SOAP vs REST: What is the Key Differences
SOAP and Rest API have their pros and cons, and knowing the differences between them can help developers and architects determine which architecture to use when building their web-based APIs.
SOAP (Simple Object Access Protocol) and REST (Representational State Transfer) have become two of the most popular protocols for developing web-based APIs.
In this article, we will briefly explore the differences between SOAP and REST APIs, with the aim of helping readers better understand the advantages, drawbacks, and use cases of each API architecture.
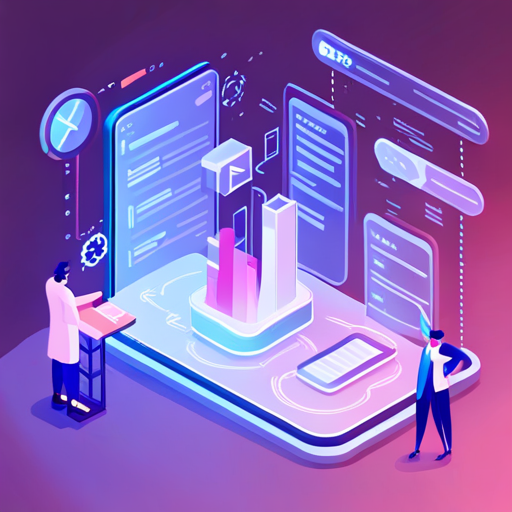
What is REST API?
REST stands as an architectural style, distinct from SOAP's protocol nature. The fundamental contrast lies in the level of coupling between client and server implementations. Unlike SOAP, where clients are tightly bound to servers, a REST client operates like a browser, with minimal prior knowledge.
Key Points of REST API:
- Protocol Independence: REST is not limited to HTTP; it embraces flexibility with any protocol featuring a standardized URI scheme.
- Not a CRUD Mapping: REST transcends a simple CRUD-to-HTTP method mapping, demanding a nuanced approach for effective implementation.
- Standardization in Usage: REST adheres to standards, with security and authentication following HTTP standards when implemented over HTTP.
- HATEOAS is Essential: REST is incomplete without Hypermedia as the Engine of Application State (HATEOAS). Clients should only be aware of the entry point URI, and resources must return links for clients to follow.
- Web Architecture Parallels: REST mirrors the web's architectural style, guiding clients through links in resource representations, similar to a user navigating a website.
When to Use REST API?
REST API is best suited for scenarios where simplicity, scalability, and flexibility are crucial. It relies on standard HTTP methods (GET, POST, PUT, DELETE) and is designed to work with stateless communication. RESTful APIs are commonly used in web and mobile applications, especially in situations where lightweight communication is preferred.
REST is well-suited for scenarios where resources can be represented as URLs and manipulated using standard HTTP methods. It is widely adopted for building web services that need to support multiple clients, making it a popular choice for public APIs and web applications that prioritize ease of use.
REST API Example
Here's a simplified example of a REST API using Flask with just one endpoint:
from flask import Flask, jsonify
app = Flask(__name__)
# Sample data
tasks = [
{'id': 1, 'title': 'Learn Python', 'done': False},
{'id': 2, 'title': 'Build RESTful API', 'done': False}
]
# Endpoint to get all tasks
@app.route('/tasks', methods=['GET'])
def get_tasks():
return jsonify({'tasks': tasks})
# Run the Flask app
if __name__ == '__main__':
app.run(debug=True)
In this example, there's only one endpoint:
GET /tasks
: Returns a list of all tasks.
This is a minimal example to get you started. Save the code in a file (e.g., app.py
) and run it. You can access the API at http://localhost:5000/tasks
in your web browser or a tool like curl or Apidog.
What is SOAP API?
SOAP API (Simple Object Access Protocol) is a protocol that facilitates communication between different software systems over the internet.
Key Points of SOAP API:
- Structure: Unlike REST, SOAP is not just an architectural style but a protocol. It defines a strict set of rules for structuring messages using XML, ensuring a standardized format for data exchange.
- Coupling Nature: SOAP APIs involve a high degree of coupling between the client and server implementations. Clients operate as custom desktop applications tightly bound to servers, necessitating constant updates with any changes.
- Rigid Contract: A SOAP client follows a rigid contract with the server, and any alteration on either side may result in potential breakdowns. This rigid structure, while ensuring adherence to standards, can be less flexible in accommodating changes.
- Documentation Emphasis: SOAP APIs typically require comprehensive documentation detailing every aspect the client needs to know for interaction. Prior knowledge is essential for clients to initiate any communication.
When to Use SOAP API?
SOAP API is more appropriate for scenarios where a strict and standardized communication protocol is required, often in enterprise-level applications. SOAP is a protocol that uses XML as its message format and operates over various transport protocols, including HTTP and SMTP.
SOAP APIs are known for their strict standards and strong typing, making them suitable for situations where a high level of security, reliability, and transactional support is needed. Enterprise-level integrations, such as those in financial systems or healthcare applications, often leverage SOAP APIs due to their ability to handle complex operations and ensure data integrity.
A SOAP Example
If you're looking for a pure XML-based example, here's a simple one using the requests
library to send a SOAP request:
import requests
# Define the SOAP request XML
soap_request = """
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:web="http://www.dneonline.com/">
<soapenv:Header/>
<soapenv:Body>
<web:Add>
<web:intA>2</web:intA>
<web:intB>3</web:intB>
</web:Add>
</soapenv:Body>
</soapenv:Envelope>
"""
# Set the SOAP endpoint
url = 'http://www.dneonline.com/calculator.asmx'
# Set the SOAPAction header
headers = {'Content-Type': 'text/xml', 'SOAPAction': 'http://www.dneonline.com/Add'}
# Make the SOAP request
response = requests.post(url, data=soap_request, headers=headers)
# Print the response
print(response.content)
This script manually constructs a SOAP request in XML format and sends it to the specified SOAP endpoint using the requests
library. The response from the server is then printed.
SOAP vs REST API: Get the Key Differences
Let's compare the implementations of the popular REST and SOAP styles:
Message Format
- In SOAP, requests and responses must be in SOAP XML format.
- In REST, there is no fixed format. We can exchange messages based on XML, JSON, or any other format. JSON is the most popular format.
Service Definition
- SOAP uses WSDL (Web Service Description Language), which is an XML-based language used to describe and access web services.
- REST does not have a standard service definition language. Although WADL was one of the earliest proposed standards, it is not very popular. Swagger or Open API are currently more widely used and accepted.
Transport
SOAP does not impose any restrictions on the type of transport protocol used. We can use HTTP web protocol or MQ, while REST is best used with the HTTP transport protocol.
Usability RESTful web services are usually easier to implement than SOAP-based web services.
- REST usually uses JSON, which is easier to parse and process. In addition, REST does not require a service definition to provide web services.
- In addition to requiring WSDL to define services, SOAP also requires effort to process and parse SOAP-XML messages.
The Pros and Cons of SOAP vs REST API
SOAP and REST are two different styles of web services that have their own advantages and disadvantages.
SOAP API Pros: | SOAP API Cons: |
---|---|
Standardized protocol and formal contract | Higher overhead due to its XML-based message format |
Built-in error handling and security features | Slower performance compared to REST API |
Support for ACID transactions | More complex and harder to implement |
Wide support for different languages/platforms | Limited support for mobile devices and web browsers |
REST API Pros: | REST API Cons: |
---|---|
Lightweight and faster performance | Lack of standardized protocol and formal contract |
Easier to implement and maintain | No built-in error handling and security features |
Better support for mobile devices/web browsers | Limited support for ACID transactions |
Scalable and flexible architecture | Limited support for different languages/platforms |
Apidog: The Powerful API Tool
Apidog is an API development and testing tool that provides a range of functionalities for testing RESTful APIs. Like Postman, Apidog offers a user-friendly interface that allows users to create, test, and document APIs using various features such as automated testing, test suites, and API documentation.
Apidog allows users to easily create and send HTTP requests to APIs, view response data, and debug potential issues. It also provides the ability to organize requests into collections, folders, and subfolders, making it easy to manage and maintain API requests. Additionally, Apidog supports collaboration tools and various integration options with other development platforms.
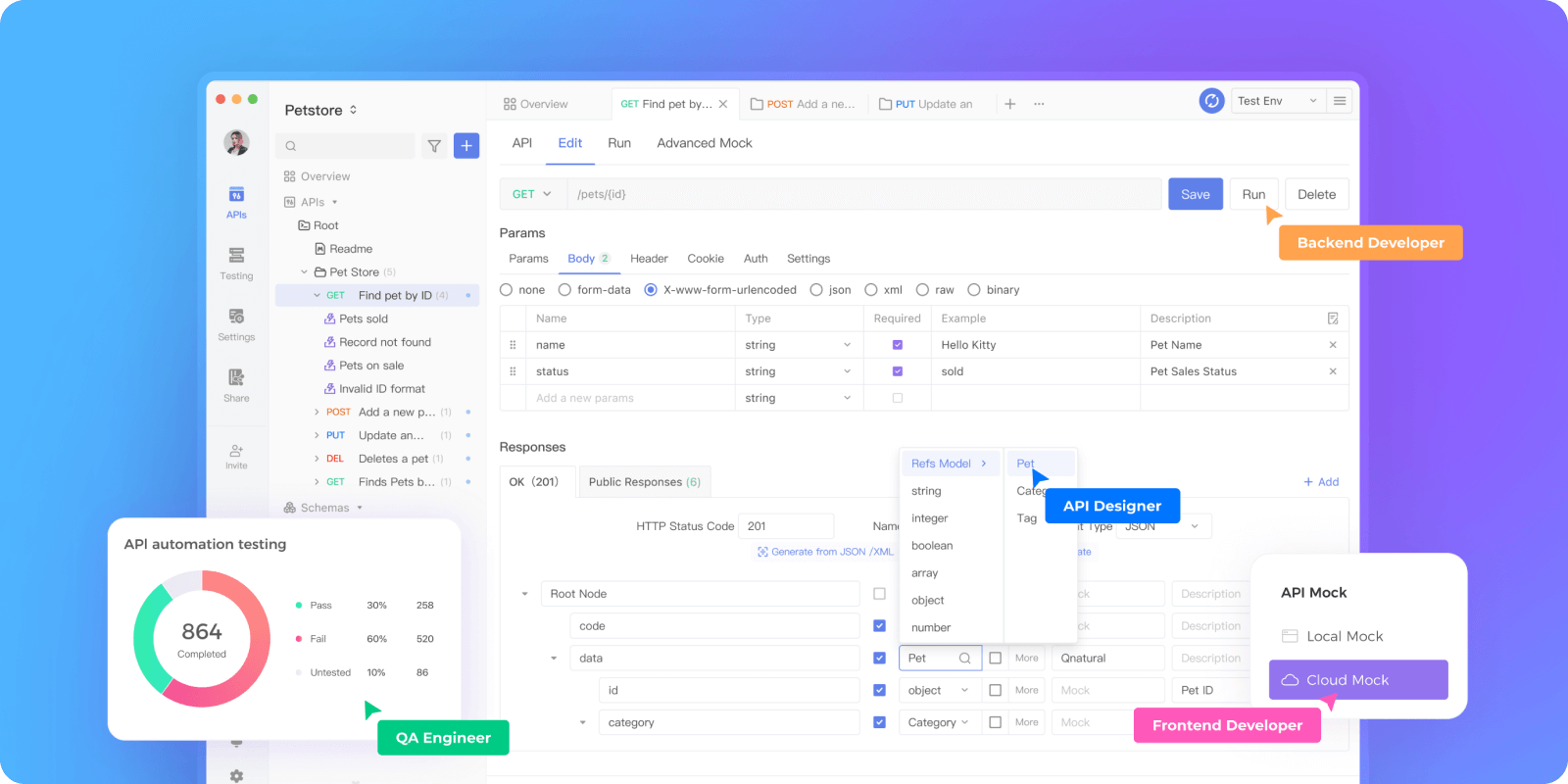
In addition to testing APIs, Apidog offers capabilities such as automated testing, performance monitoring, and scripting. It also supports advanced features such as error validation, authentication, and parameterization, making it a versatile tool for developers and testers.
In general, SOAP APIs are better suited for applications that require a high degree of security, reliability, and interoperability, while REST APIs are better suited for applications that require fast and efficient data transfer, scalability, and flexibility. However, the choice between SOAP and REST APIs ultimately depends on the specific needs of the application and the resources available to support it.