What Is gRPC? Definition, Architecture, Pros & Cons
gRPC is an open-source Remote Procedure Call (RPC) framework developed by Google. In this article, we will explore its definition, architecture, pros, and cons, and compare it with other RPC frameworks.
gRPC is an open-source Remote Procedure Call (RPC) framework developed by Google. It enables communication between client and server applications using a simple and efficient protocol, making it ideal for building distributed systems and microservices. gRPC uses Protocol Buffers (Protobuf) as its default serialization framework and HTTP/2 as its underlying transport protocol, providing high performance and efficiency.
In this article, we will explore its definition, architecture, pros, and cons, and compare it with other RPC frameworks.
Core Concepts of gRPC
Understanding its core concepts is essential for harnessing its full potential. Here are the key elements:
Protocol Buffers (Protobuf):
Protocol Buffers is a language-agnostic, efficient, and extensible data serialization format used by gRPC. It allows developers to define the structure of their data in a .proto file, specifying the data types and message formats. gRPC uses these .proto files to automatically generate code in various programming languages for message serialization and deserialization.
Streaming:
gRPC supports different types of streaming, enabling efficient communication for real-time data exchange. It offers three streaming options: server streaming, client streaming, and bidirectional streaming. In server streaming, the server sends a stream of messages in response to a single client request. Client streaming allows the client to send a stream of messages to the server. Bidirectional streaming enables both the client and server to send and receive independent streams of messages concurrently.
HTTP/2:
gRPC is built on top of HTTP/2, a major improvement over the traditional HTTP/1.1 protocol. HTTP/2 introduces features such as request and response multiplexing, header compression, and server push. These enhancements enhance performance and efficiency, reducing latency and improving data transmission over the network.
gRPC Architecture:
The gRPC architecture follows a client-server model. The client sends a request to the server, and the server responds with a corresponding reply. The communication between the client and server is facilitated by gRPC stubs and service definitions. The stubs are auto-generated code that acts as a client-side proxy, making remote calls appear like local function calls. The service definitions define the methods that the server can implement, and they are specified in the .proto files using Protocol Buffers.
What is gRPC Used For?
gRPC is used for building efficient and high-performance communication between different services and applications in modern distributed systems. It provides a framework for implementing Remote Procedure Calls (RPC) that allows client and server applications to communicate with each other over the network, making it easier to build and maintain complex microservices architectures.
Some common use cases for gRPC include:
Microservices Communication: gRPC is widely used in microservices architectures to enable seamless communication between different services. Its efficient binary serialization format (Protocol Buffers) and support for streaming make it ideal for handling large volumes of data and real-time communication.
API Development: gRPC can be used to build APIs for web, mobile, and IoT applications. Its speed, efficiency, and language-agnostic nature make it a compelling choice for developing APIs that require high-performance and real-time capabilities.
Inter-Service Communication: In distributed systems, different services often need to communicate with each other to exchange data or perform specific tasks. gRPC simplifies this communication by providing a standardized and efficient way for services to interact.
Client-Server Communication: gRPC is suitable for various client-server communication scenarios, allowing applications to make remote procedure calls to servers and receive responses efficiently.
Real-Time Applications: With its support for bidirectional streaming and server push, gRPC is well-suited for building real-time applications, such as chat applications, real-time analytics, and live tracking systems.
Performance-Critical Applications: gRPC's use of HTTP/2 and binary serialization results in improved performance and reduced latency, making it an excellent choice for applications that require high efficiency and low response times.
Cross-Platform Communication: gRPC's cross-language support allows developers to build services and applications in different programming languages, promoting interoperability across various platforms.
gRPC Advantages and Disadvantages
Here's a simple table comparing the advantages and disadvantages of gRPC:
Aspect | Advantages | Disadvantages |
---|---|---|
Performance | Efficient binary serialization (Protocol Buffers), smaller message sizes, faster parsing | Complexity compared to REST APIs, may introduce overhead in certain use cases |
Streaming | Server, client, and bidirectional streaming modes for real-time communication | Requires familiarity with Protocol Buffers and HTTP/2, may not be fully supported in older browsers |
Multiplexing | HTTP/2-based request and response multiplexing for reduced latency and improved system performance | Debugging and inspection challenges due to binary nature of messages |
Cross-Language | API contracts using Protocol Buffers allow communication between services in different programming languages | gRPC is a relatively newer technology compared to REST with a longer track record |
Code Generation | Automatic client and server code generation in multiple languages, minimizing manual coding efforts | Potential overhead compared to simpler REST communication in certain scenarios |
Bidirectional | Supports bidirectional communication, enabling real-time interactive applications | |
Community Support | Backed by Google and supported by a thriving community |
gRPC vs REST
RPC stands for Remote Procedure Call, which refers to a method of invoking a function or procedure on a remote server. In simpler terms, imagine you have two servers, A and B. The application deployed on server A needs to call a method provided by another application deployed on server B. Since they are not in the same memory space, direct invocation is not possible, and network communication is required to achieve the desired call.
From the diagram above, it can be seen that RPC is a client-server pattern. From a certain perspective, any calls outside of the application itself can be classified as RPC. Whether it's microservices, third-party HTTP interfaces, or interacting with database middleware like MySQL or Redis.
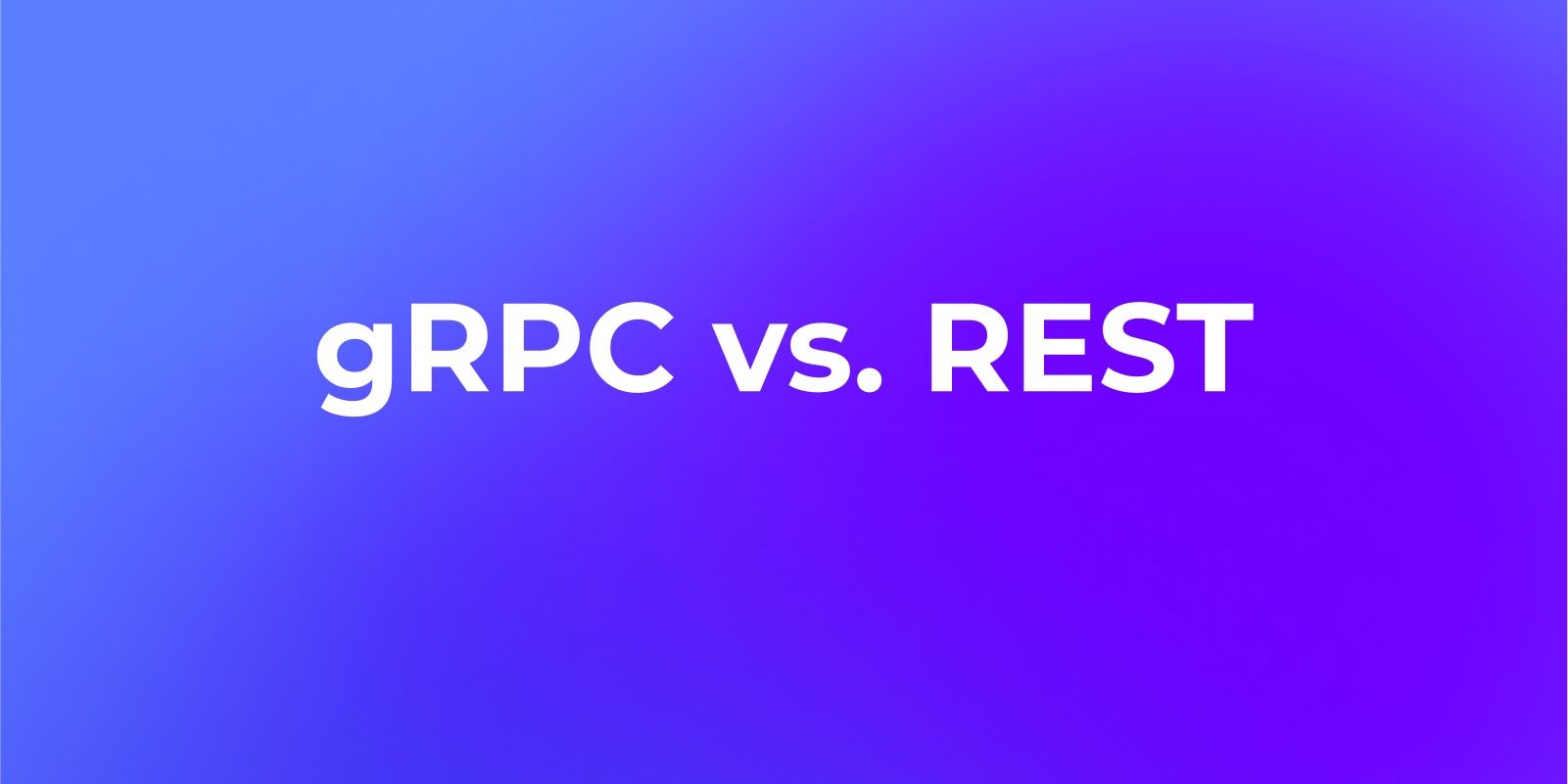
Aspect | gRPC | REST |
---|---|---|
Communication Protocol | Based on Remote Procedure Calls (RPC) | Based on Representational State Transfer |
Data Format | Binary (Protocol Buffers) | Text-based (JSON, XML) |
Streaming Support | Bidirectional, Server, and Client Streaming | No native support for bidirectional streaming |
Performance | Efficient, smaller message sizes | Simpler, but larger message sizes |
Language Support | Cross-language support using Protobuf | Cross-language support |
Code Generation | Automatic code generation | No automatic code generation |
Use Cases | High-performance, real-time applications | Simpler applications, web and mobile APIs |
gRPC Security
gRPC provides robust security through Transport Layer Security (TLS) encryption, ensuring confidential and tamper-proof data transmission between clients and servers. It supports mutual TLS authentication for verifying the identity of both parties, and developers can implement custom credential-based authentication and authorization mechanisms. With secure interceptors and HTTP/2's built-in security features, gRPC offers a comprehensive security framework for protecting sensitive data in distributed systems.
The Benefits of gRPC
gRPC is popular for several reasons:
High Performance: gRPC uses binary serialization format (Protocol Buffers) which results in smaller message sizes and faster serialization and parsing compared to traditional text-based formats like JSON. This makes data transmission more efficient, saving bandwidth and resources.
Streaming Support: gRPC offers various streaming modes, such as server streaming, client streaming, and bidirectional streaming. This makes real-time communication and handling of large data streams more straightforward and efficient.
Multiplexing: Built on HTTP/2, gRPC leverages request and response multiplexing, allowing multiple requests and responses to be sent over a single connection. This reduces latency and improves overall performance.
Cross-Language Support: By defining API contracts using Protocol Buffers, gRPC supports multiple programming languages, making communication between services simple and flexible regardless of the language used for development.
Automatic Code Generation: The gRPC protoc compiler can automatically generate client and server code in various languages, reducing the need for manual coding and speeding up development.
Bidirectional Communication: gRPC enables bidirectional communication, enabling both the client and server to initiate data transfers independently, facilitating real-time interactive applications.
Strong Community Support: Developed by Google and backed by a thriving community, gRPC enjoys widespread adoption and continuous improvements through contributions from developers and organizations.
Interoperability: gRPC is designed to interoperate with other HTTP-based systems, allowing seamless integration with existing technologies, making it an ideal choice for modernizing legacy systems.
gRPC in Apidog
Apifdoghas launched the gRPC API debugging feature, and we will demonstrate how to create a new gRPC project in Apidog and debug the interface through a sample scenario.
Note: The gRPC interface management feature requires Apidog version 2.3.0 or higher.
Server Streaming
As the icon suggests, Server Streaming means sending multiple response data in one request. For example, subscribing to all the transaction price data of stocks within one minute.
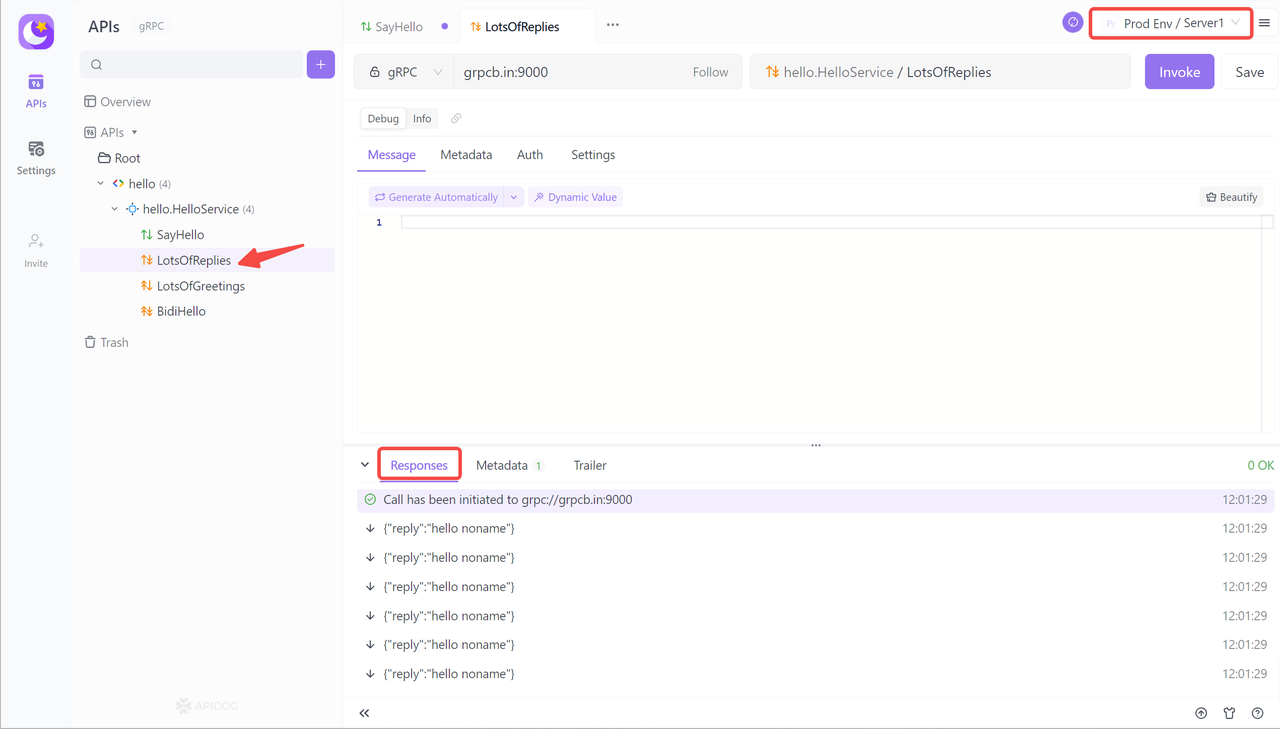
Client Streaming
In this mode, the client can continuously send multiple request messages to the server without waiting for immediate responses. Once all the requests are processed, the server sends a single response message back to the client. It is ideal for efficiently transmitting large amounts of data in a streaming fashion, reducing latency and optimizing data exchange.
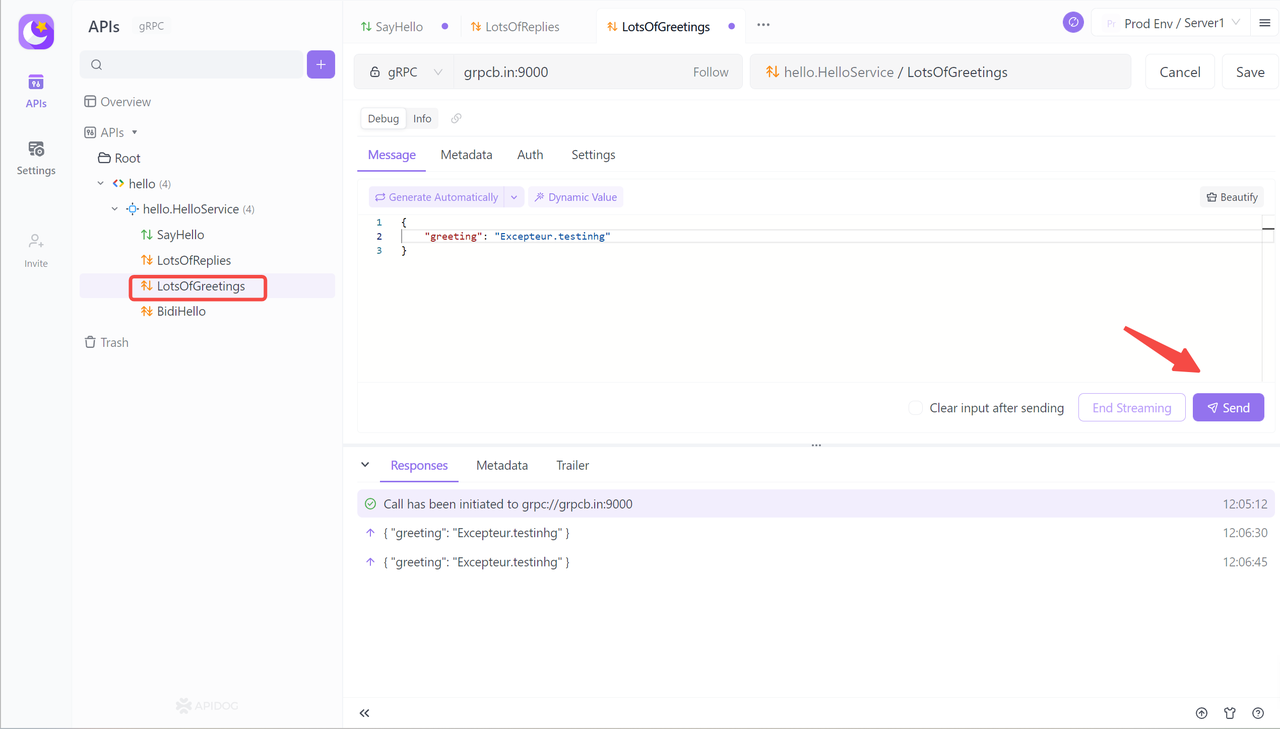
Bidirectional Streaming
Bidirectional Streaming allows clients and servers to establish persistent bidirectional communication and can transmit multiple messages at the same time. It is commonly used in online games and real-time video call software, and is suitable for real-time communication and large-scale data transmission scenarios. After initiating the call, the client and the server will maintain a session between them and receive real-time responses after sending different request contents.
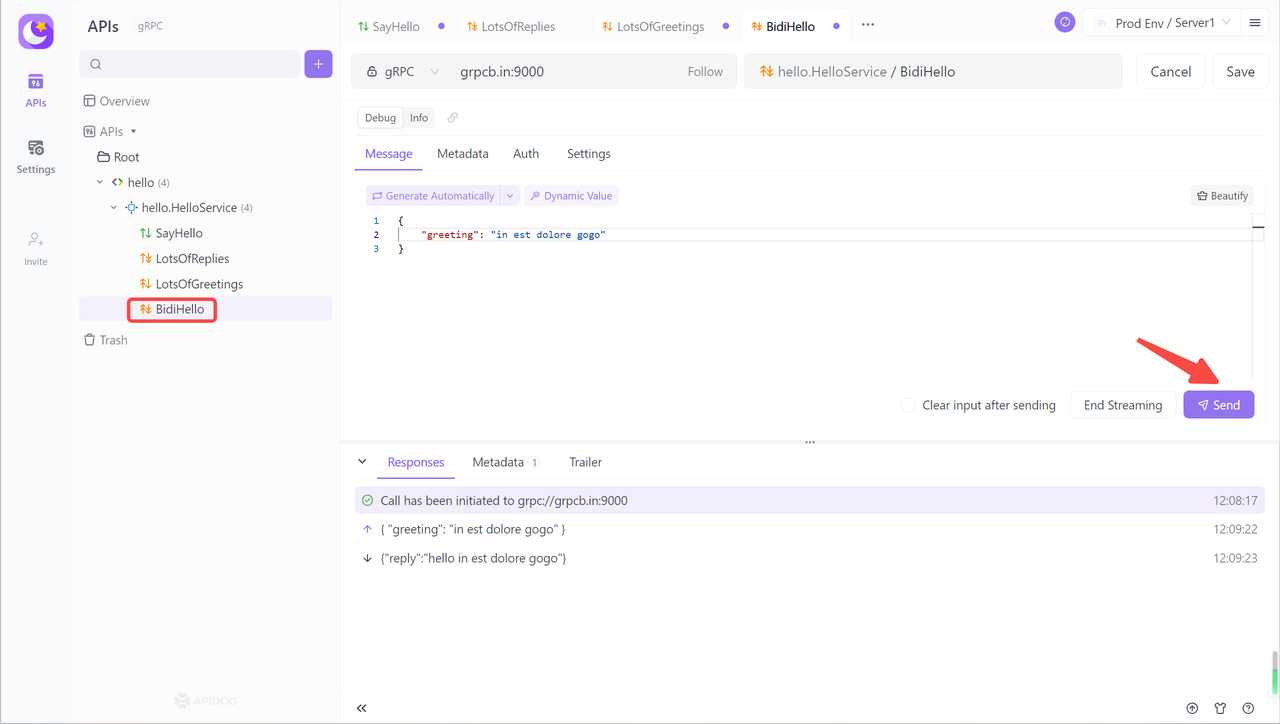
gRPC API Collaboration
Apidog can render gRPC interface documents that are more suitable for human reading based on .proto files, making it easier to collaborate on interfaces within a team. You can click on the menu button on the right side of the interface to get the collaboration link and share it with other team members to align the debugging method of the interface.
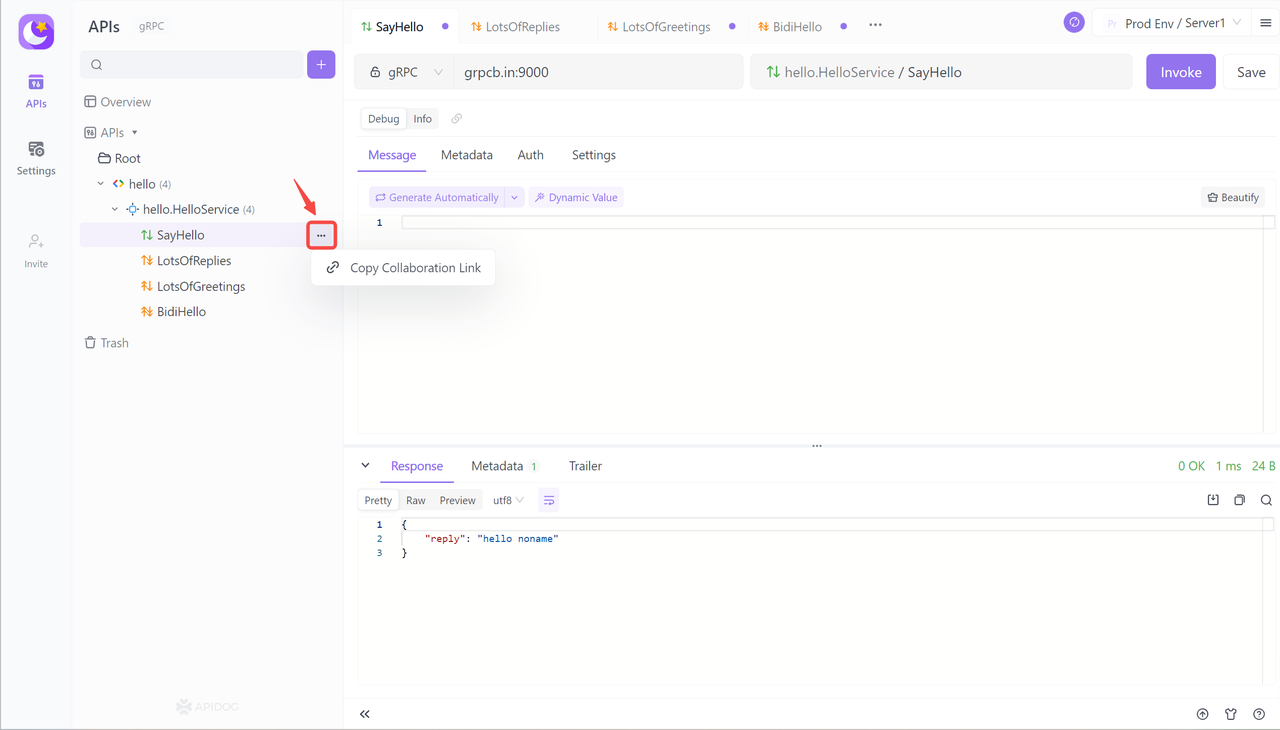