How to Call REST API from Node.JS
In this guide, we'll explore the different methods for calling REST APIs from Node.js applications. We'll go over core concepts, show example code snippets, and provide best practices for working with REST APIs in your Node.js projects.
REST (Representational State Transfer) APIs have become the standard for API development and integration. Their simple, lightweight design makes REST APIs easy to build, scale, and consume from any programming language.
In this guide, we'll explore the different methods for calling REST APIs from Node.js applications. We'll go over core concepts, show example code snippets, and provide best practices for working with REST APIs in your Node.js projects.
What are REST APIs?
First, let's briefly recap on some key aspects of REST APIs:
- REST APIs access and manipulate data using standard HTTP methods like GET, POST, PUT, and DELETE.
- Resources are accessed via endpoints - URLs that expose data entities.
- REST uses status codes like 200, 401, 404 etc to indicate API response status.
- JSON is the standard format for sending and receiving data.
- REST is stateless, so requests must contain all necessary information.
- Authentication is via tokens, API keys, etc.
These principles make REST APIs simple, lightweight, and easy to consume from any language like Node.js.
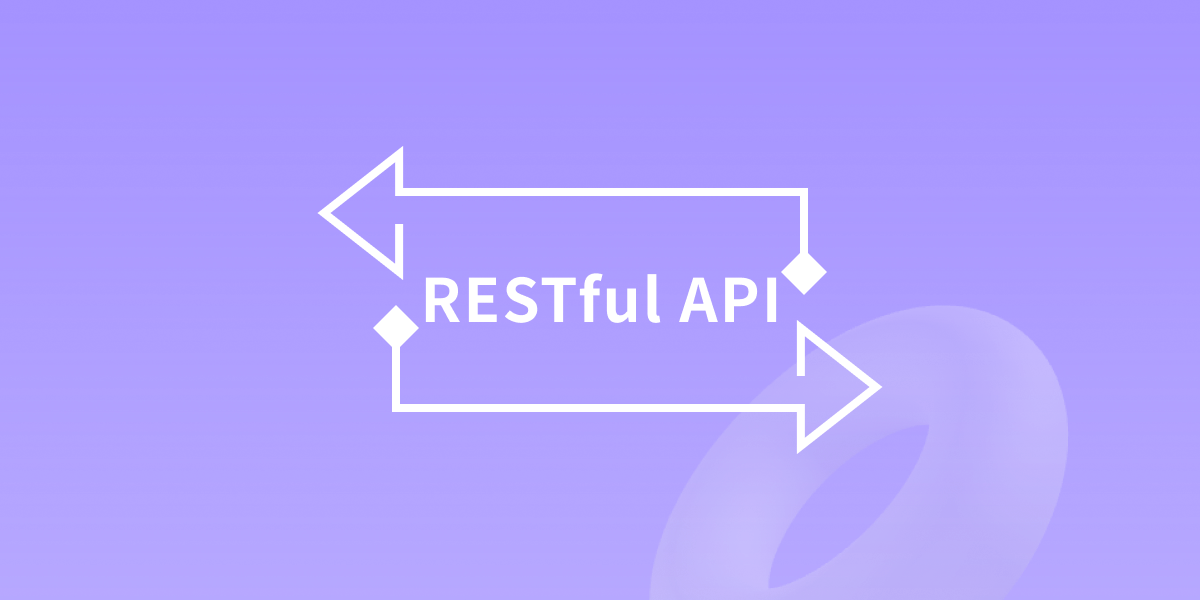
Why Use Node.js for Calling REST APIs?
Node.js is great for working with REST APIs for several reasons:
- Asynchronous, event-driven - Perfect for I/O bound work like API calls.
- Fast and scalable - Handles thousands of concurrent connections.
- Ecosystem of packages - Simplifies making HTTP requests and working with JSON.
- Popular for web apps - Integrates well with frontend JavaScript code.
- Excellent for real-time apps - Efficiently handles real-time data via REST.
So in short, Node.js is well-suited for accessing REST APIs both on server and client-side.
Using Built-in HTTP Module to Call API
Node.js provides the HTTP core module for making HTTP requests, which you can use to make requests to a server. To quickly demonstrate, here's an example code for a GET request using the HTTP module. This script is designed for Node.js to execute an API call to JSONPlaceholder.
The HTTP module allows making all kinds of requests including POST, PUT etc. However, it's quite low-level and requires manually handling callbacks, JSON parsing, authentication etc.
Here are some detailed steps for using Node.js to call REST APIs:
Step 1: Performing API Calls in Node.js with the HTTP Module
In the realm of Node.js, the absence of XHR objects or the XHR API is noticeable. Instead, Node.js equips us with the HTTP module, enabling us to carry out API calls and interact with servers through HTTP.
Step 1.1: Creating a Node.js Script for an API Call
To embark on your journey of making API calls in Node.js, let's craft a straightforward GET request to the JSONPlaceholder API using the HTTP module. Here are the steps:
Step 1.1.1: Crafting a Node.js Script
Start by crafting a file named "api-call.js," and inject the following code snippet into it. This script will be the linchpin for our API calls in Node.js.
javascriptCopy code
const http = require('http');
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/posts',
method: 'GET',
headers: {
'Content-Type': 'application/json',
},
};
const getPosts = () => {
let data = '';
const request = http.request(options, (response) => {
response.setEncoding('utf8');
response.on('data', (chunk) => {
data += chunk;
});
response.on('end', () => {
console.log(data);
});
});
request.on('error', (error) => {
console.error(error);
});
request.end();
};
getPosts();
Step 1.1.2: Executing the Script
Assuming that you've already installed Node.js, execute the script with the following command: node api-call
. This will trigger the execution of the JavaScript file using Node.js and make an "API call in Node.js" to JSONPlaceholder, ultimately fetching an array of objects.
Step 2: Adapting to Different Scenarios
As you delve into the world of "API calls in Node.js," you'll notice that configuring these calls in Node.js demands more setup compared to the simplicity of browser-based XHR objects. Thankfully, numerous external libraries exist to streamline these tasks. One such library is Axios, which we'll explore in our upcoming article.
In the above code, we retrieve all posts at the "/posts" resource from the JSONPlaceholder API. Should you desire to fetch a single post, a URL such as "https://jsonplaceholder.typicode.com/posts/1" will suffice. The key distinction is the inclusion of "/1" at the end of the URL.
Step 3: Modifying the Code
Let's make some alterations to our code to fetch only a single item:
javascriptCopy code
const http = require('http');
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/posts/1',
method: 'GET',
headers: {
'Content-Type': 'application/json',
},
};
const getPosts = () => {
let data = '';
const request = http.request(options, (response) => {
response.setEncoding('utf8');
response.on('data', (chunk) => {
data += chunk;
});
response on('end', () => {
console.log(data);
});
});
request.on('error', (error) => {
console.error(error);
});
request.end();
};
getPosts();
Now, you will receive a single object, and it might feature some Latin text, commonly employed as placeholder text in the development process.
Step 4: An Alternative Approach
In the example above, we used http.request
. However, http.get
is a simplified method for making requests with the GET HTTP method:
javascriptCopy code
const http = require('http');
const getPosts = () => {
let data = '';
const request = http.get('http://jsonplaceholder.typicode.com/posts/1', (response) => {
response.setEncoding('utf8');
response.on('data', (chunk) => {
data += chunk;
});
response.on('end', () => {
console.log(data);
});
});
request.on('error', (error) => {
console.error(error);
});
request.end();
};
getPosts();
Step 5: Making POST Requests
Now, let's delve into creating a POST request using the HTTP module. The crucial difference here is the need to structure a request body and transmit it to the request object:
const http = require('http');
// Craft the request bodyconst postData = JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
});
const options = {
hostname: 'jsonplaceholder.typicode.com',
path: '/posts',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': Buffer.byteLength(postData),
},
};
const makePost = () => {
let data = '';
const request = http.request(options, (response) => {
response.setEncoding('utf8');
response.on('data', (chunk) => {
data += chunk;
});
response.on('end', () => {
console.log(data);
});
});
request.on('error', (error) => {
console.error(error);
});
// Inject data into the request body
request.write(postData);
request.end();
};
makePost();
So for most API tasks, developers use higher level libraries as covered next.
Using Apidog to Make an API Call
Apidog simplifies REST API development, streamlining testing, documentation, security, and performance. This article demonstrates how to use Apidog and its key features. Sign up for free to boost your API development efficiency.
Step 1: Create a New Project in Apidog
- Open Apidog on your computer.
- Select "New Project" or "Starting a New API Project."
- Click "Create" to establish your new project.
You've created a project to start your API development journey.
Step 2: Create a Basic Node.js API
Now, it's time to handle requests and responses with Node.js. In this example, we'll create a simple API that provides a greeting message when accessed at "/greet."
Step 3: Set Up Your Node.js Project
- Create a new project folder using the "mkdir" command. Name it "my-api":
mkdir my-api
- Navigate to the project folder:
cd my-api
Step 4: Write Your Node.js API Code
- Create a new Node.js file named "app.js" inside the "my-api" folder.
- Open "app.js" and add the following code to create a basic Node.js API:
const express = require('express');
const app = express();
const port = 3000;
function getGreeting(req, res) {
const greeting = 'Hello, world!';
res.send(greeting);
}
app.get('/greet', getGreeting);
app.listen(port, () => {
console.log(`API is running on port ${port}`);
});
Step 5: Install Express.js
Ensure you are in the "my-api" folder and run the following command to install Express.js:
npm install express
Step 6: Run Your Node.js API
Stay within your project folder and run your Node.js file with this command:
node app.js
Step 7: Access Your API
Open a web browser and access your API by entering this URL:
http://localhost:3000/greet
You should see "Hello, world!" displayed in your web browser, indicating that your simple Node.js API is running.
With these seven steps, you've successfully created a basic API using Apidog and Node.js. This API provides a greeting message when accessed at "/greet."
Fetch
The fetch API provides a modern way to make network requests. It has native support in most browsers but a Node.js polyfill is available:
const fetch = require('node-fetch');
const response = await fetch('https://api.example.com/data');
const json = await response.json();
console.log(json);
Fetch makes working with APIs simpler through:
- Promise-based API frees you from callbacks.
- Easier handling of HTTP errors via status codes.
- Automatic parsing of response based on content-type.
- Aborting requests using AbortController.
- Familiar interface for web developers.
So if you prefer promise-based code, fetch is a great option. The API will also feel familiar if you've used fetch in the browser.