API Protocol Types | The 8 Most Commonly Used API Protocols
Today, we'll introduce you to 8 commonly used API protocols: REST, GraphQL, SOAP/Web Service, WebSocket, Socket, SSE, gRPC, and MsgPack. Each protocol has its unique traits and applications, making it essential to understand their differences and capabilities.
In the ever-evolving landscape of software development, Application Programming Interfaces (APIs) play a pivotal role in enabling seamless communication between diverse applications.
Based on data from the "2023 Global API Status Report," we're diving deep into the world of API protocols to uncover the top choices of developers worldwide. What sets these protocols apart? Why do we have so many of them? And how exactly do these protocols work?
This comprehensive article takes you on a journey through 8 of the most commonly used API protocols and interface specifications. We'll explore their unique characteristics, and use cases, and provide concrete examples to illustrate how they empower your digital initiatives:
1. REST (Representational State Transfer)
REST, which stands for Representational State Transfer, is an architectural style for designing networked applications. It is not a protocol but a set of constraints and principles that define how web services should be structured and interact with each other. REST is often used in the context of building APIs (Application Programming Interfaces) for web-based applications.
HTTP Methods: Clients can use different HTTP methods to interact with the resource. For example:
GET /books
: Retrieve a list of all books.GET /books/{id}
: Retrieve details of a specific book by its ID.POST /books
: Create a new book.PUT /books/{id}
: Update an existing book by its ID.DELETE /books/{id}
: Delete a book by its ID.
REST 's Example:
HTTP is superbly suited for applications following a request-response paradigm. For instance, if you want to retrieve information about a specific user in a RESTful API for a social media platform, you'd make a GET request to a URL like this:
GET https://api.example.com/users/123
2. GraphQL
GraphQL is a query language and runtime for APIs (Application Programming Interfaces) that allows clients to request only the data they need from a server, rather than receiving a fixed set of data. It was developed by Facebook and released as an open-source project. GraphQL provides a more efficient and flexible way to interact with APIs compared to traditional RESTful APIs.
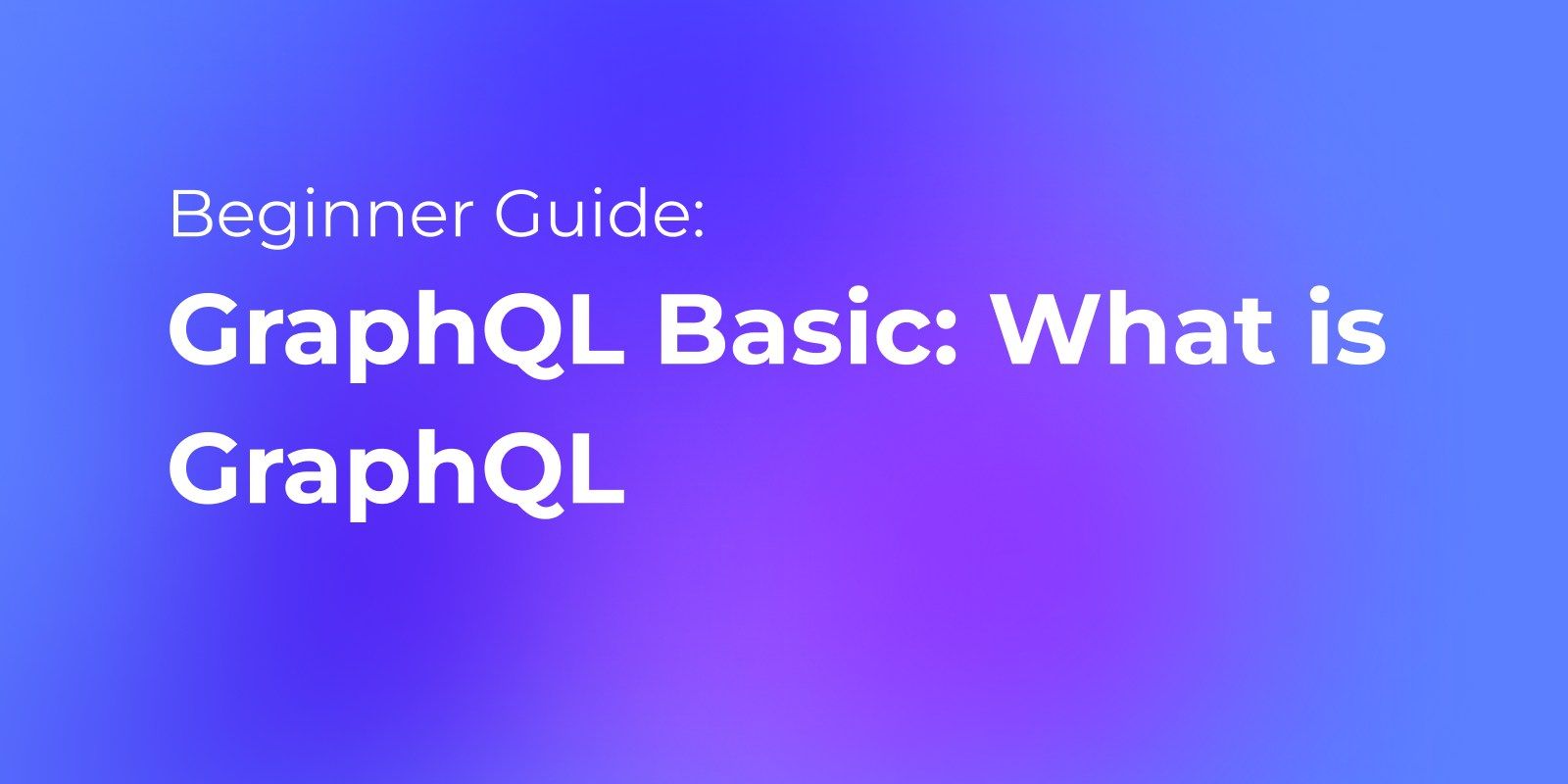
GraphQL's Example:
Here's a GraphQL schema for our post example:
type Post {
id: ID!
title: String!
body: String!
author: Author!
}
type Author {
id: ID!
name: String!
}
type Query {
posts: [Post!]!
authors: [Author!]!
}
With this schema in place, a client can make a GraphQL query to request specific data:
{
posts {
title
author {
name
}}}
In response to this query, the server will return data in the exact shape that the client requested, like:
{
"data": {
"posts": [
{
"title": "Introduction to GraphQL",
"author": {
"name": "John Doe"
}
},
{
"title": "GraphQL Best Practices",
"author": {
"name": "Jane Smith"
}
}
]
}
}
3. SOAP (Simple Object Access Protocol)/Web Service
SOAP stands for Simple Object Access Protocol. It is a protocol used for exchanging structured information in the implementation of web services over various communication protocols, typically HTTP or SMTP. SOAP is a messaging protocol, which means it defines a set of rules for structuring messages that can be sent between systems.
SOAP 's Example:
In SOAP, you define a message structure using XML. Here's a simplified example:
<SOAP-ENV:Envelope xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/" xmlns:example="http://example.com">
<SOAP-ENV:Header/>
<SOAP-ENV:Body>
<example:GetUser>
<example:UserID>123</example:UserID>
</example:GetUser>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
4. WebSocket
WebSocket is a communication protocol that provides full-duplex, bidirectional communication over a single, long-lived connection between a client and a server. Unlike traditional HTTP, which follows a request-response model, WebSocket allows data to be sent and received asynchronously without the overhead of repeatedly establishing new connections.
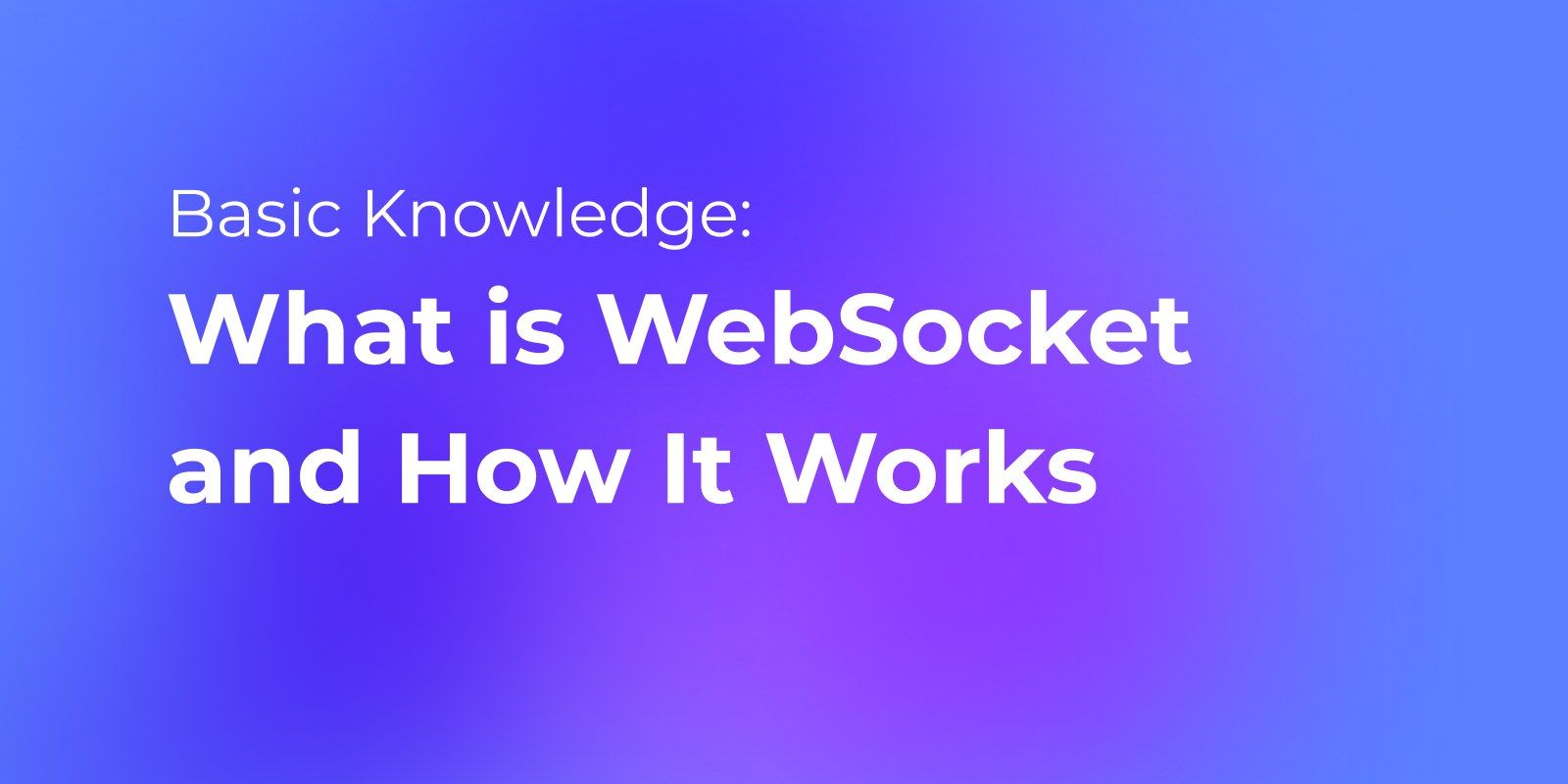
WebSocket's Example
Here's a simple JavaScript example of a WebSocket client connecting to a server:
// Client-side codeconst socket = new WebSocket("wss://example.com/socket"); // Replace with your server's WebSocket URL// Event handler for when the connection is established
socket.addEventListener("open", (event) => {
console.log("WebSocket connection opened.");
// Send data to the server
socket.send("Hello, Server!");
});
// Event handler for incoming messages from the server
socket.addEventListener("message", (event) => {
console.log(`Received message from server: ${event.data}`);
});
// Event handler for when the connection is closed
socket.addEventListener("close", (event) => {
console.log("WebSocket connection closed.");
});
// Event handler for handling errors
socket.addEventListener("error", (event) => {
console.error("WebSocket error:", event);
});
5. Socket
A socket is a software abstraction that allows programs running on different devices to communicate with each other over a network. It provides a standard interface for network communication, enabling data to be sent and received between applications running on separate computers. Sockets are commonly used in networking applications to establish connections and exchange data.
Example in Python:
Here's a simple Python example of a TCP server and client using sockets:
Server (server.py):
import socket
# Create a TCP/IP socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Bind the socket to a specific address and port
server_address = ('127.0.0.1', 12345)
server_socket.bind(server_address)
# Listen for incoming connections (max 5 clients in the queue)
server_socket.listen(5)
print("Server is listening for incoming connections...")
while True:
# Wait for a connectionprint("Waiting for a client to connect...")
client_socket, client_address = server_socket.accept()
try:
# Receive data from the client
data = client_socket.recv(1024)
print(f"Received data: {data.decode('utf-8')}")
# Send a response back to the client
response = "Hello, client!"
client_socket.send(response.encode('utf-8'))
finally:
# Clean up the connection
client_socket.close()
6. SSE (Server-Sent Events)
SSE is a real-time communication technology based on HTTP that allows servers to send asynchronous messages to clients. SSE can refer to several different things depending on the context, but one common meaning is "Sum of Squared Errors." SSE is a mathematical metric used in various fields, particularly in statistics and machine learning, to measure the accuracy of a model's predictions compared to the actual data.
Example:
SSE is often used for streaming updates. For instance, you can receive real-time stock market data updates as they happen.
7. gRPC (gRPC Remote Procedure Call)
gRPC is ideal for backend-to-backend communication, particularly in microservices architectures.
gRPC, which stands for "Google Remote Procedure Call," is an open-source framework developed by Google for building efficient and high-performance distributed systems. It's designed for enabling communication between applications or microservices over a network, making it easier for them to interact with one another.
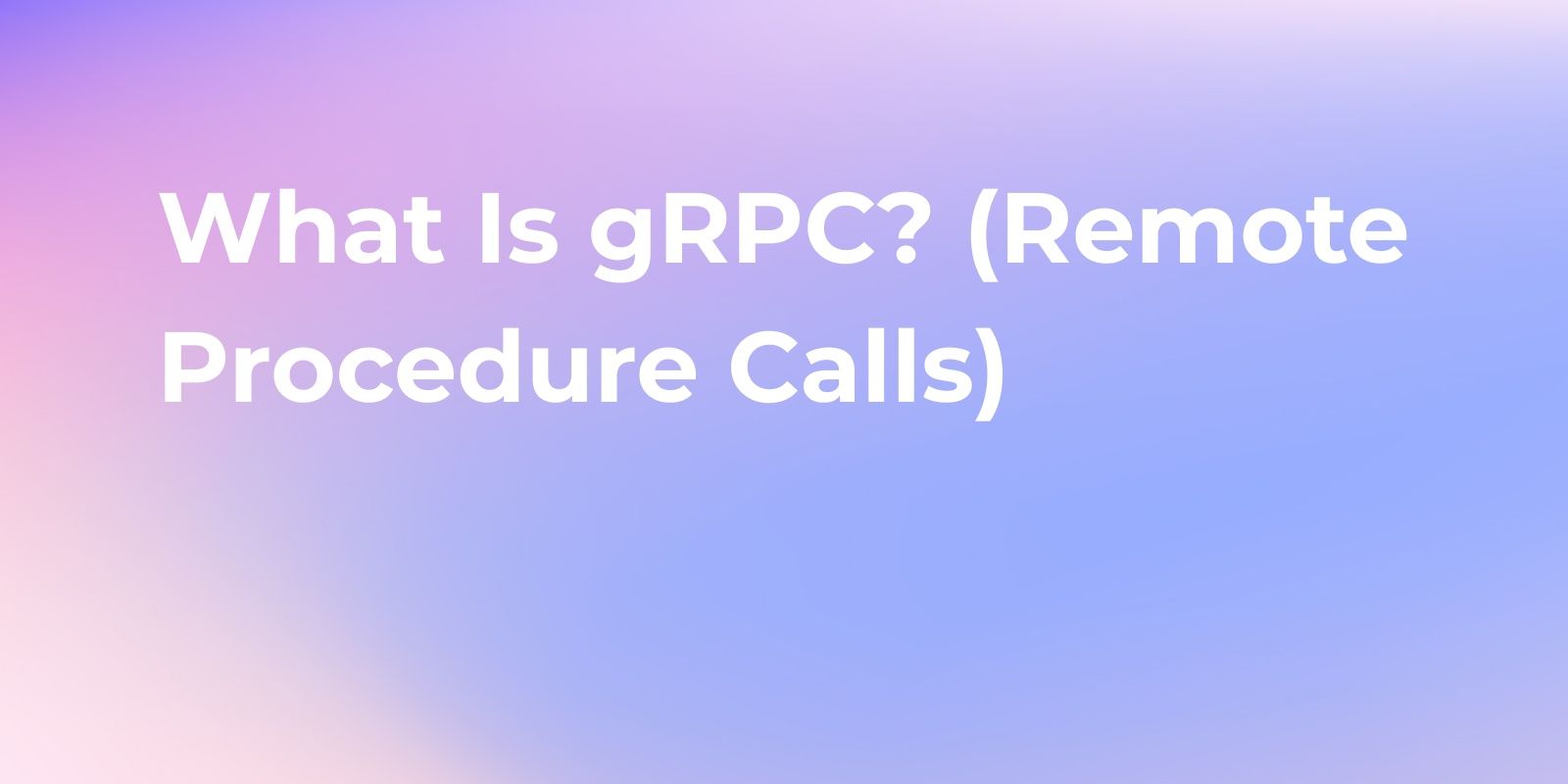
Example of gRPC
Suppose you want to create a gRPC service for a calculator with two methods: Add
and Subtract
. You'd define the service and messages like this in a Protobuf file (e.g., calculator.proto
):
syntax = "proto3";
package calculator;
service Calculator {
rpc Add(AddRequest) returns (AddResponse);
rpc Subtract(SubtractRequest) returns (SubtractResponse);
}
message AddRequest {
int32 num1 = 1;
int32 num2 = 2;
}
message AddResponse {
int32 result = 1;
}
message SubtractRequest {
int32 num1 = 1;
int32 num2 = 2;
}
message SubtractResponse {
int32 result = 1;
}
8. MsgPack (MessagePack)
MsgPack is an open standard for compact binary data serialization, ideal for efficient data transfer.
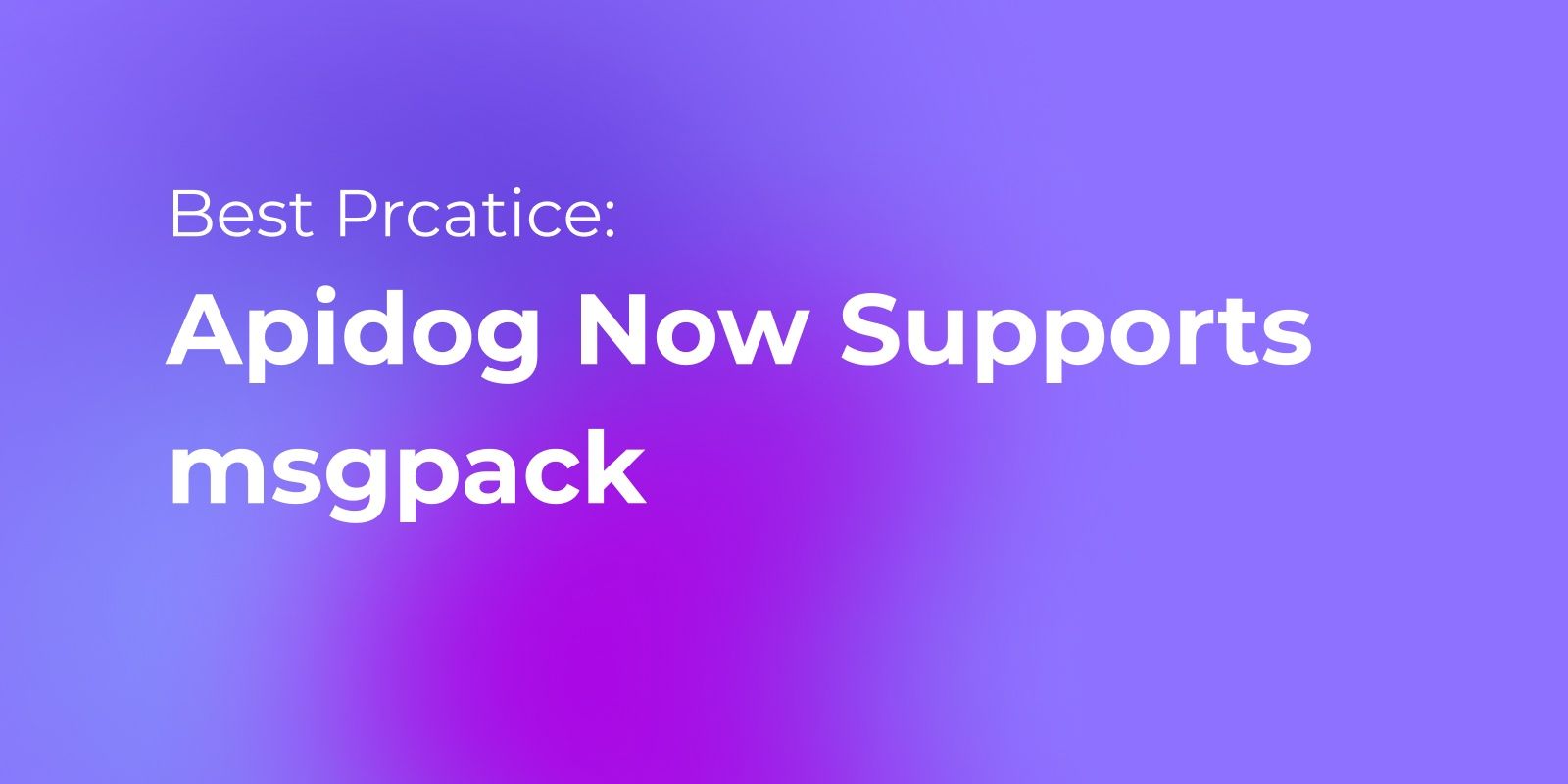
MsgPack supports a variety of data types, including integers, floating-point numbers, strings, arrays, maps (key-value pairs), and more. It is designed to be platform-agnostic, meaning that you can serialize data in one programming language and deserialize it in another without compatibility issues.
Example of MsgPack
Here's a brief example of MsgPack serialization and deserialization in Python:
import msgpack
# Creating a Python dictionary to represent some data
data = {
"name": "John Doe",
"age": 30,
"is_student": False,
"scores": [95, 88, 72]
}
# Serialize the data to a MsgPack binary format
packed_data = msgpack.packb(data)
# Deserialize the MsgPack binary data back to a Python object
unpacked_data = msgpack.unpackb(packed_data)
# Print the original data and the deserialized dataprint("Original Data:", data)
print("Deserialized Data:", unpacked_data)
Apidog: Support All Protocols in ONE
Apidog is your all-in-one solution, supporting above all protocols, making it a versatile tool for API design, development, testing, and management. Whether you're creating a RESTful API, crafting a GraphQL service, or implementing real-time communication with WebSocket, Apidog has got you covered.
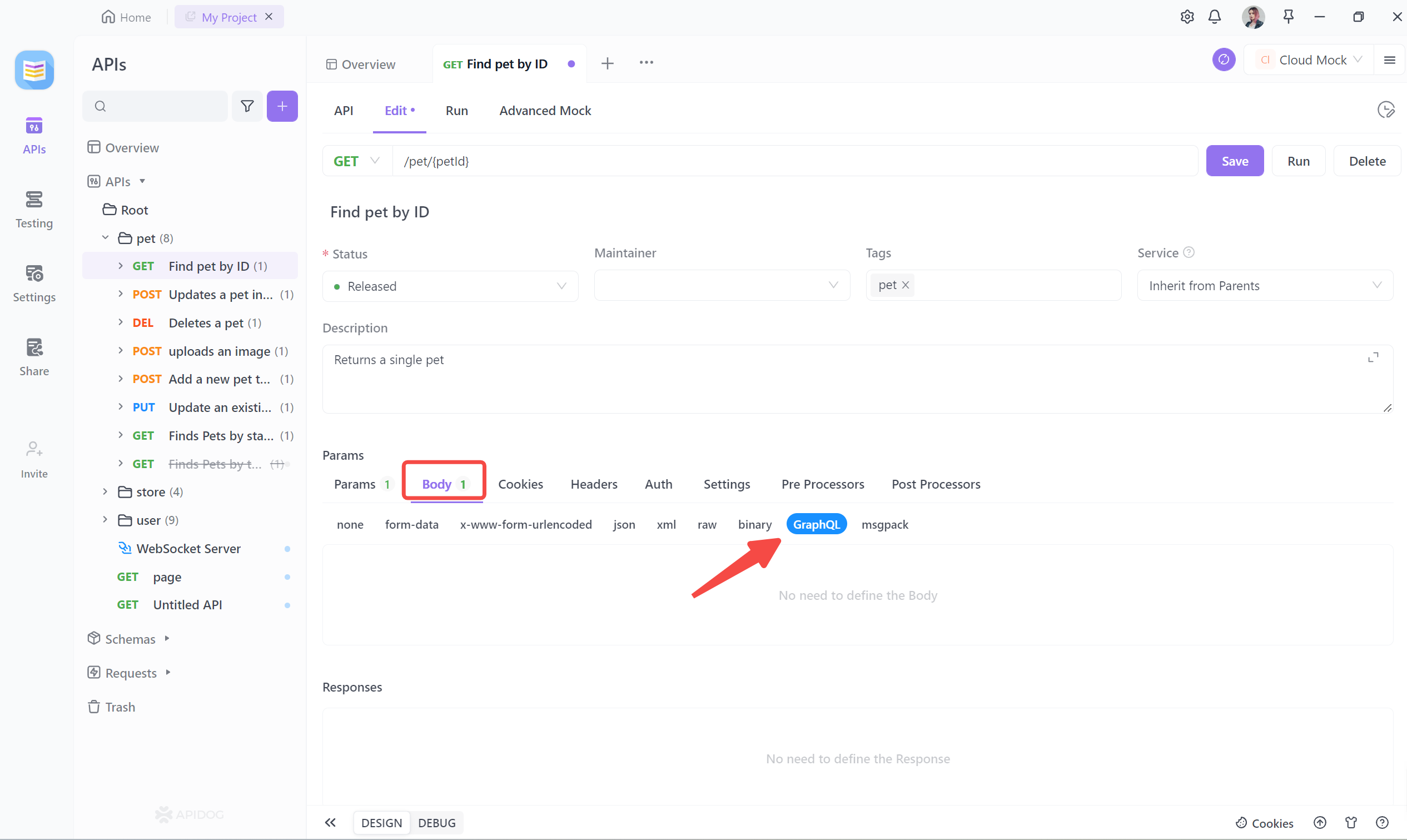
In essence, Apidog seamlessly combines the capabilities of Postman, Swagger, Mock, and JMeter into a single, comprehensive tool, offering a holistic solution for API development, testing, and management. It empowers developers and teams to work effortlessly with diverse API protocols, eliminating the need to hunt for specialized tools for each protocol.
Think of Apidog as the fusion of Postman, Swagger, Mock, and JMeter, all rolled into one. It provides a unified, top-tier API management solution for developers worldwide.