Guide: What is JavaScript HTTP POST and How to Implement Them?
JavaScript HTTP POST methods are mainly utilized for creating new resources on the server. On a few occasions, they can be used for updating existing resources. With various advantages that they may offer, learn how to implement JavaScript HTTP POST methods here!
Four common HTTP methods are used in JavaScript: GET, PUT, POST, and DELETE. Although they may seem simple to understand in English, in the context of API development, each has a different meaning and function.
For those seeking a modern and efficient API platform, Apidog may be a solution to consider. To begin exploring its capabilities, simply click the button below! 👇👇👇
What is a JavaScript HTTP POST?
In the context of JavaScript, an HTTP POST request is a method for sending data to a server mainly for creating a resource. In some scenarios, the JavaScript HTTP POST method can be used for updating an existing resource on the server.
JavaScript HTTP POST requests are mainly used to send data to a server, not to retrieve data, so you can easily remember JavaScript HTTP POST methods by the data flow, starting from the client and ending at the server.
Key Characteristics (and Components) of a JavaScript HTTP POST Method
- Method: The JavaScript HTTP POST method is used to submit data to be processed to a specified resource. It is one of the standard HTTP methods alongside GET, PUT, DELETE, etc.
- Data payload: POST requests are relatively more secure compared to other methods as the data that is sent are packaged inside the request's body. With JavaScript HHTP POST methods, you are capable of sending larger amounts of data, regardless if it is sensitive information or not.
- Headers: POST requests will most likely include headers that provide additional information about the request, such as the content type (
Content-Type
), specifying the format of the data being sent (whether it would be in JSON, form data, or any other type). - Endpoint: JavaScript HTTP POST requests will include the URL of the server resource or API endpoint to which the POST request is sent
The URL may be in a relative or absolute URL - Asynchronous: The main characteristic that allows JavaScript HTTP POST methods to shine. JavaScript POST requests are often made asynchronously to prevent blocking the other scripts from executing or webpages from rendering.
- Response handling: If a request is made, a response usually follows. JavaScript code can usually handle the response by parsing the response data (typically made with JSON or XML).
- Error handling: In the case a JavaScript HTTP POST method's request returns a failed response, proper error handling swoops into the rescue. There are common errors that a response may display, such as network and server-side errors.
Error handling will usually involve catching expectations or checking response status codes. - Content encoding: JavaScript HTTP POST methods may include encoded data before it is sent in the request body for better data security.
Why Use JavaScript HTTP POST?
With so many other technologies available, such as Fetch API, AJAX (Asynchronous JavaScript and XML), and XMLHttpRequest (XHR), JavaScript HTTP POST has its undeniable strengths and compatibilities with them, such as:
- Asynchronous Communication: JavaScript permits asynchronous communication - while the POST request is being processed by the server, the rest of the webpage can continue to function. This is crucial for web applications to remain responsive and provide a smooth user experience.
- Dynamic Content: JavaScript enables dynamic content generation on web pages. Using POST requests, you can send user input or other data to the server and receive a response that can be dynamically updated on the webpage without requiring a full page reload.
- Integration with APIs: Many modern web applications rely on APIs (Application Programming Interfaces) to interact with server-side resources. JavaScript HTTP POST requests are commonly used to interact with these APIs, allowing developers to fetch data, send updates, or perform other actions without refreshing the entire page.
- Form Submission: When submitting forms on a webpage, POST requests are often used to send the form data to the server for processing. This allows for the secure transmission of sensitive information such as passwords or payment details, as the data is sent in the request body rather than appended to the URL (as in GET requests).
- Data Manipulation: JavaScript POST requests allow more complex data manipulation and transmission than simpler methods like GET requests. With POST requests, you can send structured data in various formats (JSON, XML, etc.), making it suitable for more sophisticated applications.
JavaScript HTTP POST Method Code Example (Fetch API included!)
// Define the data you want to send in the request body
const postData = {
username: 'example',
password: 'password123'
};
// Convert the data to JSON format
const jsonData = JSON.stringify(postData);
// Define the URL of the server endpoint
const url = 'https://example.com/api/login';
// Configure the fetch options
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: jsonData
};
// Make the POST request using the fetch API
fetch(url, options)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON response
})
.then(data => {
console.log('Response:', data);
})
.catch(error => {
console.error('There was a problem with your fetch operation:', error);
});
Code Explanation (In Order):
- Defined the data we want to send in the request body as a JavaScript object (
postData
). - Converted the data to JSON format using
JSON.stringify()
and store it in thejsonData
variable. - Defined the URL of the server endpoint (
url
). - Configured the fetch options object (
options
) with the method set to'POST'
, headers indicating JSON content type, and the JSON data in the request body. - Used the
fetch
function to make the POST request with the specified URL and options. - Handled the response asynchronously using Promises, parsing the JSON response and logging it to the console.
- Handled errors using the
catch
method to log any encountered errors.
Common Use Cases for JavaScript HTTP POST Requests
In web development, there are occasions where sensitive data needs to be sent. Without a safe method of relaying data to the opposite party, such a simple process would take a much longer time, and require more resources and planning. Therefore, here are some real-world scenarios that are enabled with JavaScript HTTP POST requests, thus making them implemented in a lot of web applications today!
- Form submission: When a user fills out a form on a webpage (e.g., a login form, registration form, contact form), the form data is typically sent to the server using a POST request. This allows the server to process the user input and perform actions such as authentication, database updates, or sending emails.
- User authentication: In many web applications, user authentication involves sending credentials (e.g., username and password) to the server for verification. This is often done using a POST request to a login endpoint, where the server checks the credentials and returns a response indicating whether the user is authenticated.
- Data submission and update: JavaScript HTTP POST requests are commonly used to submit data to a server for storage or updating. For example, in a content management system (CMS), users might create or edit articles, blog posts, or other content, with the changes being sent to the server via POST requests.
- API interaction: Modern web applications often interact with APIs (Application Programming Interfaces) to fetch data from external sources or perform actions on remote servers. JavaScrip HTTP POST requests are used to send data to these APIs, such as creating new records, updating existing data, or triggering actions.
- AJAX Requests: JavaScript HTTP POST requests are frequently used in AJAX (Asynchronous JavaScript and XML) applications to fetch data from the server in the background without requiring a full page reload. This allows for dynamic updates to the webpage content based on user actions or other events.
- User-generated Content: Websites or web applications that allow users to submit content (e.g., comments, reviews, messages) often use JavaScript POST requests to send the user-generated content to the server for storage and display.
- Payment Processing: When processing payments in an e-commerce application, JavaScript HTTP POST requests are often used to send payment details securely to a payment gateway or processing service for authorization and payment confirmation.
Apidog - Fitting API Platform for Implementing JavaScript HTTP POST Requests
Apidog is an all-in-one API development platform that allows users to implement JavaScript HTTP POST requests. After building the JavaScript HTTP POST request, you can also test, mock, debug, and document it all on Apidog. You will not need another API tool to fulfill API lifecycle processes anymore!
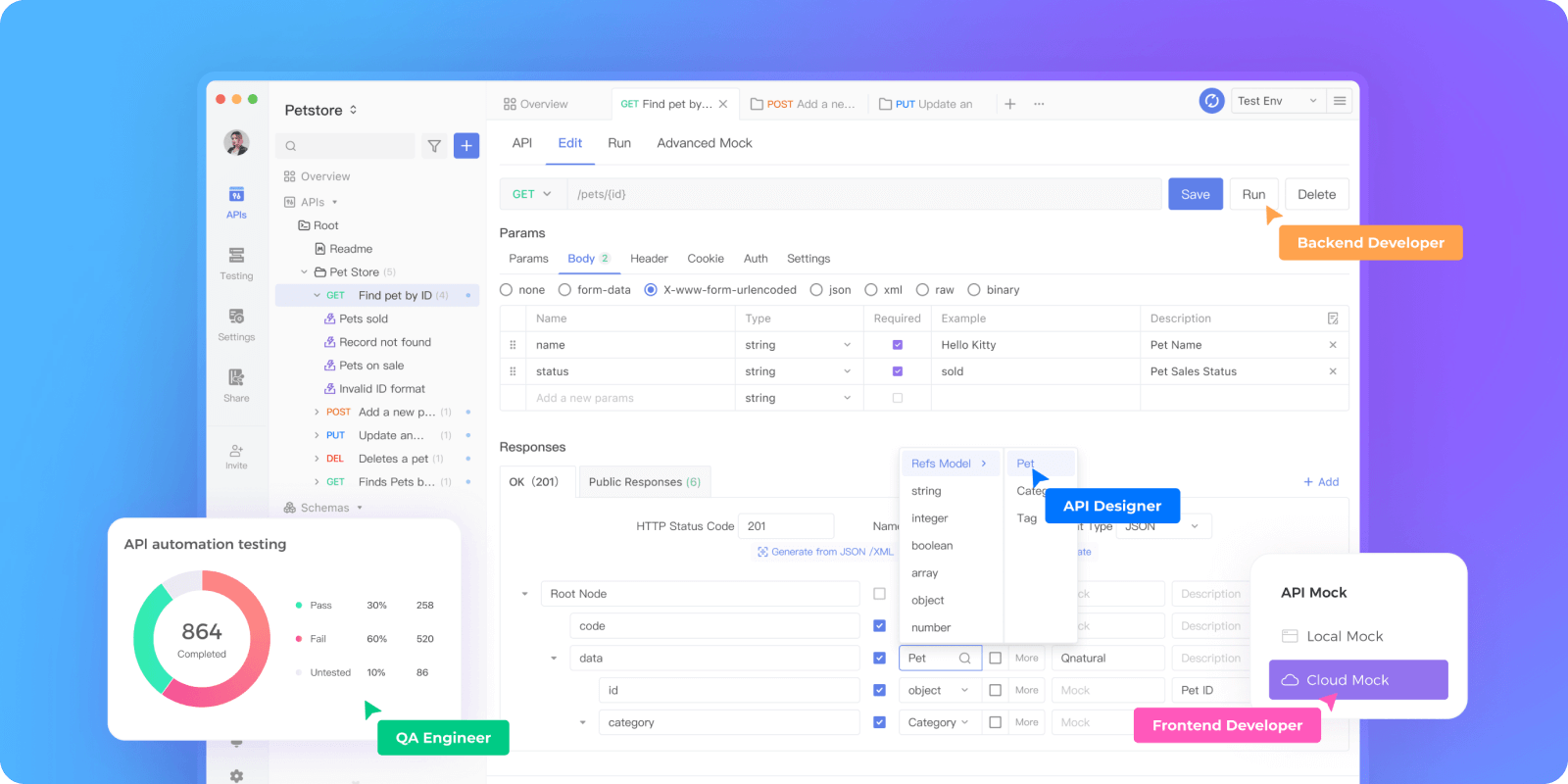
Building a New JavaScript HTTP POST Request with Apidog
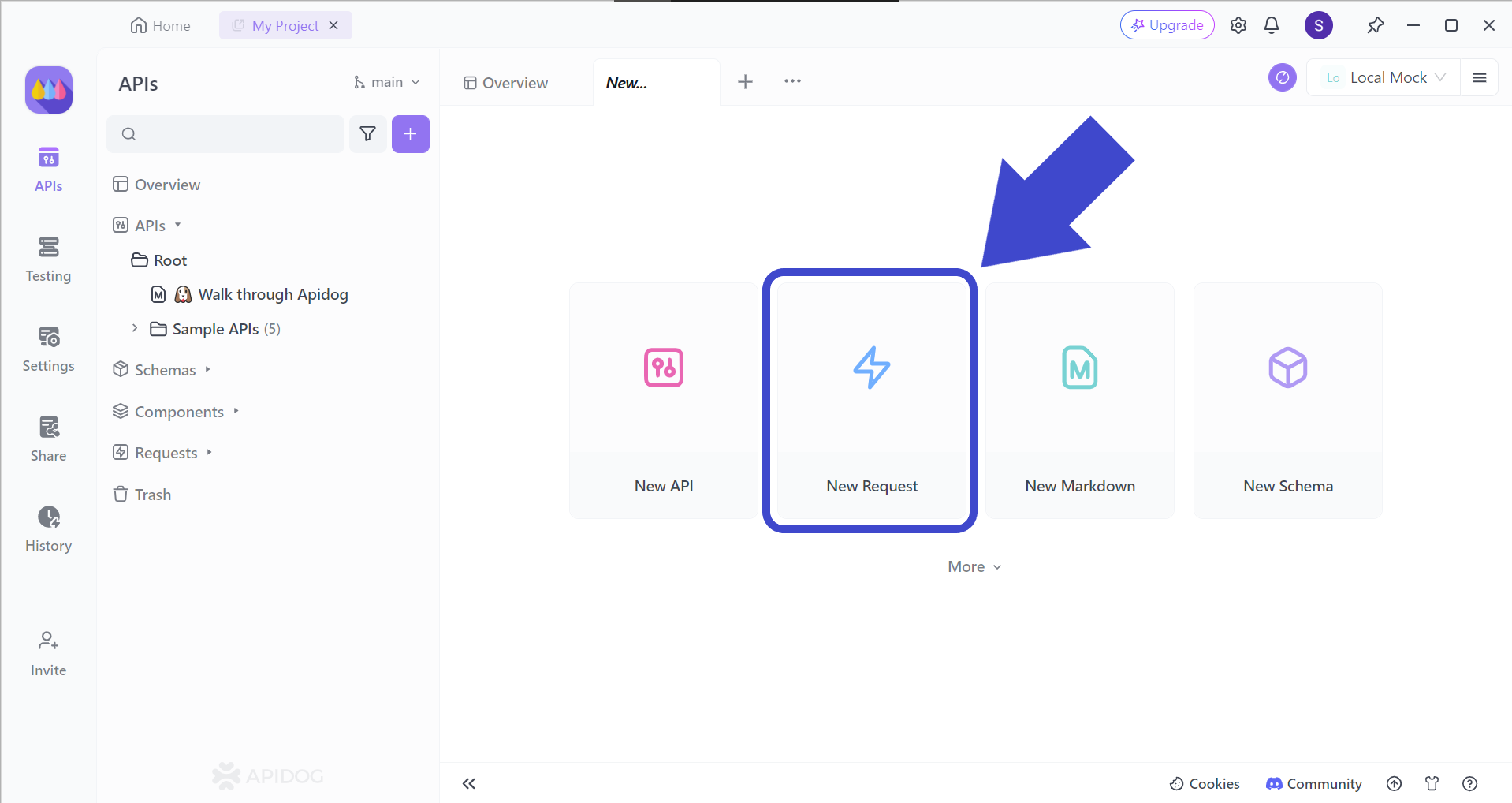
Begin by pressing the New Request
button as pointed out by the arrow in the picture above.
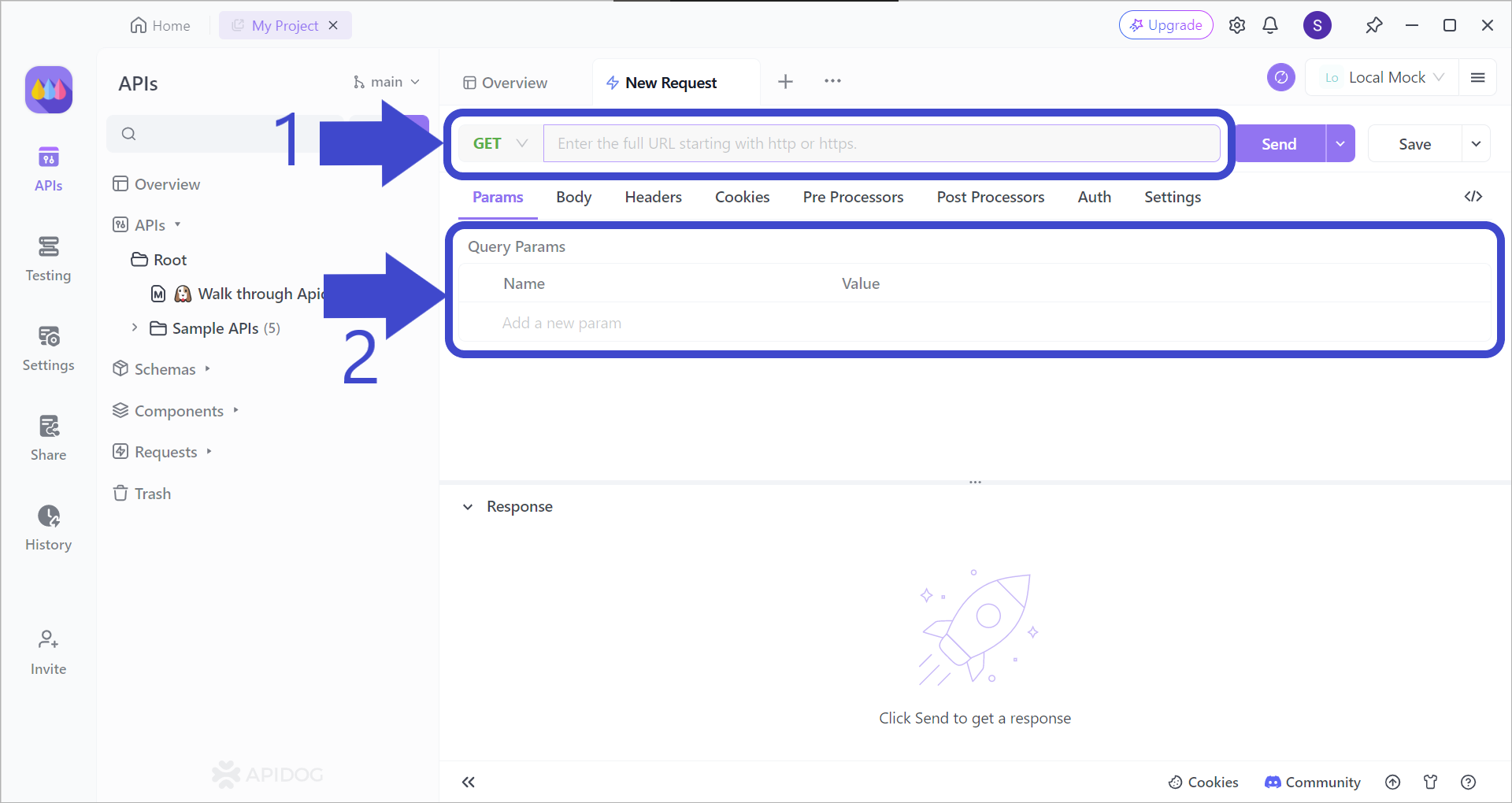
To create a JavaScript HTTP POST request, make sure to select the POST
method, and create a relevant URL. If you plan to pass multiple parameters into the POST request URL, make sure to include them in the section underneath.
Observing the Response Obtained from JavaScript HTTP POST Method Using Apidog
You can utilize Apidog's simple and intuitive user interface to analyze the response returned after the request has been sent.
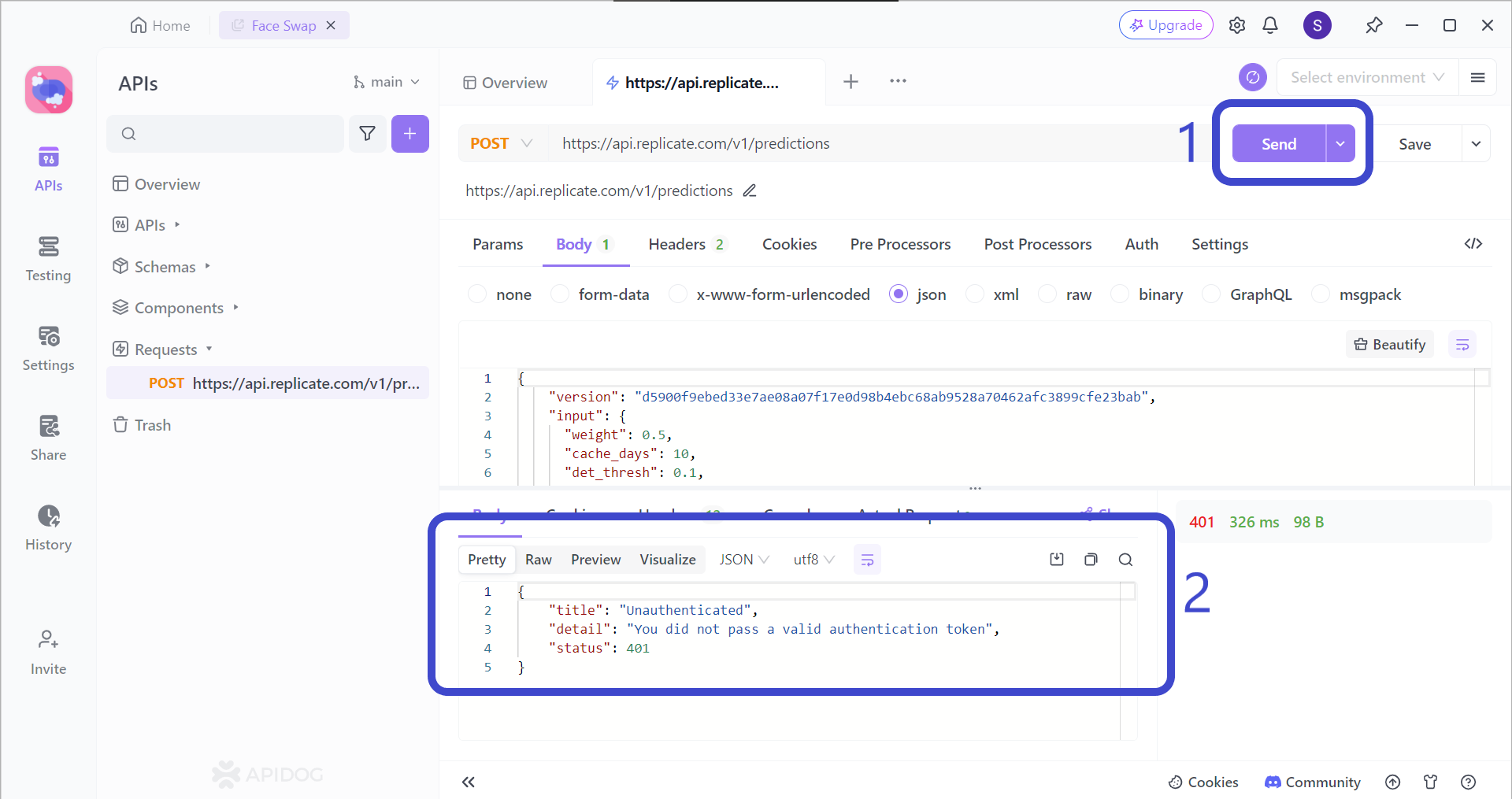
Make the JavaScript HTTP POST method request by pressing the Send
button found in the right corner of the Apidog window. Then, you should be able to view the response on the bottom portion of the screen.
Setting API Responses for Your API Using Apidog
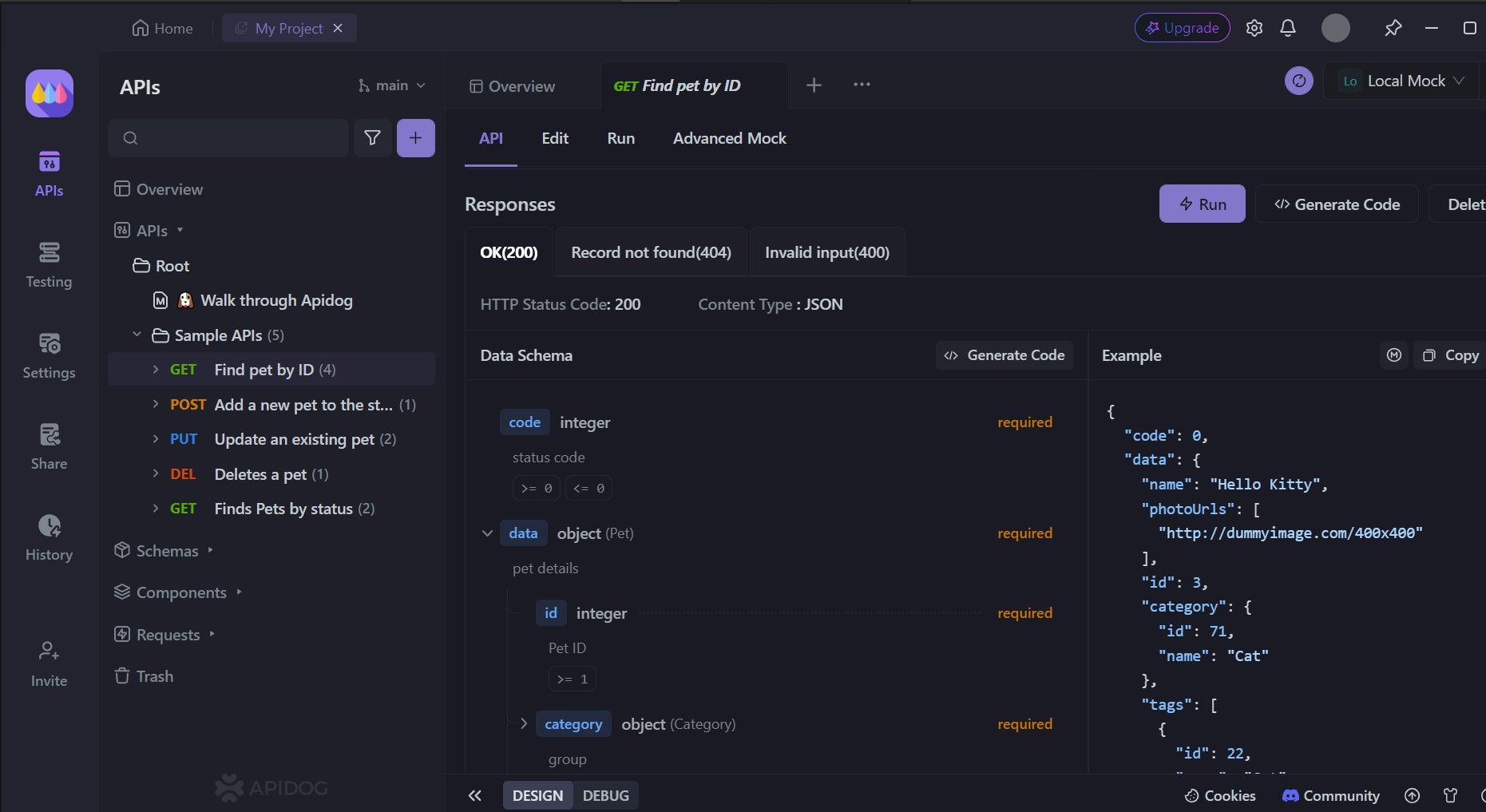
If you wish to create your API, you can also initialize your very own status codes and responses. You also can generate relevant code based on the data schema that you have created on Apidog!
Conclusion
JavaScript HTTP POST methods are a more secure way to send sensitive data from the client to the server. With the ability to support asynchronous communication, JavaScript HTTP POST methods are becoming a web developer's choice for creating resources on servers. With JavaScript HTTP POST methods, many application functionalities are turned into reality, such as:
- File uploading
- API interactions
- User authentication
As mentioned before, Apidog can be a suitable choice for developers who interact and wish to create JavaScript HTTP POST methods. Aside from creating your own APIs, you can also learn and implement other developers' APIs by using Apidog's API Hub feature - API Hub is where you get to see and connect to thousands of other APIs!