How to Get Streaming Requests with Axios (2 Best Ways)
Axios is a popular HTTP client library for making requests in JavaScript. It provides two effective methods to implement streaming requests and continuously gets server responses. Check it now.
Axios is a popular HTTP client library for making requests in JavaScript. It provides two effective methods to implement streaming requests and continuously gets server responses.
In addition, Axios provides an easy-to-use API and supports various features, including streaming requests. Streaming requests allow us to process data as it arrives, rather than waiting for the entire response to be received. Here is a detailed Axios stream guide for you.
Axios with Streaming
Axios has supported streaming requests since version 0.12. In Axios, you can listen for the data event on the response object to handle a streaming response.
Here are some ways streaming can be useful with Axios:
- For long-running requests or server-sent events, streaming allows processing the response incrementally as data arrives from the server, instead of waiting for the entire response to complete.
- Streaming can facilitate piping response data to other streams, like writing to files. Chunks of response data can be piped seamlessly as they arrive.
- For large responses that would take up too much memory, streaming prevents having to buffer the entire response before processing. The response can be handled in smaller chunks.
- Streaming enables showing progress indicators for long requests, since the progress of the response can be monitored as chunks are received.
- If the client needs to receive just a portion of the response, streaming allows aborting the response early once sufficient data is retrieved.
How to Make Streaming Requests with Axios?
Here are two common ways to implement streaming requests with Axios:
Pipe the Stream to a Writable Stream
Request with Axios () and get the response. Listen to the data
event on the response and pipe the data to a Writable Stream like fs.createWriteStream
.
const axios = require('axios');
const fs = require('fs');
const writer = fs.createWriteStream('example.pdf');
axios({
method: 'get',
url: '/example.pdf',
responseType: 'stream'
})
.then(response => {
response.data.pipe(writer);
});
Manually Listen for the data
Event
Make a request with Axios () and get the response. Listen for the data
event on the response. In the event handler, process the streaming data manually:
const axios = require('axios');
axios({
method: 'get',
url: '/example.pdf',
responseType: 'stream'
})
.then(response => {
response.data.on('data', (chunk) => {
// logic to process stream data
});
response.data.on('end', () => {
// logic for stream complete
});
});
By listening for events on the response data stream, we can handle streaming responses and process the continuously arriving data.
Axios Stream Example
Here is a simple example of building a server with Express and making a streaming request with Axios to save the response data into a file. The detailed steps are as follows:
- First, install the Axios and express modules:
npm install axios
npm install express
2. This example needs a file file.txt as the data source on the server side. You can prepare this file yourself (the file content is not specified, you can put any content in it).
3. Create a server.js file. In server.js, use express to start a web server, and define a /stream route, the code is:
// Import the express module
const express = require('express');
// Create express app
const app = express();
// Define /stream route
app.get('/stream', (req, res) => {
// Read file.txt and pipe to response
const stream = fs.createReadStream('file.txt');
stream.pipe(res);
});
// Start server listening on port 3000
app.listen(3000);
Note: The command to start the server is node server.js
4. Create a client.js file. In client.js, make a request using Axios:
// Import axios
const axios = require('axios');
const fs = require('fs');
const writer = fs.createWriteStream('output.txt');
// Make GET request to /stream route
axios({
method: 'GET',
url: 'http://localhost:3000/stream',
responseType: 'stream' // Declare response type as stream
})
.then(response => {
// Get response stream via data event
response.data.pipe(writer);
});
Note: The command to start client is node client.js
In this example, the output.txt file is generated, showing the file stream transmission is achieved.
Debugging Backend APIs with Apidog
Apidog integrates features from Postman, Swagger, Mock, JMeter, and more into one platform. Apidog supports debugging APIs over protocols like HTTP(S), WebSocket, Socket, gRPC, Dubbo, etc.
During testing after the backend developer has written the API, Apidog can be used to validate the correctness of the interfaces through its graphical API which greatly improves the efficiency of deployment.
In the example of this article, the API can be tested using Apidog. After creating a new project, select "Debug Mode" in the project, fill in the request address and you can quickly send requests and get response results. The practice case in the previous section is shown below using Apidog:
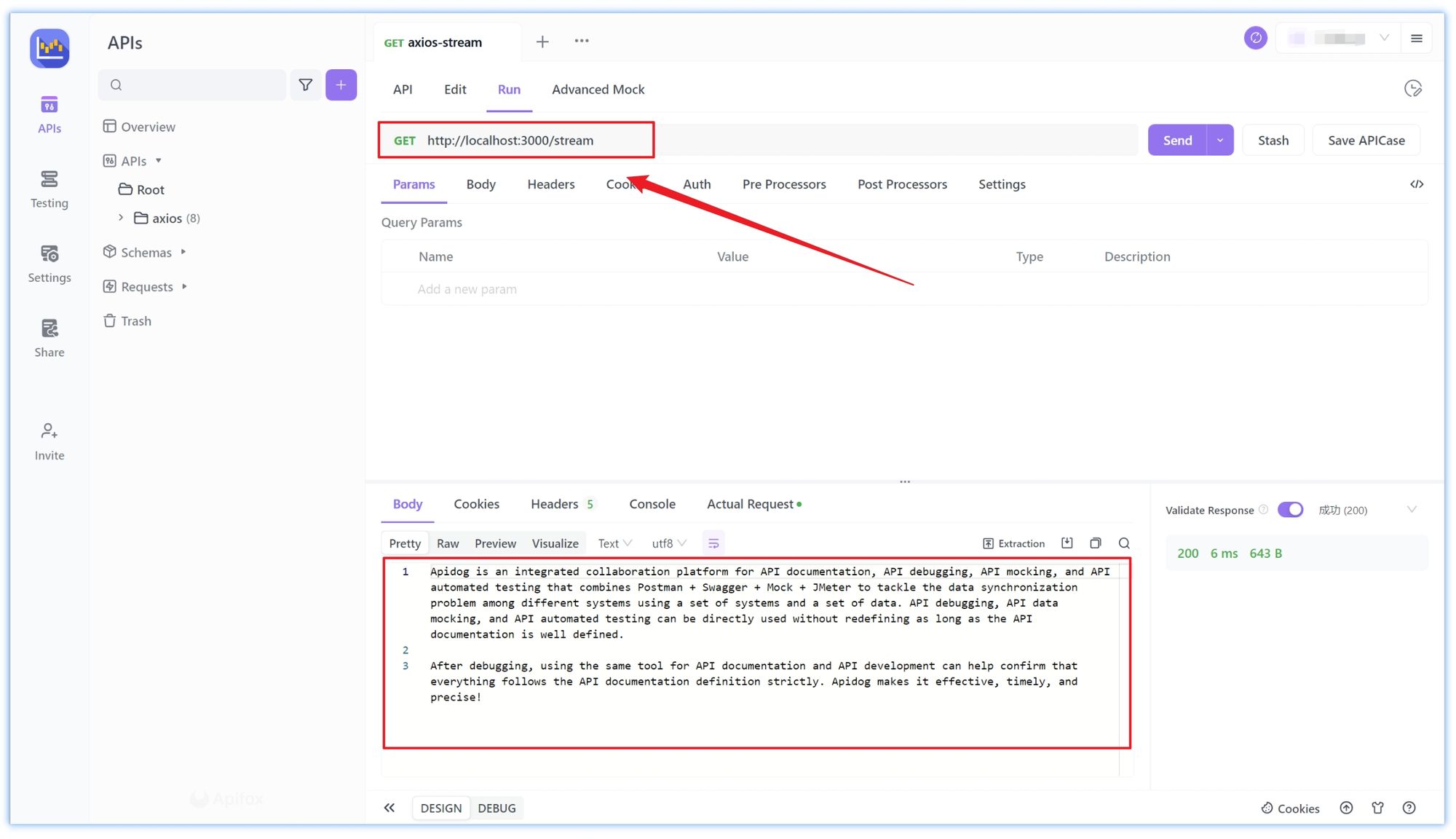
Tips and Notes
- The response type must be set to 'stream' to indicate the response is a stream.
- When piping data, stream events including finish and error must be handled properly.
- Stream requests do not support configurations like timeout, cancel token, validateStatus, etc.
- The stream can only be consumed once, a new request is needed to read again.
- Stream data may arrive out of order, so orders must be handled properly.
Conclusion
Axios enables streaming requests by listening to the data event on the response object. The main approaches are piping the stream directly or handling events manually. Using streams allows streaming file or data transmission, effective memory management, and improved efficiency. But stream events need to be handled properly.