How to send POST Request in python?
Learn how to send POST requests in Python using the requests library. This guide covers everything from setting up your environment to following best practices for secure and effective API communication.
In this blog post, we will explore the basics of POST requests, how to make them using Python Requests, and some best practices to keep in mind. Whether you’re a seasoned developer or just starting out, this guide will provide you with everything you need to know about POST requests.
HTTP Request Basics
Before we dive into the POST request, let’s first understand what an HTTP request is. HTTP stands for Hypertext Transfer Protocol, which is a protocol used to transfer data over the internet. An HTTP request is a message sent by a client to a server, requesting for a specific resource. The server then responds with the requested resource.
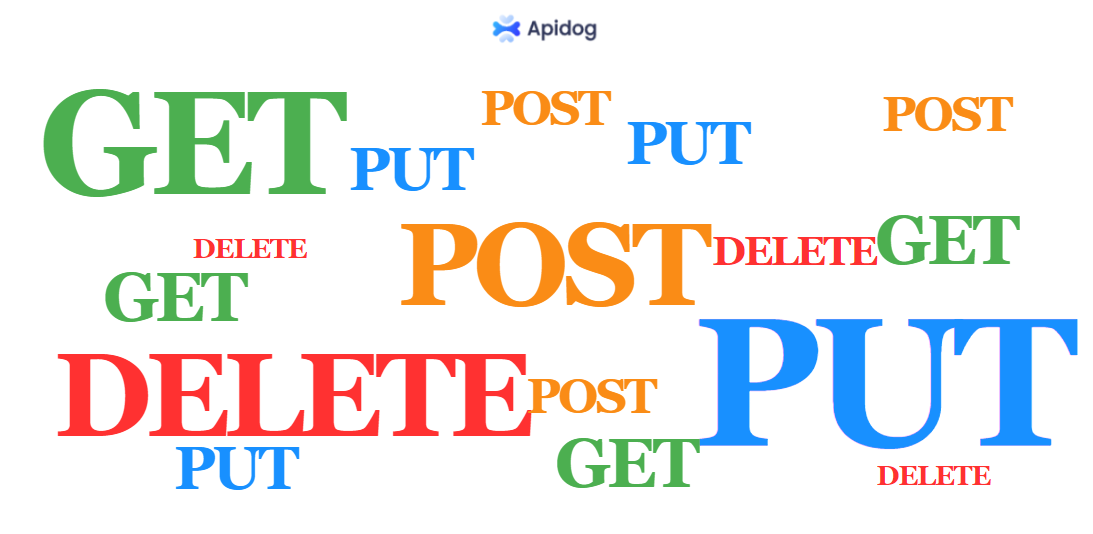
There are various HTTP methods, also referred to as HTTP requests, each serving a distinct purpose and conveying the nature of the request. The most prevalent HTTP methods include GET, POST, PUT and DELETE.
What is a POST request?
A POST request is a method used in the Hypertext Transfer Protocol (HTTP) to send data to a server to create or update a resource. The data is included in the body of the request, rather than in the URL as with a GET request. This method is commonly used for submitting form data or uploading a file.
What is Python?
Now that we've covered the basics of HTTP requests, let's talk about the programming language that's going to be our trusty companion on this journey – Python. Python is renowned for its simplicity, readability, and versatility. It's a high-level language that enables developers to write clear, logical code for projects of all sizes. The latest version of Python can be obtained by visiting the official website and downloading it from there.
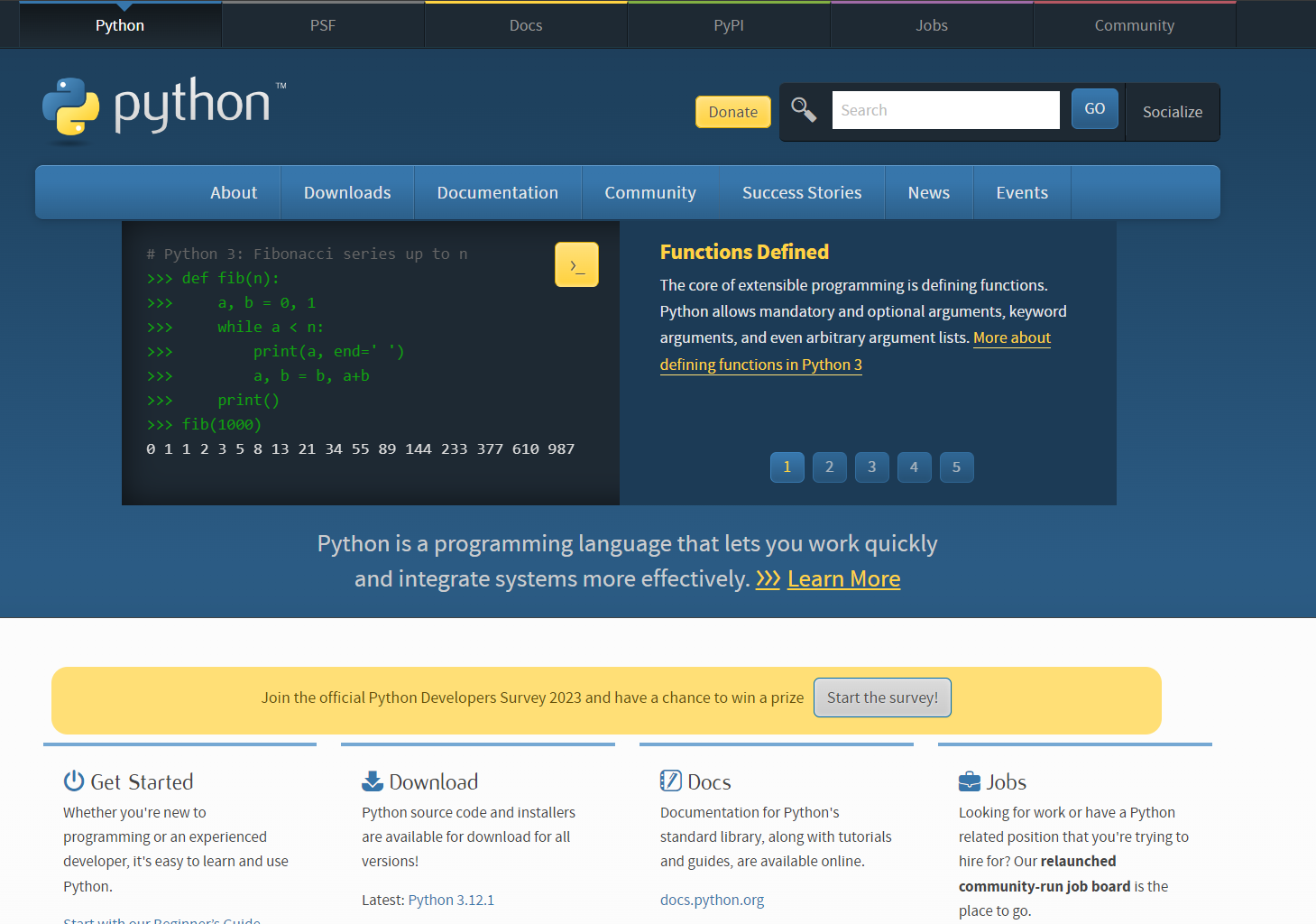
Python's extensive libraries make it a favorite among developers, and its syntax ensures that even beginners can grasp its concepts quickly.
How to make a POST request using Python
To make a POST request using Python, you can use the requests
library, which is a popular HTTP library for Python. Here’s an example of how you can make a POST request:
import requests
# Define the URL and the data to be sent in the POST request
url = 'http://example.com/test/demo_form.php'
data = {'name1': 'value1', 'name2': 'value2'}
# Make the POST request
response = requests.post(url, data=data)
# Print the status code and the response content
print(f"Status Code: {response.status_code}")
print(f"Response Content: {response.text}")
In this code snippet, we’re sending a POST request to http://example.com/test/demo_form.php
with two pieces of data: name1
with a value of value1
, and name2
with a value of value2
. The requests.post
method is used to send the POST request, and then we print out the status code and response content from the server.
Please note that to run this code, you’ll need to have the requests
library installed. You can install it using pip:
pip install requests
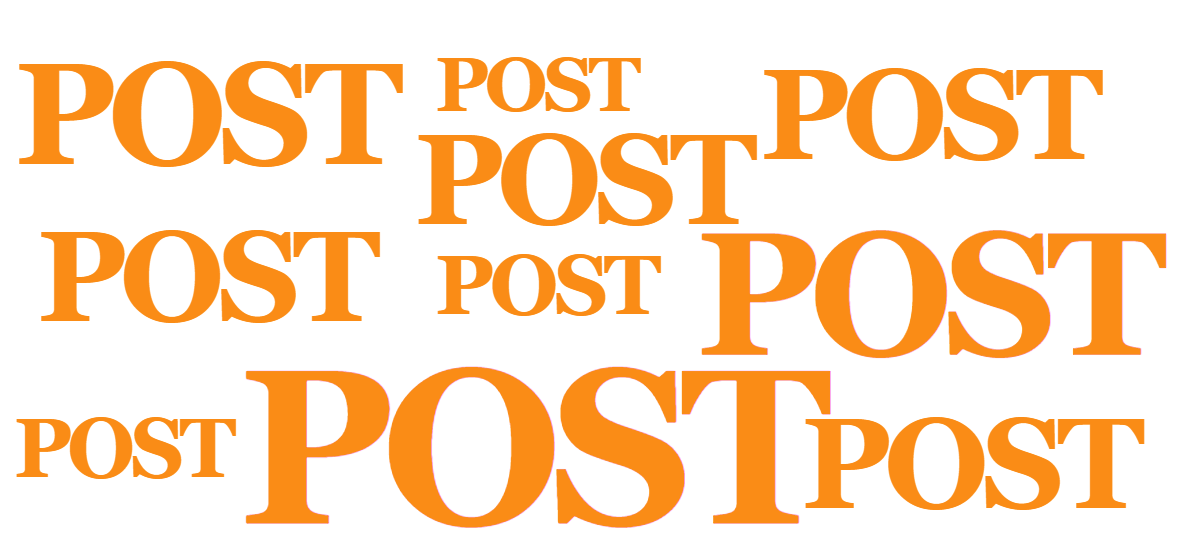
Understanding the POST request parameters in Python.
In Python, when making a POST request using the requests
library, you typically deal with the following parameters:
url
: The URL to which the POST request is sent.data
: A dictionary, list of tuples, bytes, or a file object that you want to send in the body of the request.json
: A JSON object to send in the body of the request.
Here’s a breakdown of how you can use these parameters:
import requests
# The URL for the POST request
url = 'http://example.com/api'
# Data to be sent in the body of the POST request
data = {
'key1': 'value1',
'key2': 'value2'
}
# Making the POST request
response = requests.post(url, data=data)
# Checking the response
print(response.text)
In this example, data
is a dictionary containing the data to be sent to the server. If you’re sending JSON data, you can use the json
parameter instead, which automatically sets the Content-Type
header to application/json
.
Additionally, the requests.post
function can accept several other keyword arguments (**kwargs
) such as:
headers
: A dictionary of HTTP headers to send with the request.cookies
: A dictionary of cookies to send with the request.files
: A dictionary of files to send with the request.auth
: A tuple to enable HTTP authentication.timeout
: How long to wait for the server to send data before giving up.allow_redirects
: Boolean. Set to True if POST redirection is allowed.
These parameters allow you to customize the POST request to fit the requirements of the server you’re interacting with.
Using Apidog to Test Your Python POST Request
Apidog is a powerful tool for testing APIs. It allows you to create and save API requests, organize them into collections, and share them with your team.
Here is how you can use Apidog to test your POST request:
- Open Apidog and create a new request.
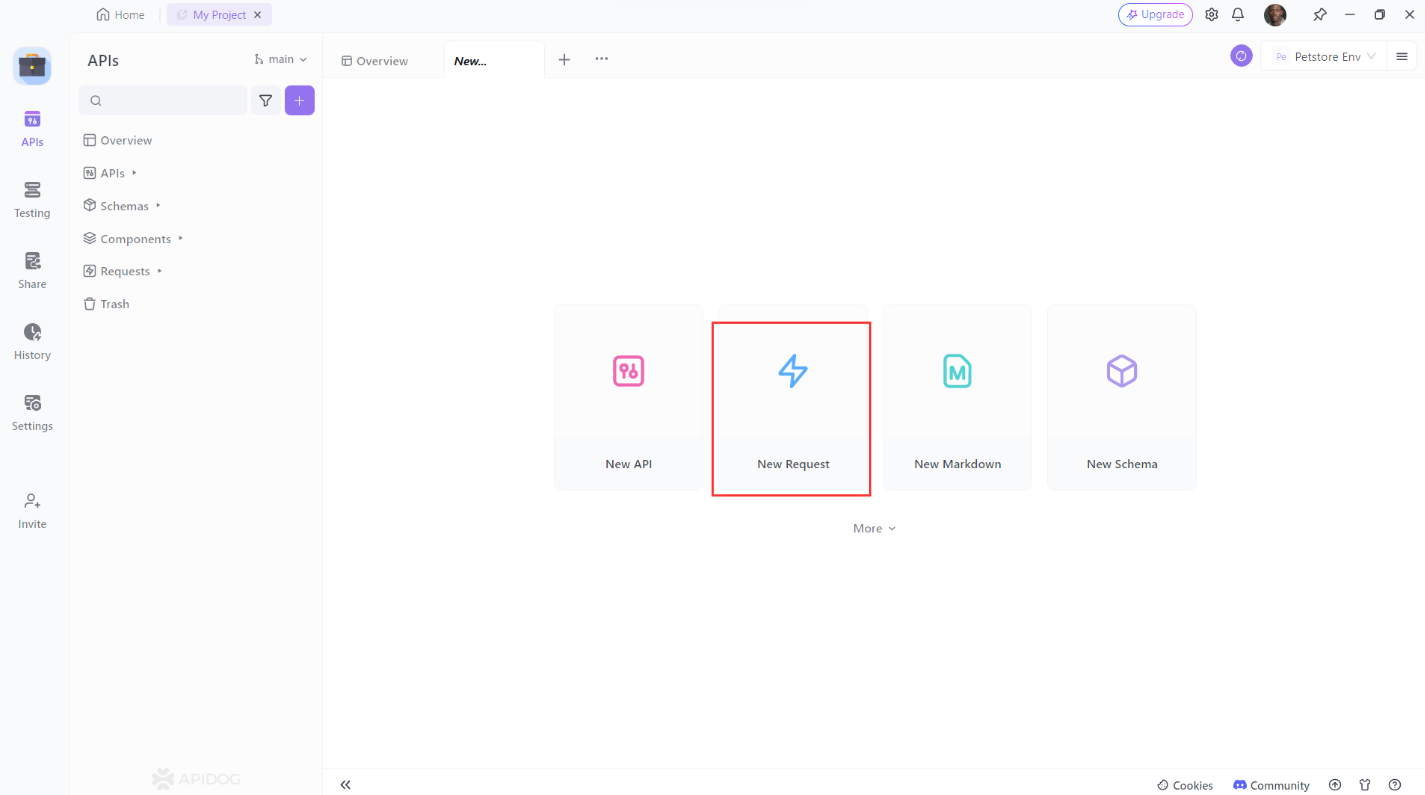
2. Set the request method to POST.
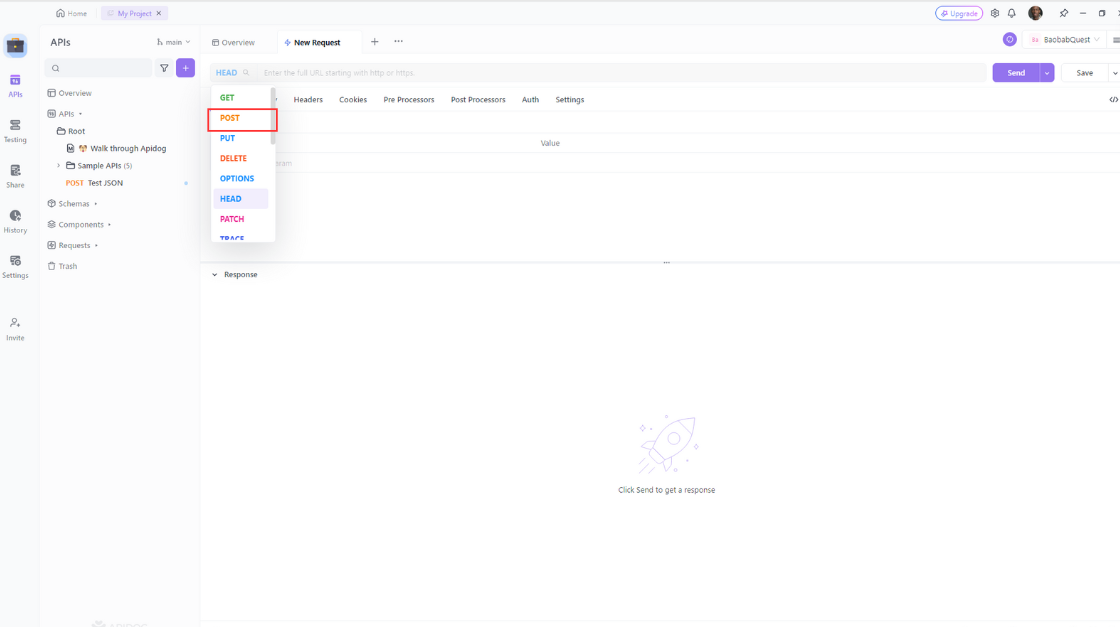
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
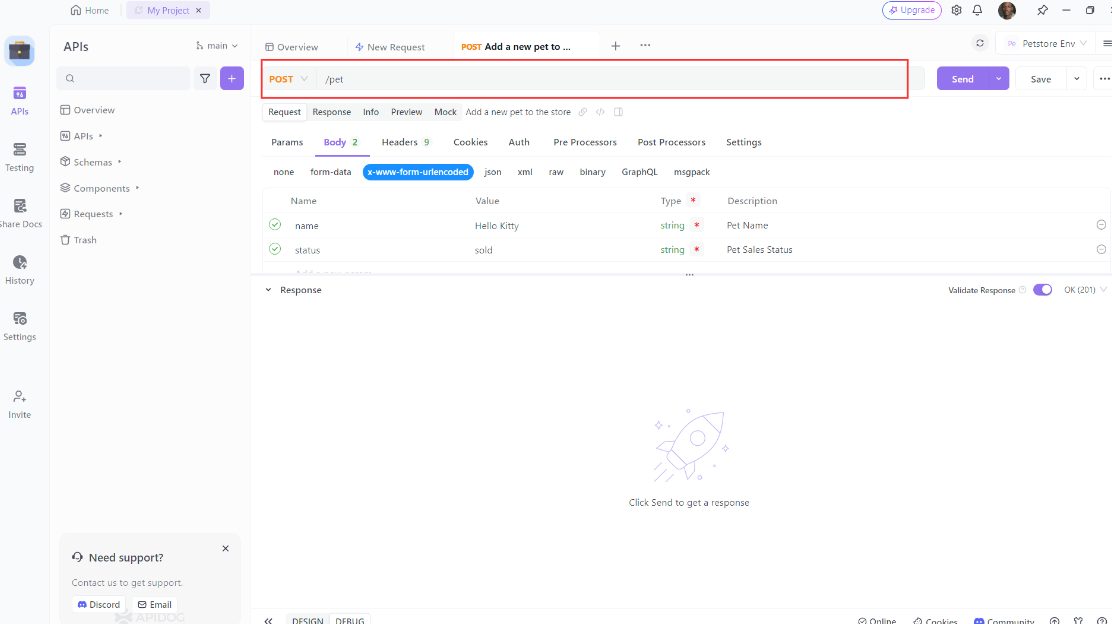
4. Verify that the response is what you expected.
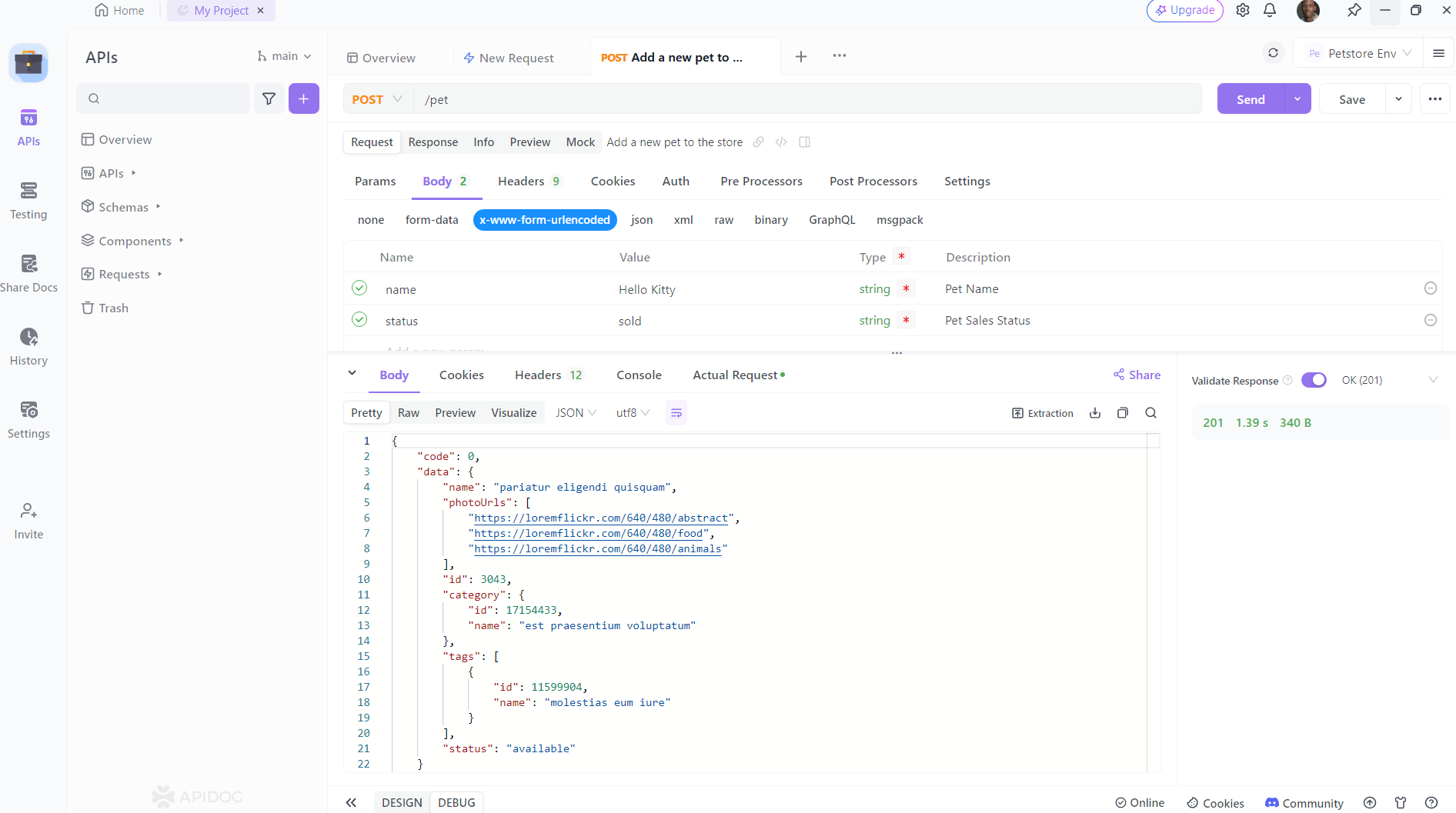
Best practices for making a POST Request.
When making a POST request, it’s important to follow best practices to ensure that your API is secure, efficient, and easy to use. Here are some key best practices:
Use JSON for Data Transfer: JSON is the standard for transferring data. It’s widely supported and easy to use with most frameworks.
Secure Your API: Use HTTPS to encrypt data in transit. Implement authentication and authorization strategies to protect sensitive information.
Handle Errors Gracefully: Return standard HTTP status codes and provide clear error messages to help clients understand what went wrong.
Support Filtering, Sorting, and Pagination: These features improve the usability of your API by allowing clients to retrieve only the data they need.
Cache Data: Caching can significantly improve the performance of your API by reducing the load on your server and speeding up response times.
Version Your API: Maintain different versions of your API to ensure backward compatibility and to allow clients to transition to new versions at their own pace.
Validate Input: Always validate and sanitize input to protect against SQL injection and other types of attacks.
Document Your API: Provide clear documentation for your API endpoints, including the expected request and response formats, to make it easier for clients to integrate with your API.
Use Proper Status Codes: Use appropriate HTTP status codes to indicate the result of the request. For example, use 201 Created
for successful POST requests that result in creation.
Avoid Overloading Query Parameters: Use query parameters for non-sensitive metadata and send main request data and sensitive information in the request body.
These practices will help you create a robust and user-friendly API. If you need more detailed information or have specific questions, feel free to ask!
Conclusion
Sending a POST request in Python is a fundamental skill for any developer working with APIs. By leveraging the requests
library, you can efficiently interact with web services, submit form data, and handle server responses. Remember to follow best practices such as using JSON for data transfer, securing your API, testing your POST request using Apidog, and validating input to ensure robust and secure applications.
With these tools and techniques, you’re well-equipped to integrate Python into your web development projects and take full advantage of the power of HTTP communication.