Understanding aiohttp GET Requests: Asynchronous Solutions for Your Web Applications
Explore the power of asynchronous programming with our deep dive into aiohttp GET requests. Learn how to enhance your web applications with efficient, non-blocking HTTP operations.
In the evolving landscape of web development, efficiency and speed are paramount. Asynchronous programming has emerged as a critical strategy to enhance performance and user experience. Python’s aiohttp
library stands out as a powerful tool, enabling developers to handle asynchronous HTTP requests with ease. In this article, we delve into the mechanics of aiohttp
GET requests, illustrating how they can streamline your web applications.
What is aiohttp?
aiohttp is more than just an HTTP client/server framework. It’s a versatile tool that integrates seamlessly with Python’s asyncio
library, enabling developers to write concurrent code that is both efficient and readable. By supporting asynchronous request handling, aiohttp
ensures that your web services can handle a large number of simultaneous connections without breaking a sweat.
The Asynchronous Advantage
Asynchronous programming allows a program to handle multiple tasks seemingly at once. For web services, this means being able to process other requests while waiting for responses from databases or APIs, thus avoiding the dreaded performance bottlenecks associated with synchronous code.
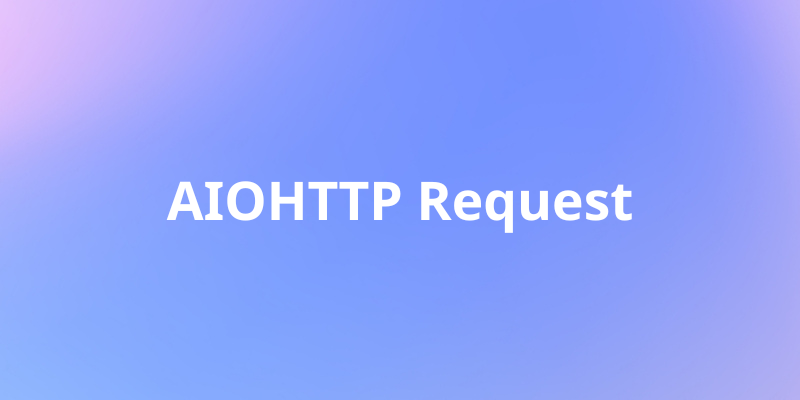
GETting Started with aiohttp
GET requests are the bread and butter of HTTP operations, tasked with retrieving data from servers. aiohttp
shines in this area, providing a simple yet powerful interface for sending GET requests and handling responses.
Example: Fetching Data Asynchronously
To demonstrate the power of aiohttp
GET requests, let’s look at a simple example where we fetch data from an API:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch(session, 'http://python.org')
print(html)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
In this snippet, we define an asynchronous function fetch
that performs a GET request to the specified URL. The main
function then creates a client session and calls fetch
to retrieve the content from Python’s official website.
How to send aiohttp get request with Apidog?
To send an aiohttp
GET request using Apidog, you would typically start by setting up your Apidog environment for API development and testing. Apidog is an all-in-one collaborative API development platform that can help you design, document, debug, mock, and automatically test your APIs.
Set Up Your Apidog Project:
- Open Apidog and create a new project.
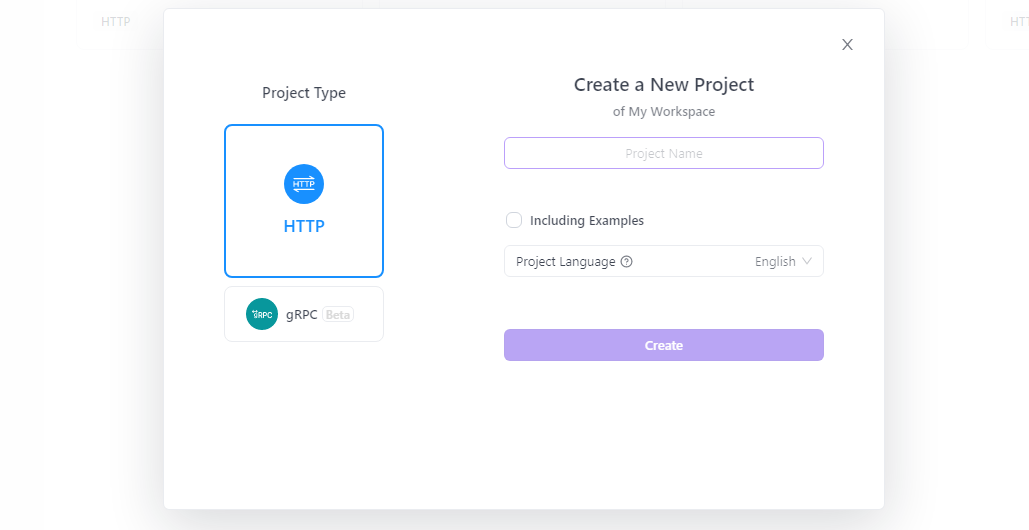
Write Your aiohttp GET Request Code:
- Use
aiohttp
to write asynchronous code for sending GET requests. - Here’s a basic example of how you might structure your
aiohttp
GET request in Python:
import aiohttp
import asyncio
async def get_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
# Replace 'your_api_endpoint' with the actual endpoint you've set up in Apidog
url = 'your_api_endpoint'
loop = asyncio.get_event_loop()
loop.run_until_complete(get_data(url))
Test Your GET Request in Apidog:
- Within Apidog, navigate to the API debugging or testing section.
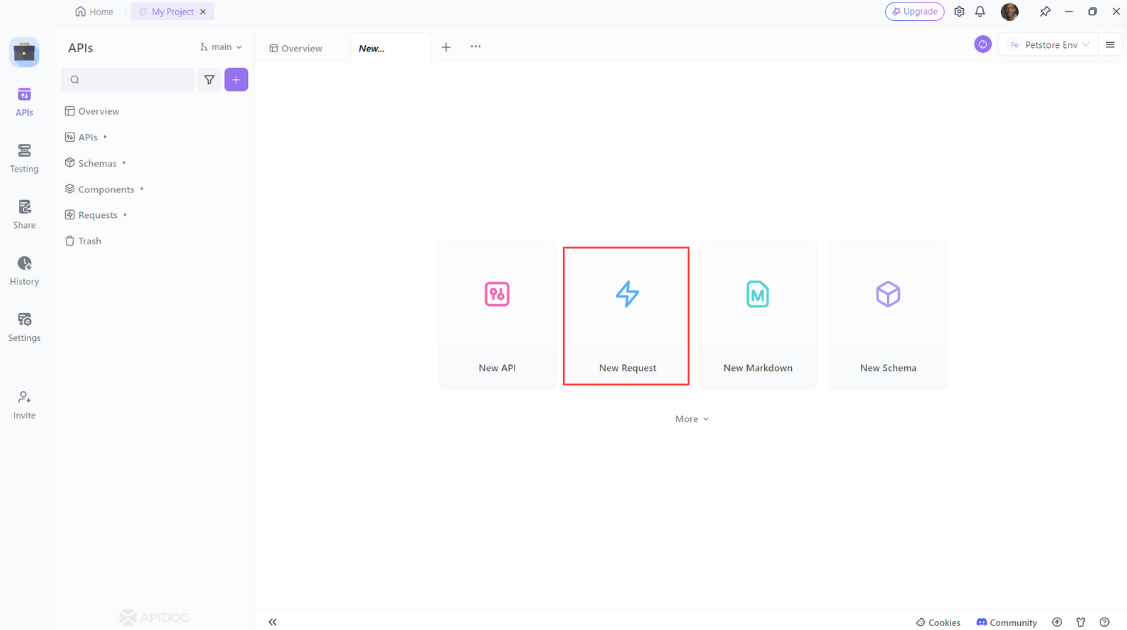
- Input the details of your GET request, including the endpoint and any necessary headers or parameters.
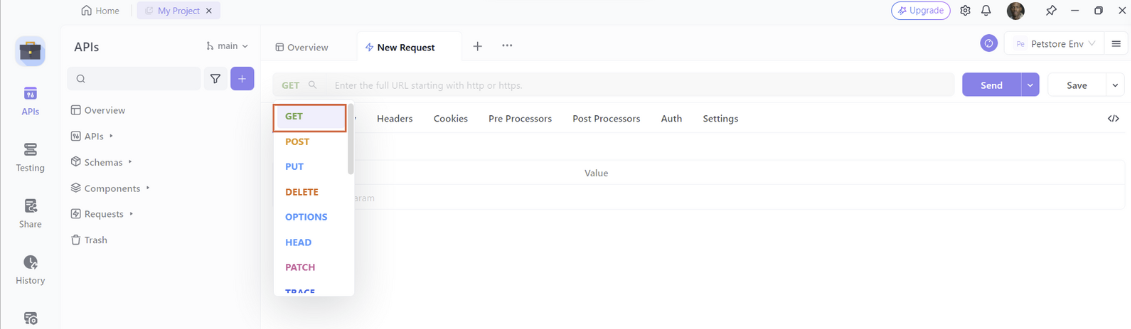
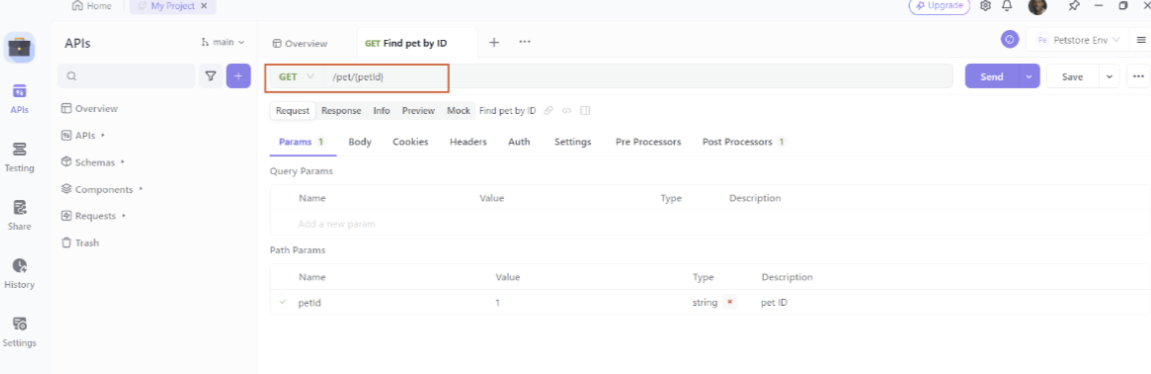
- Send the request and analyze the response directly within Apidog.
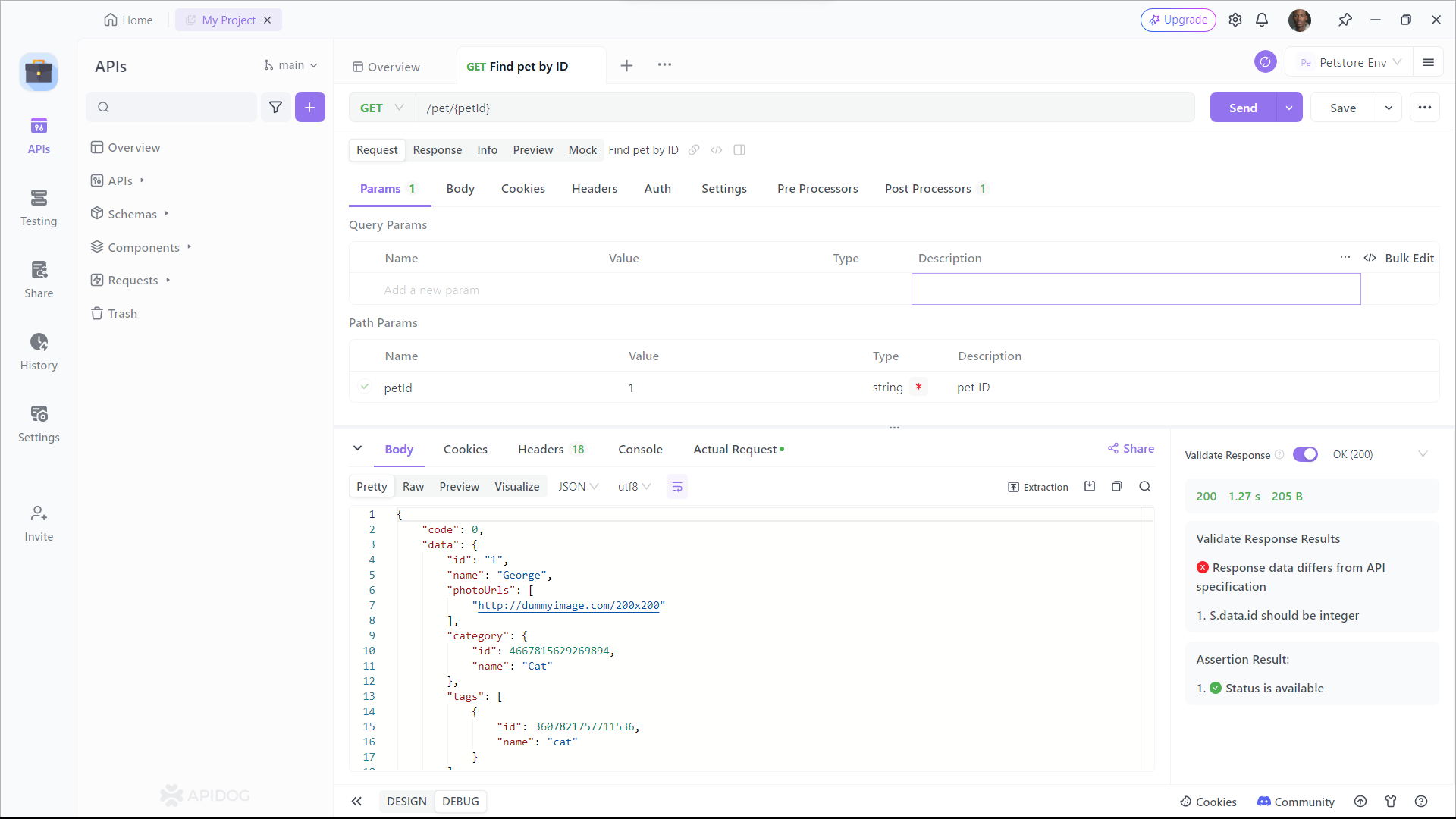
Iterate and Refine:
- Based on the response, make any necessary adjustments to your
aiohttp
code or your API configuration in Apidog. - Repeat the testing process until you achieve the desired outcome.
Conclusion
As we’ve explored the capabilities of aiohttp
for handling GET requests, it’s clear that asynchronous programming is a game-changer for developing high-performance web applications. By utilizing aiohttp
, you can ensure that your services are scalable, efficient, and ready to meet the demands of modern web traffic.
Tools like Apidog further streamline the development process, offering a collaborative platform for designing, testing, and refining your APIs. With aiohttp
and Apidog, you’re well-equipped to build and maintain robust web services that stand the test of time and technology.