Mastering API Testing with React Mock API and Axios
Discover how to master API testing in React with Axios and mock APIs. Learn the essentials of setting up Axios, creating mock APIs, and enhancing your testing workflow with Apidog.
Ever found yourself in the middle of a React project, stuck on how to test your API calls without hitting the actual server every time? If you have, you're in the right place. Today, we're diving deep into the world of React mock API with Axios. Whether you're a seasoned developer or just starting, this guide will walk you through everything you need to know.
What is API Testing?
API testing is a type of software testing that involves testing application programming interfaces (APIs) directly. It checks the functionality, reliability, performance, and security of the APIs. In simpler terms, it’s about ensuring that your app communicates correctly with other systems, services, or applications.
Why is API Testing Important?
APIs are the backbone of modern web applications. They allow different systems to interact seamlessly. Without robust API testing, you risk having faulty interactions which can lead to a poor user experience, data breaches, and system failures.
Understanding Axios in React
If you’ve been working with React, you’ve probably heard of Axios. It’s a promise-based HTTP client for JavaScript, making it a breeze to send asynchronous HTTP requests to REST endpoints.
Key Features of Axios:
- Easy to use: With a simple and intuitive API.
- Supports Promises: Making it perfect for handling asynchronous requests.
- Interceptors: Allow you to transform requests and responses.
- Cancellation: Easily cancel requests.
Why Mock APIs are Essential
Mock APIs play a crucial role in development and testing. They allow you to simulate responses from your API without hitting the actual server. This means you can develop and test your frontend independently from your backend.
Benefits of Mock APIs:
- Speed: Reduce the time spent waiting for API responses.
- Isolation: Test frontend logic without backend dependencies.
- Consistency: Ensure consistent responses for predictable tests.
Setting Up Axios in a React Project
Let’s get our hands dirty and set up Axios in a React project. If you don’t have a React project yet, create one using Create React App.
npx create-react-app my-app
cd my-app
npm install axios
Now, let's create a service to handle our API calls. Create a new file apiService.js
in the src
directory:
import axios from 'axios';
const apiService = axios.create({
baseURL: 'https://api.example.com',
timeout: 1000,
headers: {'X-Custom-Header': 'foobar'}
});
export default apiService;
Creating a Mock API with Axios
Mocking API responses in Axios is straightforward. We’ll use axios-mock-adapter
, a simple library to mock Axios requests.
Install axios-mock-adapter
:
npm install axios-mock-adapter
Now, let’s create our mock API. In src
, create a new file mockApi.js
:
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
// This sets the mock adapter on the default instance
const mock = new MockAdapter(axios);
// Mock any GET request to /users
// arguments for reply are (status, data, headers)
mock.onGet('/users').reply(200, {
users: [{ id: 1, name: 'John Smith' }]
});
// Mock any POST request to /login
// arguments for reply are (status, data, headers)
mock.onPost('/login').reply(200, {
user: { id: 1, name: 'John Smith' },
token: 'abcd1234'
});
With the mock API set up, you can now test your components without hitting the real server.
Integrating Apidog for Enhanced API Testing
Apidog is a powerful tool that enhances your API testing capabilities. It allows you to design, test, and mock APIs all in one place. Here's how to integrate Apidog with your React project.
To test your API with Apidog, you need to follow these steps:
Step 1: Open Apidog and create a new request.
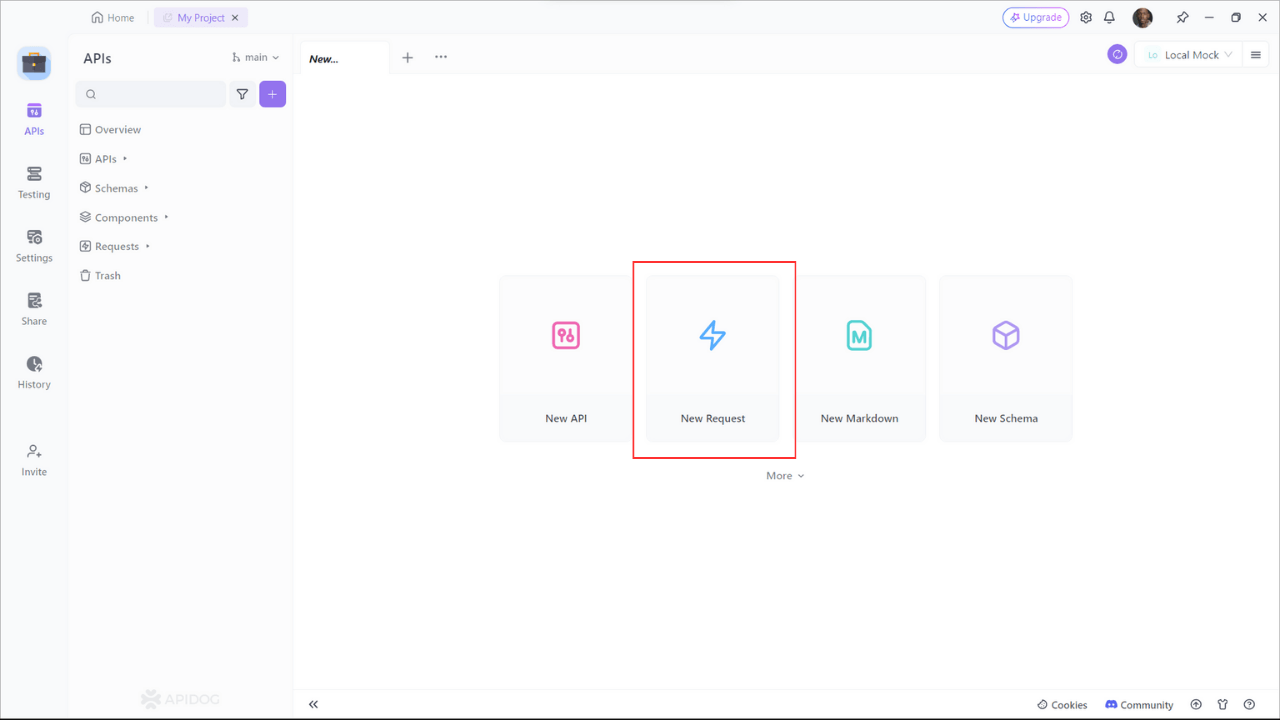
Step 2: In the test editor, enter the URL of your API endpoint, select the HTTP method, and add any headers, parameters, or body data that you need. For example, you can test the route that returns a simple message that we created earlier:
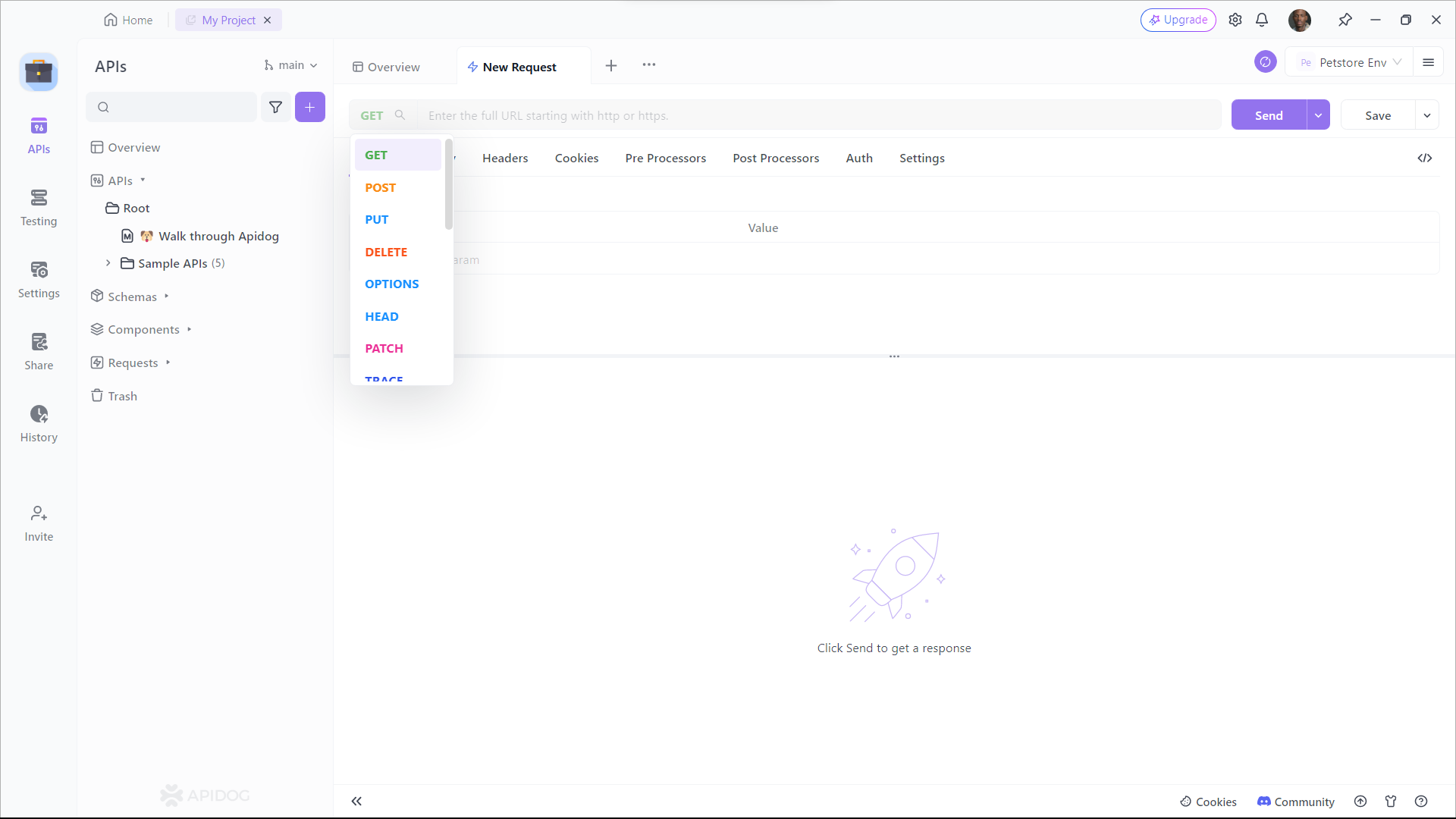
Step 3: Click on the Send button and see the result of your test. You should see the status code, the response time, and the response body of your API. For example, you should see something like this:
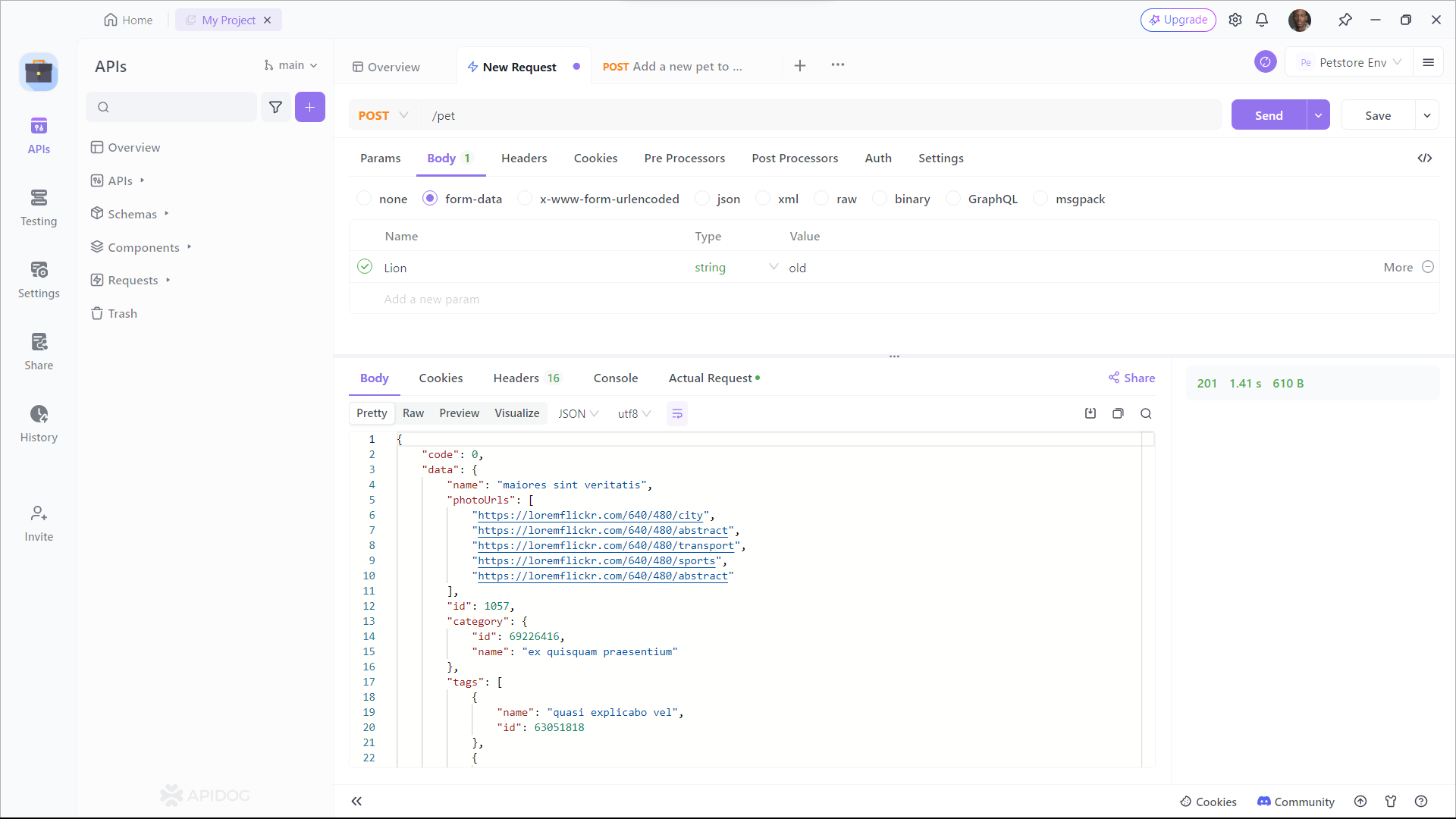
Apidog is a great tool for testing your APIs, as it helps you ensure the quality, reliability, and performance of your web services. It also saves you time and effort, as you don’t need to write any code or install any software to test your APIs. You can just use your browser and enjoy the user-friendly interface and features of Apidog.
Step-by-Step Guide to Mastering Mock APIs with Apidog
Step 1. Create a New Project: Apidog uses projects to manage APIs. You can create multiple APIs under one project. Each API must belong to a project. To create a new project, click the "New Project" button on the right side of the Apidog app homepage.
Step 2. Create a New API: To demonstrate the creation of an API for user details, follow these steps:
- Request method: GET.
- URL: api/user/{id}, where {id} is the parameter representing the user ID.
- Response type: json.
- Response content:
{
id: number, // user id
name: string, // username
gender: 1 | 2, // gender: 1 for male, 2 for female
phone: string, // phone number
avatar: string, // avatar image address
}
To create a new interface, go to the homepage of the previously created project and click on the "+" button on the left side.
Fill in the corresponding interface information and save it.
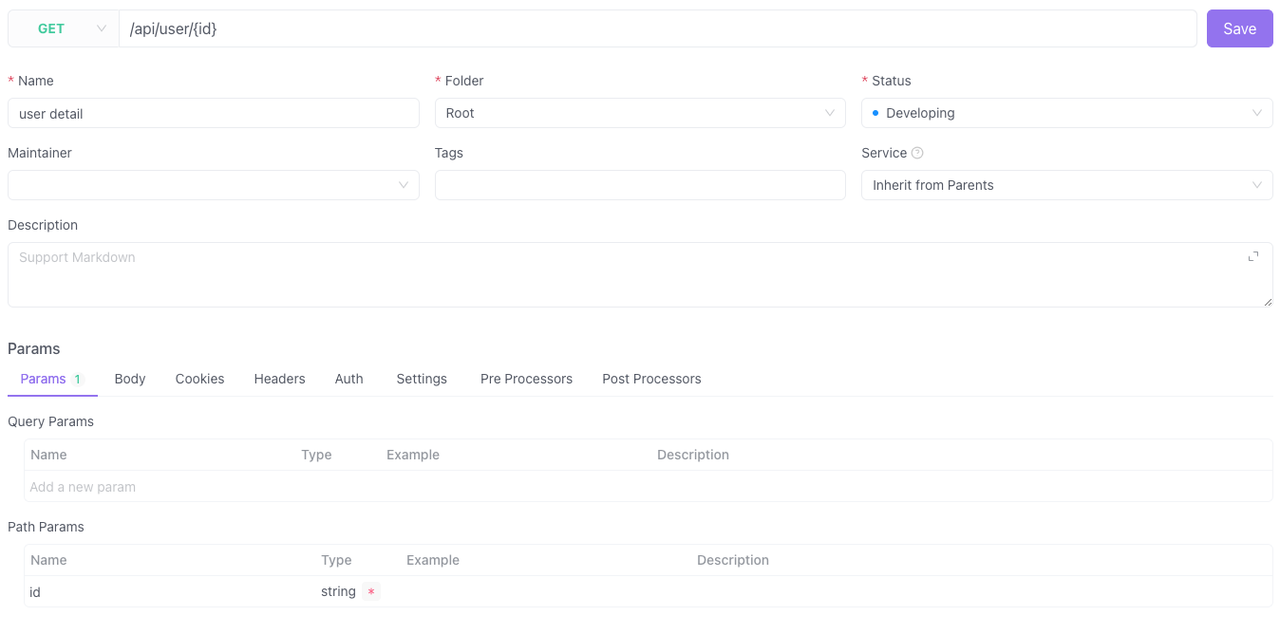
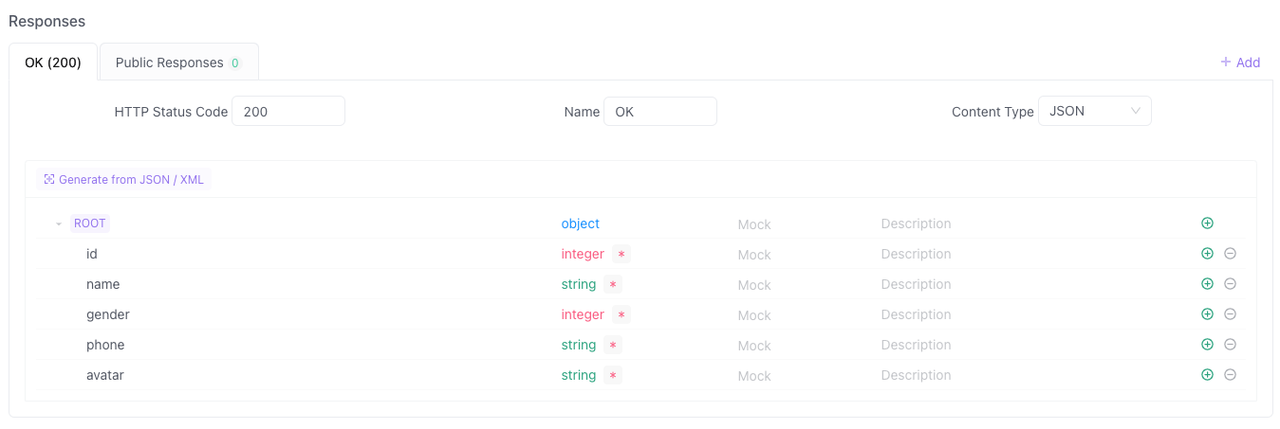
With this, the user detail interface has been created. Meanwhile, Apidog has automatically generated a mock for us based on the format and type of the response fields. Click on the " Request" button under Mock to view the mock response.
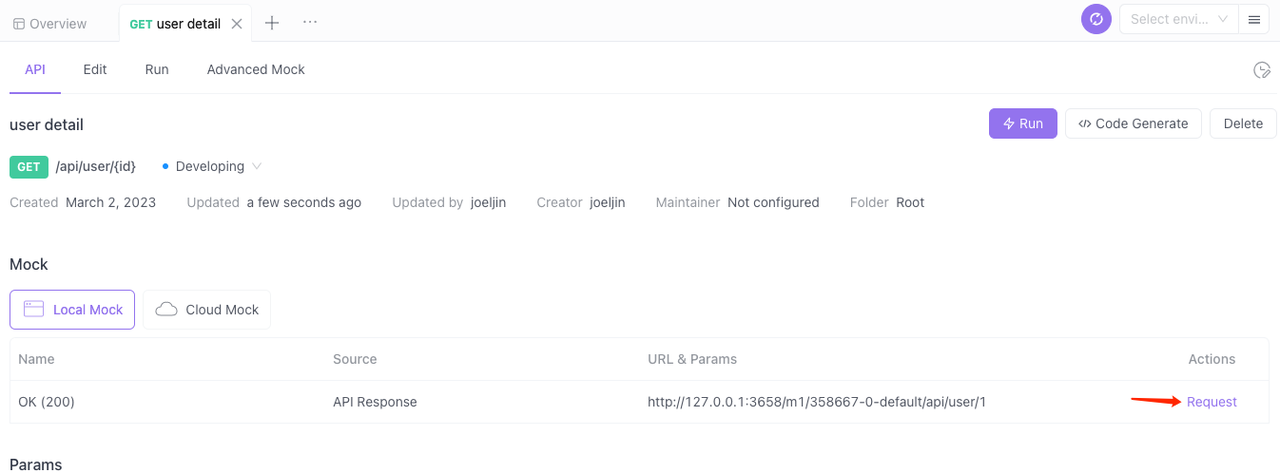
Let's take a look at the mock response. Click on the "Request" button and then click "Send" on the page that opens.
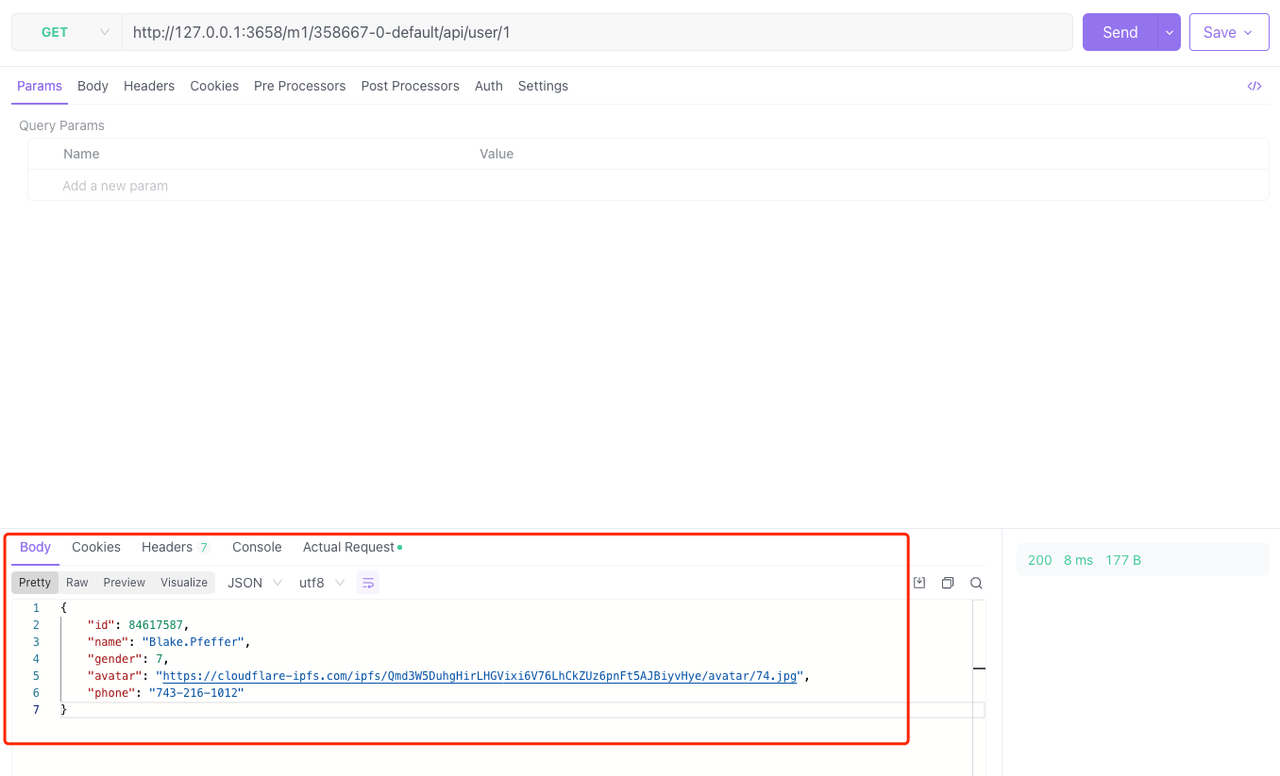
Step 3. Set Mock Matching Rules: Have you noticed something magical? Apidog set the "name" field to string type, yet it returns names; set the "phone" field to string type, yet it returns phone numbers; set the "avatar" field to string type, yet it returns image addresses.
The reason is that Apidog supports setting matching rules for Mock. Apidog has built-in rules, and users can also customize their own rules. These rules can be found in Project Settings > Feature Settings > Mock Settings.
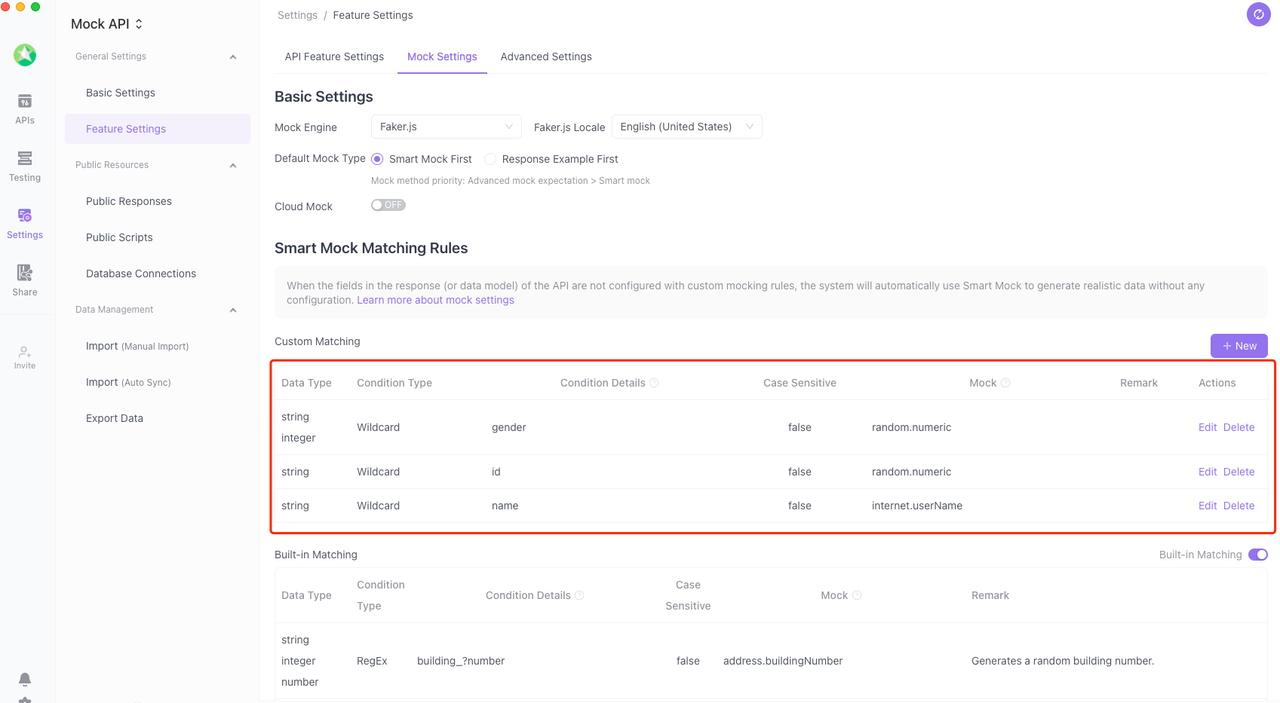
You can also set dedicated Mock rules for each field. Click on the "Mock" next to the field:
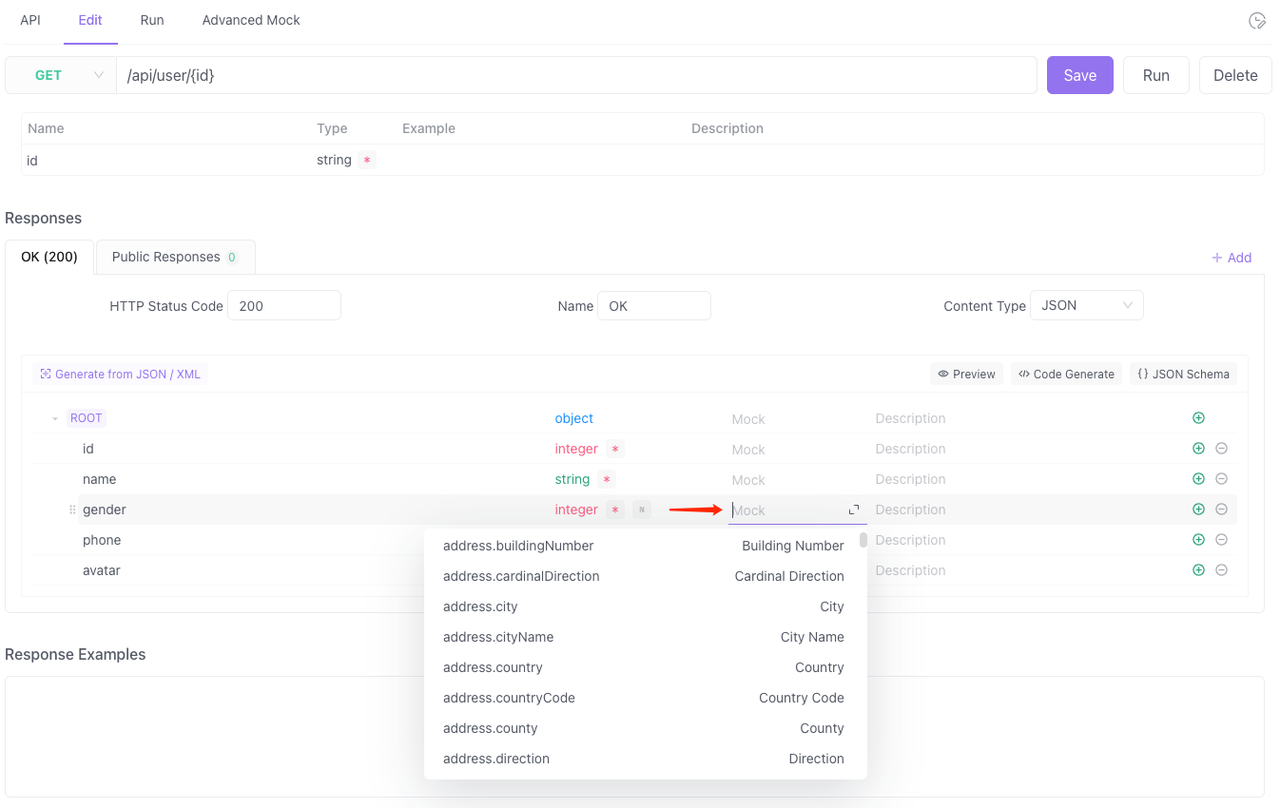
Apidog Mock matching rules are as follows:
- When a field meets a matching rule, the response will return a random value that satisfies the Mock rule.
- If a field does not meet any matching rule, the response will return a random value that meets the data type of the field.
There are three types of matching rules:
- Wildcards: * matches zero or more characters, and ? matches any single character. For example, *name can match user name, name, and so on.
- Regular expressions.
- Exact match.
Mock rules are fully compatible with Mock.js, and have extended some syntax that Mock.js does not have (such as domestic phone numbers "@phone"). Commonly used Mock rules include:
- @integer: integer. @integer(min, max).
- @string: string. @string(length): specifies the length of the string.
- @regexp(regexp): regular expression.
- @url: URL.
During development, we may encounter flexible and complex Mock scenarios, such as returning customized content based on different request parameters. For example, return normal user information when the ID is 1, and report an error when the ID is 2. Apidog also supports these scenarios, and those interested can check out the Advanced Mock document.
Best Practices for Mocking APIs
Mocking APIs is a powerful technique, but it’s essential to follow best practices to ensure effective testing.
1. Keep Mocks Up-to-Date
Ensure your mock APIs reflect the latest changes in your real APIs. This avoids discrepancies and ensures accurate testing.
2. Use Realistic Data
Use realistic data in your mocks to simulate real-world scenarios. This helps identify issues that might arise in production.
3. Limit Mock Usage
While mocks are great for testing, don’t rely on them exclusively. Test with real APIs whenever possible to catch issues that mocks might miss.
Common Pitfalls and How to Avoid Them
Mocking APIs can introduce challenges if not done correctly. Here are some common pitfalls and how to avoid them.
1. Over-Mocking
Relying too heavily on mocks can give a false sense of security. Balance mock tests with integration tests using real APIs.
2. Inconsistent Mocks
Ensure consistency in your mock data to avoid flaky tests. Use tools like Apidog to generate consistent mock data.
3. Ignoring Edge Cases
Don’t just test the happy paths. Use mocks to simulate edge cases and ensure your application handles errors gracefully.
Conclusion
Mocking APIs with Axios in a React project can significantly improve your development workflow and testing efficiency. By using tools like Apidog, you can streamline your API testing process, ensuring your application is robust and reliable.
Remember, while mocks are powerful, they should complement, not replace, testing with real APIs. Keep your mocks up-to-date, use realistic data, and balance mock tests with integration tests. Happy coding!