Handling API requests and responses in Python can be a game-changer for your projects. Whether you're building a web application, a mobile app, or even a simple script, understanding how to interact with APIs is crucial. In this comprehensive guide, we'll dive deep into the world of Python requests and responses, giving you the tools you need to become an API master. And hey, don't forget to download Apidog for free - it will make your API development and testing a breeze!
Introduction to APIs
First things first, let's talk about what an API is. API stands for Application Programming Interface. It's a set of rules that allows different software entities to communicate with each other. Think of it as a waiter taking your order (your request) and bringing back your food (the response). APIs are everywhere – from weather apps to social media platforms.
APIs allow developers to access the functionality of other software programs. This can range from simple tasks, like fetching data from a web server, to more complex operations, like interacting with a machine learning model hosted on a cloud service.
Why Use APIs?
Using APIs can save you a lot of time and effort. Instead of reinventing the wheel, you can leverage existing services and data. Plus, it allows your applications to be more versatile and integrated.
Key Terms
Before we dive in, here are some key terms you should know:
- Endpoint: The URL where the API can be accessed.
- Request: The message sent to the API to perform an action.
- Response: The message the API sends back with the result of the request.
- Status Code: A code that indicates the result of the request (e.g., 200 for success, 404 for not found).
Understanding Python Requests
Python's requests
library is a powerful tool for making HTTP requests. It's simple, yet very flexible, allowing you to interact with APIs effortlessly. Let's get started with installing the requests
library.
pip install requests
Now that we have the library installed, let's move on to making our first API request.
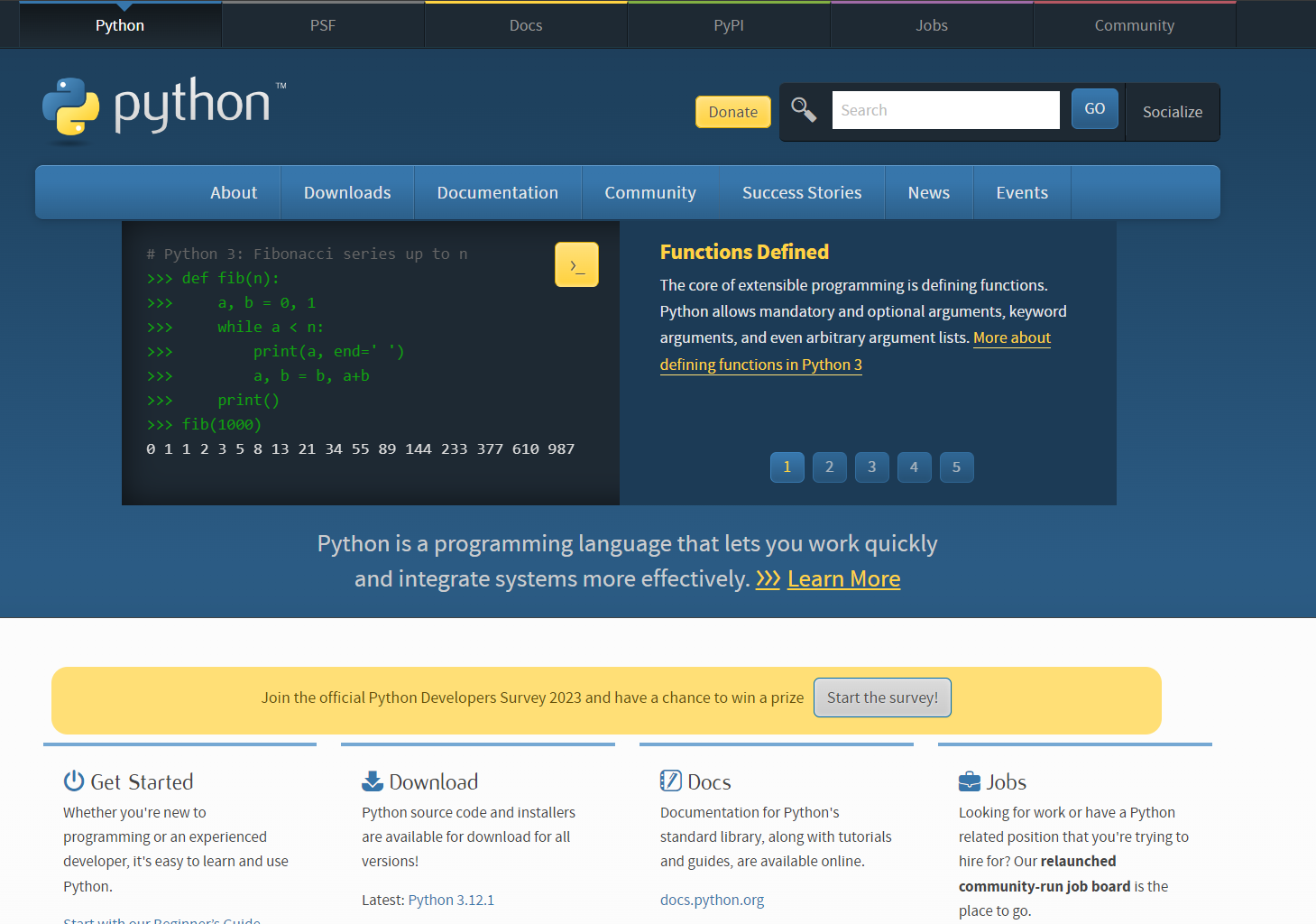
Making Your First API Request
To make an API request, you'll need an API endpoint. For this example, we'll use the JSONPlaceholder API, a free fake online REST API for testing and prototyping.
Here's a simple example of how to make a GET request:
import requests
url = 'https://jsonplaceholder.typicode.com/posts'
response = requests.get(url)
print(response.status_code)
print(response.json())
In this example, we're sending a GET request to the /posts
endpoint. The requests.get
function sends the request, and the response is stored in the response
variable. We then print the status code and the JSON response.
Understanding the Response
The response object contains all the information returned by the server. Here are some useful properties of the response object:
status_code
: The HTTP status code returned by the server.headers
: A dictionary of HTTP headers.text
: The raw response body as a string.json()
: A method that parses the response body as JSON.
Making a POST Request
Sometimes, you'll need to send data to the API. This is where POST requests come in. Here's an example:
import requests
url = 'https://jsonplaceholder.typicode.com/posts'
data = {
'title': 'foo',
'body': 'bar',
'userId': 1
}
response = requests.post(url, json=data)
print(response.status_code)
print(response.json())
In this example, we're sending a POST request to the /posts
endpoint with some JSON data. The requests.post
function sends the request, and the response is handled similarly to the GET request.
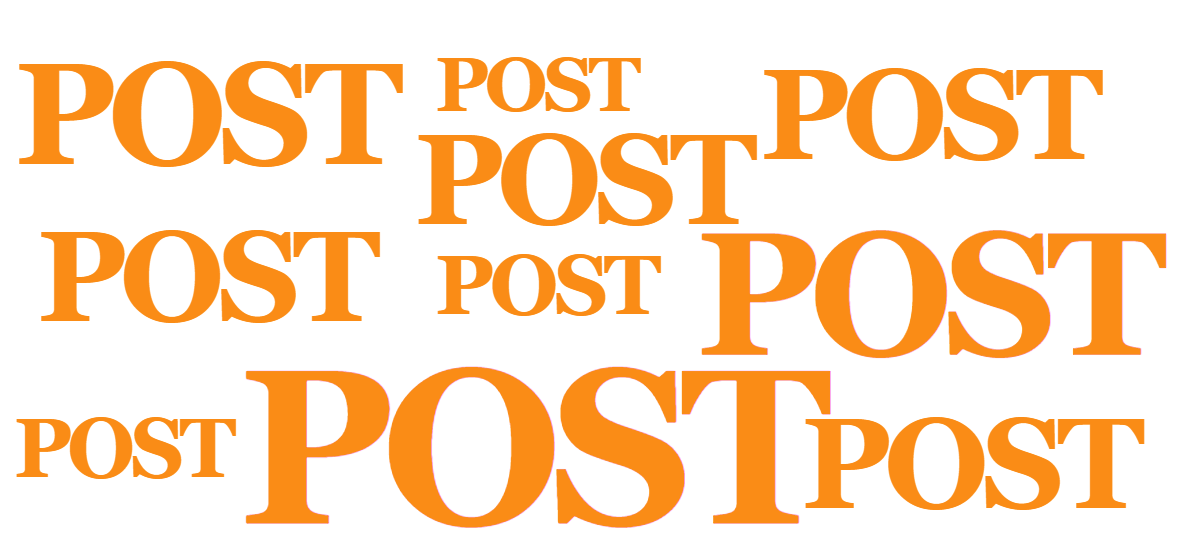
Handling API Responses
Now that we've made some requests, let's talk about handling the responses. It's important to check the status code to ensure the request was successful.
Checking Status Codes
Here's a simple example of how to check the status code:
import requests
url = 'https://jsonplaceholder.typicode.com/posts/1'
response = requests.get(url)
if response.status_code == 200:
print('Success!')
print(response.json())
else:
print('Error:', response.status_code)
In this example, we're checking if the status code is 200
, which indicates success. If the request was successful, we print the JSON response. Otherwise, we print an error message with the status code.
Parsing JSON Responses
Most APIs return data in JSON format. The response.json()
method makes it easy to parse the JSON data. Here's an example:
import requests
url = 'https://jsonplaceholder.typicode.com/posts/1'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print('Title:', data['title'])
print('Body:', data['body'])
else:
print('Error:', response.status_code)
In this example, we're parsing the JSON response and printing the title
and body
fields.
Error Handling with Requests
Error handling is crucial when working with APIs. You need to be prepared for various scenarios, such as network issues, invalid endpoints, and server errors.
Handling Network Errors
Network errors can occur when the server is unreachable or the connection is lost. The requests
library provides built-in support for handling these errors using exceptions.
Here's an example:
import requests
url = 'https://jsonplaceholder.typicode.com/posts/1'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as errh:
print("Http Error:", errh)
except requests.exceptions.ConnectionError as errc:
print("Error Connecting:", errc)
except requests.exceptions.Timeout as errt:
print("Timeout Error:", errt)
except requests.exceptions.RequestException as err:
print("OOps: Something Else", err)
In this example, we're using a try-except block to catch different types of errors. The raise_for_status()
method raises an HTTPError if the status code is not 200.
Handling Server Errors
Server errors (status codes 500-599) indicate a problem on the server side. Here's an example of how to handle server errors:
import requests
url = 'https://jsonplaceholder.typicode.com/invalid-endpoint'
response = requests.get(url)
if response.status_code >= 500:
print('Server Error:', response.status_code)
elif response.status_code == 404:
print('Not Found:', response.status_code)
elif response.status_code == 400:
print('Bad Request:', response.status_code)
else:
print('Other Error:', response.status_code)
In this example, we're checking if the status code is in the 500 range, which indicates a server error. We're also handling 404 (Not Found) and 400 (Bad Request) errors.
Advanced Tips and Tricks
Now that we've covered the basics, let's explore some advanced tips and tricks to make your life easier when working with APIs in Python.
Using Query Parameters
Query parameters allow you to pass data to the API as part of the URL. Here's an example:
import requests
url = 'https://jsonplaceholder.typicode.com/posts'
params = {'userId': 1}
response = requests.get(url, params=params)
print(response.status_code)
print(response.json())
In this example, we're passing a userId
parameter to the /posts
endpoint. The params
parameter of the requests.get
function takes a dictionary of query parameters.
Using Headers
Headers are used to send additional information with the request. Here's an example:
import requests
url = 'https://jsonplaceholder.typicode.com/posts'
headers = {'Content-Type': 'application/json'}
response = requests.get(url, headers=headers)
print(response.status_code)
print(response.json())
In this example, we're setting the Content-Type
header to application/json
. The headers
parameter of the requests.get
function takes a dictionary of headers.
Authentication
Many APIs require authentication. The requests
library supports various authentication methods, including Basic Auth and OAuth. Here's an example of how to use Basic Auth:
import requests
from requests.auth import HTTPBasicAuth
url = 'https://api.example.com/user'
auth = HTTPBasicAuth('username', 'password')
response = requests.get(url, auth=auth)
print(response.status_code)
print(response.json())
In this example, we're using Basic Auth to authenticate with the API. The auth
parameter of the requests.get
function takes an instance of HTTPBasicAuth
.
How to Test Your Python POST Request
Apidog is a powerful tool for testing APIs. It allows you to create and save API requests, organize them into collections, and share them with your team.
Here is how you can use Apidog to test your POST request:
- Open Apidog and create a new request.
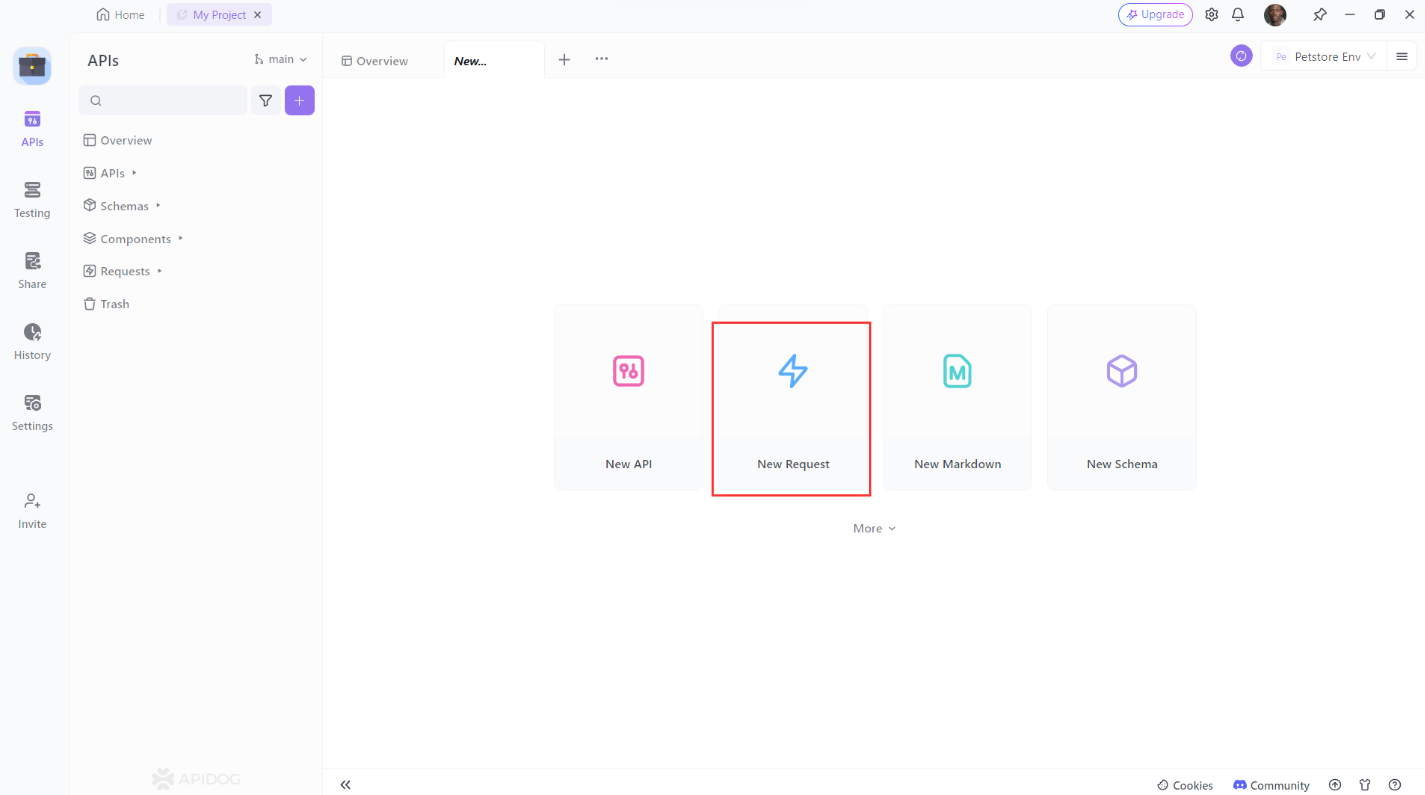
2. Set the request method to POST.
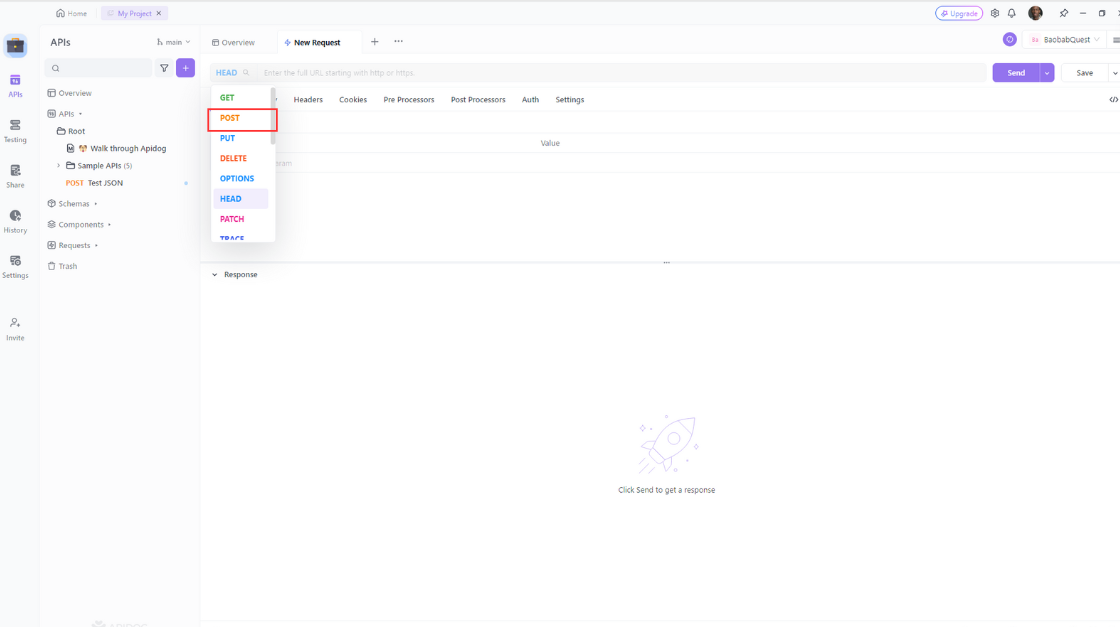
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
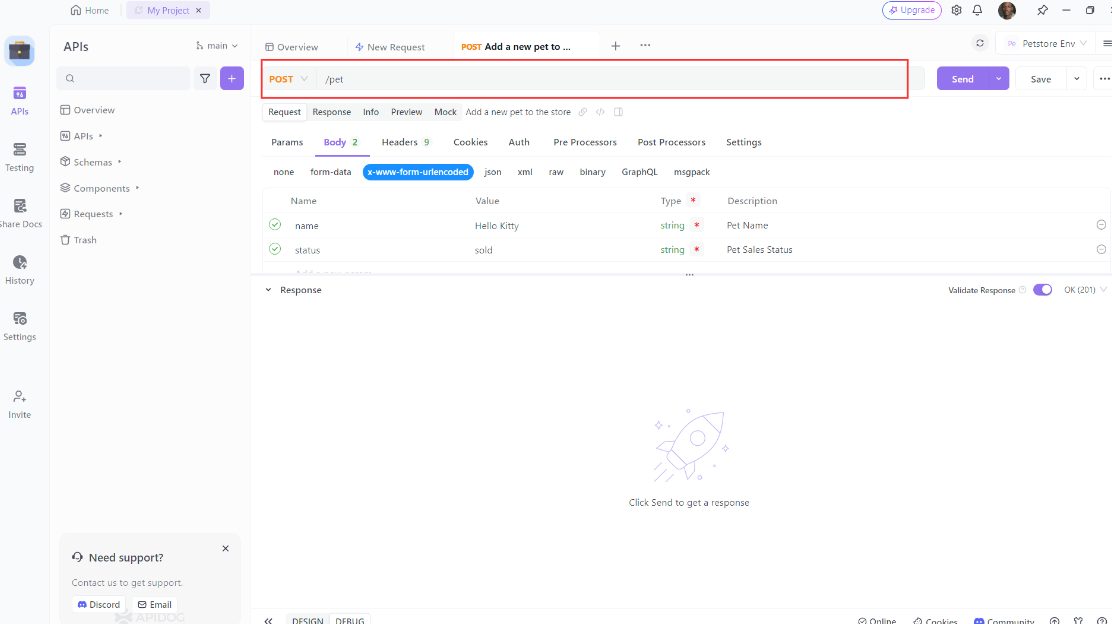
4. Verify that the response is what you expected.
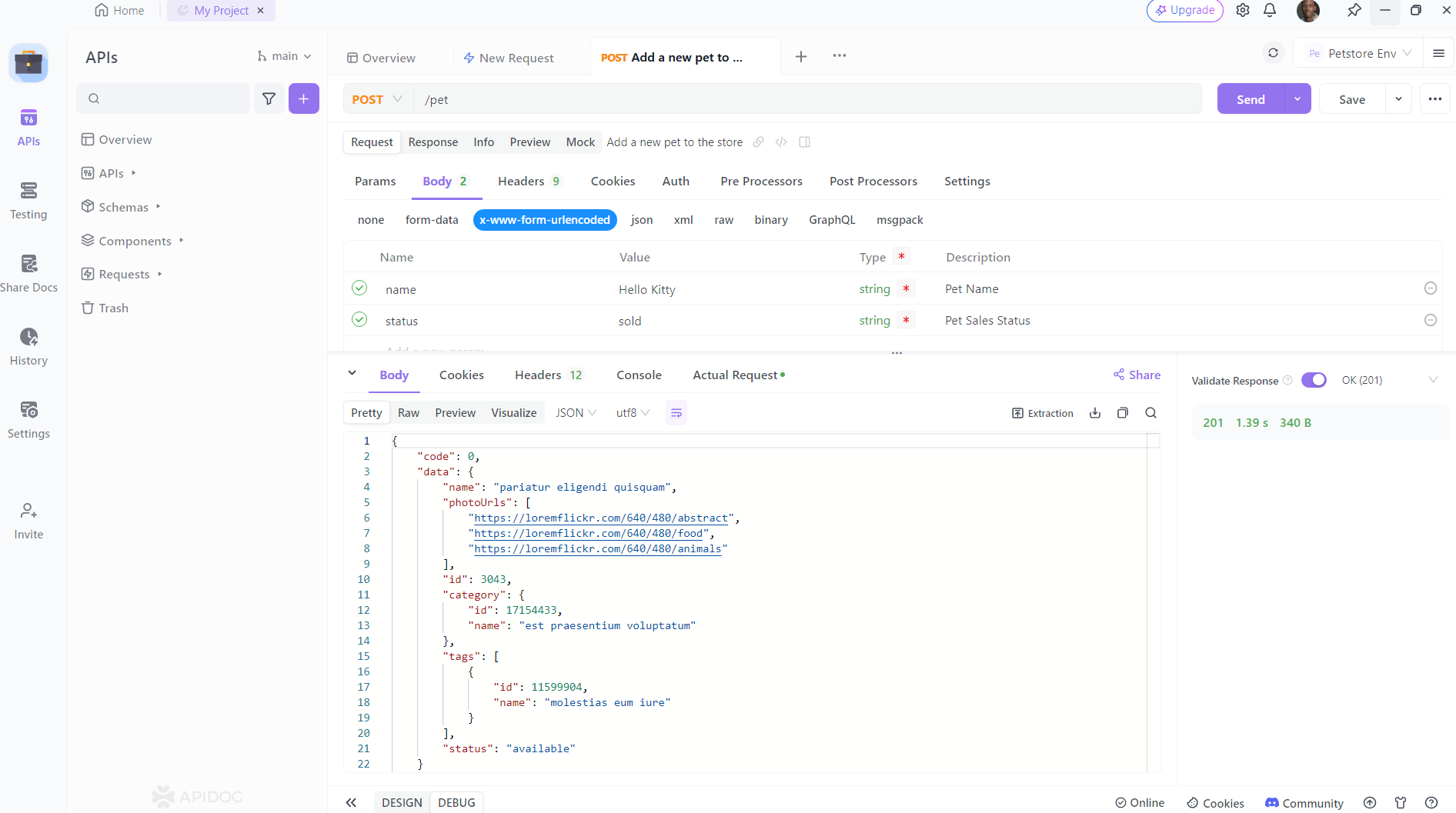
Conclusion
In this guide, we've covered the essentials of handling API requests and responses in Python. We've looked at making GET and POST requests, handling responses, and dealing with errors. We've also explored some advanced tips and tricks to make your life easier. Remember, APIs are a powerful tool that can open up a world of possibilities for your projects. So go ahead, experiment, and start building amazing things!
And don't forget to download Apidog for free to streamline your API development and testing. Happy coding!