Guide: What is JavaScript HTTP DELETE and How to Implement Them?
The JavaScript HTTP DELETE method is essential for removing server resources. It offers versatility in user account management, content deletion, and data cleanup, enhancing web application functionality and adhering to RESTful API principles
The JavaScript HTTP DELETE method is one of four famous HTTP methods that many web developers use today: GET, PUT, POST, and DELETE. With each HTTP responsible for different operations, they have their uses in various web applications.
What distinguishes Apidog is its user-friendly visual interface, facilitating swift adaptation for users. For those seeking a potent API solution, Apidog emerges as a highly attractive choice. Click the button below to dive right into Apidog! 👇 👇 👇
What is JavaScript HTTP DELETE?
To put it simply, JavaScript HTTP DELETE refers to a method used for making requests to servers solely for deleting a resource.
JavaScript HTTP DELETE methods are commonly used together with APIs to remove data or resources permanently from a server.
Key Characteristics (and Components) of a JavaScript HTTP DELETE Method
- HTTP Method: The DELETE method is part of the Hypertext Transfer Protocol (HTTP) and is used to request the deletion of a resource identified by the provided URL.
- URL: The URL (Uniform Resource Locator) specifies the location of the resource to be deleted on the server. This is typically provided as an argument to the DELETE request.
- Request Headers: Optionally, you may include headers in the DELETE request to provide additional information or customization, such as authentication tokens or content type.
- Payload: DELETE requests usually do not have a request body or payload. Unlike POST or PUT requests, which often include data to be added or updated, DELETE requests simply identify the resource to be removed through the URL.
- Response: Upon receiving a DELETE request, the server responds with an HTTP status code to indicate the outcome of the operation. A successful deletion typically returns a status code of 200 (OK) or 204 (No Content), while errors might result in status codes like 404 (Not Found) or 403 (Forbidden).
- Asynchronous Nature: In client-side JavaScript, HTTP requests are typically made asynchronously to prevent blocking the main thread of execution. This means that you'll often use callbacks, promises, or async/await syntax to handle the response from the server.
- Irreversible: Once the DELETE method is made, there is no other way to reverse the deletion or retrieve the erased resource. It's important to ensure that only authorized users can initiate DELETE requests and that sensitive data is handled securely.
Why Use JavaScript HTTP DELETE Methods?
With JavaScript HTTP DELETE Methods, you can manage data on servers more efficiently. You can create more memory space for resources that matter to your application!
- Resource Deletion: The primary purpose of the DELETE method is to delete resources on the server. This could be anything from a user account to a specific file or record in a database.
- RESTful API Compliance: When developing web applications that adhere to the principles of Representational State Transfer (REST), using HTTP methods such as DELETE helps maintain consistency and clarity in the API design. In RESTful APIs, each HTTP method has a specific meaning, and DELETE is used to delete resources.
- Client-Server Communication: In client-server architectures, JavaScript DELETE requests allow the client (typically a web browser) to communicate with the server to perform actions like deleting user accounts, removing items from a shopping cart, or deleting posts in a social media application.
- Asynchronous Operations: JavaScript HTTP DELETE requests are typically made asynchronously, which means they don't block the main execution thread of the web page. This asynchronous nature allows for smooth user interactions without freezing the browser while waiting for a response from the server.
- Efficiency and Performance: Using HTTP DELETE methods can lead to more efficient and performant web applications. By removing unnecessary data or resources from the server, you can optimize storage space and improve overall system performance.
- API Integration: When working with external APIs, JavaScript DELETE methods enable integration with various services or platforms that provide APIs for managing resources. This can include deleting files from cloud storage, removing entries from a database, or revoking access tokens.
JavaScript HTTP DELETE Method Code Example (Fetch API Included)
// URL of the resource to be deleted
const url = 'https://api.example.com/resource/123';
// Configuration object for the fetch request
const options = {
method: 'DELETE',
headers: {
'Content-Type': 'application/json', // Specify the content type if necessary
// Additional headers can be included as needed, such as authentication tokens
},
};
// Send the DELETE request using fetch
fetch(url, options)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
console.log('Resource deleted successfully');
})
.catch(error => {
console.error('There was a problem with the DELETE request:', error.message);
});
Code Explanation (In order):
- Defined the URL of the resource to be deleted.
- Created an options object specifying the method as DELETE. You can include additional headers here if required.
- Used the
fetch
function to send the DELETE request to the specified URL with the provided options. - Handled the response using promises. If the response is successful (status code 2xx), we log a success message. Otherwise, we catch any errors that occur during the request.
Common Use Cases for JavaScript HTTP DELETE Methods
Deleting resources sounds more important than it seems. It is vital to be able to remove resources from a server as leftover sensitive data may pose a security issue for third parties.
- Deleting User Accounts: In web applications with user authentication systems, DELETE requests can be used to delete user accounts when requested by the user. This could involve removing user data from the database and revoking access tokens.
- Removing Items from a Shopping Cart: In e-commerce applications, DELETE requests can be used to remove items from a user's shopping cart. This allows users to manage their cart contents before proceeding to checkout.
- Deleting Posts or Comments: In social media platforms or content management systems, DELETE requests can be used to delete posts, comments, or other user-generated content. This helps users manage their contributions and maintain the integrity of the platform.
- Managing File Storage: When working with cloud storage services or file-sharing platforms, DELETE requests can be used to delete files or folders from the server. This allows users to clean up their storage space and remove unwanted files.
- Unsubscribing from Mailing Lists: In email marketing systems, DELETE requests can be used to unsubscribe users from mailing lists or newsletters. This ensures compliance with privacy regulations and gives users control over their email preferences.
- Revoking Access Tokens: In authentication and authorization systems, DELETE requests can be used to revoke access tokens issued to clients or users. This helps enhance security by invalidating tokens and preventing unauthorized access to resources.
- Canceling Reservations or Bookings: In booking or reservation systems, DELETE requests can be used to cancel reservations, bookings, or appointments. This allows users to manage their schedules and free up reserved slots for others.
- Removing Personal Data: In compliance with data privacy regulations such as the General Data Protection Regulation (GDPR), DELETE requests can be used to delete personal data stored on the server upon user request. This ensures that user data is handled responsibly and following legal requirements.
Apidog - Fool-Proof API Development Tool for Developers
Ensure that you do not make fatal mistakes when designing APIs by using Apidog!
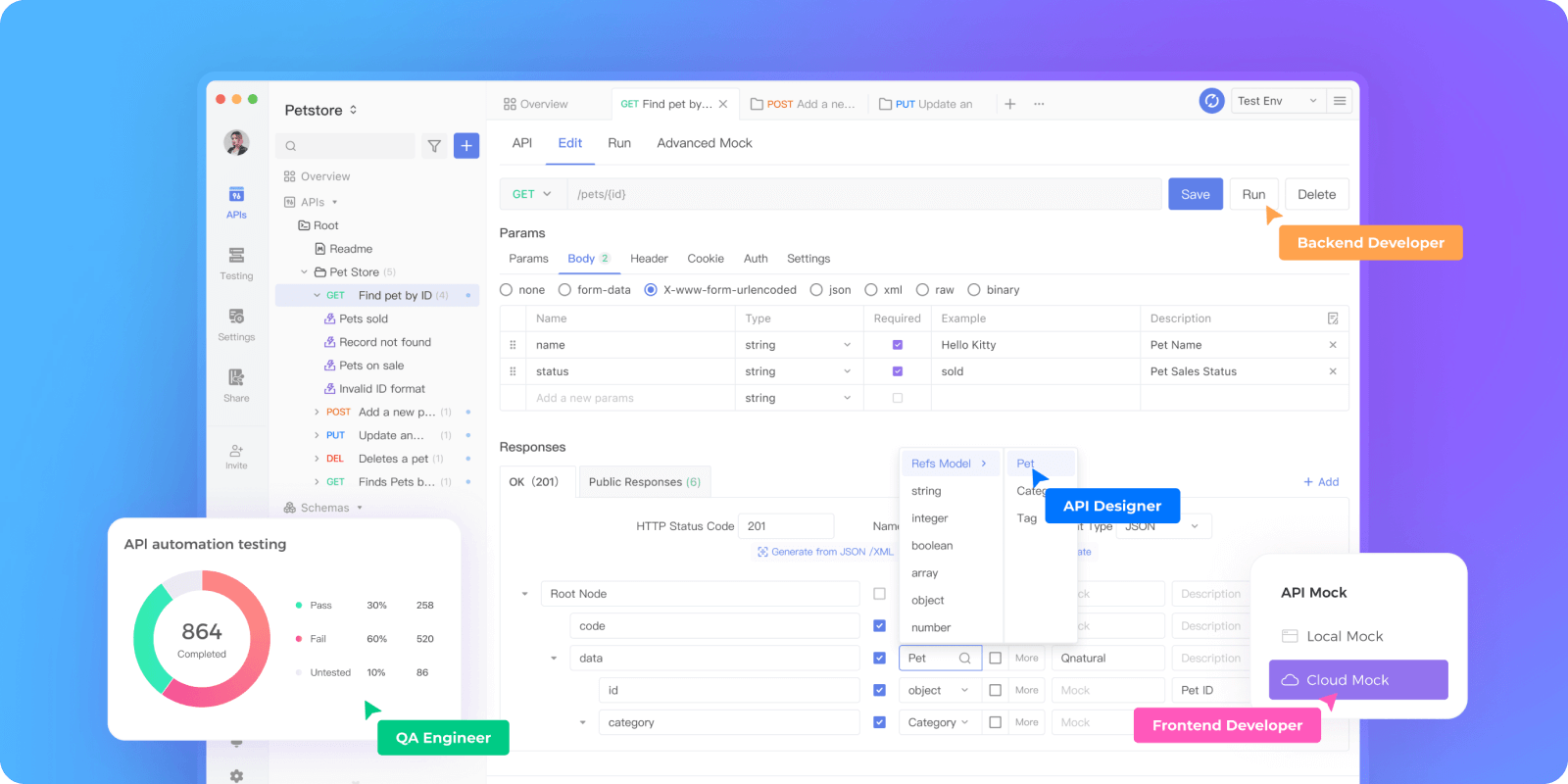
With a clear and simple user interface, Apidog users can vividly differentiate different functions. It is extremely easy to learn Apidog's functionalities and interface, so new Apidog users can quickly adapt to their new environment.
Creating a New JavaScript HTTP DELETE Request with Apidog
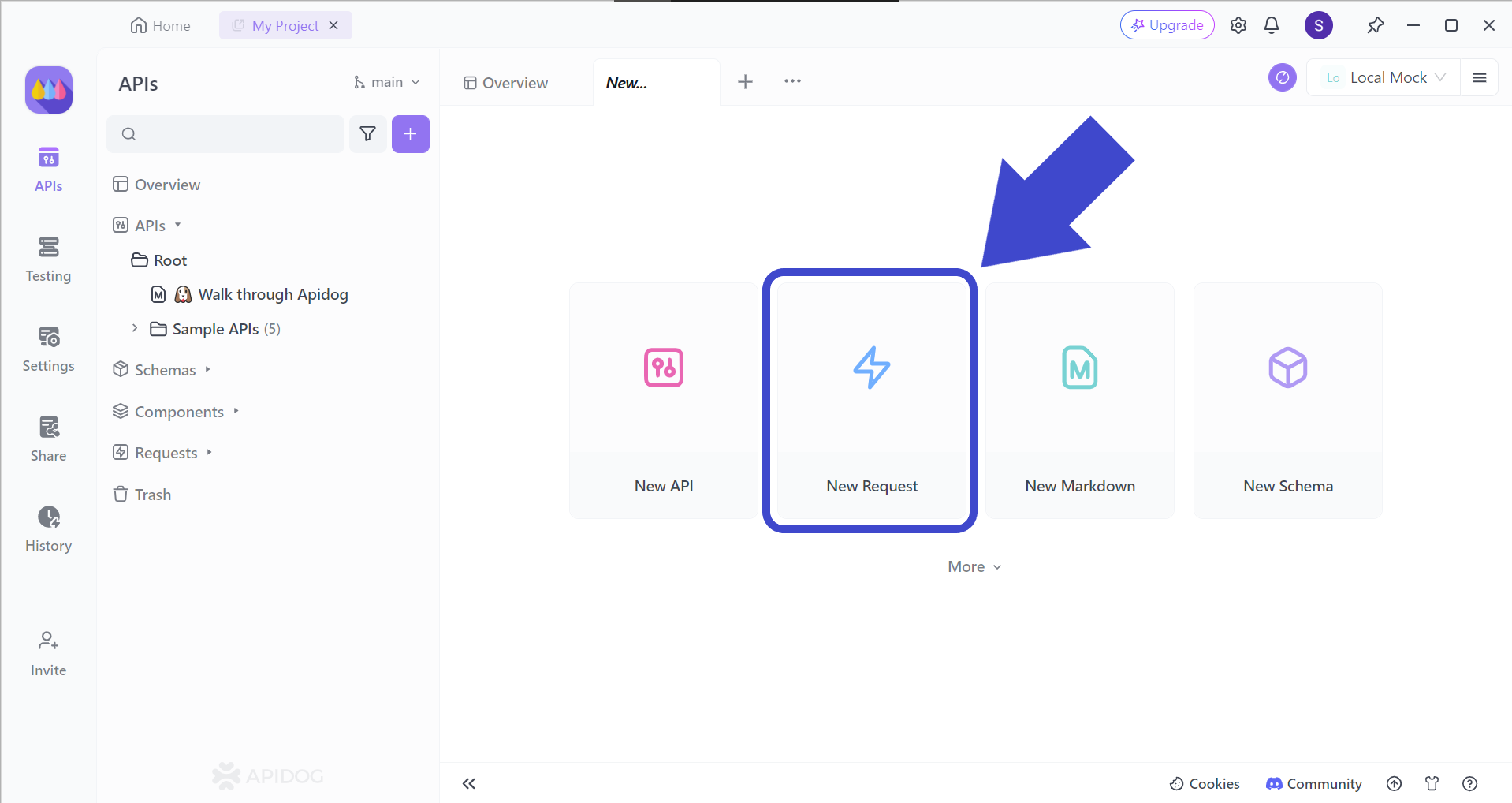
Begin by pressing the New Request
button as pointed out by the arrow in the picture above.
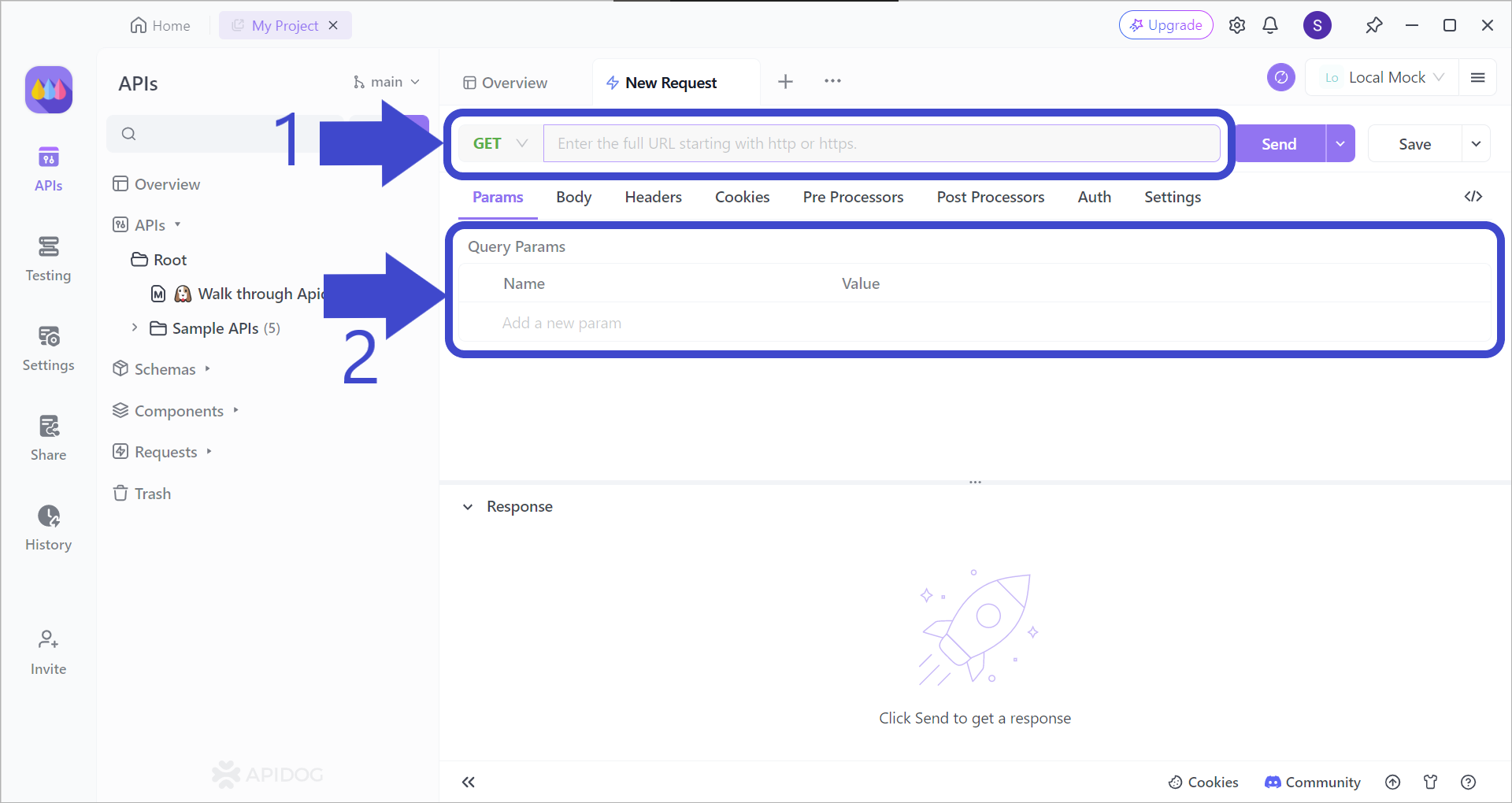
To create a JavaScript HTTP DELETE request, make sure to select the DELETE
method, and create a relevant URL. You may want to pass multiple parameters to correctly point at the specific resource you want to delete.
Observing the JavaScript HTTP DELETE Method Response Using Apidog
You can check to see whether the JavaScript HTTP DELETE METHOD was successful depending on the response you receive.
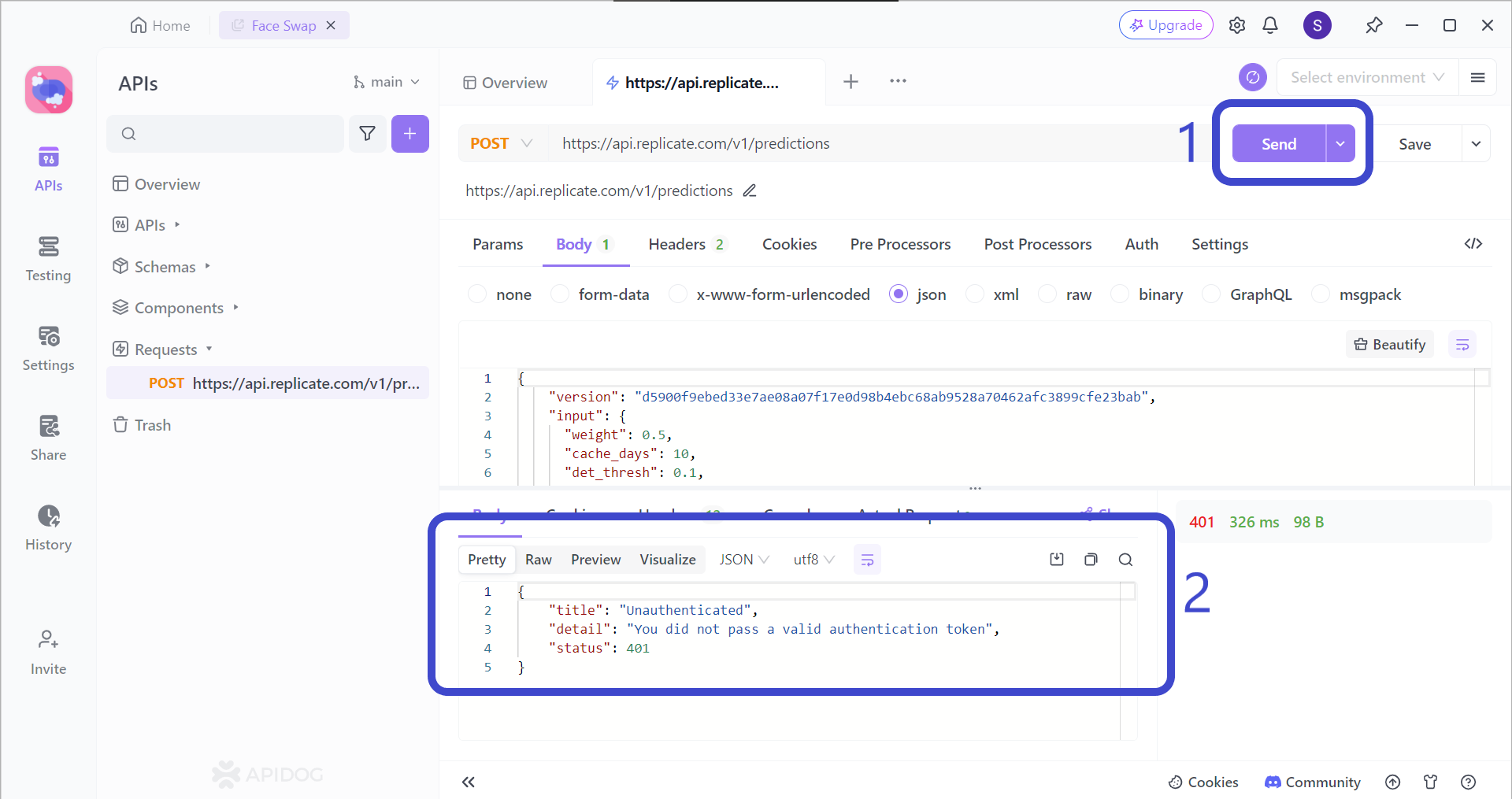
Make the JavaScript HTTP PUT method request by pressing the Send
button found in the right corner of the Apidog window. Then, you should be able to view the response on the bottom portion of the screen.
If your response includes a number that corresponds to a successful status code, it indicates that you have successfully deleted your targetted resource(s). Else, you will have to provide error handling.
Conclusion
The JavaScript HTTP DELETE method serves as a crucial tool for web developers when it comes to managing resources on the server. Its versatility and simplicity make it an integral part of client-server communication, allowing for the deletion of various types of data and resources.
By leveraging the DELETE method, developers can implement features such as user account deletion, content management, resource cleanup, and more in their web applications. This enhances user experience by providing control and flexibility while also ensuring data integrity and security.
Consider Apidog today over alternatives as Apidog can display API details with clarity, reducing the possibility of misreading. Aside from an easy-to-learn user interface, you can conduct other API operations, such as building, testing, and documenting APIs.