How to Make DELETE Requests with Axios
Axios is a popular HTTP request library that can send asynchronous requests in both browser and Node.js environments. This article will focus on how to use Axios for DELETE requests and explore different ways of passing parameters.
Axios is a popular HTTP request library that can send asynchronous requests in both browser and Node.js environments. Using JavaScript libraries like Axios for handling HTTP requests has become very common. This article will focus on how to use Axios for DELETE requests and explore different ways of passing parameters.
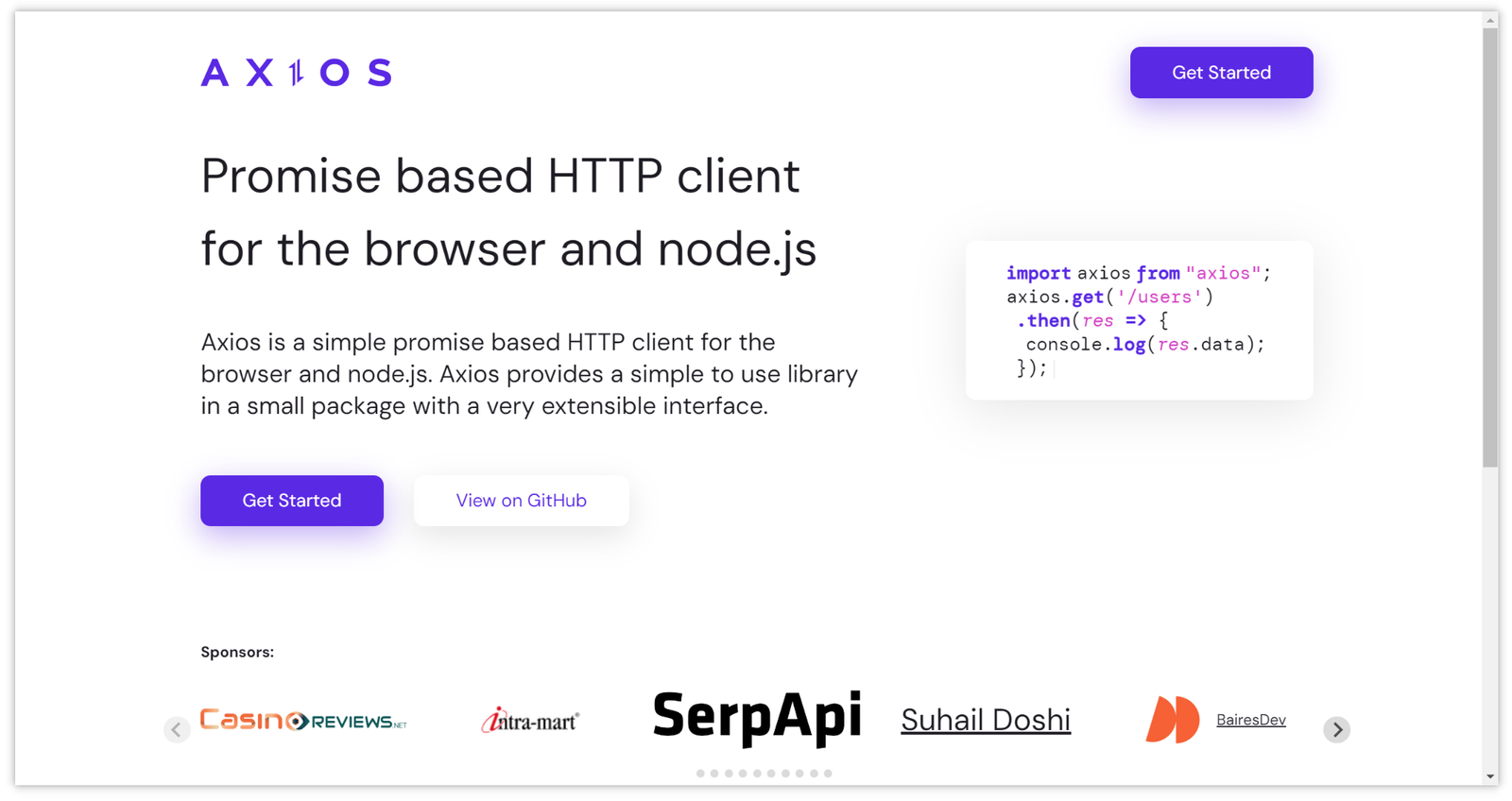
Axios DELETE Requests
DELETE requests are used to send a request to the server to delete a resource. It is an essential method in RESTful APIs used to delete a specified resource.In Axios, sending a POST request requires specifying the target URL and optionally passing some parameters like request headers and body.
Axios DELETE Requests Body and Headers
Sending a request body with an Axios DELETE request is a little bit difference from POST and PUT requests. Although technically possible, DELETE requests should not contain a request body according to RESTful API design principles.
Since DELETE requests are meant to be idempotent operations for removing a specified resource, the best practice is to use query parameters or headers to pass any additional data needed for the deletion, rather than including it in the request body.
Instead of passing the request body as the second argument, you need to include it in the data
property of the configuration object, which is the second argument. Here's an example:
javascriptCopy codeconst response = await axios.delete('https://httpbin.org/delete', {
data: { answer: 42 }
});
console.log(response.data.data); // { answer: 42 }
In this case, we're sending a DELETE request to https://httpbin.org/delete
with a request body containing { answer: 42 }
. The request body is included in the data
property of the configuration object, which is the second argument passed to axios.delete()
.
The response from the API is then available in response.data.data
because the https://httpbin.org/delete
API wraps the request body in a data
property. For other APIs, the request body might be accessible directly from response.data
.
Remember, you can't pass the request body directly as the second argument to axios.delete()
like you can with axios.post()
or axios.put()
. Always include the request body in the data
property of the configuration object.
How to Make Axios DELETE Requests Axios React
Let's practice with a simple example where we'll use Axios to send a DELETE request to delete a user.
1.Install json-server
First, you need to install json-server using npm or yarn in your project directory.
npm install -g json-server
Next, create a JSON file in your project directory to simulate your data. Assuming you want to simulate user data, create a file named "users.json" and define user data in it.
Example content of the "users.json" file:
{
"users": [
{ "id": 1, "name": "John Doe", "email": "john@example.com" },
{ "id": 2, "name": "Jane Smith", "email": "jane@example.com" }
]
}
Finally, run the following command in the terminal to start json-server and specify the mock data file:
json-server --watch users.json --port 3000
This will start a mock server, listen on port 3000, and use the data from the "users.json" file as simulated resources.
2.Send a DELETE Request
The routes provided by json-server for DELETE requests can be:
DELETE http://localhost:3000/users/:userId
First, create a new JavaScript file (e.g., "deleteUser.js") in your IDE editor and paste the following code. Then, run it in the console using the node deleteUser.js
command.
const axios = require('axios');
const userId = 1; // ID of the user to delete
axios.delete('http://localhost:3000/users/' + userId)
.then(response => {
console.log('User deleted successfully:', response.data);
})
.catch(error => {
console.error('Error deleting user:', error);
});
Note: If you encounter errors, make sure you have axios installed by running npm install axios
.
In the code above, we used the first method, directly passing parameters (user ID) in the URL to delete the corresponding user.
How to Use Apidog to Debug DELETE Request
Apidog supports debugging API for various protocols such as HTTP(s), WebSocket, Socket and gRPC. When backend developers complete service interfaces, you can use Apidog to verify the correctness of the API in the testing phase, greatly improving project deployment efficiency.
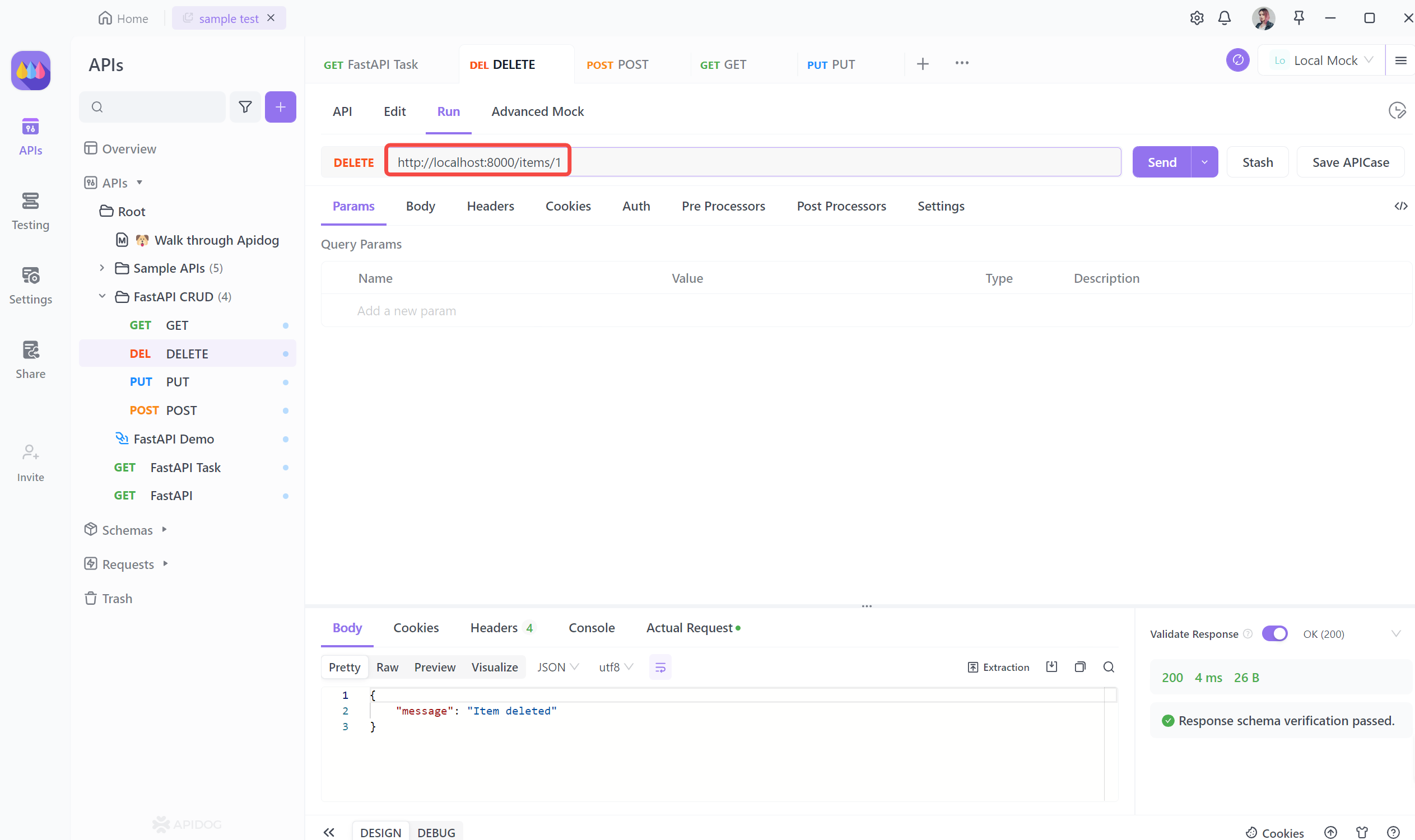
In the example in this article, you can use Apidog to test the API. Create a new project and select "Debug Mode" within the project. Enter the request address, and you can quickly send requests and get response results. The practice example mentioned above is shown in the image.