Python Requests: How to POST JSON Data with Ease in 2024
Learn how to use Python Requests to POST JSON data with ease in 2024. Our step-by-step guide will help you get started with this powerful library and make your API calls more efficient and effective. Read on to find out more!
Are you looking to send JSON data to a server using Python Requests? If so, you’re in the right place! In this blog post, we’ll go over how to use Python Requests to POST JSON data. We’ll cover everything from the basics of sending a POST request to handling the response received from the server, and even handling errors that may occur along the way. So, let’s get started!
Python Requests
Python Requests is a Python 3.7+ library that simplifies the process of sending HTTP/1.1 requests. It provides a simple API for interacting with HTTP methods such as GET, POST, PUT, PATCH and more.
Python Requests: POST HTTP Method
The following code snippet demonstrates how to use the requests
library to send an HTTP POST request:
import requests
url = 'https://www.example.com/api'
data = {'username': 'my_username', 'password': 'my_password'}
response = requests.post(url, data=data)
print(response.status_code)
print(response.content)
In this example, we are sending an HTTP POST request to https://www.example.com/api
with a JSON payload containing a username and password. The requests.post()
method is used to send the request, and the response status code and content are printed to the console.
You can install the requests
library using the following command:
python -m pip install requests
In this blog post, we’ll be focusing on how to use Python Requests to send a POST request with JSON data.
What is JSON?
JSON stands for JavaScript Object Notation. It's a standardized format for representing structured data. It is a text-based format that uses human-readable text to store and transmit data objects consisting of attribute-value pairs and arrays (or other serializable values). JSON is commonly used for transmitting data in web applications, such as sending data from the server to the client, or vice versa.
JSON can represent six types of data natively: strings, numbers, booleans, null, arrays, and objects. For example, here is a JSON representation of a blog post:
{
"id": 1001,
"title": "What is JSON?",
"author": {
"id": 1,
"name": "James Walker"
},
"tags": [
"api",
"json",
"programming"
],
"published": false,
"publishedTimestamp": null
}
This example demonstrates all the JSON data types. It also illustrates the concision of JSON-formatted data, one of the characteristics that’s made it so appealing for use in APIs.
In other terms, it’s a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. JSON is a text format that is completely language-independent, making it an ideal choice for data exchange between different programming languages.
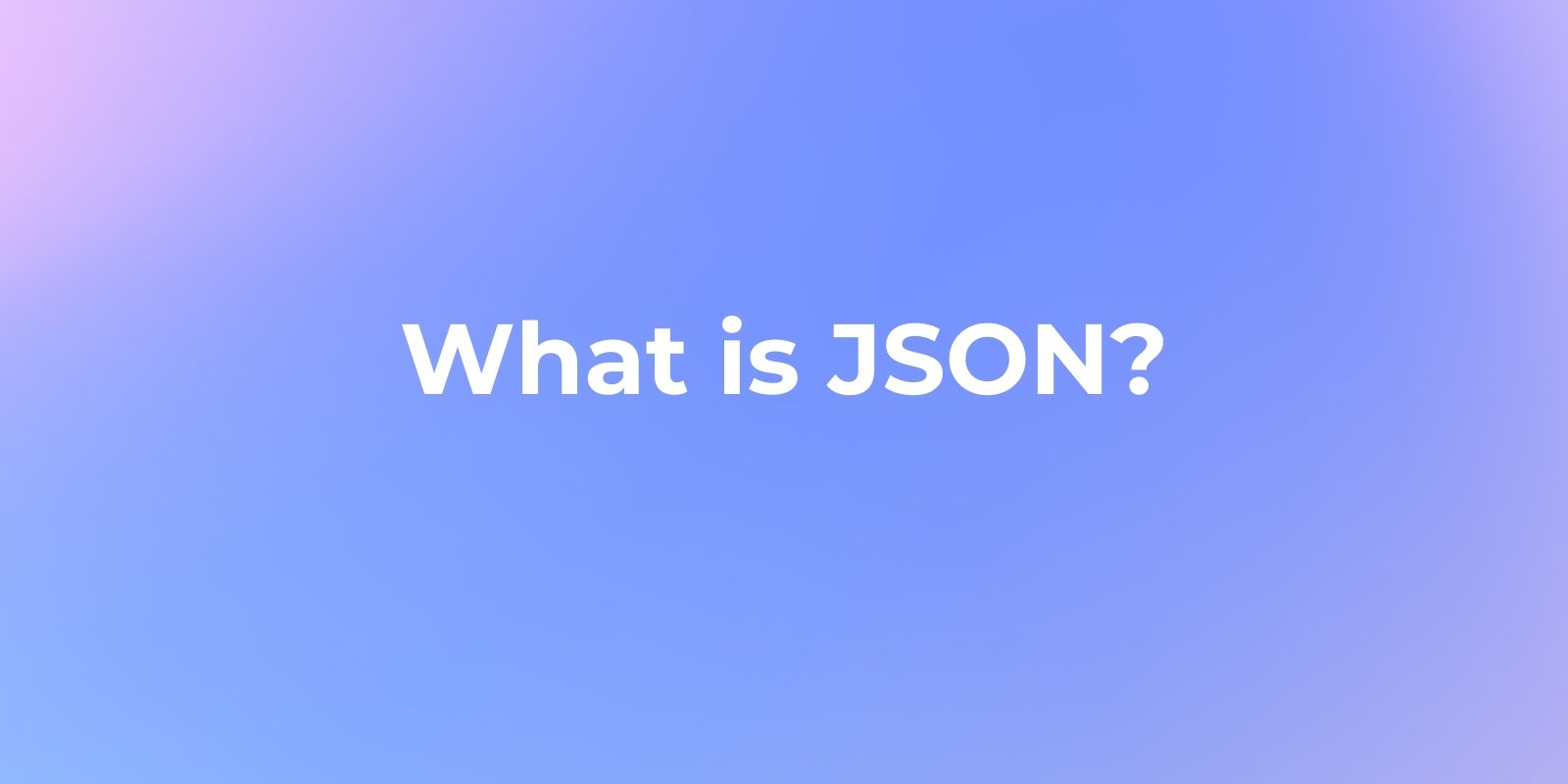
Understand the POST request method
A POST request is a type of HTTP request method that is used to send data to a server to create or update a resource. It is often used when uploading a file or when submitting a completed web form. The POST request method requests that a web server accepts the data enclosed in the body of the request message, most likely for storing it.
JSON's standardized format for representing objects and data makes it a popular choice for sending data in POST requests. By using JSON, developers can ensure that the data sent to the server is well-organized and easily understandable, enhancing the efficiency of resource creation and updates.
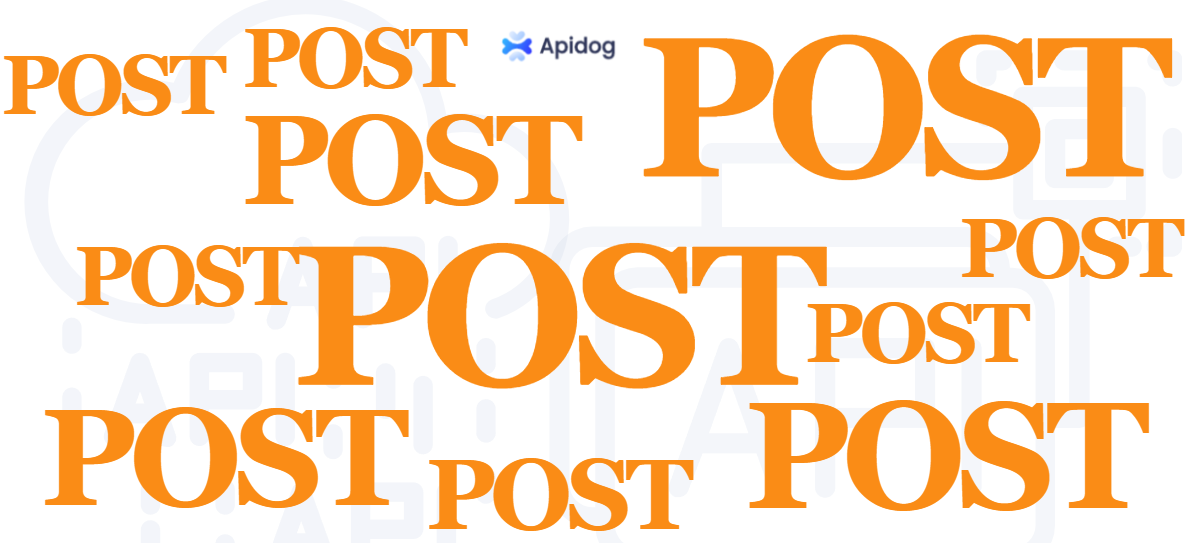
In contrast to the HTTP GET request method, which retrieves information from the server, the POST request method requests that a web server accept the data enclosed in the body of the request message, most likely for storing it. An arbitrary amount of data of any type can be sent to the server in the body of the request message.
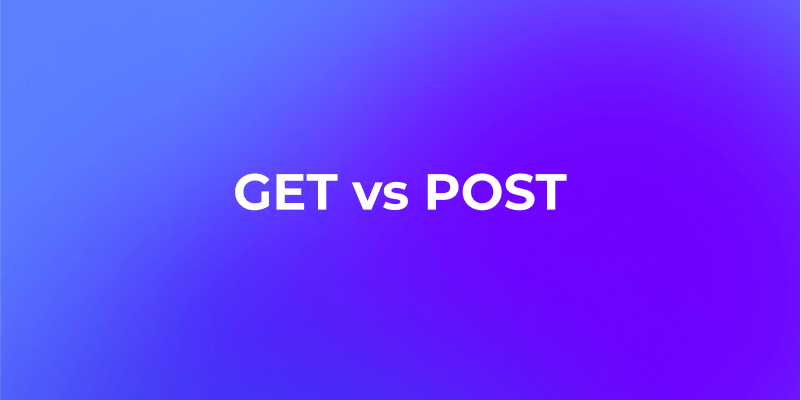
Here’s an example of how to use the requests
library to send an HTTP POST request:
import requests
url = 'https://www.example.com/api'
data = {'username': 'my_username', 'password': 'my_password'}
response = requests.post(url, data=data)
print(response.status_code)
print(response.content)
In this example, we are sending an HTTP POST request to https://www.example.com/api
with a JSON payload containing a username and password. The requests.post()
method is used to send the request, and the response status code and content are printed to the console.
How to Use Python Requests to POST JSON Data?
Now that we have a basic understanding of what Python Requests and JSON are, let’s dive into how to use Python Requests to POST JSON data. Here’s an example of how to do it:
import requests
import json
url = 'https://www.example.com/api'
data = {'username': 'my_username', 'password': 'my_password'}
headers = {'Content-type': 'application/json'}
response = requests.post(url, data=json.dumps(data), headers=headers)
print(response.status_code)
print(response.content)
In this example, we are sending an HTTP POST request to https://www.example.com/api
with a JSON payload containing a username and password. The requests.post()
method is used to send the request, and the response status code and content are printed to the console. Note that we are using the json.dumps()
method to convert the data
dictionary to a JSON string before sending it in the request body.
You can install the requests
library using the following command:
python -m pip install requests
Handling the Response
After sending the POST request, we’ll receive a response from the server. We can handle the response using the response
object that we created earlier. Here’s an example of how to handle the response:
import requests
url = 'https://www.example.com/api'
data = {'username': 'my_username', 'password': 'my_password'}
headers = {'Content-type': 'application/json'}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 200:
print('Success!')
else:
print('An error occurred.')
In this example, we’re checking the status code of the response to see if the request was successful. If the status code is 200, we’ll print “Success!”. Otherwise, we’ll print “An error occurred.”.
Handling Errors
Sometimes, things don’t go as planned. In the event that an error occurs while sending the POST request, we’ll want to handle it gracefully. Here’s an example of how to handle errors:
import requests
url = 'https://www.example.com/api'
data = {'username': 'my_username', 'password': 'my_password'}
headers = {'Content-type': 'application/json'}
try:
response = requests.post(url, json=data, headers=headers)
response.raise_for_status()
except requests.exceptions.HTTPError as err:
print(err)
In this example, we’re using a try
/except
block to catch any errors that may occur while sending the POST request. If an error occurs, we’ll print the error message.
How to Send Python POST Requests with JSON Data in Apidog?
Apidog is a powerful testing solution that provides developers with a more robust interface testing experience. Its advanced features like creating custom test cases, generating reports, and load testing empower developers with greater flexibility and capabilities within their testing workflows. Compared to Postman, Apidog stands out as a more comprehensive and powerful testing solution.
To send a POST request with JSON data in Apidog, follow these steps:
Step 1: Open Apidog and create a new request.
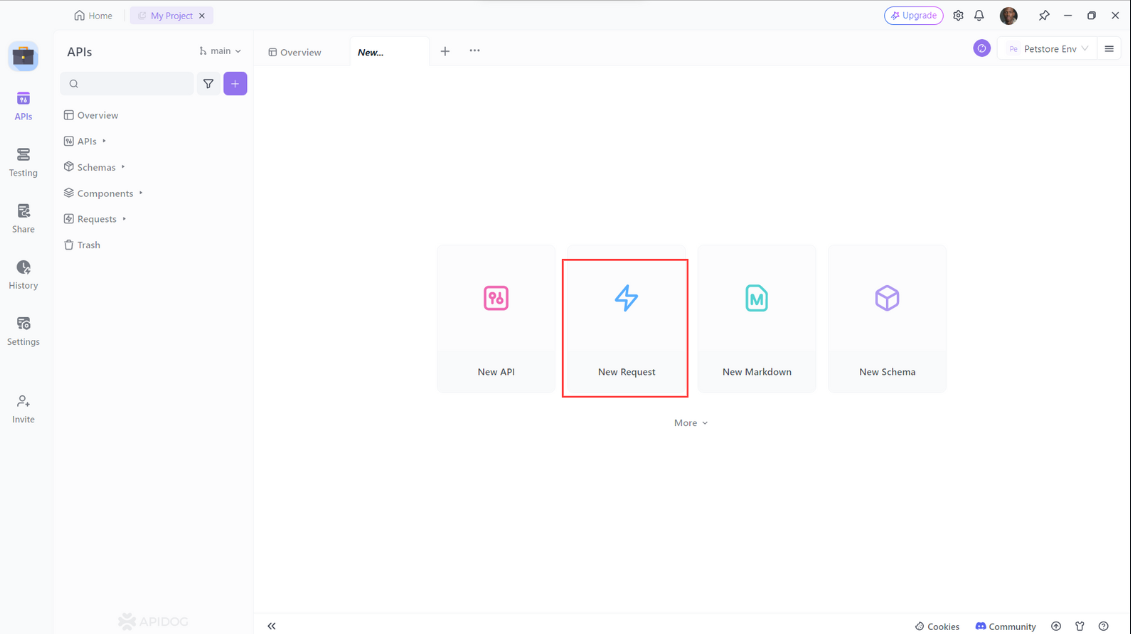
Step 2: Click on the Request tab and select POST from the dropdown menu.
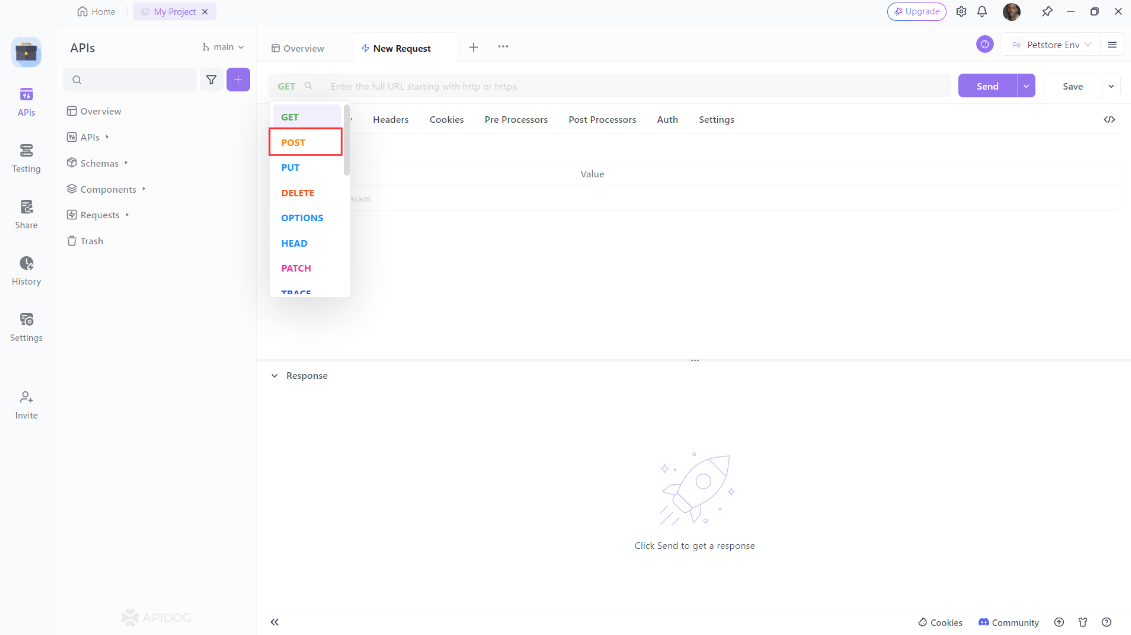
Step 3: Enter the URL of the API endpoint you want to test, in the Headers section, add any required headers. In the Body section, select JSON from the dropdown menu and enter the JSON data you want to send in the request body.
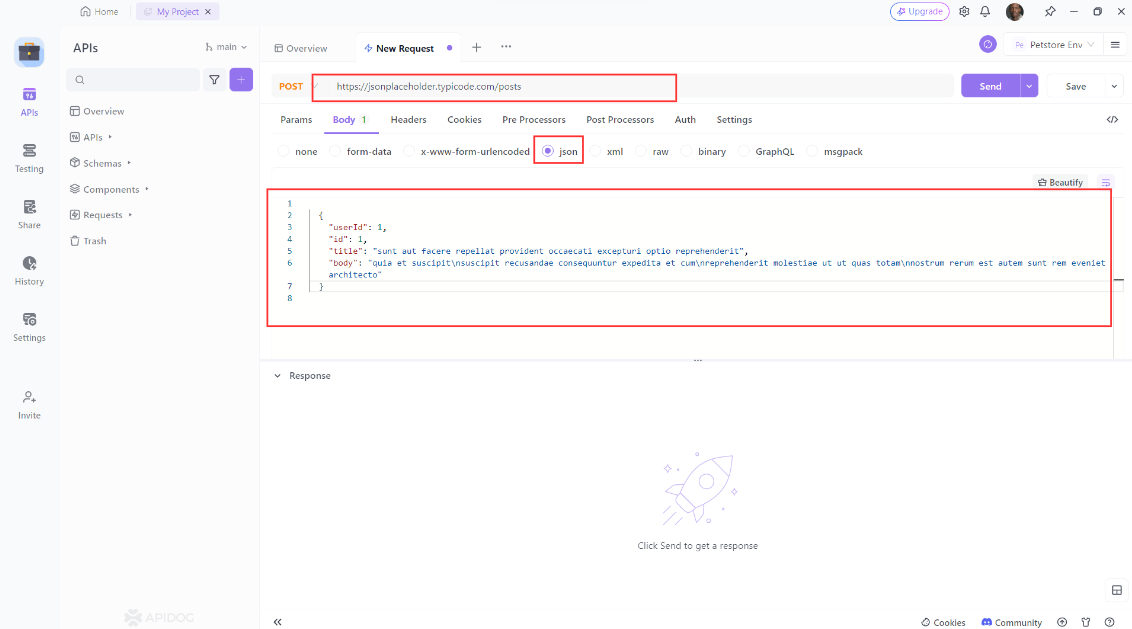
Step 4: Click on the Send button to send the request and check the response.
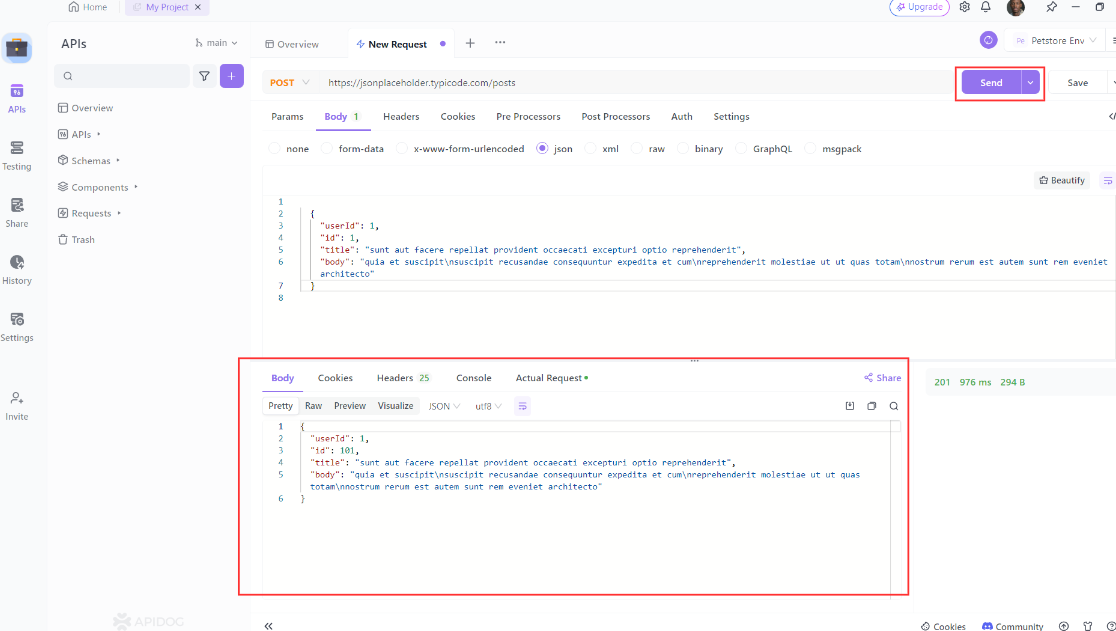
That’s it! You have successfully sent a Python POST request with JSON data in Apidog.
Conclusion
In this blog post, we’ve covered how to use Python Requests to POST JSON data. We’ve gone over the basics of sending a POST request, handling the response, and handling errors. We hope that this blog post has been helpful to you, and that you’re now able to use Python Requests to POST JSON data with ease!