How to Use an API in Python: A Step-by-Step Guide
Learn how to harness the power of APIs with Python in this step-by-step guide. From making your first API call to handling responses, we’ve got you covered.
Are you looking to unlock the power of APIs in your Python development? Look no further! In this blog post, we will cover everything you need to know to get started with using APIs in Python. From understanding the basics of APIs to advanced techniques for working with them, we will provide you with the knowledge and skills necessary to integrate APIs into your projects with ease. Whether you're a seasoned developer or just starting out, this post will help you take your API integration to the next level.
What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. It enables developers to use functionalities that are provided by another service without having to understand the intricate details of how the service is implemented.
For example, if you’re creating an app and you want to include weather data, you don’t need to create a system to gather and process weather information. Instead, you can use a weather service’s API to send a request and receive the weather data you need.
APIs are essential for building software and applications in today’s interconnected digital world, as they allow for the integration of different systems and services, making them more powerful and versatile. They can be used for web services, operating systems, databases, and many other purposes.
Why Should You Care About APIs?
APIs are important for several reasons, and here’s why you should care about them:
- Integration with other systems: APIs allow different software programs to communicate, making it possible to integrate and connect various systems within an organization. This can lead to more efficient operations and better data management.
- Flexibility and control: With APIs, you can customize the user experience by creating tailored interfaces or integrating features from other services into your own application.
- Innovation and growth: APIs enable developers to build upon existing platforms, fostering innovation and allowing businesses to leverage new functionalities without reinventing the wheel.
- Engagement with customers and vendors: By exposing certain APIs, you can allow customers or vendors to interact directly with your systems, which can streamline processes and improve service delivery.
- Monetization: APIs can be used to create new revenue streams by allowing third parties to access your services or data in a controlled manner.
In essence, APIs are the building blocks of modern software development, enabling businesses to be more agile, innovative, and customer-centric. They are crucial for anyone involved in technology or digital services.
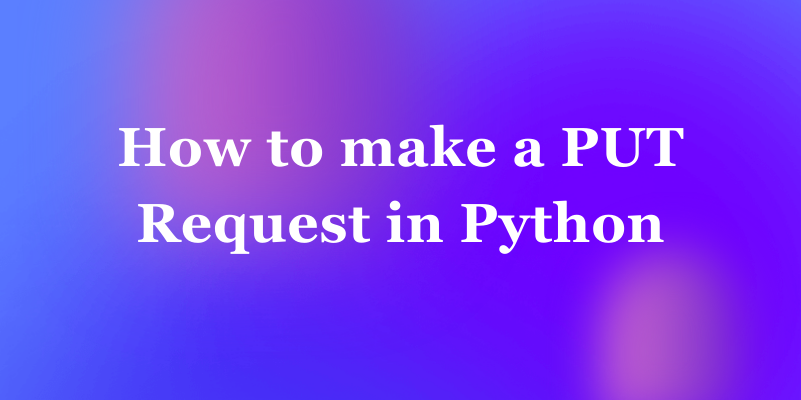
Why Python for APIs?
Python is a fantastic language for working with APIs. Its simplicity and readability make it accessible to beginners, while its powerful libraries and frameworks are robust enough for complex applications. Plus, with Python, you can focus on the logic of your application without getting bogged down by verbose syntax.
Getting Started: Python and APIs
Getting started with Python and APIs is an exciting journey that opens up a world of possibilities for automating tasks, integrating systems, and accessing vast amounts of data. Here’s a simple guide to help you begin:
Understand the Basics:
- Learn what an API is and how it works.
- Familiarize yourself with HTTP method like GET, POST, PUT, and DELETE.
Set Up Your Environment:
- Install Python on your system if you haven’t already.
- Ensure you have a code editor, like Visual Studio Code, to write your scripts.
Learn to Use requests
:
- The
requests
library in Python is essential for making API calls. - Install it using pip:
pip install requests
.
Read API Documentation:
- Before you can use an API, you need to understand its documentation to know the endpoints and data format.
Make Your First API Call:
- Start with a simple API that doesn’t require authentication, like a public data API.
- Use the
requests
library to send a request and handle the response.
Work with JSON Data:
- Most APIs will return data in JSON format, so get comfortable with parsing and using JSON in Python.
Handle API Keys and Authentication:
- For APIs that require authentication, learn to manage API keys securely.
Practice:
- The best way to learn is by doing. Try integrating different APIs into your projects.
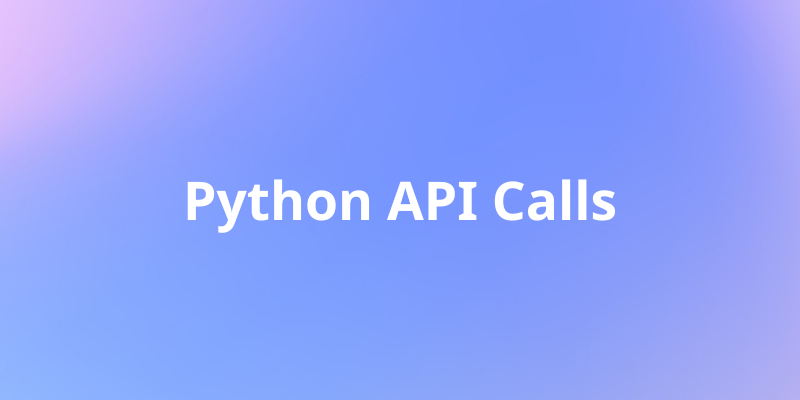
Making Your First API Request with Python
Making your first API request in Python is a great way to start interacting with web services. Here’s a step-by-step guide to help you make your first API request:
Choose an API: For your first request, choose a simple API that doesn’t require authentication. An example could be an API that provides random facts or data.
Install requests
Library: Python’s requests
library is the de facto standard for making HTTP requests. You can install it using pip:
pip install requests
Import requests
: At the beginning of your Python script, import the library:
import requests
Make a GET Request: Use the get
method to make a GET request to the API’s endpoint:
response = requests.get('API_ENDPOINT')
Check the Response: It’s good practice to check the response status code to ensure the request was successful:
if response.status_code == 200:
print("Success!")
else:
print("Error:", response.status_code)
Process the Data: If the response is successful, you can process the data, which is often returned in JSON format:
data = response.json()
print(data)
Here’s a complete example that makes a request to an API that provides random facts:
import requests
# Replace 'API_ENDPOINT' with the actual endpoint of the API you are using
response = requests.get('API_ENDPOINT')
if response.status_code == 200:
# Parse the response as JSON
data = response.json()
# Print a random fact
print(data['fact'])
else:
print("Failed to retrieve data:", response.status_code)
Remember to replace 'API_ENDPOINT'
with the actual endpoint URL of the API you want to use.
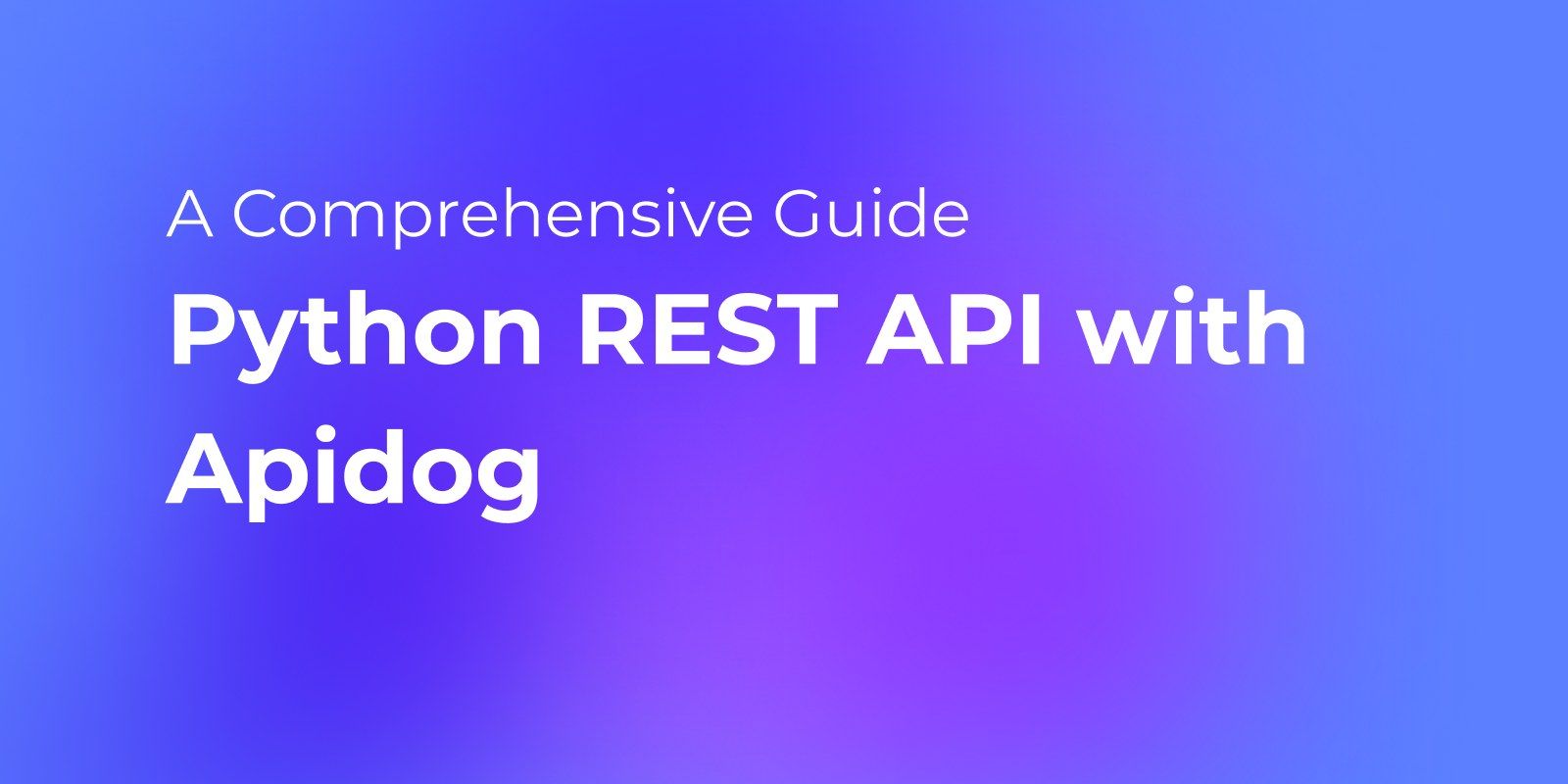
Understanding API Responses with Python
Understanding API responses in Python is crucial for effectively working with APIs. Here’s a brief guide on how to interpret and handle these responses:
Status Codes: The response from an API request includes a status code that indicates the result of the request. Common status codes are:
200 OK
: The request was successful.400 Bad Request
: The request was not understood by the server.401 Unauthorized
: Authentication is required and has failed or has not been provided.403 Forbidden
: The request was valid, but the server is refusing action.404 Not Found
: The requested resource could not be found.500 Internal Server Error
: An unexpected condition was encountered by the server.
Response Content: The body of the response often contains the data you requested, usually in JSON format. You can parse this data using Python’s json
library.
Headers: Response headers can provide useful information such as content type, server, date, and more.
Error Handling: It’s important to write code that gracefully handles errors by checking the status code and providing appropriate feedback to the user or logs.
Here’s an example of handling an API response in Python:
import requests
# Make an API request
response = requests.get('API_ENDPOINT')
# Check the status code of the response
if response.status_code == 200:
# Parse the response content as JSON
data = response.json()
# Process the data
print(data)
elif response.status_code == 404:
print("Resource not found.")
else:
print(f"Error: {response.status_code}")
Replace 'API_ENDPOINT'
with the actual endpoint of the API you’re using. This code checks the status code and prints out the data if the request was successful, or an error message if it wasn’t.
Test your Python API with Apidog
Testing your Python API with Apidog can streamline the process and ensure that your API functions as expected. Apidog is a tool that can help you design, develop, debug, and test your APIs.
- Open Apidog and create a new request.
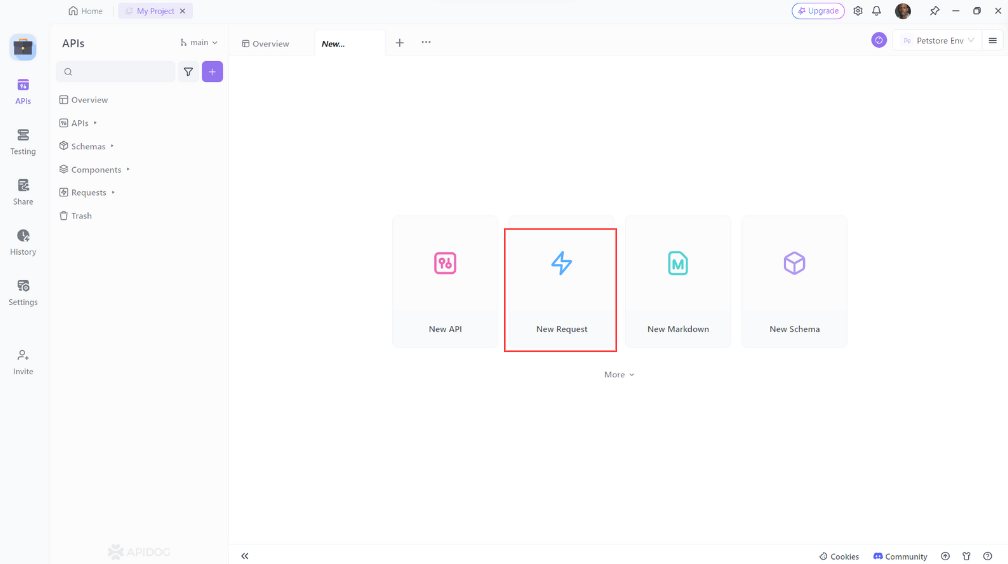
2. Set the request method to GET.
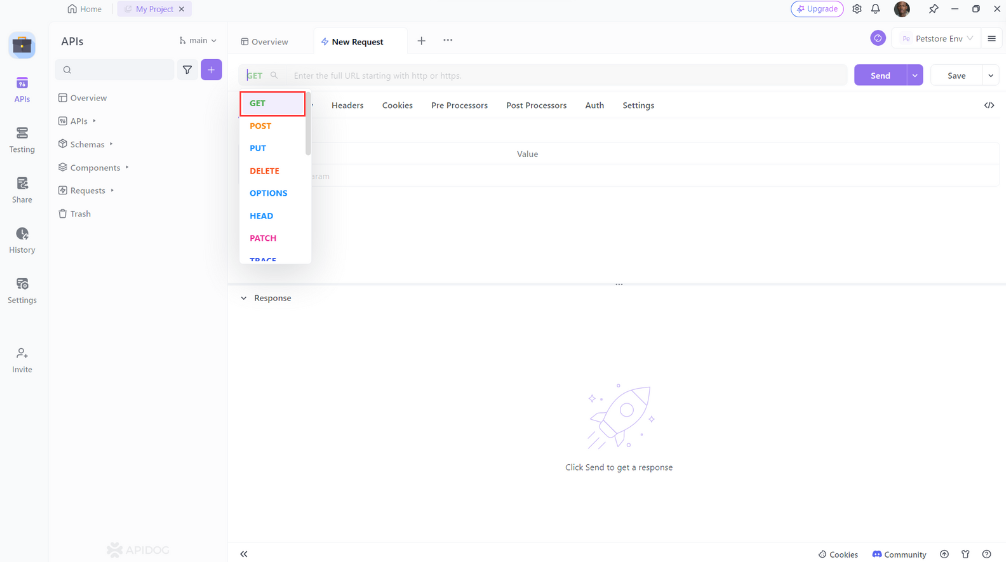
3. Enter the URL of the resource you wish to update. You can also add any additional headers or parameters you want to include, and then click the 'Send' button to send the request
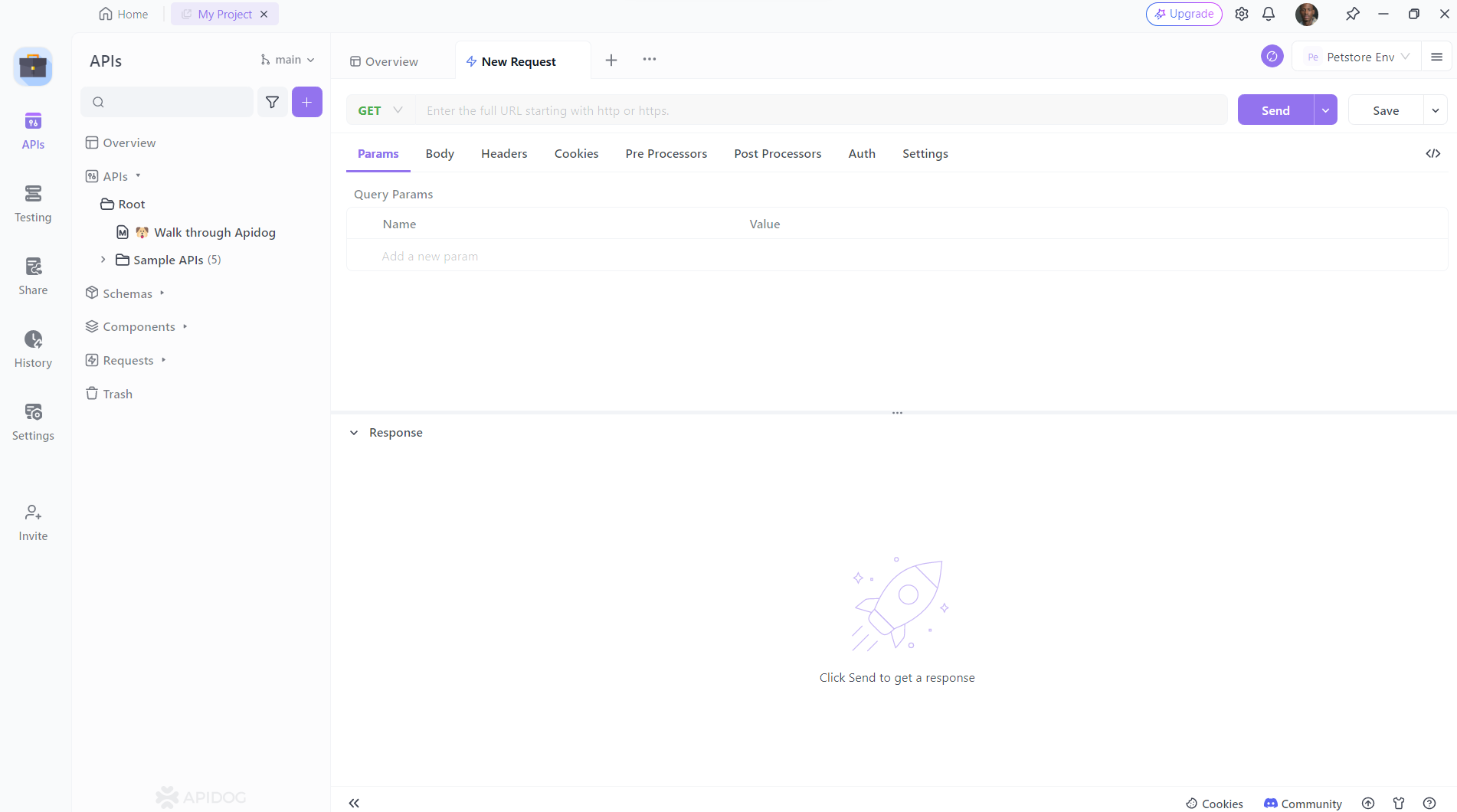
4. Confirm that the response matches your expectations.
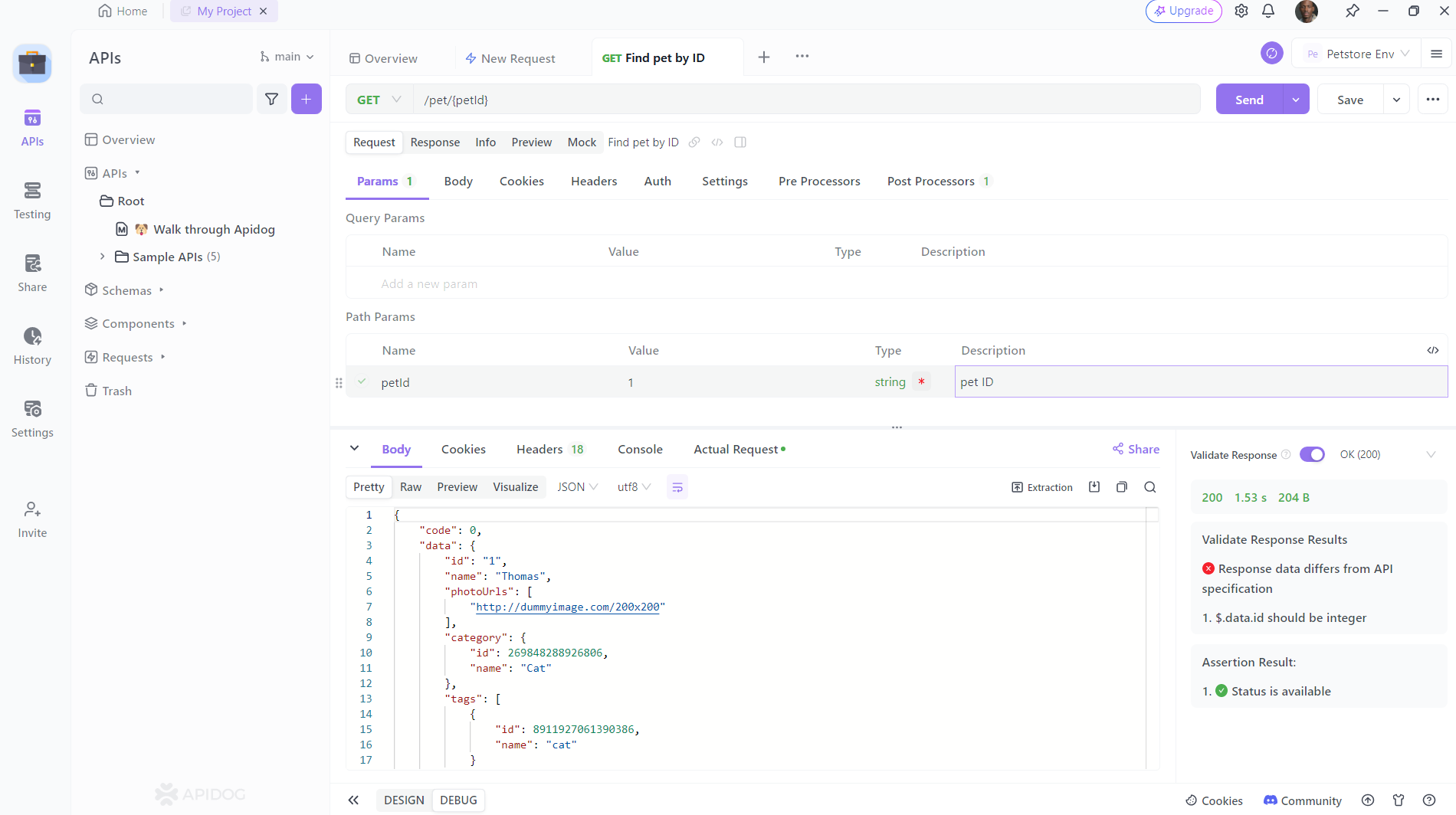
Apidog provides a comprehensive guide that explores leveraging its features to enhance your Python REST API development, making it faster, more efficient, and a pleasure to work with.
Python Libraries for API Interaction
Apart from requests
, Python offers other librarie that can help you interact with APIs effectively.
- Urllib: Part of Python’s standard library,
urllib
provides a high-level interface for fetching data across the web. It’s useful for basic operations but lacks some of the conveniences provided byrequests
. - HTTPx: A fully featured HTTP client for Python 3, which provides async capabilities and is often considered as a next-generation
requests
library. - Flask: While primarily a micro web framework, Flask can be used to create APIs and handle API requests within a web application context.
- Django REST Framework: A powerful and flexible toolkit for building Web APIs in Django applications.
- FastAPI: A modern, fast web framework for building APIs with Python 3.7+ based on standard Python type hints.
- Tornado: A Python web framework and asynchronous networking library, originally developed at FriendFeed, which is particularly well-suited for long polling, WebSockets, and other applications that require a long-lived connection to each user.
- Sanic: A Flask-like Python 3.7+ web server that’s written to go fast. It’s particularly good at handling asynchronous requests.
These libraries can help you make requests to APIs, handle the responses, and even create your own APIs. Each has its own strengths and use cases, so the choice of library will depend on the specific requirements of your project and your familiarity with the library.
Conclusion
Integrating APIs into your Python development can greatly enhance the functionality and interactivity of your projects. By following the tips and techniques outlined in this blog post, you'll be able to access a wide range of external data sources and build more robust and powerful applications. Remember, API integration is a crucial part of modern software development, and with practice and experience, you can become a master of working with APIs in Python.
By incorporating Apidog into your workflow, you can design, execute, and manage tests with ease, giving you the confidence that your API is ready for the real world. So, what are you waiting for? Start integrating APIs into your projects
today and see the difference it can make!