How to Send POST Request with Axios
Axios stands out as a valuable JavaScript HTTP client library, particularly in facilitating seamless communication with APIs. Given that the POST method is extensively employed in HTTP transactions, mastering its implementation in Axios becomes crucial.
Axios stands out as a valuable JavaScript HTTP client library, particularly in facilitating seamless communication with APIs. Given that the POST method is extensively employed in HTTP transactions, mastering its implementation in Axios becomes crucial. You may know how to make an Axios GET request, furthermore, this article aims to guide users through the process of sending POST requests using Axios, shedding light on the fundamental steps involved.
Additionally, the article delves into the intricacies of passing parameters during the transmission of Axios POST requests, elucidating on the nuances of data exchange between the client and the server. Beyond Axios, the article introduces an alternative method that prioritizes intuitiveness, providing users with a straightforward approach to sending API requests and receiving responses.
Axios POST Requests
Axios is a versatile JavaScript library commonly used for making HTTP requests in web applications. It simplifies the process of sending asynchronous requests to servers, making it a popular choice for frontend developers. One of the essential features of Axios is its support for various HTTP methods, including the widely used POST method.
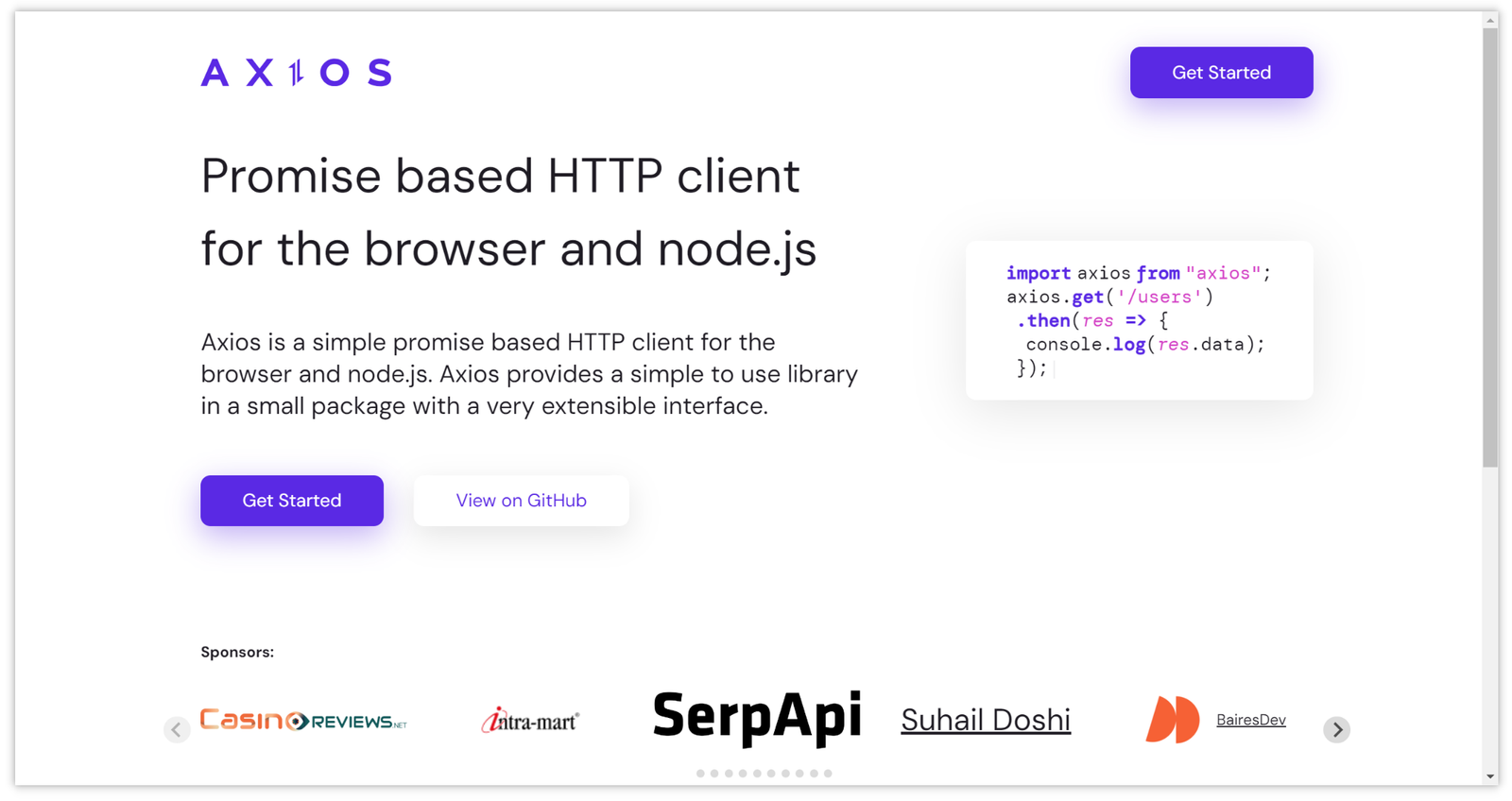
The POST method is employed in scenarios where data needs to be sent to a server to create or update a resource. This could include submitting form data, uploading files, or interacting with APIs that require data input. Axios provides a straightforward and elegant way to handle POST requests, allowing developers to seamlessly send data to a server and process the server's response.
To initiate a POST request with Axios, developers use the axios.post
method, specifying the target URL and the data to be sent in the request body. This method returns a promise, enabling the use of asynchronous programming techniques such as .then()
and .catch()
to handle the server's response or any potential errors.
How to Send POST Request with Axios
1.Install Axios
Before you can start using Axios, you need to install it. If you are working with a Node.js project, you can use npm or yarn to install Axios. Open your terminal and run:
npm install axios
# or
yarn add axios
For front-end projects, you can include Axios via a script tag in your HTML file or use a module bundler like Webpack.
htmlCopy code
<!-- If using a script tag --><script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"</script
2. Import Axios
In your JavaScript file, you need to import Axios before you can use it. If you are using a module bundler like Webpack, you can use the import
statement:
javascriptCopy code
// CommonJSconst axios = require('axios');
// ES6 Modulesimport axios from 'axios';
3. Sending a Basic POST Request
To send a basic POST request, use the axios.post()
method. Provide the URL you want to send the request to and the data you want to include in the request body.
axios.post('https://api.example.com/post-endpoint', {key1: 'value1',key2: 'value2',
})
.then(response => {console.log('Response:', response.data);
})
.catch(error => {console.error('Error:', error);
});
Replace the URL and data with your specific endpoint and payload.
4. Handling Headers
You can include custom headers in your POST request by passing an object containing headers as the third argument to the axios.post()
method.
axios.post('https://api.example.com/post-endpoint', {key1: 'value1',key2: 'value2',
}, {headers: {'Content-Type': 'application/json','Authorization': 'Bearer YOUR_ACCESS_TOKEN',
},
})
.then(response => {console.log('Response:', response.data);
})
.catch(error => {console.error('Error:', error);
});
5. Handling Asynchronous Operations
Axios returns a Promise, making it easy to work with asynchronous code. You can use async/await
or the traditional then
and catch
syntax.
async function postData() {try {const response = await axios.post('https://api.example.com/post-endpoint', {key1: 'value1',key2: 'value2',
});
console.log('Response:', response.data);
} catch (error) {console.error('Error:', error);
}
}
postData();
Apidog: Another Easy Way to Send POST Request
Apidog is a free and user-friendly API testing tool, specifically designed to handle POST requests. It is equipped with robust features that empower developers and testers to seamlessly send real-time HTTP requests to APIs.
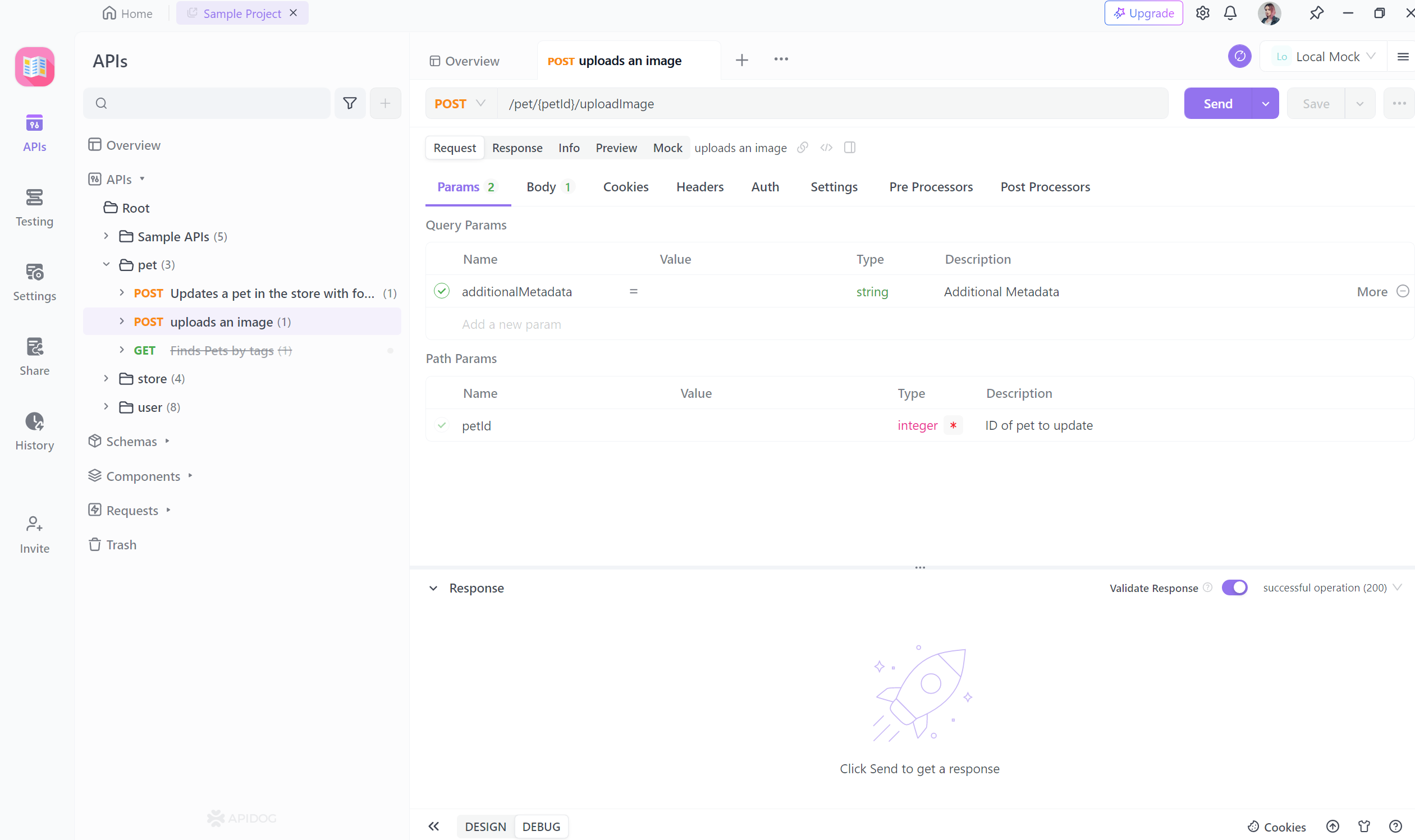
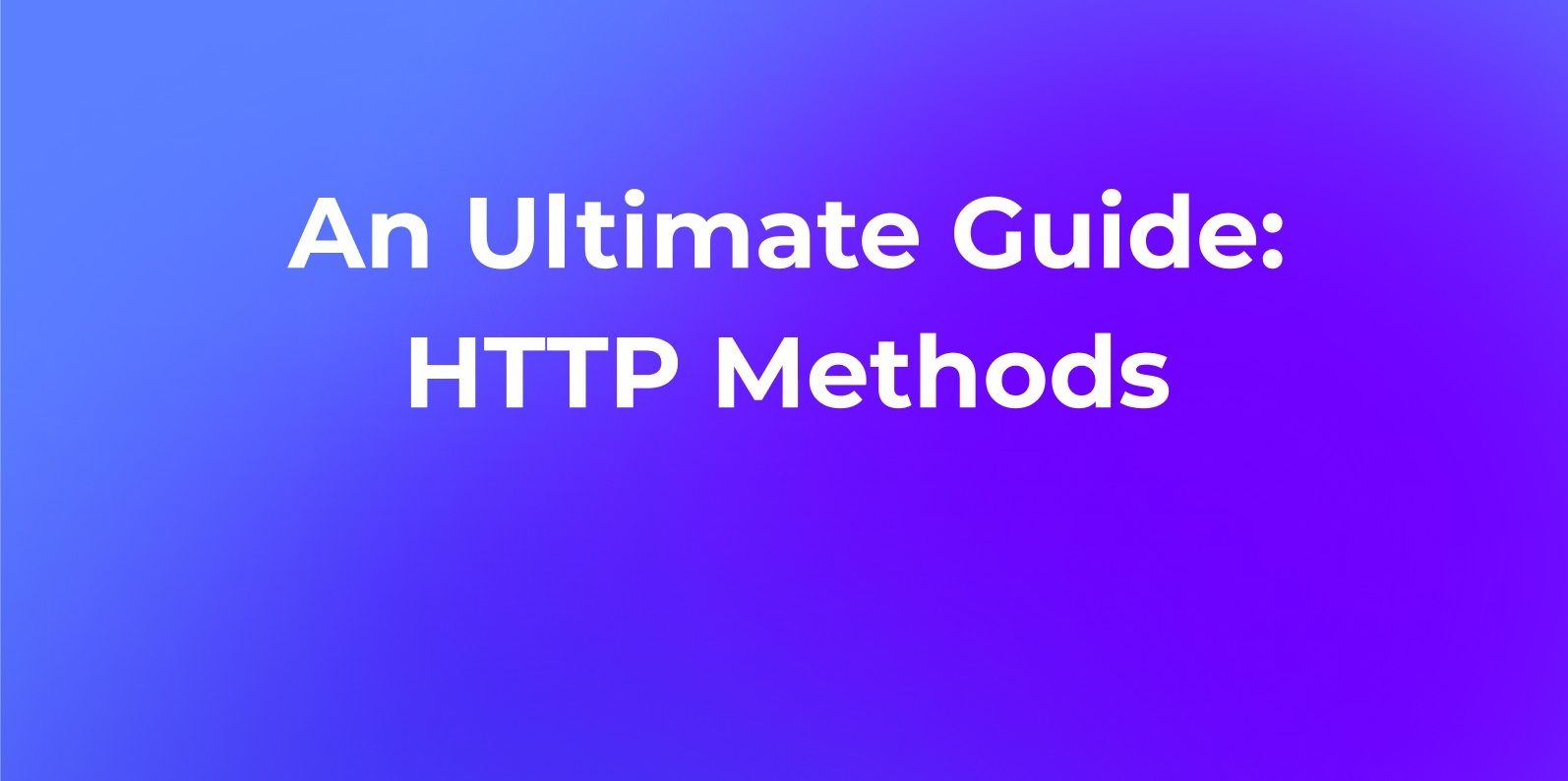
Its simplicity makes it accessible to both beginners and experts, allowing users to easily customize parameters for various testing scenarios. The tool also includes additional features such as test data generation, request and response validation, and smooth integration with CI tools for automation.
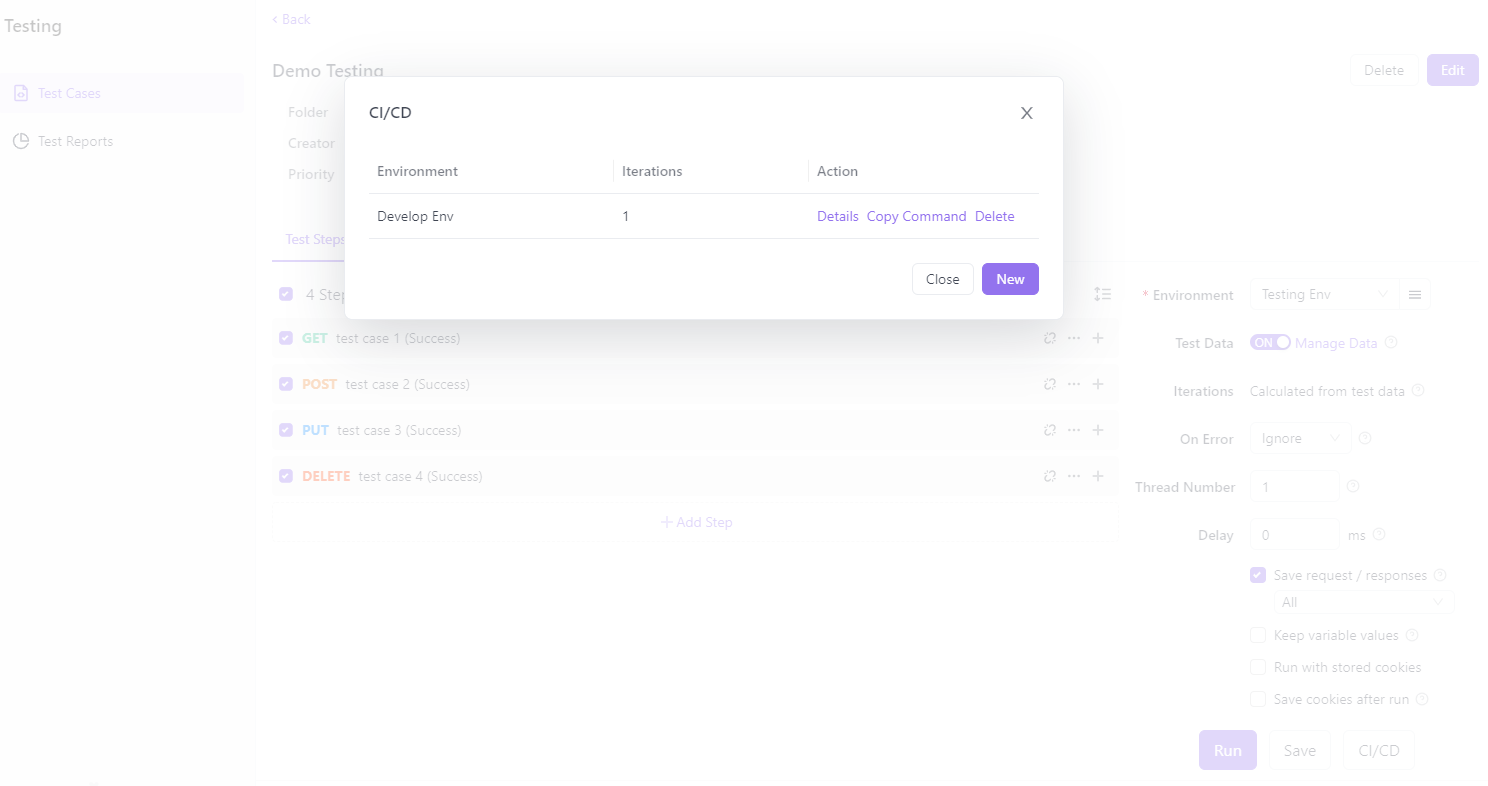
Conclusion
Using Axios to send POST requests is straightforward and powerful. Whether you're building a frontend application or working on the server side, Axios simplifies the process of interacting with APIs. Keep in mind that handling responses and errors appropriately is crucial for building robust applications. Now that you have the basics, you can explore more advanced features of Axios, such as interceptors and request/response transformations, to further enhance your HTTP request handling.