Making an AIOHTTP Request to Any API
AIOHTTP empowers Python devs with asynchronous HTTP requests, ideal for blasting through high volumes of web interactions. It utilizes non-blocking I/O and connection pooling to handle numerous requests concurrently, maximizing performance.
AIOHTTP is an asynchronous HTTP client library for Python, allowing its users to make asynchronous requests to web servers. With AIOHTTP, you can interact with web APIs and services with ease. However, what comprises an AIOHTTP request?
To speed up the Python coding process, try out Apidog, a comprehensive API development tool that code generation features for client code language. Within a few clicks, you will have your code ready for implementation!
If you are interested in trying Apidog, click the button below to begin! 👇 👇 👇
What are AIOHTTP Requests?
AIOHTTP requests are requests used for making a large number of HTTP requests concurrently and efficiently. This means you do not need to make requests individually, thus saving much time.
AIOHTTP Requests' Key Features
AIOHTTP requests have a few specialties that you should be aware of.
1. Asynchronous Operations:
- Non-Blocking I/O (Input/Output): AIOHTTP leverages asynchronous I/O, meaning it doesn't wait for a single request to finish before starting another. This allows it to handle multiple requests concurrently, improving performance compared to traditional synchronous libraries.
- Event Loop Integration: AIOHTTP works seamlessly with Python's asyncio library and integrates with the event loop. The event loop efficiently manages concurrent tasks and network I/O, ensuring the smooth execution of multiple requests.
2. Flexible Request Management:
- HTTP Methods Support: AIOHTTP supports all standard HTTP methods like GET, POST, PUT, DELETE, etc., allowing you to interact with web servers in various ways.
- Customizable Headers & Data: You have full control over request headers and data. You can specify custom headers like authorization tokens or content type, and send data in various formats like JSON, form data, or raw bytes.
- Timeouts and Retries: Define timeouts for requests to prevent hanging applications. Additionally, AIOHTTP allows implementing retry logic for failed requests, improving robustness.
3. Advanced Features:
- Streaming Responses: AIOHTTP allows streaming large responses in chunks, processing data incrementally without loading the entire response into memory. This is beneficial for handling large files or real-time data streams.
- Proxies: You can configure AIOHTTP to use proxies for making requests, providing additional flexibility for network routing or security purposes.
- Cancellation: Requests can be canceled before completion, allowing you to gracefully stop processing if needed.
4. Integration and Ecosystem:
- Asynchronous Framework Compatibility: AIOHTTP integrates well with asynchronous web frameworks like Quart or Sanic, enabling efficient handling of HTTP requests within these frameworks.
- Third-Party Libraries: A rich ecosystem of libraries built on top of AIOHTTP exists, providing functionalities like JSON parsing, authentication handling, and more.
AIOHTTP Requests' Key Strengths Over its Alternatives
1. Superior Performance for Concurrent Requests:
- Non-Blocking I/O: Unlike synchronous libraries like Requests, AIOHTTP utilizes non-blocking I/O. This allows it to initiate multiple requests simultaneously, improving performance significantly compared to waiting for each request to finish before starting the next.
- Event Loop Integration: AIOHTTP seamlessly integrates with Python's asyncio library and event loop. This ensures efficient management of concurrent tasks and network I/O, maximizing resource utilization and minimizing waiting times.
2. Powerful ClientSession Management:
- Connection Pooling: AIOHTTP's ClientSession maintains a pool of reusable connections. Instead of establishing new connections for every request, it reuses existing ones whenever possible. This significantly reduces connection overhead, leading to faster communication.
- Simplified State Management: ClientSession automatically handles cookies between requests within a session. This eliminates the need for manual cookie management, simplifying state handling for authenticated interactions.
- Robust Error Handling: ClientSession acts as a context manager, ensuring proper connection closure even if exceptions occur. This prevents resource leaks and improves application reliability.
3. Advanced Features and Flexibility:
- Streaming Responses: AIOHTTP allows you to process large responses in chunks. This is particularly beneficial for handling massive files or real-time data streams, as it avoids loading the entire response into memory at once.
- Proxy Support: You can configure AIOHTTP to use proxies for making requests. This provides additional flexibility for network routing or security purposes.
- Cancellation Capabilities: Requests can be canceled before completion. This allows for graceful termination of operations if needed, adding another layer of control.
4. Rich Ecosystem and Integrations:
- Asynchronous Framework Compatibility: AIOHTTP integrates well with asynchronous web frameworks like Quart or Sanic. This enables efficient handling of HTTP requests within these frameworks, leading to performant and scalable web applications.
- Third-Party Library Support: A rich ecosystem of libraries built on top of AIOHTTP exists. These libraries offer functionalities like JSON parsing, authentication handling, and more, extending AIOHTTP's capabilities for various use cases.
AIOHTTP Requests Coding Examples
1. Simple GET request (httpbin.org is a popular service for testing HTTP requests):
import asyncio
async def fetch_data():
async with aiohttp.ClientSession() as session:
async with session.get('https://httpbin.org/get') as response:
data = await response.json()
print(data)
asyncio.run(fetch_data())
Code explanation:
This code fetches data from the /get
endpoint of httpbin.org using a GET request. The response is later parsed as JSON, and printed.
2. POST request with data:
import asyncio
async def send_data():
async with aiohttp.ClientSession() as session:
data = {'key': 'value'}
async with session.post('https://api.example.com/data', json=data) as response:
response.raise_for_status() # Raise an exception for non-2xx status codes
print(await response.text())
asyncio.run(send_data())
Code explanation:
This example sends a POST request to an API endpoint https://api.example.com/data
with JSON data ( data
dictionary). The raise_for_status()
method is there to ensure an exception is raised for any unsuccessful response (status code outside the 2xx range).
3. Downloading a file:
import asyncio
import aiohttp
async def download_file():
async with aiohttp.ClientSession() as session:
async with session.get('https://example.com/file.zip', stream=True) as response:
response.raise_for_status()
with open('file.zip', 'wb') as f:
async for chunk in response.content():
f.write(chunk)
print("Download complete!")
asyncio.run(download_file())
Code explanation:
The code downloads a file ( file.zip
) from a URL, and uses the stream=True
parameter to download the file in chunks, improving the memory efficiency for larger files.
4. Using headers and timeouts:
import asyncio
async def request_with_headers():
async with aiohttp.ClientSession() as session:
headers = {'Authorization': 'Bearer YOUR_TOKEN'}
async with session.get('https://api.example.com/private', headers=headers, timeout=5) as response:
# Process the response based on status code
if response.status == 200:
data = await response.json()
print(data)
else:
print(f"Error: {response.status}")
asyncio.run(request_with_headers())
Code explanation:
The code example shows sending a GET request with custom headers (authorization token(, and a timeout of 5 seconds.
Apidog - Generate AIOHTTP Python Client Code for your Application
If you are struggling with client-sided coding, worry no longer!
Introducing Apidog, an all-in-one API development tool that allows users to create APIs from scratch, test them, and even run modifications on existing APIs! Once you have finished designing your API, you can also proceed with generating beautiful API documentation for your API consumers to read.
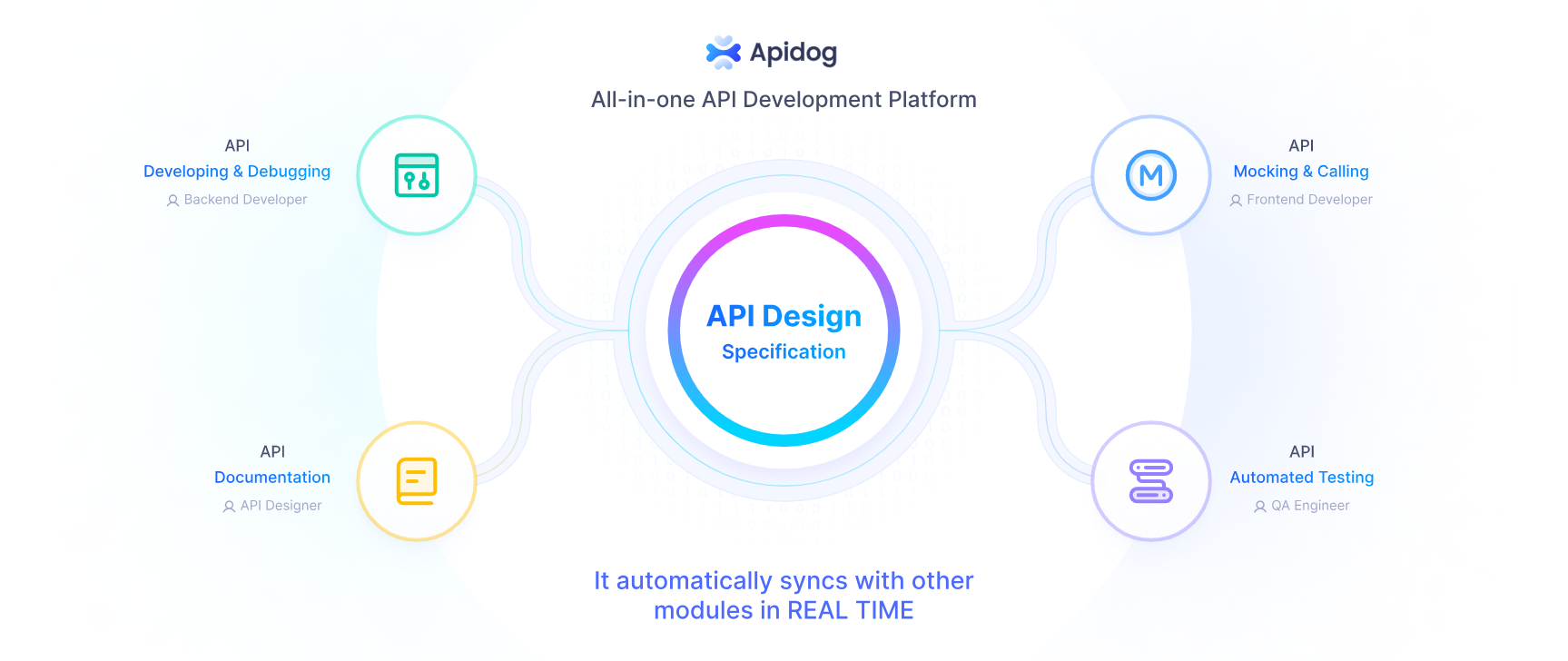
Apidog is particularly useful for newer developers learning how to create applications and APIs. Apidog's code generation feature can provide code templates within a few clicks of a button. Let's see how to do it on Apidog!
Generating AIOHTTP Python Code Using Apidog
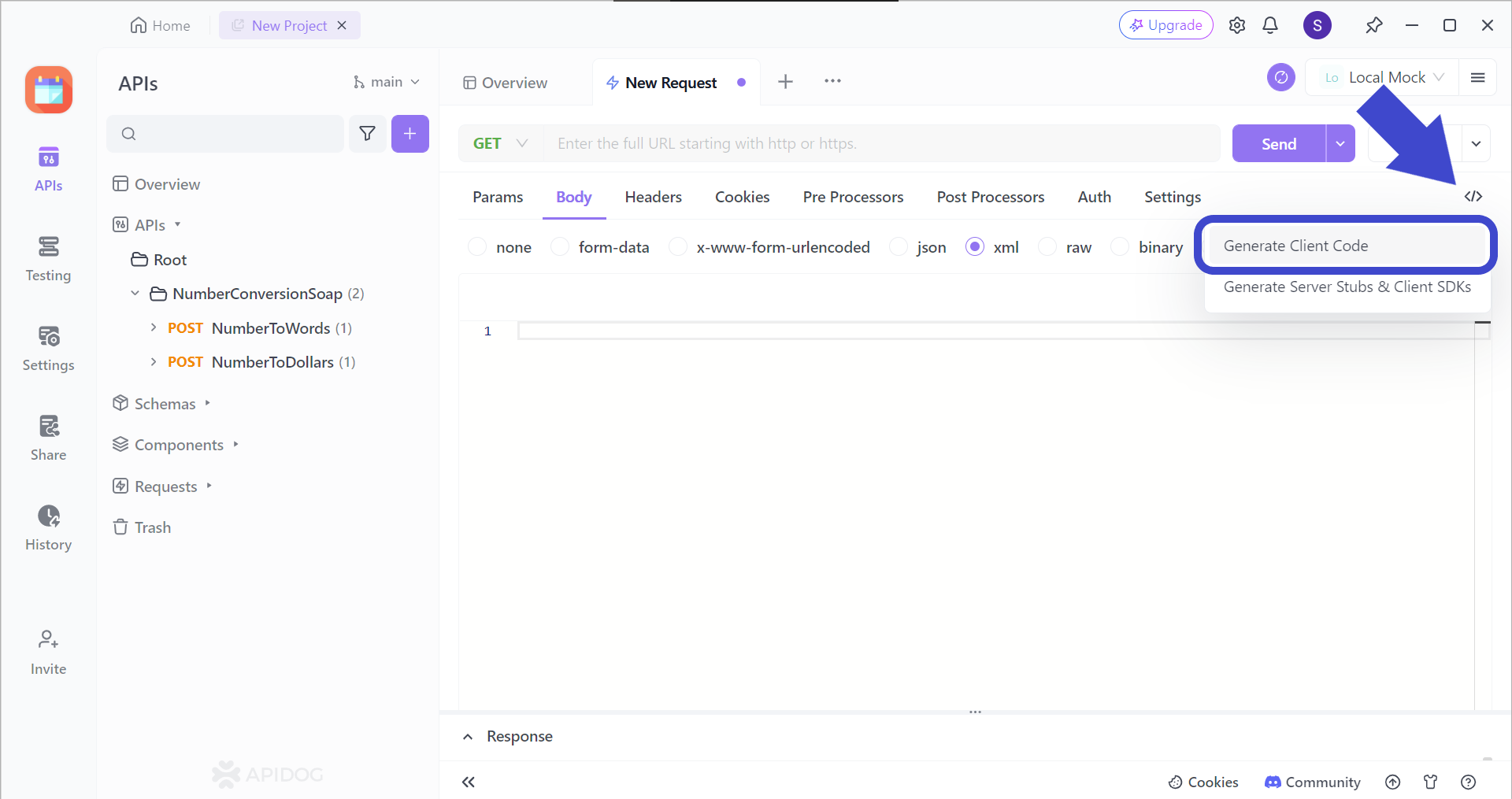
To utilize Apidog's code generation feature, begin by clicking the </>
button found on the top right corner of the Apidog window, and press Generate Client Code
.
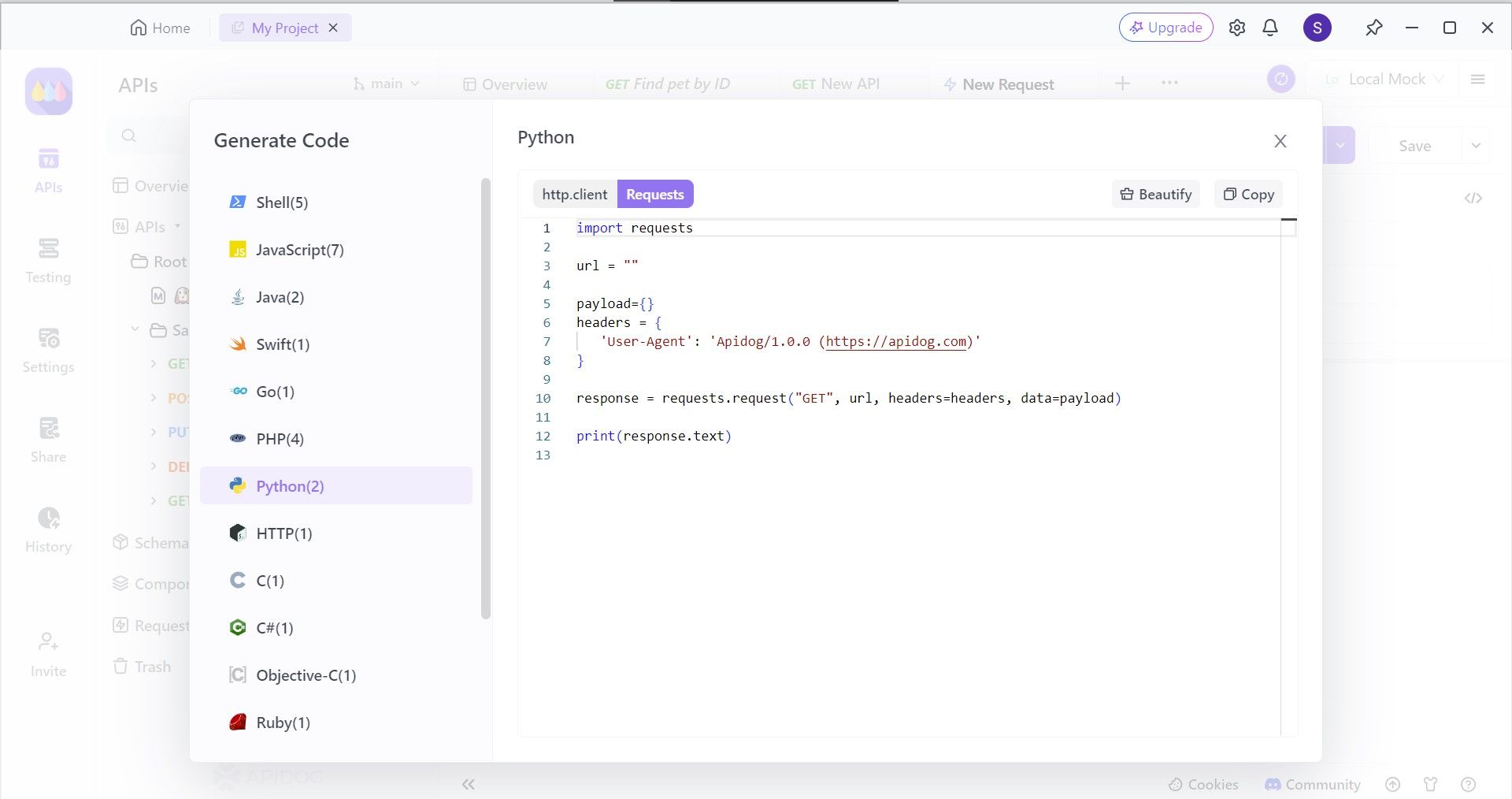
Next, select the Python
section, where you can find different frameworks for the JavaScript language. In this step, select Requests
, and copy the code. You can then paste it over to your other code platform to implement an AIOHTTP request!
Apidog's API Hub
If you are looking for more APIs to consume for your application or project, you can also consider checking out Apidog's API Hub, where you can find thousands of third-party APIs in the form of projects.
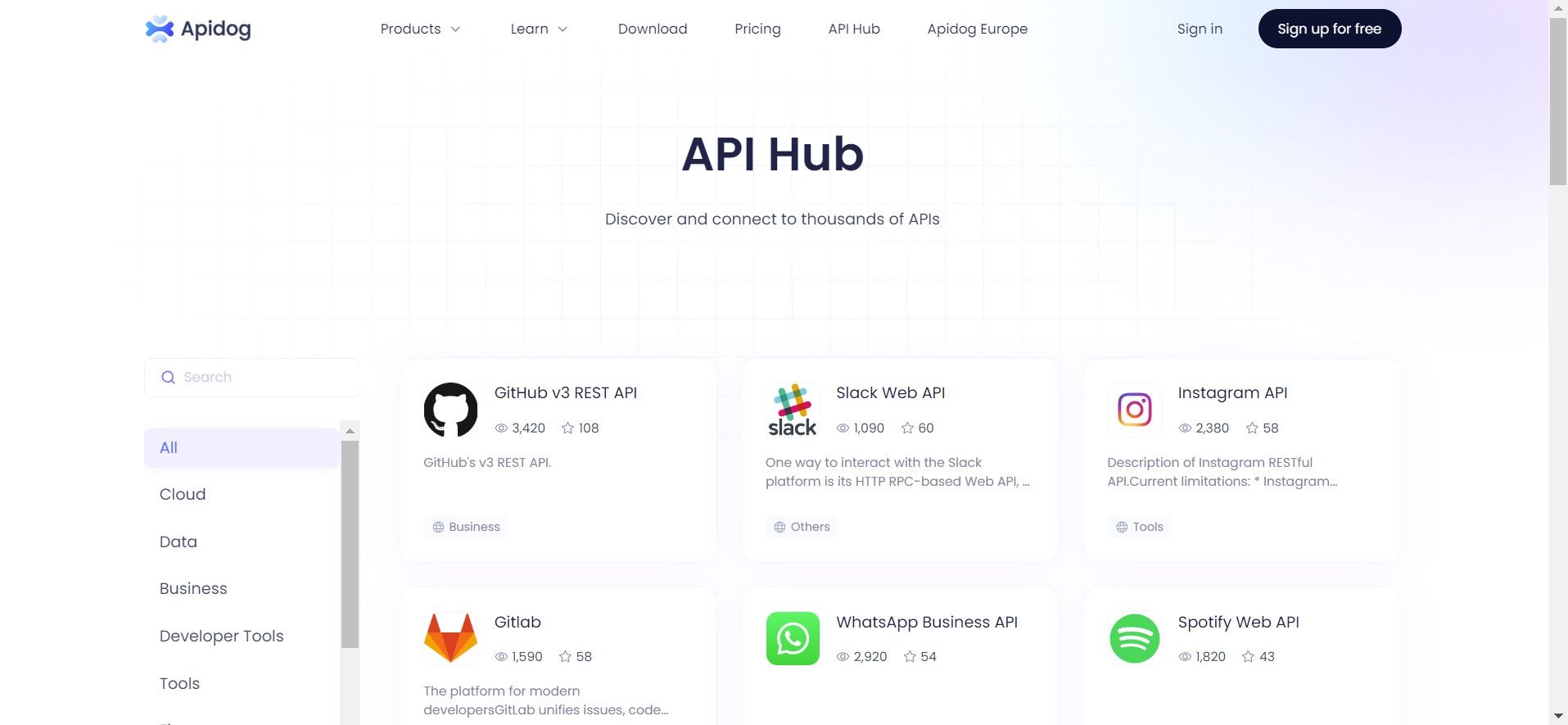
All you need to do is find one that interests you, and you can then check out the APIs in detail, so you can understand how the API functions - and perhaps create your very own API!
Conclusion
AIOHTTP requests are a powerful tool for making asynchronous HTTP requests in Python. They excel at handling a high volume of concurrent requests efficiently. This is achieved through features like non-blocking I/O, event loop integration, and connection pooling within the ClientSession class.
AIOHTTP also offers advanced features like streaming responses, proxy support, and cancellation capabilities, making it a versatile solution for various web communication needs.
Additionally, its rich ecosystem of third-party libraries and smooth integration with asynchronous web frameworks solidify AIOHTTP's position as a preferred choice for building performant and scalable web applications that heavily rely on asynchronous HTTP communication.
Lastly, Apidog can be the perfect API development tool for you if you are trying to save more time or focus on other aspects of API developing. With advanced features like code generation and multiple-step testing, you can boost your work efficiency by a mile.