Axios vs Fetch: Which is best for HTTP requests in 2024?
There are many ways to make HTTP requests in JavaScript, but two of the most popular ones are Axios and fetch(). In this post, we will compare and contrast these two methods and see which one is best for different scenarios.
HTTP requests are essential for communicating with servers and APIs in web applications. There are many ways to make HTTP requests in JavaScript, but two of the most popular ones are Axios and fetch(). In this post, we will compare and contrast these two methods and see which one is best for different scenarios.
What is Axios and why should you use it?
Axios is a third-party library that provides a promise-based HTTP client for making HTTP requests. Axios is widely used in the JavaScript community and is known for its simplicity and flexibility
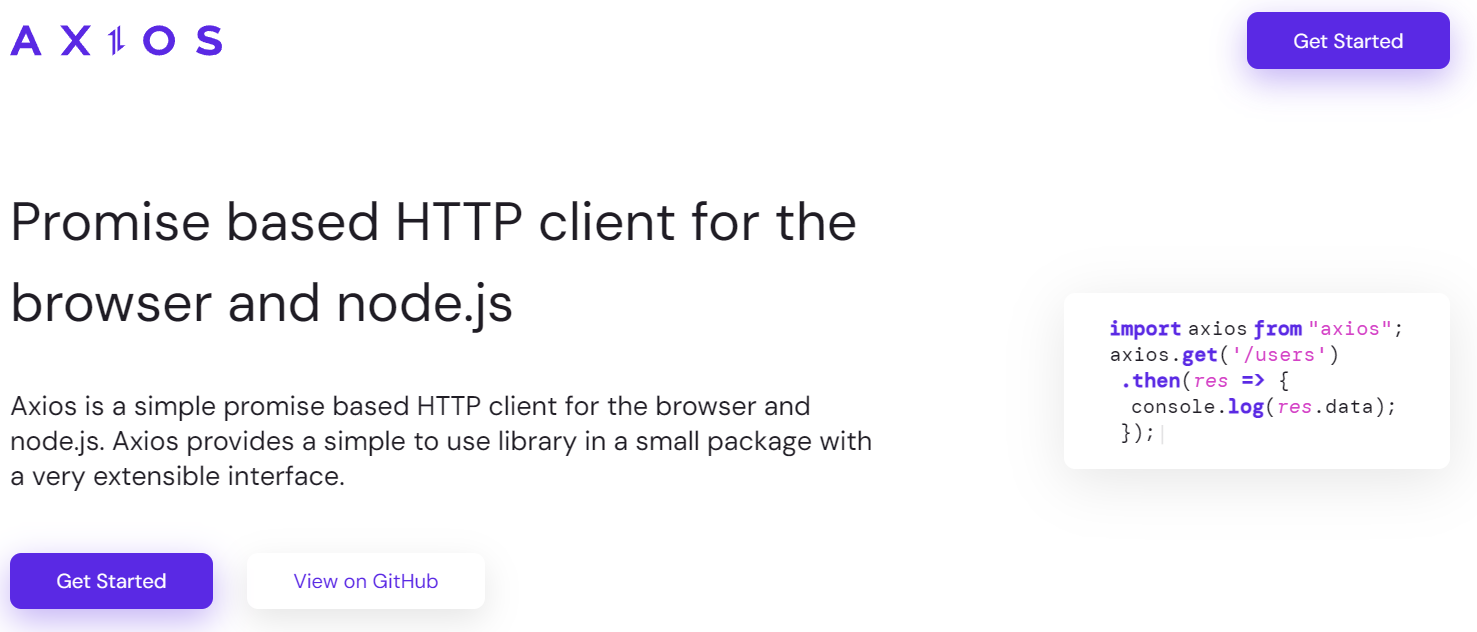
It has many features that make it easy and convenient to use, such as:
- Automatic JSON data transformation: Axios automatically converts the data to and from JSON, so you don’t have to manually parse or stringify it.
- Response timeout: Axios allows you to set a timeout for your requests, so you can handle errors if the server takes too long to respond.
- HTTP interceptors: Axios lets you intercept requests and responses before they are handled by then or catch, so you can modify them or add additional logic.
- Download progress: Axios can track the progress of your downloads and uploads, so you can display feedback to the user or cancel the request if needed.
- Simultaneous requests: Axios can make multiple requests at the same time and combine them into a single response using axios.all and axios.spread.
What is fetch()?
fetch() is a built-in API that comes with native JavaScript. It is an asynchronous web API that returns the data in the form of promises. fetch() is supported by all modern browsers, so you don’t need to import any external library to use it. Some of the features of fetch() are:
- Basic syntax: fetch() has a simple and concise syntax that takes the URL of the resource you want to fetch as the first argument and an optional options object as the second argument.
- Backward compatibility: fetch() can be used in older browsers that don’t support it by using a polyfill, such as whatwg-fetch or fetch-ponyfill.
- Customizable: fetch() gives you more control over your requests and responses, as you can customize the headers, body, method, mode, credentials, cache, redirect, and referrer policies.
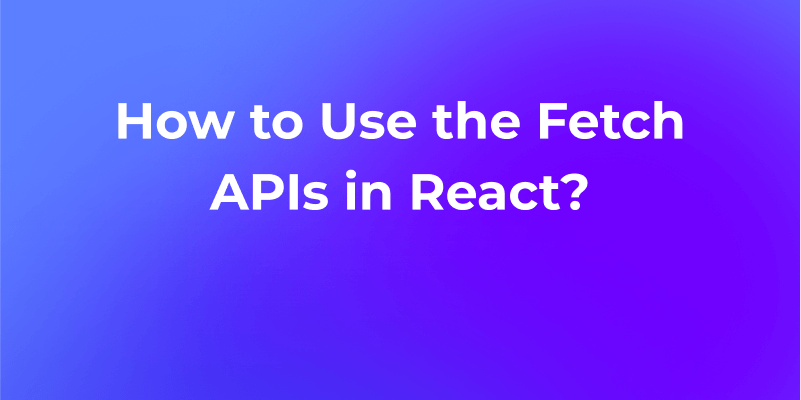
How to use Axios to make HTTP requests?
To use Axios, you need to install it using npm or yarn:
npm install axios
And here’s how to install Axios using yarn:
yarn add axios
If you prefer to use pnpm, you can install Axios using the following command:
pnpm install axios
Alternatively, you can use a content delivery network (CDN) to include Axios in your project. Here’s how to include Axios using a CDN:
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
Then, you can import it in your JavaScript file and use it to make HTTP requests. For example, to make a GET request to a URL, you can use axios.get():
import axios from 'axios';
axios.get('https://example.com/api')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
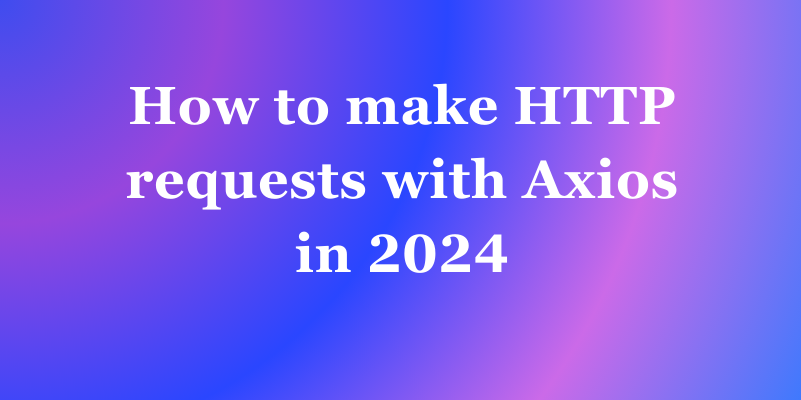
Making HTTP requests with fetch
To use fetch(), you don’t need to install anything, as it is already available in the browser. You can use the fetch() function to make HTTP requests. For example, to make a GET request to a URL, you can use fetch() like this:
fetch('https://example.com/api')
.then(response => {
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
Notice that:
- Axios automatically transforms the data to and from JSON, while fetch() requires you to call response.json() to parse the data to a JavaScript object.
- Axios provides the data in the response object, while fetch() provides the response object itself, which contains other information such as status, headers, and url.
- Axios handles errors in the catch block, while fetch() only rejects the promise if there is a network error, not if the response has an error status.
Comparing the syntax of Fetch and Axios
The syntax of Fetch and Axios are similar, but there are some differences. Here are some of the main ones:
- Fetch accepts the URL as the first argument and an optional options object as the second argument. Axios can take the URL as the first argument and an options object as the second argument, or an object that contains both the URL and the options as the first argument.
- Fetch uses the body property to send data to the server, while Axios uses the data property. Fetch also requires the data to be stringified, while Axios automatically converts the data to and from JSON.
- Fetch returns the response object itself, which contains other information such as status, headers, and url. Axios returns the data in the response object, which is more convenient for accessing the data directly.
- Fetch only rejects the promise if there is a network error, not if the response has an error status. Axios handles errors in the catch block, which makes it easier to deal with error responses.
They have some similarities and differences in their syntax:
- Sending a GET request with query parameters:
// Axios
axios.get('/api/data', {
params: {
name: 'Alice',
age: 25
}
})
.then(response => {
// handle response
})
.catch(error => {
// handle error
});
// Fetch
const url = new URL('/api/data');
url.searchParams.append('name', 'Alice');
url.searchParams.append('age', 25);
fetch(url)
.then(response => response.json())
.then(data => {
// handle data
})
.catch(error => {
// handle error
});
- Sending a POST request with a JSON body:
// Axios
axios.post('/api/data', {
name: 'Bob',
age: 30
})
.then(response => {
// handle response
})
.catch(error => {
// handle error
});
// Fetch
fetch('/api/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
name: 'Bob',
age: 30
})
})
.then(response => response.json())
.then(data => {
// handle data
})
.catch(error => {
// handle error
});
- Setting a timeout for the request:
// Axios
axios.get('/api/data', {
timeout: 5000 // 5 seconds
})
.then(response => {
// handle response
})
.catch(error => {
// handle error
});
// Fetch
const controller = new AbortController();
const signal = controller.signal;
setTimeout(() => {
controller.abort(); // abort after 5 seconds
}, 5000);
fetch('/api/data', { signal })
.then(response => response.json())
.then(data => {
// handle data
})
.catch(error => {
// handle error
});
- Using async/await syntax:
// Axios
async function getData() {
try {
const response = await axios.get('/api/data');
// handle response
} catch (error) {
// handle error
}
}
// Fetch
async function getData() {
try {
const response = await fetch('/api/data');
const data = await response.json();
// handle data
} catch (error) {
// handle error
}
}
Error Handling: Axios vs Fetch
Error handling is an important aspect of making HTTP requests, as it allows you to handle different scenarios such as network failures, server errors, or invalid responses. Fetch and Axios have different ways of handling errors, which I will compare with some examples.
- In Axios, if there is a request failure, it throws an error and you can easily handle the error with a try-catch block and get the error data from
error.response.data
. For example:
// Axios error handling with try-catch
try {
const response = await axios.get('/api/data');
// handle response
} catch (error) {
// handle error
console.log(error.response.data);
}
- In Fetch, if there is a request failure, it does not throw an error, but returns a response object with an
ok
property set to false. You have to check theok
property and throw an error manually if you want to use a try-catch block. Alternatively, you can use theresponse.ok
property to handle different cases in thethen
method. For example:
// Fetch error handling with try-catch
try {
const response = await fetch('/api/data');
// check response status
if (!response.ok) {
// throw error if status is not ok
throw new Error(`HTTP error! status: ${response.status}`);
}
// handle response
} catch (error) {
// handle error
console.log(error.message);
}
// Fetch error handling with response.ok
fetch('/api/data')
.then(response => {
// check response status
if (response.ok) {
// handle response
} else {
// handle error
console.log(`HTTP error! status: ${response.status}`);
}
})
.catch(error => {
// handle network error
console.log(error.message);
});
- Another difference between Fetch and Axios is how they handle non-200 status codes. Axios considers any status code outside the range of 2xx as an error and rejects the promise. Fetch considers any valid HTTP response (even 4xx or 5xx) as a success and resolves the promise. This means that you have to handle non-200 status codes differently in Fetch and Axios. For example:
// Axios error handling for non-200 status codes
axios.get('/api/data')
.then(response => {
// handle response
})
.catch(error => {
// handle error
if (error.response) {
// server responded with a status code outside 2xx
console.log(error.response.status);
console.log(error.response.data);
} else {
// network error or request was aborted
console.log(error.message);
}
});
// Fetch error handling for non-200 status codes
fetch('/api/data')
.then(response => {
// check response status
if (response.ok) {
// handle response
} else {
// server responded with a status code outside 2xx
console.log(response.status);
return response.json();
}
})
.then(data => {
// handle error data
console.log(data);
})
.catch(error => {
// handle network error or request was aborted
console.log(error.message);
});
Axios or Fetch: Which one is better?
There is no definitive answer to which one is better, as it depends on your preferences and needs. However, here are some general guidelines to help you decide:
- Use Axios if you want a simple and convenient way to make HTTP requests, with features such as automatic JSON data transformation, response timeout, HTTP interceptors, download progress, and simultaneous requests.
- Use fetch() if you want a native and customizable way to make HTTP requests, with features such as backward compatibility, custom headers, body, method, mode, credentials, cache, redirect, and referrer policies.
Generate Axios/Fetch code with Apidog
Apidog is an all-in-one collaborative API development platform that provides a comprehensive toolkit for designing, debugging, testing, publishing, and mocking APIs. Apidog enables you to automatically create Axios code for making HTTP requests.
Here's the process for using Apidog to generate Axios code:
Step 1: Open Apidog and select new request
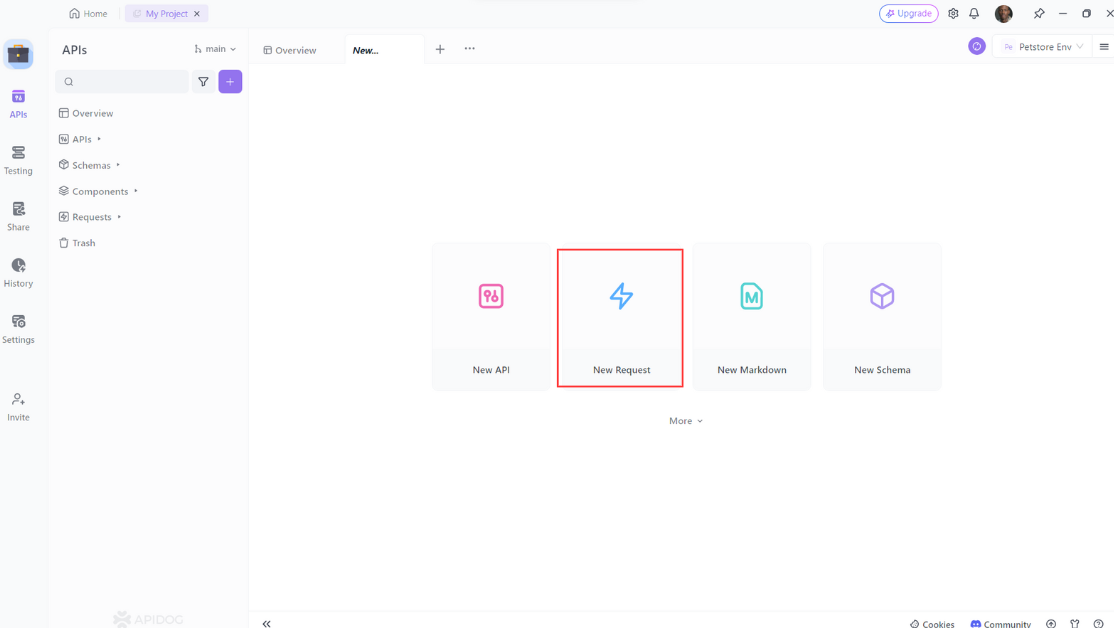
Step 2: Enter the URL of the API endpoint you want to send a request to,input any headers or query string parameters you wish to include with the request, then click on the "Design" to switch to the design interface of Apidog.
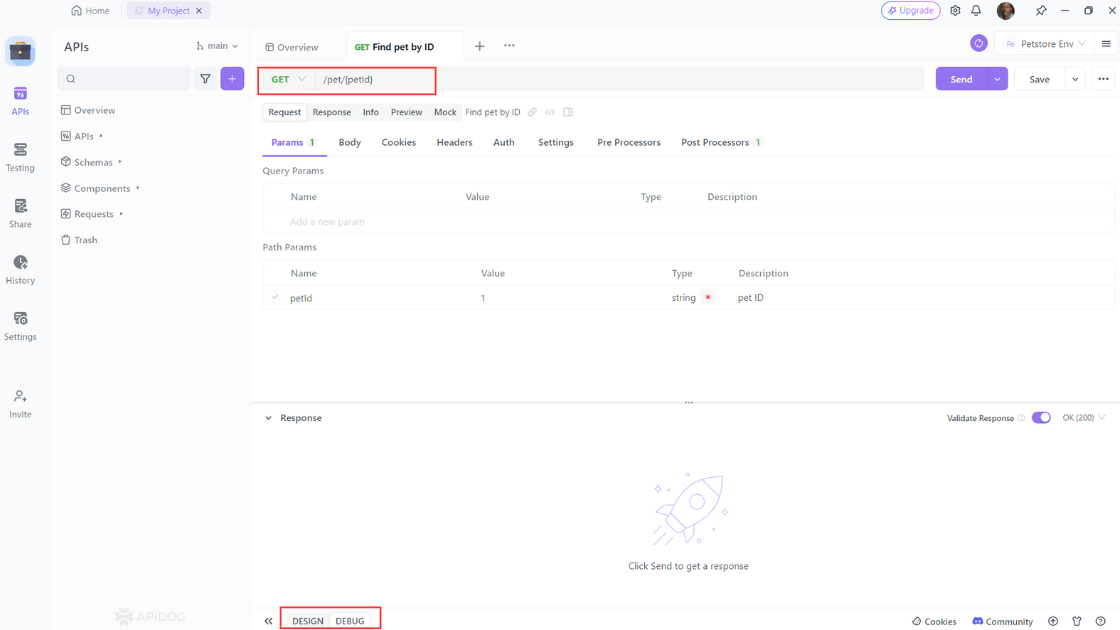
Step 3: Select "Generate client code " to generate your code.
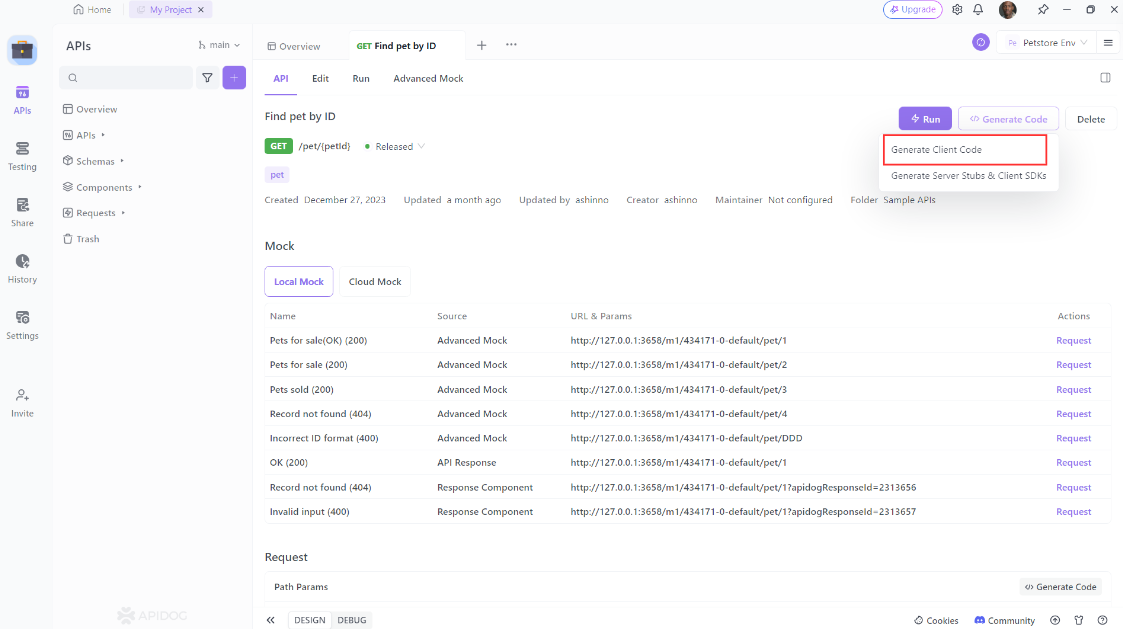
Step 4: Copy the generated Axios code and paste it into your project.
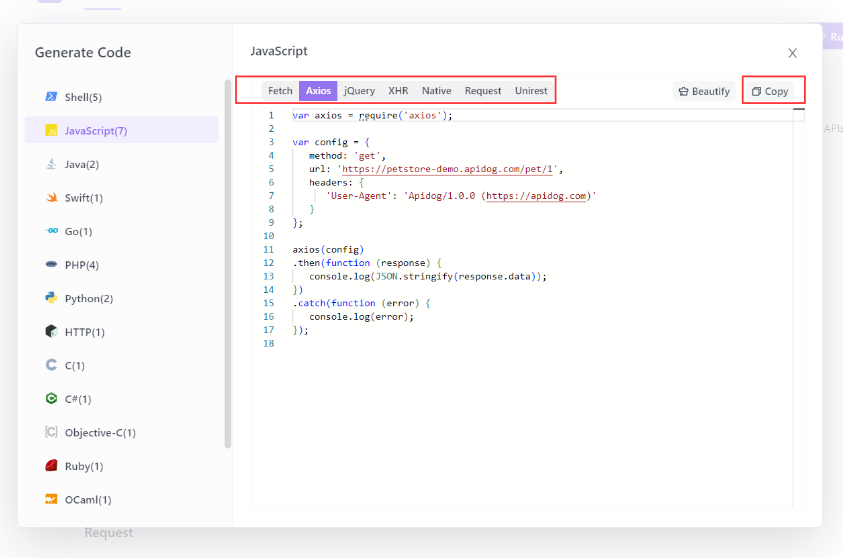
Using Apidog to Send HTTP Requests
Apidog offers several advanced features that further enhance its ability to test HTTP requests. These features allow you to customize your requests and handle more complex scenarios effortlessly.
Step 1: Open Apidog and create a new request.
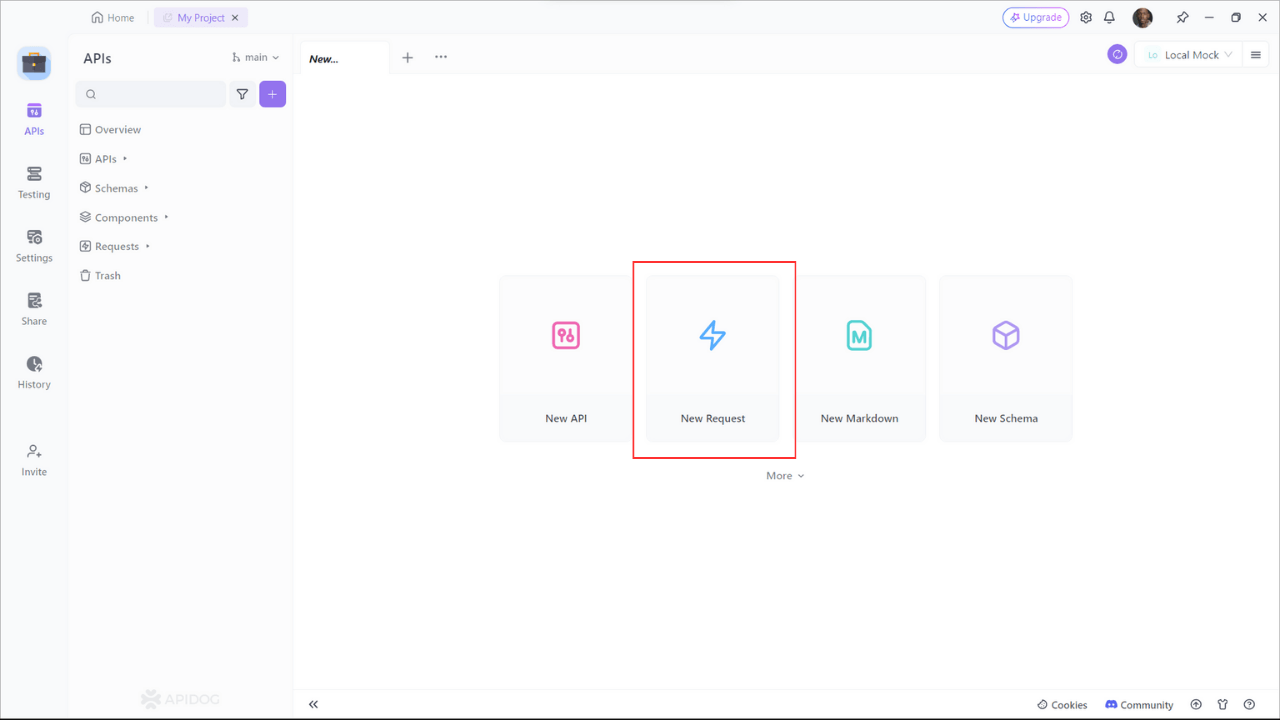
Step 2: Find or manually input the API details for the POST request you want to make.
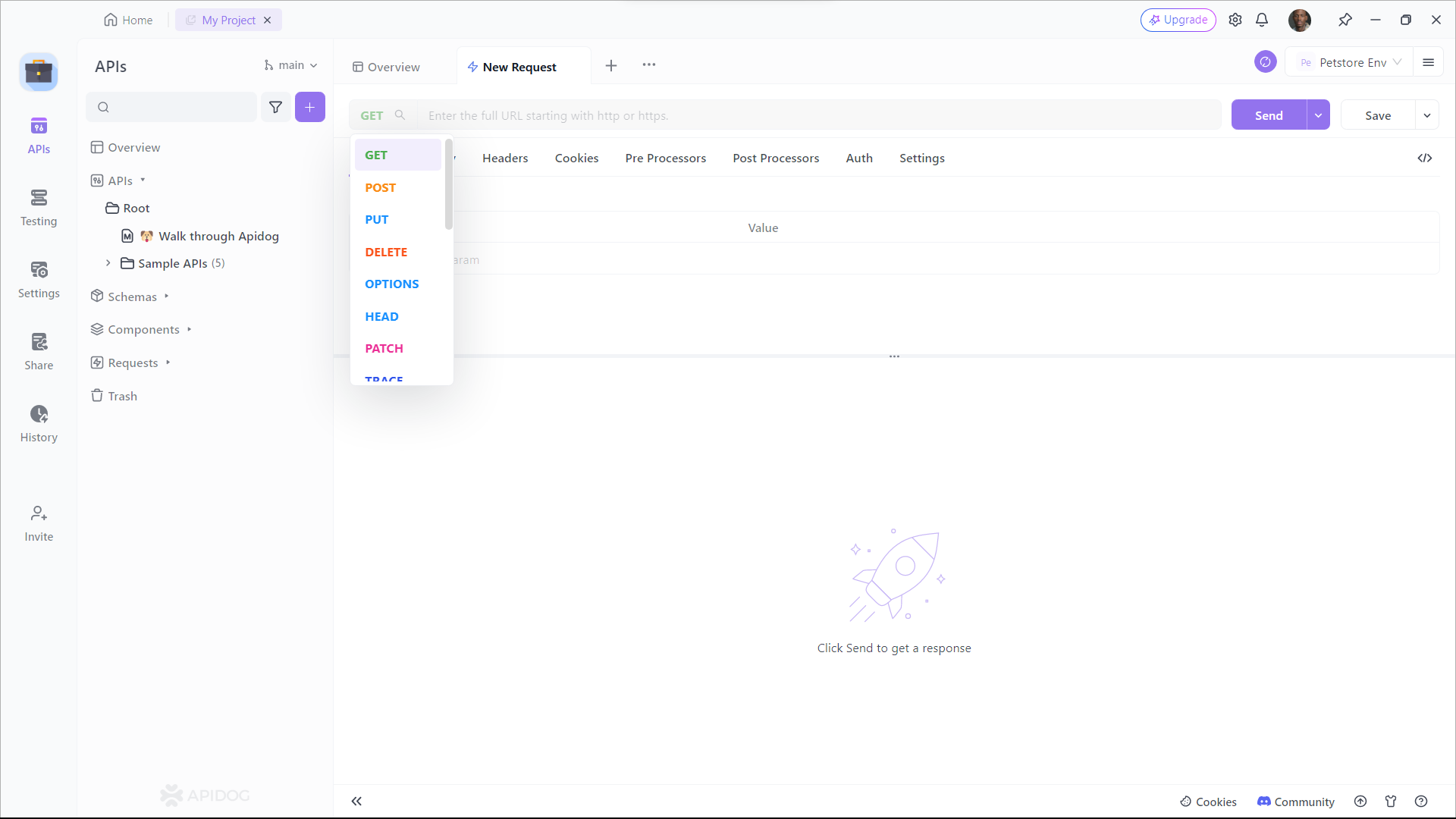
Step 3: Fill in the required parameters and any data you want to include in the request body.
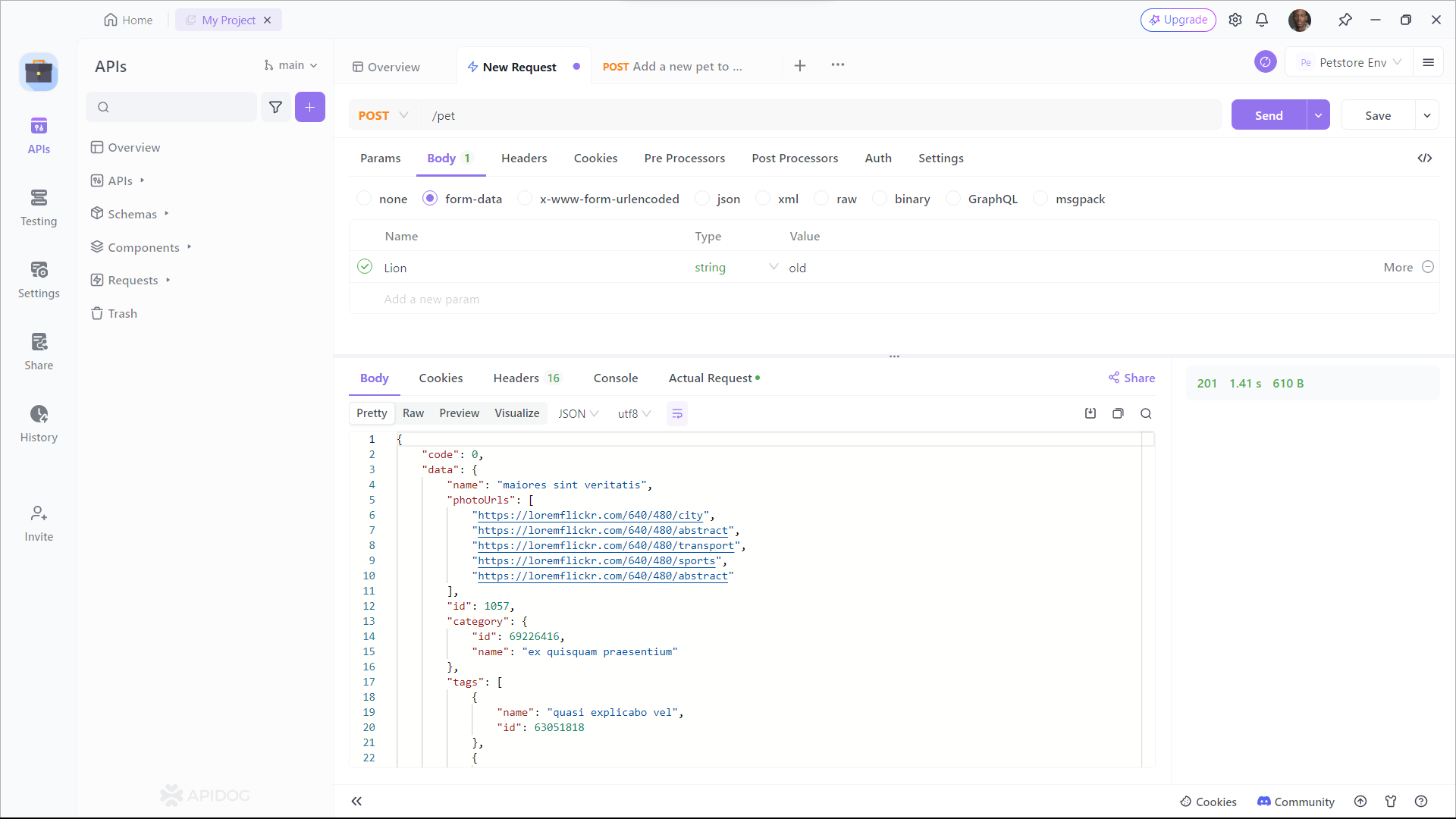
Conclusion
Both Axios and fetch() are powerful and reliable methods for making HTTP requests in JavaScript. You can choose the one that suits your project and style better, or even use both of them for different purposes. The important thing is to understand how they work and how to use them effectively.
Using Apidog not only saves you valuable time and effort but also ensures that your code is accurate and error-free. With its user-friendly interface and intuitive features, Apidog is a must-have tool for any developer working with Axios requests. Happy coding!