Picture this: You’re building a sleek web application. The front end looks perfect, the user experience is smooth, and everything seems in place. But then, out of nowhere, your API calls start failing intermittently. It’s a nightmare scenario for any developer. So, what’s the solution? Enter Axios retry requests. In this blog post, we’ll explore how to implement and optimize Axios retry requests to handle those pesky network errors gracefully. And guess what? By the end, you’ll be a pro at handling API requests like a champ!
Why Axios?
First things first, why should you use Axios for your API requests? Axios is a promise-based HTTP client for JavaScript. It’s popular for its ease of use and versatility, allowing you to make requests to both backend and third-party APIs. It supports all the HTTP methods and works seamlessly with Node.js and browser applications. Plus, it comes with a bunch of helpful features like interceptors, automatic JSON data transformation, and, most importantly for us, retry requests.
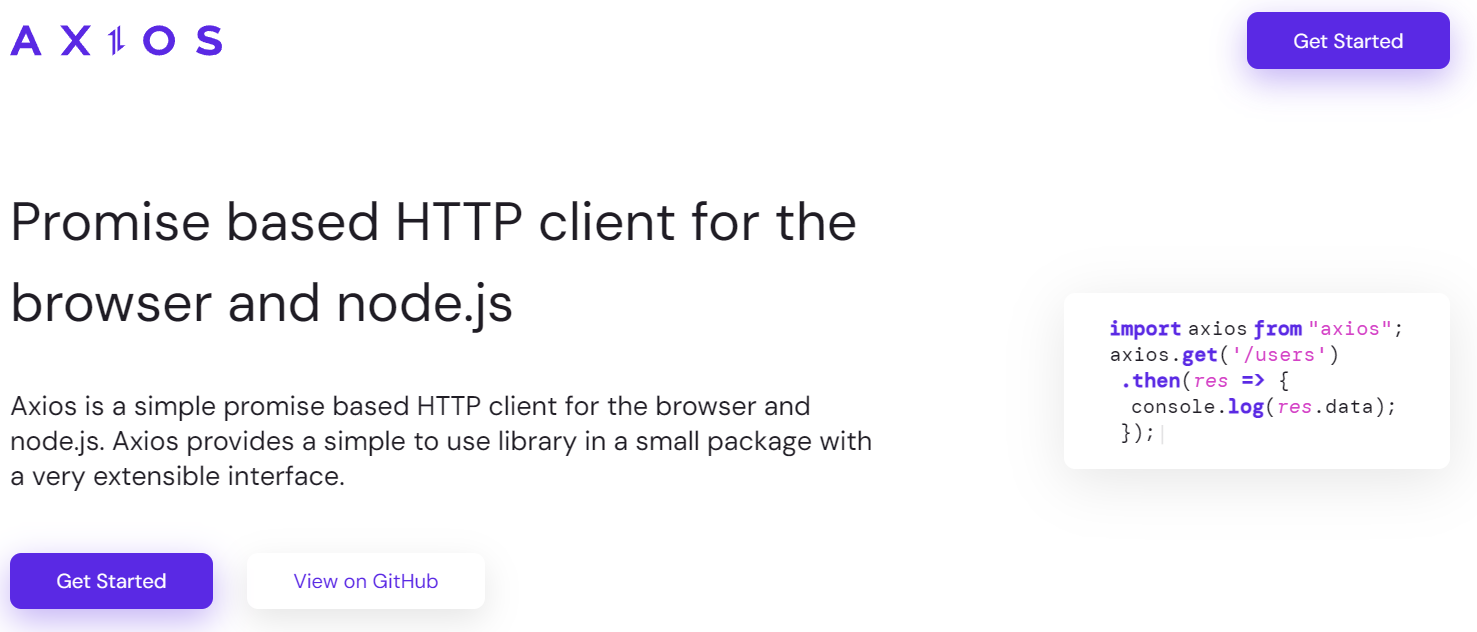
The Problem with Unreliable Networks
Imagine you’re working on an application that relies heavily on API calls. It could be a weather app, a social media dashboard, or an e-commerce site fetching product details. Your users expect real-time data. However, network reliability can be unpredictable. A brief server hiccup or a minor network glitch can result in failed API requests. This is where Axios retry requests come to the rescue, allowing your application to automatically retry failed requests and improve the overall user experience.
Setting Up Axios
Before we can implement retry requests, we need to set up Axios in our project. If you haven’t already installed Axios, you can do so using npm or yarn:
npm install axios
or
yarn add axios
Once installed, you can import Axios and start making API requests. Here’s a simple example:
import axios from 'axios';
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
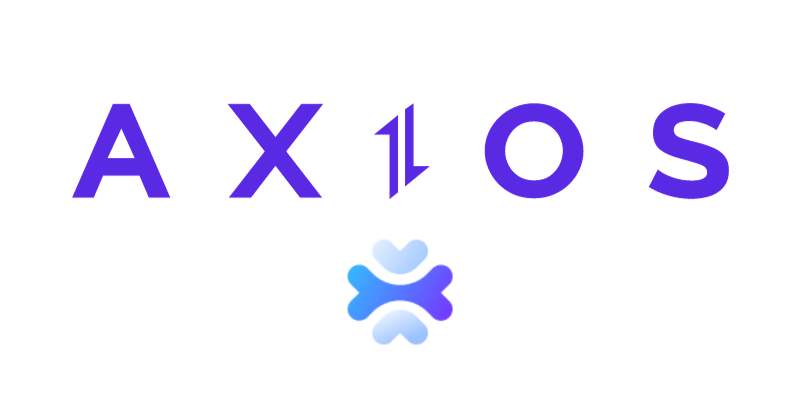
Introducing Axios Retry
So, how do we make Axios retry a request when it fails? The answer lies in Axios interceptors. Interceptors allow you to run your code or modify the request/response before the request is sent or after the response is received. For retrying requests, we’ll use request interceptors.
We’ll need to install an additional package, axios-retry
, which makes implementing retries straightforward. Install it using npm or yarn:
npm install axios-retry
or
yarn add axios-retry
Next, let’s set up Axios retry in our project:
import axios from 'axios';
import axiosRetry from 'axios-retry';
axiosRetry(axios, { retries: 3 });
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example, we’ve configured Axios to retry failed requests up to three times. It’s that simple!
Customizing Axios Retry
The basic setup is great, but we can do much more with Axios retry. For instance, we can customize the retry logic based on the error type, delay between retries, and even exponential backoff.
Custom Retry Logic
Let’s customize the retry logic to retry only on network errors or specific status codes:
import axios from 'axios';
import axiosRetry from 'axios-retry';
axiosRetry(axios, {
retries: 3,
retryCondition: (error) => {
return error.response.status === 500 || axiosRetry.isNetworkOrIdempotentRequestError(error);
}
});
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example, Axios will retry the request if the response status is 500 or if it’s a network error.
Delaying Between Retries
You can also add a delay between retries to avoid bombarding the server with requests in quick succession. Here’s how:
import axios from 'axios';
import axiosRetry from 'axios-retry';
axiosRetry(axios, {
retries: 3,
retryDelay: (retryCount) => {
return retryCount * 1000; // 1 second delay between retries
}
});
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
This setup introduces a 1-second delay between retries.
Exponential Backoff
For a more sophisticated approach, consider using exponential backoff, which increases the delay exponentially with each retry. This can be particularly useful to give the server some breathing room to recover.
import axios from 'axios';
import axiosRetry from 'axios-retry';
axiosRetry(axios, {
retries: 3,
retryDelay: axiosRetry.exponentialDelay
});
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Handling Specific HTTP Methods
Sometimes, you may want to retry only specific HTTP methods. By default, axios-retry
retries GET requests, but you can customize this:
import axios from 'axios';
import axiosRetry from 'axios-retry';
axiosRetry(axios, {
retries: 3,
shouldResetTimeout: true,
retryCondition: (error) => {
return axiosRetry.isRetryableError(error) && error.config.method === 'get';
}
});
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Real-World Use Case: Fetching Data from an API
Let’s bring this all together with a real-world example. Imagine you’re building a weather app that fetches data from a third-party API. Network issues are common, and you want to ensure a smooth user experience.
Here’s how you can implement Axios retry in your weather app:
import axios from 'axios';
import axiosRetry from 'axios-retry';
// Set up Axios retry
axiosRetry(axios, {
retries: 3,
retryDelay: axiosRetry.exponentialDelay,
retryCondition: (error) => {
return error.response.status === 503 || axiosRetry.isNetworkOrIdempotentRequestError(error);
}
});
// Function to fetch weather data
const fetchWeatherData = async (city) => {
try {
const response = await axios.get(`https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=${city}`);
return response.data;
} catch (error) {
console.error('Error fetching weather data:', error);
throw error;
}
};
// Usage
fetchWeatherData('San Francisco')
.then(data => {
console.log('Weather data:', data);
})
.catch(error => {
console.error('Failed to fetch weather data after retries:', error);
});
In this example, we’ve set up Axios to retry requests up to three times with an exponential delay, specifically for network errors or a 503 status code.
Apidog: a free tool to generate your Axios code
Apidog is an all-in-one collaborative API development platform that provides a comprehensive toolkit for designing, debugging, testing, publishing, and mocking APIs. Apidog enables you to automatically create Axios code for making HTTP requests.
Here's the process for using Apidog to generate Axios code:
Step 1: Open Apidog and select new request
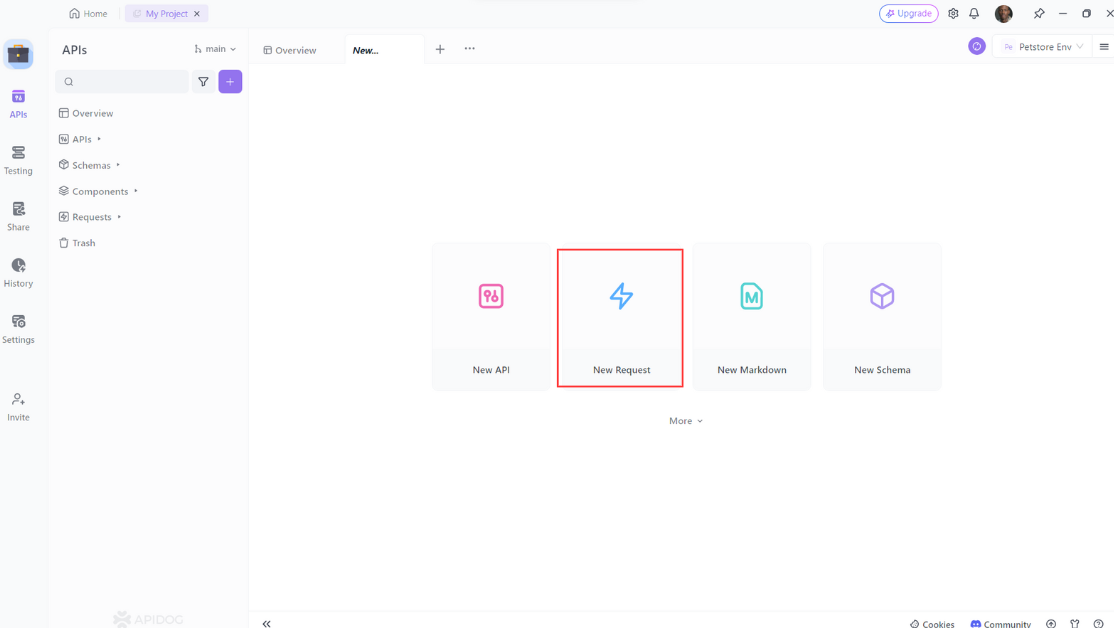
Step 2: Enter the URL of the API endpoint you want to send a request to,input any headers or query string parameters you wish to include with the request, then click on the "Design" to switch to the design interface of Apidog.
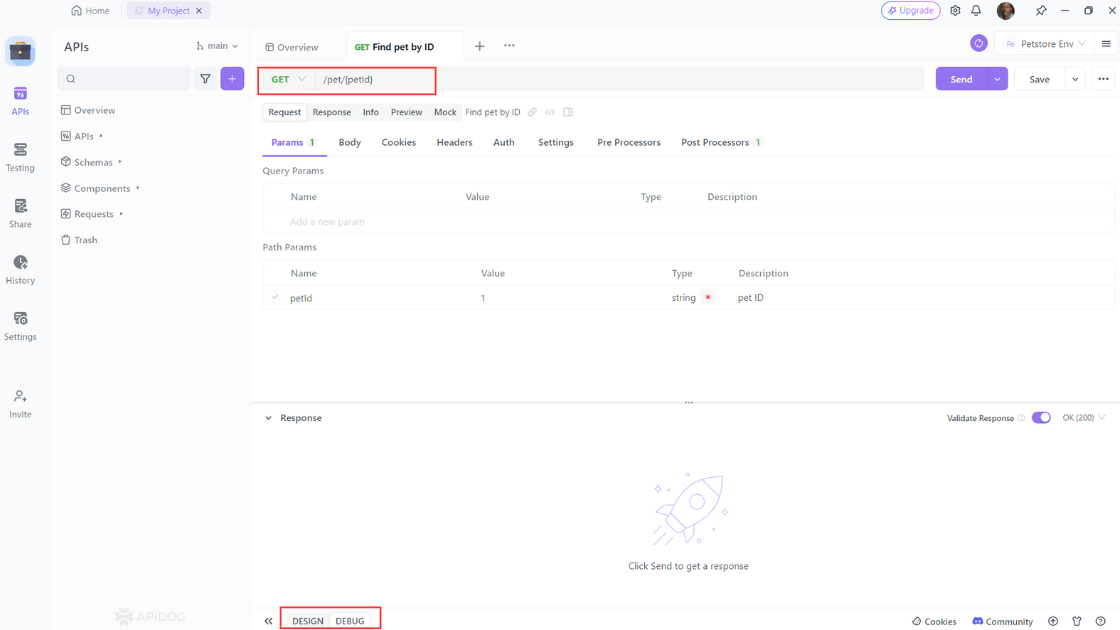
Step 3: Select "Generate client code " to generate your code.
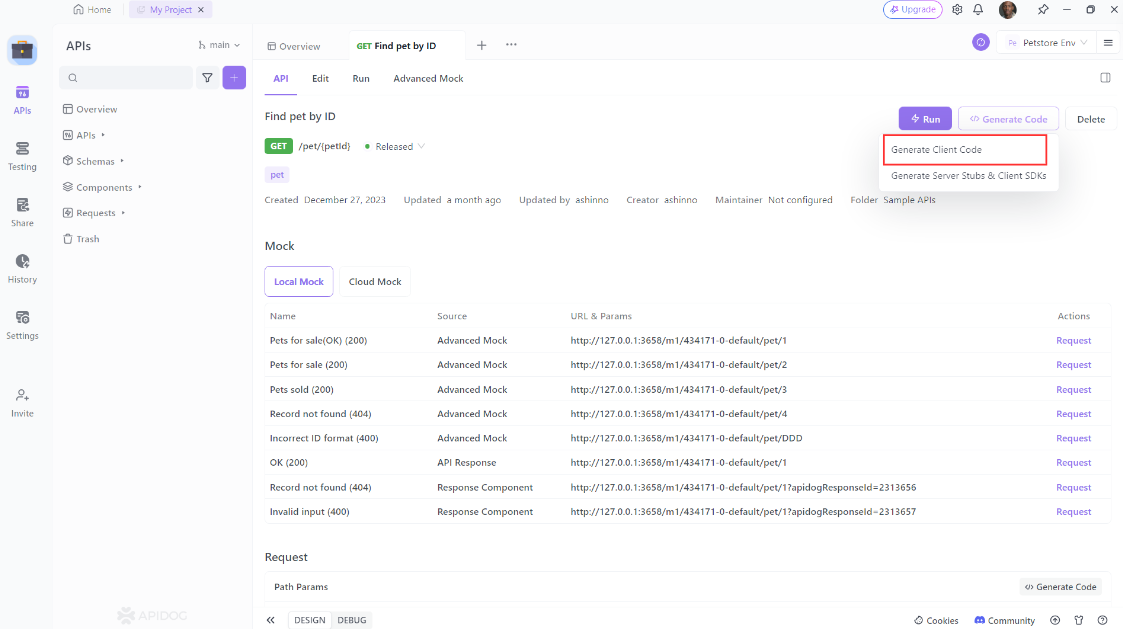
Step 4: Copy the generated Axios code and paste it into your project.
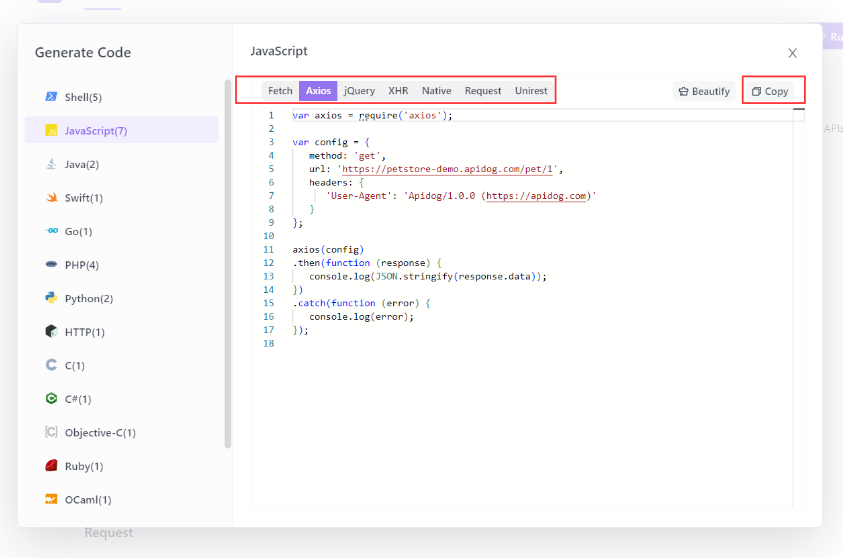
Using Apidog to Send HTTP Requests
Apidog offers several advanced features that further enhance its ability to test HTTP requests. These features allow you to customize your requests and handle more complex scenarios effortlessly.
Step 1: Open Apidog and create a new request.
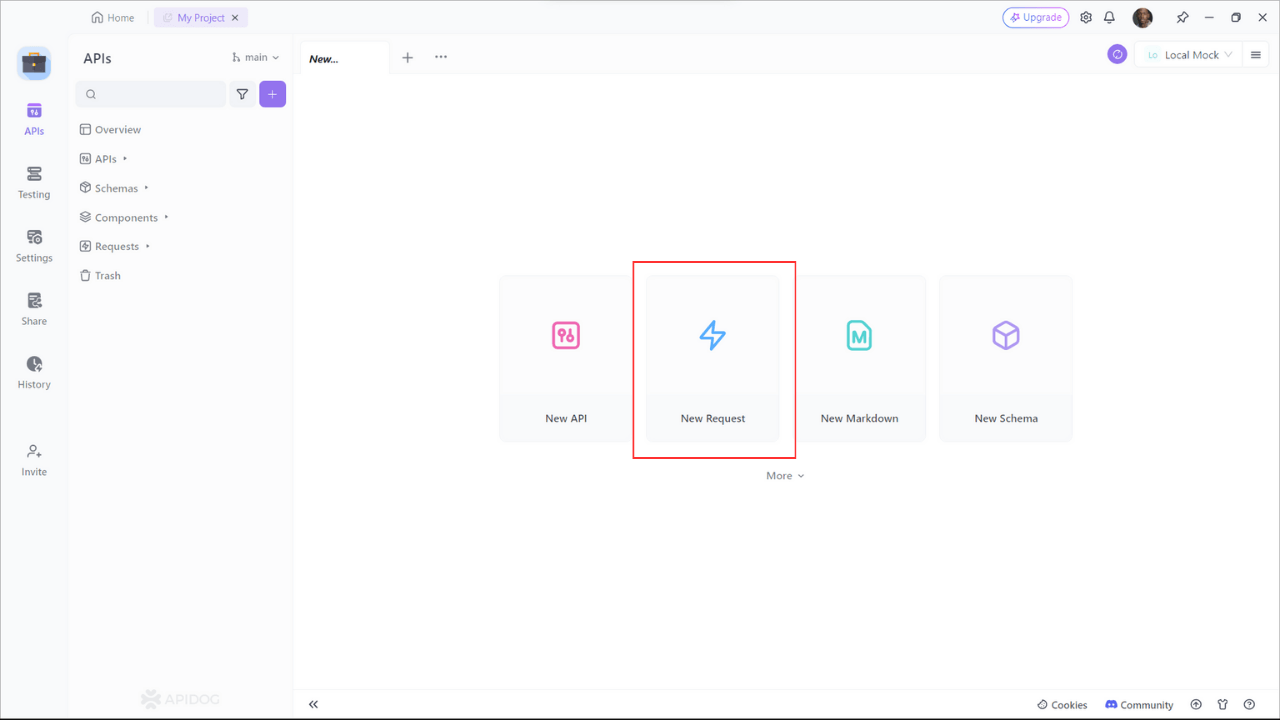
Step 2: Find or manually input the API details for the POST request you want to make.
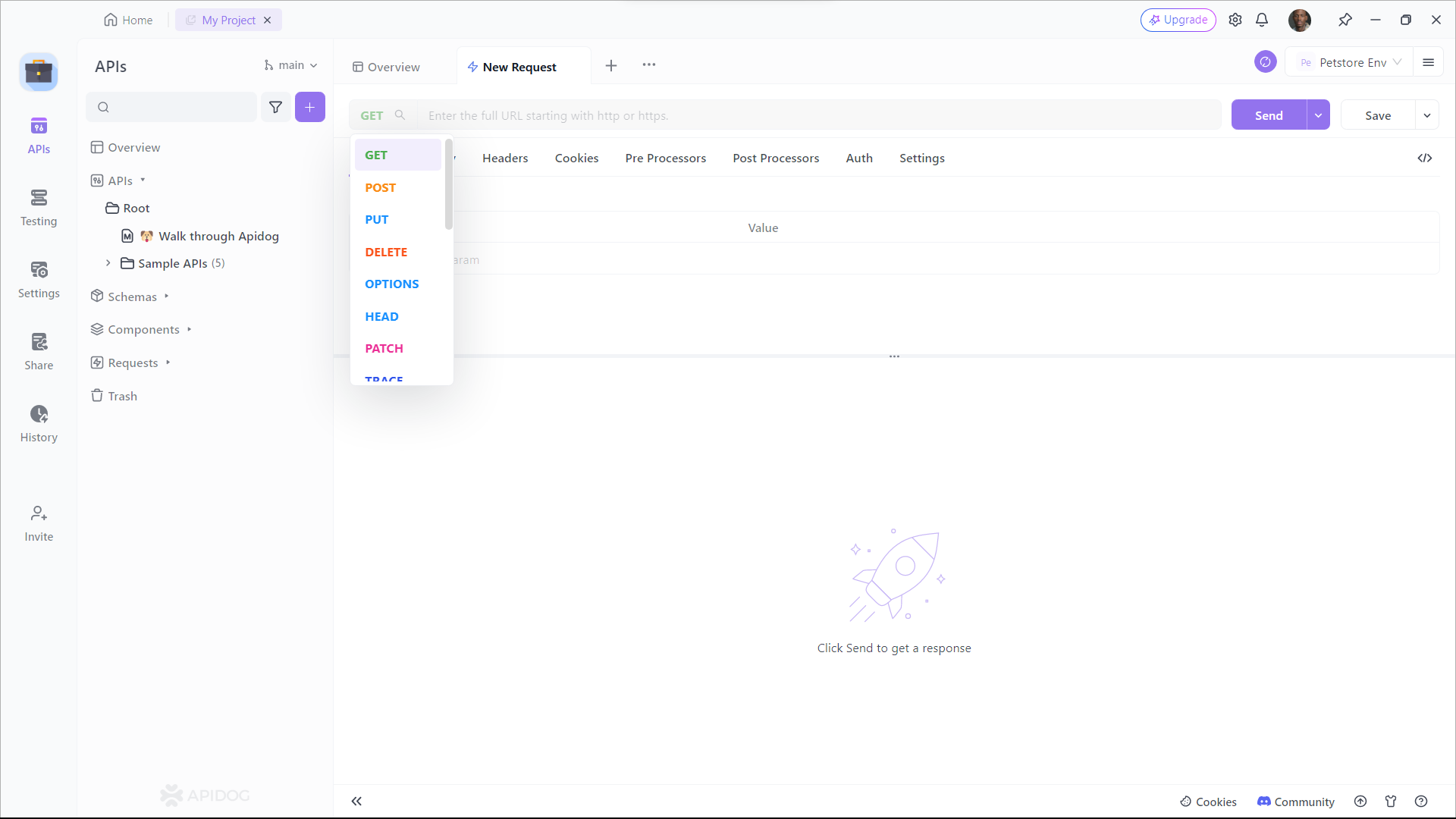
Step 3: Fill in the required parameters and any data you want to include in the request body.
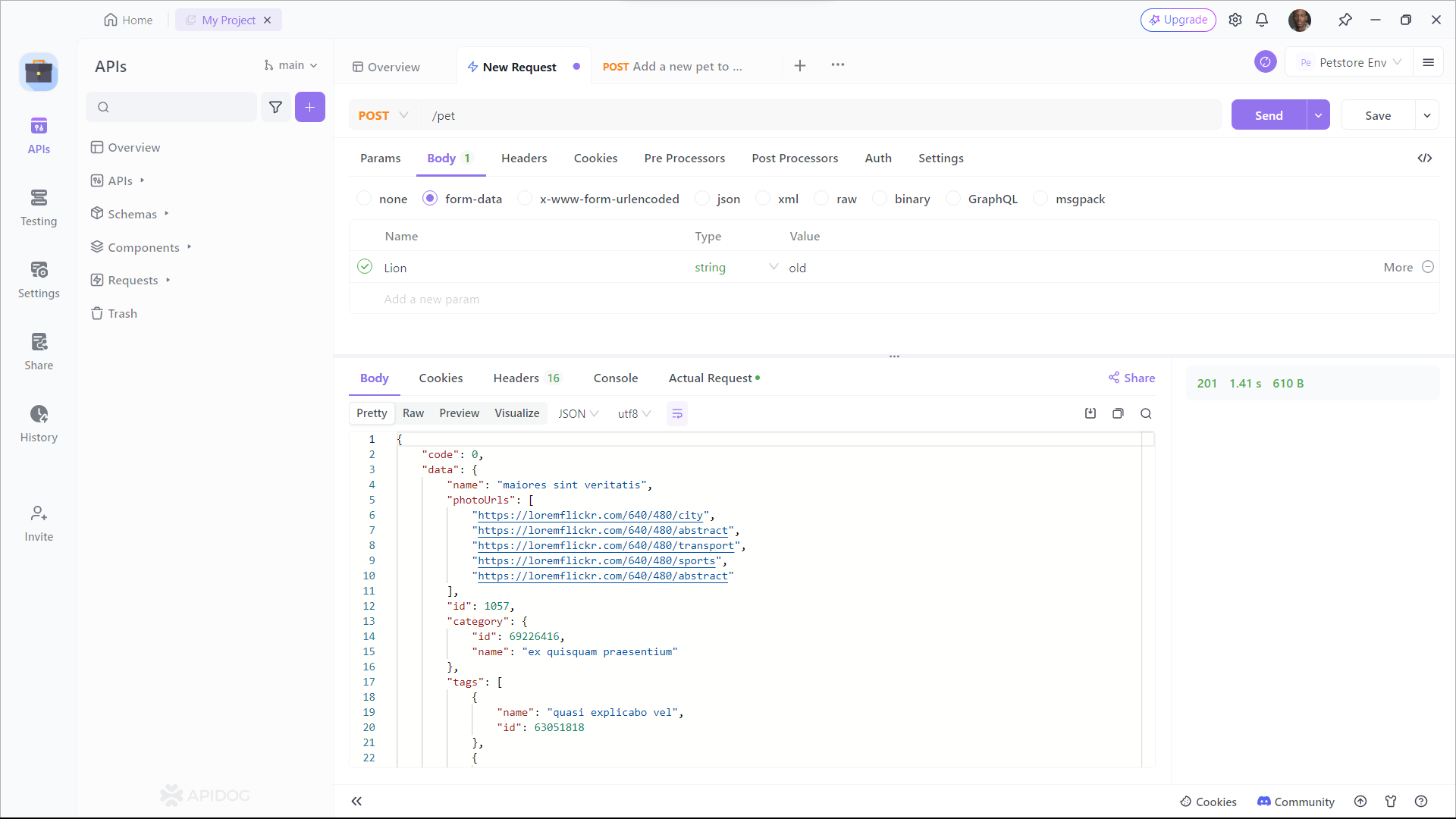
Best Practices
Monitor Retry Attempts
While retries can improve reliability, they can also lead to increased load on your server. Monitor retry attempts and adjust your retry logic as needed. Tools like Apidog can help you monitor and test your APIs effectively.
Handle Different Scenarios
Customize your retry logic based on the specific needs of your application. For example, you might want to handle rate limiting errors differently by implementing a longer delay or a different retry strategy.
Keep the User Informed
Inform your users when retries are happening, especially if it results in a noticeable delay. This can be as simple as showing a loading spinner or a message indicating that the app is trying to reconnect.
Limit Retry Attempts
Avoid infinite retries by setting a reasonable limit. This prevents your application from getting stuck in an endless loop of failed requests.
Conclusion
Mastering Axios retry requests can significantly improve the reliability and user experience of your web applications. By customizing retry logic, introducing delays, and handling different scenarios, you can ensure your app gracefully recovers from network issues.
Ready to take your API development to the next level? Don’t forget to check out Apidog for a comprehensive toolset that simplifies API development and testing. With Apidog, you’ll be equipped to handle any API challenge that comes your way.