How to Use HTTP Authorization Header ?
Learn how to use HTTP authorization header to access APIs securely and efficiently, and how to handle common errors and challenges with it.
If you are working with APIs, you probably know what HTTP authorization header is. It is a way of sending credentials to a server to authenticate a request. It is often used to access protected resources or perform actions that require permission. But do you know how to use it effectively and securely? In this blog post, we will show you how to use HTTP authorization header like a pro, using a tool called apidog.
By the end of this blog post, you will have a better understanding of HTTP authorization header and how to use it with confidence. Let’s get started!
What is HTTP Authorization Header?
HTTP authorization header is a part of the HTTP protocol that allows you to send credentials to a server to authenticate a request. It is usually formatted as:
Authorization: <type> <credentials>
The <type>
indicates the authorization scheme, such as Basic, Bearer, Digest, etc. The <credentials>
are the actual data that the server needs to verify your identity, such as a username and password, a token, a hash, etc.
HTTP authorization header is often used to access protected resources or perform actions that require permission. For example, you may need to send HTTP authorization header to:
- Access a user’s profile or data on a social media platform
- Upload or download files from a cloud storage service
- Make a payment or a transaction on an e-commerce site
- Manage or monitor a server or a device on a network
- And many more
HTTP authorization header is a simple and flexible way of authenticating requests, but it also comes with some challenges and risks. You need to choose the right authorization scheme for your API, generate and send the HTTP authorization header correctly, handle errors and responses from the server, secure your HTTP authorization header from attacks, and document your HTTP authorization header for other developers.
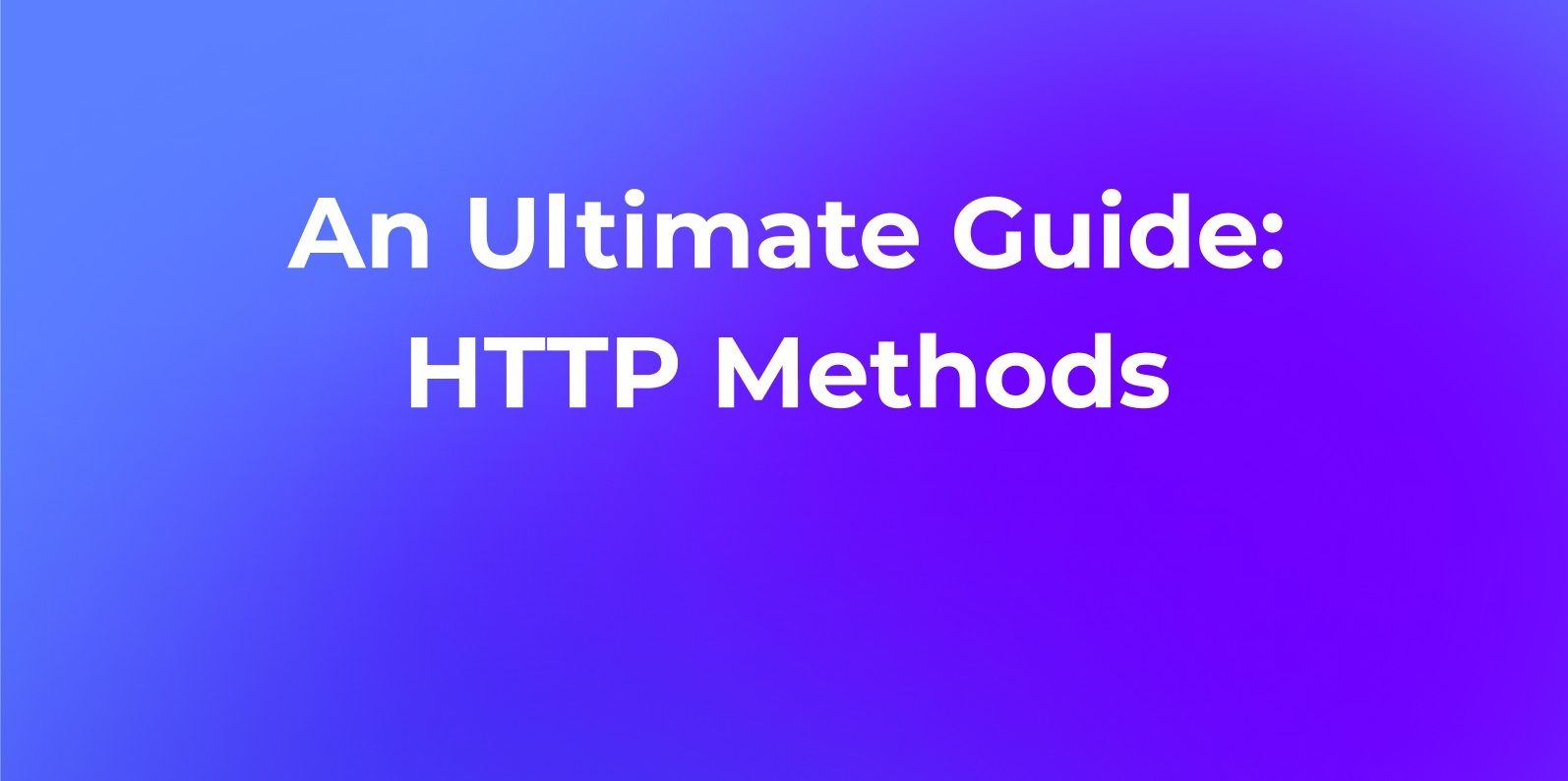
How to Choose the Right Authorization Scheme for Your API
There are many authorization schemes that you can use with HTTP authorization header, such as Basic, Bearer, Digest, OAuth, etc. Each scheme has its own advantages and disadvantages, and you need to choose the one that suits your API’s needs and security requirements. Here are some factors that you should consider when choosing an authorization scheme for your API:
- Complexity: Some authorization schemes are simpler and easier to implement than others. For example, Basic and Bearer are very straightforward and only require a single header, while OAuth and Digest are more complex and require multiple steps and headers. You should choose an authorization scheme that is easy to understand and use for both you and your API consumers.
- Security: Some authorization schemes are more secure and robust than others. For example, Basic and Bearer are vulnerable to eavesdropping and replay attacks, while OAuth and Digest are more resistant to these threats. You should choose an authorization scheme that provides adequate protection for your API’s data and functionality.
- Performance: Some authorization schemes are more efficient and faster than others. For example, Basic and Bearer are stateless and do not require any additional requests or database queries, while OAuth and Digest are stateful and may require extra overhead. You should choose an authorization scheme that minimizes the latency and bandwidth consumption of your API.
- Standardization: Some authorization schemes are more widely adopted and supported than others. For example, Basic and Bearer are very common and compatible with most HTTP clients and servers, while OAuth and Digest are more specific and may require special libraries or tools. You should choose an authorization scheme that is easy to integrate and maintain for your API.
To help you choose the right authorization scheme for your API, here is a table that summarizes the main features and differences of some popular authorization schemes:
Scheme | Complexity | Security | Performance | Standardization |
---|---|---|---|---|
Basic | Low | Low | High | High |
Bearer | Low | Medium | High | High |
Digest | Medium | Medium | Medium | Medium |
OAuth | High | High | Low | Medium |
Of course, this table is not exhaustive and there may be other authorization schemes that are not listed here. You should always do your own research and testing before choosing an authorization scheme for your API.
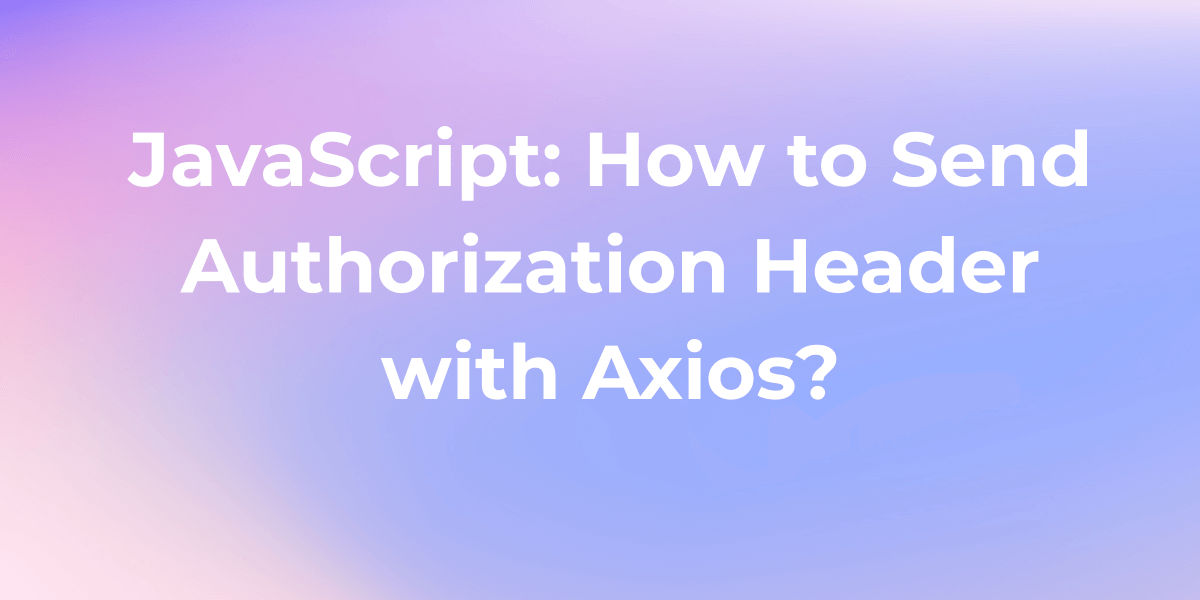
How to use HTTP authorization header with basic authentication
Basic authentication is one of the simplest and most widely used types of HTTP authorization header. It works by sending the username and password of the requester in plain text, encoded with base64, to the server. The server then decodes the credentials and checks if they match the ones stored in its database. If they do, the server grants access to the requested resource. If they don’t, the server returns an error message.
To use HTTP authorization header with basic authentication, you need to follow these steps:
- Encode your username and password with base64. You can use any online tool or library to do this. For example, if your username is “alice” and your password is “secret”, the base64-encoded string would be “YWxpY2U6c2VjcmV0”.
- Add the prefix "Basic " to the encoded string. This indicates that you are using basic authentication. For example, the final string would be “Basic YWxpY2U6c2VjcmV0”.
- Set the value of the HTTP authorization header to the final string. For example, the HTTP authorization header would look like this:
Authorization: Basic YWxpY2U6c2VjcmV0
- Send the request to the server. The server will decode the credentials and authenticate the request. For example, if you are using curl, the command would look like this:
curl -H "Authorization: Basic YWxpY2U6c2VjcmV0" https://example.com/api
- Receive the response from the server. If the credentials are valid, the server will return the requested resource. If the credentials are invalid, the server will return an error message with status code 401 (Unauthorized).
Using HTTP authorization header with basic authentication is simple and easy, but it also has some drawbacks. The main drawback is that the credentials are sent in plain text, which means they can be intercepted and compromised by anyone who can see the network traffic. Therefore, basic authentication should only be used over HTTPS, which encrypts the data and prevents eavesdropping. Another drawback is that basic authentication does not support any form of session management, which means the credentials have to be sent with every request, which can be inefficient and insecure. Therefore, basic authentication should only be used for simple and stateless APIs, where the security requirements are low and the performance impact is minimal.
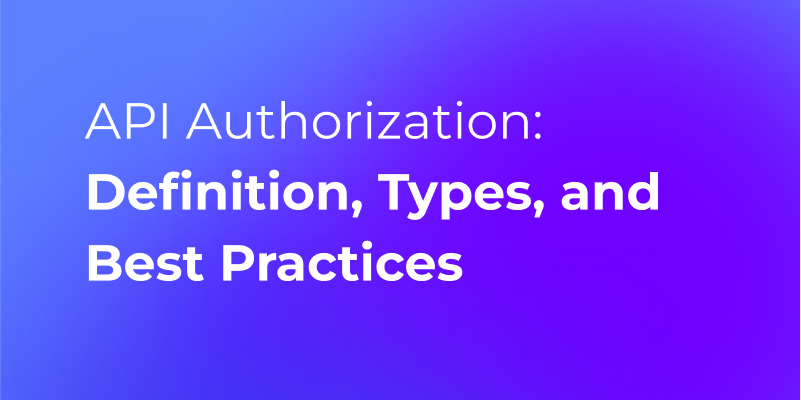
How to use HTTP authorization header with bearer token
Bearer token is another popular type of HTTP authorization header. It works by sending a token, which is a string of characters that represents the identity and permissions of the requester, to the server. The server then validates the token and checks if it grants access to the requested resource. If it does, the server returns the resource. If it doesn’t, the server returns an error message.
To use HTTP authorization header with bearer token, you need to follow these steps:
- Obtain a token from the server or a third-party service. The token can be generated and validated using various methods and standards, such as JSON Web Token (JWT), which is a self-contained and secure way of encoding and verifying claims. To obtain a token, you usually need to provide some credentials, such as username and password, or an API key, to the server or the service. The server or the service will then return a token that contains the information and permissions of the requester. For example, if you are using JWT, the token would look something like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJhbGljZSIsIm5hbWUiOiJBbGljZSBCb2IiLCJyb2xlIjoiYWRtaW4iLCJleHAiOjE2MjEwMjQwMDB9.6y0jZt7xg8GxhXUq3TJrcQ4aR7fZ0v0t5DLGJ4Z5C8k
The token consists of three parts, separated by dots: the header, the payload, and the signature. The header contains the algorithm and the type of the token. The payload contains the claims, which are the information and permissions of the requester. The signature is the result of applying the algorithm to the header and the payload, using a secret key. The signature ensures the integrity and authenticity of the token.
2. Add the prefix "Bearer " to the token. This indicates that you are using bearer token authentication.
3. Set the value of the HTTP authorization header to the final string.
4. Send the request to the server. The server will decode and verify the token and authenticate the request.
5. Receive the response from the server. If the token is valid, the server will return the requested resource. If the token is invalid, the server will return an error message with status code 401 (Unauthorized) or 403 (Forbidden).
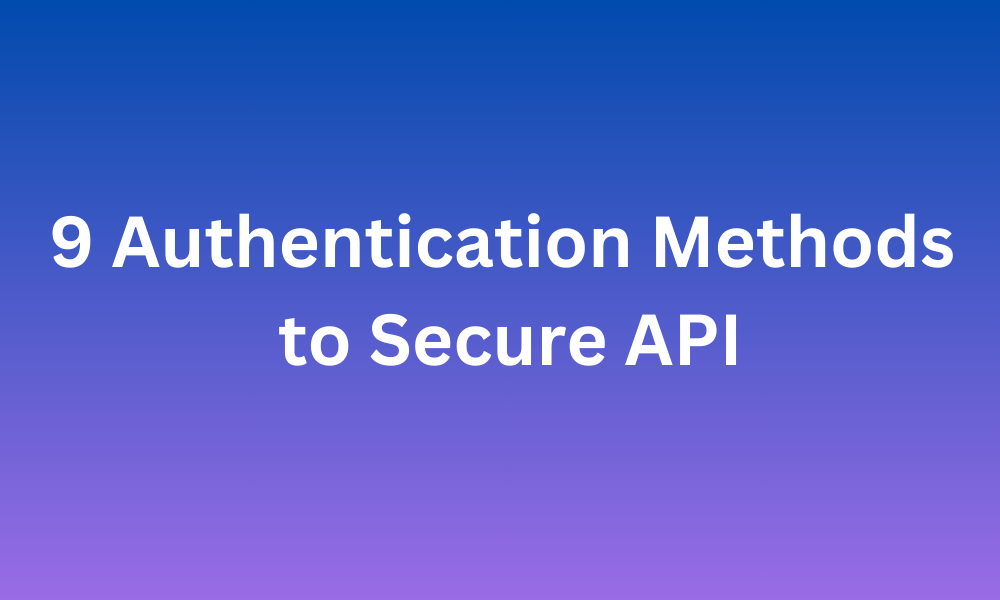
How to use HTTP authorization header with digest authentication
Digest authentication is a more advanced and secure type of HTTP authorization header than basic authentication. It works by sending a hash, which is a result of applying a mathematical function to a string of characters, of the credentials and some other information, such as a nonce and a timestamp, to the server. The server then calculates the same hash using the same information and compares it with the one sent by the requester. If they match, the server grants access to the requested resource. If they don't, the server returns an error message.
To use HTTP authorization header with digest authentication, you need to follow these steps:
- Receive a challenge from the server. The challenge is a message that contains some information that the server uses to verify the credentials, such as a nonce, a realm, and a qop. The challenge is sent by the server when the requester tries to access a protected resource without authentication, or with invalid credentials. The challenge is sent with status code 401 (Unauthorized) and a header called WWW-Authenticate.
- Calculate the hash of the credentials and the challenge. The hash is calculated using a mathematical function called MD5, which produces a 32-digit hexadecimal number from any input. The hash is composed of three parts: the HA1, the HA2, and the response. The HA1 is the hash of the username, the realm, and the password. The HA2 is the hash of the HTTP method and the URI of the request. The response is the hash of the HA1, the nonce, the nonce count, the client nonce, the qop, and the HA2. The nonce count is a number that indicates how many times the nonce has been used. The client nonce is a random string that is generated by the requester.
How to Send HTTP Authorization Header with Apidog
Once you have chosen an authorization scheme for your API, you need to generate and send the HTTP authorization header with your requests. This can be done easily with Apidog, a web-based tool that helps you test, debug, and document your APIs. Apidog allows you to:
- Create and save multiple API requests with different parameters, headers, and body
- Send and receive API requests and responses in real-time
- View and analyze the API response status, headers, and body
- Validate and format the API response body with JSON, XML, HTML, etc.
- Generate and share API documentation with other developers.
To use Apidog, you need to follow these steps:
- Click on the "New Request" button.
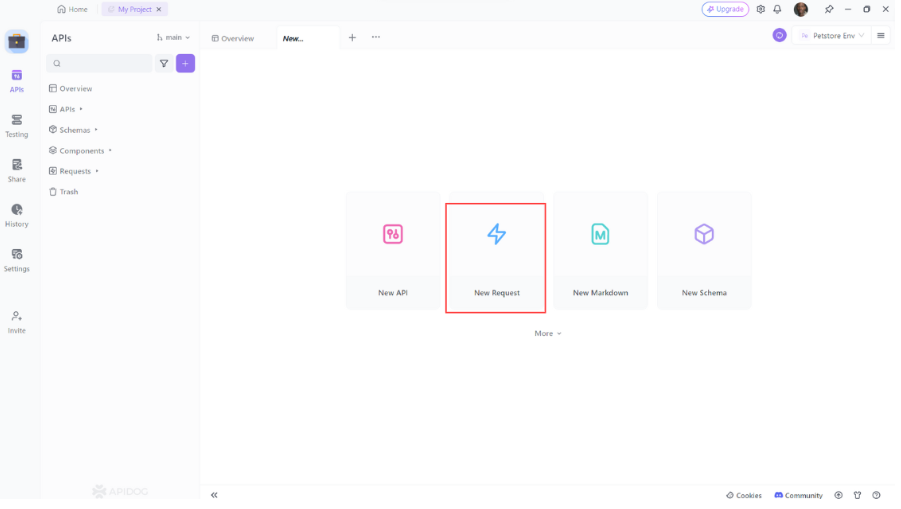
2. Select the HTTP method you want to use and enter the URL of the API you want to access.
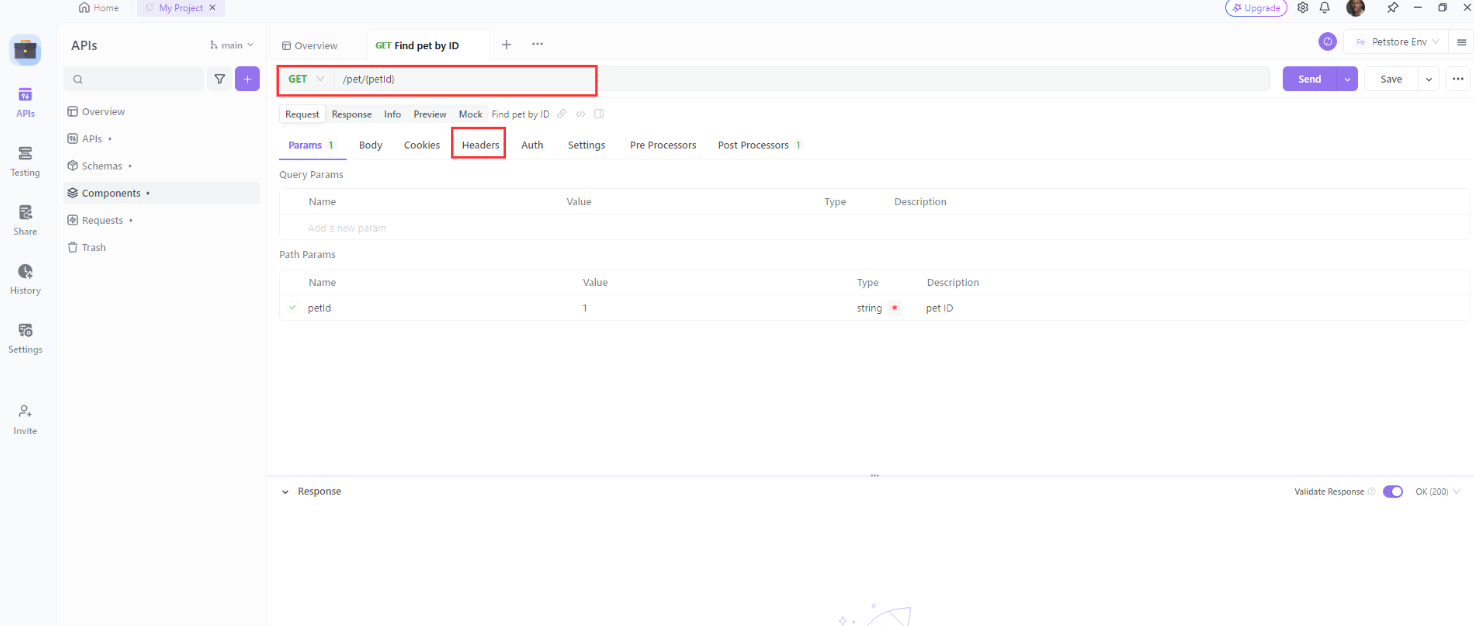
3. Click on the "Headers" tab and add the HTTP authorization header and Auth to modify your authorization type.
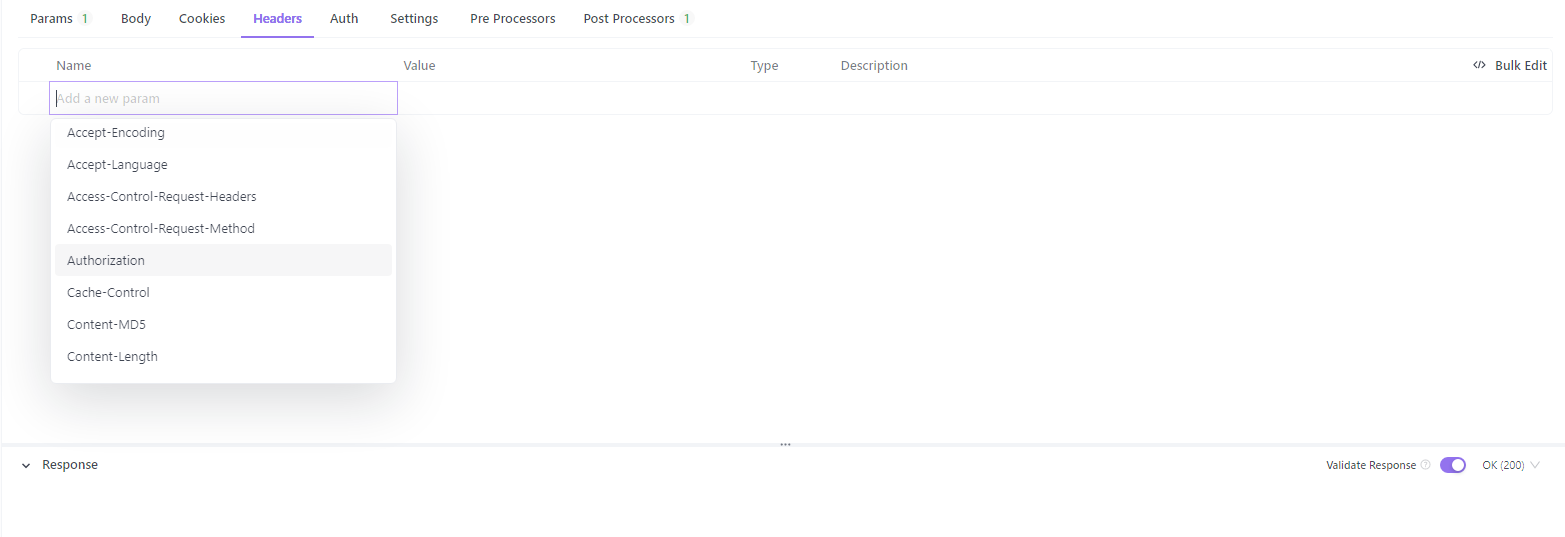
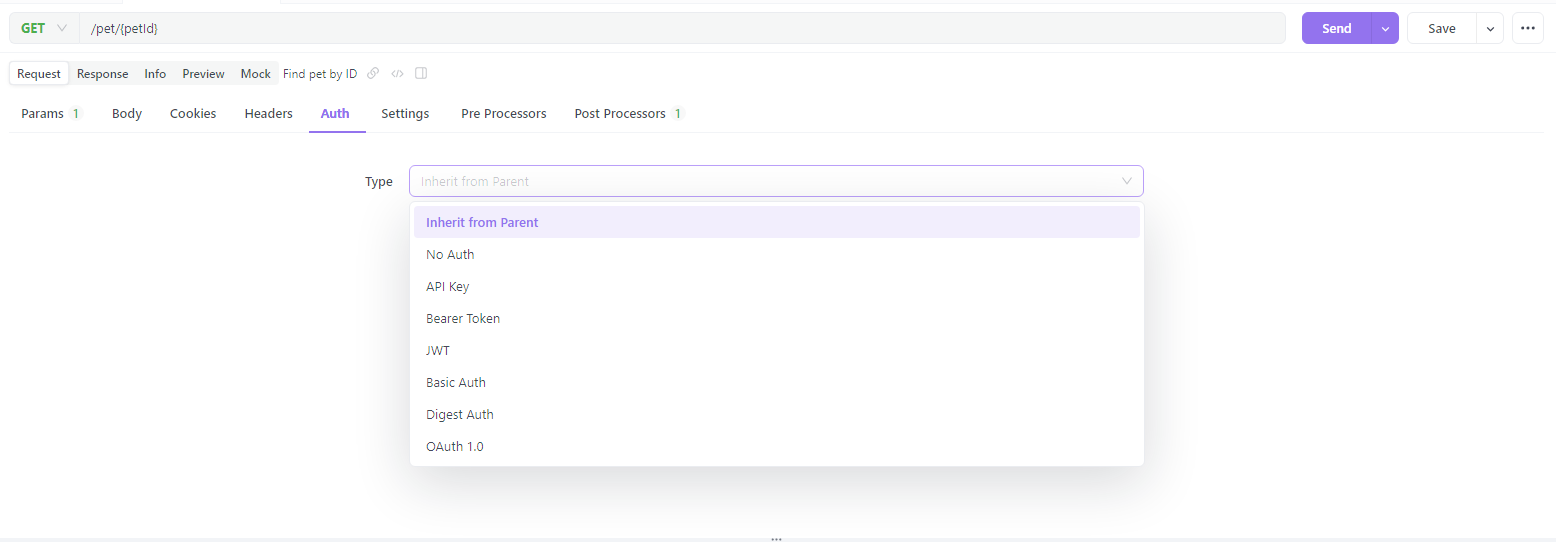
4. Click on the "Send" button and check the response status code, headers, and body. If the token is valid, the status code should be 200 (OK) and the body should contain the requested resource. If the token is invalid, the status code should be 401 (Unauthorized) or 403 (Forbidden) and the body should contain an error message.
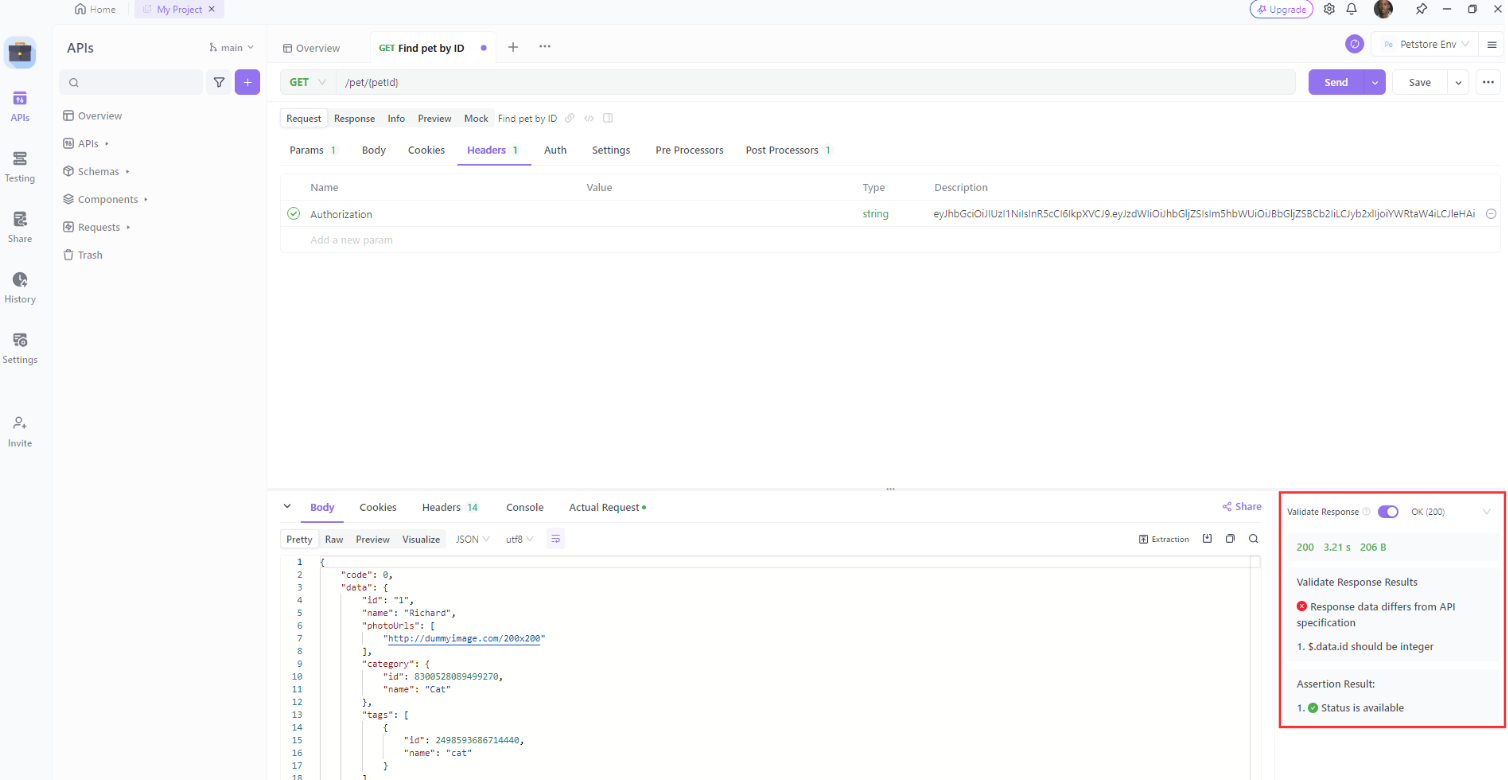
Check the response status code, headers, and body. If the token is valid, the status code should be 200 (OK) and the body should contain the requested resource. If the token is invalid, the status code should be 401 (Unauthorized) or 403 (Forbidden) and the body should contain an error message.
As you can see, apidog makes it very easy and convenient to generate and send the HTTP authorization header with your API requests. You can also use apidog to test and debug other aspects of your API, such as the parameters, headers, and body of your requests and responses.
How to Handle Common Errors and Challenges with HTTP Authorization Header
When you use HTTP authorization header to authenticate your API requests, you may encounter some errors and challenges that you need to handle properly. Some of the common errors and challenges are:
- Invalid or missing credentials: One of the most common errors with HTTP authorization header is when the credentials are invalid or missing. This can happen when the user enters the wrong username or password, the token expires or is revoked, the hash is incorrect or tampered with, or the header is malformed or omitted. To handle this error, you should always check the response status code and the WWW-Authenticate header from the server. If the status code is 401 (Unauthorized), it means that the server requires authentication and provides a challenge that indicates the supported schemes and parameters. You should then prompt the user for the correct credentials, or obtain a new token, and retry the request with the appropriate authorization header. If the status code is 403 (Forbidden), it means that the server rejects the credentials or the token, and does not allow access to the resource. You should then inform the user of the reason and the possible actions, such as contacting the administrator or requesting a new permission.
- Replay attacks: Another common challenge with HTTP authorization header is when the credentials or the token are reused by an attacker who intercepts the request or the response. This can compromise the security and integrity of the API and the data. To prevent this challenge, you should always use HTTPS, which encrypts the data and prevents eavesdropping. You should also use schemes that include nonce, timestamp, and signature, such as digest authentication and JWT, which make the credentials or the token unique and verifiable. You should also use schemes that have expiration time and revocation mechanism, such as OAuth 2.0, which limit the validity and usability of the credentials or the token.
- Performance and scalability: Another common challenge with HTTP authorization header is when the authentication process affects the performance and scalability of the API and the server. This can happen when the authentication scheme is complex and computationally intensive, such as hashing, encrypting, and signing, or when the authentication requires multiple requests and responses, such as obtaining and refreshing tokens. To overcome this challenge, you should always choose the right authentication scheme for your API, based on the security requirements, the functionality, and the user experience. You should also optimize the authentication process, such as caching the credentials or the token, using efficient algorithms and libraries, and reducing the network overhead.
- Documentation and communication: Another common challenge with HTTP authorization header is when the authentication scheme is not well documented and communicated to the users and developers of the API. This can lead to confusion, errors, and frustration. To avoid this challenge, you should always document and communicate the authentication scheme for your API, such as the type, the format, the parameters, the errors, and the examples of the authorization header.
Conclusion
The HTTP Authorization Header is a powerful and flexible way to secure your APIs and provide authentication and authorization for your clients. By following the best practices and tips discussed in this post, you can ensure that your APIs are robust, reliable, and compliant with the standards and specifications.
You can also use various tools and frameworks, such as Apidog, to design, debug, develop, mock, and test your APIs with the HTTP Authorization Header. Apidog helps you connect the entire API lifecycle and implement the best practices for API Design-first development.