HTTP Request Parameters in REST API
Request parameters allow you to pass data to an API endpoint when making a request. They are an important part of designing and using REST APIs.
Request parameters allow you to pass data to an API endpoint when making a request. They are an important part of designing and using REST APIs. In this guide, we'll cover everything you need to know about request parameters, including how to use them with different HTTP methods like GET, POST, PUT and DELETE in Apidog.
What Are Request Parameters in HTTP Request?
Request parameters, also known as query parameters or URL parameters, are key-value pairs that are appended to the URL when making an HTTP request. Parameters are visible in the URL and allow the client to pass data to the API in a simple and standardized way.
Some examples of request parameters:
/users?id=1234
/posts?category=tech&sort=asc
/search?q=hello+world
The parameters come after the ?
in the URL, separated by an &
. The server can then access these parameters to handle the request accordingly.
Why Use Request Parameters?
Here are some of the main benefits of using REST API parameters:
- Simplicity - Appending key-value pairs to the URL is an easy way to pass data. The client doesn't need to construct a complex request body.
- Flexibility - Parameters can be combined in different ways to support various use cases. New parameters can easily be introduced without breaking existing clients.
- Caching - URLs with different parameters can be cached separately by browsers and CDNs.
- Bookmarks - URLs with parameters can be bookmarked for later use.
- Logging - Parameter values appear directly in server access logs for tracking and analytics.
- Encoding - URLs easily support encoding of parameter values, such as spaces to %20.
Overall, request parameters provide a simple, flexible way to pass data to APIs that fit nicely into the REST architectural style.
4 Types of API Parameters
There are two main types of request params:
Query Parameters
Query parameters are the most common type. They are appended to the URL path after a ?
character:
/users?page=1&per_page=20
Query params work well for filtering, sorting, pagination, and simple lookups.
Path Parameters
Path parameters are Baked into the URL path itself:
/users/{userId}
This allows identifiers and fixed attributes to appear directly in the resource path for more RESTful, self-documenting APIs. Path params are also required for many URI patterns like collection/element.
Most modern API frameworks like Express support both query and path parameters.
Header Parameters
Header parameters are components of an HTTP request that contain metadata about the request or the client making the request. They typically include information like the content type of the request body, authentication credentials, cache controls, and more. These parameters are transmitted in the request header, separate from the request body.
For example:
Content-Type: application/json
Authorization: Bearer <token>
Request Body Parameters
Request body parameters are the data sent along with the request in the body of the HTTP message. They carry the actual payload or data that the client wants to send to the server. These parameters are commonly used in POST, PUT, and PATCH requests to transmit data like JSON objects, form data, or file uploads.
For example:
{
"username": "example",
"password": "password123"
}
Using Parameters with HTTP Methods
Request parameters can be used with any HTTP method like GET, POST, PUT and DELETE. However, there are best practices around which to use in different situations.
GET Request Parameters
GET requests should use query parameters exclusively. Path parameters can also work for simple lookups by ID.
GET requests with query params are safe, cacheable, and easy to construct. This makes them ideal for:
- Filtering results
- Pagination
- Sorting
- Partial response
For example:
GET /users?status=active&sort=-createdAt
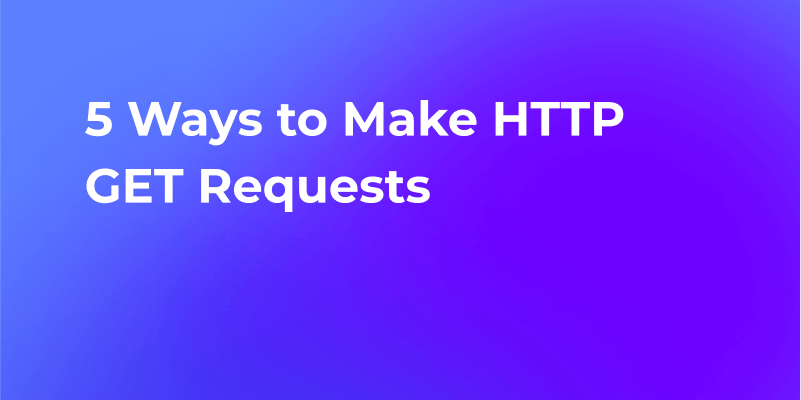
POST Request Parameters
POST requests support both query and path parameters.
However, it's best practice to avoid query parameters for POSTs in REST APIs. This keeps the request data together in the body and avoids potential issues with parameter encoding and caching.
Instead, use path parameters to identify resources and put the remaining data in the request body as JSON:
POST /users/{userId}/comments
{
"text": "Hello World!"
}
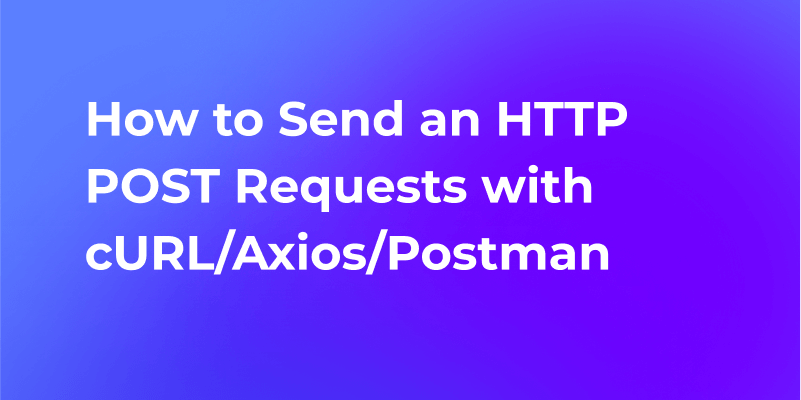
PUT Request Parameters
Like POST, PUT requests should avoid query parameters and use path parameters to identify the resource being updated. The update data itself should go in the request body:
PUT /users/{userId}
{
"firstName": "Jane"
}
PATCH Request Parameters
PATCH requests are used in HTTP for making partial updates to existing resources. They allow clients to specify only the data that needs to be changed, rather than replacing the entire resource. This helps optimize network traffic and improve efficiency.
Example:
PATCH /api/users/123
{
"age": 40,
"city": "New York"
}
In this example, the client is sending a PATCH request to update the user with ID 123. Only the "age" and "city" fields are included in the request body, indicating that only these fields should be modified. The "age" field is updated to 40, and the "city" field is set to "New York".
HTTP Request Parameters in Apidog
Apidog is an API documentation tool that allows you to generate documentation for your APIs based on request parameters and other relevant information. To introduce Apidog using request parameters, you can follow these ways:
Request Parameter Documentation: In Apidog, you can document request parameters by specifying their names, types, descriptions, and any validation rules or constraints. This ensures that developers using your API understand what data is expected in each request.
Endpoint Description: You can associate request parameters with specific API endpoints. For each endpoint, you can describe its purpose and functionality, making it clear how the request parameters fit into the overall API workflow.
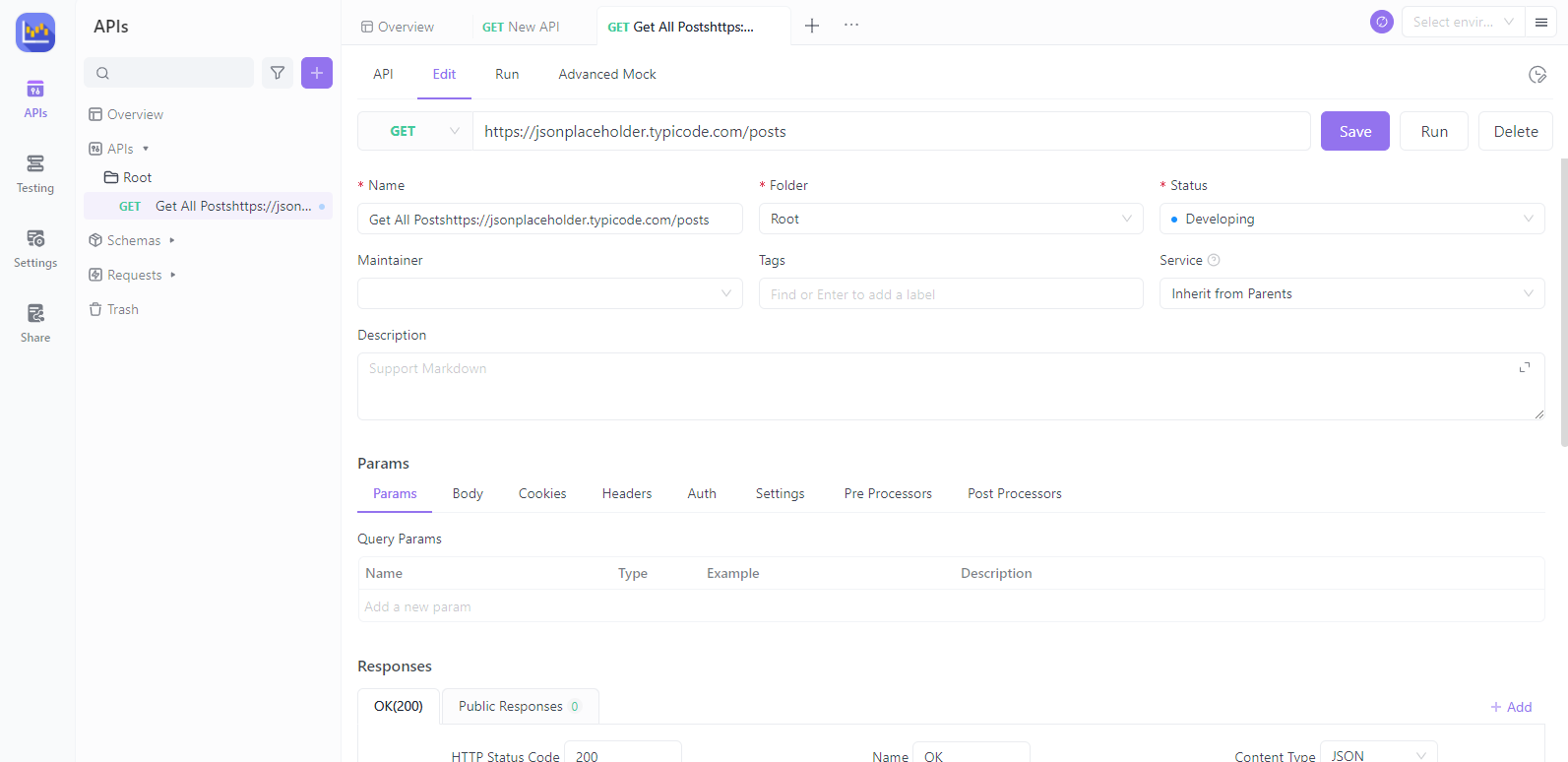
Example Requests: Apidog allows you to include sample request examples that demonstrate how to send parameters in a request. These examples can include the HTTP method (e.g., GET, POST), endpoint URL, and the request body or query parameters.
Accessing Parameters in Code
On the server side, access and validate parameter values from the request before using them.
In Express, parameters are available in the req.params
and req.query
objects:
app.get('/users', (req, res) => {
const sort = req.query.sort;
const limit = req.query.limit;
// ...
})
Frameworks like Express will decode parameter values from the URL encoding for you.
On the client side, there are libraries like qs that help generate and parse parameter strings.
Parameters Validation
It's important to validate parameters coming from clients to avoid security issues and bad data:
- Type Check - Make sure the parameter is the expected type like number, string, etc.
- Value Check - Validate against an allowable set of values, range, regex pattern, etc.
- Required - Ensure required parameters are present.
- Sanitize - Trim, escape, encode, etc. to prevent XSS and other injection attacks.
Doing proper parameter validation is critical for secure, production-ready APIs. Frameworks like Express Validator make validation much easier.
In Summary
Request parameters allow passing simple data to APIs in a standardized way that fits REST principles.
Follow these best practices when using request parameters:
- Stick to query params for GET, path params for POST/PUT/DELETE.
- Avoid query params in request bodies - put data in body instead.
- Validate, sanitize, and document parameters properly.
- Keep parameters consistent across APIs.