Guide: What are JavaScript Query Parameters and How to Implement Them?
JavaScript query parameters, also known as URL parameters or query string parameters, are data packets attached to URLs after a question mark (?). They offer a convenient way to pass information between web pages and the server.
Niches in specific client languages are created to increase efficiency. With the unceasing use of query parameters in a great number of web addresses today, it's no surprise that the JavaScript language has its very own version of JavaScript query parameters.
Come utilize Apidog's straightforward and intuitive user interface to learn more about JavaScript query parameters by clicking the button below! 👇 👇 👇
After reading this article, you should be able to create industry-level JavaScript query parameters, as well as identify the best practices for creating JavaScript query parameters. Additionally, this article will specify the best scenarios to implement JavaScript query parameters.
What are JavaScript Query Parameters
JavaScript query parameters are a subset of general query parameters - they are niche JavaScript tools that are responsible for passing information between web pages and the server found after the question marks ( ?
) of web addresses (or URLs).
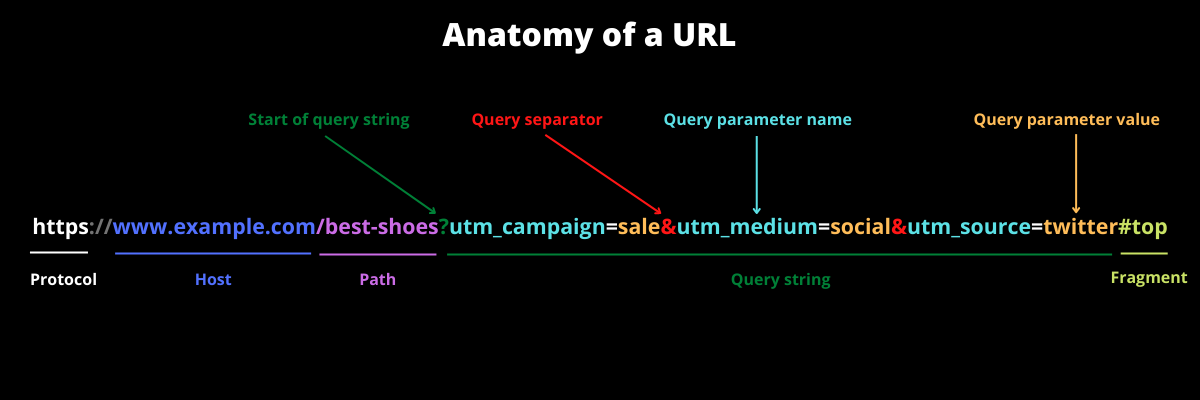
Basic Components of a JavaScript Query Parameter
Every JavaScript query string will have the following components:
- A base URL: A base URL is the core URL that uniquely identifies a web page or resource.
Famous examples of base URLs that you may have seen or used before arewww.facebook.com
andwww.google.com
! - A question mark (
?
): Question marks act as a separator for query parameters. It makes it easier to differentiate what is part of the query string. - Key-value pair(s): If the web address you are on has a query string, you will definitely see at least one key-value pair.
A key-value pair always consists of two components, a key and a value.
Keys are a unique identifier for data that are being passed, you will notice that most keys are descriptive and that they follow consistent naming conventions.
Values are the actual data contained. They may come in different forms, such as text, numbers, and encoded structures like JSON. - Ampersand(s) (
&
): The ampersand separates multiple key-value pairs found on the query string.
Suitable Scenarios For Implementing JavaScript Query Parameters
- Passing small amounts of data: JavaScript query parameters are well-suited for sending a few key-value pairs between pages. They are lightweight and efficient for simple data exchange.
- Temporary data: If the data you need to pass only needs to be used for the current session or doesn't require permanent storage on the server, using JavasScript query parameters is a good option. For example, sorting preferences or temporary user selections can be effectively managed using them.
- Sharing data between applications: JavaScript query parameters can be used to exchange data between different web applications running on the same domain. This can be helpful for scenarios like single sign-on or passing user information between applications.
- Bookmarking page states: You can leverage JavaScript query parameters to encode the current state of a web page (such as filters and sorting) and store it as a bookmark for later access. This allows users to return to the same view they left off.
Scenarios to Avoid Implementing JavaScript Query Parameters
- Sensitive data: Since JavaScript query parameters are visible in the URL, they should never be used to transmit sensitive information like passwords, credit card details, or any other data that could be compromised if exposed.
- Large amounts of data: Sending large datasets through JavaScript query parameters can become cumbersome and inefficient. The URL length has limitations, and large strings might get truncated. For such cases, you should consider alternative methods like POST requests with JSON payloads, which can handle larger data volumes more effectively.
Good and Bad Practices to Know When Implementing JavaScript Query Parameters
There are welcomed practices and avoided characteristics for JavaScript query parameters.
Good JavaScript Query Parameters Features
- Simple and easy to use: JavaScript query parameters are relatively easy to understand and implement, making them a good choice for basic data exchange.
- Lightweight: JavaScript query parameters are suitable for sending small amounts of data, as they don't add significant overhead to the URL length.
- Temporary data: JavaScript query parameters are well-suited for transmitting temporary data that doesn't need to be persisted on the server, like sorting preferences or filter selections.
- Sharing data between applications: JavaScript query parameters can be used to exchange data between different web applications on the same domain, facilitating functionalities like single sign-on.
- Bookmarking: You can use JavaScript query parameters to encode the current state of a page (e.g., filters, sorting) into a bookmark, allowing users to easily return to that specific view later.
Bad JavaScript Query Parameters Features to Avoid
- Limited length: Browsers have limitations on the total length of a URL, including the query string. Excessively long JavaScript query parameters can get truncated, causing data loss.
- Security concerns: Since JavaScript query parameters are visible in the URL, they should never be used to transmit sensitive information like passwords or credit card details. This information can be easily intercepted if not properly secured.
- Readability and maintainability: Long and complex JavaScript query parameters can make URLs cluttered and harder to understand, especially for new developers who may not be familiar with the code. This can also hinder maintainability in the long run.
- Limited data types: While they can handle various data types, complex data structures might become cumbersome to encode and decode within a query string.
Summary of Comparison Between Good and Bad JavaScript Query String Features
Feature | Good Query String | Bad Query String |
---|---|---|
Conciseness | Short and focused, containing only essential data pairs. | Excessively long, containing unnecessary information.(?sort=name&limit=10 ) |
Clarity | Uses descriptive and well-defined key names. | Uses cryptic or unclear key names. |
Structure | Follows a consistent structure with proper encoding. | Lacks a clear structure or uses improper encoding. |
Example (Search) | ?https://www.example.com/search?q=javascript |
?https://www.example.com/search?query=javascript+tutorial&sort=relevance |
Common Methods to Work With Query Parameters:
URLSearchParams
object: This is the modern and recommended way to parse and manipulate query parameters. It provides methods such as get()
to retrieve the value of a specific key, getAll()
to get an array of all values for a key (if there are duplicates), and methods to iterate over all parameters.
Here is a JavaScript code sample showing how to parse the string, access specific key-value pairs, and modify the query string.
const urlParams = new URLSearchParams(window.location.search);
// Accessing a specific value:
const value1 = urlParams.get('key1');
console.log(value1); // Output: value1
// Checking for existence of a key:
const hasKey2 = urlParams.has('key2');
console.log(hasKey2); // Output: true
// Looping through all key-value pairs:
for (const [key, value] of urlParams) {
console.log(key, value);
}
Regular expressions: While less common nowadays, you can also use regular expressions to extract specific query parameters from the window.location.search
string.
Although it is not recommended, here is a code sample to show what it looks like:
const queryString = window.location.search;
console.log(queryString); // Output: ?key1=value1&key2=value2
Apidog - Clear and Concise API Tool
Apidog is an all-in-one API development tool for developers who wish to visually develop APIs. With Apidog, users can build, mock, test, and document APIs all within just one application.
Importing Files Onto Apidog
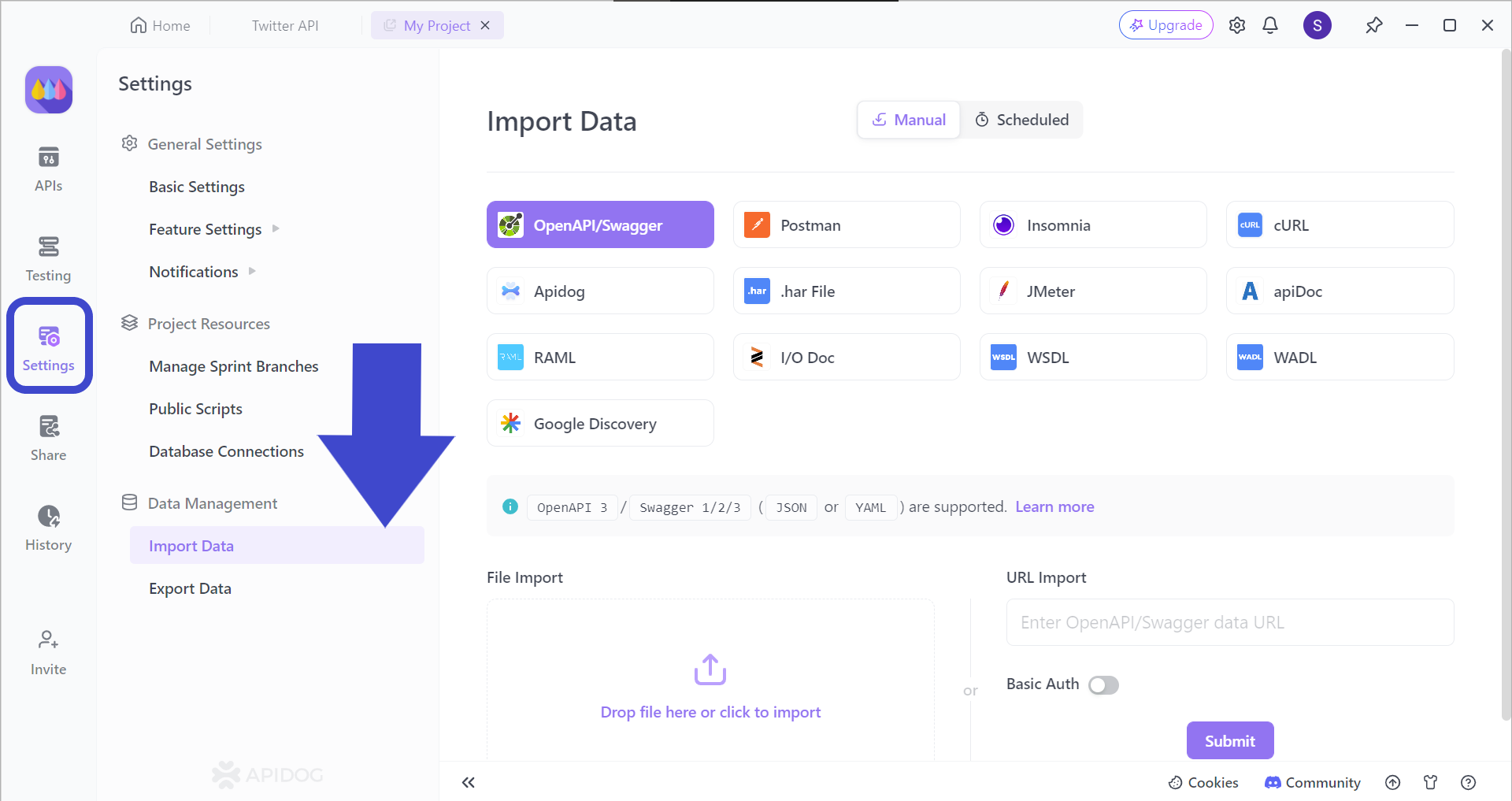
As the caption of the image above mentions, Apidog supports the import of various types of API files. This also includes Postman, Insomnia, and Swagger API files! Let's take a look at how we can import such files.
Start by locating the Settings
button found on the left vertical toolbar. You can then locate the Import Data
section under the Data Management
, and drag the API file you wish to import onto Apidog.
Observing Responses After Sending Requests With Apidog
With Apidog, you can easily analyze API responses after sending a request.
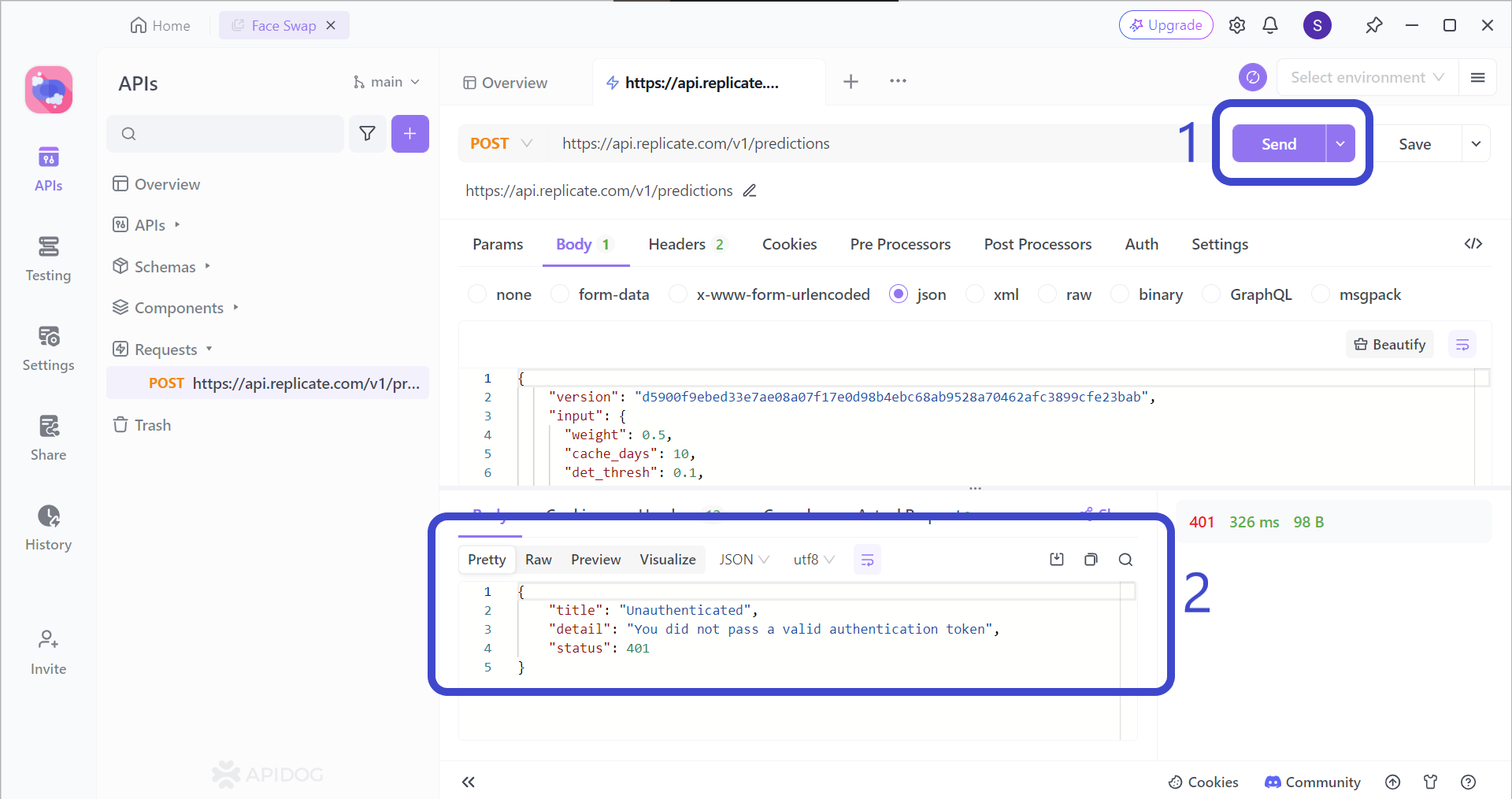
To receive a response, you first have to send a request. Press the Send
button. You can then head to the lower section of the screen to observe the response you received.
Conclusion
JavaScript query parameters are not so different from ordinary query parameters, however, you will need to understand how to acquire access to JavaScript query parameters, you may use the URLSearchParams
method to work with JavaScript query parameters.
Apidog is an all-in-one API tool that allows you to flexibly work with APIs, regardless of whether you want to continue an existing project or develop a brand new API from scratch. With Apidog, you can quickly learn and adapt using the application with the assistance of an easy-to-learn user interface and functions.