React Fetch vs Axios: Comparing HTTP Request Libraries in React
Fetch is a native JS interface for HTTP requests supported by modern browsers. Axios is a more full-featured third-party library requiring installation. Axios provides richer features like automatic JSON parsing, error handling, interceptors.
When building React applications, we often need to make HTTP requests to retrieve or send data to a server. The two most popular methods for making HTTP requests in React are the native Fetch API and the third party Axios library. Both have their pros and cons and can accomplish the same tasks. In this article we will compare Fetch and Axios to help you decide which one may work best for your React app.
React Fetch vs Axios
Here is a comparison of Fetch and Axios.js for making HTTP requests for a quick look.
Fetch is a native JS interface for HTTP requests supported by modern browsers. Axios is a more full-featured third-party library requiring installation. Axios provides richer features like automatic JSON parsing, error handling, interceptors.
Difference | Axios | Fetch |
---|---|---|
Library Type | Third party library, needs to be installed via npm | Native JS interface, no extra installation needed |
Browser Support | Supports all popular browsers | Supports all modern browsers except IE |
Syntax | More humane syntax | Concise syntax |
HTTP Methods | Supports all HTTP methods | Only supports GET and POST |
Parameter Passing | Supports multiple ways of parameter passing | Can only pass params in body or URL |
Error Handling | Has built-in error handling mechanism | Need to manually catch and handle errors |
Response Result | Parses response to JSON by default | Returns raw response, needs manual parsing |
Configuration | Provides richer configuration options | Limited configuration options |
Interceptors | Supports adding interceptors | Does not support intercepting requests and responses |
Fetch API
The Fetch API is native to most modern browsers and utilizes promises. Some key things to know about Fetch:
- Native to browsers so no need to install a separate library
- Uses promises and is therefore familiar to JS developers used to async/await
- Simple syntax but requires manually handling some things like errors
- Cannot monitor request progress like upload progress
- Requires parsing JSON response with .json()
- Limited browser support on older browsers
Here is an example Fetch request to get JSON data in React:
Copy code
fetch('/api/data')
.then(response => response.json())
.then(data => {
// use data
})
Axios
Axios is a third party library that can be installed into React apps. Some key aspects of Axios:
- Small size with minimal dependencies
- Supports older browsers
- Automatic transforms for JSON data
- Monitoring of request progress
- Response timeout handling
- Automatic error handling
- Ability to cancel requests
- Support for security features like CORS
Here is an example Axios request for getting JSON data:
axios.get('/api/data')
.then(response => {
// use response.data
})
As you can see, the syntax is quite similar to Fetch. However, Axios handles some things automatically that you would need to handle manually with Fetch.
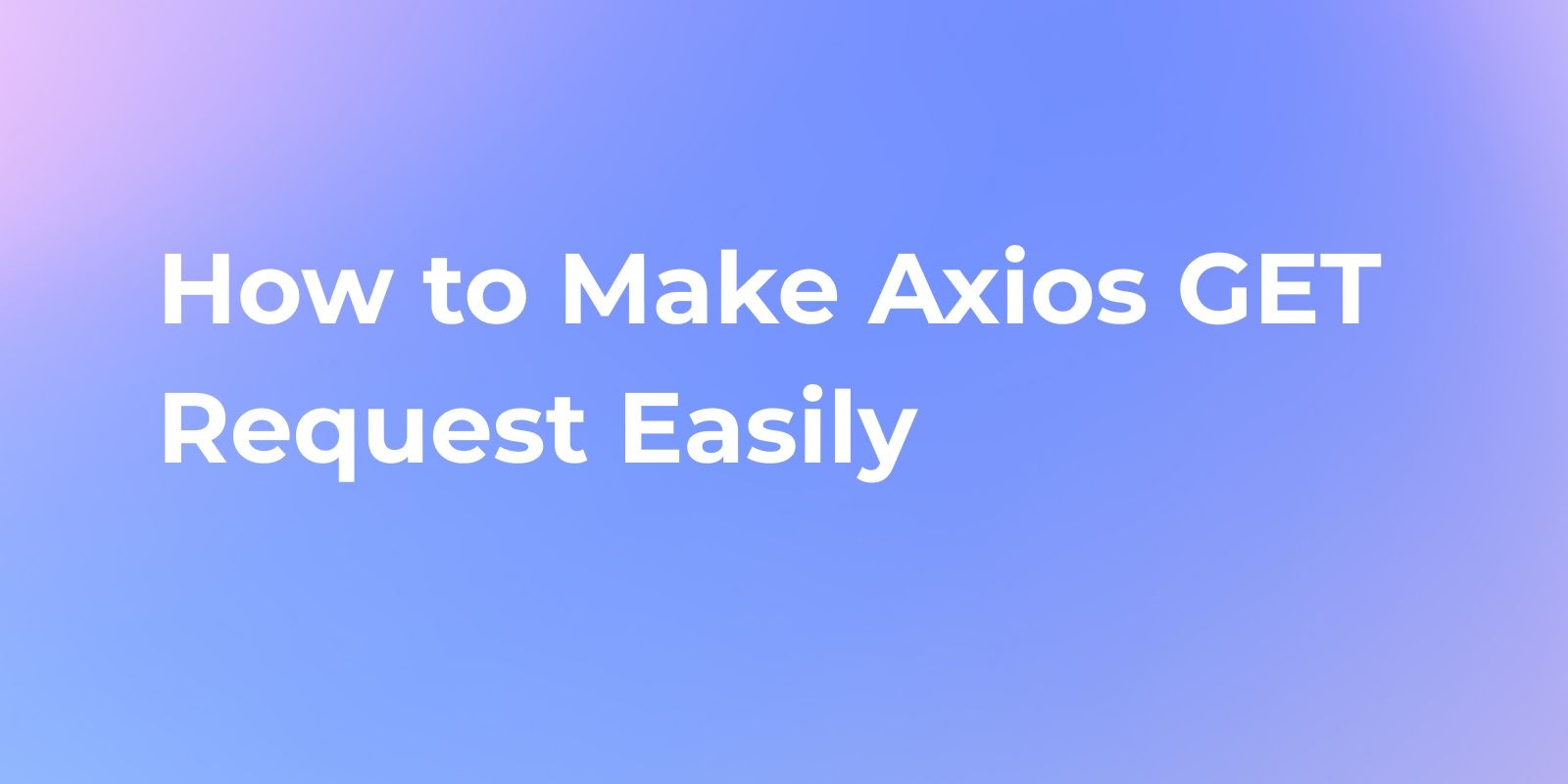
Key Differences
- Fetch is native while Axios is a third party library
- Fetch requires manually parsing JSON but Axios does this automatically
- Fetch has less browser support while Axios supports older browsers
- Axios has ability monitor progress, timeout, and cancel requests
- Axios automatically transforms errors while Fetch requires manual error handling
- Axios has built-in security features like CORS support
What is Apidog
Fetching data is critical for React apps, but manually coding API requests can be tedious. Apidog streamlines this process through automatic client code generation from backend API specs.
Simply click the Apidog icon to produce tailored Fetch requests matched to the needed endpoints and data structures. This saves developers effort and avoids errors that stem from hand-coding HTTP requests.
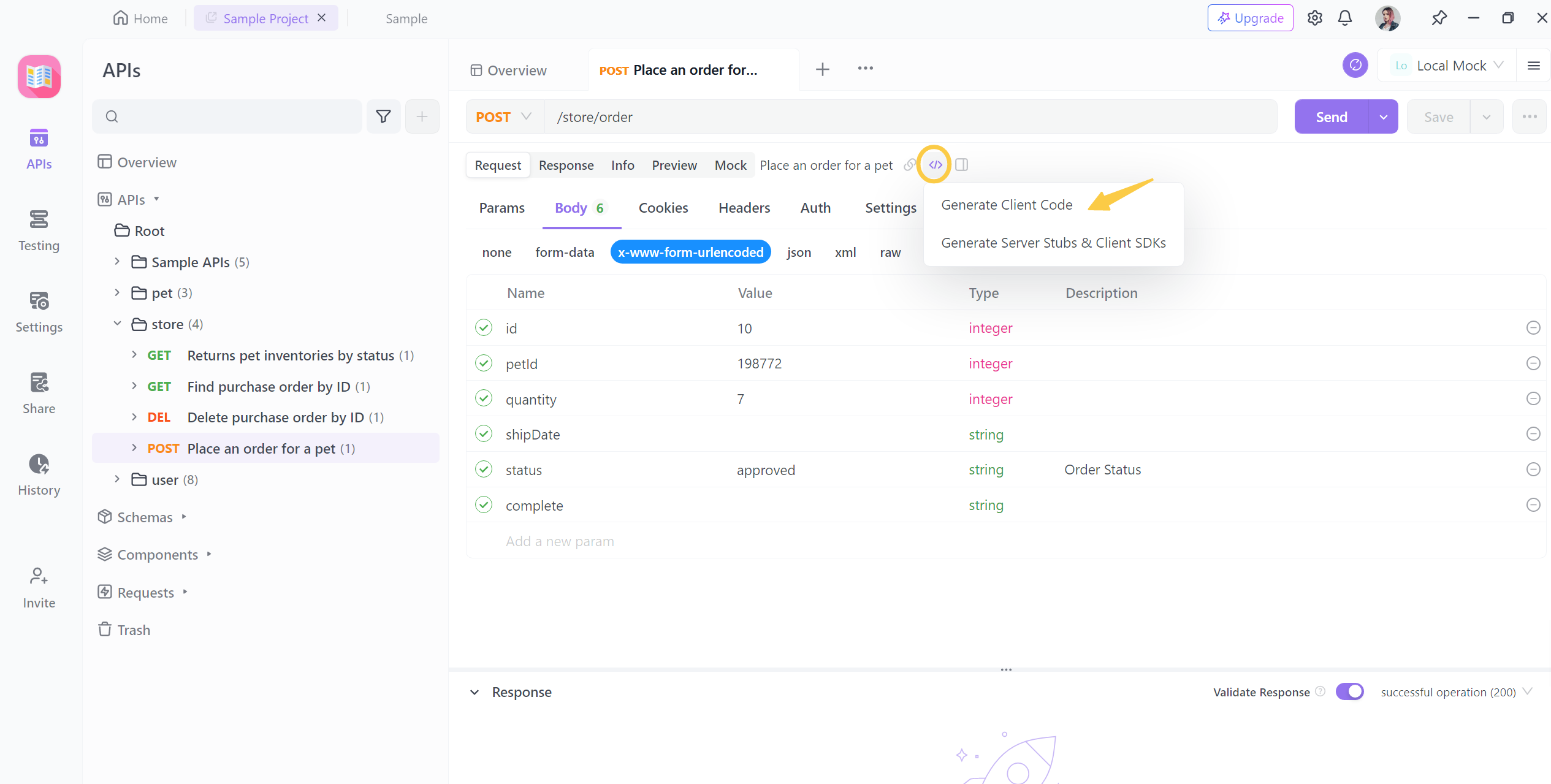
Apidog also empowers developers to mock responses before backends are ready, enabling frontend work to proceed independently. With just one click, fake data can stand in to support UI/UX development and application logic.
By automating client code generation and mocking, Apidog enables smooth data integration in React apps without the headache. It removes friction from linking frontends to backends, letting developers concentrate on building robust and user-friendly applications.
The auto-generated Fetch code, modifiable endpoints, and response mocking maximize productivity while laying the groundwork for robust and sustainable React projects.
Conclusion
In summary, Fetch and Axios can both be used to make API calls in React. Fetch is simpler syntactically but Axios includes more built-in capabilities. If you need these additional features or wider browser support, Axios may be a better choice.
However, Fetch can be useful for basic HTTP requests in newer browser environments. Consider the needs of your application to determine if native Fetch or Axios is more suitable.