Getting Started on API Automation Testing Framework
This article provides you with a comprehensive learning guide on interface automation testing frameworks to help you get started quickly and build an efficient testing tool.
API Testing
API (Application Programming Interface) testing is a type of software testing that focuses on verifying the functionality, reliability, performance, and security of APIs. An API is a set of rules, protocols, and tools that allow different software applications to communicate with each other.
4 Steps for API Testing
Generally, there are four basic steps involved in interface testing:
- Step 1: Preparing the interfaces to be tested.
- Step 2: Set up the expected results.
- Step 3: Testing the interfaces using a testing framework or an API tool.
- Step 4: Check the results to see if they match. However, the actual process can be more complex since continuous integration, testing logic, and testing reports must also be considered.
Plan for API testing
There are two different approaches to interface testing. One is using an automated testing framework written in programming languages, and another is using API tools. The most popular testing framework is Python+Pytest+Allure, while API tools like Apidog are also quite useful and convenient.
Using Automated Testing Frameworks to Test APIs
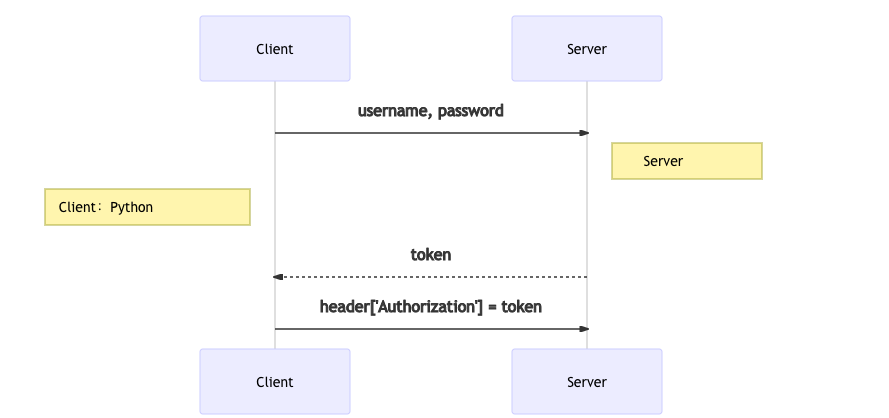
The technology stacks involved are: Requests, Python, Pytest, and Allure, and the next one or two are explained to you.
Requests
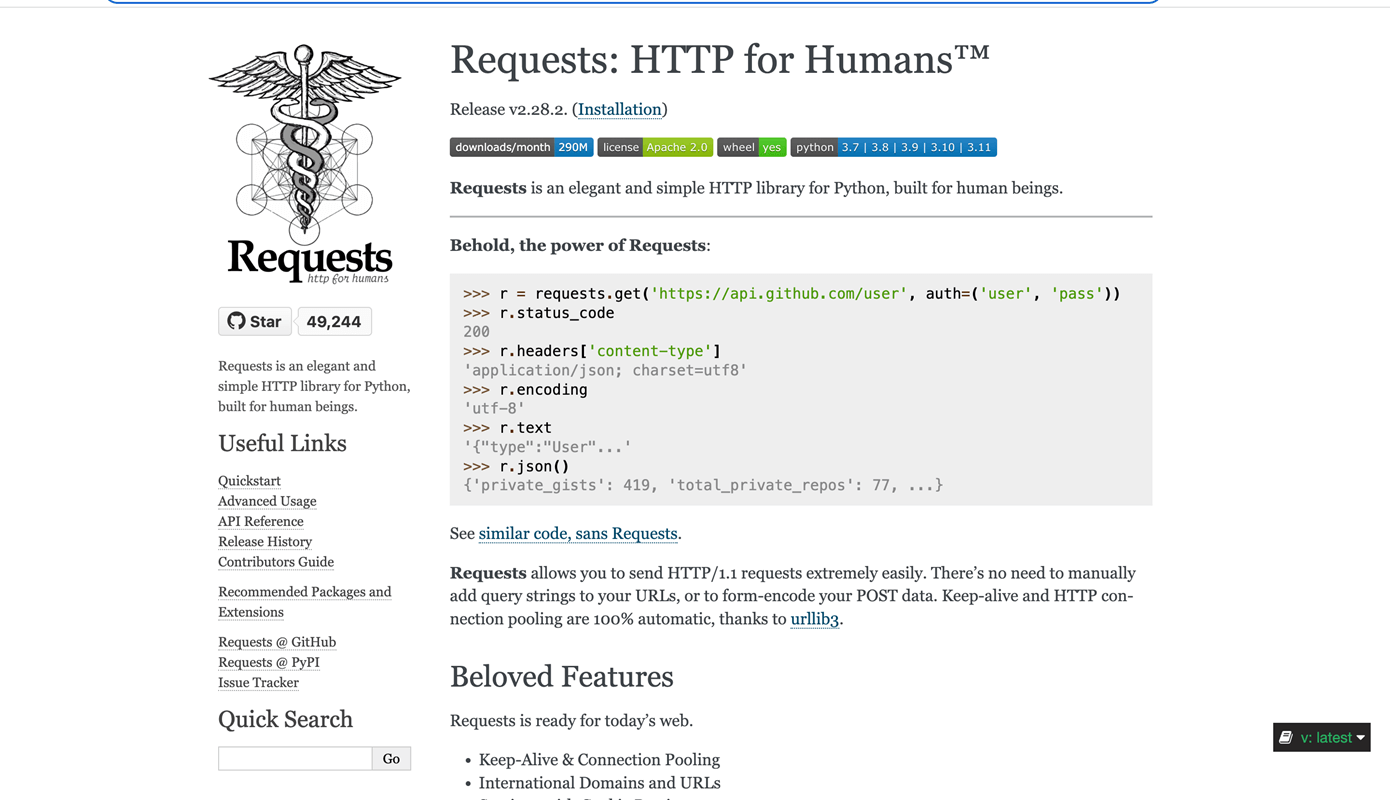
You can use Requests to send requests to the interface and get the returned responses, and Requests support almost all request methods.
r = requests.get(url, params, kwargs)
r = requests.post(url, data, json, kwargs)
And you can modify the response results according to the actual situation.
r.json()
r.text()
These are the main parameters in the response.
# HTTP response staus code
r.status_code
# Reason for response failure
r.reason
# Request object corresponding to the response
r.request
# Convert response data to text
r.text
# URL where the response is from
r.url
# Response content
r.content
Requests also support Session, which is used for authentication and other operations.
s = requests.Session()
s.header[key] = value
s.auth = (username, password)
Pytest
Pytest is an automated testing framework for Python, and its derived plugins include allure-pytest, which supports parameterization and can be integrated with Jenkins. It also supports parameterization and other features.
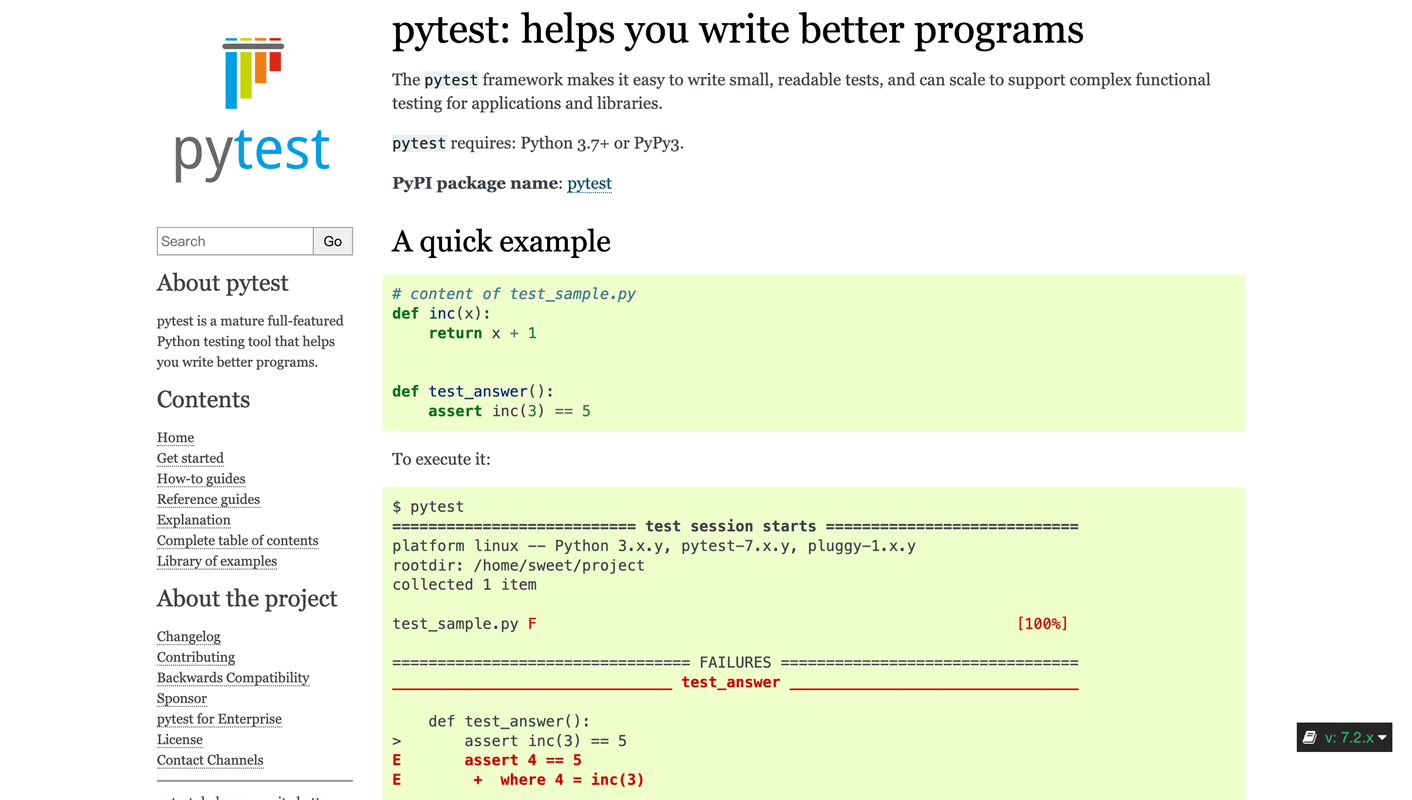
The basic test code can be written below:
@pytest.mark.login
def test_login():
pass
@pytest.mark.logout
def test_logout():
pass
Pytest supports specific execution of a class, a function, or a file, and is very flexible.
debugging in the main file
# specify running a single testing directory
pytest.main(['./test_case'])
# specify running a single testing file
pytest.main(['./test_case/test_func.py'])
# specify running a test class
pytest.main(['./test_case/test_func.py::TestFunc'])
# specify running a specify method in test class
pytest.main(['./test_case/test_func.py::TestFunc::test_add_by_class'])
# specify running a single test function
pytest.main(['./test_case/test_func.py::test_add_by_func_aaa'])
Pytest.ini supports direct output to logs.
[pytest]
log_cli = 1
log_cli_level = DEBUG
log_cli_date_format = %Y-%m-%d-%H-%M-%S
log_cli_format = %(asctime)s - %(filename)s - %(module)s - %(funcName)s - %(lineno)d - %(levelname)s - %(message)sv
log_file_level = DEBUG
log_file_date_format = %Y-%m-%d-%H-%M-%S
log_file_format = %(asctime)s - %(filename)s - %(module)s - %(funcName)s - %(lineno)d - %(levelname)s - %(message)s
log_file = ./log/Test.log
Allure
Allure is used for generating test reports and it is integrated into many frameworks such as Pytest, Junit, TestNG, and more. Additionally, Allure provides some decorators to transform Pytest test cases, all of which are ultimately intended to prepare the test report.
@allure.feature # Used to describe the requirements of the product being tested
@allure.story # Used to describe the user scenarios of a feature, i.e., testing requirements
with allure.step # Used to describe testing steps that will be output to the report
allure.attach # Used to input some additional information into the test report, usually some testing data, screenshots, etc.
@pytest.allure.step # Used to output some common functions as testing steps to the report, and calling the function will output the step to the report.
Using Apidog to test API interfaces
Apidog is an integrated API collaboration platform where you can perform various operations, such as API documentation, API debugging, API mocking, and API automation. It also provides a comprehensive API management solution.
By using Apidog, you can design, debug, test, and collaborate on your APIs on a unified platform, eliminating the need to switch between different tools and the problem of inconsistent data. The data is updated in real-time, which is more conducive to achieving efficient work efficiency.
Apidog is a more advanced API design/development/testing tool. Using Apidog for API testing is your best choice, as it does not require you to perform too many complex operations. You only need to click a few times, and you don't need to write a lot of code, just the basic test scripts.
Apidog Automated Testing
Apidog provides automated testing functionality, but you must first define the API interfaces you need to test, such as defining five interfaces here and writing two simple test scripts for each interface.
- Test if the status code is 200.
- Test if the response time is less than 200ms.
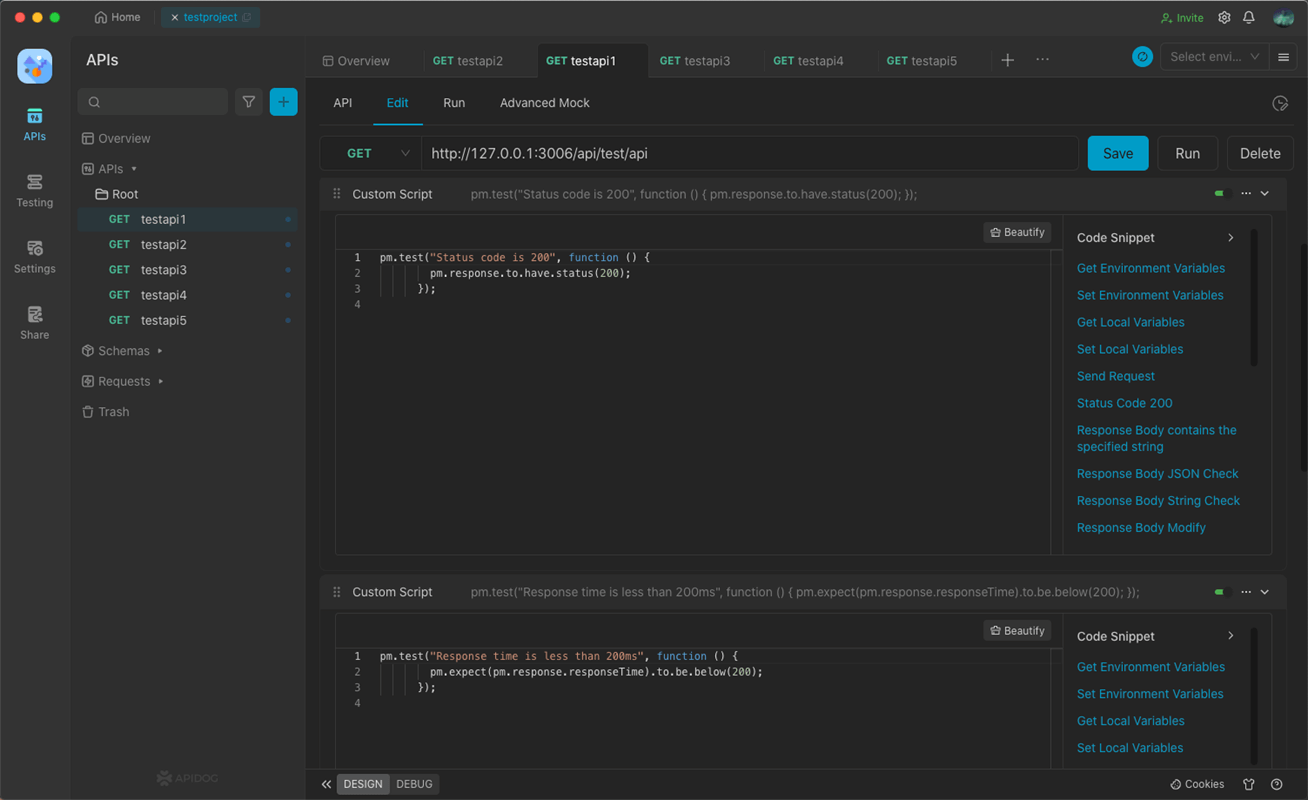
After defining the interface, you need to enter the automated testing process and import the interface into Apidog. Apidog supports the import of interfaces and interface test cases, and you can flexibly choose according to your needs. Next, you need to set some parameters, such as the number of loops, delay time, threads, and environment. These parameters are relatively semantic and easy to understand.
Bonus Tips:
- Number of loops: Number of times to run the test
- Delay time: Delay time between each loop
- Number of threads: Number of lines to run
- Environment: Testing environment to run the test in.
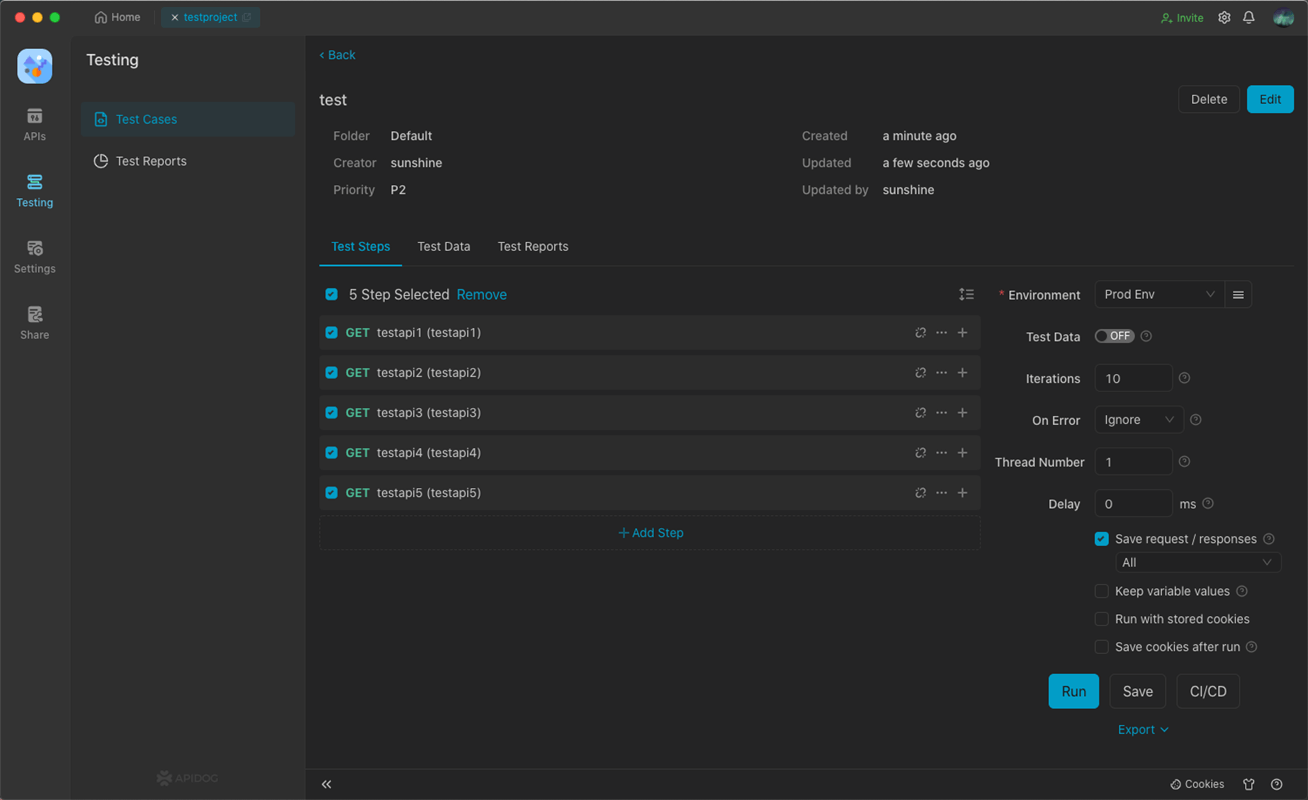
Apidog Test Reports
After setting the parameters, you need to run it to see the results after running. Apidog provides a one-click export report function, so you can export the test report for viewing after running.
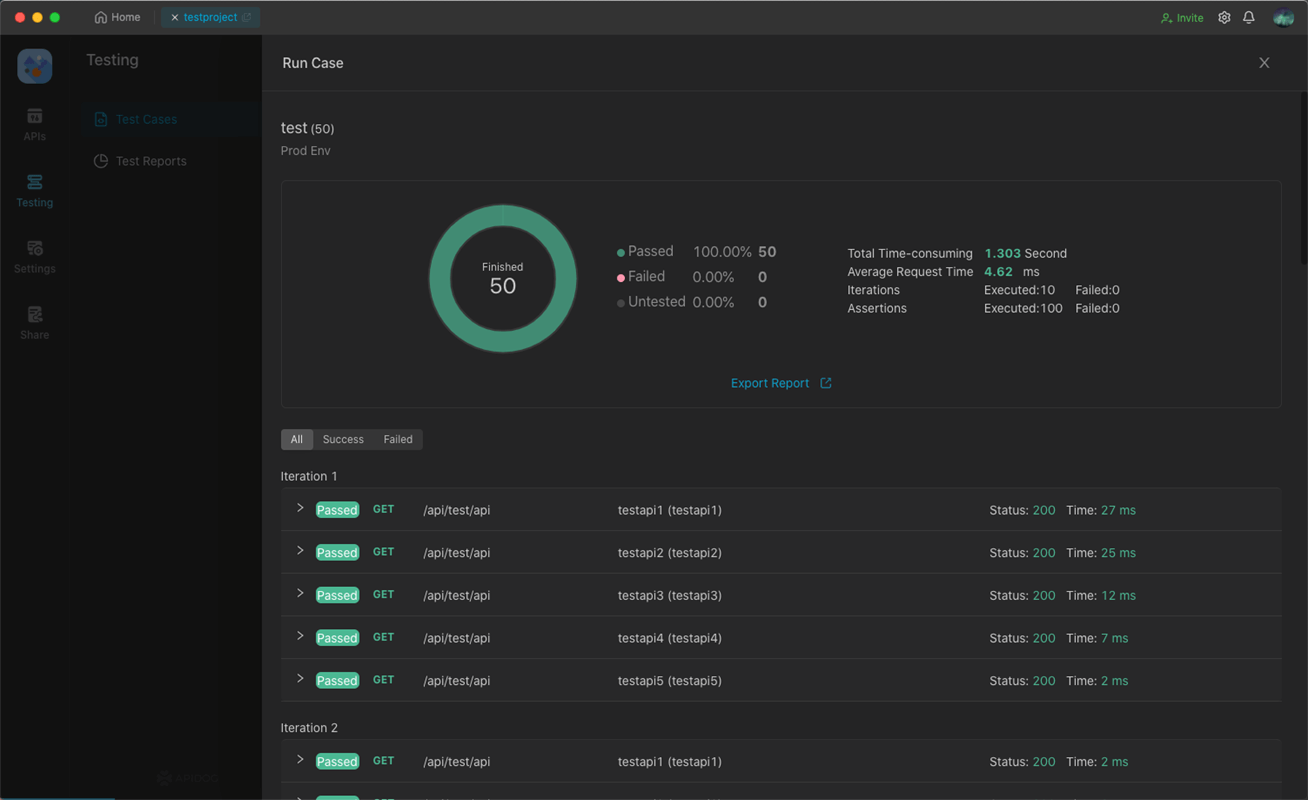
After exporting, you can see the test report on the HTML page, which contains some important information about the test and how each interface was tested.
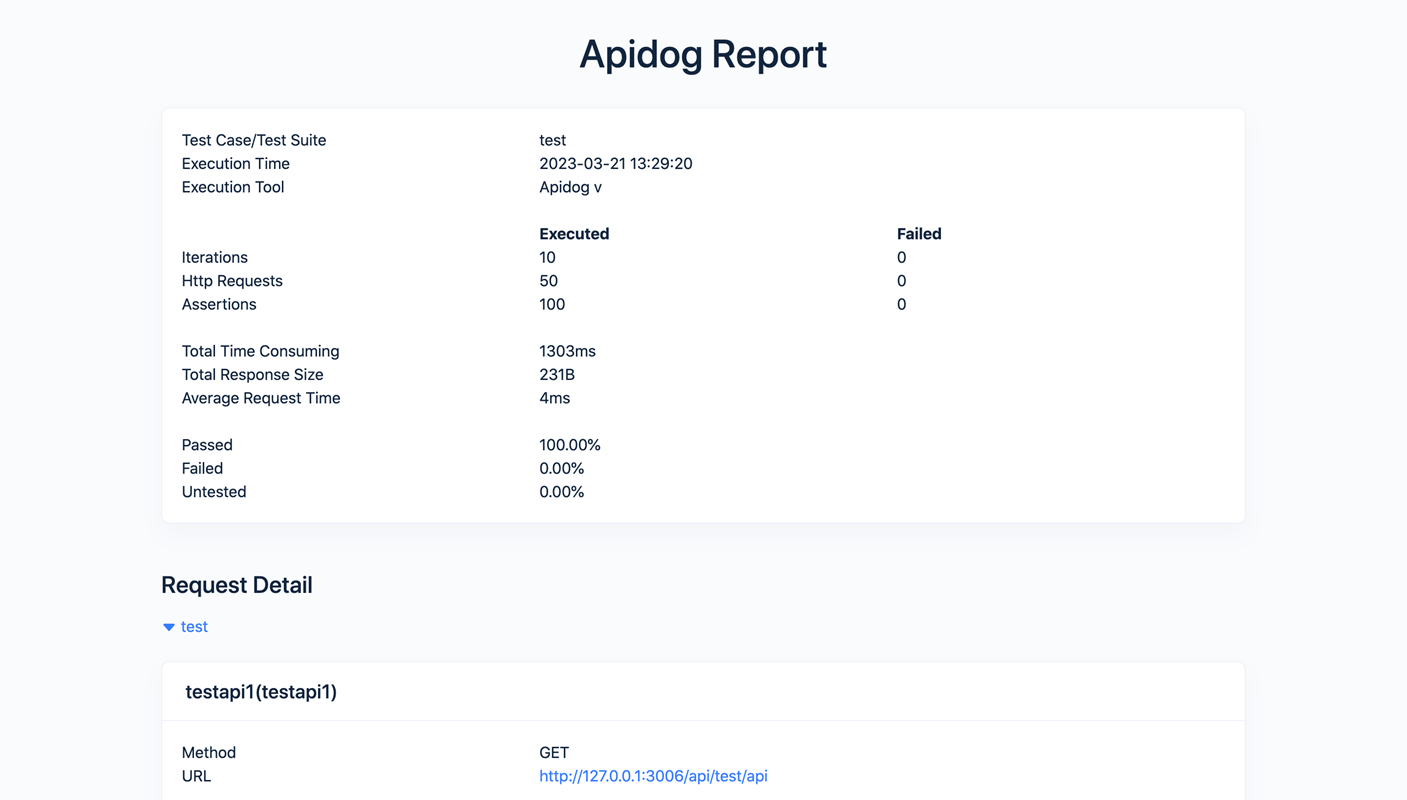