API Authorization: Definition, Types, and Best Practices
This article provides an overview of Authorization in APIs, covering the types of authorization such as API Key, OAuth 1.0, JWT, and Basic Authentication. It also explains how to implement authorization in APIs by determining the method, implementation, and testing.
APIs have become a crucial part of modern technology, with businesses relying on them to share data and functionality across different applications and services. However, with the increase in connectivity and data sharing, there comes an increased risk of unauthorized access and security breaches.
Therefore, authorization is a critical aspect of API development that helps ensure only authorized users or clients can access sensitive data and functionality. In this article, we will explore the importance of authorization in APIs, the various authorization methods that can be used, and the role of API toolkits in helping to ease the process of authorization in APIs.
What Does Authorization Mean in APIs
Authorization is the process of verifying that a user or client has the necessary permissions to access specific resources or functions in an API. This is critical to API security as it ensures that only authorized users or clients can access sensitive data and functionality. Authorization is often implemented in conjunction with authentication, which is the process of verifying a user or client's identity.
Types of Authorization in APIs
Several types of authorization can be implemented in APIs, depending on the specific use case and security requirements. Here are some of the common types of authorization in APIs.
API Key Authorization
API key authorization is a simple method where a unique key is generated for each API client. The client must include the API key in each API request to authenticate and authorize their access to the API. This is a basic security measure that prevents unauthorized access to the API. This method is particularly useful for applications that don't require complex user authentication and don't store sensitive user data.
OAuth 1.0 Authorization
OAuth 1.0 is a widely used authorization framework that allows users to grant third-party applications access to their resources without sharing their credentials. It is commonly used for social media APIs, where users want to share their data with other apps. OAuth 1.0 is based on the concept of access tokens, which are issued to authorized clients and used to access protected resources. The access tokens are short-lived, which helps prevent unauthorized access to the API.
JSON Web Tokens (JWT)
JWT is a popular method for securely transmitting information between parties as a JSON object. JWT can be used to create access tokens that can be used to authenticate API requests. The access tokens contain information about the user or client and the authorization scope, which helps the API validate the access rights. JWTs are self-contained, containing all the necessary information for authorization, including expiration times and cryptographic signatures.
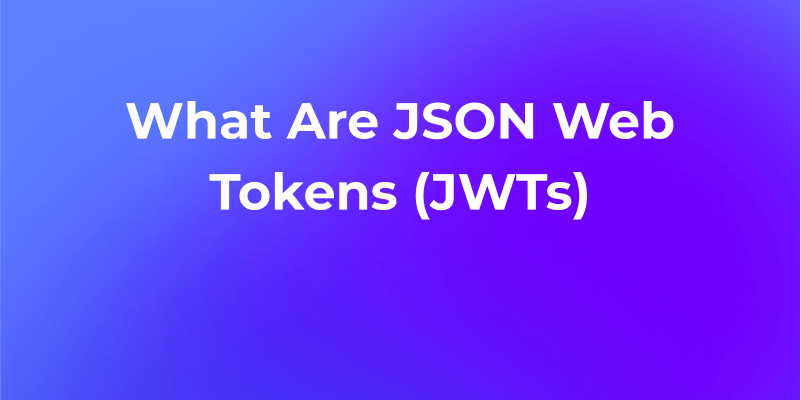
Basic Authentication
Basic authentication is a widely used method for authenticating API requests using a username and password. The username and password are transmitted in the HTTP header, which is encrypted over HTTPS. This method is simple to implement and widely supported by web servers and browsers. However, it's not suitable for applications that require high security, as the username and password can be easily intercepted.
How to Implement Authorization in APIs
Depending on the specific use case and security requirements, there are several ways to achieve authorization in APIs. However, the following are some of the general steps that can be taken to enforce authorization in APIs.
The UI clearly displays the token's expiration date, and you can refresh the token with one click when needed. This makes testing APIs that require OAuth 2.0 authentication extremely convenient within Apidog's powerful API management platform.
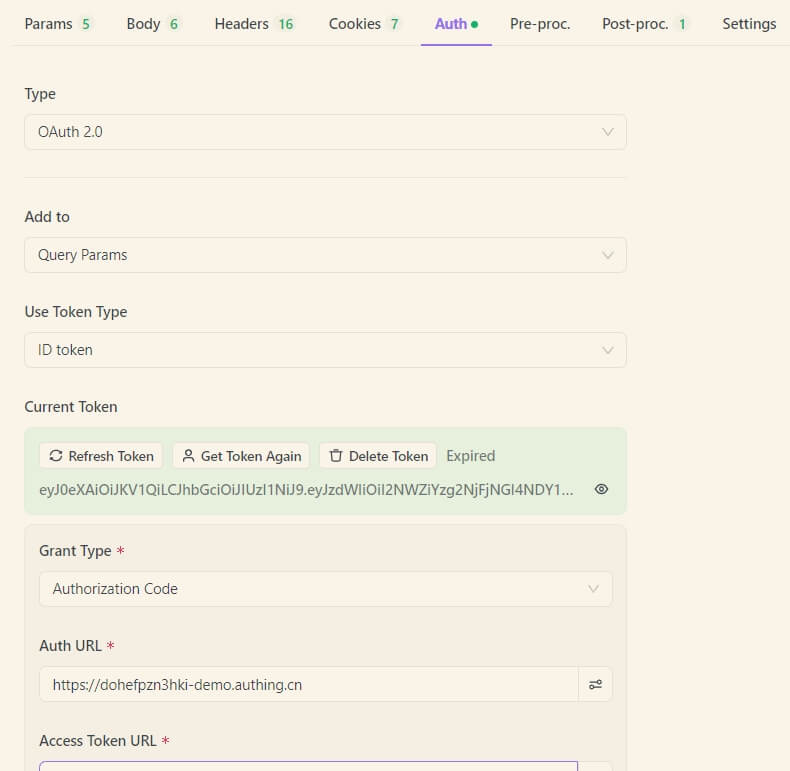
Determine the Authorization Method
Choose the best method for your use case and security requirements. Consider factors such as the level of security required, the complexity of the API, and the nature of the shared data.
Implement Authorization
Implement the chosen authorization method in the API code. This typically involves adding authentication and authorization checks to the API endpoints. For example, using OAuth 1.0, you would need to implement the OAuth 1.0 framework in the API code and generate access tokens for authorized clients.
Secure the API
After implementing the authorization method, it's important to secure the API against common security threats. This includes implementing secure coding practices, encryption to protect sensitive data, and rate limiting to prevent API abuse.
Test the Authorization
Testing the authorization is critical to ensure that only authorized users or clients can access the API. It involves testing different scenarios, such as incorrect credentials, expired tokens, and unauthorized access attempts, to ensure that the API is secure.
However, the user's choice of an appropriate tool for implementing API authorization is equally important. Let’s head on to the next section to explore more!
Apidog: All-in-One Workspace for APIs
Apidog is an API documentation and testing tool that helps developers design, document, debug, test, and mock their APIs. It is designed to simplify the process of creating and managing APIs by providing an intuitive and user-friendly interface. Apidog is available in both cloud-based and self-hosted versions and supports multiple programming languages and API frameworks.
Authorization Techniques Explained Using Apidog
There are several types of authorization techniques as mentioned above. Now, we will see three examples of authorization techniques using Apidog.
Basic Authorization
Basic Authorization is a simple authorization method that involves sending a username and password with each API request. The username and password are encoded in Base64 format and sent as part of the Authorization header. The server then verifies the credentials and grants access to the resource if they are valid.
Here is an example of how to implement Basic Authorization in Apidog:
1. Open Apidog and create a new API endpoint. Select the API request you want to perform, like GET, PUT, POST, etc., and enter the field you want to access, like studentId, as highlighted in the image below.
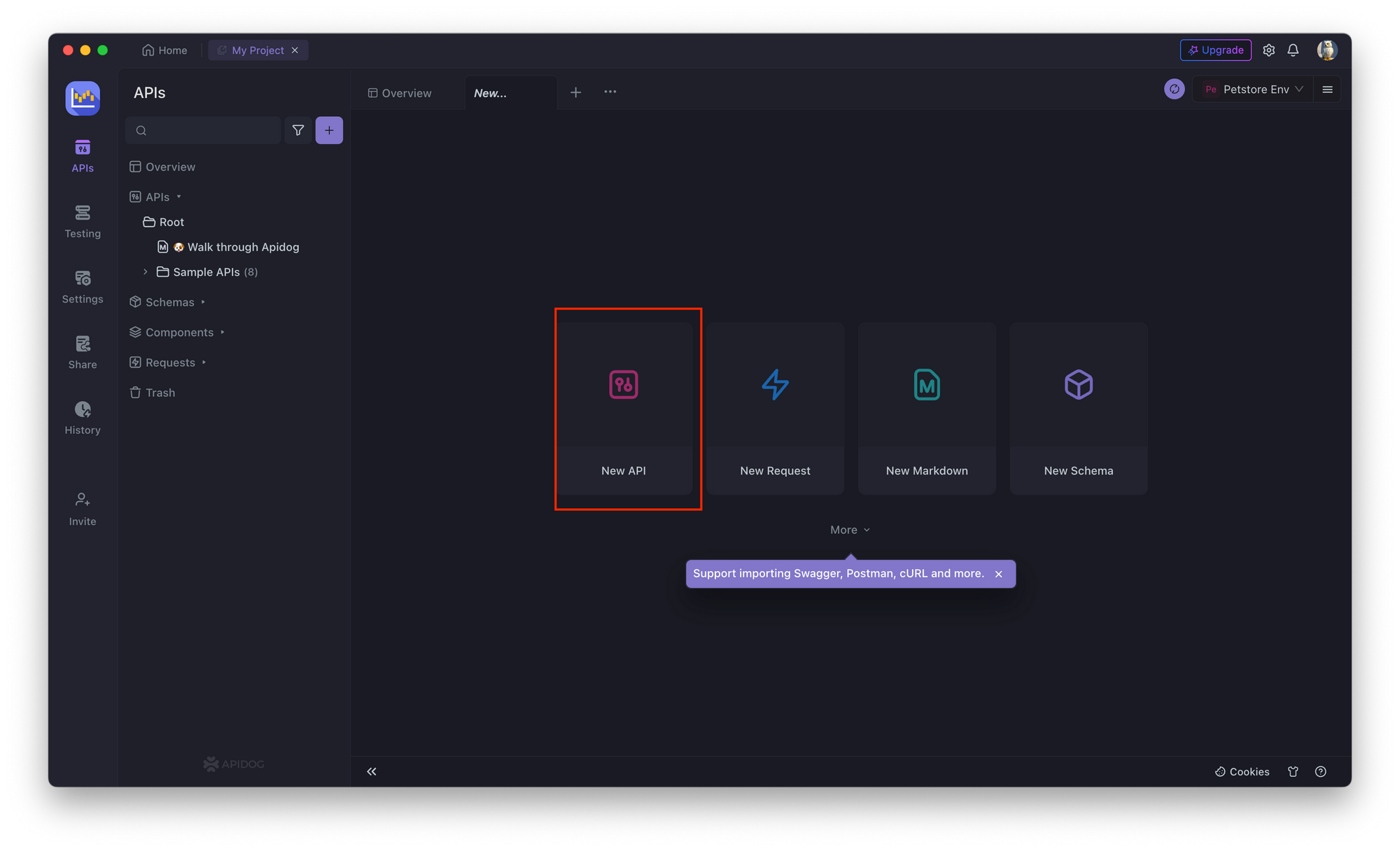
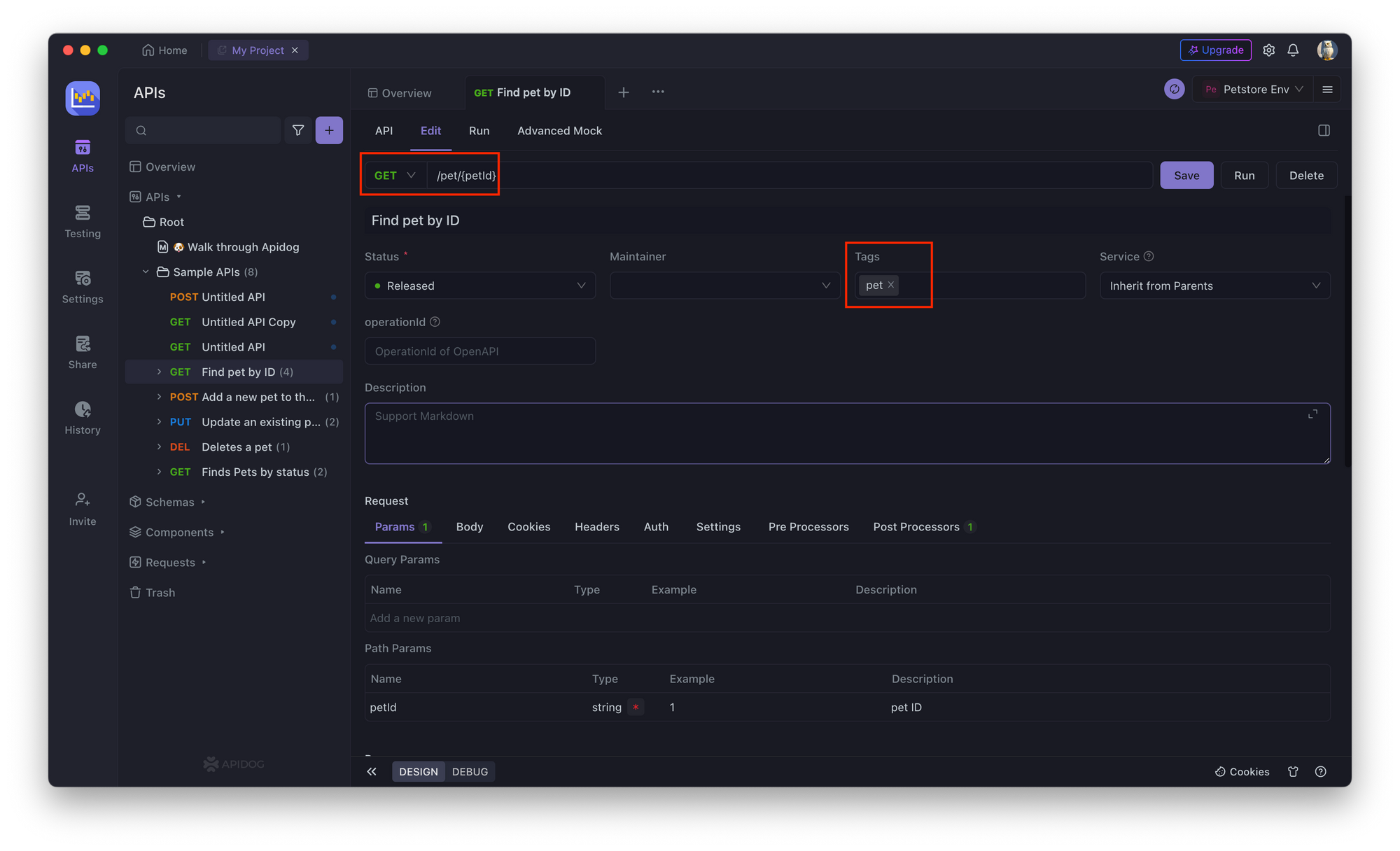
2. In the API endpoint, go to the Authorization tab and select Basic Authorization.
3. Enter a username and password for the API endpoint.
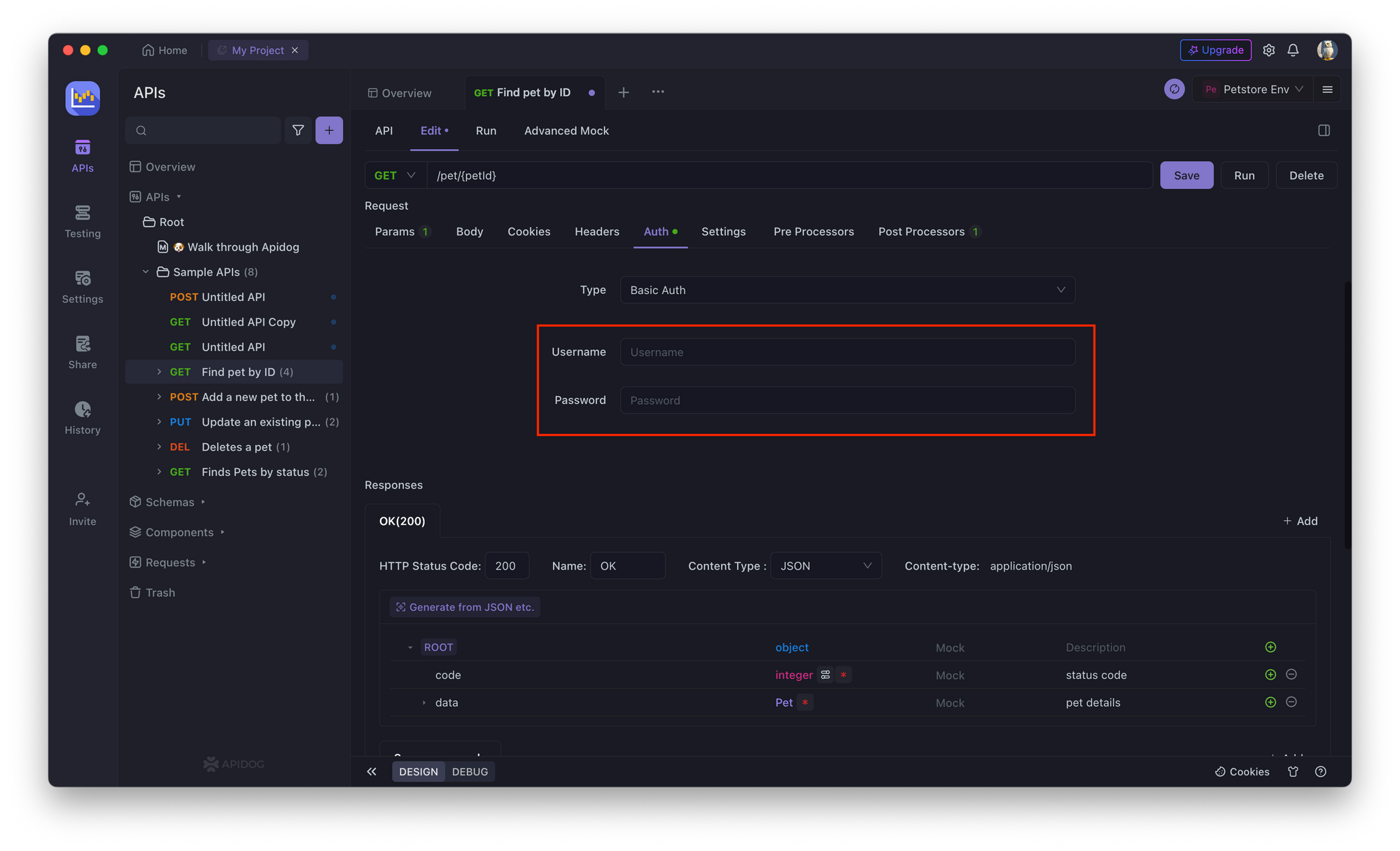
4. Save the changes and test the API endpoint by clicking on the Save
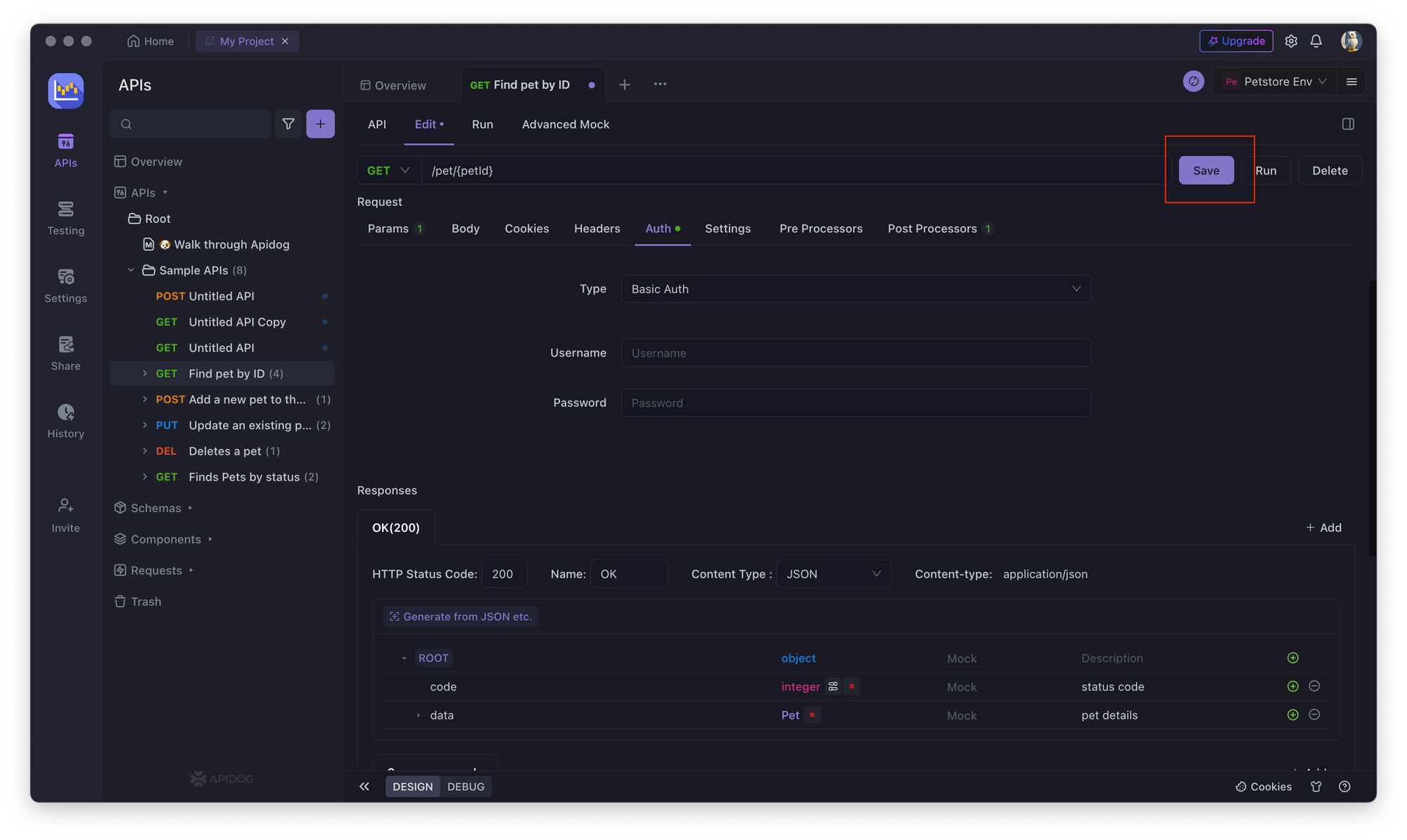
API Key Authorization
API Key Authorization is another popular method that involves generating a unique API key for each user or application. The API key is sent with each API request as part of the Authorization header or query parameter. The server then verifies the API key and grants access to the resource if it is valid.
Here is an example of how to implement API Key Authorization in Apidog:
GET /api/v1/users/1234 HTTP/1.1
Host: 127.0.0.1
Authorization: APIKEY 0123456789ABCDEF
In this example, the API key is provided in the Authorization header using the APIKEY scheme. The general steps for applying this authorization in Apidog are as follows:
1. Open Apidog and create a new API endpoint. Select the API request you want to perform, like GET, PUT, POST, etc., and enter the field you want to access, like studentId, as highlighted in the image below.
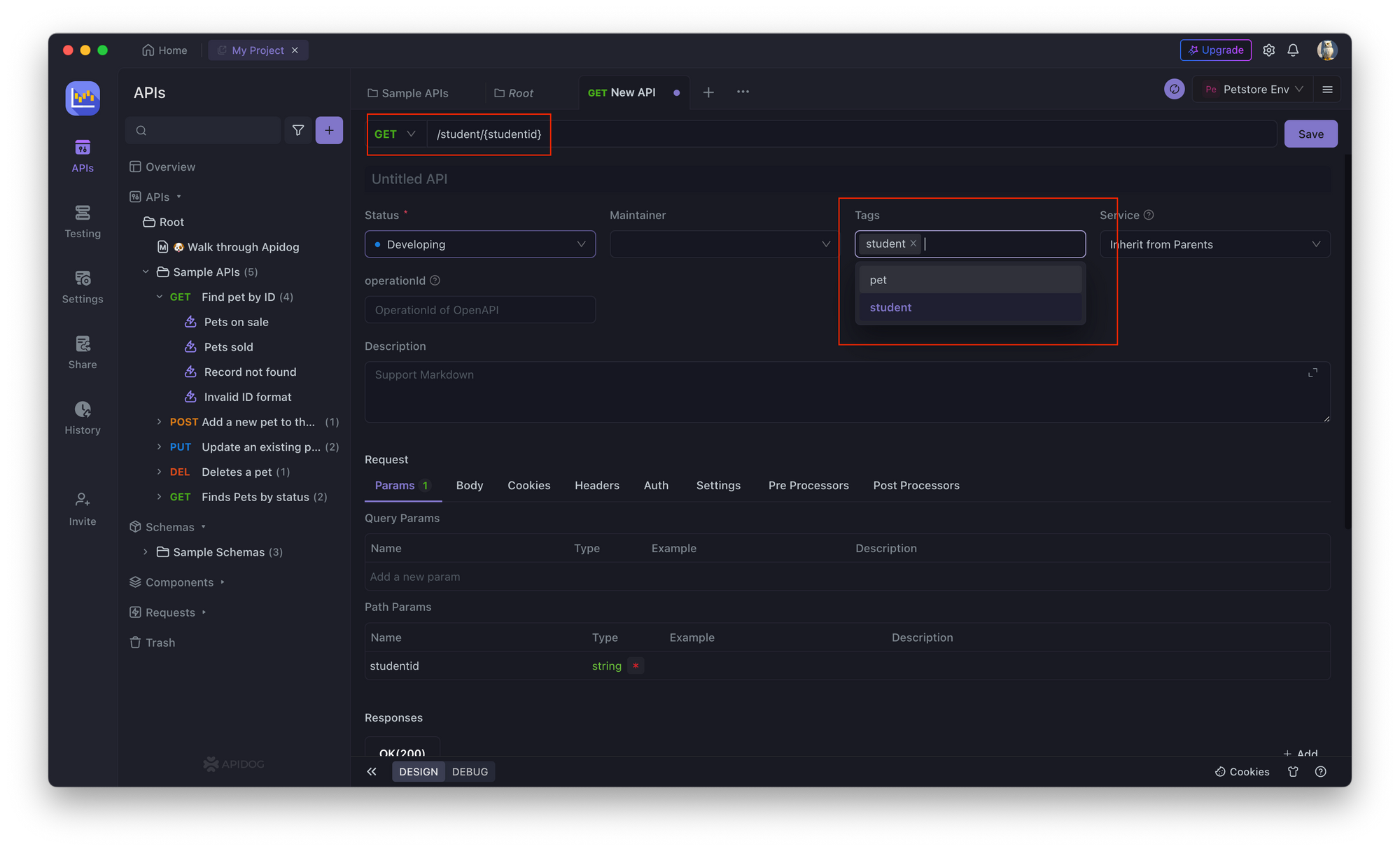
2. In the API endpoint, go to the Authorization tab and select API Key.
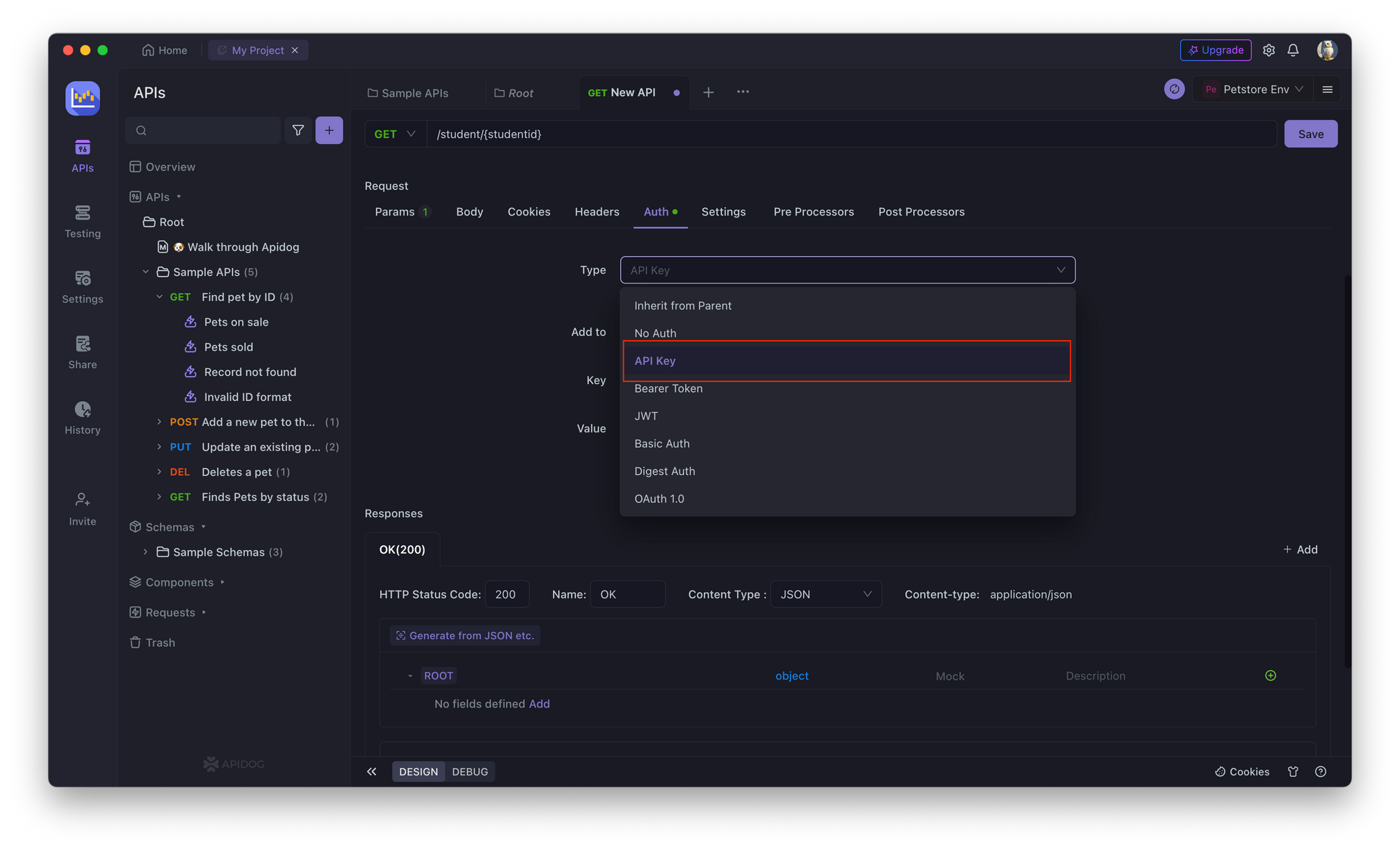
3. Enter a unique API key for the API endpoint.
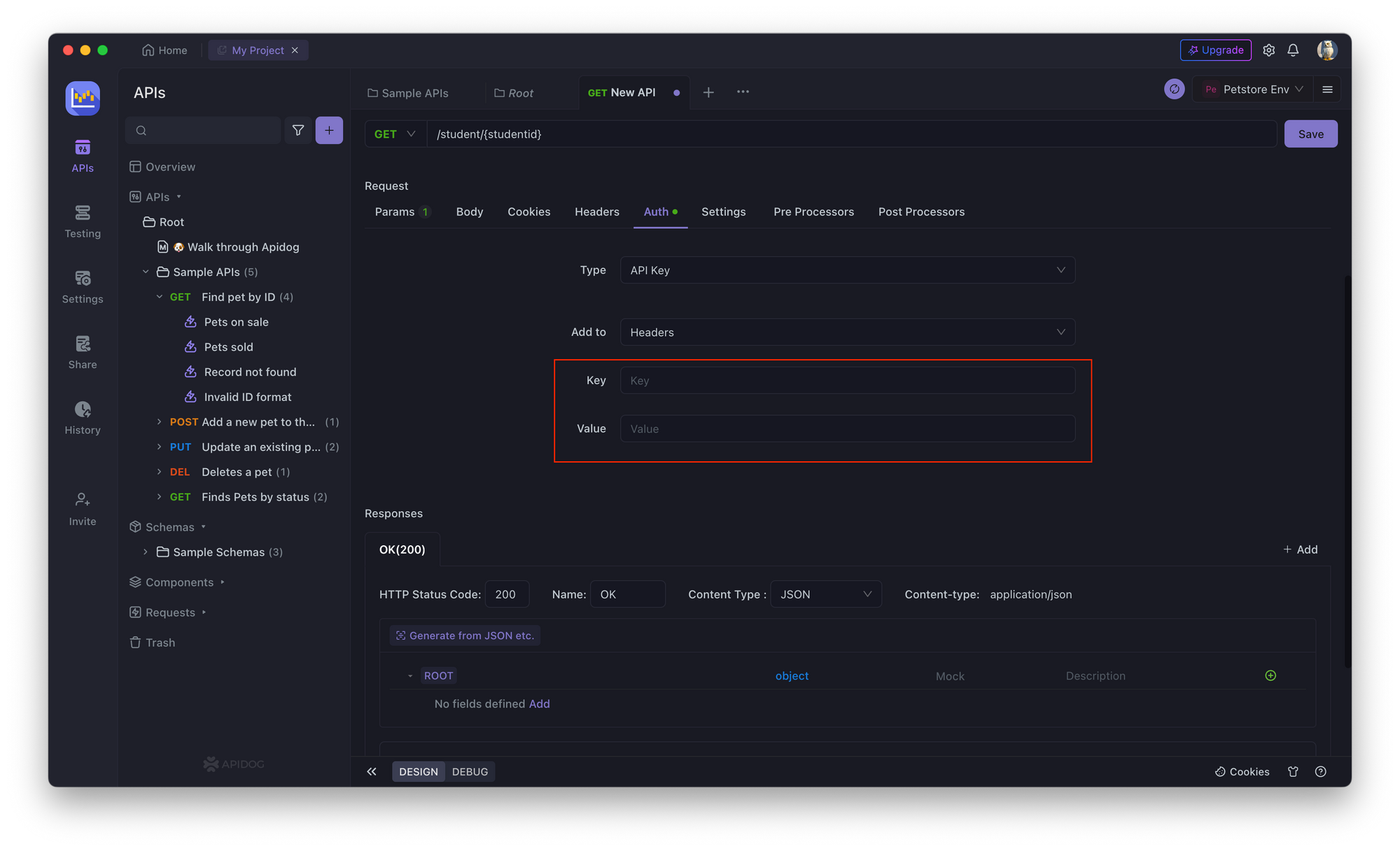
4. Save the changes and test the API endpoint.
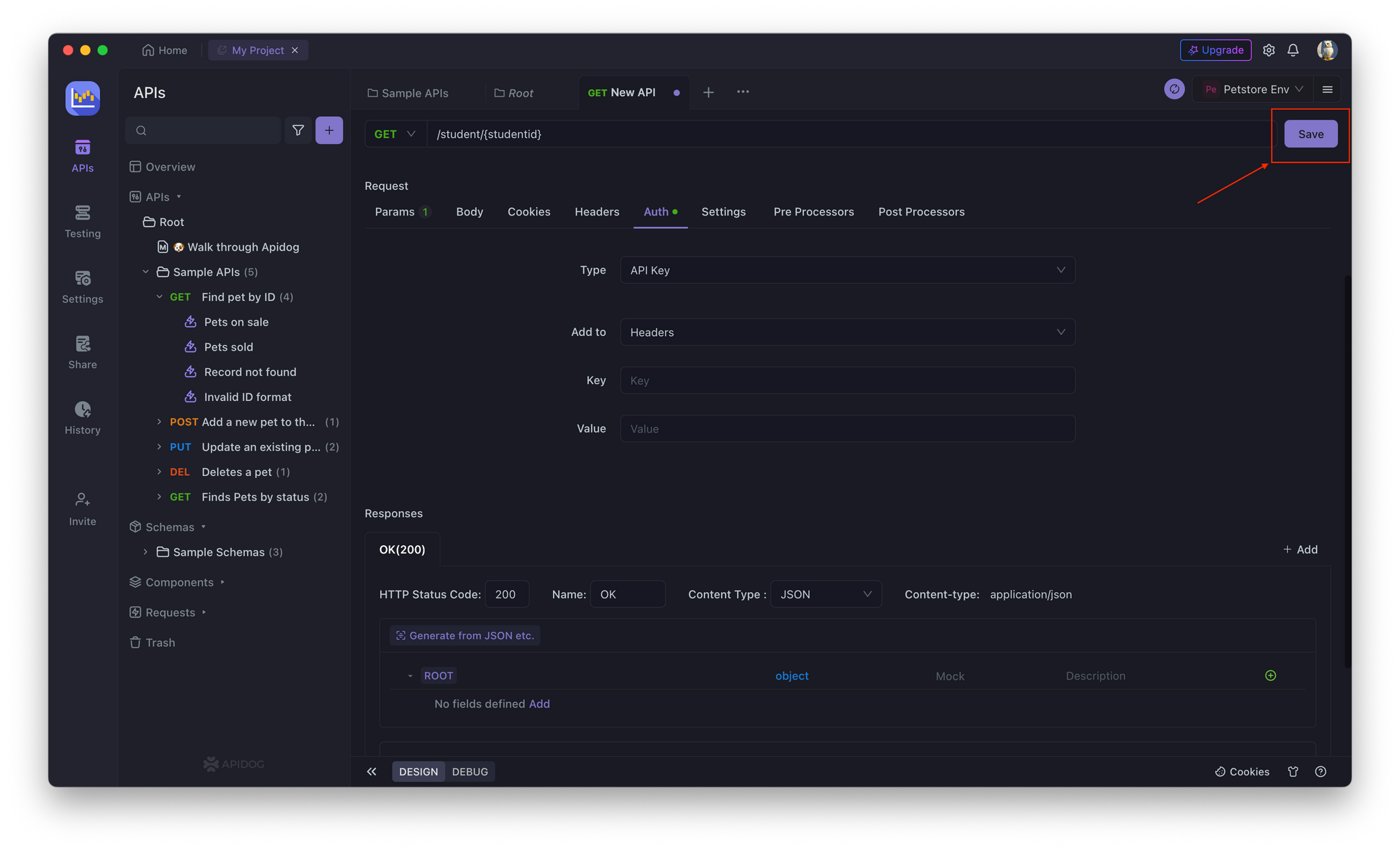
OAuth 1.0 Authorization
OAuth 1.0 Authorization is a more advanced authorization method that involves delegating access to resources from one application to another. It is commonly used in third-party applications that require user data access. OAuth 1.0 uses access tokens to grant access to resources, and it supports several grant types, including authorization codes, client credentials, and passwords.
Here is an example of OAuth 1.0 authorization in Apidog:
POST /token HTTP/1.1
Host: 127.0.0.1
Content-Type: application/x-www-form-urlencoded grant_type=password&username=abc&password=abc123
In this example, the client requests the API's token endpoint to obtain an access token. The client provides the user's credentials in the request body, and the API issues an access token if the credentials are valid. The general steps for applying this authorization in Apidog are as follows:
1. Open Apidog and create a new API endpoint. Select the API request you want to perform, like GET, PUT, POST, etc., and enter the field you want to access, like studentId, as highlighted in the image below.
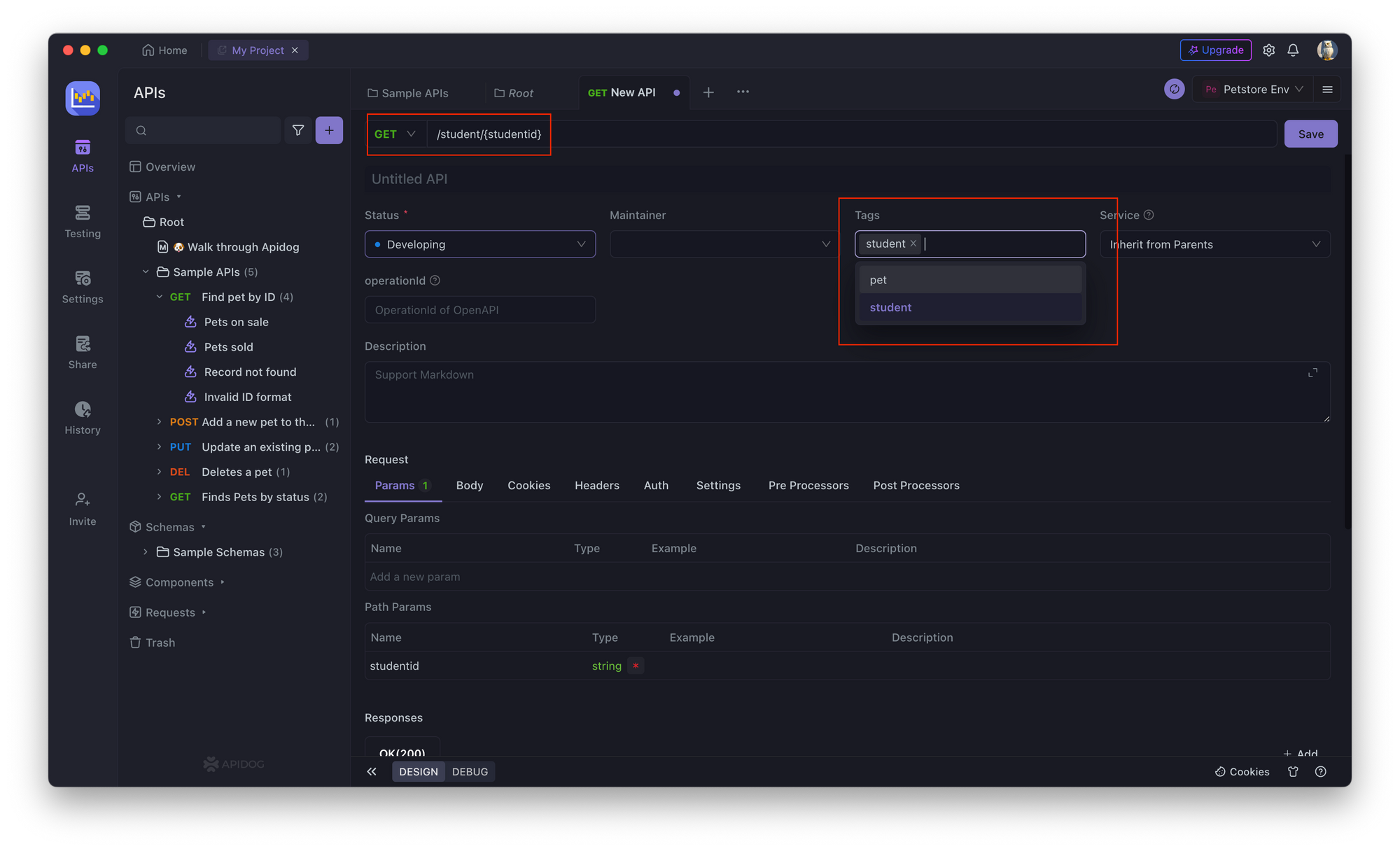
2. In the API endpoint, go to the Authorization tab and select OAuth 1.0.
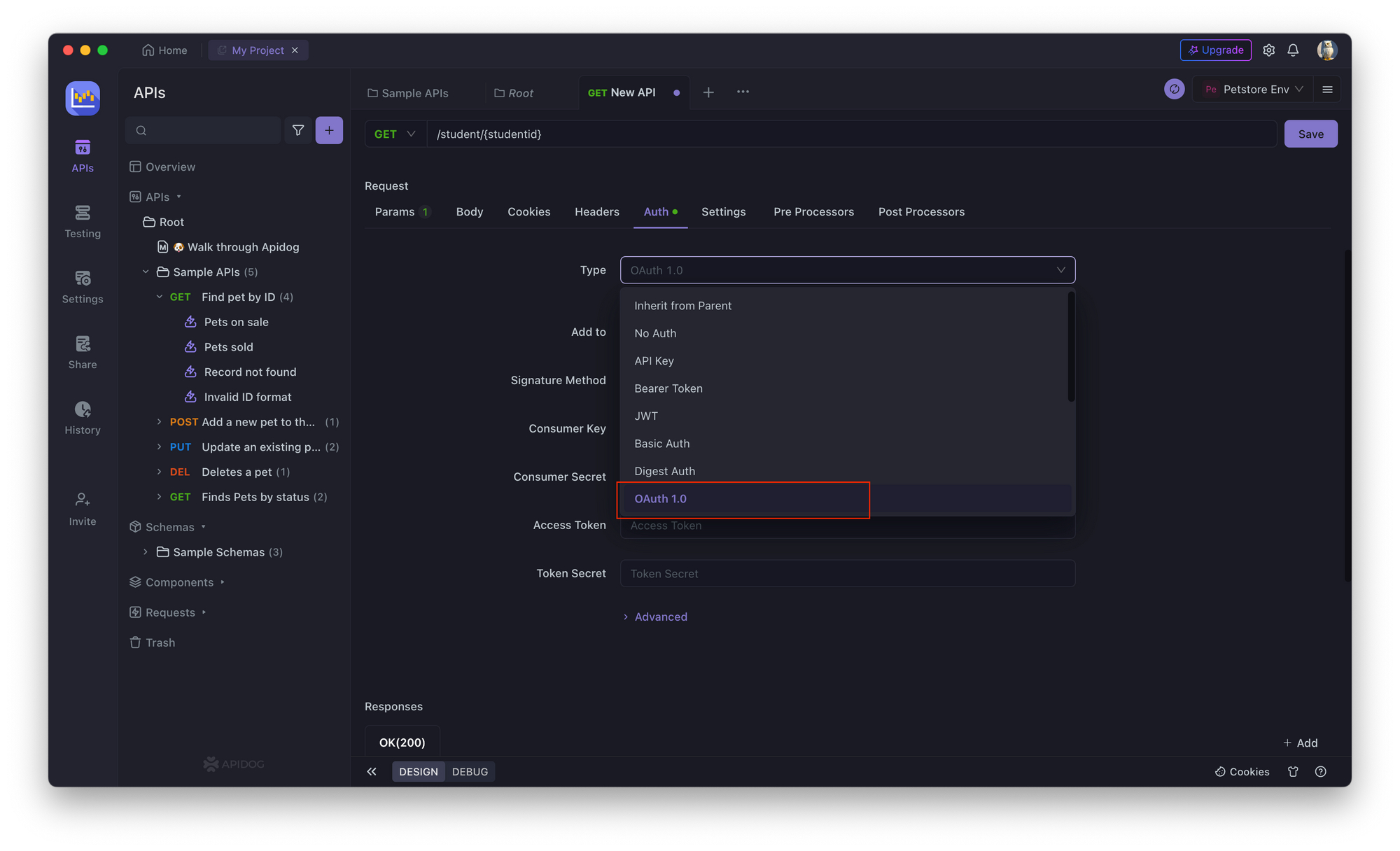
3. Enter the authorization endpoint (consumer key), token endpoint (access token), client ID (access token), and client secret (token secret) for the API endpoint.
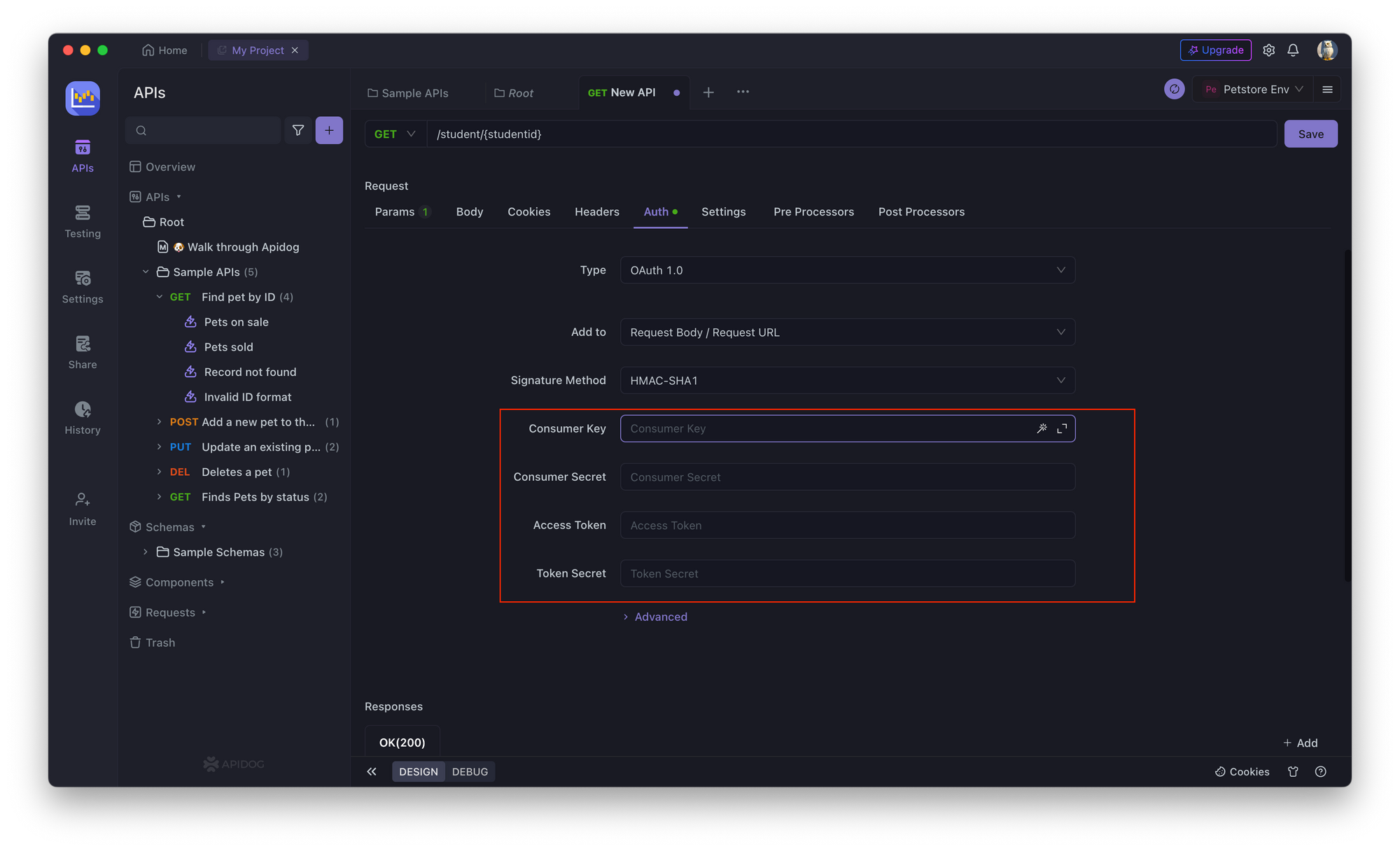
4. Save the changes and test the API endpoint.
JSON Web Tokens (JWT)
JSON Web Tokens (JWT) are a compact, URL-safe means of representing claims to be transferred between two parties. JWTs are often used as access tokens in APIs. They consist of three parts: a header, a payload, and a signature. The header and payload are Base64-encoded and separated by a period (.).
Here is an example of JWT authorization in Apidog:
GET /api/v1/users/1234 HTTP/1.1
Host: 127.0.0.1
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJz
The access token is provided in the Authorization header using the Bearer scheme in this example. The general steps for applying this authorization in Apidog are as follows:
1. Open Apidog and create a new API endpoint. Select the API request you want to perform, like GET, PUT, POST, etc., and enter the field you want to access, like studentId, as highlighted in the image below.
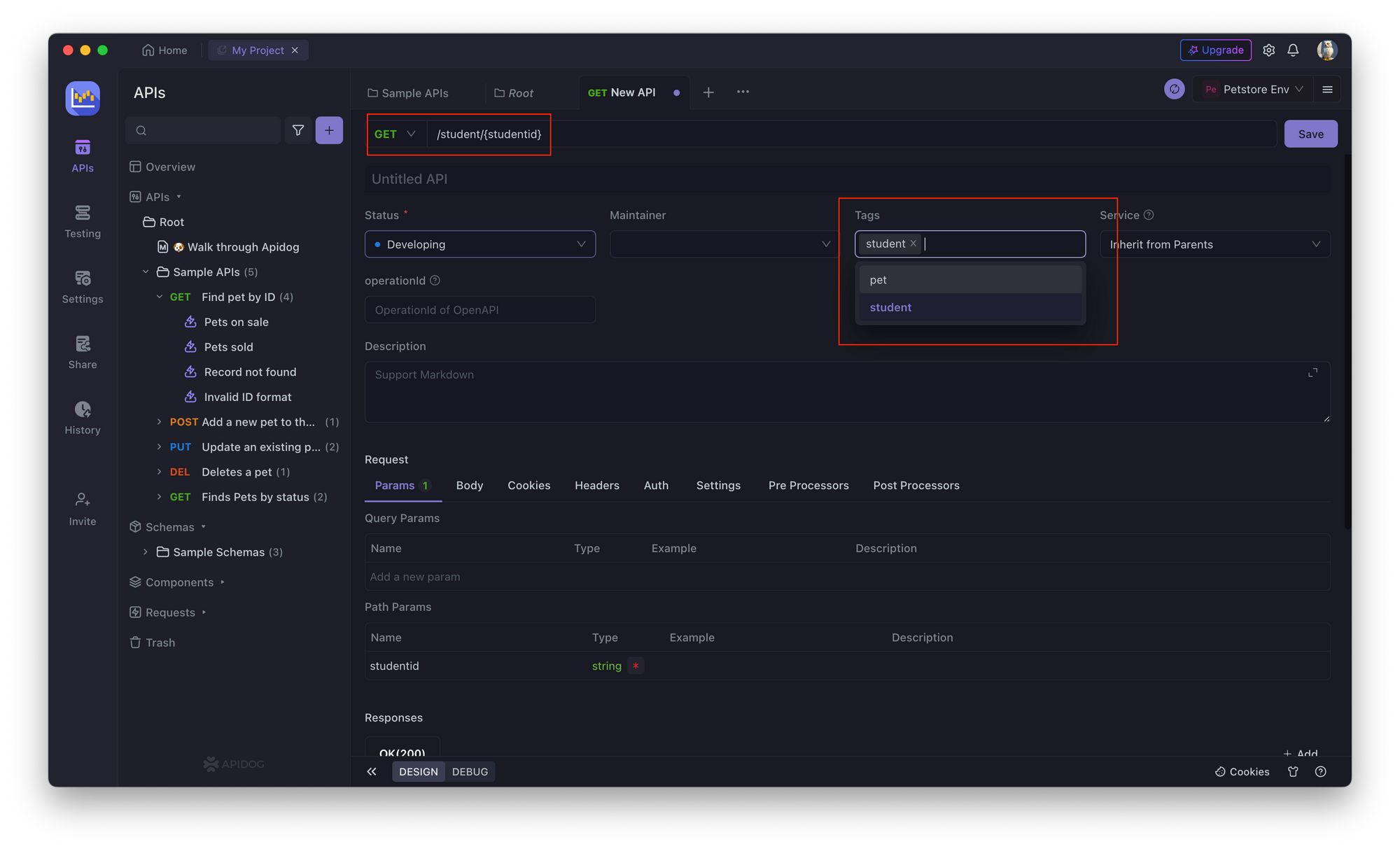
2. In the API endpoint, go to the Authorization tab and select Bearer Token.
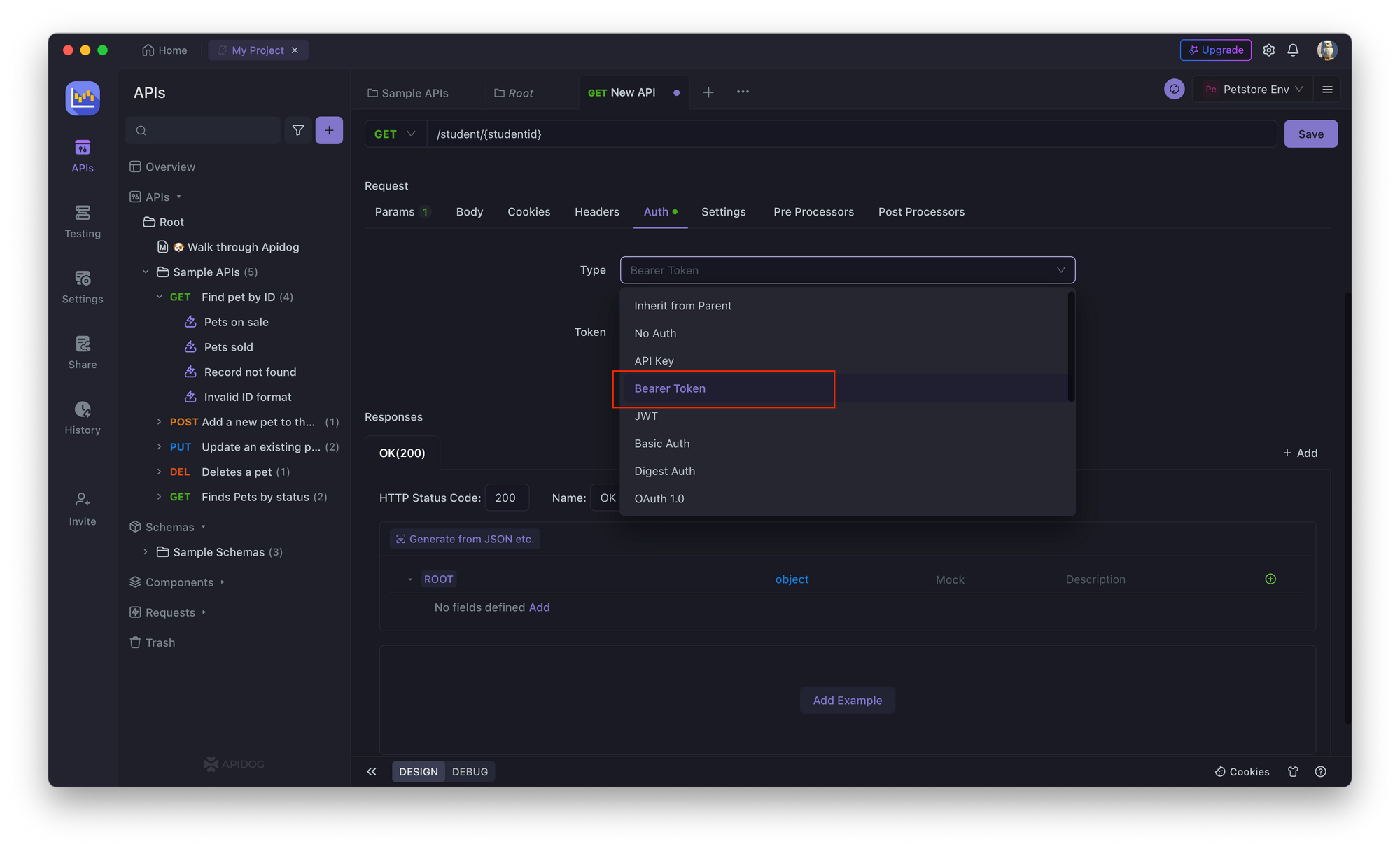
2. Enter the bearer token.
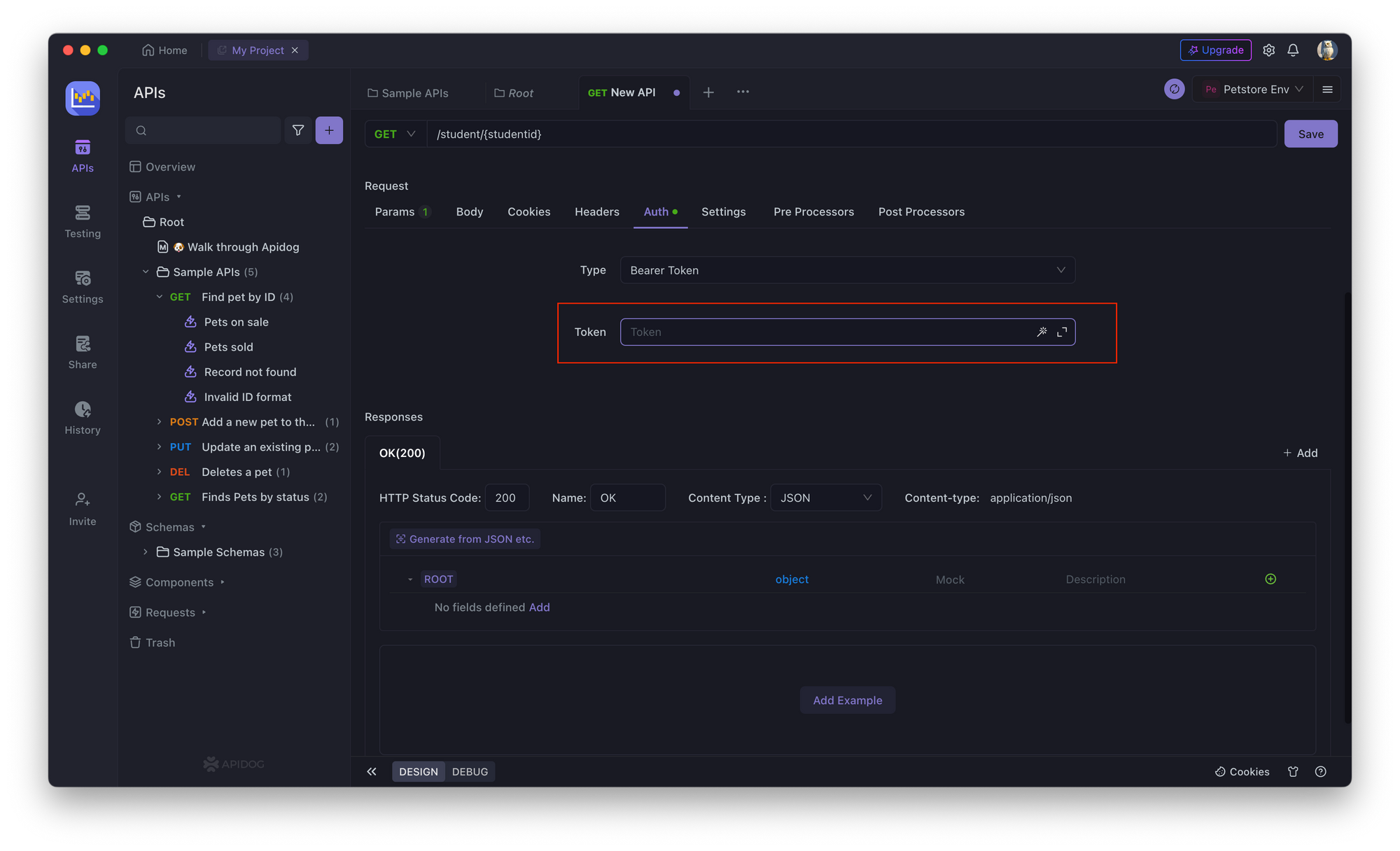
3. Save the changes and test the API endpoint.
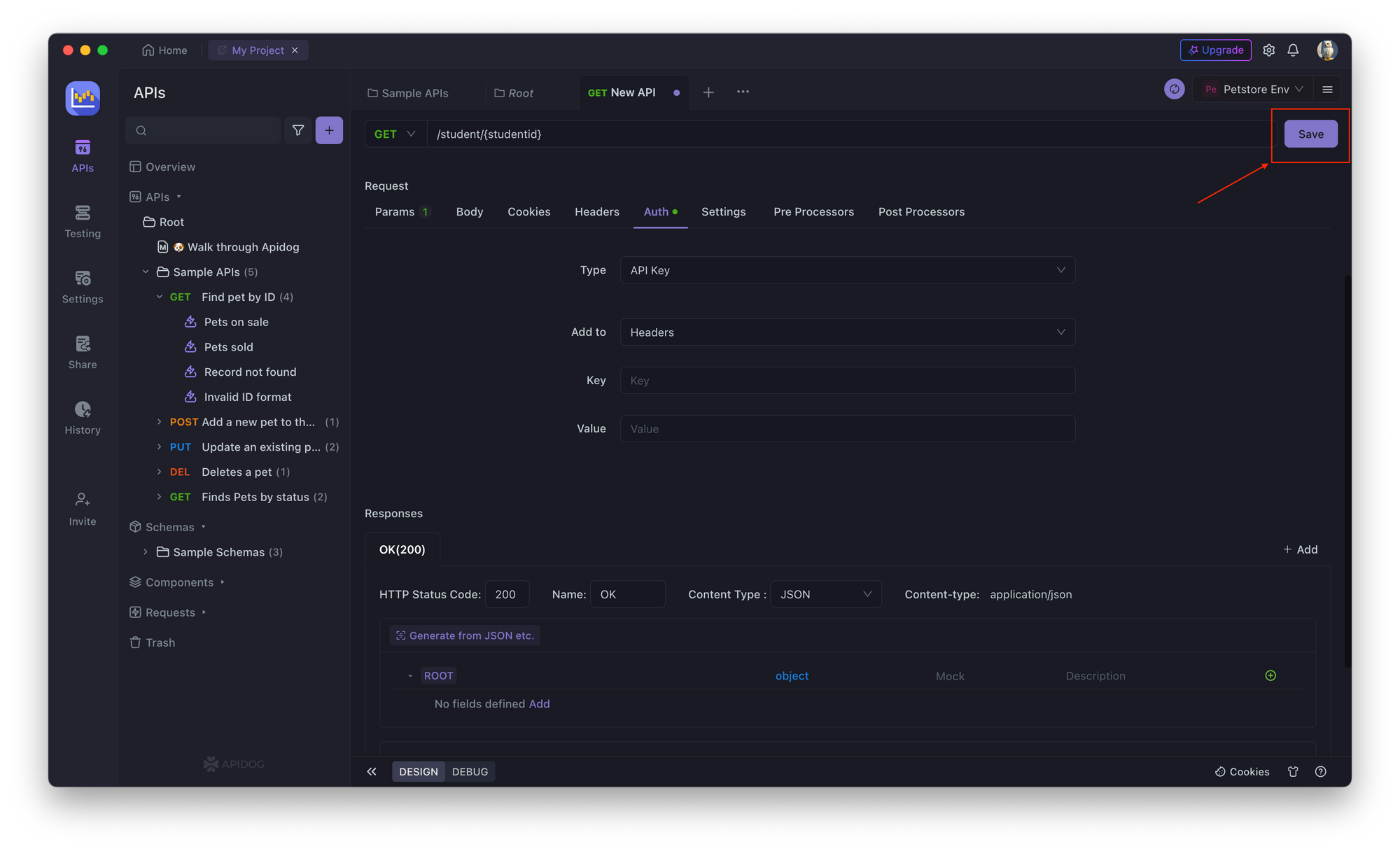
Best Practices for Authorization in APIs
Implementing authorization in APIs is not enough to ensure API security. Here are some best practices for authorization in APIs that can help increase security and reduce the risk of security breaches:
● Use Strong Authentication: Use strong authentication methods such as multi-factor authentication to prevent unauthorized access to the API.
● Limit Access: Limit access to the API to only the resources that users or clients need to perform their tasks. It reduces the risk of data loss and security breaches.
● Use HTTPS: Use HTTPS to encrypt data transmitted between the API and the client. It helps prevent data interception and man-in-the-middle attacks.
● Implement Rate Limiting: Implement rate limiting to prevent API abuse and protect against DDoS attacks.
● Use Short-Lived Tokens: Use short-lived access tokens to grant access to resources, and make sure to revoke them when they are no longer needed.
● Use API Key Management: Use API key management tools to manage and track API keys. It helps to prevent unauthorized access to your APIs and helps you to track usage.
● Regularly Monitor and Audit: Regularly monitor and audit the API to detect and prevent security breaches. This includes monitoring access logs and audit trails to detect suspicious activities.
Conclusion
Authorization is a crucial component of securing APIs and ensuring that only authorized users and applications can access sensitive data. By using Apidog to design, document, and test APIs, developers can easily implement various authorization methods, including Basic Authorization, API Key Authorization, and OAuth 1.0 Authorization.
Additionally, following best practices for authorization in APIs, such as using HTTPS, limiting access, and using short-lived tokens, can help to strengthen the security of APIs further. With Apidog's user-friendly interface and the ability to easily test and manage APIs, developers can confidently implement authorization in their APIs and help protect against unauthorized access to sensitive data.