AIOHTTP Python | Build Asynchronous Web Apps With This Framework!
AIOHTTP: Powerful Python lib for async HTTP. Learn how to build fast web clients & servers that can handle high traffic with ease using Python's asyncio library!
AIOHTTP is a Python library that empowers developers to create asynchronous HTTP clients and servers. It excels in building high-performing web applications by utilizing the asyncio library.
If you are unfamiliar with AIOHTTP or Python in general, try using Apidog, a comprehensive API tool that has code generation features. Within a few clicks of your mouse, you can have code ready to be pasted into your IDE (Integrated Development Environment).
If you want to start becoming more efficient in developing apps and APIs, click the button below to begin! 👇 👇 👇
What is AIOHTTP Python?
AIOHTTP is a Python framework that utilizes the asyncio library, allowing you to write non-blocking and concurrent code for building web applications.
AIOHTTP Python Key Features
Asynchronous Core:
- Non-Blocking I/O: Unlike traditional frameworks, AIOHTTP doesn't wait for a single operation (like a network request) to finish before moving on. It can handle multiple requests concurrently, making it ideal for applications dealing with high traffic. This is achieved through coroutines, a special type of function that can suspend and resume execution as needed.
Client-Side Operations:
- Making HTTP Requests: AIOHTTP provides an asynchronous API for making HTTP requests to external servers. You can fetch data, send information, and handle responses efficiently.
- Client Sessions: For repeated interactions with a server, AIOHTTP offers client sessions. These sessions manage connection pooling, reducing overhead and improving performance.
Server-Side Development:
- Building Web Servers: AIOHTTP allows you to construct web servers that can handle incoming HTTP requests. You can define routes that map URLs to specific functions in your application, responsible for generating responses.
- WebSockets: It offers built-in support for WebSockets, a two-way communication channel that enables real-time data exchange between the server and client. This is perfect for applications like chat rooms or live updates.
Benefits of Using AIOHTTP Python
AIOHTTP Python's core strength lies in its asynchronous nature which is powered by asyncio. This therefore translates to several key benefits that elevate anyone's development experience:
1. Enhanced Scalability:
- Concurrent Connection Handling: AIOHTTP doesn't get bogged down by waiting for individual operations to finish. It thrives on handling multiple requests simultaneously. This is particularly beneficial for applications that experience high traffic volumes. Imagine a web server bombarded with requests – AIOHTTP can juggle them efficiently, ensuring smooth operation even under load.
2. Unlocking Performance Gains:
- Non-Blocking I/O: Traditional frameworks often get stuck waiting for I/O operations (like network requests) to complete before moving on. AIOHTTP eliminates this bottleneck. It utilizes coroutines, functions that can suspend and resume execution as needed. This allows it to initiate new tasks while waiting for responses, maximizing resource utilization and boosting overall application responsiveness.
3. The Magic of Concurrency:
- Efficient Task Management: With AIOHTTP, you can write code that tackles multiple requests concurrently. This translates to a more efficient use of system resources. Instead of having your application grind to a halt waiting for a single response, AIOHTTP keeps things moving, improving overall application efficiency.
4. Streamlined Development Process:
- Cleaner Code with Coroutines: AIOHTTP leverages coroutines, promoting a more concise and readable codebase. Imagine writing code that clearly expresses the asynchronous nature of your application – coroutines make this a breeze.
AIOHTTP Python Code Examples
Here are some code examples showcasing AIOHTTP Python in both client-side and server-side applications:
1. Making asynchronous HTTP requests (Client-side):
import aiohttp
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
data = await response.json()
return data
else:
print(f"Error: {response.status}")
return None
# Example usage
loop = asyncio.get_event_loop()
data = loop.run_until_complete(fetch_data("https://api.github.com/users/bard"))
if data:
print(f"Name: {data['name']}")
Code explanation:
The code example defines an asynchronous function fetch_data
that takes a URL as input. It then uses a ClientSession
to make a GET request to the specified URL.
If the response is successful (status code 200
), it parses the JSON data and returns it, else it prints an error message.
2. Building a simple web server (Server-side):
from aiohttp import web
async def hello(request):
return web.Response(text="Hello, world!")
app = web.Application()
app.add_routes([web.get('/', hello)])
if __name__ == '__main__':
web.run_app(app)
The code example above demonstrates a basic web server using AIOHTTP, defining an asynchronous hello
function that returns a "Hello, world" response.
The app
object configures routes to map the root path ('/') to the hello
function. The web.run_app
then starts the server.
3. Handling POST requests with Data (Server-side):
from aiohttp import web
async def handle_post(request):
data = await request.json()
name = data.get('name')
if name:
return web.Response(text=f"Hello, {name}!")
else:
return web.Response(text="Missing name parameter", status=400)
app = web.Application()
app.add_routes([web.post('/greet', handle_post)])
if __name__ == '__main__':
web.run_app(app)
The code example above builds on the previous web-server example by handling a POST request to the /greet
endpoint. This is made possible by retrieving the JSON data from the request body and extracting the name
parameter.
If present, the code will return a personalized greeting, else it returns a 400
Bad Request error.
Real-life Scenarios Where AIOHTTP Python are Applicable
1. Building Scalable Web APIs:
When you're developing web APIs that need to handle a large number of requests simultaneously, AIOHTTP shines. Its asynchronous capabilities allow it to process multiple API calls efficiently without getting bogged down. This is ideal for APIs that power mobile applications, real-time data feeds, or microservices architectures.
2. Web Scraping at High Speed:
Imagine scraping data from multiple websites concurrently. AIOHTTP excels at this task. It can initiate requests to various URLs simultaneously, retrieving data much faster than traditional synchronous approaches. This is beneficial for tasks like market research, price aggregation, or competitor analysis.
3. Real-Time Applications (WebSockets):
AIOHTTP's built-in WebSocket support makes it a great choice for building real-time applications like chat rooms, collaborative editing tools, or live dashboards. WebSockets enable two-way communication between client and server, allowing for seamless data exchange and updates in real time.
4. High-Performance Data Fetching:
Applications that rely heavily on fetching data from external sources can benefit significantly from AIOHTTP. Its asynchronous nature allows it to make multiple data requests concurrently, improving the overall responsiveness of your application. This is valuable for applications that display content from various APIs or social media feeds.
5. Building Chatbots and Messaging Platforms:
AIOHTTP's asynchronous capabilities are well-suited for building chatbots and messaging platforms that need to handle a high volume of incoming and outgoing messages simultaneously. It can efficiently process user requests, retrieve data, and generate responses, leading to a smooth user experience.
Apidog - Python Client Code Generation
As AIOHTTP is a Python framework, you will need to understand Python programming to create client or server-sided applications.
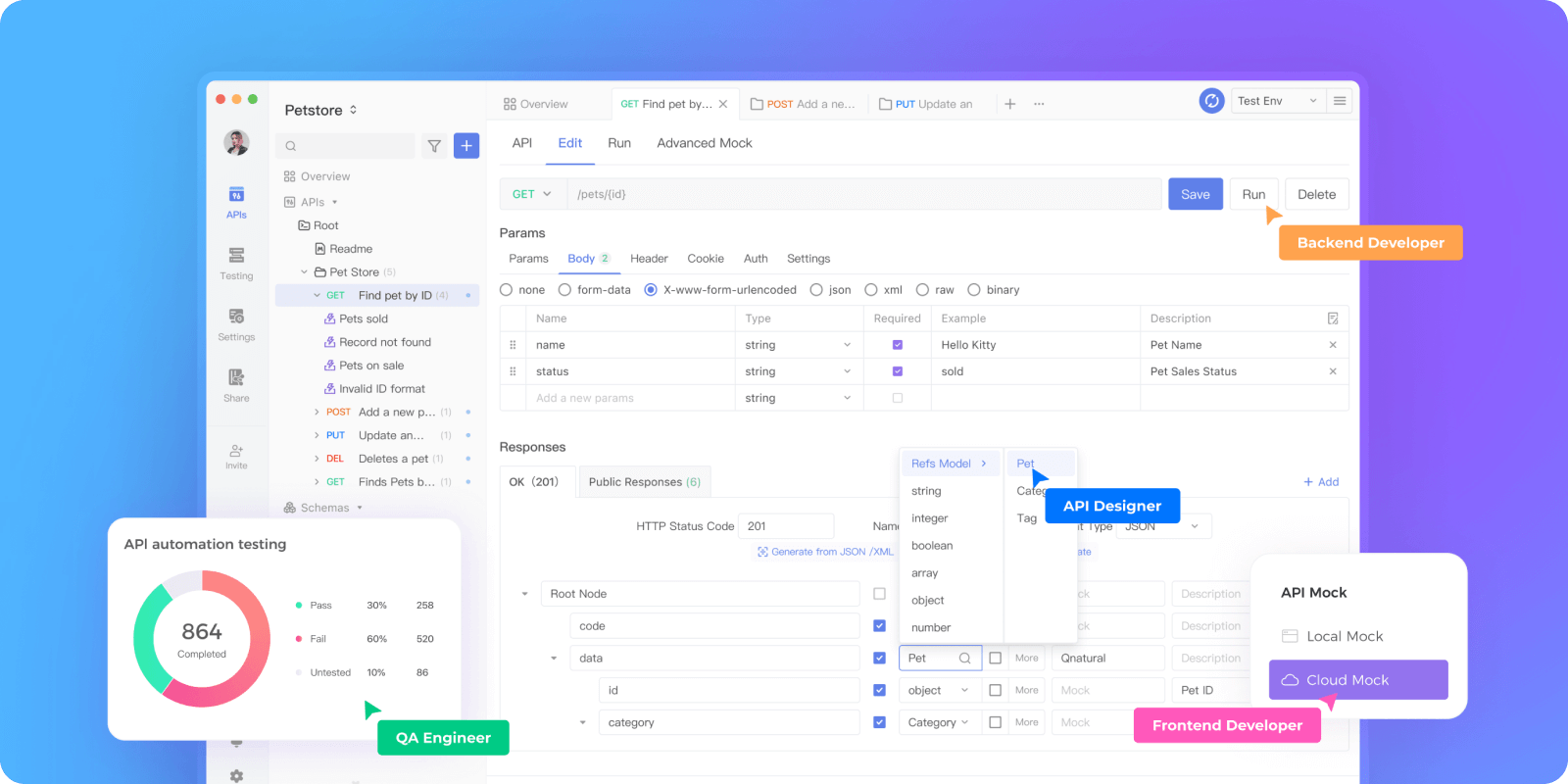
To create high-performing applications, you will need a good understanding of APIs to include useful functionalities in your application. Therefore, you should consider using Apidog, an all-in-one API development tool that allows you to build, modify, test, and document APIs.
Another significant reason why Apidog is strongly recommended is due to its code generation feature. This allows developers to quickly develop their codes on IDEs, and prepare their applications for testing!
Generating AIOHTTP Python Code Using Apidog
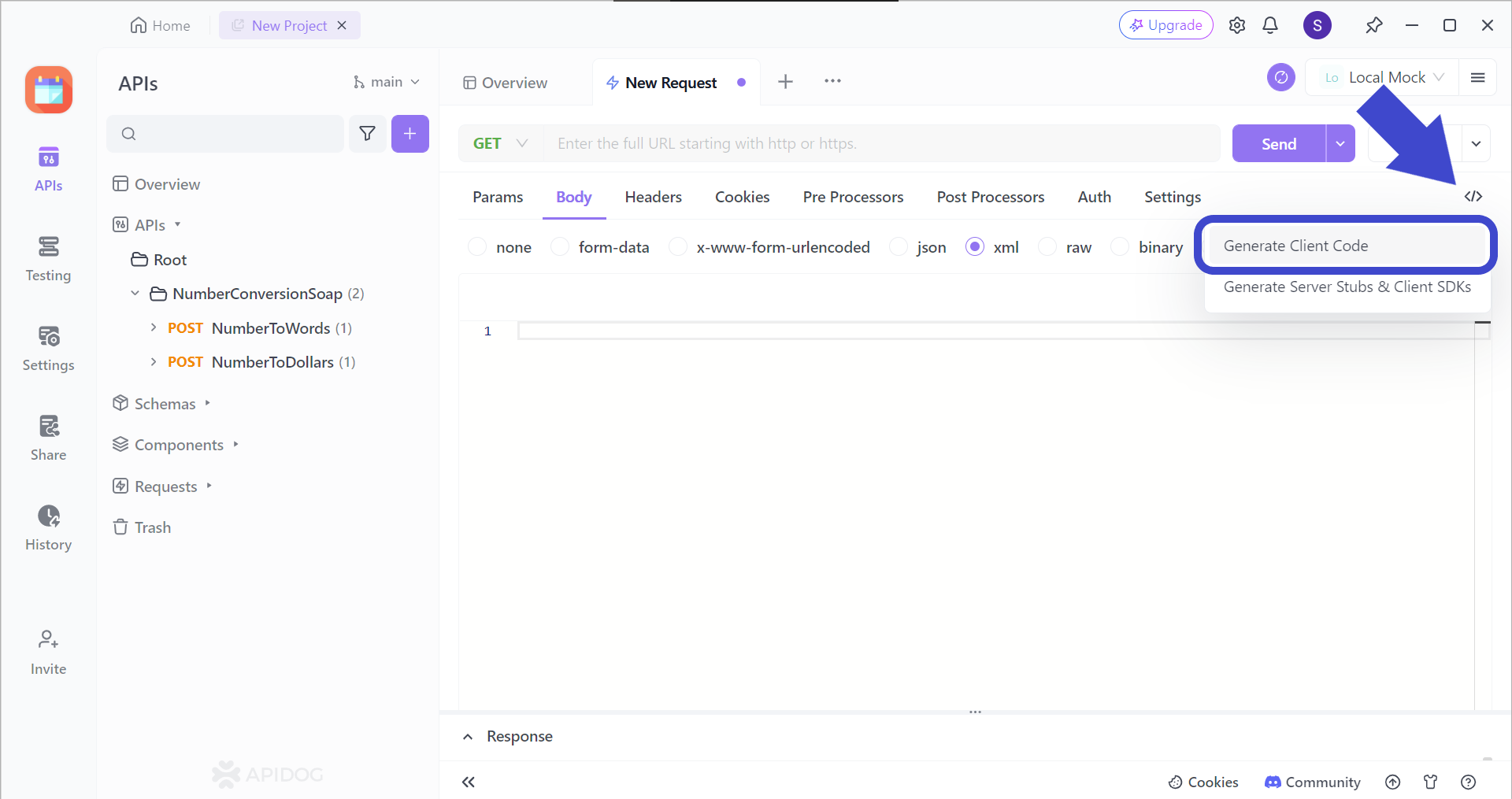
To utilize Apidog's code generation feature, begin by clicking the </>
button found on the top right corner of the Apidog window, and press Generate Client Code
.
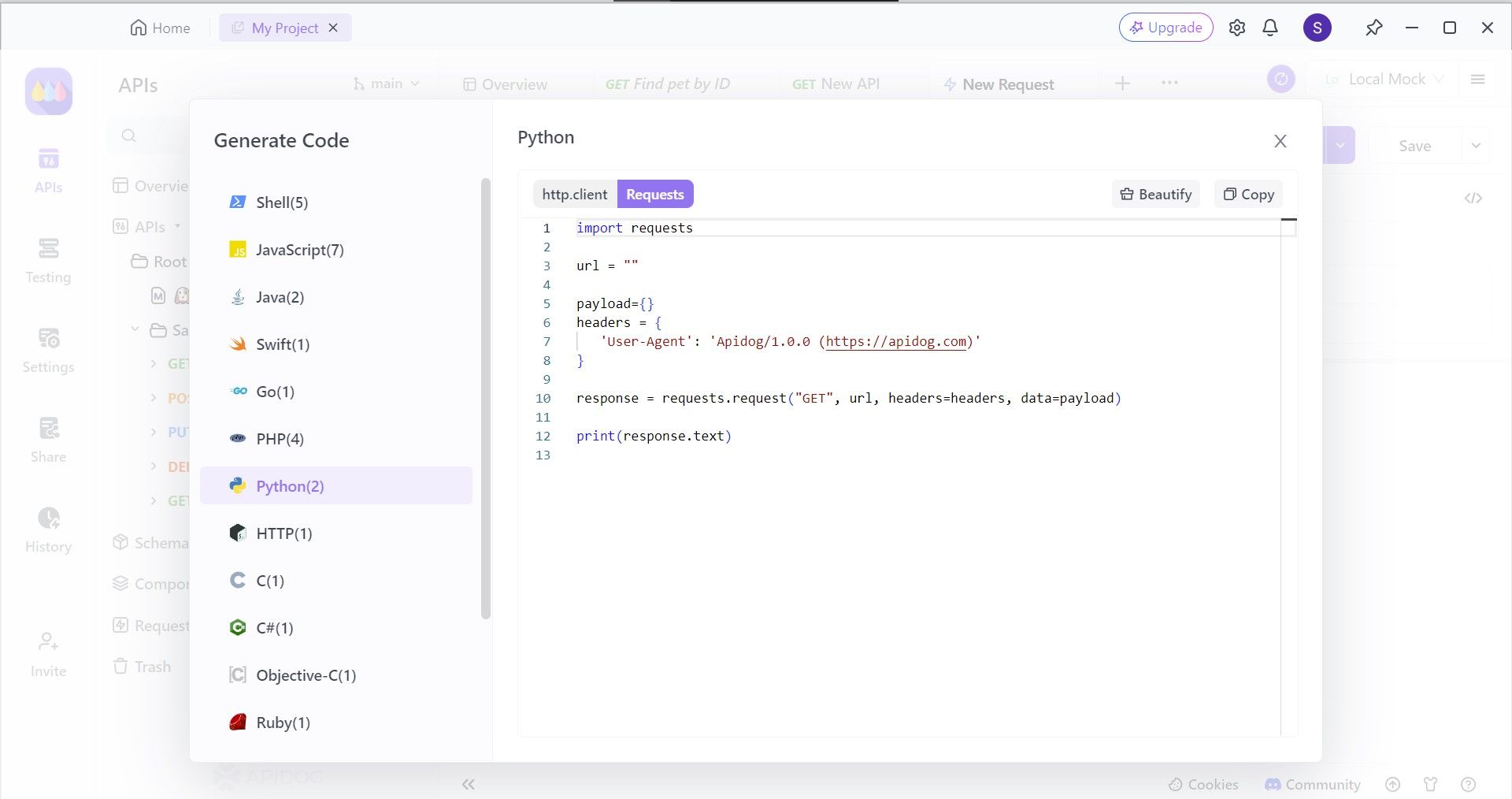
Next, select the Python
section, where you can find different frameworks for the JavaScript language. In this step, select Requests
, and copy the code. You can then paste it over to your IDE to implement the AIOHTTP framework!
Generate Descriptive API Documentation with Apidog
As AIOHTTP might be unfamiliar to some developers, you can help them understand what your application is running on through creating proper API documentation.
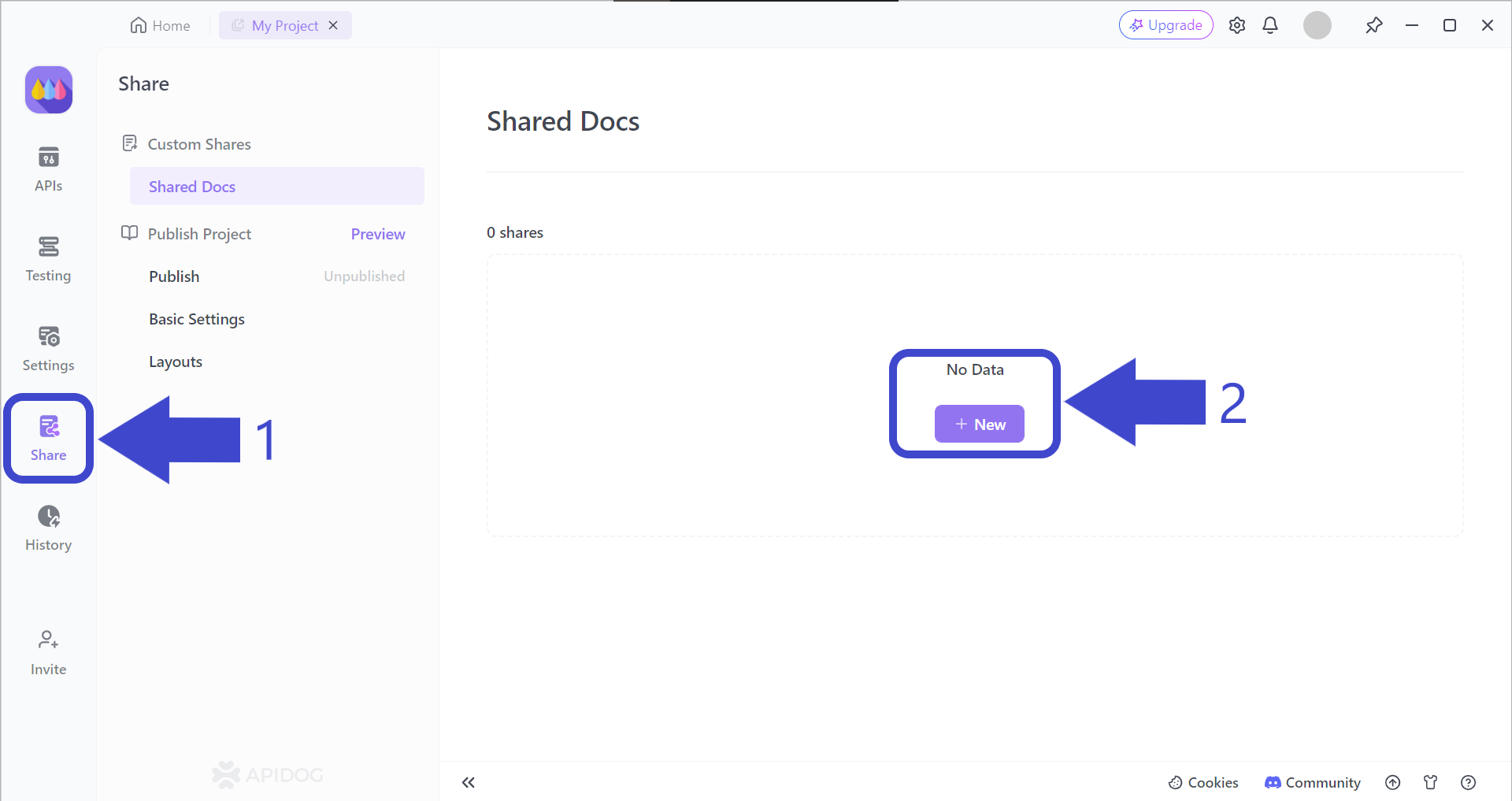
Arrow 1 - First, press the Share
button on the left side of the Apidog app window. You should then be able to see the "Shared Docs" page, which should be empty.
Arrow 2 - Press the + New
button under No Data
to begin creating your very first Apidog API documentation.
Select and Include Important API Documentation Properties
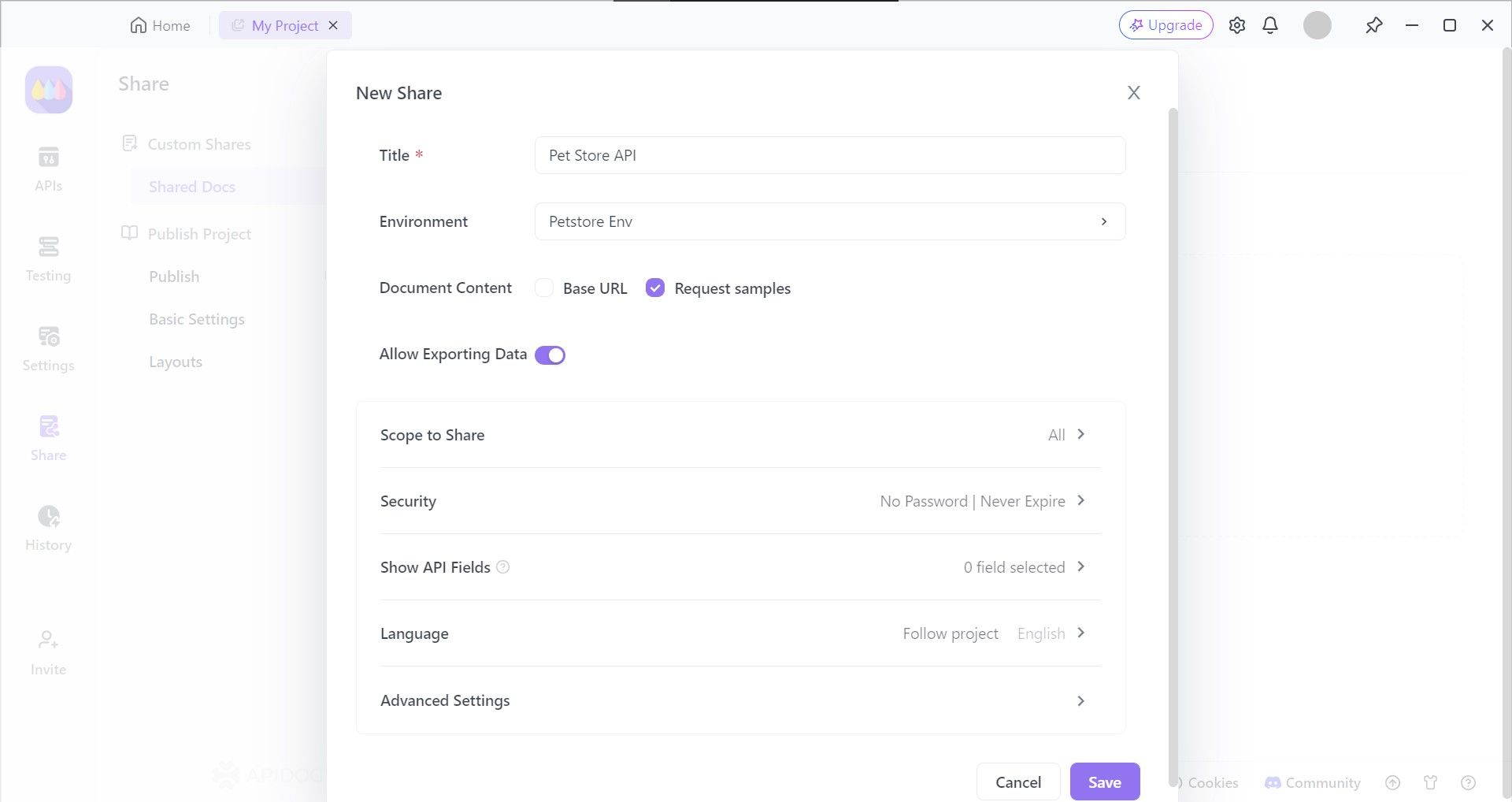
Apidog provides developers with the option of choosing the API documentation characteristics, such as who can view your API documentation and setting a file password, so only chosen individuals or organizations can view it.
View or Share Your API Documentation
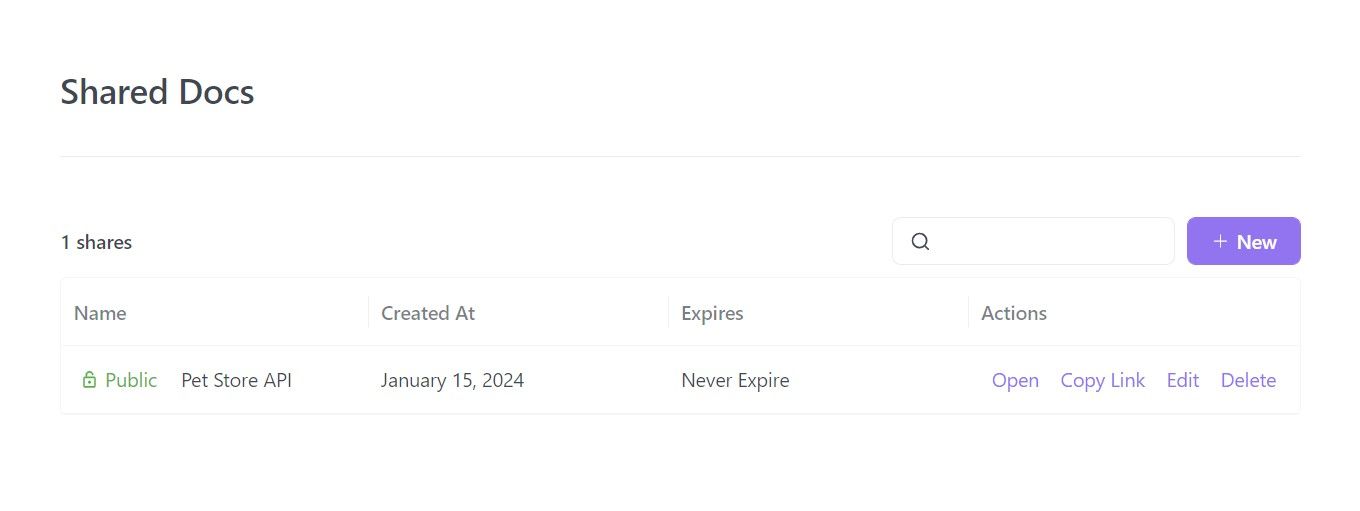
Apidog compiles your API project's details into an API documentation that is viewable through a website URL. All you have to do is distribute the URL so that others can view your API documentation!
If more details are required, read this article on how to generate API documentation using Apidog:
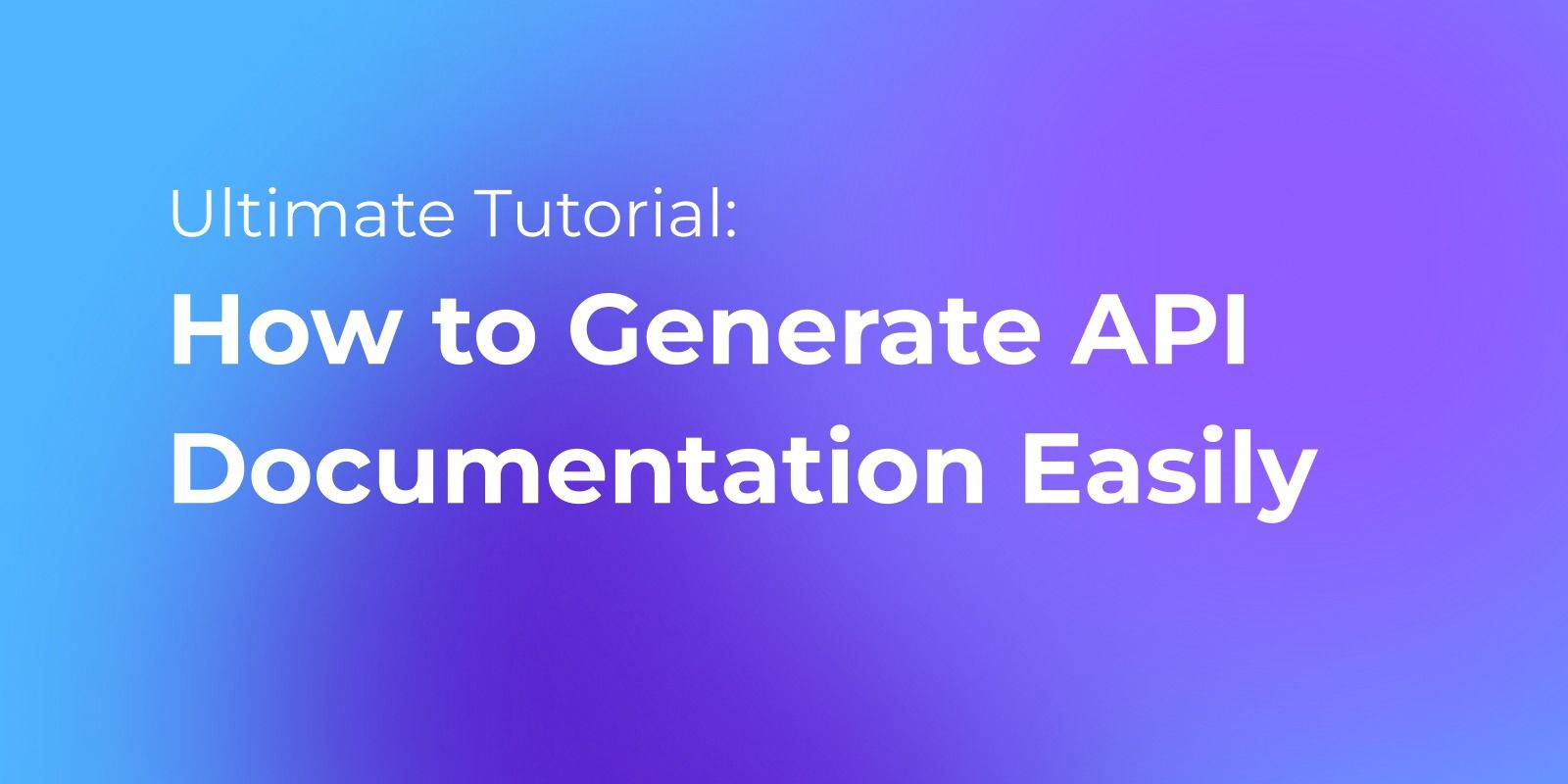
Conclusion
In conclusion, AIOHTTP shines as a versatile tool for crafting high-performance web applications in Python. Thanks to Python's asynchronous library asyncio
, it tackles heavy traffic with ease, making it perfect for modern web development.
AIOHTTP boasts a well-designed API that simplifies HTTP interactions and server creation. From building web scrapers and API clients to full-fledged web services, AIOHTTP offers a comprehensive solution for your web development needs.
If you want to learn more about AIOHTTP, make sure to check these articles:
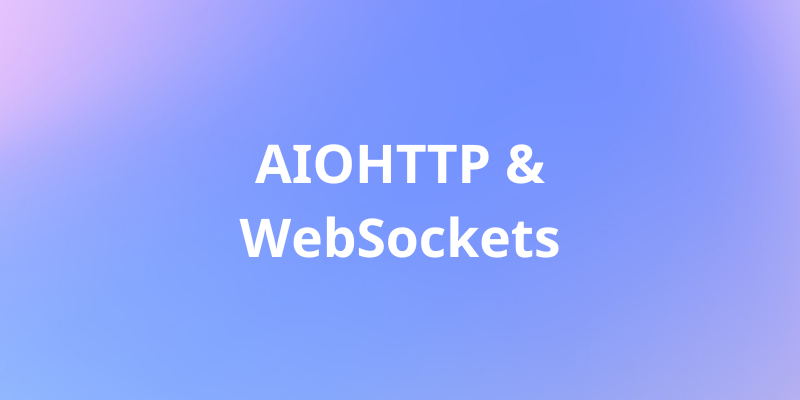
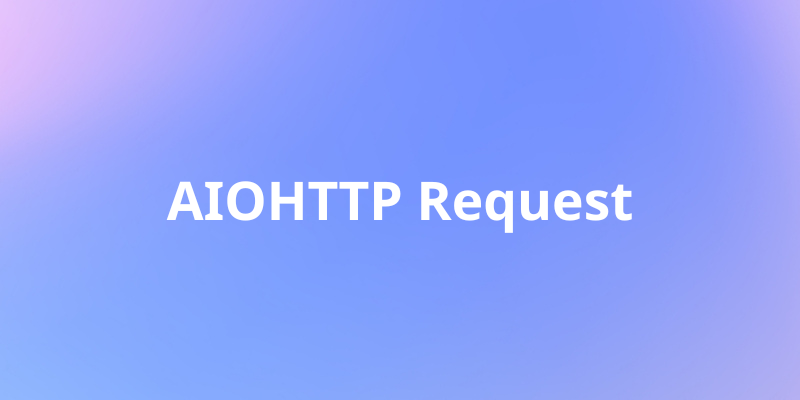
Apidog is a comprehensive API tool that can be very useful, especially for newer developers who are trying to create scalable, fast-performing APIs. With Apidog's simple and intuitive user interface, both experienced and new developers can quickly understand the gist of Apidog, so try it out today!