How to Use JSON Web Token (JWT) in Node.js
JSON Web Token (JWT) is an open standard that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. This article will provide a quick overview of how JWTs work.
JSON Web Tokens (JWTs) have emerged as a popular way to handle authentication and authorization in Node.js apps. Compared to sessions, JWTs are simple, compact tokens that can be easily passed in HTTP requests to authenticate users and transmit information securely.
This article will provide a quick overview of how JWTs work, discuss their benefits, and offer a step-by-step guide to implementing JWT-based auth in a Node.js. You'll learn the core concepts needed to enable a seamless JWT authentication flow for securely accessing protected resources in your Node APIs.
What is JSON Web Token (JWT)?
JSON Web Token (JWT) is an open standard that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. It is used for authentication and authorization - after a user logs in, the server generates a JWT with user information and signs it to prevent tampering.
The client stores this JWT and sends it back in HTTP headers for protected API routes. The JWT consists of three Base64-encoded parts - a header, a payload, and a signature.
JWT Usage Scenarios
When does it make sense to use JWT? Here are some common usage scenarios:
User authentication: JWT can be used to authenticate a user. When a user logs in, the server can generate a JWT for them, and the client can send this JWT in subsequent requests to prove their identity.
Single Sign-On (SSO): JWT can be used to implement Single Sign-On, which allows a user to log in once and access multiple related applications without having to log in repeatedly.
API Authorization: JWTs can include authorization information in API calls to verify on the server side that a user has permission to perform a particular action.
Password Reset: JWT can be used to securely generate a link containing a password reset token to allow a user to reset their password.
Mobile Application Authentication: Mobile applications can use JWTs to authenticate with back-end servers to ensure that only authorized users can access back-end resources.
JWT Basic Concepts
To use JWT in Node.js, you need to understand the following basic concepts:
JWT Structure: JWT consists of three parts, namely Header, Payload and Signature. The Header contains the algorithm and token type, the Payload contains the information to be passed, and the Signature is used to verify the authenticity of the token.
Key: To generate and validate a JWT, you need a key. The key can be symmetric (same key for signing and verification) or asymmetric (different keys for signing and verification).
Signature Verification: The server that receives the JWT uses the key to verify the signature to ensure that the JWT has not been tampered with. If the signature verification is successful, the server can trust the information in the JWT.
Common Method
Here is an optimized translation of the common methods for JWT authentication and authorization in Node.js:
1. Generate JWT
Use thejsonwebtoken
library to generate JWTs. First install the library:
npm install jsonwebtoken
Then generate a JWT like this:
const jwt = require('jsonwebtoken');
const payload = { userId: 123, role: 'admin' };
const secretKey = 'your-secret-key';
const token = jwt.sign(payload, secretKey, { expiresIn: '1h' });
2. Verify JWT
When the server receives a JWT, it needs to verify its authenticity. Use this to verify:
const jwt = require('jsonwebtoken');
const token = 'your-jwt-token';
const secretKey = 'your-secret-key';
try {
const decoded = jwt.verify(token, secretKey);
console.log(decoded);
} catch (error) {
console.error('JWT verification failed');
}
3. Middleware
In Express.js, you can create JWT verification middleware to protect routes:
const jwt = require('jsonwebtoken');
const secretKey = 'your-secret-key';
function authenticateToken(req, res, next) {
const token = req.header('Authorization');
if (!token) return res.status(401).send('Access denied');
try {
const decoded = jwt.verify(token, secretKey);
req.user = decoded;
next();
} catch (error) {
res.status(403).send('Invalid token');
}
}
Example Application
Here is an example Express.js app using JWT for authentication and authorization:
// Import libraries
const express = require('express');
const jwt = require('jsonwebtoken');
// App setup
const app = express();
const secretKey = 'your-secret-key';
// Login route, generate JWT
app.post('/login', (req, res) => {
// Authenticate credentials and create token
const token = jwt.sign({ username: 'user' }, secretKey);
res.json({ token });
});
// Protected route, verify JWT
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'Protected data' });
});
// JWT verification middleware
function authenticateToken(req, res, next) {
// Verify JWT and decode payload
const decoded = jwt.verify(token, secretKey);
req.user = decoded;
next();
}
app.listen(3000);
So in summary, we are using JSON web tokens to create and verify JWTs, and middleware to protect routes.
Apidog: A Powerful API Tool
If you are a Node.js developer, you often need to deal with APIs to ensure your applications function properly. This is where a powerful API testing tool comes in handy.
It also supports JSON Web Tokens (JWT) with visual tools and intuitive features, providing a seamless experience for handling authentication and authorization within your API requests.
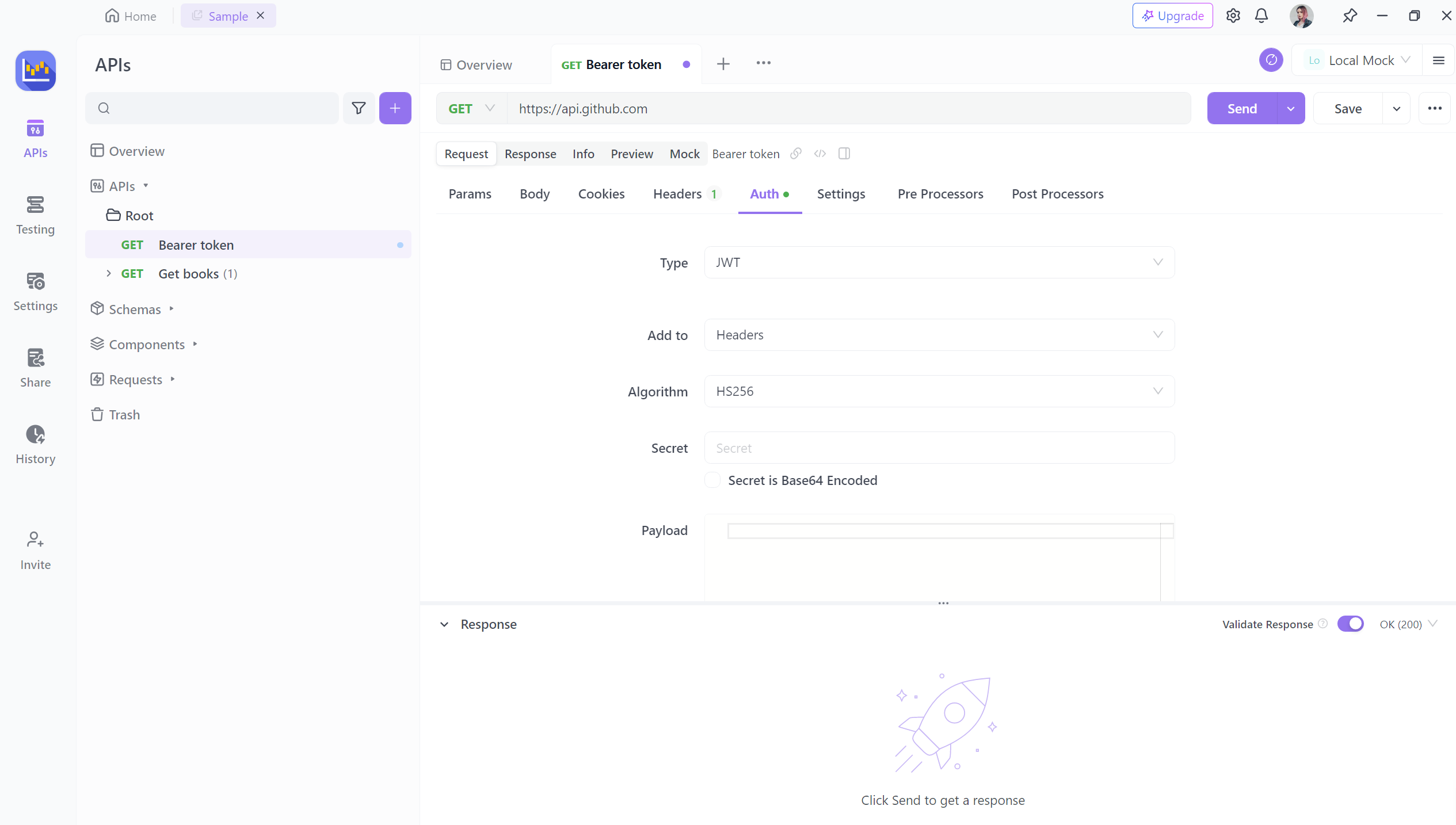
In addition, Apidog is an API testing tool more powerful than Postman. It supports debugging HTTP (s), WebSocket, Socket, gRPC, and other protocol interfaces, making it a very comprehensive API testing tool.
Conclusion
JWT authentication and authorization in Node.js is a powerful method to ensure your applications are secure and user privacy is protected. This article provided the basics of JWTs, common methods, and a practical example to help you start using JWTs in your Node.js apps. By appropriately leveraging JWTs, you can build secure web apps and APIs.