How to Send Query Parameters in POST Request
Learn the simple and effective way to send query parameters in a POST request with our step-by-step guide. Master the art of sending data efficiently!
When building APIs and web applications, you'll often need to send data from the client to the server. The two main methods for transmitting this data are through request bodies or query parameters.
But can you use query parameters with POST requests, or are they limited to GET requests? In this post, we'll cover the details of how to send query parameters with POST requests in JavaScript, REST Assured, and provide a powerful API tool to send Query parameters POST request easily .
Query Parameters
First, let's define what query parameters are. Query parameters are key-value pairs that are appended to the URL to pass data to the server. For example:
https://example.com/api?name=john&age=30
Here, name
and age
are query parameters. Their values, john
and 30
, are passed to the server through the URL.
Query parameters are very commonly used with GET requests. Appending them to the URL is an easy way to filter data or pass options to GET endpoints. However, query parameters can also be used with POST, PUT, DELETE, and other HTTP verbs.
What is a POST Request API?
A POST request API, or simply a POST API, refers to an Application Programming Interface that utilizes the HTTP POST method for communication. In web development, APIs often use different HTTP methods to perform various actions. The POST method is commonly employed when a client wants to send data to a server to create a new resource or perform a specific operation.
In the context of a POST request API, clients send data in the body of the request, allowing them to submit information, such as form data or JSON payloads, to the server for processing. This method is crucial for actions that involve data submission and creation, making it a fundamental aspect of many web-based applications and services.
Can POST Requests Have Query Parameters?
Yes, absolutely. While less common than with GET requests, query parameters can be appended to POST request URLs just like any other request type.
For example, you could make a POST request like:
POST https://example.com/api/createUser?notify=true
The notify=true
query parameter here could tell the server to send a notification after creating the user.
The key thing to understand is that query parameters are separate from the request body. The body holds the main data you want to send, while the query parameters hold metadata or options about how to process that data.
So in summary - yes, POST requests can definitely have query parameters!
Sending Query Parameters in POST Requests with JavaScript
Let's look at some examples of how to send query parameters with POST requests in JavaScript.
With the standard XMLHttpRequest
object, you can append query parameters to the URL like:
js
Copy code
const xhr = new XMLHttpRequest();
xhr.open('POST', '/api/createUser?notify=true');
xhr.send(JSON.stringify({
name: 'John',
age: 30
}));
The query parameter is added directly to the URL passed to open()
.
If using the Fetch API
, you can construct the URL with query parameters like:
js
Copy code
const url = '/api/createUser?notify=true';
fetch(url, {
method: 'POST',
body: JSON.stringify({
name: 'John',
age: 30
})
});
Or with Axios, append the query params to the URL:
js
Copy code
axios.post('/api/createUser?notify=true', {
name: 'John',
age: 30
});
The process is the same no matter which request library you use - just construct the URL with the query parameters included.
Sending Query Parameters in REST Assured
REST Assured is a popular Java library for testing REST APIs. You can also send query parameters with POST requests in REST Assured.
For example:
java
Copy code
given()
.queryParam("notify", "true")
.when()
.post("/api/createUser")
.then()
.assertThat()
.statusCode(201);
This will append a notify=true
query parameter to the POST request.
Or you can construct the full URL with params:
java
Copy code
.post("https://example.com/api/createUser?notify=true")
So REST Assured makes it very easy to add query parameters to any type of request.
What is Apidog?
Apidog is a powerful tool for documenting APIs. It provides a straightforward way to create comprehensive API documentation that includes details about request parameters, endpoints, response structures, and more.
In addition to request parameters, Apidog enables you to document authentication methods and headers required for accessing your API. This ensures that developers have all the necessary information to make successful requests.
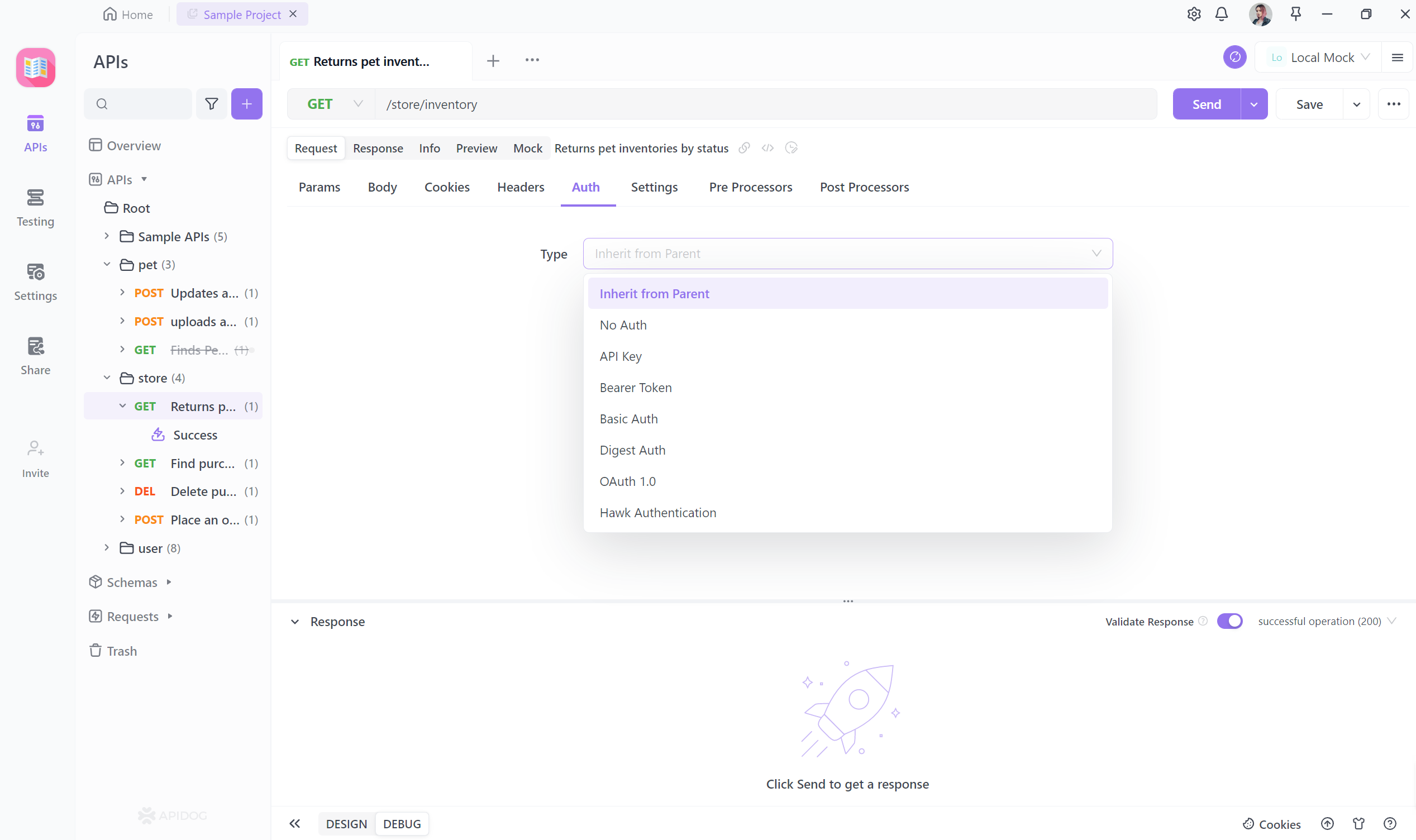
Best Practices for Query Parameters
When should you use query parameters versus a request body? Here are some best practices:
- Use query parameters for non-sensitive metadata like filters, options, etc.
- Send main request data and sensitive information in the request body.
- Don't overload the URL with too many query parameters as it may exceed max length.
- For GET requests, use query parameters instead of request bodies.
- Consider if the data format works - URL encoding for query params vs JSON for request body.
Following these guidelines will ensure you use the right approach for sending data to your API endpoints.
Conclusion
To summarize this article what we learned:
- Query parameters can be used with any HTTP verb like POST, not just GET. They are added to the request URL.
- In JavaScript, you can append query params to the URL when calling
open()
,fetch()
, oraxios.post()
. - REST Assured and other libraries make it easy to add query parameters to POST and other requests.
- Best practices are to use query parameters for non-sensitive metadata and request bodies for main data.
So in conclusion - yes, POST requests absolutely can have query parameters! Just construct the URL appropriately whenever you need to pass extra options or filters to your API endpoints.