Socket. IO vs. WebSocket: Keys Differences
Two popular technologies that facilitate real-time communication are Socket.IO and WebSocket. In this article, we'll dive deep into Socket.IO and WebSocket, exploring their key differences, use cases, and advantages.
In today's interconnected world, real-time communication has become crucial for web applications. Whether it's online gaming, live chat, or collaborative platforms, the demand for seamless and efficient communication between the client and server has grown significantly.
Two popular technologies that facilitate real-time communication are Socket.IO and WebSocket. Although they both serve the same purpose, they have distinct characteristics and are suited for different scenarios. In this article, we'll dive deep into Socket.IO and WebSocket, exploring their key differences, use cases, and advantages.
What is WebSocket?
WebSocket is a protocol that provides a full-duplex communication channel over a single TCP connection. It allows the server to send data to the client and vice versa without the need for the client to continuously send requests.
WebSocket uses a persistent connection, which means that once the connection is established, it remains open until either the client or the server decides to close it. This makes WebSocket suitable for applications that require real-time updates, such as chat applications, real-time collaboration tools, and financial trading platforms.
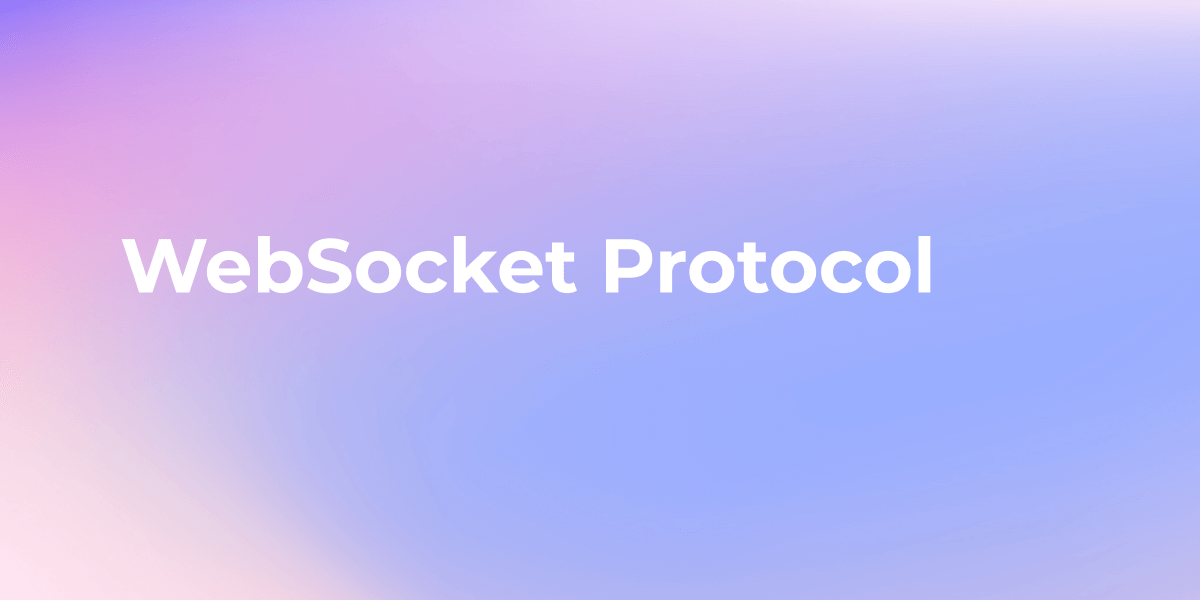
Advantages of WebSocket and Use Cases
Advantages of WebSocket
WebSocket has several advantages over traditional HTTP polling or long polling techniques. Firstly, it reduces the overhead of establishing and maintaining a connection compared to HTTP. Once the initial handshake is complete, WebSocket allows for continuous communication without the need to send additional HTTP requests. This results in lower latency and improved performance.
Another advantage of WebSocket is its ability to handle real-time data streaming. Unlike traditional HTTP requests, which are request-response based, WebSocket allows for bidirectional communication, making it ideal for applications that require real-time updates, such as chat applications, stock market tickers, and multiplayer games.
WebSocket is also highly scalable and can handle a large number of concurrent connections. This is achieved through its efficient use of resources and the ability to multiplex multiple WebSocket connections over a single TCP connection. This makes it suitable for applications that require high scalability, such as real-time collaboration tools or live-streaming platforms.
WebSocket is supported by most modern web browsers and can be used with various programming languages and frameworks. It provides a simple API for developers to work with, making it easy to implement and integrate into existing applications.
Disadvantages of WebSocket:
- Limited features out of the box (e.g., no automatic reconnection, no packet buffering)
- Requires more manual implementation for advanced features
Suitable Use Cases for WebSocket
In terms of use cases, WebSocket is well-suited for applications that require real-time communication and data streaming. It is particularly useful in scenarios where instant updates are crucial, such as real-time collaboration tools, multiplayer games, and financial applications. WebSocket can also be used for push notifications, live chat applications, and real-time monitoring systems.
In summary, WebSocket offers several advantages over traditional HTTP-based communication methods. Its low latency, bidirectional communication, scalability, and support for real-time data streaming make it a powerful tool for building modern web applications.
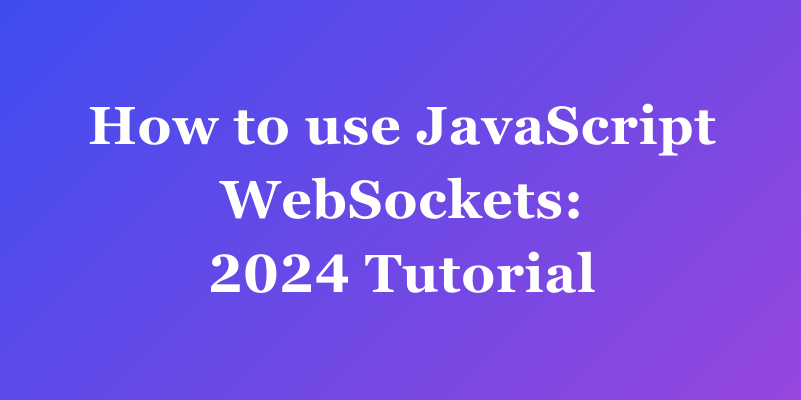
However, it is important to consider the specific requirements of your application before choosing between WebSocket and other communication protocols like Socket.IO.
Writing Real-Time Code
How do they play out when writing real-time applications?
Simple WebSocket Server
In the Node.js program below, we'll create a WebSocket server exposed on port 3001. Each time a client connects, a unique ID will be assigned to their session. When a client sends a message, the server will reply in the format: "[]: ", confirming the successful message delivery.
const WebSocket = require("ws");
const UUID = require("uuid");
const wss = new WebSocket.Server({ port: 3000 });
wss.on("connection", ws => {
ws.id = UUID();
ws.on("message", message => {
ws.send(`[${ws.id}]: ${message}`);
});
});
But what if we want to broadcast the message to all connected clients? By default, WebSocket doesn't support message broadcasting. However, with plain WebSocket, implementing message broadcasting is still straightforward:
const WebSocket = require("ws");
const UUID = require("uuid");
const wss = new WebSocket.Server({ port: 3000 });
function broadcast(clientId, message) {
wss.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(`[${clientId}]: ${message}`);
}
});
}
wss.on("connection", ws => {
ws.id = UUID();
ws.on("message", message => broadcast(ws.id, message));
});
As you can see, the WebSocket.The server will track each connected client, allowing us to loop through them and send the desired message to each one! We've just implemented the simplest chat server ever! You can test the above code using any WebSocket client, whether on the client-side or through a Chrome extension.
Using Socket.IO to Achieve the Same
const io = require("socket.io");
const server = io.listen(3002);
server.on("connection", socket => {
socket.on("message", message => {
socket.emit(`[${socket.id}]: ${message}`);
socket.broadcast.emit(`[${socket.id}]: ${message}`);
});
});
As you can see, using Socket.IO's native broadcast
method, we won't send the message back to the sender; instead, we send it to all other clients. Therefore, we need to manually send the message to the client in this case.
However, there's one issue: you can't test it with a standard WebSocket client (as we saw in the previous example). That's because, as mentioned earlier, Socket.IO doesn't use plain WebSocket but combines multiple technologies to support as many clients as possible (and avoid certain issues, as mentioned earlier). So, how do we test it?
Using Socket.IO Client
<html>
<head>
</head>
<body>
<script type="text/javascript" src="socket.io-client.js"></script>
<script type="text/javascript">
ioClient = io.connect("http://localhost:3000");
ioClient.on("connect", socket => {
ioClient.send("Hello everybody!");
ioClient.on("message", msg => console.log(msg));
});
</script>
</body>
</html>
You need to use a Socket.IO client. In the example above, we use the client provided by a CDN, which allows us to do some quick and dirty testing in the web browser.
As you can see, these two examples might not appear very different, but in terms of compatibility, remember that Socket.IO will always be used with its own client library. Therefore, you won't be able to use it for purposes outside of web development. WebSocket, on the other hand, can be utilized for solving various problems, such as p2p communication and real-time server-to-server data transfer, among others.
What is Socket.IO?
Socket.IO is a powerful library that provides real-time bidirectional communication between the server and the client. It is built on top of the WebSocket protocol and offers several advantages over using WebSocket alone. In this section, we will explore the advantages of Socket.IO and discuss its suitable use cases.
Advantages of Socket.IO and Use Cases
The Advantages of Socket.IO
Fallback options: One of the major advantages of Socket.IO is its ability to fall back to other transport mechanisms if WebSocket is not supported by the client or the network. Socket.IO supports several fallback options, including long polling, JSONP polling, and iframe-based transport. This ensures that communication can still happen even in environments where WebSocket is not available.
Real-time event-based communication: Socket.IO provides a simple and intuitive API for emitting and listening to events. This allows developers to build real-time applications easily, where server-side events can be instantly propagated to connected clients. Socket.IO also supports broadcasting, allowing events to be sent to all connected clients or specific groups of clients.
Automatic reconnection: Socket.IO automatically handles reconnection in case of network disruptions. It attempts to reconnect to the server and restore the connection seamlessly. This is especially useful in mobile applications where network connections can be unstable.
Room-based communication: Socket.IO allows clients to join and leave rooms, enabling targeted communication between specific groups of clients. This is useful in scenarios where you want to send messages only to a subset of connected clients, such as chat applications or multiplayer games.
Disadvantages of Socket.IO:
- Additional overhead and complexity compared to plain WebSocket
- Reliance on a third-party library
- Not a standardized protocol
Clarifying Misconceptions:
There are several misconceptions that need clarification regarding WebSocket and Socket.IO:
- Ease of Use: Contrary to popular belief, Socket.IO isn't necessarily easier to use than WebSocket. The following examples provide more insight into this.
- Browser Support: It's a common misconception that WebSocket lacks broad browser support. The detailed information below dispels this notion.
- Connection Handling: A misunderstanding exists about Socket.IO's connection downgrade for older browsers. In actuality, Socket.IO assumes an older browser and initiates an AJAX connection with the server, upgrading to WebSocket once supported, after initial data exchange.
Suitable Use Cases for Socket.IO
- Real-time applications: Socket.IO is well-suited for building real-time applications, such as chat applications, collaborative editing tools, and live dashboards. Its event-based communication model and automatic reconnection make it ideal for scenarios where instant updates are required.
- Multiplayer games: Socket.IO's room-based communication and real-time event propagation make it a great choice for building multiplayer games. It allows for efficient communication between players and enables real-time updates of game state.
- Collaborative applications: Socket.IO's ability to handle multiple clients and support targeted communication makes it a good fit for collaborative applications. It allows multiple users to work together in real-time, making it suitable for applications like document editing tools, project management systems, and collaborative drawing tools.
- Monitoring and analytics: Socket.IO can be used to build monitoring and analytics systems that require real-time data updates. It enables real-time visualization of data and allows for instant notifications or alerts based on specific events.
What is the Difference Between WebSocket vs Socket.IO?
Here's a simplified comparison of WebSocket vs Socket. IO :
Aspect | WebSocket | Socket.IO |
---|---|---|
Protocol/Library | Protocol established over TCP connections | Library designed to enhance WebSocket functionality |
Communication | Facilitates full-duplex communication on TCP | Enables event-based communication between browsers |
Support | Lacks inherent support for proxies and load balancers | Supports connection with proxies and load balancers |
Broadcasting | Doesn't inherently support broadcasting | Supports broadcasting |
Fallback Option | Doesn't provide a built-in fallback option | Incorporates fallback options when WebSocket is unavailable |
WebSocket vs Socket.IO, Which One to Use?
When it comes to choosing between WebSocket and Socket.IO for real-time communication in your web application, here is my recommendation on whether to use WebSocket or Socket.IO:
For most projects, I would recommend using Socket.IO over plain WebSocket.
The key reasons to favor Socket.IO are:
- Ease of Development: Socket.IO provides a higher-level abstraction and more features out-of-the-box, making it easier and faster to develop real-time functionality compared to using raw WebSocket.
- Cross-Browser Support: Socket.IO automatically handles fallback options like long-polling for environments where WebSocket is not supported, ensuring better cross-browser compatibility.
- Additional Features: Socket.IO includes useful features like automatic reconnection, multiplexing, broadcasting, and namespacing, which would need to be implemented manually with WebSocket.
- Consistent API: Socket.IO has consistent APIs for both client-side (JavaScript) and server-side (Node.js), simplifying development across different environments.
- Community and Documentation: Socket.IO has a larger community and more extensive documentation, making it easier to get started and find support.
However, there are some cases where using plain WebSocket may be preferable:
- Lightweight Requirements: If you need an extremely lightweight solution and do not require Socket.IO's additional features, WebSocket can result in a smaller client-side payload.
- Strict Performance Needs: In some scenarios with stringent performance requirements, the overhead introduced by Socket.IO's abstraction layer may be undesirable, although the difference is likely negligible in most cases.
- Extensive Customization: If you need a highly customized solution that doesn't align with Socket.IO's design choices, implementing with WebSocket directly can provide more control and flexibility.
- Non-JavaScript Environments: If your server-side environment is not JavaScript/Node.js, using WebSocket directly may be simpler than integrating Socket.IO with other language-specific libraries.
Using Apidog to Debug WebSocket Service
If you have developed a WebSocket service and want to debug it, we suggest using some excellent API debugging tools, such as Apidog, which has the ability to debug WebSocket services.
Apidog Google Chrome Extension
Apidog has both a web version and a client, if you are using the web version and want to debug a local service, you need to install Apidog's Google Chrome extension. Apidog online link: Apidog Google Chrome extension
Step 1. Creating a WebSocket Request
In the most recent update of Apidog, accessing a WebSocket interface is made easy by simply clicking the "+" button on the left side of your project and selecting "New WebSocket API(Beta)".
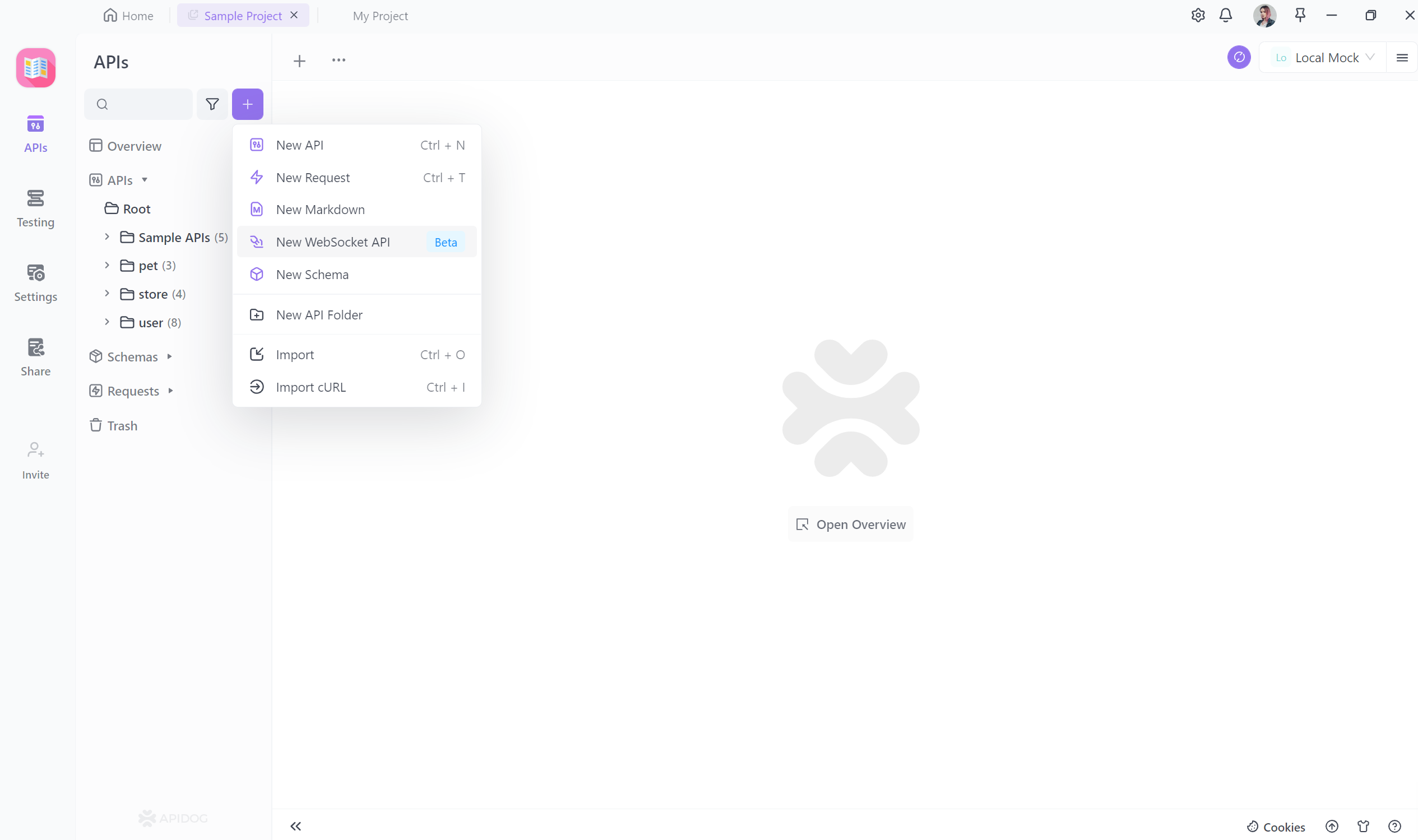
From there, you can enter the URL of your WebSocket interface to establish a connection and seamlessly send and receive messages. Below, we will provide more detailed instructions.
Step 2. Setting Message and Params
In the Message section, you can fill in the information you want to send to the server side, and the server will receive the information you transmit.
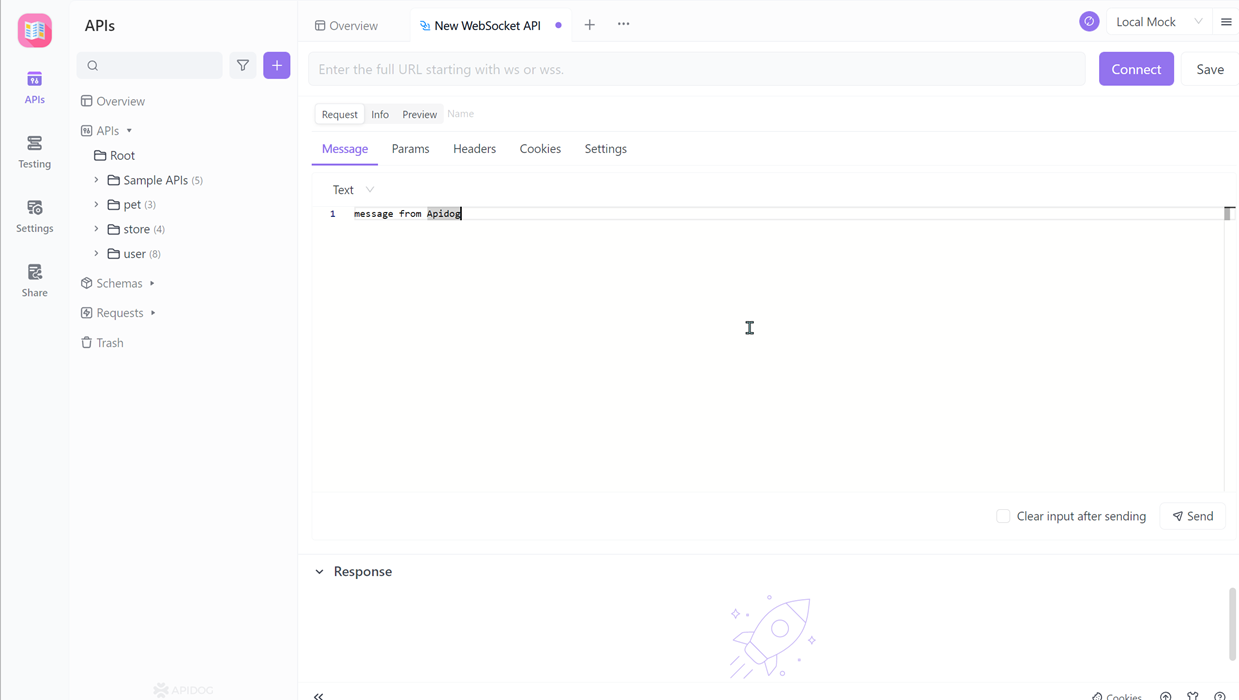
During the transmission process, parameters can also be carried out. Query parameters can be carried on the address, and the data types that can be carried include string, integer, number, array.
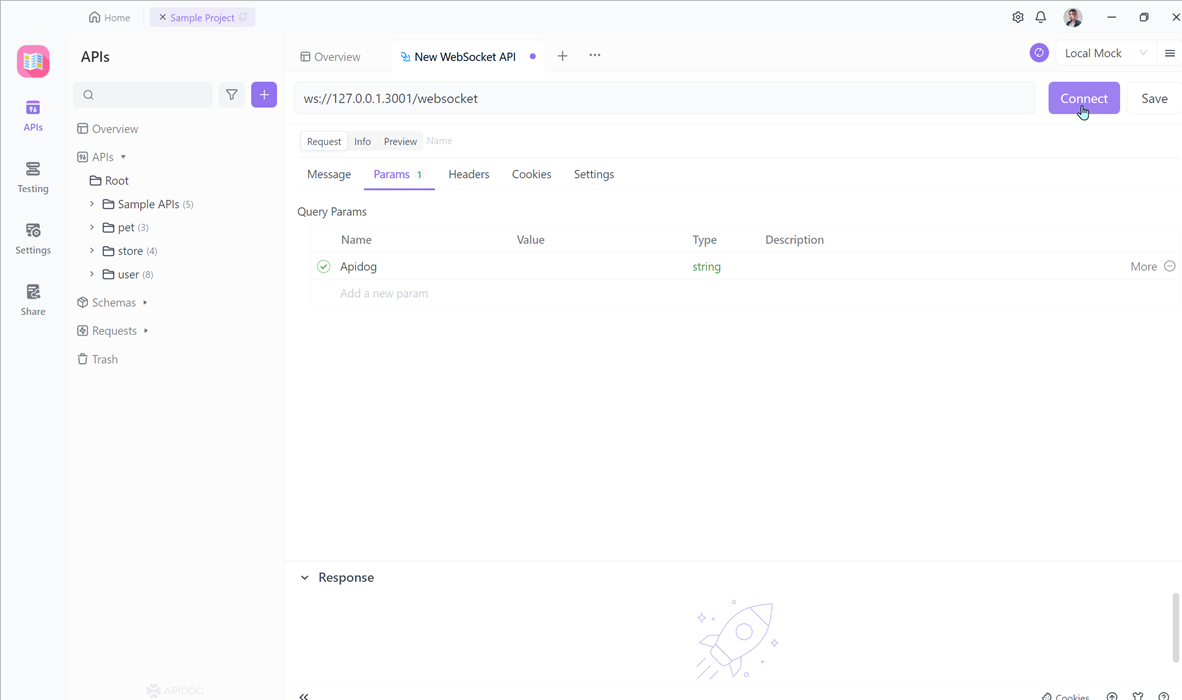
Step 3. Saving Request
After completing the required information, you can click the save button to save it for future use, making it easy to access again next time.
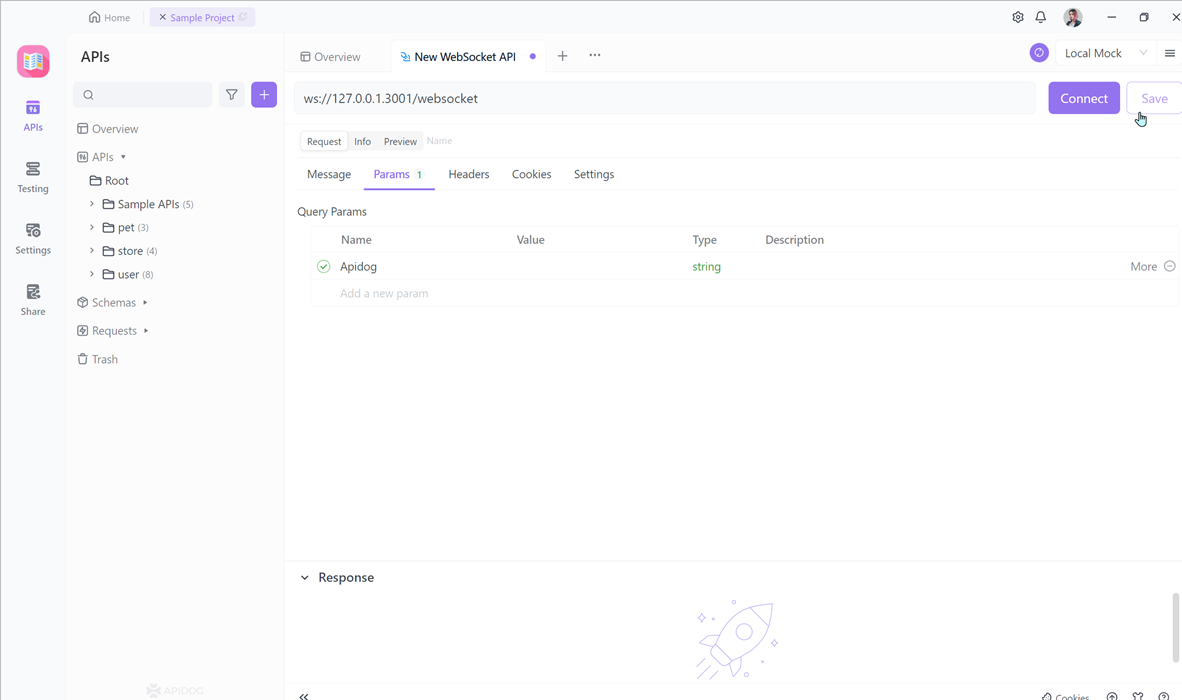
Step 4. Connect and Send WebSocket Requests
To communicate with the WebSocket server, we need to establish a WebSocket connection. We can simply click the "connect" button to do so.
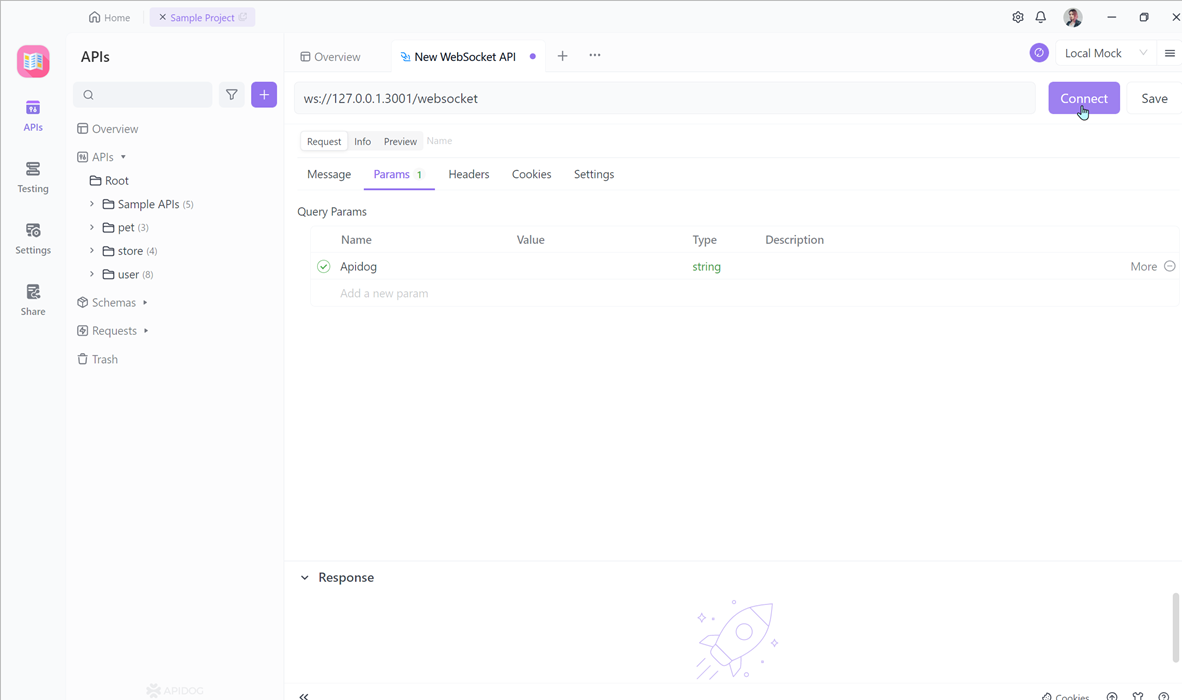
After a successful connection, Apidog will display a success promptly.On the server side, a response will also be made due to a successful connection.
Step 5. Sending WebSocket Request
Then we can communicate with the server via WebSocket. We can use Apidog to click the send button to send messages and parameters to the server.
Step 6. Communicating with the WebSocket service
After sending, the server can also send information to the client. For example, in my case, I set the server to send the current timestamp to the client every second. This is the WebSocket function of Apidog, which is very convenient.
Conclusion
In conclusion, both Socket.IO and WebSocket are essential tools for enabling real-time communication between clients and servers. WebSocket offers superior performance and is ideal for applications demanding low-latency communication. On the other hand, Socket.IO provides added flexibility with fallback mechanisms, making it suitable for projects that require cross-browser compatibility and automatic reconnection.
Ultimately, the choice between Socket.IO and WebSocket depends on the specific needs of your web application. Consider factors like application requirements, user base, and performance priorities to make an informed decision. By understanding their key differences, you can harness the full potential of real-time communication and deliver a seamless user experience.