GraphQL request: The Lightweight Library for Your API Needs
Discover the power of graphql-request, a lightweight library for making GraphQL API requests effortlessly. Learn how to use it, integrate with apidog, and explore best practices for real-world applications. Perfect for developers seeking efficiency and simplicity.
If you're diving into the world of APIs and looking for a sleek, efficient way to handle your GraphQL queries, you're in the right place. Today, we’re talking about graphql-request, a minimalistic yet powerful library that’s making waves in the API community. Whether you're a seasoned developer or a curious newcomer, this guide will walk you through everything you need to know about graphql-request, and why it might just be the tool you’ve been searching for.
Are you ready to supercharge your GraphQL development? Download Apidog FOR FREE today to enhance your API management and testing experience! With seamless integration with GraphQL, Apidog offers robust testing environments, automatic documentation generation, and efficient monitoring tools. Take your GraphQL queries to the next level 🚀
What is a GraphQL request?
Graphql request is a lightweight library designed to simplify making GraphQL requests. It's a straightforward client that wraps around the GraphQL API, enabling you to send queries and mutations with ease. Unlike bulkier libraries, GraphQL request focuses on being minimal and efficient, which makes it perfect for projects where simplicity and performance are paramount.
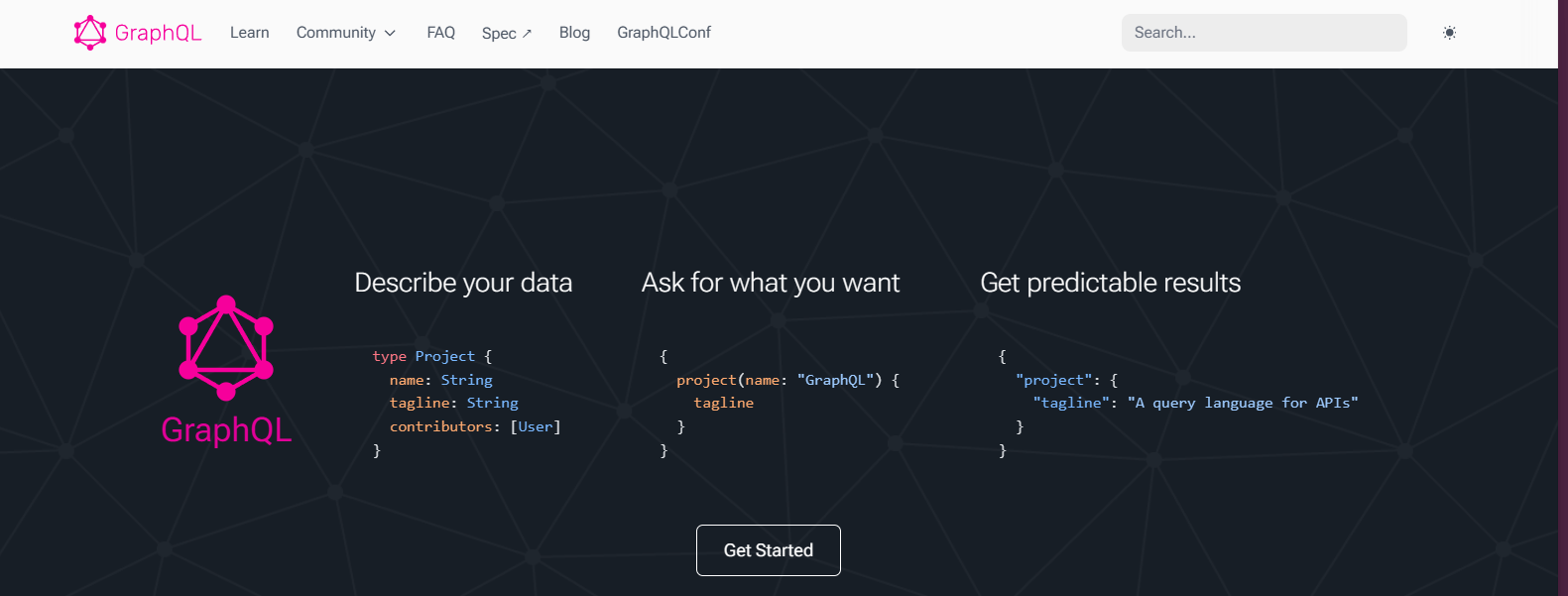
Why Choose GraphQL request?
- Minimalistic Approach: No unnecessary bloat. It does what it’s supposed to do and does it well.
- Ease of Use: Its simple syntax makes it easy to learn and implement.
- Flexibility: Perfect for both small projects and large applications.
- Efficiency: Low overhead means faster performance and lower resource consumption.
These features make GraphQL request an ideal choice for developers who want to keep things simple and efficient. But let’s not just skim the surface—let’s dive deep into how you can leverage this tool in your projects.
Getting Started with GraphQL request
First things first, let's get GraphQL request set up in your project. Whether you're starting a new project or integrating it into an existing one, the process is straightforward.
Installation
To install GraphQL request, you’ll need npm or yarn. Open your terminal and run the following command:
npm install graphql-request
Or, if you prefer using yarn:
yarn add graphql-request
That’s it! You’re ready to start using GraphQL request in your project.
Basic Usage
Using GraphQL request is refreshingly simple. Here’s a basic example to get you started:
import { request, gql } from 'graphql-request';
const endpoint = 'https://api.spacex.land/graphql/';
const query = gql`
{
launchesPast(limit: 5) {
mission_name
launch_date_utc
rocket {
rocket_name
}
links {
video_link
}
}
}
`;
request(endpoint, query).then((data) => console.log(data));
In this example, we’re querying the SpaceX GraphQL API to get information about past launches. The gql
tag is used to parse the query, and the request
function sends it to the specified endpoint. The response is then logged to the console.
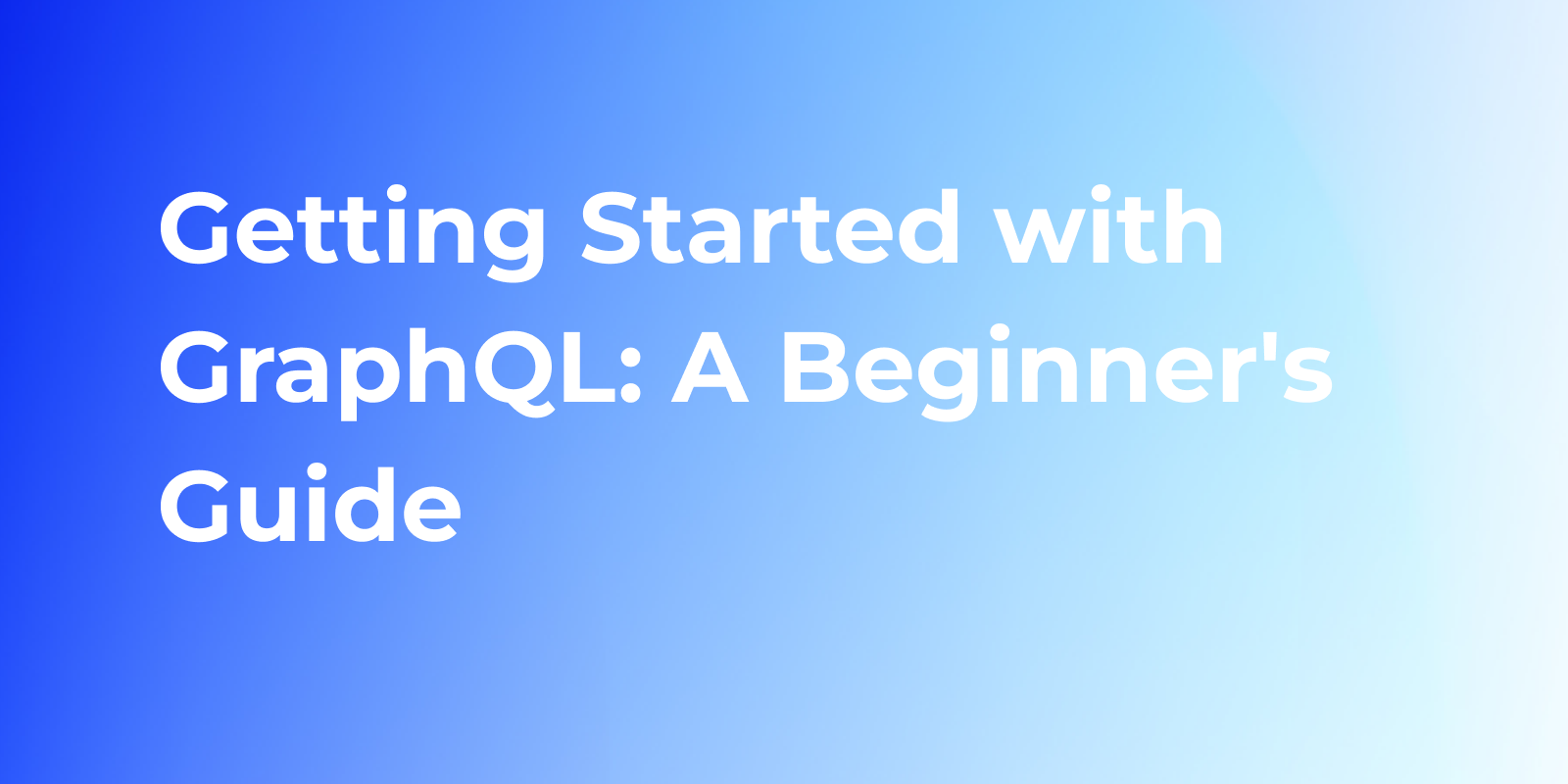
Advanced Features and Usage
Now that we've covered the basics, let’s explore some advanced features and scenarios where GraphQL request truly shines.
Variables in Queries
GraphQL queries often require variables. GraphQL request handles this seamlessly. Here’s how you can include variables in your queries:
const query = gql`
query getLaunches($limit: Int!) {
launchesPast(limit: $limit) {
mission_name
launch_date_utc
rocket {
rocket_name
}
links {
video_link
}
}
}
`;
const variables = {
limit: 3,
};
request(endpoint, query, variables).then((data) => console.log(data));
In this example, we’re passing a limit
variable to control the number of past launches returned by the query. This makes your queries more dynamic and powerful.
Error Handling
No one likes errors, but they’re a part of life—and coding. GraphQL request makes error handling straightforward:
request(endpoint, query, variables)
.then((data) => console.log(data))
.catch((error) => console.error(error));
By chaining a .catch
method to your request, you can easily handle any errors that arise.
Setting Headers
Sometimes, you need to set headers for authentication or other purposes. graphql-request lets you do this effortlessly:
const headers = {
Authorization: 'Bearer YOUR_ACCESS_TOKEN',
};
request(endpoint, query, variables, headers).then((data) => console.log(data));
This is particularly useful when working with APIs that require authentication.
How to create GraphQL request in Apidog
If you're already using Apidog or considering it, you'll be pleased to know that GraphQL request integrates smoothly with it. Apidog is a powerful tool for API management and testing, and combining it with GraphQL request can streamline your workflow.
To create a new GraphQL request in a project, click on "Body" → "GraphQL" in sequence.
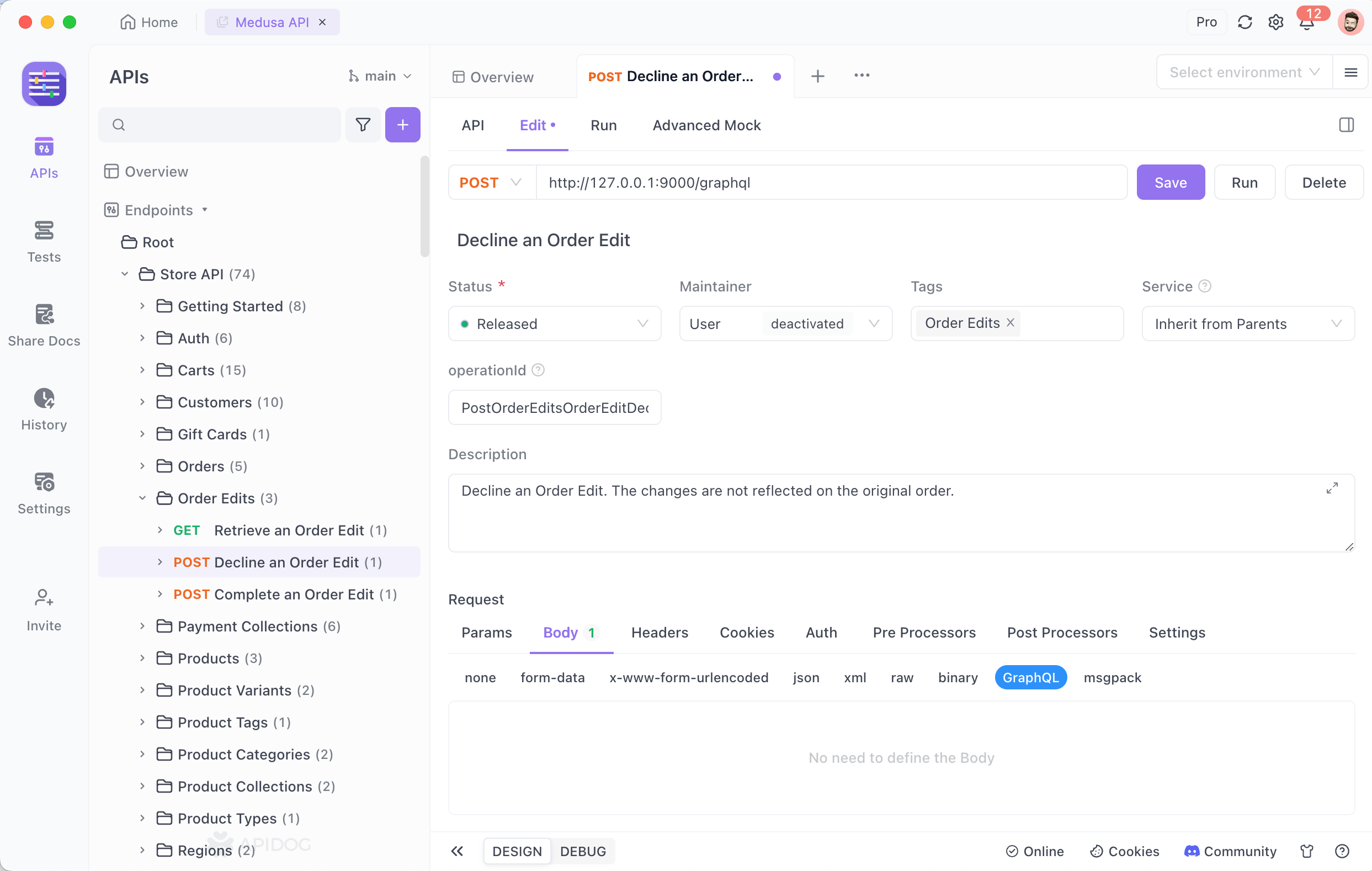
Enter your query in the Query box on the "Run" tab. You can also click the manual Fetch Schema button in the input box to enable the "code completion" feature for Query expressions, assisting in entering Query statements.
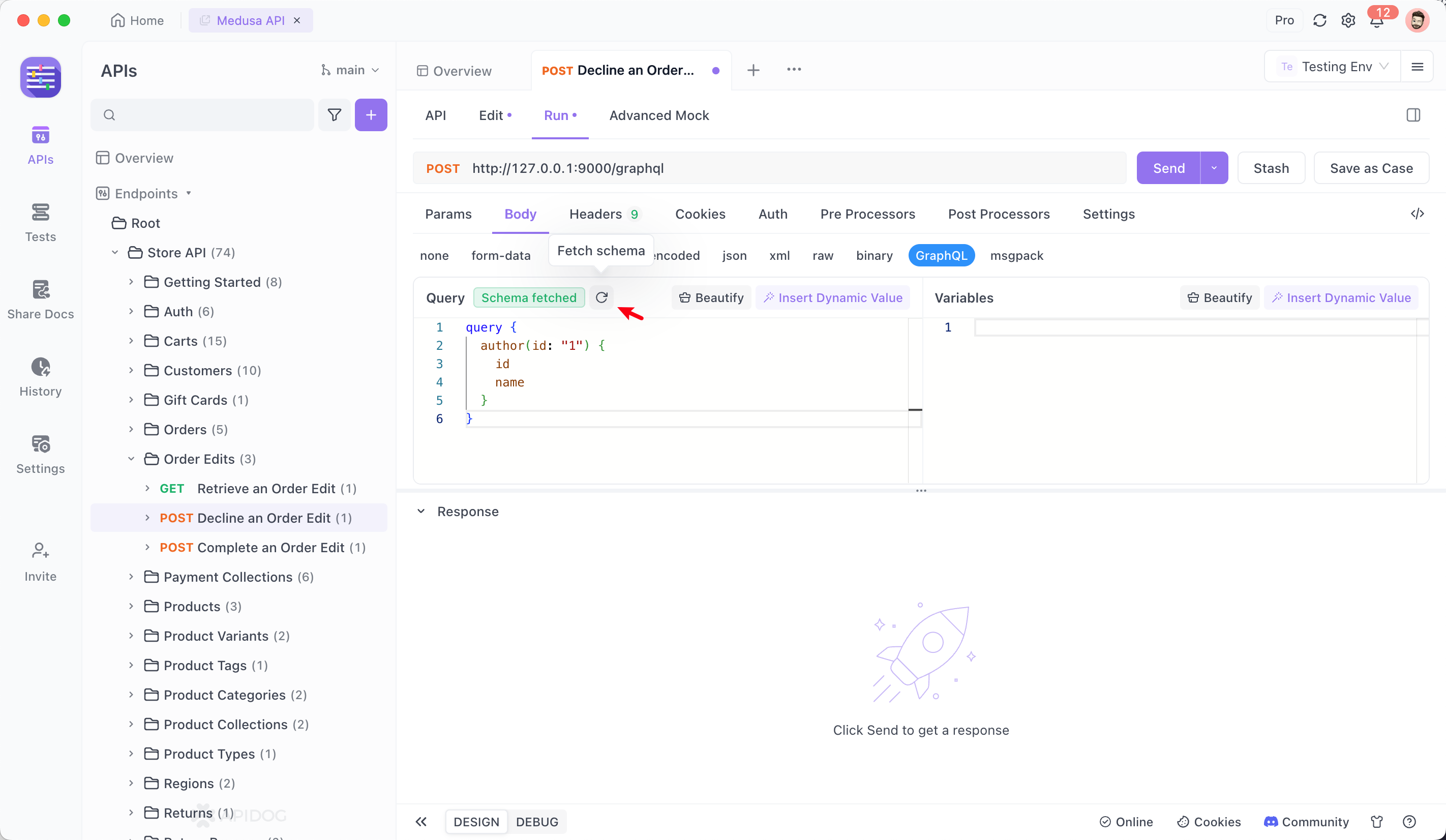
Query statements support using GraphQL variables for requesting. For specific usage, please refer to the GraphQL syntax.
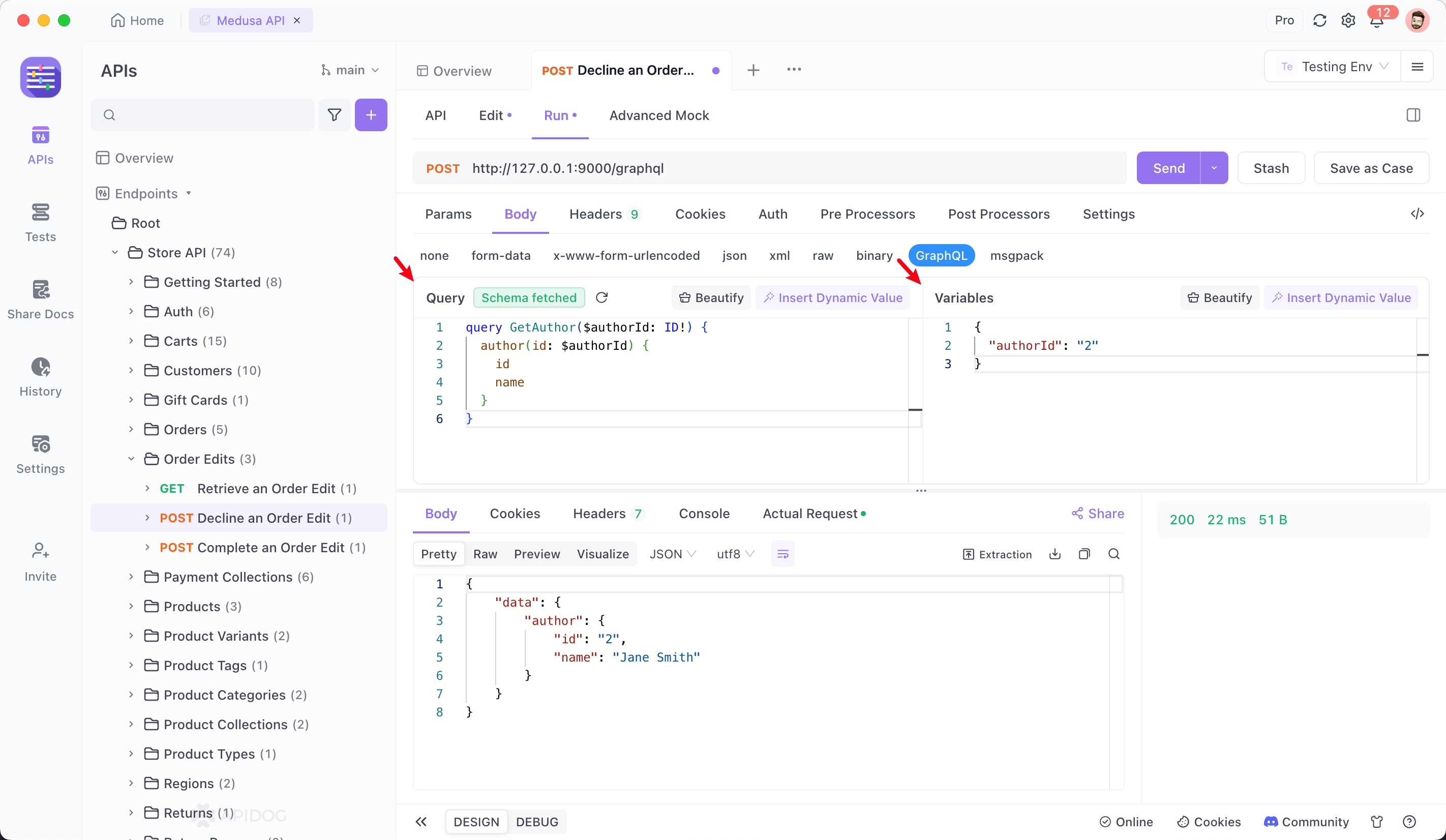
Real-World Use Cases
To truly appreciate the power of GraphQL request, let’s look at some real-world use cases.
Single Page Applications (SPAs)
For SPAs, performance is key. GraphQL request is lightweight, making it perfect for environments where you need to minimize overhead. By using GraphQL request, you can fetch data efficiently and keep your application running smoothly.
Server-Side Rendering (SSR)
In SSR scenarios, you want your data to be ready when the page is served. GraphQL request’s simplicity and speed make it a great choice for fetching data server-side. Whether you’re using Next.js, Nuxt.js, or another SSR framework, GraphQL request can fit seamlessly into your data-fetching strategy.
Mobile Applications
Mobile applications often need to be as efficient as possible due to constraints like network speed and device capabilities. GraphQL request’s minimal footprint ensures that your app remains responsive and efficient, providing a better user experience.
Best Practices
To get the most out of GraphQL request, here are some best practices to keep in mind:
Modularize Your Queries
Keep your queries organized by modularizing them. This makes your codebase easier to maintain and understand.
// queries.js
import { gql } from 'graphql-request';
export const GET_LAUNCHES = gql`
{
launchesPast(limit: 5) {
mission_name
launch_date_utc
rocket {
rocket_name
}
links {
video_link
}
}
}
`;
// main.js
import { request } from 'graphql-request';
import { GET_LAUNCHES } from './queries';
const endpoint = 'https://api.spacex.land/graphql/';
request(endpoint, GET_LAUNCHES).then((data) => console.log(data));
Error Logging
Implement robust error logging to quickly identify and fix issues. This is crucial for maintaining the reliability of your applications.
request(endpoint, query)
.then((data) => console.log(data))
.catch((error) => {
console.error('GraphQL request failed:', error);
// Additional logging logic
});
Utilize TypeScript
If you're using TypeScript, take advantage of its type-checking capabilities to catch errors early in the development process.
import { request, gql } from 'graphql-request';
const query = gql`
{
launchesPast(limit: 5) {
mission_name
launch_date_utc
rocket {
rocket_name
}
links {
video_link
}
}
}
`;
interface Launch {
mission_name: string;
launch_date_utc: string;
rocket: {
rocket_name: string;
};
links: {
video_link: string;
};
}
request<{ launchesPast: Launch[] }>(endpoint, query)
.
then((data) => console.log(data))
.catch((error) => console.error(error));
Conclusion
GraphQL request is a powerful, efficient, and easy-to-use library that can greatly simplify your GraphQL queries. Whether you're working on a small personal project or a large-scale application, it offers the performance and flexibility you need.
By integrating GraphQL requests with tools like Apidog, you can enhance your development workflow, making it easier to test, document, and manage your APIs. With real-world use cases ranging from SPAs to mobile applications, GraphQL request proves to be a versatile tool in any developer's toolkit.
So, what are you waiting for? Give GraphQL requests a try in your next project and experience the benefits for yourself.