Unlocking the Power of FastAPI Authentication: Secure Your APIs with Ease
Learn how to secure your APIs with FastAPI authentication. This comprehensive guide covers OAuth2 implementation, password hashing, and leveraging tools like Apidog.com to streamline your API development. Get started with FastAPI today and build high-performance, secure APIs effortlessly.
Whether you're a seasoned developer or just getting started, ensuring that your APIs are secure is critical. FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+, offers an excellent solution. Today, we’ll dive into the ins and outs of FastAPI authentication, explore how to implement it, and why it’s a game-changer for your projects. Plus, we'll touch on how tools like Apidog can make your life even easier.
Why FastAPI for Your APIs?
Before we jump into the nitty-gritty of authentication, let's talk about why you might choose FastAPI in the first place. FastAPI is designed to be fast and simple, enabling you to build and deploy high-performance APIs quickly. It’s built on top of Starlette for the web parts and Pydantic for the data parts, meaning you get both speed and reliability.
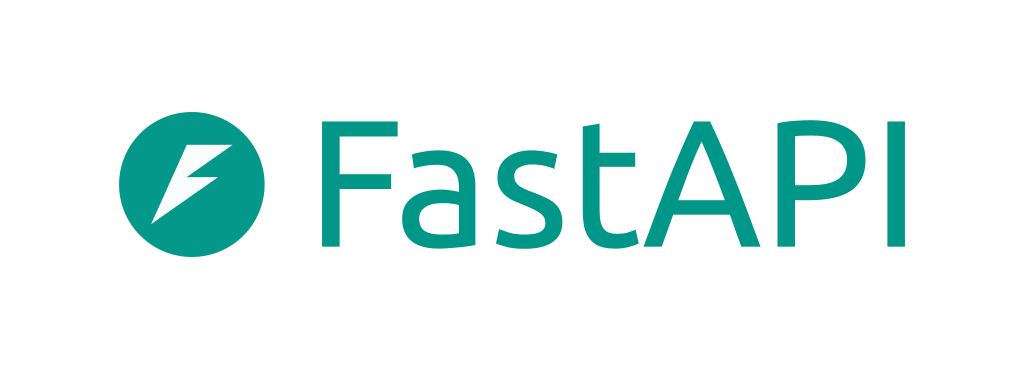
Key Benefits of FastAPI
- Performance: FastAPI is one of the fastest Python frameworks available.
- Ease of Use: Its syntax is intuitive and straightforward, making it accessible even for beginners.
- Automatic Interactive API Documentation: FastAPI automatically generates interactive API documentation with Swagger UI and ReDoc.
- Type Safety: Leveraging Python type hints, FastAPI ensures robust and error-free code.
The Importance of Authentication
When building APIs, securing them with authentication mechanisms is essential to protect sensitive data and ensure that only authorized users can access certain endpoints. Authentication helps verify the identity of users or systems interacting with your API, providing a layer of security and trust.
Types of Authentication
FastAPI supports multiple types of authentication, including:
- Basic Authentication: A straightforward method using a username and password.
- OAuth2: A more secure and flexible method, often used for third-party authentication.
- JWT (JSON Web Tokens): A compact and self-contained way to securely transmit information.
Setting Up FastAPI Authentication
Now, let’s get into the heart of the matter—setting up authentication in FastAPI. We’ll cover a basic implementation of OAuth2 with Password (and hashing), one of the most common and secure ways to handle authentication.
Step 1: Install FastAPI and Dependencies
First, ensure you have FastAPI and the necessary dependencies installed. You can do this using pip:
pip install fastapi[all] uvicorn
The [all]
installs all dependencies needed for authentication, including pydantic
and python-jose
for JWT.
Step 2: Create a Basic FastAPI Application
Let's start with a simple FastAPI application. Create a file named main.py
:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
You can run this application using Uvicorn:
uvicorn main:app --reload
Step 3: Implementing OAuth2 with Password
OAuth2 is a widely used authorization framework that provides specific authorization flows for different types of applications. We'll use OAuth2 with Password (and hashing) to manage authentication.
Creating User Models
First, define your user models. Create a file named models.py
:
from pydantic import BaseModel
class User(BaseModel):
username: str
email: str
full_name: str
disabled: bool = False
class UserInDB(User):
hashed_password: str
Password Hashing Utility
Next, you'll need a utility to hash and verify passwords. Create a file named security.py
:
from passlib.context import CryptContext
pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto")
def verify_password(plain_password, hashed_password):
return pwd_context.verify(plain_password, hashed_password)
def get_password_hash(password):
return pwd_context.hash(password)
OAuth2 Password Flow
Now, configure OAuth2 Password Flow. Update your main.py
to include the following:
from fastapi import Depends, HTTPException, status
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import JWTError, jwt
from pydantic import BaseModel
from typing import Optional
import time
# Secret key to encode JWT
SECRET_KEY = "your_secret_key"
ALGORITHM = "HS256"
ACCESS_TOKEN_EXPIRE_MINUTES = 30
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
# User database simulation
fake_users_db = {
"johndoe": {
"username": "johndoe",
"full_name": "John Doe",
"email": "johndoe@example.com",
"hashed_password": "$2b$12$...",
"disabled": False,
}
}
class Token(BaseModel):
access_token: str
token_type: str
class TokenData(BaseModel):
username: Optional[str] = None
def authenticate_user(fake_db, username: str, password: str):
user = fake_db.get(username)
if not user:
return False
if not verify_password(password, user["hashed_password"]):
return False
return user
def create_access_token(data: dict, expires_delta: Optional[int] = None):
to_encode = data.copy()
if expires_delta:
expire = time.time() + expires_delta
else:
expire = time.time() + ACCESS_TOKEN_EXPIRE_MINUTES * 60
to_encode.update({"exp": expire})
encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM)
return encoded_jwt
@app.post("/token", response_model=Token)
async def login_for_access_token(form_data: OAuth2PasswordRequestForm = Depends()):
user = authenticate_user(fake_users_db, form_data.username, form_data.password)
if not user:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Incorrect username or password",
headers={"WWW-Authenticate": "Bearer"},
)
access_token = create_access_token(data={"sub": user["username"]})
return {"access_token": access_token, "token_type": "bearer"}
@app.get("/users/me", response_model=User)
async def read_users_me(token: str = Depends(oauth2_scheme)):
credentials_exception = HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Could not validate credentials",
headers={"WWW-Authenticate": "Bearer"},
)
try:
payload = jwt.decode(token, SECRET_KEY, algorithms=[ALGORITHM])
username: str = payload.get("sub")
if username is None:
raise credentials_exception
token_data = TokenData(username=username)
except JWTError:
raise credentials_exception
user = fake_users_db.get(username, None)
if user is None:
raise credentials_exception
return user
This code sets up a simple OAuth2 password flow. Users can obtain a token by providing their username and password to the /token
endpoint, and they can use this token to authenticate themselves on protected endpoints like /users/me
.
Step 4: Testing Your Authentication
To test your authentication, you can use an API testing tool like Postman or Apidog. Here’s how you can do it with Apidog:
Create a New request: Open Apidog and create a new request.
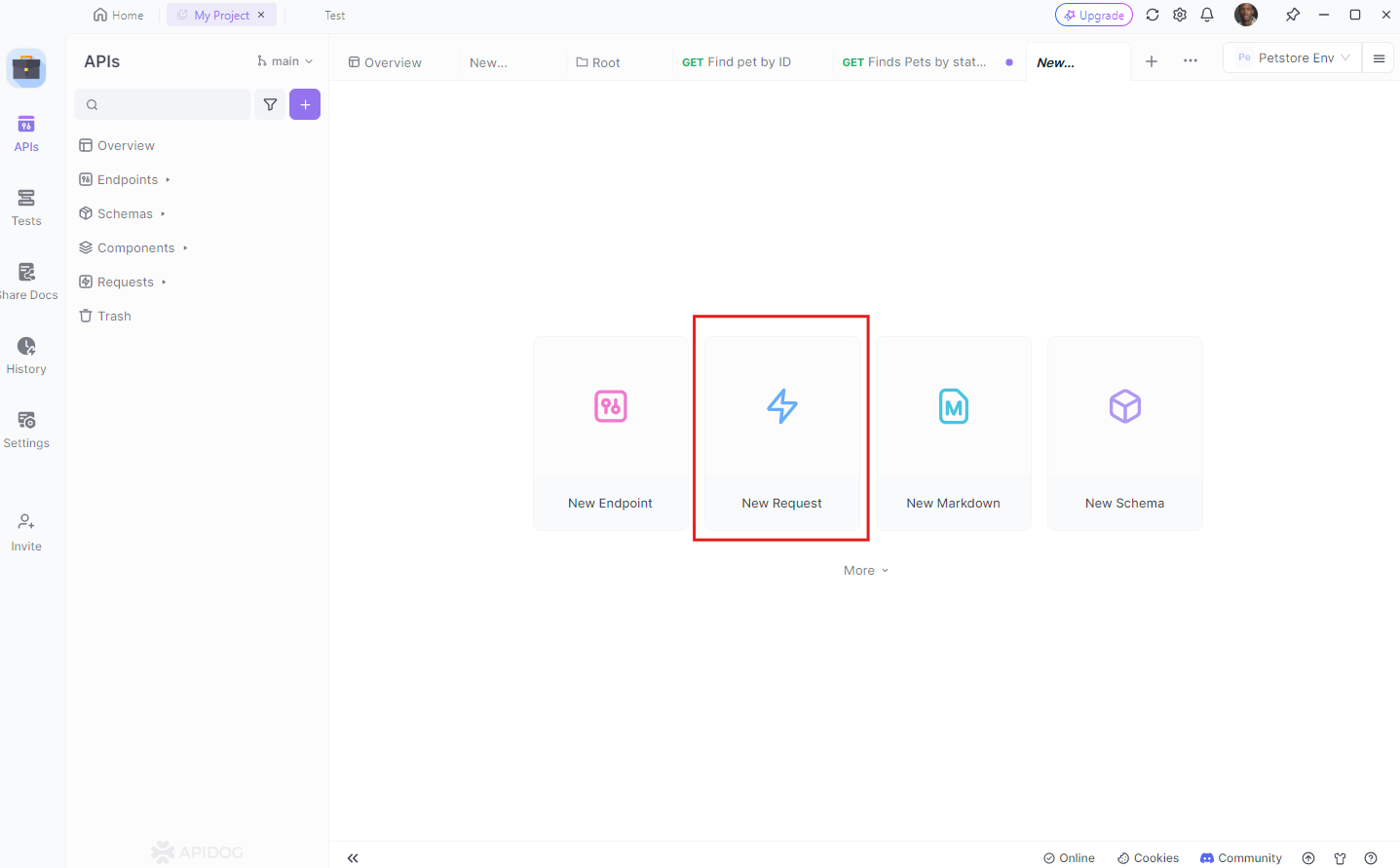
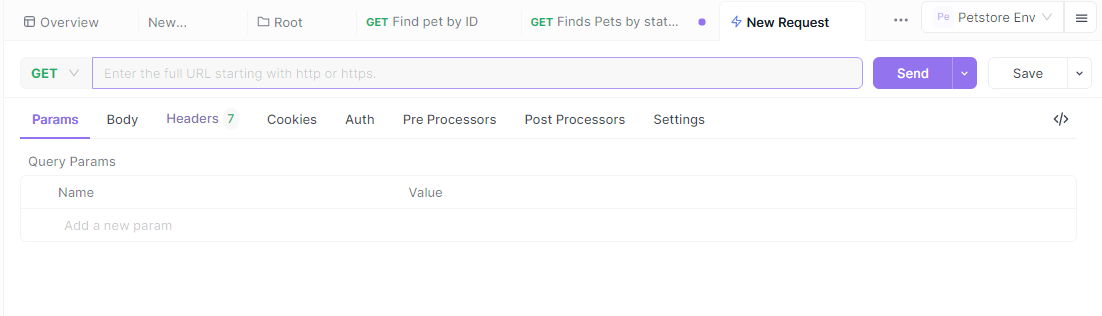
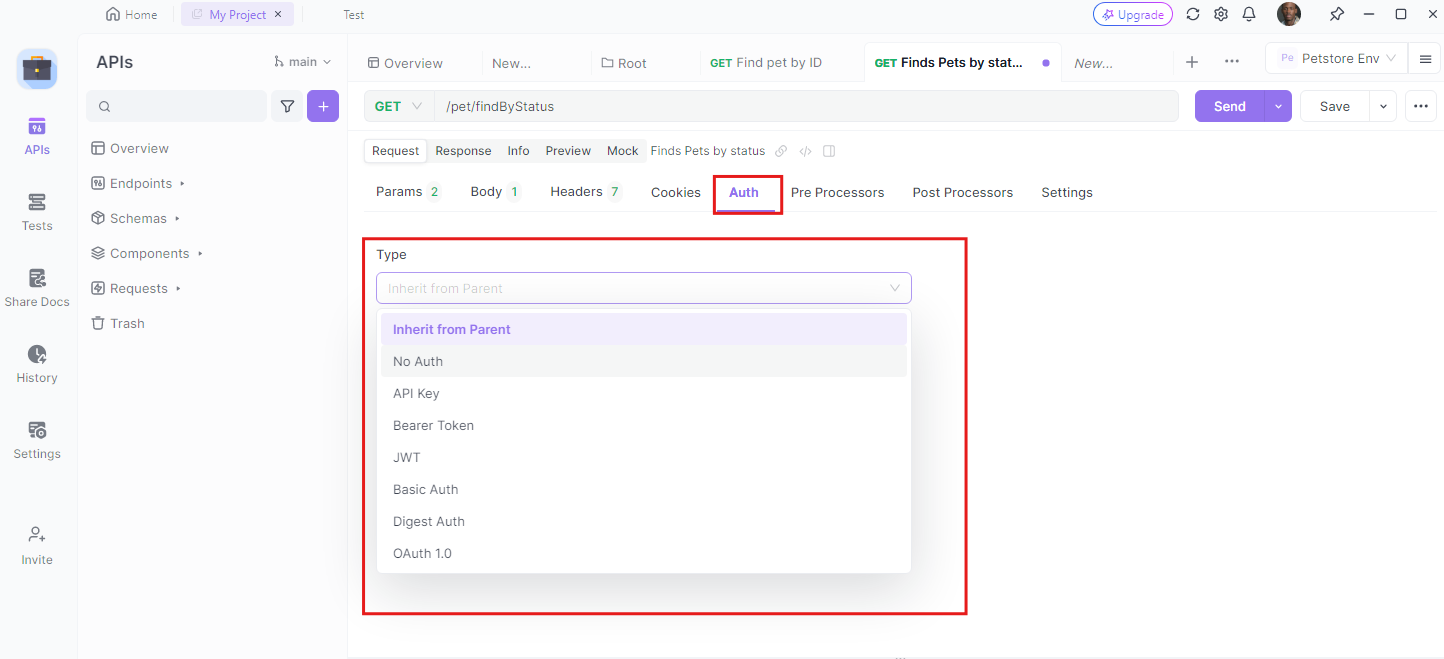
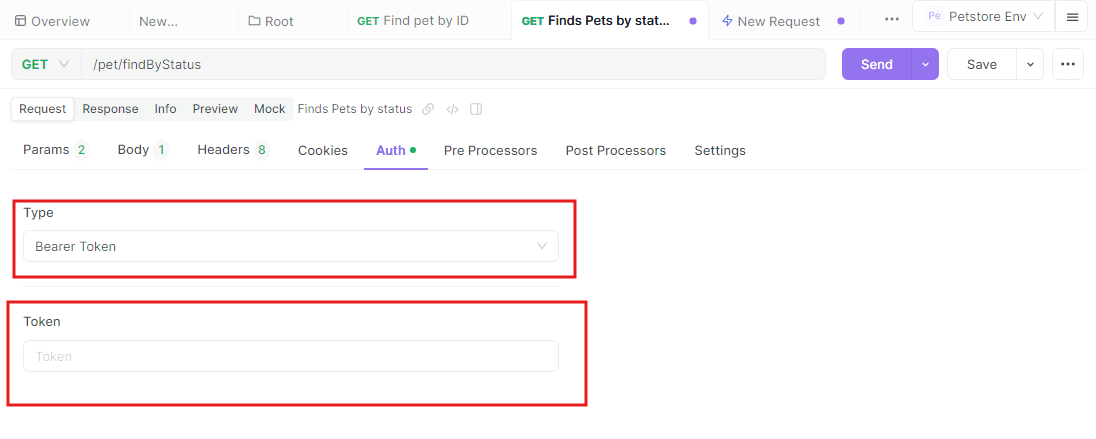
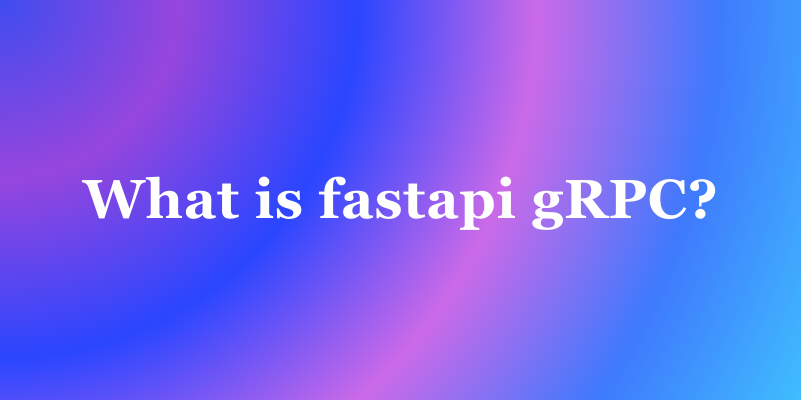
Enhancing Your FastAPI Authentication with Apidog
While FastAPI makes setting up authentication straightforward, tools like Apidog can further streamline the process by providing a platform to design, document, and test your APIs. Apidog offers a user-friendly interface to manage your API endpoints, simulate responses, and collaborate with your team.
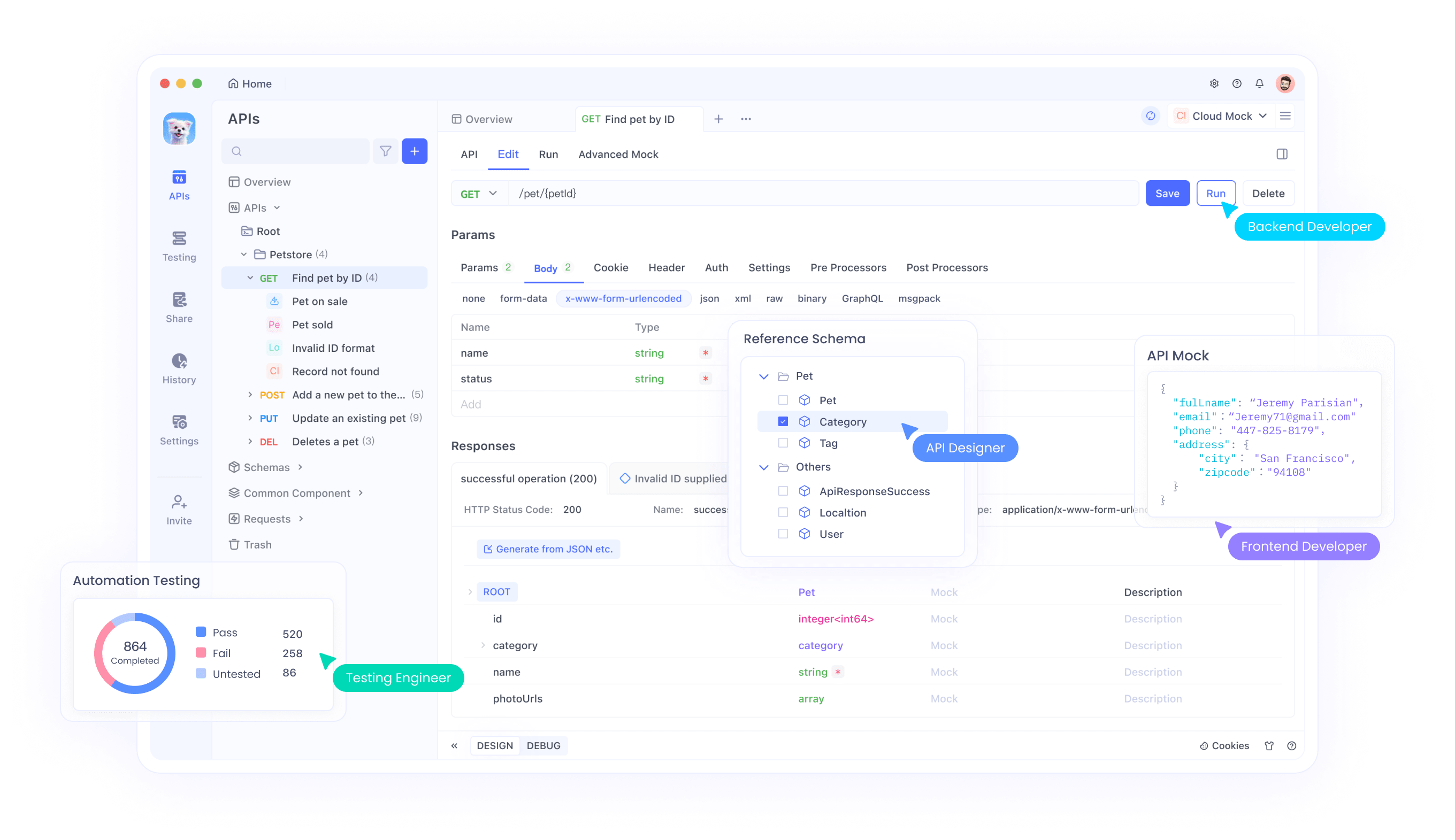
Benefits of Using Apidog
- Comprehensive API Management: Design, test, and document your APIs in one place.
- Collaboration: Work with your team in real-time, share endpoints, and review changes.
- Mock Responses: Simulate API responses for testing without hitting the actual endpoints.
Conclusion
FastAPI simplifies the process of building and securing APIs, making it a top choice for developers looking for performance and ease of use. By implementing authentication with FastAPI, you ensure your APIs are secure and only accessible to authorized users. Additionally, leveraging tools like Apidog can enhance your API development experience by providing robust management and testing capabilities.
Now that you know how to implement FastAPI authentication, you can confidently secure your APIs and protect your data. Happy coding!