How to Use Assert in Node.js
By incorporating assertion statements into their code and writing tests using the assert() function, developers can validate assumptions, detect errors, and improve the quality of their Node.js applications.
Testing is an integral part of the development process in Node.js, ensuring that code behaves as expected and meets specified requirements. Among the various testing tools, the assert module in Node.js plays a crucial role in simplifying the process of verifying code correctness.
In this article, we will delve into the assert module, explore its functionalities, and learn how to use the assert() function effectively in Node.js development.
What is the Assert Module?
The assert module is a built-in module in Node.js that provides a set of assertion functions for performing assertions in code. Assertions are statements that validate assumptions about the state of the code during runtime. The assert module facilitates these assertions by providing functions that check whether certain conditions hold and throw errors if they do not.
One of the primary purposes of the assert module is to aid in writing tests for Node.js applications. By using assertion functions provided by the assert module, developers can assert the correctness of values, behaviors, and conditions in their code, thus ensuring its reliability and stability.
What is the Assert Function in JavaScript?
In JavaScript, the assert function is a built-in function that checks whether a given expression evaluates to true. If the expression evaluates to false, the assert function throws an AssertionError, indicating that the assumption made by the developer does not hold true.
The assert function is commonly used in JavaScript programming to validate assumptions and detect inconsistencies in code execution. It helps developers identify and fix bugs by providing feedback when code behavior deviates from expectations.
Node.js assert() Function:
The assert() function in Node.js is an integral part of the assert module, offering a range of assertion methods for validating code behavior. The assert() function takes two parameters: a value to be tested and an optional error message to be displayed if the assertion fails.
One of the most commonly used assertion methods provided by the assert() function is strict equality checking. This method compares two values using the strict equality operator (===) and throws an error if the values are not strictly equal.
Additionally, the assert() function supports deep equality checking, which compares the properties of objects and arrays recursively to determine if they are deeply equal.
Common Assertion Methods
- assert.ok(value[, message]): Throws an error with an optional message if the value is falsy.
- assert.strictEqual(actual, expected[, message]): Asserts that the actual and expected values are strictly equal without type conversion.
- assert.deepEqual(actual, expected[, message]): Asserts that the actual and expected values are deeply equal, performing type conversion.
- assert.throws(block[, error][, message]): Asserts that the block function throws an error, with an optional error parameter to validate the thrown error type.
- assert.doesNotThrow(block[, error][, message]): Asserts that the block function does not throw an error.
How to Validate Server Behavior with Assert: A Test-Driven Approach
Assuming we have a simple Node.js server that handles GET requests and returns a welcome message. We will use the assert module to write test cases to validate the server's behavior.
First, install the required dependencies:
npm install express
Then create a file named server.js and write the following code:
const assert = require('assert');
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Node.js assert!');
});
const server = app.listen(3000, () => {
console.log('Server is running on port 3000');
});
// Test cases
assert.ok(server !== undefined, 'Server should be defined');
assert.strictEqual(2 + 2, 4, '2 + 2 should be equal to 4');
console.log('All tests passed');
In the above code, we first create a simple Express server, then write two test cases using different assertion methods to validate the behavior of the server and a mathematical expression. Running this script will output "All tests passed" if all tests pass.

Apidog: Enhanced API Testing for Node.js Developers
As a Node.js developer, handling APIs is a common task to ensure the proper functioning of your application. In such scenarios, having a robust interface testing tool is essential.
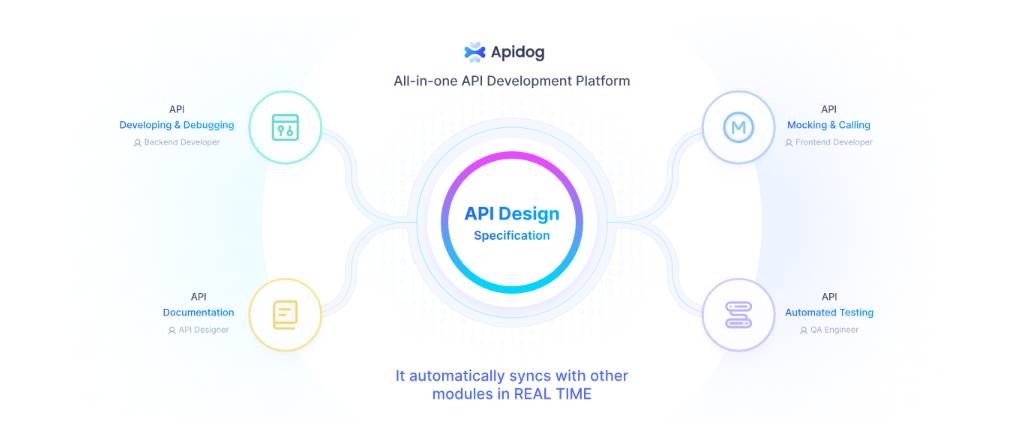
Apidog emerges as a more powerful interface testing tool compared to Postman. Apidog offers the combined features of Postman, Swagger, Mock, and JMeter. It supports debugging interfaces of various protocols including HTTP(S), WebSocket, Socket, gRPC, and more, and seamlessly integrates with the IDEA plug-in.
Once the backend team finishes writing the service interface, Apidog can be utilized to verify its correctness during the testing phase. With its intuitive graphical interface, Apidog significantly enhances the efficiency of project launches.
Conclusion:
In conclusion, the assert module in Node.js provides a powerful set of assertion functions for ensuring code reliability and correctness. By incorporating assertion statements into their code and writing tests using the assert() function, developers can validate assumptions, detect errors, and improve the quality of their Node.js applications.
As you continue to explore Node.js development, mastering the assert module will empower you to write more robust and reliable code, ultimately leading to better software outcomes. So, embrace the assert module in your testing workflows, and let it guide you towards building high-quality Node.js applications.