If you’re a developer who works with APIs, you probably know how important it is to test and debug them before deploying them to production. You want to make sure that your APIs are reliable, secure, and performant, and that they meet the expectations of your clients and users.
But how do you test and debug your APIs, especially if they use gRPC, a modern and efficient protocol for communication between microservices? That’s where grpc-curl comes in. grpc-curl is a command-line tool that lets you interact with gRPC servers simply and intuitively.
In this blog post, I’ll show you how to use grpc-curl to test and debug your APIs, and why you should consider using it for your gRPC projects.
What is grpc-curl?
grpc-curl is a command-line tool that lets you invoke RPC methods on a gRPC server from the command-line. It supports various RPC methods, including unary, server-streaming, client-streaming, and bi-directional streaming. You can also browse the schema for gRPC services, either by querying a server that supports server reflection or by loading in compiled protoset files.
grpc-curl is written in .NET, and it has some advantages over gRPCurl, such as:
- It supports both .NET Core and .NET Framework, and it can run on Windows, Linux, and Mac OS.
- It has a more concise and expressive syntax, and it can infer the service and method names from the URL.
- It can automatically format the JSON output with colors and indentation, and it can also output XML or plain text.
- It can handle nested messages and repeated fields better, and it can also accept input from files or standard input.
- It can use environment variables for common options, such as the server URL, the headers, and the TLS settings.
- It can generate code snippets for C#, Java, Python, and Go, to help you write your own client code.
grpc-curl is available as a NuGet package, and you can install it with the following command:
dotnet tool install -g grpc-curl
How to Use grpc-curl?
To use grpc-curl, you need to have a gRPC server running, and you need to know its URL and the service and method names that you want to call. You also need to have the schema for the gRPC services, either by using server reflection or by loading protoset files.
For this example, I’ll use a simple gRPC server that implements a calculator service, with four methods: Add, Subtract, Multiply, and Divide. The server is written in C#, and it supports server reflection.
To start the server, run the following command from the project folder:
dotnet run
The server will listen on port 5000 by default. You can change the port number in the appsettings.json file.
To call the Add method on the server, run the following command from another terminal:
grpc-curl http://localhost:5000 Calculator/Add -d '{"x": 3, "y": 5}'
The output should look something like this:
{
"result": 8
}
As you can see, grpc-curl automatically infers the service and method names from the URL, and it formats the JSON output with colors and indentation. You can also use the -o option to specify a different output format, such as xml or text.
To call the Subtract method, run the following command:
grpc-curl http://localhost:5000 Calculator/Subtract -d '{"x": 10, "y": 4}'
The output should look something like this:
{
"result": 6
}
To call the Multiply method, run the following command:
grpc-curl http://localhost:5000 Calculator/Multiply -d '{"x": 2, "y": 7}'
The output should look something like this:
{
"result": 14
}
To call the Divide method, run the following command:
grpc-curl http://localhost:5000 Calculator/Divide -d '{"x": 15, "y": 3}'
The output should look something like this:
{
"result": 5
}
If you try to divide by zero, you’ll get an error message, like this:
grpc-curl http://localhost:5000 Calculator/Divide -d '{"x": 15, "y": 0}'
{
"error": "Cannot divide by zero."
}
grpc-curl also supports streaming methods, which can send or receive multiple messages in a single call. For example, let’s say we have a server-streaming method called Fibonacci, which takes a number n as input and returns the first n Fibonacci numbers as a stream of messages. The server code for this method looks something like this:
public override async Task Fibonacci(Number request, IServerStreamWriter<Number> responseStream, ServerCallContext context)
{
int n = request.Value;
int a = 0;
int b = 1;
for (int i = 0; i < n; i++)
{
await responseStream.WriteAsync(new Number { Value = a });
int temp = a;
a = b;
b = temp + b;
}
}
To call this method with grpc-curl, run the following command:
grpc-curl http://localhost:5000 Calculator/Fibonacci -d '{"value": 10}'
The output should look something like this:
{
"value": 0
}
{
"value": 1
}
{
"value": 1
}
{
"value": 2
}
{
"value": 3
}
{
"value": 5
}
{
"value": 8
}
{
"value": 13
}
{
"value": 21
}
{
"value": 34
}
As you can see, grpc-curl prints each message as it receives it from the server, and it closes the connection when the stream is complete.
grpc-curl also supports client-streaming methods, which can send multiple messages to the server and receive a single response. For example, let’s say we have a client-streaming method called Sum, which takes a stream of numbers as input and returns their sum as a response. The server code for this method looks something like this:
public override async Task<Number> Sum(IAsyncStreamReader<Number> requestStream, ServerCallContext context)
{
int sum = 0;
await foreach (var number in requestStream.ReadAllAsync())
{
sum += number.Value;
}
return new Number { Value = sum };
}
To call this method with grpc-curl, we need to provide the input messages from a file or from standard input. For example, we can create a file called numbers.json with the following content:
{"value": 1}
{"value": 2}
{"value": 3}
{"value": 4}
{"value": 5}
Then, we can run the following command:
grpc-curl http://localhost:5000 Calculator/Sum -d @numbers.json
The output should look something like this:
{
"value": 15
}
Alternatively, we can use the - symbol to indicate that the input messages are coming from standard input, and then type or paste the messages in the terminal, followed by Ctrl+D to end the input. For example:
grpc-curl http://localhost:5000 Calculator/Sum -d -
{"value": 1}
{"value": 2}
{"value": 3}
{"value": 4}
{"value": 5}
^D
The output should be the same as before.
grpc-curl also supports bi-directional streaming methods, which can send and receive multiple messages in both directions. For example, let’s say we have a bi-directional streaming method called Chat, which takes a stream of messages as input and returns a stream of messages as output. The server code for this method looks something like this:
public override async Task Chat(IAsyncStreamReader<Message> requestStream, IServerStreamWriter<Message> responseStream, ServerCallContext context)
{
await foreach (var message in requestStream.ReadAllAsync())
{
Console.WriteLine($"Received: {message.Text}");
var reply = new Message { Text = $"Echo: {message.Text}" };
await responseStream.WriteAsync(reply);
Console.WriteLine($"Sent: {reply.Text}");
}
}
To call this method with grpc-curl, we need to provide the input messages from a file or from standard input, and we need to use the -s option to indicate that we want to receive the output messages as a stream. For example, we can create a file called chat.json with the following content:
{"text": "Hello"}
{"text": "How are you?"}
{"text": "Goodbye"}
Then, we can run the following command:
grpc-curl http://localhost:5000 Calculator/Chat -d @chat.json -s
The output should look something like this:
{
"text": "Echo: Hello"
}
{
"text": "Echo: How are you?"
}
{
"text": "Echo: Goodbye"
}
As you can see, grpc-curl sends each message to the server, and prints each reply from the server, until the input stream is exhausted.
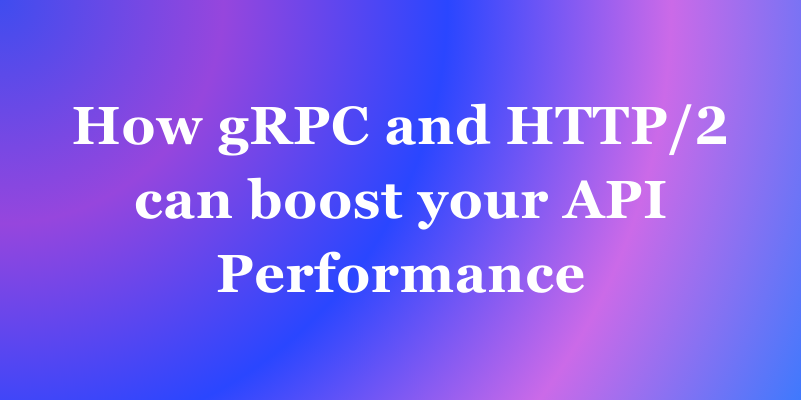
Why use grpc-curl?
grpc-curl is a handy tool for testing and debugging your gRPC APIs, because it allows you to:
- Invoke any kind of RPC method, including streaming methods, from the command-line, without writing any client code.
- Browse the schema for gRPC services, either by querying a server that supports server reflection, or by loading protoset files.
- Format the output in different ways, such as JSON, XML, or plain text, with colors and indentation.
- Handle nested messages and repeated fields better than gRPCurl, and accept input from files or standard input.
- Use environment variables for common options, such as the server URL, the headers, and the TLS settings.
- Generate code snippets for C#, Java, Python, and Go, to help you write your own client code.
grpc-curl is also easy to install and use, and it works on multiple platforms, such as Windows, Linux, and Mac OS. It’s compatible with both .NET Core and .NET Framework, and it supports both HTTP/1.1 and HTTP/2 protocols.
grpc-curl is not only useful for developers, but also for testers, QA engineers, DevOps engineers, and anyone who needs to interact with gRPC servers. It can help you verify the functionality, performance, security, and reliability of your APIs, and identify and fix any issues or bugs.
How to use grpc-curl with apidog?
If you want to take your gRPC testing and debugging to the next level, you might want to use grpc-curl with apidog, a powerful and flexible tool for API documentation and testing.
Apidog is a tool that lets you create, manage, and share API documentation and tests, using a simple and intuitive interface. You can use apidog to document and test any kind of API, including REST, SOAP, GraphQL, and gRPC.
With apidog, you can:
- Import your API schema from various sources, such as OpenAPI, Swagger, WSDL, GraphQL, or protoset files.
- Create and organize your API endpoints, methods, parameters, headers, and responses, using a graphical editor or a code editor.
- Add descriptions, examples, validations, and annotations to your API elements, using Markdown or HTML.
- Generate interactive and beautiful API documentation, with live examples, code snippets, and diagrams.
- Create and run API tests, using a built-in test runner or a command-line tool.
- Monitor and debug your API calls, using a built-in proxy or a browser extension.
- Collaborate and share your API projects with your team or your clients, using a cloud-based platform or a self-hosted solution.
Server Streaming
Server Streaming, as the name implies, involves sending multiple response data in a single request. For instance, it could involve subscribing to all the transaction price data of stocks within a one-minute timeframe.
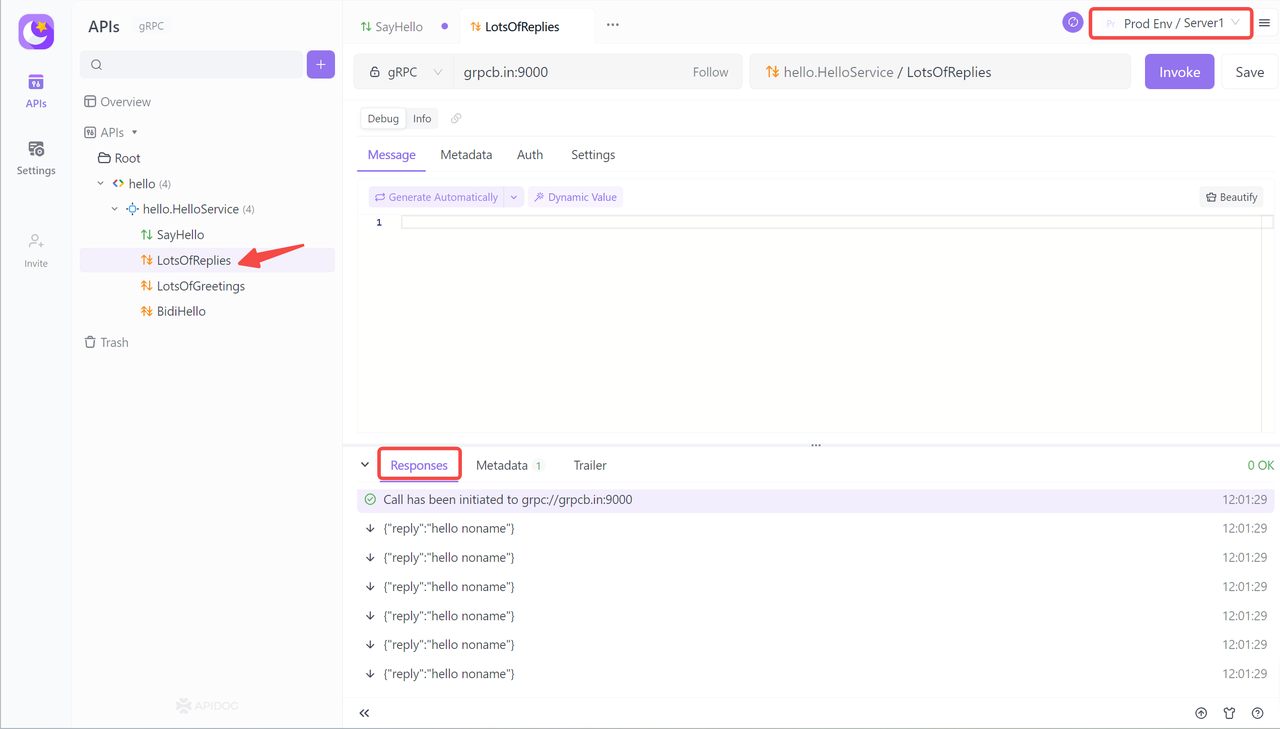
Client Streaming
In this mode, the client can continuously send multiple request messages to the server without waiting for immediate responses. After processing all the requests, the server sends a single response message back to the client. This approach is well-suited for efficiently transmitting large amounts of data in a streaming manner, which helps reduce latency and optimize data exchange.
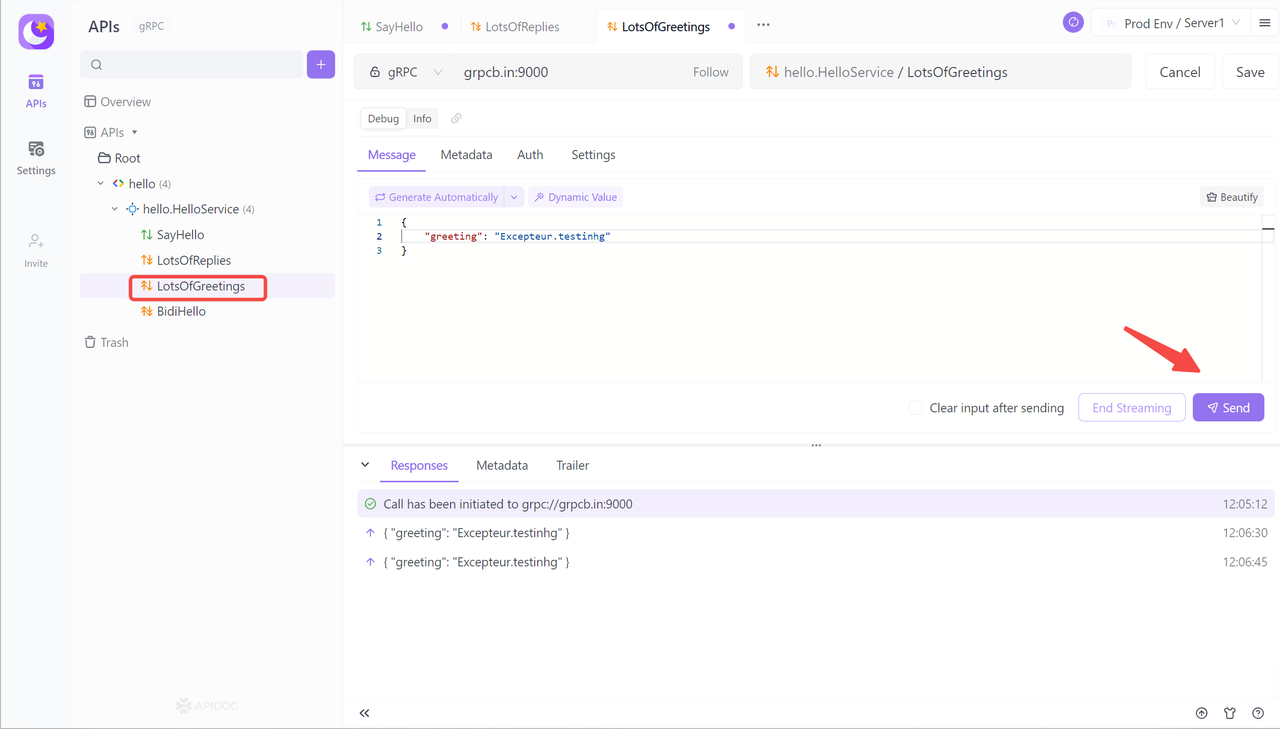
Bidirectional Streaming
Bidirectional Streaming enables clients and servers to establish persistent bidirectional communication and transmit multiple messages simultaneously. It is commonly employed in online games and real-time video call software, and is well-suited for real-time communication and large-scale data transmission scenarios. After initiating the call, the client and the server maintain a session between them and receive real-time responses after sending different request contents.
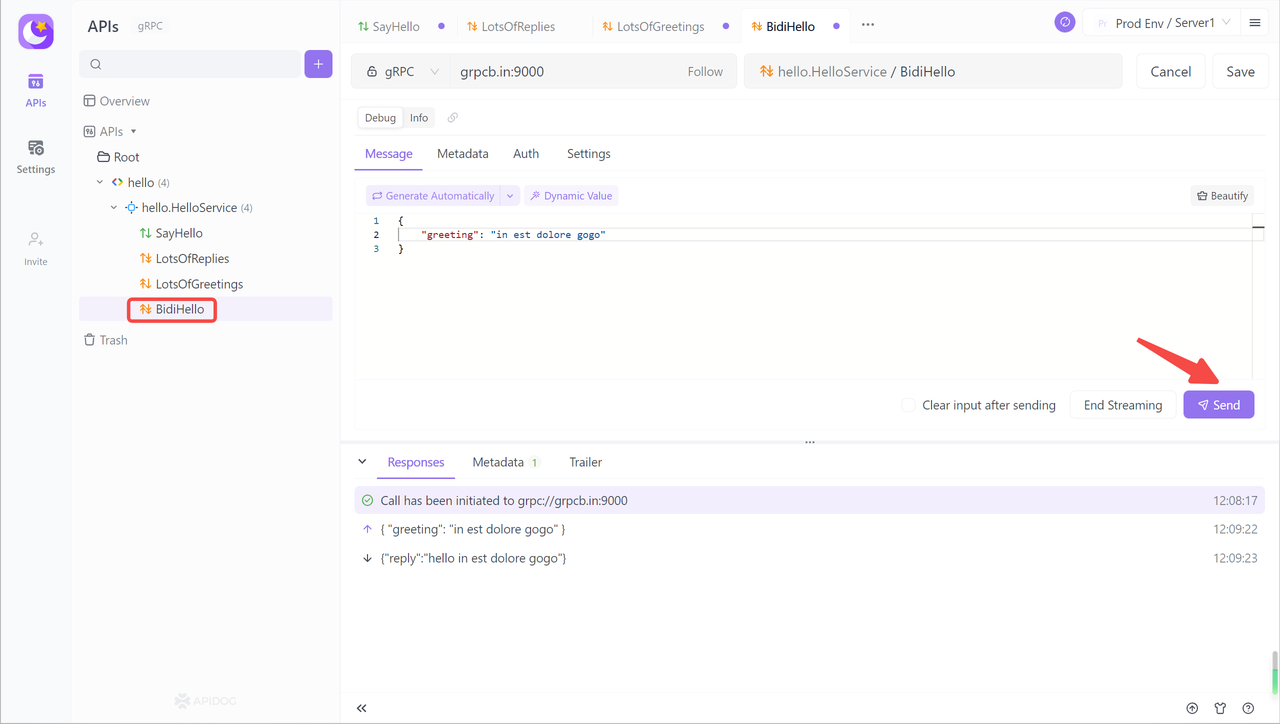
Collaborating on gRPC APIs
Apidog can generate human-readable gRPC interface documents from .proto files, facilitating team collaboration on interfaces. Click the menu button on the right side of the interface to obtain the collaboration link and share it with other team members to align the interface debugging approach.
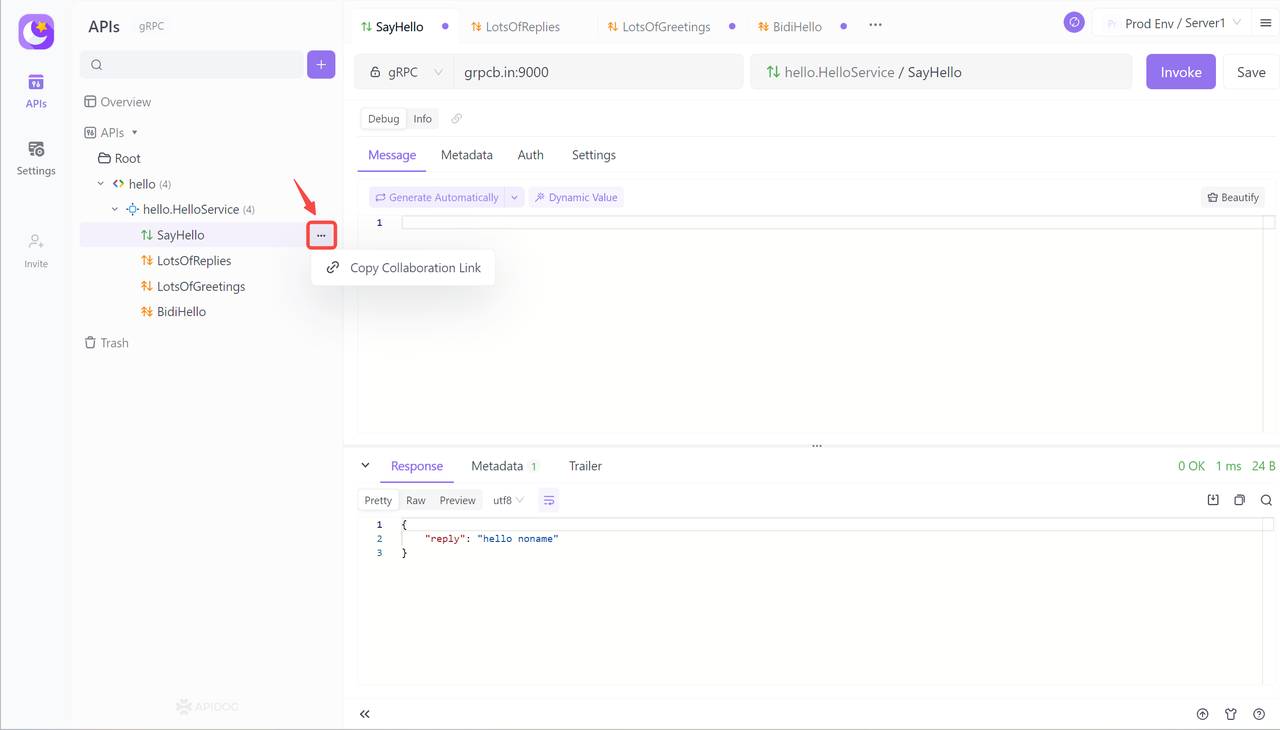
- Create an apidog account, and log in to the apidog web app.
- Create a new API project, and give it a name and a description.
- Import your gRPC schema from a protoset file, or create it manually using the graphical editor or the code editor.
- Add your gRPC endpoints, methods, parameters, headers, and responses, and fill in the details and the examples.
- Generate your API documentation, and view it in the browser or export it as a PDF or HTML file.
- Create your API tests, and run them using the built-in test runner or the command-line tool.
- Monitor and debug your API calls, using the built-in proxy or the browser extension.
- Share your API project with your team or your clients, using the cloud-based platform or the self-hosted solution.
To learn more about apidog, and how to use it with grpc-curl, you can visit the apidog website, or check out the apidog documentation.
Conclusion
grpc-curl is a command-line tool that lets you interact with gRPC servers in a simple and intuitive way. It’s based on gRPCurl, but it adds some features and improvements that make it more user-friendly and powerful. You can use grpc- curl to test and debug your gRPC APIs, and to generate code snippets for your own client code.
grpc-curl is also compatible with apidog, a web-based tool for API documentation and testing. You can use apidog to create, manage, and share your API projects, and to run and monitor your API tests.
If you’re a developer who works with gRPC, or if you’re interested in learning more about gRPC, you should definitely give grpc-curl and Apidog a try. They can help you improve the quality and the usability of your APIs, and make your development process easier and faster.