Harnessing the Power of React API Calls
React API calls are a reference to the process of how React applications send a request to an external web API and receive a response. Without React API calls, it would not be possible for your application to communicate and exchange data with other third-party systems.
React is a free, open-source JavaScript library that many web developers use to create sophisticated and alluring web pages. It is also part of the popular tech development framework stack MERN that many developers rely on to create web or mobile apps.
If you have API files that you would like to test, you can import them onto Apidog and start testing them out. All you have to do is click the button below to begin! 👇 👇 👇
For React applications to interact or communicate with third-party systems, it has to execute an API call. To fully grasp this concept, this article will introduce React API calls, prime examples of React API calls with code snippets included, and real-world examples of React API calls that are commonly used.
What are [React] API Calls?
API (Application Programming Interface) calls are essential in enabling communication and data between different programs or systems. API calls can be considered the full package, acting as requests and responses between two software.
To understand how React API calls work, a simple demonstration will be shown.
Showing How to Make a React API call
It first begins when a React app needs to access a certain piece of data or functionality from another program. This is when it sends an API call, which can also be referred to as a structured request. The typical API call request will include:
- An endpoint: Endpoints are the specific addresses within the API that define what information is being requested or what action needs to be taken.
- A method: Methods are the types of operations that are being requested. They come in forms such as
GET
to retrieve data records,POST
to create new data records,PUT
to update existing data records, andDELETE
to remove data records. - Any additional data: There are many optional additional information you may choose to include in the request for the receiving program to utilize. These may include additional path or query parameters.
Once the receiving program receives the React API call, it will process your request and send back a response. An API call response will typically include:
- Data requested: The response can include the data that you requested, or perhaps the result of whether the operation or action was a success or not.
- Status code: A status code indicates the state of the call/ There are status codes for success (
200
) and failure (400
and404
).
Positive Aspects of React API Calls
By using API calls for React applications, you will be encouraged to follow a certain structure, which can be assured, will be beneficial for you in the long run.
- Modular development: Building with APIs encourages modular design, allowing developers to build smaller, self-contained components that can easily interact with each other.
- Code reusability: With API calls, code can be reused by multiple programs, promoting efficient code development and maintenance.
- Data sharing: APIs facilitate data exchange between different systems, enabling powerful and integrated functionalities.
Real-World Examples of React API Calls
1. E-commerce websites:
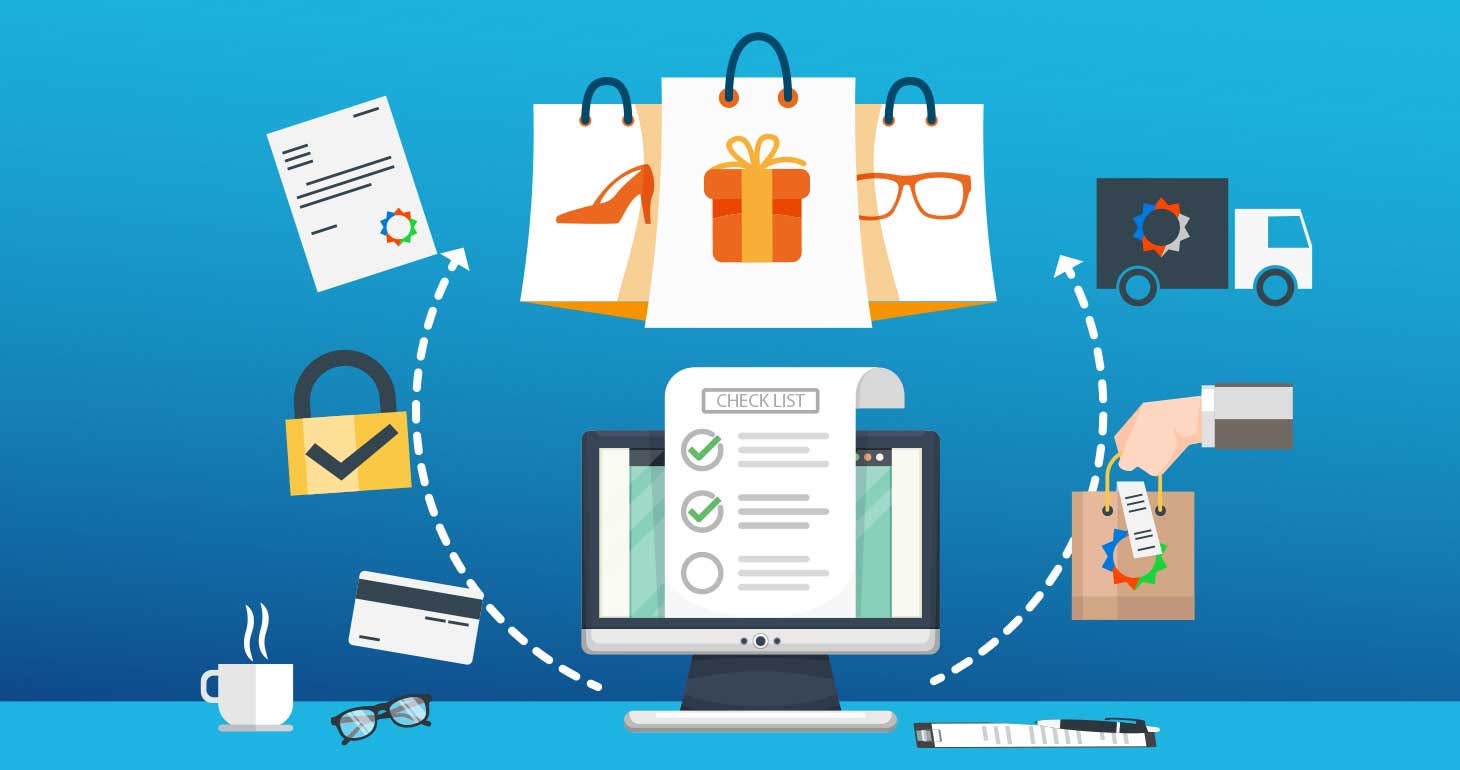
- Product listings: React can fetch product information and images from an API to populate product listings on the website. Users can then filter, sort, and search through these products based on various criteria.
- Shopping carts: When a user adds an item to their cart, React can send an API call to update the shopping cart data on the server, ensuring consistency and allowing further actions like a checkout.
2. Social media platforms:
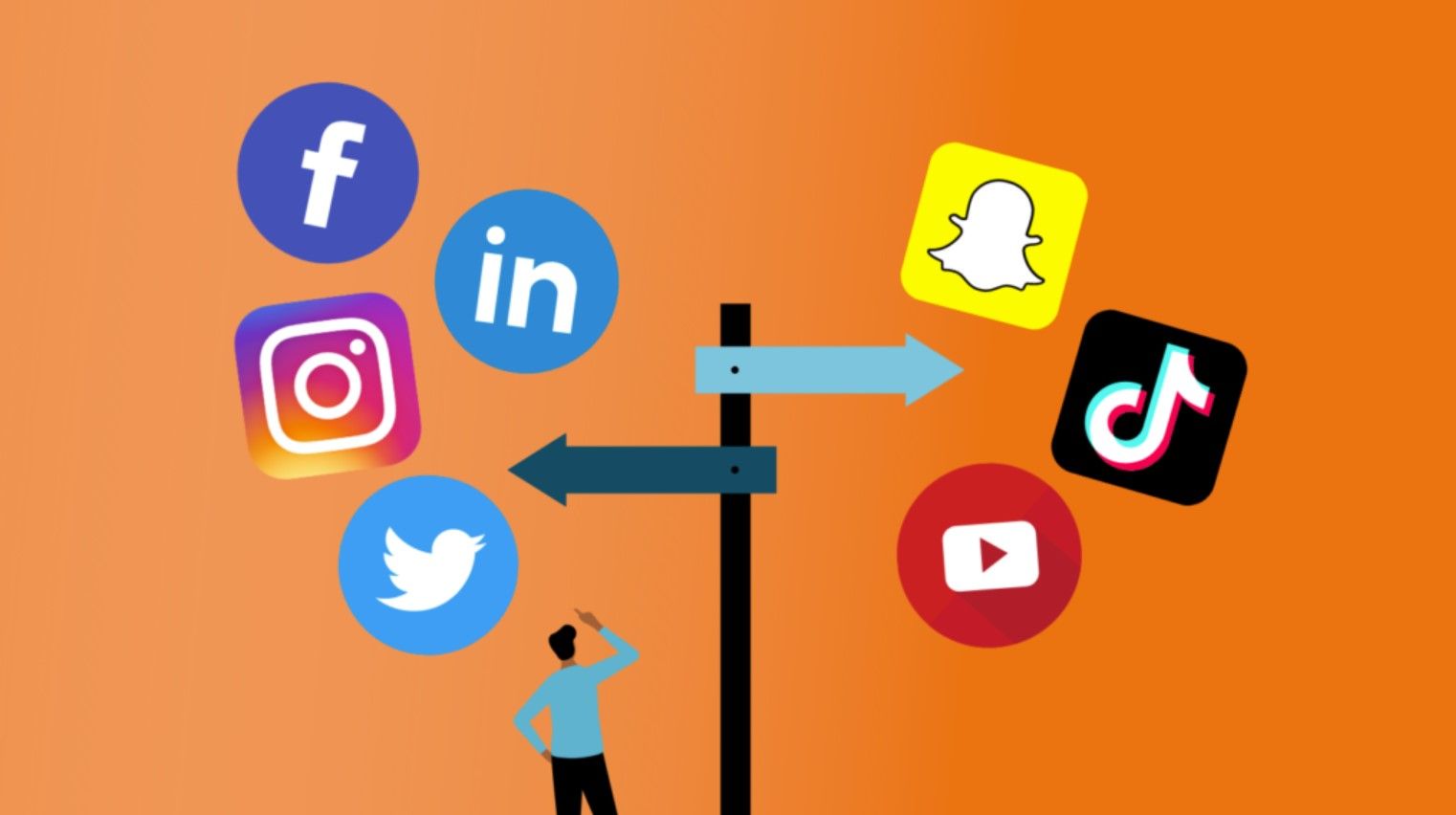
- Feeds and updates: React can fetch data from social media APIs to display user feeds, news updates, or trending topics. This allows for dynamic content updates without full-page reloads.
- Likes, comments, and shares: When a user interacts with a post (likes, comments, shares), React can send an API call to update the corresponding information on the server, reflecting the action and updating the UI for other users in real time.
3. Weather applications:
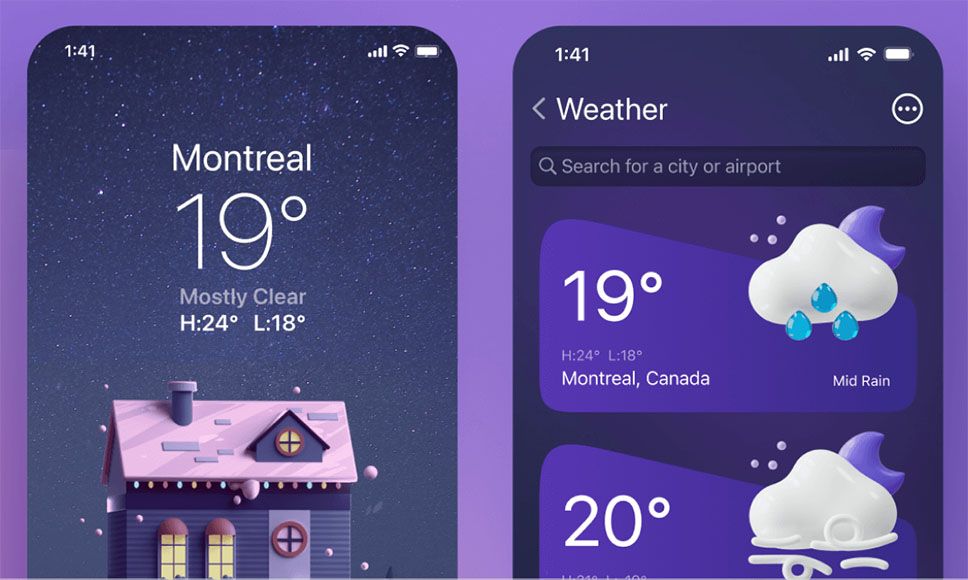
- Fetching weather data: React can use weather APIs to retrieve current and forecast weather data for a specific location. This data can then be displayed in a user-friendly format with beautified visuals.
- Location updates: Based on user input or geolocation, React can dynamically send API calls to update the displayed weather information depending on the user's current location.
React API Call Code Example: Fetching data from Public API Using Fetch API
Here is an example of how to fetch data from a public API using the Fetch API for updating the state of a React application (or component).
import React, { useState, useEffect } from 'react';
function App() {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const jsonData = await response.json();
setData(jsonData);
};
fetchData();
}, []);
return (
<div>
<h1>Fetched data</h1>
{data.length > 0 ? (
<ul>
{data.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
) : (
<p>Loading data...</p>
)}
</div>
);
}
export default App;
Code Explanation:
1. Importing the necessary components:
useState
is used for managing the state of the component (data).useEffect
is used for performing other operations like fetching data.
2. Define the App
component:
useState
hook creates a state variabledata
to store the fetched data, initialized as an empty array.
3. useEffect
hook:
- This hook runs after the component mounts (similar to lifecycle methods).
- the
useEffect
hook defines an async functionfetchData
to fetch data. - The Fetch API is used to send a GET request to the specified API endpoint.
- The response is parsed as JSON using
response.json()
. - The fetched data is then used to update the
data
state usingsetData
.
4. Return statement:
- The return statement renders a
<h1>
element with the title "Fetched data". - The return statement also conditionally renders the data: if
data
has elements, it iterates through them and displays each post's title in an<li>
element. - If data is still loading, it displays a
Loading data...
message.
Apidog - API Development Platform for Testing React API Calls
Apidog is an all-in-one API development tool created with a design-first orientation. With a simplistic and intuitive UI design, Apidog encourages its users to visually design and build APIs.
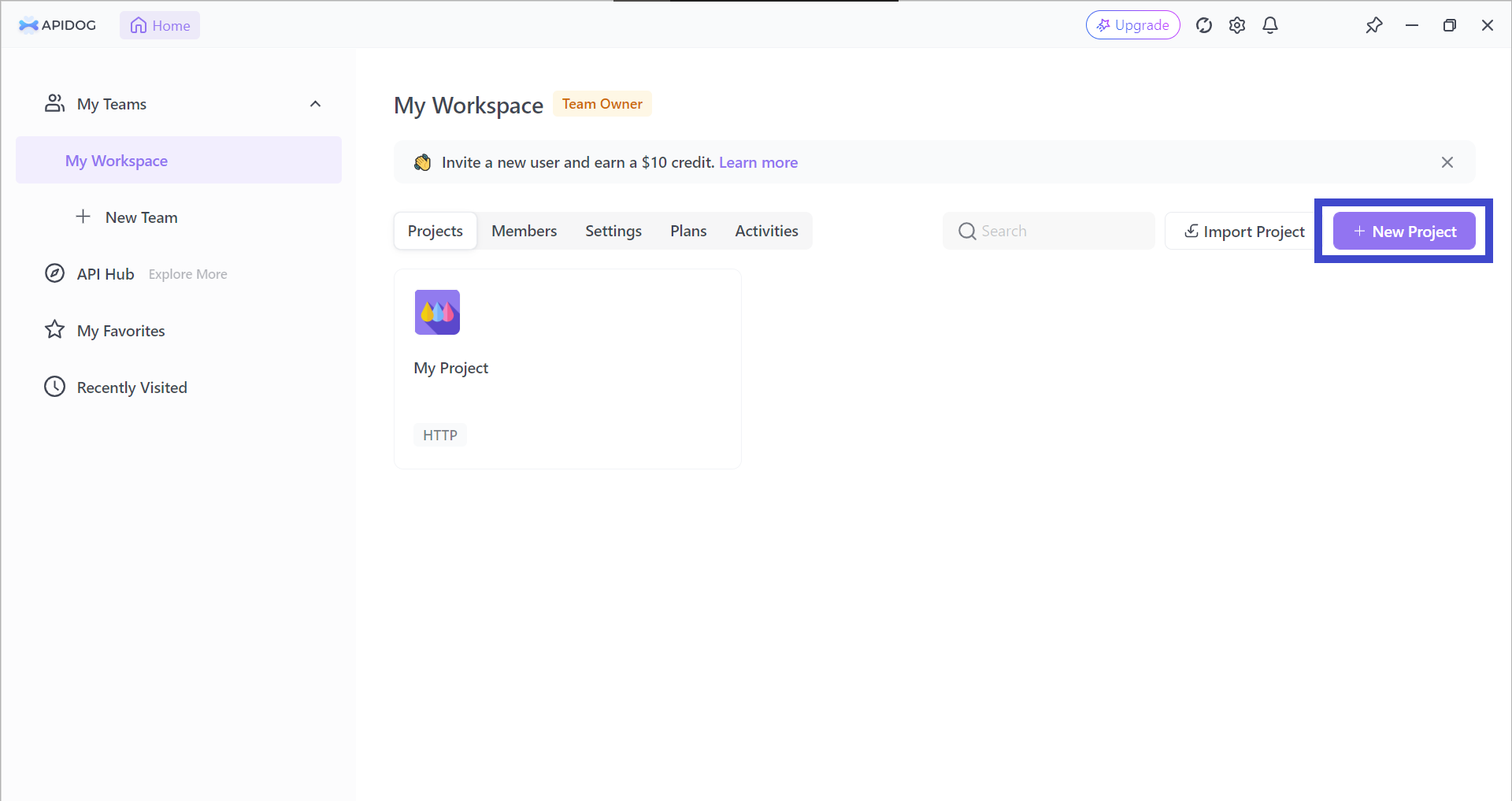
If you need to test your React API calls, you can use Apidog to do so! This next section will show a simple demonstration.
Importing React API Call Onto Apidog
Before we can start testing your React API call, we need to first import the file.
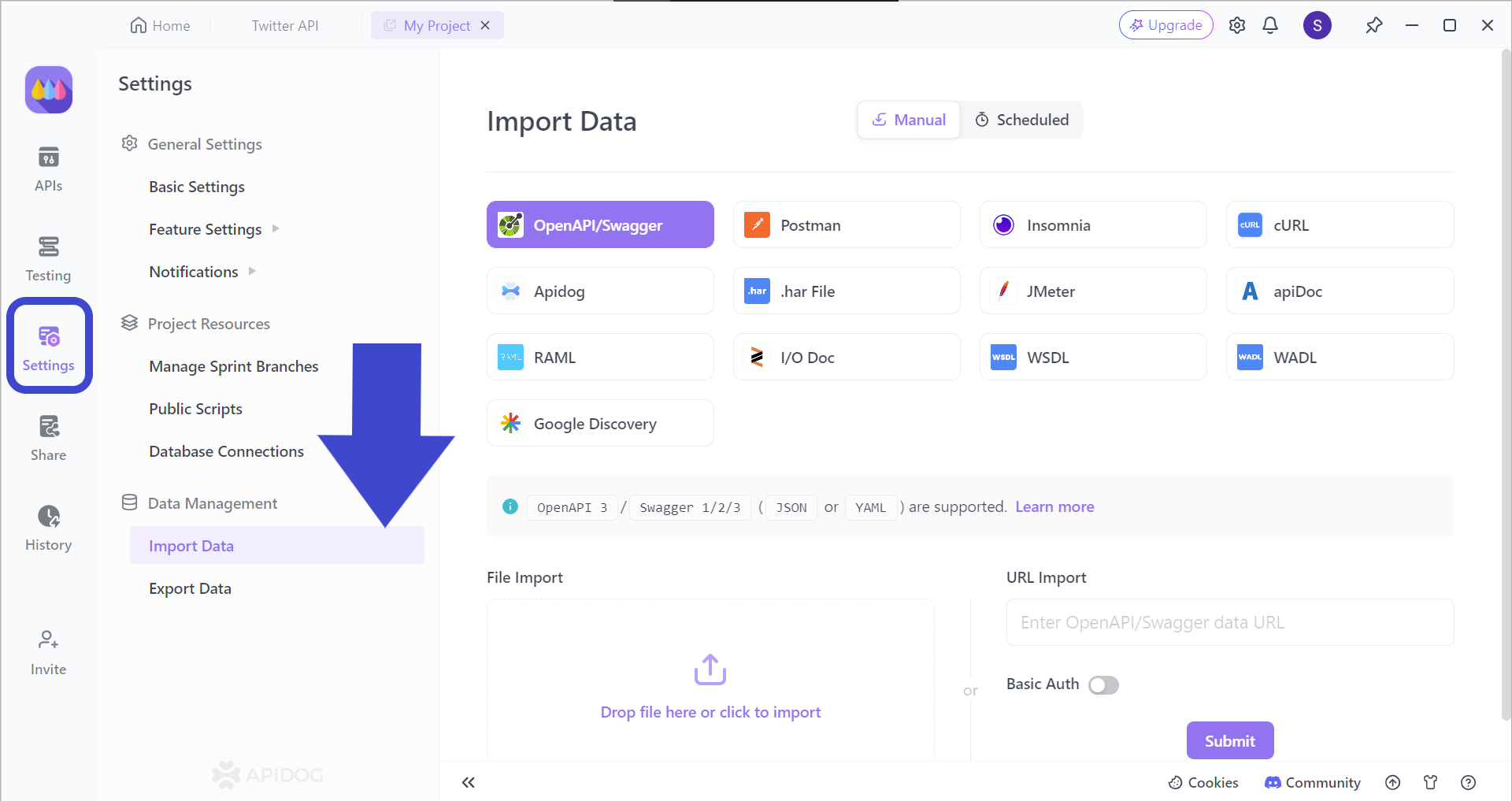
First press the Import Data
section found after pressing the Settings
button, as shown in the picture above. Here, you can drag your React API file to the Apidog window.
Generating React Fetch API Code Using Apidog
If you need help with writing React Fetch API code, you can rely on Apidog to provide you with a one-click client code generator.
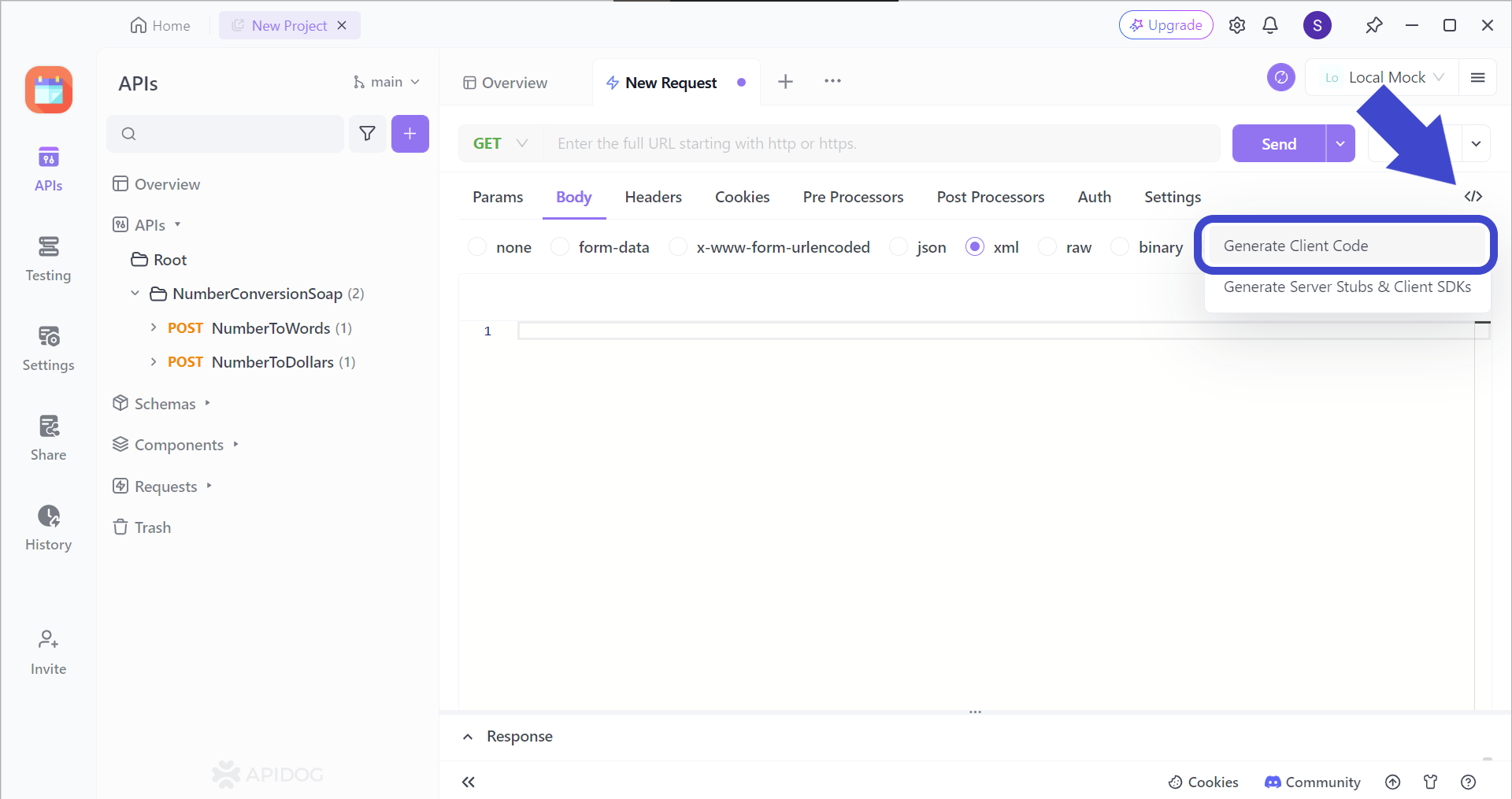
Firstly, locate the </>
button that can be found around the top right corner of the Apidog window application.
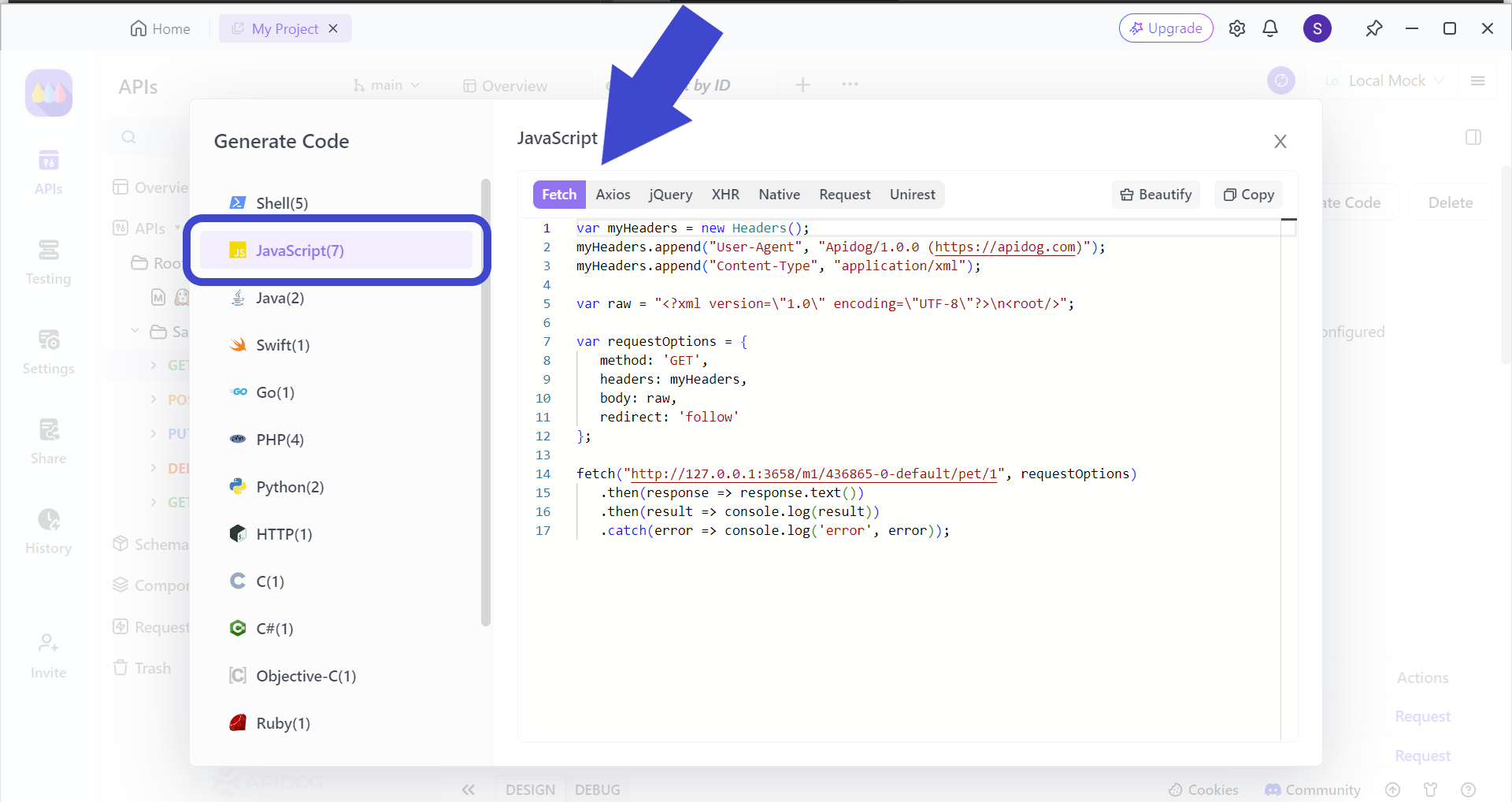
You can then select JavaScript
. The default choice is the Fetch code that we can copy for further use.
Conclusion
React API Calls are an important component to understand, especially if you are planning to create React-based applications. With React API calls, you can create a variety of applications - all you need to do is find compatible APIs that provide the functionalities or data that you need.
Apidog is an suitable all-in-one API platform that provides a comfy yet elegant interface for users to enjoy. If you need a place to test out your React API calls, try out Apidog!