How to Use ReactJS Query to Fetch Data from APIs
Learn how to use ReactJS Query, a library that simplifies data fetching and caching for React applications, to fetch data from APIs and display it on your website.
In this blog post, I will show you how to use ReactJS Query to fetch data from APIs and display it on your website and how to generate your ReactJS Query code with Apidog
What is ReactJS Query and Why Should You Use It?
ReactJS Query is a library that helps you manage data fetching, caching, and updating in your React apps. It’s based on the idea of queries, which are functions that fetch data from an API or any other source. ReactJS Query handles the loading, caching, refetching, and error handling of these queries for you, so you don’t have to worry about them.
ReactJS Query also provides you with mutators, which are functions that update data on the server or any other source. ReactJS Query handles the optimistic updates, rollback, and error handling of these mutators for you, so you don’t have to worry about them either.
ReactJS Query also integrates seamlessly with React, using custom hooks and components that let you access and manipulate data in your components. ReactJS Query also supports features like pagination, infinite scrolling, background fetching, dependent queries, and more.
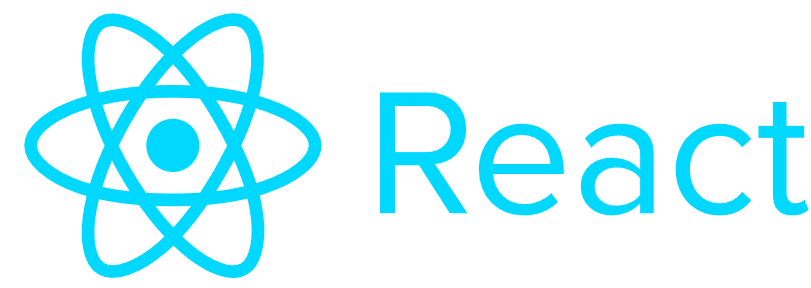
So why should you use ReactJS Query? Here are some of the benefits of using ReactJS Query in your React apps:
- It simplifies your code: ReactJS Query reduces the amount of code you have to write to fetch, cache, and update data in your apps. You don’t have to use Redux, Axios, SWR, or any other library to manage data. You just use ReactJS Query and its hooks and components, and you’re good to go.
- It improves your performance: ReactJS Query optimizes the performance of your apps by caching data and avoiding unnecessary requests. It also allows you to control when and how often data is refetched, and how stale data is handled. ReactJS Query also supports features like suspense, concurrent mode, and prefetching, which can further enhance your app’s performance.
- It enhances your user experience: ReactJS Query improves the user experience of your apps by providing smooth and consistent data loading and updating. It also allows you to customize the loading, error, and success states of your data, and provide feedback to your users. ReactJS Query also supports features like optimistic updates, which can make your app feel more responsive and interactive.
How to install and set up ReactJS
ReactJS is a JavaScript library for building user interfaces. To install and set up ReactJS on your computer, you can follow these steps:
- Step 1: Install Node.js, a JavaScript runtime environment, on your computer. You can download the Node.js installer for free from the official website. The long-term support (LTS) version is recommended even though the current version has the latest features.
- Step 2: Install ReactJS using create-react-app, a tool that installs all of the dependencies to build and run a full ReactJS application. You can use
npm
oryarn
, which are package managers for Node.js, to do that. For example, you can run this command in your terminal:
npx create-react-app my-app
This command will create a directory called my-app
inside the current folder, and generate the initial project structure and install the transitive dependencies.
- Step 3: Start the development server and open your app in the browser. You can use
npm
oryarn
to do that. For example, you can run these commands in your terminal:
cd my-app
npm start
These commands will start the development server and open your app in the browser at http://localhost:3000/. You can edit the files in the src
folder and see the changes in the browser.
That’s it! You have successfully installed and set up ReactJS on your computer, and you have created and run your first ReactJS app. You can learn more about ReactJS from its official website, where you can find the documentation, examples, tutorials, and more.
How to create and use queries with ReactJS Query
Now that you know how to install and set up ReactJS Query, let’s see how to create and use queries with ReactJS Query. Queries are the core concept of ReactJS Query, and they are the main way to fetch and display data from APIs.
As we saw in the previous section, you can use the useQuery
hook to create and use queries in your React components. The useQuery
hook takes two arguments: a query key and a query function. The query key is a unique identifier for your query, and the query function is a function that fetches data from an API endpoint and returns a promise.
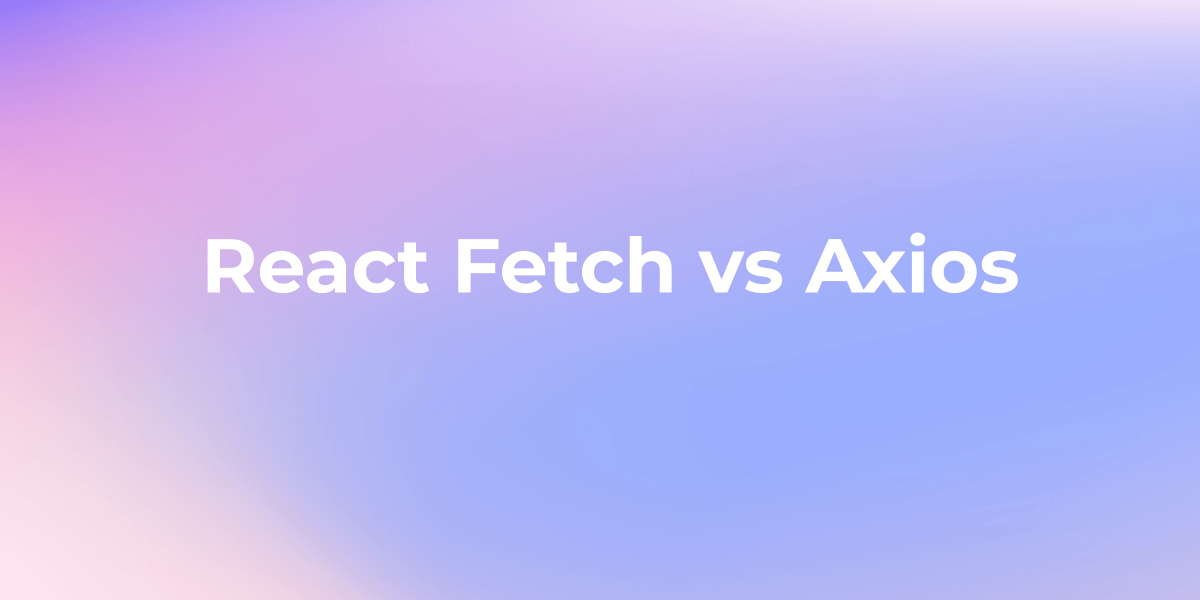
The useQuery
hook returns an object that contains the following properties:
data
: The data that is fetched from the API endpoint. It isundefined
when the query is loading or has an error.status
: The status of the query. It can be one of the following values:'idle'
,'loading'
,'error'
, or'success'
.error
: The error that is thrown by the query function. It isundefined
when the query is loading or has a success.isIdle
: A boolean that indicates whether the query is idle. It istrue
when the query is not enabled or has not started fetching data yet.isLoading
: A boolean that indicates whether the query is loading. It istrue
when the query is fetching data from the API endpoint.isError
: A boolean that indicates whether the query has an error. It istrue
when the query function throws an error or rejects the promise.isSuccess
: A boolean that indicates whether the query has a success. It istrue
when the query function resolves the promise and returns the data.
How to Fetch Data with ReactJS Query
The core feature of ReactJS Query is fetching data with queries. Queries are functions that fetch data from an API or any other source. ReactJS Query provides you with a custom hook called useQuery, which lets you use queries in your components.
The useQuery hook takes two arguments: a query key and a query function. The query key is a unique identifier for your query, which can be a string or an array. The query function is a function that returns a promise that resolves with the data you want to fetch.
For example, let’s say you want to fetch a list of posts from a fake API using ReactJS Query. You can create a query function that uses the fetch API to get the data:
// Define a query function that fetches posts
const fetchPosts = async () => {
// Use the fetch API to get the data
const response = await fetch('https://jsonplaceholder.typicode.com/posts')
// Parse the response as JSON
const data = await response.json()
// Return the data
return data
}
Then, you can use the useQuery hook in your component, passing the query key ‘posts’ and the query function fetchPosts:
// Use the useQuery hook to fetch posts
const { data, isLoading, isError, error } = useQuery('posts', fetchPosts)
The useQuery hook returns an object with several properties that you can use to access and manipulate the data. The most important ones are:
- data: The data returned by the query function, or undefined if the query is loading or has an error.
- isLoading: A boolean that indicates if the query is loading or not.
- isError: A boolean that indicates if the query has an error or not.
- error: The error object returned by the query function, or undefined if the query is loading or has no error.
You can use these properties to render your component based on the state of the query. For example, you can show a loading spinner while the query is loading, an error message if the query has an error, or a list of posts if the query is successful:
// Render the component based on the state of the query
return (
<div>
{isLoading && <div>Loading...</div>}
{isError && <div>Error: {error.message}</div>}
{data && (
<ul>
{data.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)}
</div>
)
That’s how you fetch data with ReactJS Query. ReactJS Query will automatically cache the data for you, and refetch it when the component mounts, unmounts, or the query key changes. You can also customize the behavior of the query using various options, such as staleTime, refetchOnWindowFocus, retry, and more. You can find the full list of options and their descriptions in the ReactJS Query documentation.
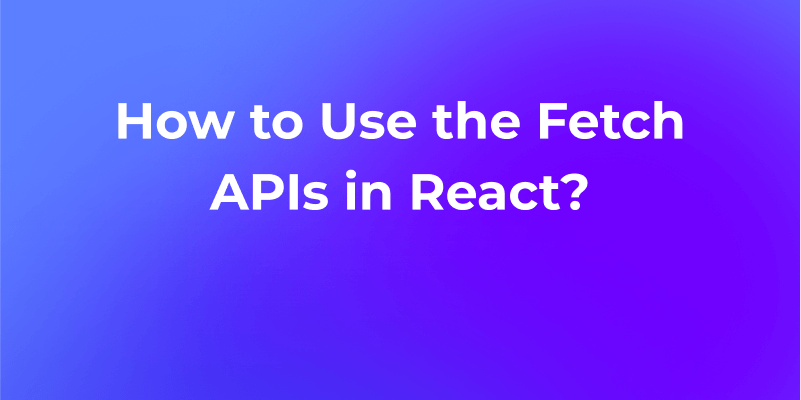
How to use Apidog to generate ReactJS Query client code
Apidog is a web-based platform that helps you discover, test, and integrate web APIs with ease. One of the features that Apidog offers is the ability to generate Fetch client code with one click, based on the API specification that you provide or select.
- Click on the “New API” button on the dashboard or select an existing API from the list.
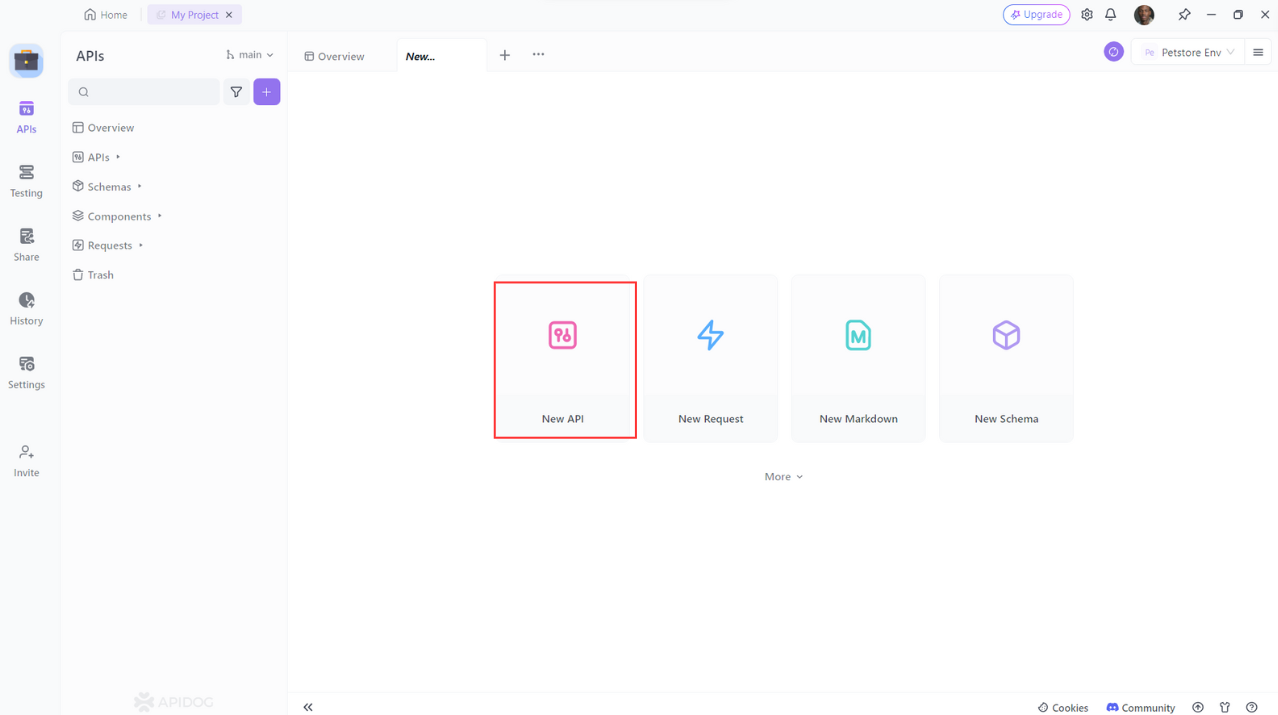
- Click on the “Generate Client Code”.Apidog will use the OpenAPI Generator engine to generate the Fetch client code for your API, matching the endpoints and data structures that you defined or selected.
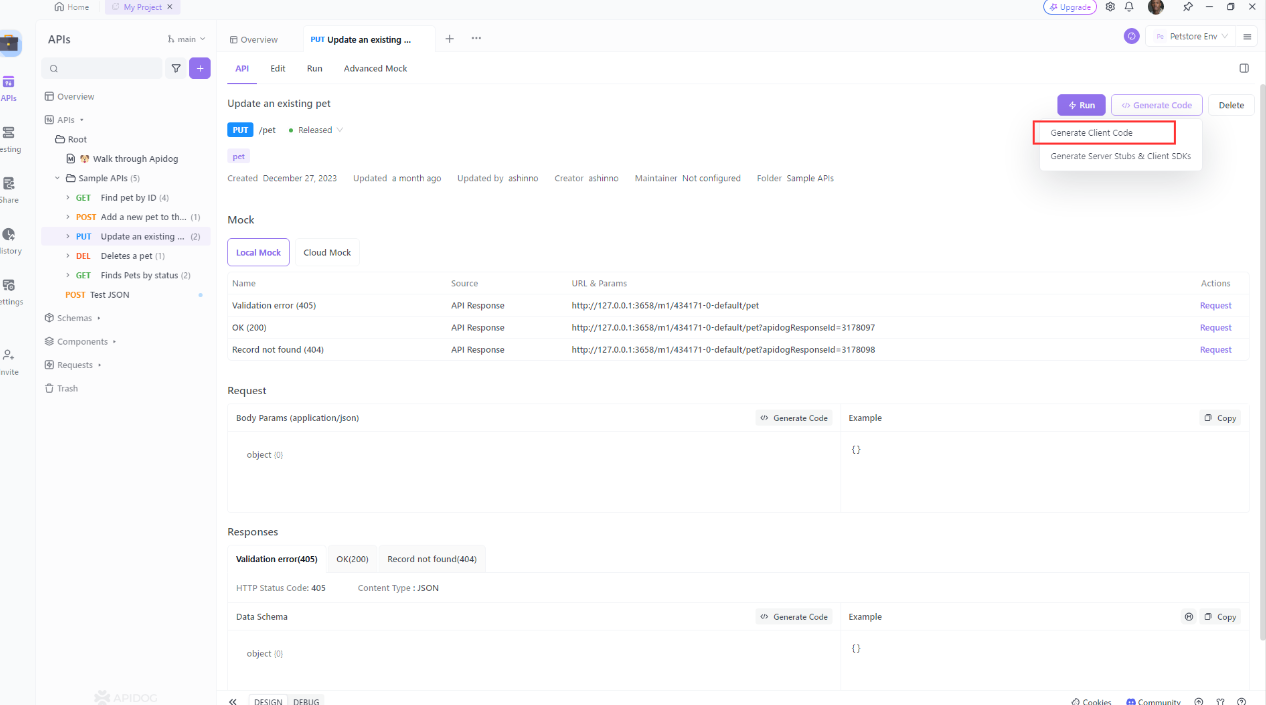
- You will see a modal window with the generated code, which you can copy to your clipboard.
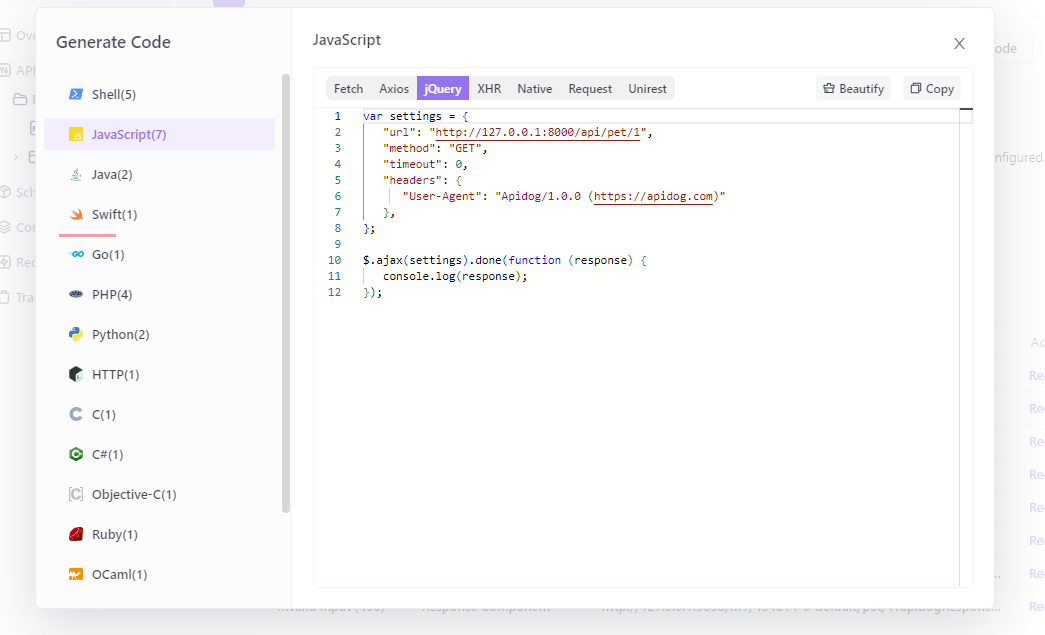
You can use the generated code in your own project, or modify it as you wish. You can also test the code with the Apidog mock server, which simulates the API responses based on your specification.
Conclusion
ReactJS Query is a powerful and versatile library that can make our data fetching experience much easier and better. It can work with any API endpoint that returns JSON data, and it can integrate with any React component or library.
In this blog post, we have learned how to use ReactJS Query to fetch data from APIs and display it on our website. We have seen how ReactJS Query simplifies data fetching and caching for React applications, and how to generate ReactJS Query code Apidog. Thank you for reading and happy coding!