How to make HTTP requests with Axios in 2024
Learn how to make HTTP requests like a pro with Axios. This tutorial covers everything you need to know about using Axios to make HTTP requests, including how to make an Axios POST request with axios.post().
Axios is a popular JavaScript library that simplifies the process of making HTTP requests from a web application. It provides an easy-to-use interface for sending and receiving data from APIs, and it’s widely used by developers around the world.
In this blog post, we’ll be discussing how to make HTTP requests with Axios, a popular JavaScript library for making HTTP requests, and provide code samples to demonstrate its usage, then we’ll provide some tips and tricks for generating your code using Apidog.
Apidog will simplify the process of generating and testing API, your development will be more efficient, so click the download button below to use Apidog for completely free.
Whether you’re a seasoned developer or just getting started with web development, this post will provide you with the knowledge you need to use Axios effectively and efficiently. So, let’s get started!
What is Axios?
Axios is a JavaScript library that allows you to make HTTP requests from your JavaScript code. It is a promise-based library that provides an easy-to-use interface for making HTTP requests. Axios is widely used in the JavaScript community and is known for its simplicity and flexibility. Axios is a client HTTP API based on the XMLHttpRequest
interface provided by browsers.
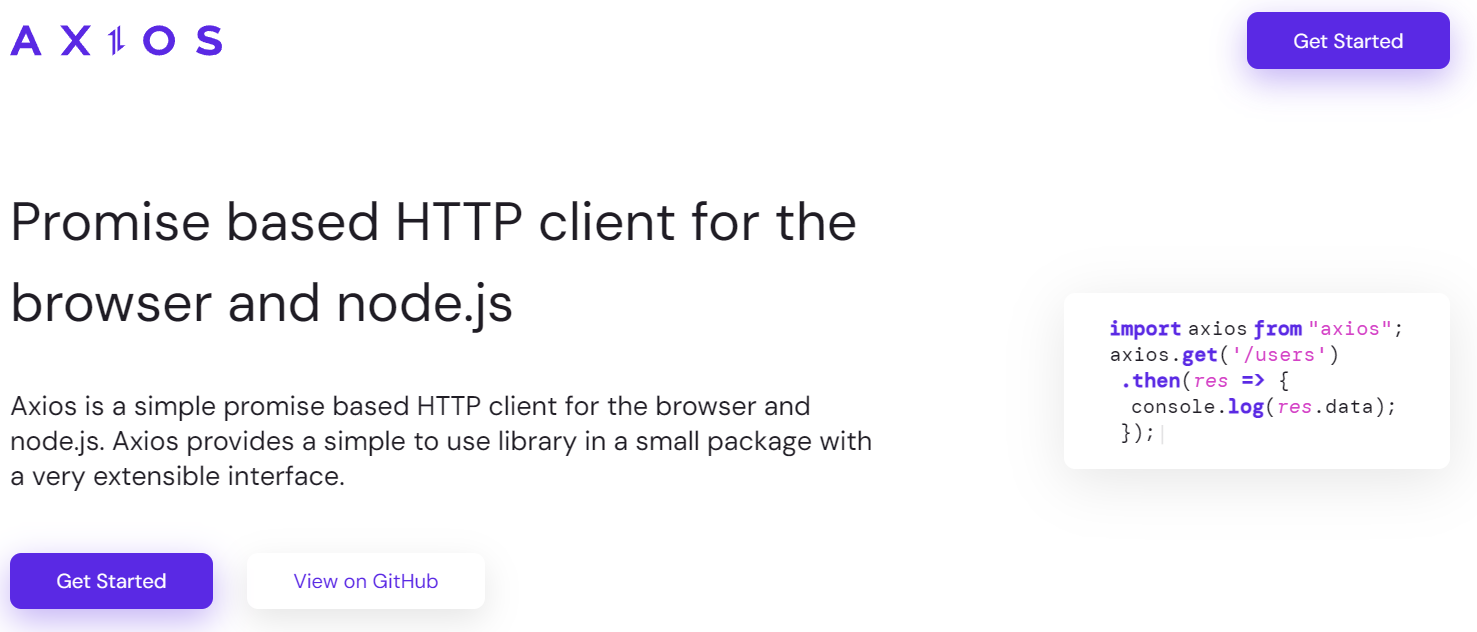
Why use Axios?
Axios provides several advantages over other HTTP libraries. Here are some reasons why you should use Axios:
- Promise-based: Axios is promise-based, which makes it easy to handle asynchronous operations. This means that you can use the
async/await
syntax to make your code more readable and easier to maintain. - Simple and intuitive interface: Axios provides a simple and intuitive interface for making HTTP requests. You can use the
axios.get()
,axios.post()
,axios.put()
, andaxios.delete()
methods to make GET, POST, PUT, and DELETE requests respectively. - Automatic JSON data transformation: Axios automatically transforms JSON data for you, making it easy to work with APIs that return JSON data.
- Support for older browsers: Axios provides several features that make it easy to work with older browsers. For example, it provides support for XMLHttpRequest, which is used by older browsers to make HTTP requests.
- Good defaults: Axios has good defaults to work with JSON data. Unlike alternatives such as the Fetch API, you often don’t need to set your headers or perform tedious tasks like converting your request body to a JSON string.
- Better error handling: Axios throws 400 and 500 range errors for you. Unlike the Fetch API, where you have to check the status code and throw the error yourself.
- Interceptors: Axios provides interceptors that allow you to intercept requests or responses before they are handled by
then
orcatch
.
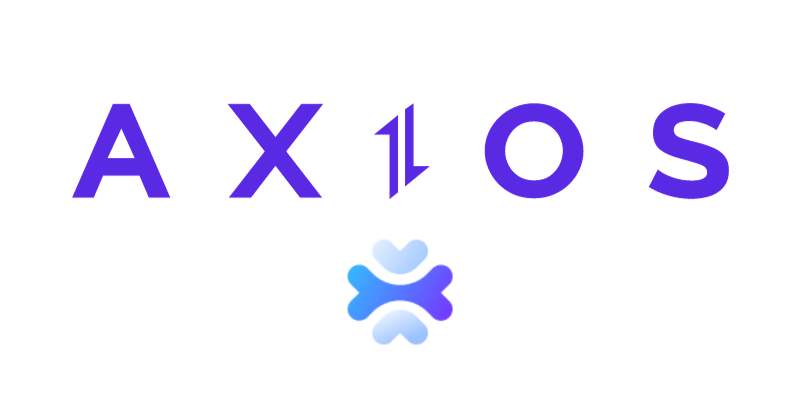
Installing Axios
To install Axios, you can use the Node.js package manager (npm) or yarn. Here’s how to install Axios using npm:
npm install axios
And here’s how to install Axios using yarn:
yarn add axios
If you prefer to use pnpm, you can install Axios using the following command:
pnpm install axios
Alternatively, you can use a content delivery network (CDN) to include Axios in your project. Here’s how to include Axios using a CDN:
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
Once you have installed Axios, you can start making HTTP requests with it. Axios provides a simple and intuitive interface for making HTTP requests. You can use the axios.get()
, axios.post()
, axios.put()
, and axios.delete()
methods to make GET, POST, PUT, and DELETE requests respectively.
Making HTTP requests with Axios
Now that we have Axios installed, let’s take a look at how to make HTTP requests with Axios. Axios provides several methods for making HTTP requests, including GET, POST, PUT, and DELETE.
How to make a GET request with Axios
Sending an HTTP request with Axios is straightforward; you simply pass a configuration object to the axios function. To send a GET request with Axios, you can use the axios.get()
method. Here is an example of how to use it:
axios.get('https://your-api-endpoint.com')
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
In this example, axios.get()
is used to send a GET request to the specified API endpoint. The .then()
method is used to handle the response from the server, while the .catch()
method is used to handle any errors that may occur during the request.
If you need to pass parameters with your GET request, you can do so by appending them to the URL. For example:
axios.get('https://your-api-endpoint.com?param1=value1¶m2=value2')
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
Here, param1
and param2
are the names of the parameters being passed, while value1
and value2
are their respective values.
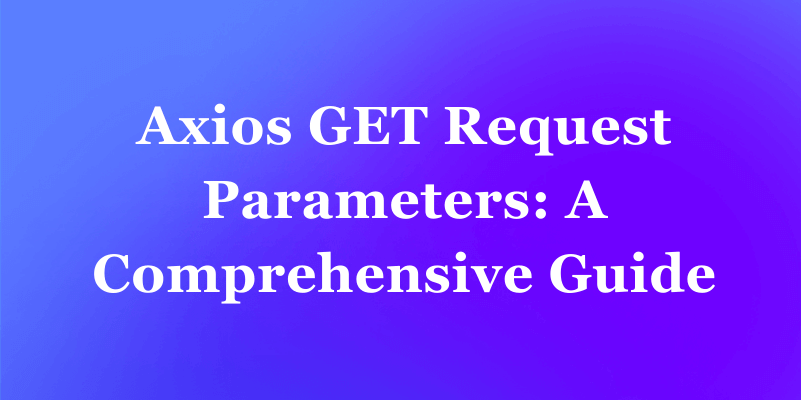
Sending a POST request with Axios
When you use Axios to make a POST request, you can "post" data to a specific endpoint and trigger events. To make a POST request with Axios, you can use the axios.post()
method. Here’s an example:
axios.post('https://api.example.com/data', {
firstName: 'John',
lastName: 'Doe'
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
In this example, we’re sending a POST request to https://api.example.com/data
. We’re also sending some data along with the request. When the request is complete, we’re logging the response data to the console. If there’s an error, we’re logging the error to the console.
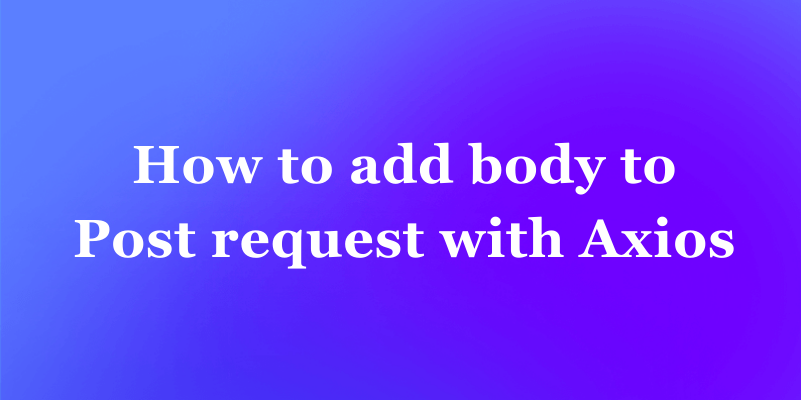
Making a PUT request with Axios
To make a PUT request with Axios, you can use the axios.put()
method. Here is an example of how to use it:
axios.put('/api/article/123', {
title: 'Making PUT Requests with Axios',
status: 'published'
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
In this example, axios.put()
is used to send a PUT request to the specified API endpoint. The .then()
method is used to handle the response from the server, while the .catch()
method is used to handle any errors that may occur during the request.
If you need to pass parameters with your PUT request, you can do so by including them in the request body. For example:
axios.put('/api/article/123', {
title: 'Making PUT Requests with Axios',
status: 'published',
param1: 'value1',
param2: 'value2'
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
Here, param1
and param2
are the names of the parameters being passed, while value1
and value2
are their respective values.
How to send a DELETE request with Axios?
To send a DELETE request with Axios, you can use the axios.delete()
method. Here is an example of how to use it:
axios.delete('/api/article/123')
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
In this example, axios.delete()
is used to send a DELETE request to the specified API endpoint. The .then()
method is used to handle the response from the server, while the .catch()
method is used to handle any errors that may occur during the request.
If you need to pass parameters with your DELETE request, you can do so by including them in the request body. For example:
axios.delete('/api/article/123', {
data: {
param1: 'value1',
param2: 'value2'
}
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
Here, param1
and param2
are the names of the parameters being passed, while value1
and value2
are their respective values.
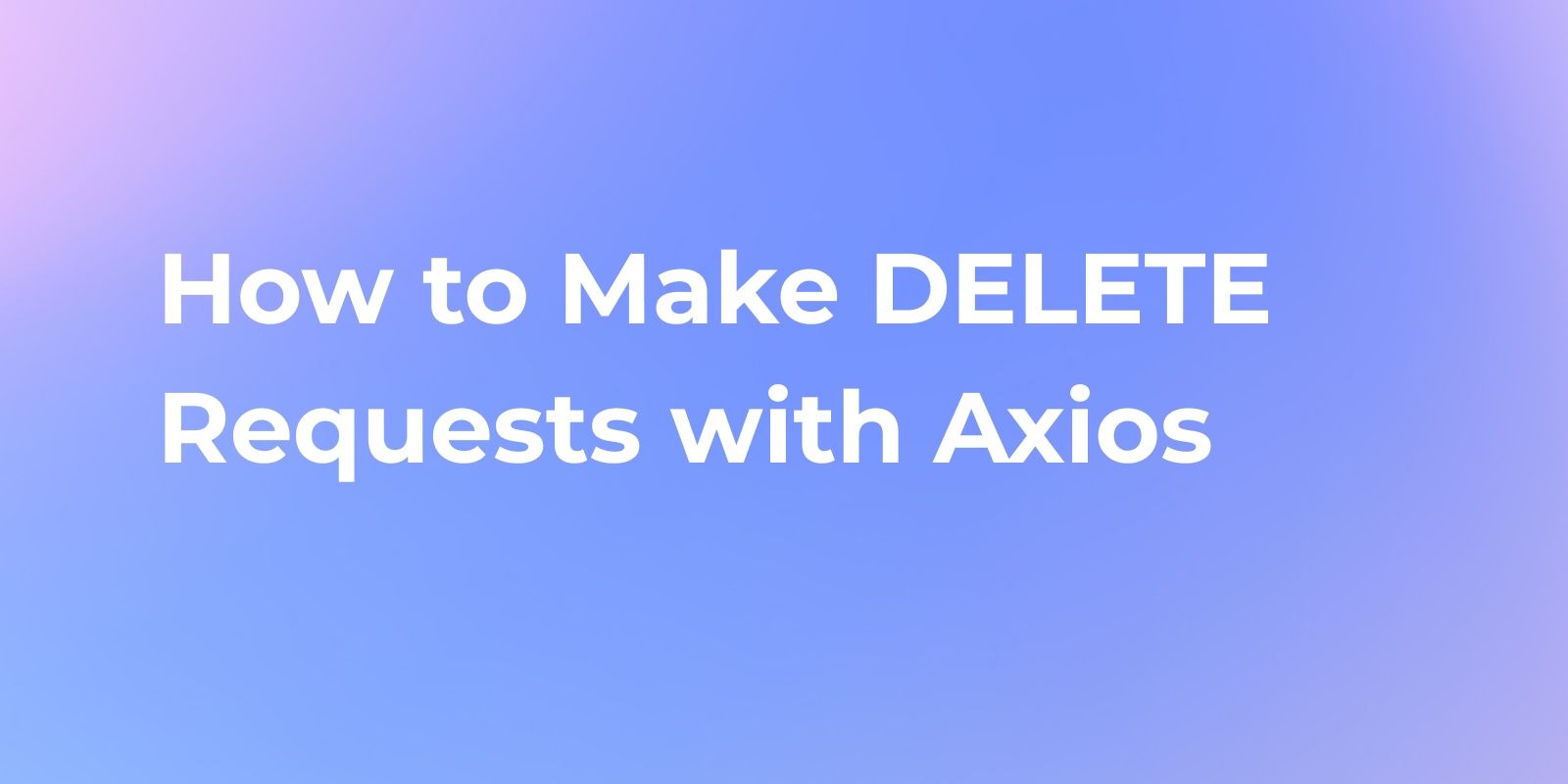
Using Axios with async and await
async
and await
are JavaScript keywords that allow you to write asynchronous code in a synchronous style. When using async
and await
with Axios, you can write cleaner and more readable code.
To use Axios with async
and await
, you can use the axios.get()
, axios.put()
, and axios.delete()
methods. Here are examples of how to use them:
async function getData() {
try {
const response = await axios.get('https://your-api-endpoint.com');
console.log(response);
} catch (error) {
console.error(error);
}
}
async function putData() {
try {
const response = await axios.put('/api/article/123', {
title: 'Making PUT Requests with Axios',
status: 'published'
});
console.log(response);
} catch (error) {
console.error(error);
}
}
async function deleteData() {
try {
const response = await axios.delete('/api/article/123', {
data: {
param1: 'value1',
param2: 'value2'
}
});
console.log(response);
} catch (error) {
console.error(error);
}
}
In these examples, axios.get()
, axios.put()
, and axios.delete()
are used to send GET, PUT, and DELETE requests to the specified API endpoints, respectively. The try
block is used to handle the response from the server, while the catch
block is used to handle any errors that may occur during the request.
If you need to pass parameters with your GET or DELETE request, you can do so by appending them to the URL or including them in the request body, respectively. For example:
async function getDataWithParams() {
try {
const response = await axios.get('https://your-api-endpoint.com?param1=value1¶m2=value2');
console.log(response);
} catch (error) {
console.error(error);
}
}
async function deleteDataWithParams() {
try {
const response = await axios.delete('/api/article/123', {
data: {
param1: 'value1',
param2: 'value2'
}
});
console.log(response);
} catch (error) {
console.error(error);
}
}
When you utilize the async and await syntax, it is customary to enclose your code within a try-catch block. This approach guarantees that you handle errors effectively and offer feedback for an improved user experience.
Apidog: a free tool to generate your Axios code
Apidog is an all-in-one collaborative API development platform that provides a comprehensive toolkit for designing, debugging, testing, publishing, and mocking APIs. Apidog enables you to automatically create Axios code for making HTTP requests.
Here's the process for using Apidog to generate Axios code:
Step 1: Open Apidog and select new request
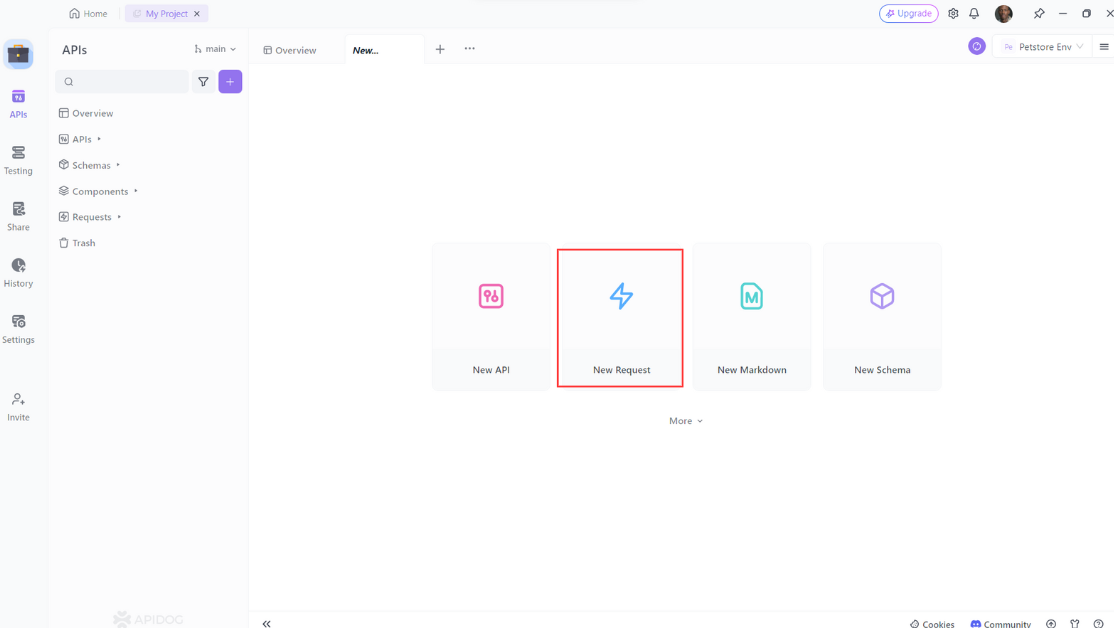
Step 2: Enter the URL of the API endpoint you want to send a request to,input any headers or query string parameters you wish to include with the request, then click on the "Design" to switch to the design interface of Apidog.
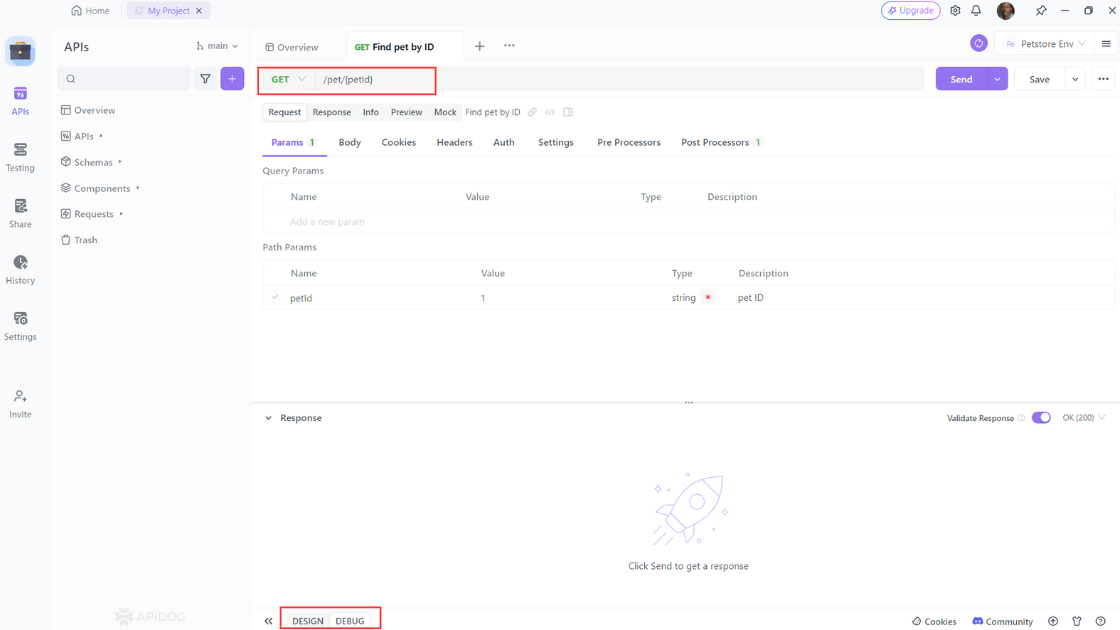
Step 3: Select "Generate client code " to generate your code.
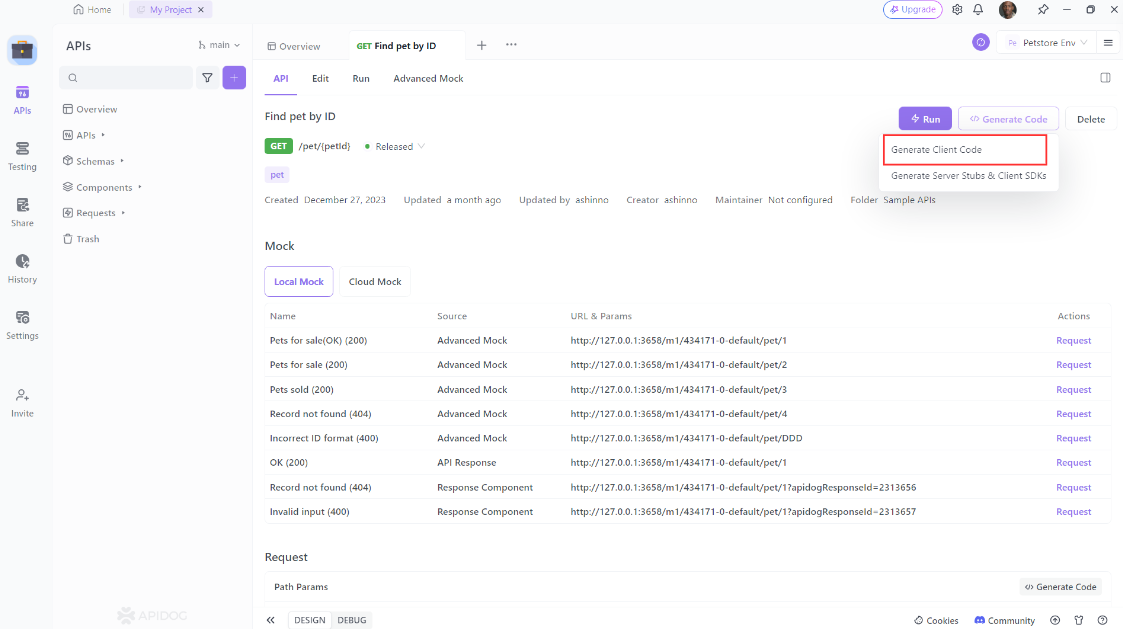
Step 4: Copy the generated Axios code and paste it into your project.
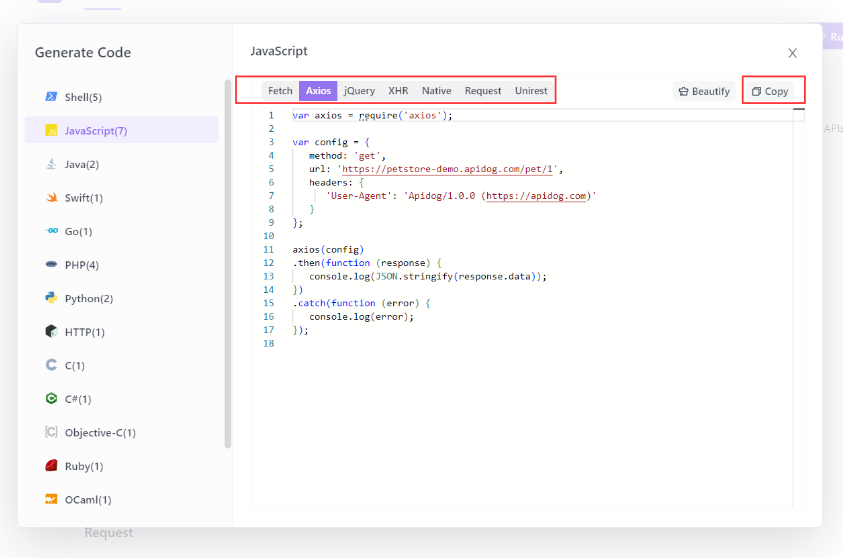
Using Apidog to Send HTTP Requests
Apidog offers several advanced features that further enhance its ability to test HTTP requests. These features allow you to customize your requests and handle more complex scenarios effortlessly.
Step 1: Open Apidog and create a new request.
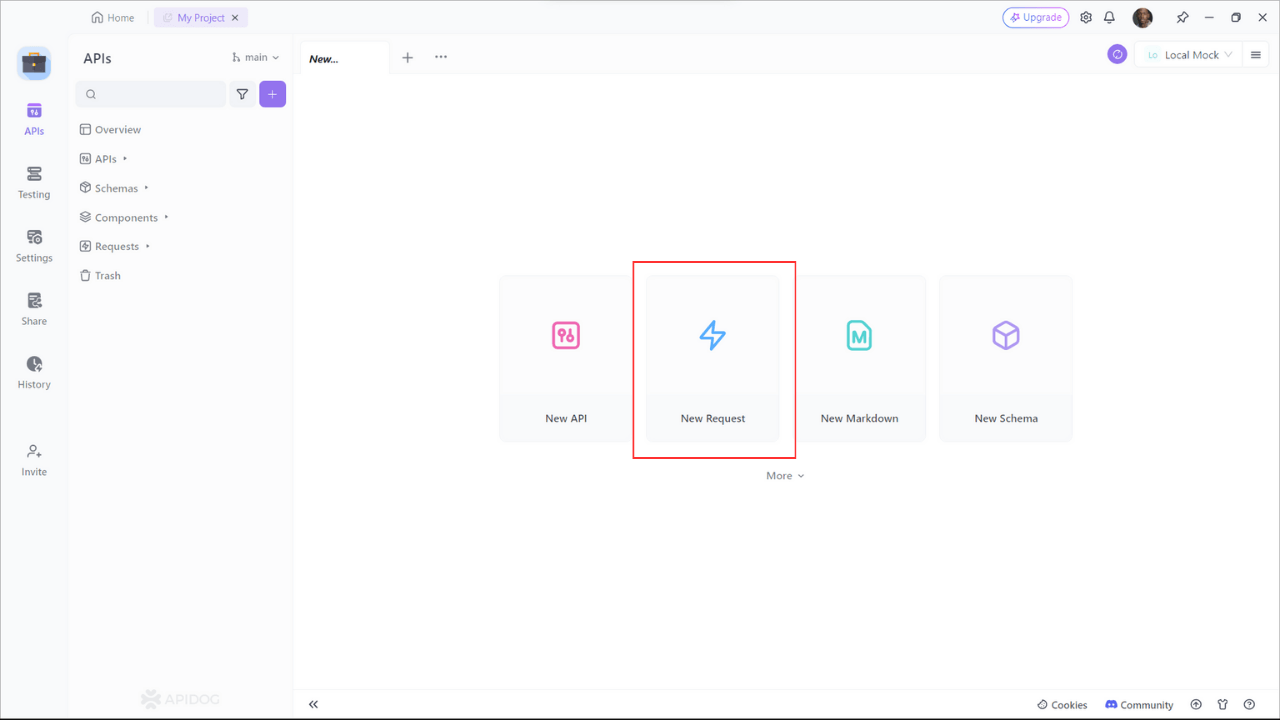
Step 2: Find or manually input the API details for the POST request you want to make.
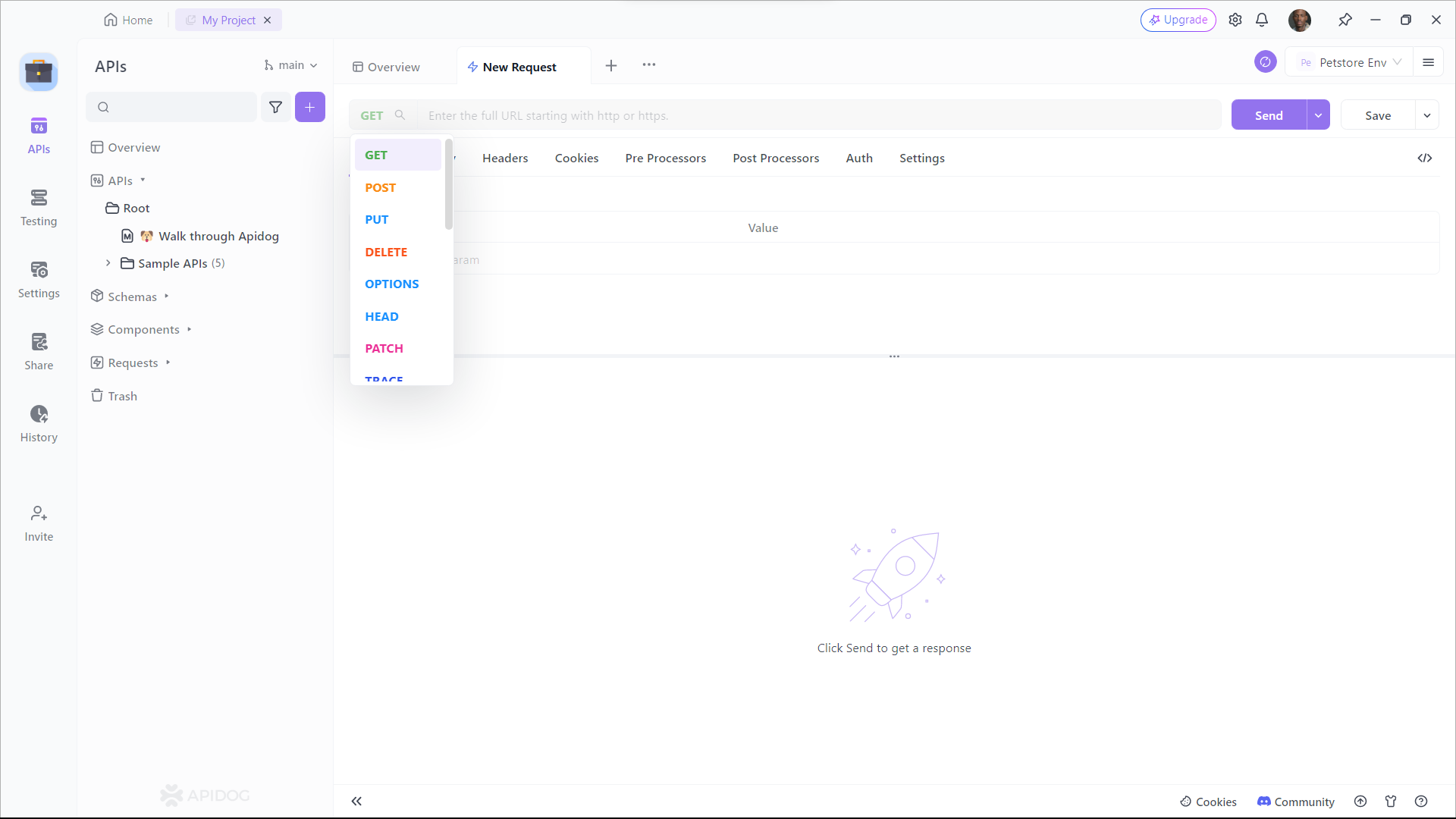
Step 3: Fill in the required parameters and any data you want to include in the request body.
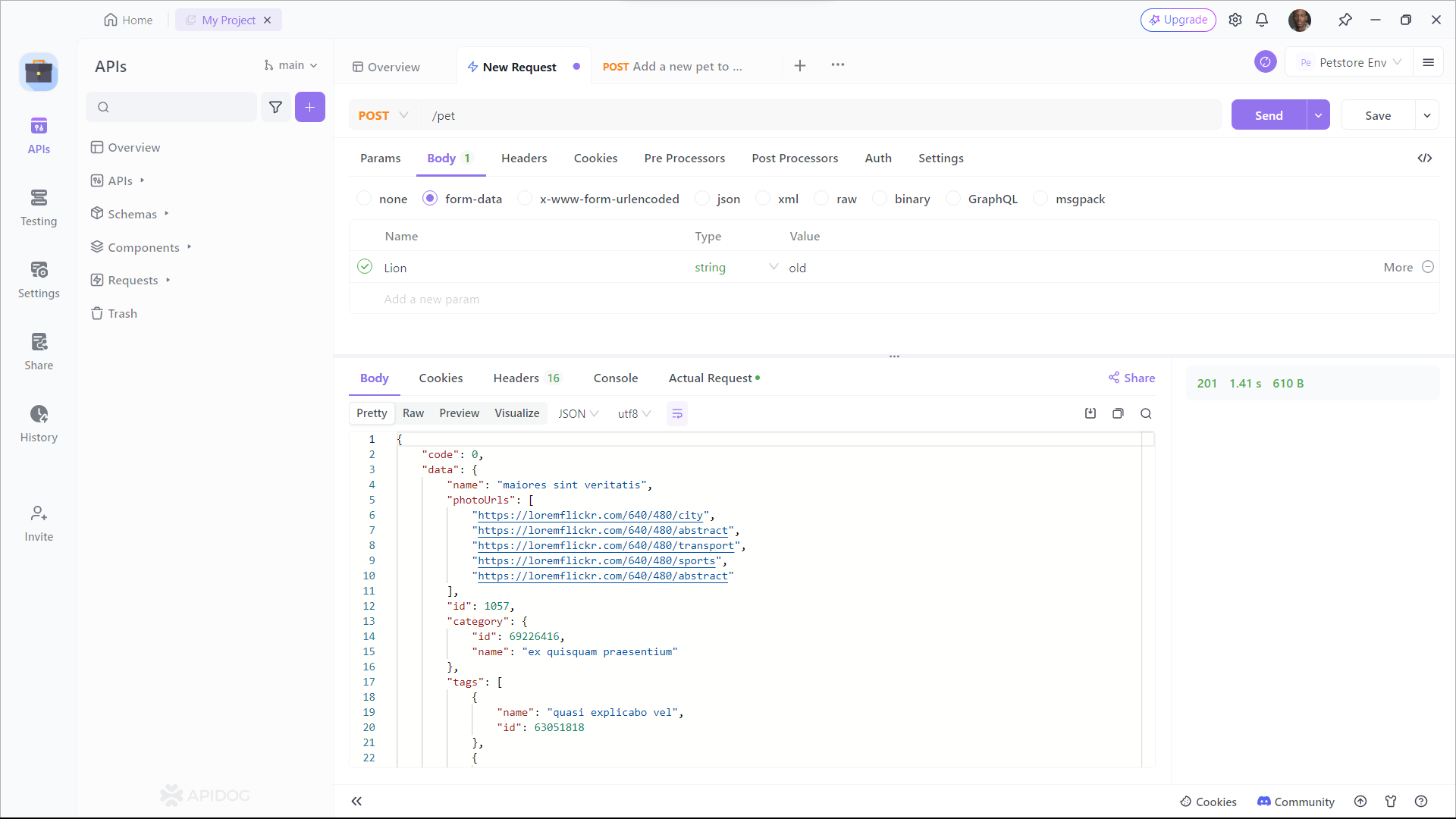
Conclusion
Axios is highly favored by developers for good reason. In this article, we've covered how to utilize Axios for making HTTP requests. We’ve covered the basics of Axios, including what it is, why you should use it, and how to install it.
By understanding how to make HTTP requests with Axios, you can enhance your web applications. With the help of Axios and tools like Apidog, you can streamline the process of making HTPP requests and focus on building robust and efficient applications.
Using Apidog not only saves you valuable time and effort but also ensures that your code is accurate and error-free. With its user-friendly interface and intuitive features, Apidog is a must-have tool for any developer working with Axios requests.