How to Send Axios PUT Request React
Axios can handle various HTTP methods, including GET, POST, and PUT. In this text, we will focus on the PUT method and introduce how to make PUT requests with Axios.
AXIOS is a JavaScript HTTP client library. It provides a simple and intuitive interface by wrapping native HTTP communication methods like XMLHttpRequest and the fetch API.
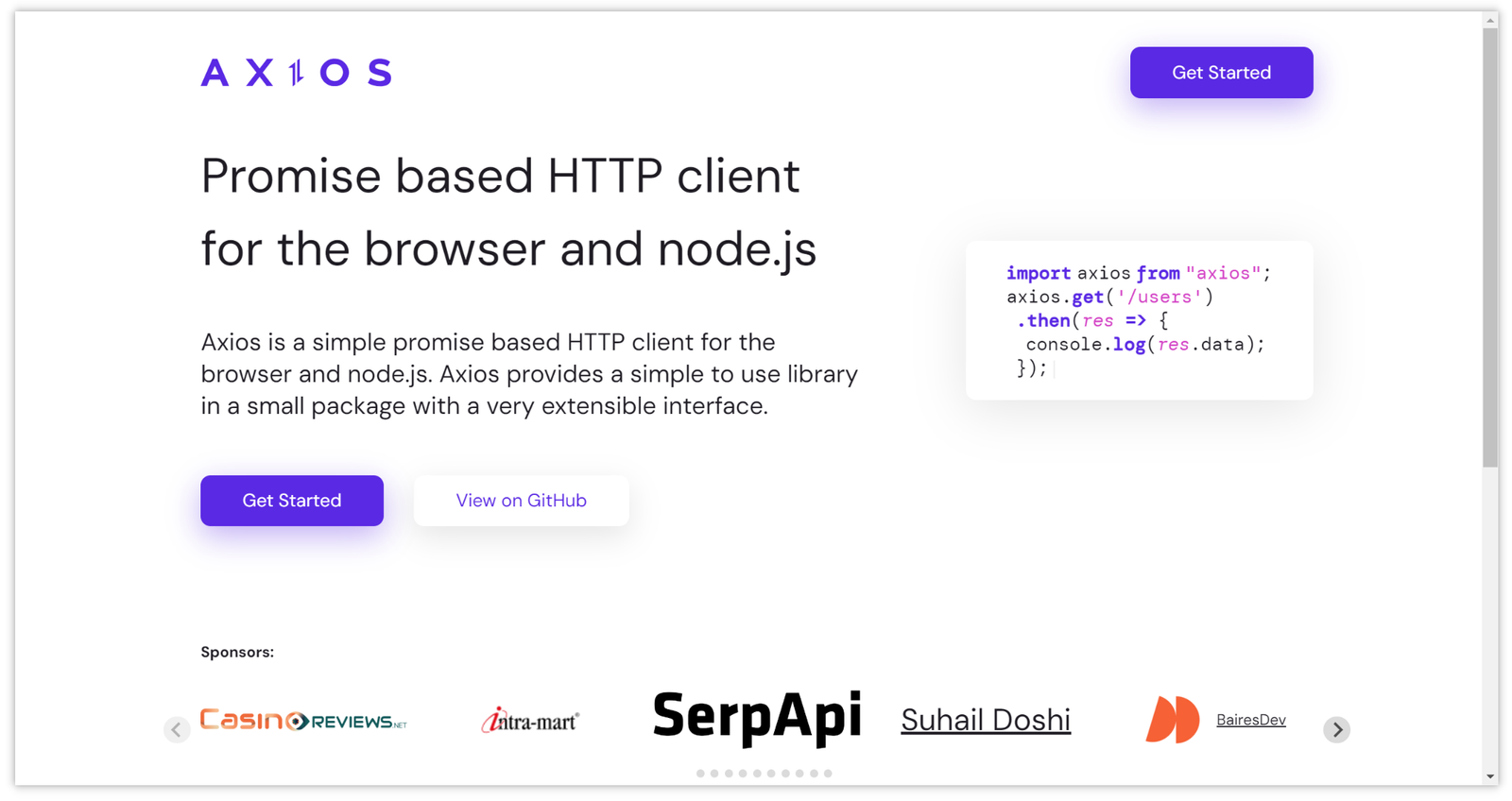
Axios can handle various HTTP methods, including GET, POST, and PUT. In this text, we will focus on the PUT method and introduce how to make PUT requests with Axios.
Axios PUT Requests React
Before using Axios, make sure it is properly installed on your computer. Similarly, Axios also offers a convenient way to send GET requests, which are used to retrieve data from the server. GET requests are typically employed for resource retrieval purposes. If it's not installed yet, you can install Axios using the following commands:
Installing Axios
Using npm:
npm install axios
Using yarn:
yarn add axios
Importing Axios and Implementing PUT Requests
Next, follow these steps to import Axios in your code and send PUT requests:
Step 1: Inside your JavaScript file, import Axios with the following code:
import axios from 'axios';
Step 2: You can use the following code to send a PUT request with Axios:
axios.put(url, data, config)
.then(response => {
// Handle the response
})
.catch(error => {
// Handle errors
});
In the above code, (url, data, config)
can be explained as follows:
url
: The URL of the server where the PUT request is sent.data
: The data you want to send with the PUT request. It's usually a JavaScript object but will be converted to JSON format when sent to the server.config
: Optional parameters configuration (Axios PUT request with headers, timeout, etc.).
Passing Parameters in PUT Requests with Axios
Now, let's explore how to pass parameters PUT requests with Axios.
Passing Parameters in the URL
You can directly add parameters to the URL and pass them to the server. This is the most common way to pass parameters:
const userId = 123;
const newData = {
name: 'John Doe',
age: 30
};
axios.put(`/api/users/${userId}`, newData)
.then(response => {
// Handle the response
})
.catch(error => {
// Handle errors
});
In the above sample code, we append userId
to the end of the URL and send newData
as the request body.
Using the params Option
Axios also provides the params option for passing parameters:
const userId = 123;
const newName = 'John Doe';
axios.put('/api/users', null, {
params: {
id: userId,
name: newName
}
})
.then(response => {
// Handle the response
})
.catch(error => {
// Handle errors
});
In this sample code, parameters are passed as a single object to the params
field, and Axios appends them to the URL.
Using Request Body
Apart from the above methods, you can also pass data in the HTTP request body:
const userData = {
id: 123,
name: 'John Doe',
age: 30
};
axios.put('/api/users', userData)
.then(response => {
// Handle the response
})
.catch(error => {
// Handle errors
});
In the above sample code, we create an object called userData
and send it to the /api/users
endpoint using Axios's put
method.
Apidog: Send PUT Requests with Just One Click!
If you need to send PUT requests to update resource data, how can you implement it? In this section, we will introduce you to how you can send PUT requests with just one click using Apidog, an API management tool. Apidog allows you to save both the sent information and the received results when sending PUT requests. You can create API specifications and easily share them with other team members, making it very convenient.
When sending PUT requests with Apidog, you can utilize all PUT request parameters, Axios PUT request with headers and body settings. You can also pass data in various formats such as form-data, x-www-form-urlencoded, JSON, XML, raw, or binary, making it highly versatile and useful.
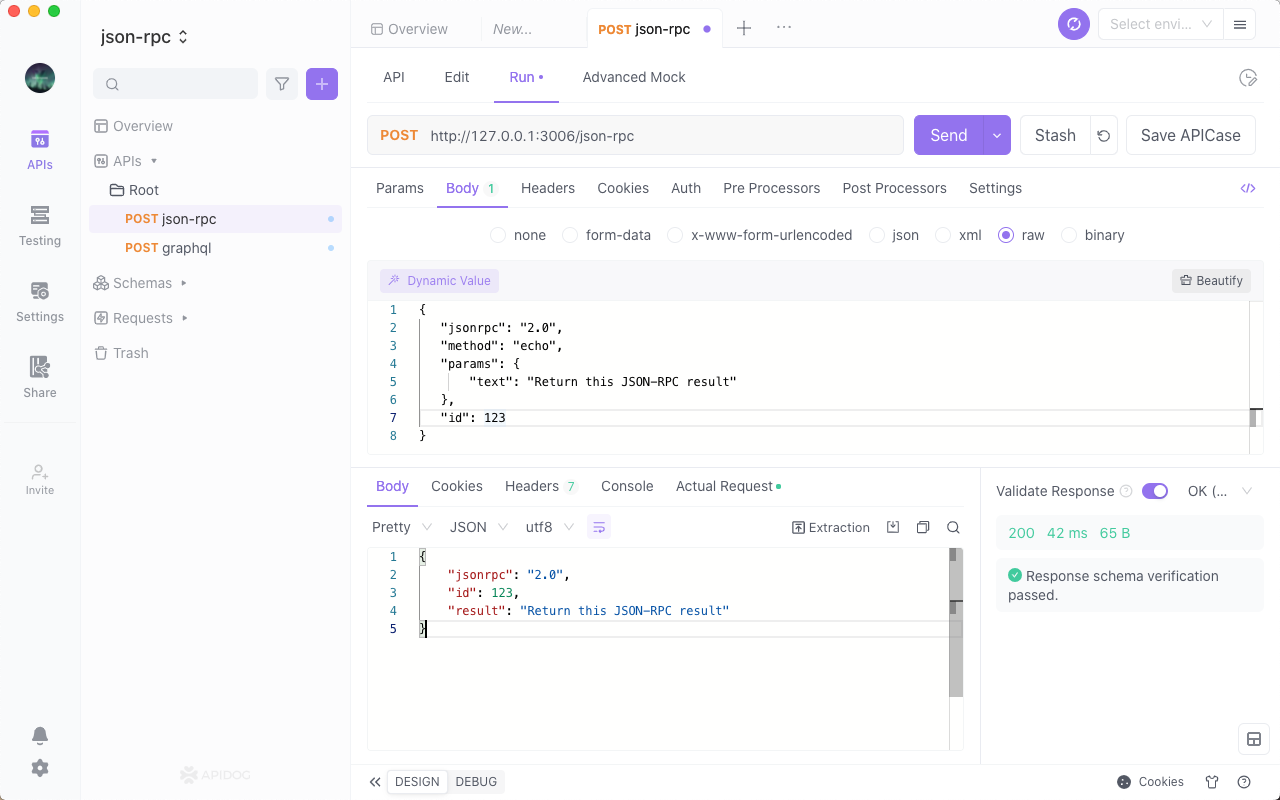