In today's rapidly evolving digital world, APIs (Application Programming Interfaces) are the building blocks that enable different software applications to communicate with each other. Whether you're developing a mobile app, integrating with third-party services, or building a robust web platform, understanding the various types of API calls is essential. But, what are API calls, and how do they work? Let’s dive deep into this topic, exploring the different types of API calls and why they matter in modern software development.
What is an API Call?
Let's start with the basics. An API call is essentially a request made by one software application to another, asking for data or actions to be performed. Think of it as a way for different pieces of software to communicate and share resources. When an API call is made, the requesting application asks the server for information, and the server responds with the requested data. This exchange happens in a matter of milliseconds, enabling seamless functionality across platforms and devices.
APIs can be classified into various categories, and understanding the types of API calls can help developers choose the right approach for their projects. So, what types of API calls are there? Let’s explore.
Understanding the Types of API Calls
APIs are like the glue that holds different applications together. They are versatile and can be used in a multitude of ways, depending on the needs of the application. Here’s a breakdown of the most common types of API calls:
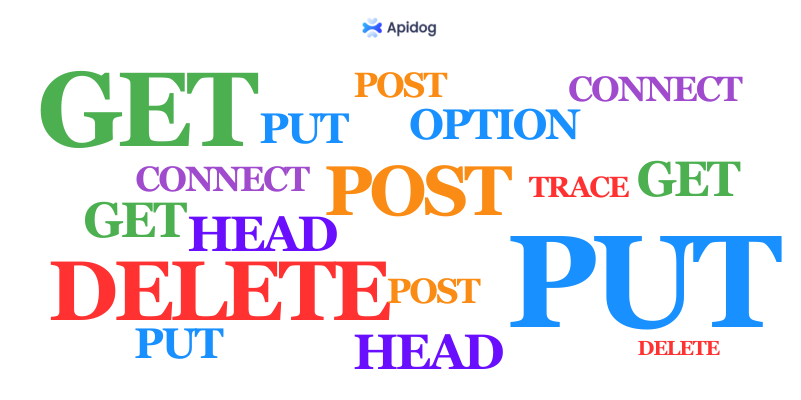
1. GET Requests
GET requests are the most common type of API call. As the name suggests, a GET request is used to retrieve data from a server. Imagine you're visiting a website to view a list of products. When you click on a link to view more details about a product, your browser sends a GET request to the server. The server then responds by sending back the product details, which are displayed on your screen.
GET requests are simple, efficient, and widely used in various applications. They are considered idempotent, meaning that making the same request multiple times will produce the same result. This is particularly useful for retrieving static data, such as images, product details, or user profiles.
Example:
GET /api/products/12345 HTTP/1.1
Host: www.example.com
2. POST Requests
Next up is the POST request, which is used to send data to a server to create or update a resource. Unlike GET requests, which are used for retrieving data, POST requests are used for submitting data. For example, when you fill out a form on a website and click "Submit," a POST request is made to the server with the form data.
POST requests are not idempotent, which means that sending the same POST request multiple times can create multiple records. This is why POST requests are often used for actions like creating a new user account, submitting a contact form, or making a payment.
Example:
POST /api/products HTTP/1.1
Host: www.example.com
Content-Type: application/json
{
"name": "New Product",
"price": 29.99,
"description": "A brand new product"
}
3. PUT Requests
PUT requests are similar to POST requests, but with a key difference: PUT requests are used to update an existing resource. When you send a PUT request, you’re telling the server to replace the existing resource with the data you’re providing.
PUT requests are also idempotent, meaning that making the same request multiple times will result in the same outcome. This makes PUT requests ideal for updating user profiles, modifying product details, or changing settings.
Example:
PUT /api/products/12345 HTTP/1.1
Host: www.example.com
Content-Type: application/json
{
"name": "Updated Product",
"price": 24.99,
"description": "An updated description for the product"
}
4. DELETE Requests
As the name implies, DELETE requests are used to delete a resource from the server. If you need to remove a record, such as a product from your inventory or a user from your database, a DELETE request is the way to go.
DELETE requests are typically idempotent. Sending a DELETE request for a non-existent resource won’t cause any harm, and the result will be the same whether the resource existed or not.
Example:
DELETE /api/products/12345 HTTP/1.1
Host: www.example.com
5. PATCH Requests
PATCH requests are used to make partial updates to an existing resource. Unlike PUT requests, which replace the entire resource, PATCH requests modify only the specified fields. This makes PATCH requests more efficient when you only need to update a small part of a resource.
PATCH requests are particularly useful for making minor updates, such as changing a user’s email address or updating the stock quantity of a product.
Example:
PATCH /api/products/12345 HTTP/1.1
Host: www.example.com
Content-Type: application/json
{
"price": 19.99
}
6. OPTIONS Requests
OPTIONS requests are a bit different from the others we've discussed so far. Instead of being used to retrieve or modify data, OPTIONS requests are used to find out what HTTP methods are supported by a server or endpoint. This is often used in Cross-Origin Resource Sharing (CORS) scenarios to check if a server allows specific HTTP methods from a particular domain.
When an OPTIONS request is made, the server responds with a list of allowed methods (e.g., GET, POST, PUT, DELETE). This helps clients understand what actions they can perform on the server.
Example:
OPTIONS /api/products/12345 HTTP/1.1
Host: www.example.com
7. HEAD Requests
A HEAD request is similar to a GET request, but with one key difference: it doesn’t return the body of the response, only the headers. This is useful when you need to check the status of a resource or inspect its metadata without downloading the entire resource.
HEAD requests are commonly used to check if a resource exists, determine its size, or see when it was last modified.
Example:
HEAD /api/products/12345 HTTP/1.1
Host: www.example.com
8. TRACE Requests
TRACE requests are used to echo back the received request, allowing the client to see what intermediate servers are receiving or modifying the request. This can be useful for debugging purposes, as it helps identify how a request is being altered as it passes through proxies or gateways.
However, TRACE requests are rarely used in modern development, as they can expose sensitive information and pose security risks.
Example:
TRACE /api/products/12345 HTTP/1.1
Host: www.example.com
9. CONNECT Requests
The CONNECT method is used to establish a network connection to a web server over HTTP. It’s primarily used for HTTPS connections, where the client asks the server to create a tunnel to a destination, allowing secure communication.
CONNECT requests are most commonly seen in proxy servers and are used to facilitate secure communications between the client and server.
Example:
CONNECT www.example.com:443 HTTP/1.1
Host: www.example.com
10. WebSocket Requests
WebSocket requests are a bit different from the typical HTTP methods we've discussed so far. WebSockets provide full-duplex communication channels over a single, long-lived connection. This allows for real-time data transfer between the client and server, which is especially useful in applications like chat apps, live sports updates, or online gaming.
While WebSocket requests are not part of the traditional HTTP methods, they play a crucial role in modern web development, enabling seamless, real-time interactions.
Example:
const socket = new WebSocket('ws://www.example.com/socket');
11. GraphQL Queries
GraphQL is a query language for APIs that allows clients to request specific data, reducing the amount of data transferred over the network. Unlike REST APIs, where multiple endpoints might be needed to fetch different pieces of data, GraphQL allows clients to query exactly what they need in a single request.
GraphQL queries can include multiple types of operations, such as queries (for fetching data), mutations (for modifying data), and subscriptions (for real-time updates).
Example:
query {
product(id: "12345") {
name
price
description
}
}
Send and Test GET Requests in Apidog
Apidog stands as a versatile and user-friendly API documentation and testing tool, designed to simplify the complexities of API interactions. Apidog excels in customizable, visually appealing API response documentation and user-friendly testing tools with assertions and testing branches.
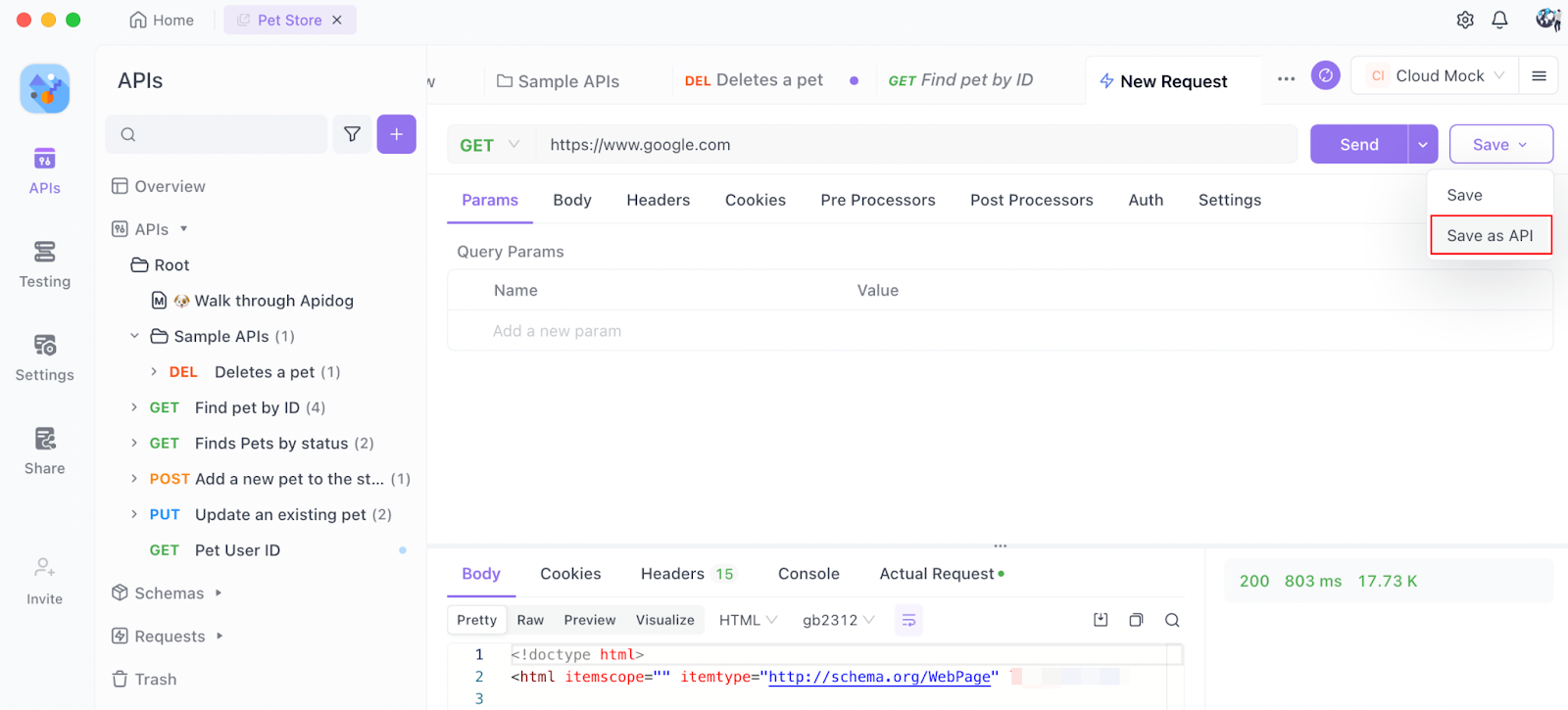
Specifically tailored for ease of use, Apidog provides a quick and visual means of sending and testing GET requests. Its user-friendly interface empowers developers to define intricate API endpoints with simplicity, set up diverse test scenarios effortlessly, and execute tests in real-time, all within an intuitive platform.
Developers can leverage Apidog's visual capabilities to streamline the process of testing GET requests, making it a recommended choice for those who value simplicity, efficiency, and an integrated approach to API testing.
Understanding API Responses
Now that we've covered the different types of API calls, it's important to also understand the nature of API responses. When an API call is made, the server sends back a response, which includes not only the requested data but also additional information, such as the status of the request.
HTTP Status Codes
HTTP status codes are a crucial part of API responses. They indicate whether the request was successful or if there was an error. Here are some of the most common status codes you might encounter:
- 200 OK: The request was successful, and the server returned the requested data.
- 201 Created: The request was successful, and a new resource was created.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: The client must authenticate itself to get the
requested response.
- 403 Forbidden: The client does not have permission to access the requested resource.
- 404 Not Found: The server could not find the requested resource.
- 500 Internal Server Error: The server encountered an error and could not complete the request.
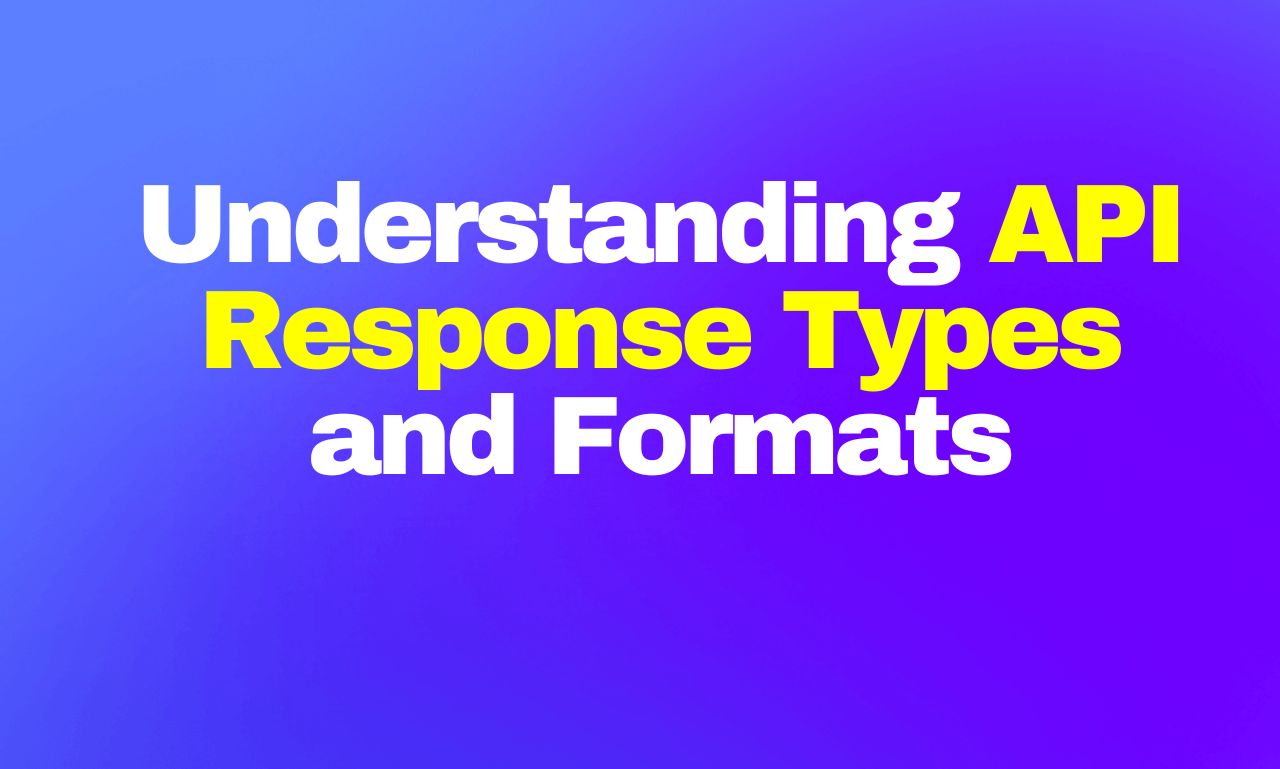
Data Formats
API responses often include data in specific formats. The most common formats are:
- JSON (JavaScript Object Notation): JSON is a lightweight data format that is easy to read and write. It is the most commonly used format for API responses.
- XML (eXtensible Markup Language): XML is another data format that is more verbose than JSON. It is still used in some APIs, particularly older ones.
- HTML: In some cases, API responses might include HTML, particularly if the API is used to render web pages.
Headers
API responses also include headers, which provide additional information about the response. Common headers include:
- Content-Type: Indicates the format of the data (e.g.,
application/json
). - Content-Length: Specifies the size of the response body.
- Authorization: Contains authentication information if the request required authentication.
When to Use Different Types of API Calls
Understanding the types of API calls is crucial, but knowing when to use each type is equally important. Let’s explore some scenarios where different types of API calls would be appropriate.
Retrieving Data: Use GET Requests
Whenever you need to retrieve data from a server, a GET request is your go-to option. Whether you’re fetching user details, product information, or a list of resources, GET requests are designed for retrieving data efficiently.
Submitting Data: Use POST Requests
When you need to submit data to a server, such as when creating a new user account or submitting a form, a POST request is the right choice. POST requests are used to send data to the server for processing and are ideal for creating new resources.
Updating Data: Use PUT or PATCH Requests
If you need to update an existing resource, you have two options: PUT or PATCH requests. Use PUT if you want to replace the entire resource, and use PATCH if you only need to update specific fields. Both methods are effective for updating data, but PATCH is often more efficient for minor changes.
Deleting Data: Use DELETE Requests
To remove a resource from the server, use a DELETE request. DELETE requests are straightforward and are the best option when you need to delete data, such as removing a product from a catalog or deleting a user account.
Checking Available Methods: Use OPTIONS Requests
If you need to find out what HTTP methods are supported by a server or endpoint, use an OPTIONS request. This is particularly useful in scenarios involving CORS or when working with APIs that have strict method restrictions.
Debugging: Use HEAD and TRACE Requests
For debugging purposes, HEAD and TRACE requests can be useful tools. HEAD requests allow you to inspect headers without downloading the full response, while TRACE requests let you see how your request is being processed by intermediate servers.
Establishing Secure Connections: Use CONNECT Requests
When working with secure connections, particularly through proxy servers, CONNECT requests are essential. They allow you to establish a secure tunnel to the destination server, facilitating encrypted communication.
Real-Time Interactions: Use WebSocket Requests
For real-time interactions, such as chat applications or live updates, WebSocket requests are the way to go. WebSockets enable full-duplex communication, allowing data to be sent and received simultaneously.
Flexible Data Retrieval: Use GraphQL Queries
If you need to retrieve specific data in a flexible and efficient manner, consider using GraphQL queries. GraphQL allows you to request exactly the data you need, reducing the amount of data transferred and improving performance.
Optimizing API Performance
Understanding the types of API calls is just one part of the equation. To build efficient and scalable applications, it's also important to optimize API performance. Here are some tips to help you get started:
1. Reduce API Calls
One of the simplest ways to optimize API performance is to reduce the number of API calls your application makes. This can be achieved by:
- Batching requests: Combine multiple requests into a single API call.
- Caching: Store frequently accessed data locally to avoid unnecessary API calls.
- Using GraphQL: With GraphQL, you can fetch all the required data in a single request, reducing the need for multiple API calls.
2. Optimize Data Payloads
Sending large amounts of data over the network can slow down your application. To optimize data payloads:
- Use pagination: Break down large data sets into smaller chunks that can be fetched in multiple requests.
- Compress data: Use data compression techniques to reduce the size of the data being sent.
- Filter data: Only request the data you need, rather than retrieving entire resources.
3. Leverage Asynchronous Requests
Asynchronous requests allow your application to continue processing other tasks while waiting for the API response. This can significantly improve the user experience by reducing perceived wait times.
4. Monitor and Log API Performance
Regularly monitoring and logging API performance is essential for identifying bottlenecks and areas for improvement. Tools like Apidog can help you track API performance metrics, such as response times and error rates, allowing you to make data-driven decisions.
5. Implement Rate Limiting
To protect your API from being overwhelmed by too many requests, consider implementing rate limiting. Rate limiting restricts the number of requests a client can make within a certain time frame, ensuring fair usage and preventing abuse.
Conclusion
APIs are the backbone of modern software development, enabling seamless communication between different applications. Understanding the various types of API calls is crucial for building efficient, scalable, and secure applications. Whether you're retrieving data with GET requests, submitting data with POST requests, or optimizing your API performance, knowing when and how to use each type of API call is essential.
And remember, if you’re looking for a tool to simplify your API development process, download Apidog for free. Apidog offers a comprehensive suite of tools for API testing, management, and documentation, making it easier than ever to work with APIs.