How to Create a REST API with Node.js and Express
Rest APIs provide a structured and scalable way for different software components to communicate over the Internet. Node.js, a server-side JavaScript runtime, and Express, a popular Node.js framework, make for a powerful combination to create RESTful APIs.
In today's interconnected world, building robust and scalable APIs has become a necessity for enabling seamless communication between diverse software components. REST APIs have emerged as the de facto standard for facilitating data exchange over the web, leveraging the power of the HTTP protocol.
Node.js, a JavaScript runtime built on Chrome's V8 engine, has gained traction for creating efficient, lightweight APIs. Its event-driven, non-blocking I/O model excels at handling concurrent connections, making it ideal for scalable, real-time APIs.
Frameworks like Express.js simplify development, allowing developers to quickly build robust APIs for diverse use cases. For web developers seeking to create RESTful APIs, Node.js and Express offer a powerful combination to streamline the process.
What is REST API?
A REST API is an architectural style that leverages the HTTP protocol to facilitate data exchange between client and server applications. It is based on a set of constraints and principles, including a uniform interface, stateless communication, and the use of standard HTTP methods (GET, POST, PUT, DELETE) for performing operations on resources.
What is Node.js?
Node.js, a server-side JavaScript runtime, and Express, a popular Node.js framework, make for a powerful combination to create RESTful APIs. In this blog post, we'll explore the process of creating a REST API using Node.js and Express, step by step, with practical examples.
Prerequisites
Before we dive into building our REST API, make sure you have the following tools and knowledge:
- Node.js and npm: Install Node.js, which includes npm (Node Package Manager), from the official website.
- Text Editor or IDE: Choose your preferred code editor. Some popular choices are Visual Studio Code, Sublime Text, or WebStorm.
- Basic JavaScript Knowledge: Understanding of JavaScript is crucial for Node.js development.
Step-by-step Guide to Make a REST API with Node.js
Step 1: Initialize Your Project
Let's start by creating a new directory for your project and initializing it with npm.
mkdir my-rest-api
cd my-rest-api
npm init -y
This will create a package.json
file with default settings for your project.
Step 2: Install Dependencies
We need a couple of packages to get our REST API up and running:
- Express: The web application framework for Node.js.
- Body-parser: A middleware for parsing incoming request bodies.
- Nodemon (Optional): A tool that helps in automatically restarting the server during development.
Install these packages using npm:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
const port = process.env.PORT || 3000;
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Step 3: Create an Express App
Now, let's create an Express application and set up a basic server.
javascriptCopy codeconst express = require('express');const bodyParser = require('body-parser');const app = express();use(bodyParser.json());const port = process.env.PORT || 3000;listen(port, () => {console.log(`Server is running on port ${port}`);
Here, we've created a basic Express app, added the body-parser middleware to parse JSON data, and started a server on port 3000.
Step 4: Define Routes
In a REST API, routes define the endpoints for different HTTP methods (GET, POST, PUT, DELETE). Let's create a simple example with a GET request.
app.get('/api/hello', (req, res) => {
res.json({ message: 'Hello, World!' });
});
This code defines a route for /api/hello
that responds with a JSON message when accessed via a GET request.
Step 5: Run Your API
You can run your API using Node.js, but during development, it's handy to use Nodemon, which will automatically restart your server on code changes.
npm start
Now, if you access http://localhost:3000/api/hello
, you should see the "Hello, World!" message.
Step 6: Add More Routes
To make a useful API, you'll need to define more routes and implement CRUD (Create, Read, Update, Delete) operations for your resources. Here's an example of a simple "To-Do List" API。
let todos = [];
app.get('/api/todos', (req, res) => {
res.json(todos);
});
app.post('/api/todos', (req, res) => {
const newTodo = req.body;
todos.push(newTodo);
res.status(201).json(newTodo);
});
// Implement PUT and DELETE as an exercise
In this example, we've added routes to list and create to-do items. You can extend this by implementing PUT and DELETE for updating and deleting tasks.
Step 7: Testing Your API
Testing your API is crucial to ensure it works as expected. Tools like Apidog or Insomnia can help you send requests and verify the responses.
Step 8: Deploy Your API
When you're ready to share your API with the world, you can deploy it on platforms like Heroku, AWS, or Azure.
An alternative way to Create a REST API with Apidog
Apidog simplifies REST API development, streamlining testing, documentation, security, and performance. This article demonstrates how to use Apidog and its key features.
Step 1: Download and Install Apidog
First, you need to create an account on Apidog. This can be done by going to the Apidog website and clicking the "Sign up" button. After registration, you will be redirected to the Apidog homepage.
Step 2: Create an API
Within the project, you can create a new API. The documentation is the blueprint of the REST API, describing its resources, operations, and parameters. To create a new API, click the "+" button on the project page.
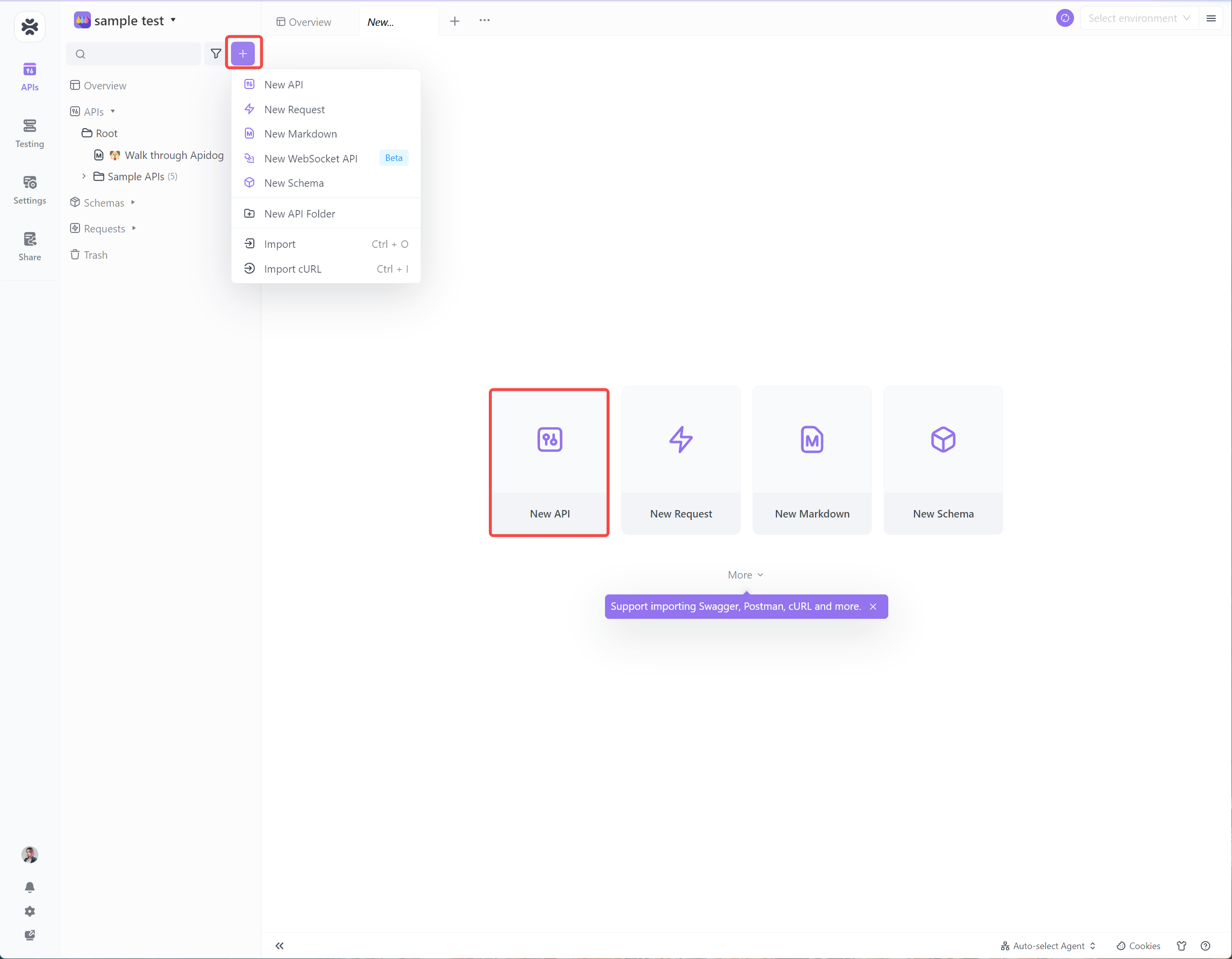
Step 3: Set the Parameters of REST APIs
Fill in the HTTP methods, request/response models, query parameters, headers, etc.
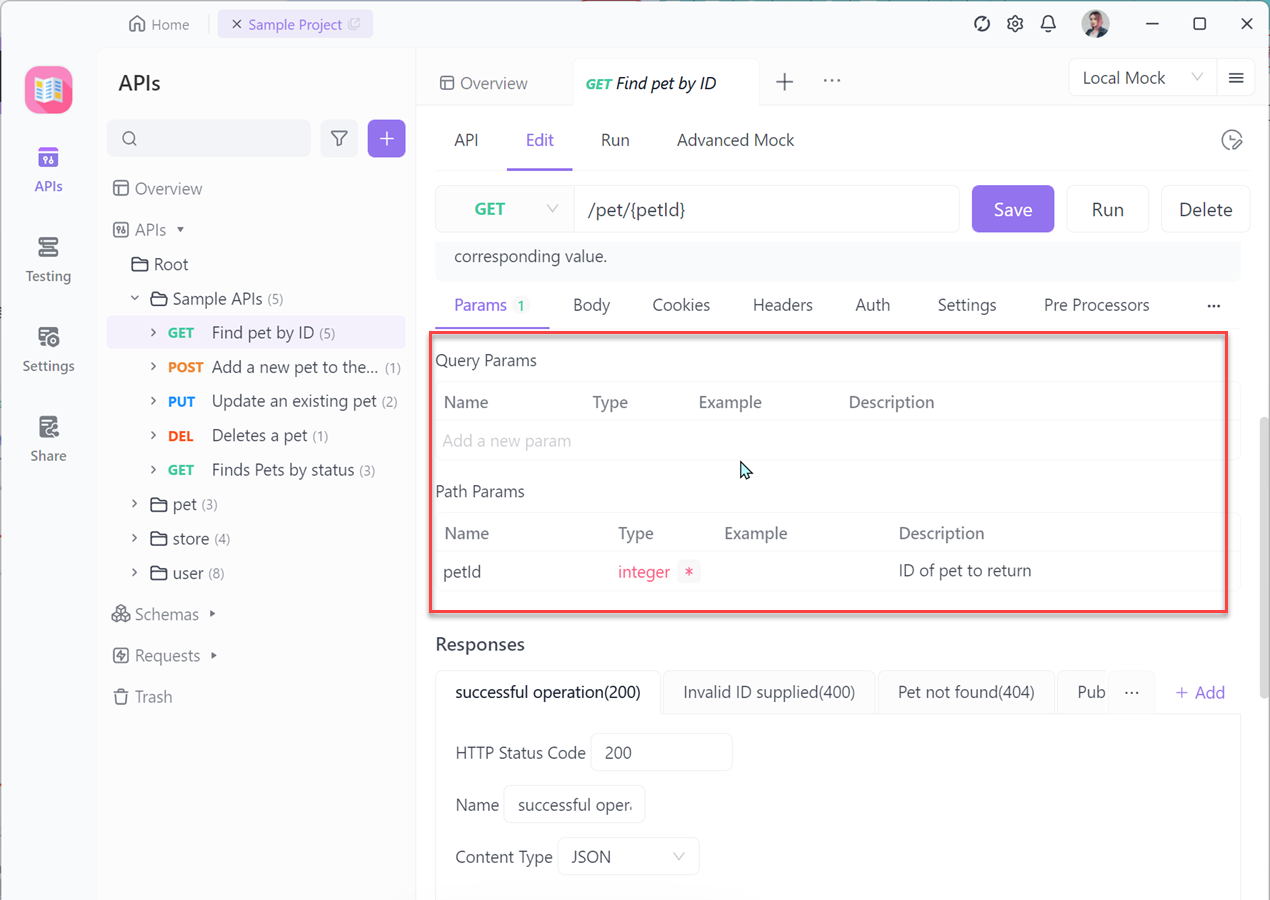
Step 4: Testing REST API
After all APIs have been developed, you can click "Send" button to test your APIs.
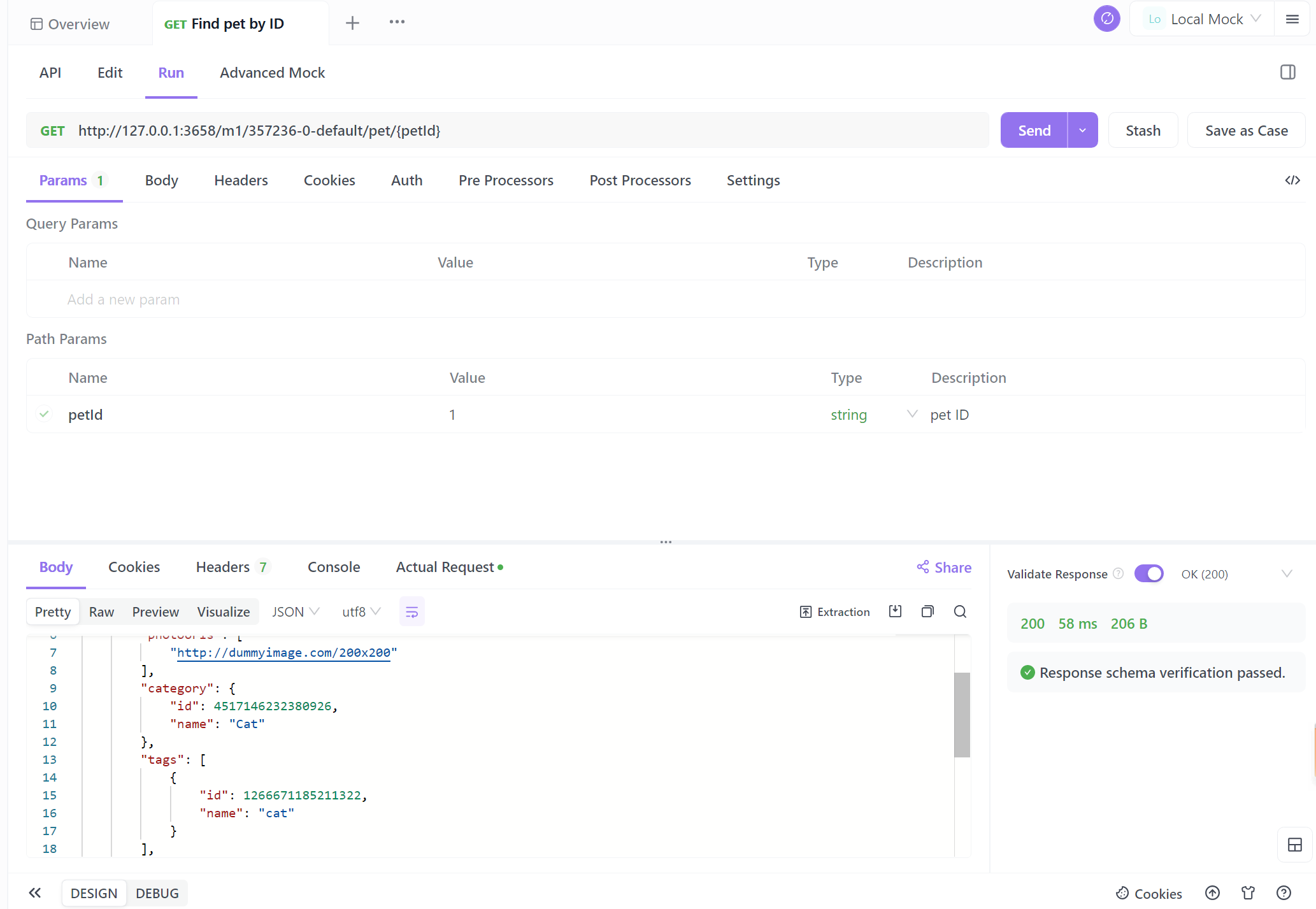
Conclusion
Creating a REST API with Node.js and Express is a powerful skill for any web developer. In this blog post, we covered the essential steps from project setup to defining routes, and even touched on testing and deployment. Remember, practice is key, so don't hesitate to build your own APIs, experiment with different functionalities, and explore more advanced features as you become more proficient with Node.js and Express.
With this foundation, you'll be well on your way to developing robust and scalable web applications with RESTful APIs. Happy coding!