A Practical Guide to Concurrent Requests with AIOHTTP in Python
Python's AIOHTTP framework is a notable solution for API developers looking for an answer to sluggish web applications. With utilizing the power of asyncio, learn to make your apps faster and more responsive with AIOHTTP concurrent requests!
In today's fast-paced world, web applications are constantly striving for efficiency. One key aspect of achieving this is handling multiple requests simultaneously. This is where AIOHTTP, a powerful Python library, shines. By enabling concurrent requests, AIOHTTP empowers developers to unlock the true potential of asynchronous programming.
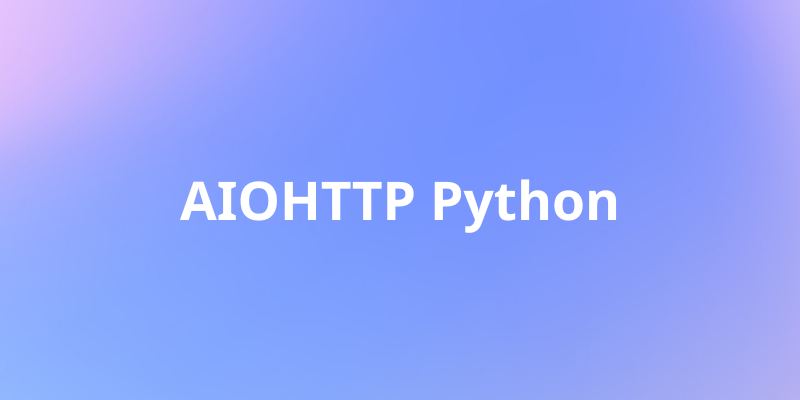
To magnify its benefits, make sure to use an equally powerful API development tool like Apidog to support your AIOHTTP-based applications. With Apidog, you can also quickly generate AIOHTTP-related coding within a few clicks.
To get started with streamlining your API development with Apidog for free, click the button below! 👇
This article delves into the practicalities of making concurrent requests with AIOHTTP, providing a step-by-step guide and exploring its benefits for optimizing application performance.
Let's first refresh our memory with brief descriptions on a couple of important concepts that we will encounter through this article:
AIOHTTP
A Python library designed for building modern, high-performance web applications. It leverages the power of asyncio, another Python library, to handle multiple HTTP requests concurrently.
Concurrent Requests
The ability to send and process multiple API requests simultaneously.
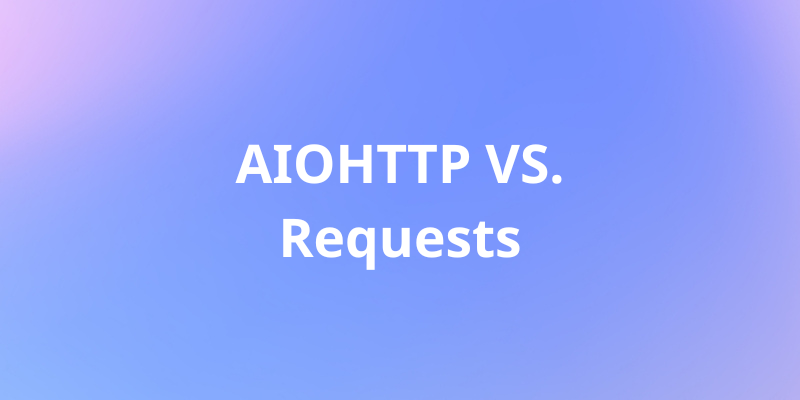
What are AIOHTTP Concurrent Requests?
AIOHTTP concurrent requests referes to the the ability to initiate and manage multiple HTTP requests asynchronously within a single Python application utilizing the AIOHTTP library.
This approach leverages the power of asyncio to avoid blocking on individual requests, allowing the application to handle them simultaneously and improve overall performance.
Key Aspects of AIOHTTP Concurrent Requests
1. Asynchronous Programming with asyncio:
AIOHTTP builds upon the foundation of asyncio, a Python library for asynchronous programming. Asynchronous code allows your application to handle multiple tasks seemingly at once.
In the context of AIOHTTP, this translates to sending and receiving HTTP requests concurrently without being blocked by individual responses. This maximizes CPU utilization and improves application responsiveness.
2. Tasks and Coroutines:
AIOHTTP uses asyncio concepts like tasks and coroutines to manage concurrent requests. A task represents a unit of asynchronous work, and a coroutine is a function designed to be used with asyncio. AIOHTTP allows you to create coroutines that handle individual HTTP requests. These coroutines are then launched as tasks, enabling them to run concurrently.
3. Async with and Async for Loops:
AIOHTTP provides asynchronous versions of common loop constructs like async with
and async for
. These are used to manage asynchronous operations like making requests and handling responses within your coroutines.
The async with
statement is used for asynchronous context management (e.g., opening and closing connections), while async for
loops are ideal for iterating through asynchronous results (e.g., processing multiple responses).
4. Async HTTP Client:
AIOHTTP offers an AsyncClient
class for making asynchronous HTTP requests. This client provides methods like get
, post
, put
, and delete
that take URLs and parameters as arguments. These methods return coroutines that can be launched as tasks to initiate concurrent requests.
5. Handling Responses:
Once a concurrent request completes, the corresponding coroutine receives the response object. You can use methods like status
, text()
, and json()
on the response object to access the status code, response body as text, or decoded JSON data, respectively.
6. Error Handling:
Robust error handling is crucial for managing concurrent requests. AIOHTTP allows you to handle exceptions raised during request execution or response processing within your coroutines.
This ensures that your application doesn't crash due to unexpected errors and can gracefully handle failed requests.
7. Benefits:
- Improved Performance: Concurrent requests significantly enhance performance by utilizing resources efficiently and reducing overall waiting time for responses.
- Increased Scalability: AIOHTTP applications can handle a high volume of concurrent requests, making them suitable for web applications with heavy traffic.
- Enhanced Responsiveness: Applications remain responsive even under heavy load as they're not blocked waiting for individual responses.
8. Important Considerations:
- API Rate Limits: Be mindful of rate limits imposed by APIs when making concurrent requests to avoid overloading their servers.
- Error Handling Complexity: Managing errors across multiple concurrent requests can become complex. Implement robust error handling strategies to ensure graceful operation.
- Resource Management: While AIOHTTP improves resource utilization, monitor resource usage to avoid overwhelming your application's processing power.
Examples on AIOHTTP Concurrent Requests
1. Fetching Multiple URLs Concurrently
import aiohttp
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
data = await response.text()
print(f"URL: {url}, Data: {data[:50]}...") # Truncate for brevity
else:
print(f"Error fetching {url}: {response.status}")
async def main():
urls = ["https://www.example.com", "https://www.python.org", "https://www.google.com"]
tasks = [aiohttp.create_task(fetch_url(url)) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
Code explanation:
The code example above defines an async
function fetch_url
that takes a URL as input. It uses an async with
statement to manage the ClientSession
and makes a GET request to the provided URL. The response is then handled, and the data or error message is printed.
The main
function creates a list of URLs and uses aiohttp.create_task
to create tasks for each URL. Finally, asyncio.gather
is used to run all tasks concurrently and wait for them to complete.
2. Downloading Multiple Files Concurrently
import aiohttp
import asyncio
async def download_file(url, filename):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
with open(filename, 'wb') as f:
while True:
chunk = await response.content.read(1024)
if not chunk:
break
f.write(chunk)
print(f"Downloaded {filename}")
else:
print(f"Error downloading {filename}: {response.status}")
async def main():
urls = [
("https://example.com/file1.zip", "file1.zip"),
("https://example.com/file2.pdf", "file2.pdf"),
]
tasks = [aiohttp.create_task(download_file(url, filename)) for url, filename in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
Code explanation:
The code example builds upon the previous one, demonstrating file downloads. The download_file
function takes a URL and filename as input. It retrieves the content and writes it chunk-by-chunk to a file with the specified name.
The main
function creates a list of URL-filename pairs and launches tasks for each download using aiohttp.create_task
. Similar to the previous example, asyncio.gather
is used for concurrent execution.
Important Note: Remember to replace the example URLs with the actual URLs you want to fetch or download. These examples serve as a foundation for building your own concurrent request functionalities using AIOHTTP.
Apidog - Quickly Generate AIOHTTP Code to Streamline Development
The AIOHTTP framework is based on thhe Python programming language, therefore you may have to learn the said programming language if you have no prior exposure to it. Luckily, there is an omni-potent API development tool that can assist you with code generation for client code called Apidog.
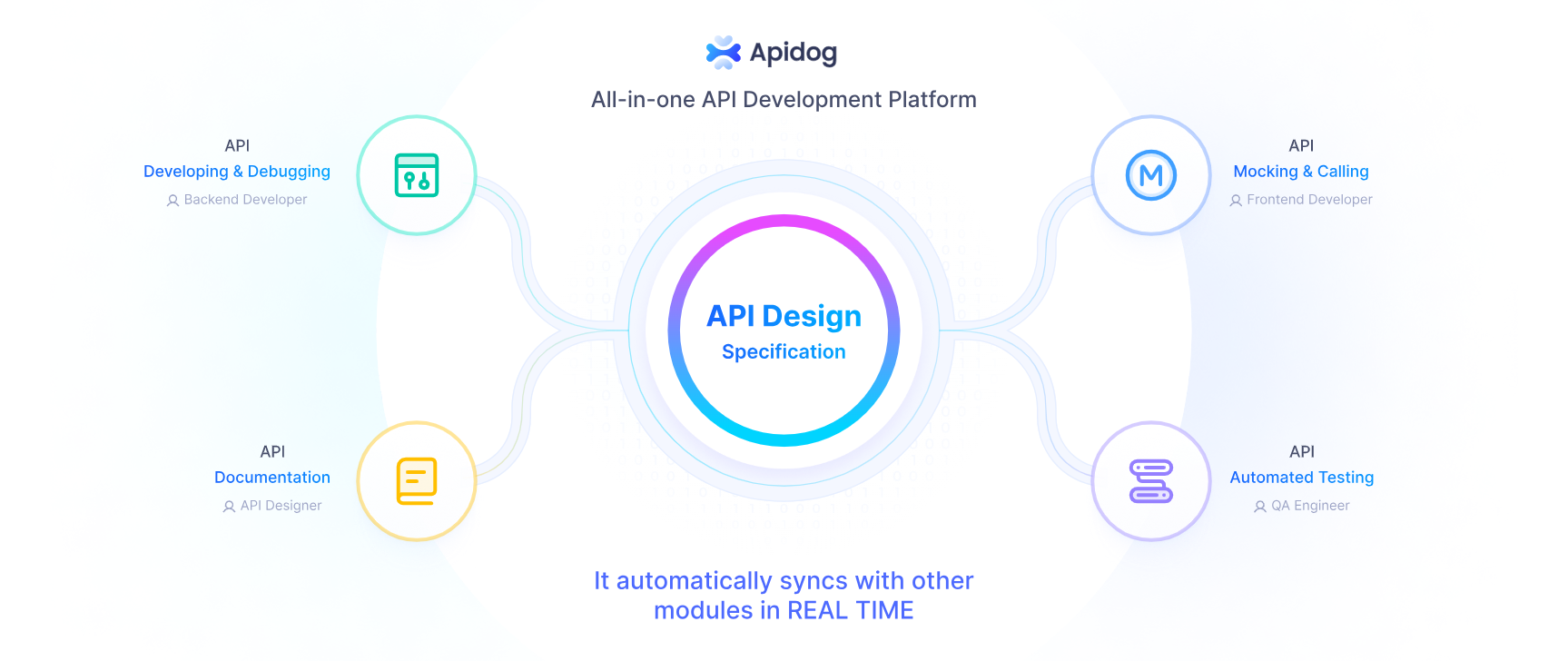
With Apidog, you can have the necessary Python client code for creating AIOHTTP-based applications. Proceed to the next section to find out how.
Generating Python Client Code Using Apidog
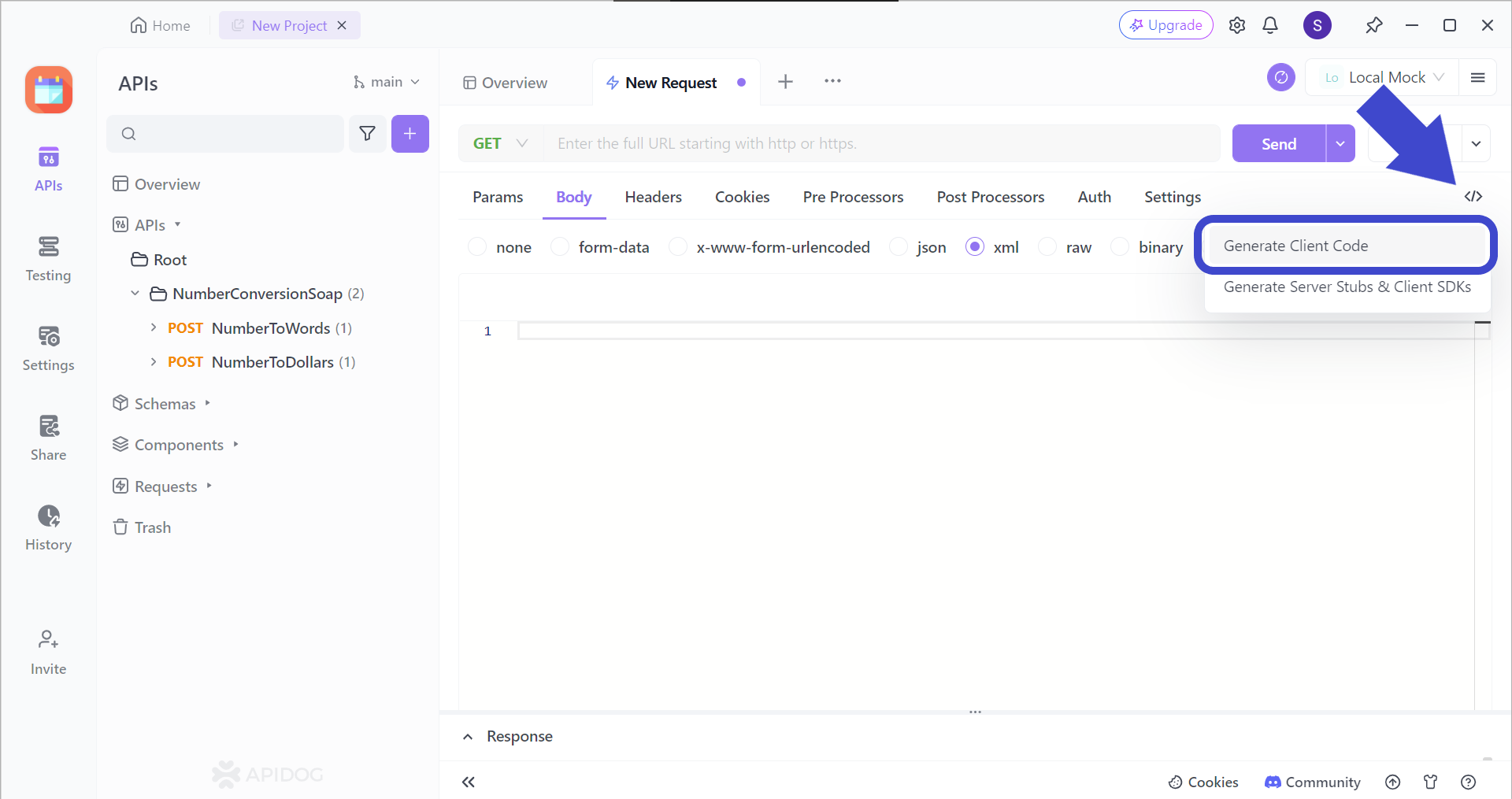
To utilize Apidog's code generation feature, begin by clicking the </>
button found on the top right corner of the Apidog window, and press Generate Client Code
.
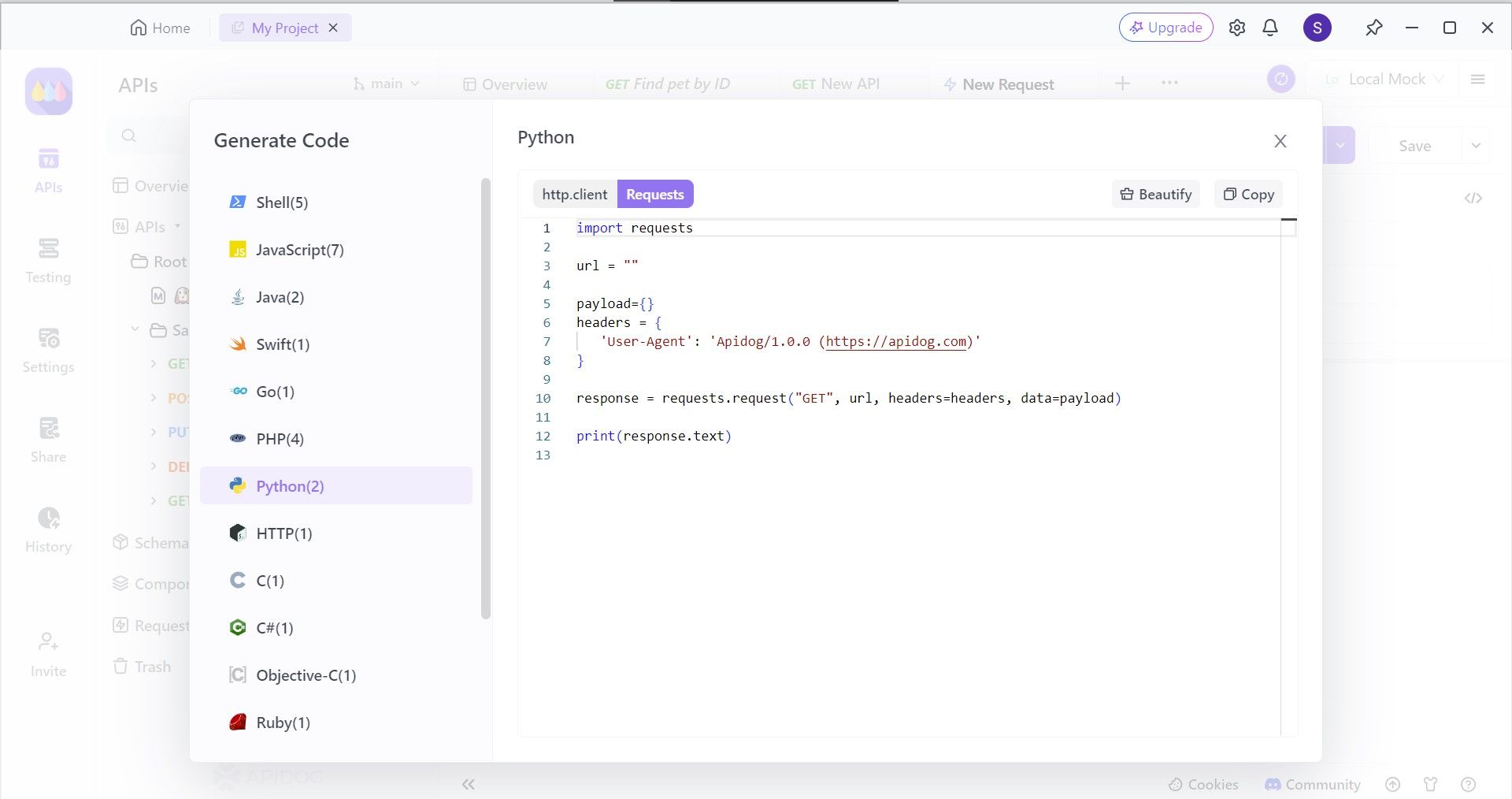
Next, select the Python
section, where you can find different frameworks for the JavaScript language. In this step, select Requests
, and copy the code. You can then paste it over to your IDE to implement the AIOHTTP framework!
Building Requests Using Apidog
You may need to create more than one requests for your application, more so significant if your application is going to be complex.
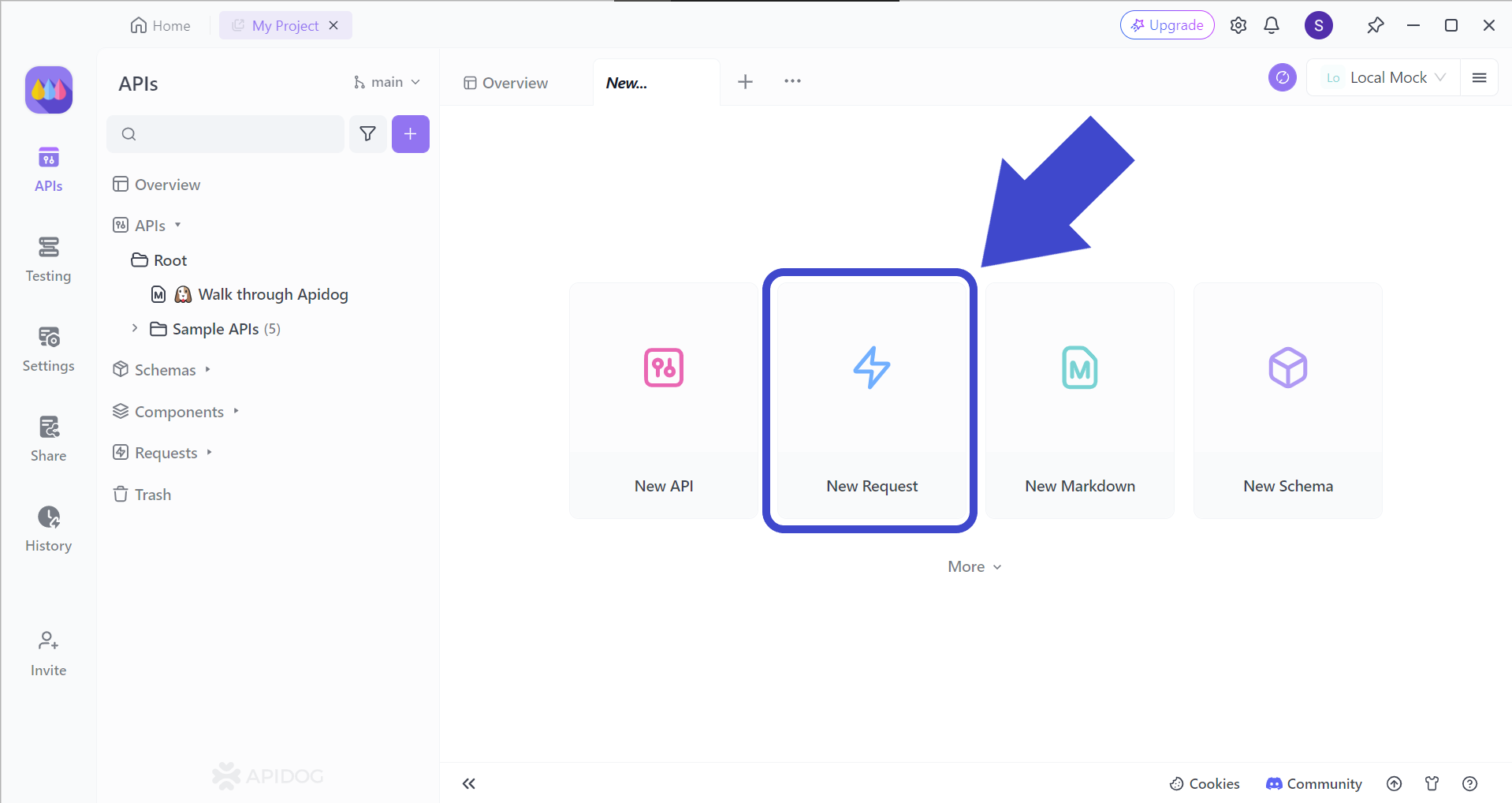
First, begin by initializing a new request on Apidog.
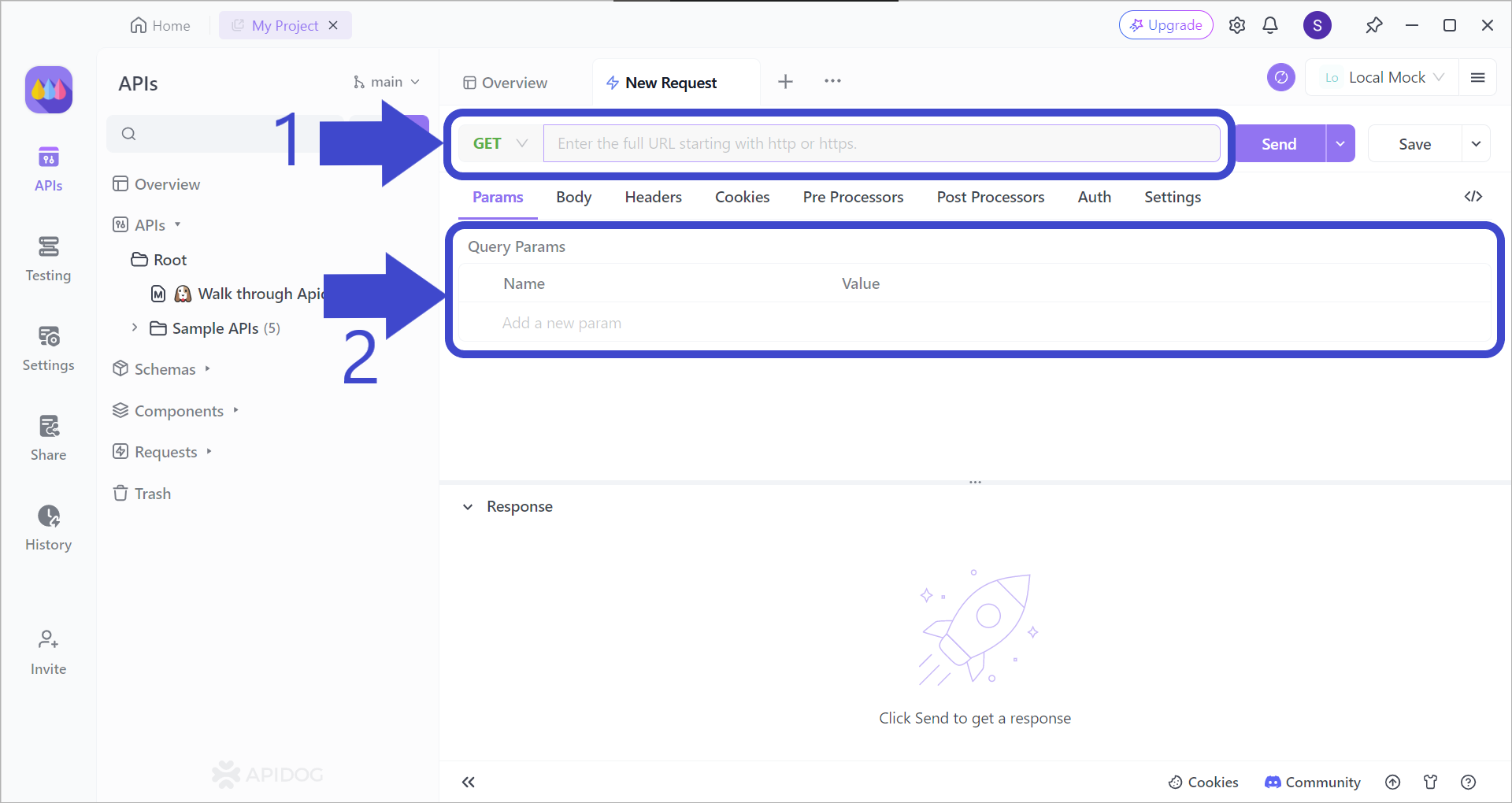
Next, select the POST
HTTP method, and craft a proper REST API URL. You can use a mix of path and query parameters, along with multiple IDs to create a more specific API URL.
Once you have finished including all the details, you can save the progress by clicking the Save
button.
Conclusion
This exploration of AIOHTTP and concurrent requests in Python has equipped you with the skills to significantly enhance the performance and efficiency of your web applications. By embracing the power of asynchronous programming with asyncio, AIOHTTP allows you to send and receive multiple HTTP requests simultaneously. This eliminates the traditional wait times associated with sequential requests, leading to faster data retrieval and a more responsive user experience.
The provided examples have demonstrated the practical applications of AIOHTTP for fetching data and downloading files concurrently. Remember to keep API rate limits in mind when crafting your concurrent request strategies. Additionally, implementing robust error handling practices will ensure your applications gracefully handle unexpected situations and maintain smooth operation under heavy loads. With the knowledge gained from this guide, you can confidently leverage AIOHTTP concurrent requests to build high-performing and scalable web applications in Python.
Apidog is an API development platform that provides API developers with the necessary tools for the entire API lifecycle. Aside from code generation, you can also utilize Apidog's API Hub feature if you ever get stuck on a coder's "mental block".