In the evolving landscape of web development, efficiency and scalability are paramount. Flask gRPC emerges as a beacon of innovation, offering developers the tools to craft robust microservices with ease. This guide delves into the intricacies of Flask gRPC, from setting up your environment to testing with Apidog. Whether you’re a seasoned developer or new to the scene, mastering Flask gRPC will elevate your projects to new heights. Join us as we explore the synergy of Flask’s simplicity and gRPC’s performance to revolutionize your development workflow.
What is gRPC?
gRPC is a modern open source Remote Procedure Call (RPC) framework developed by Google that can run in any environment and connect services in and across data centers, distributed computing and devices. It supports load balancing, tracing, health checking and authentication with Protocol Buffers and bi-directional streaming.
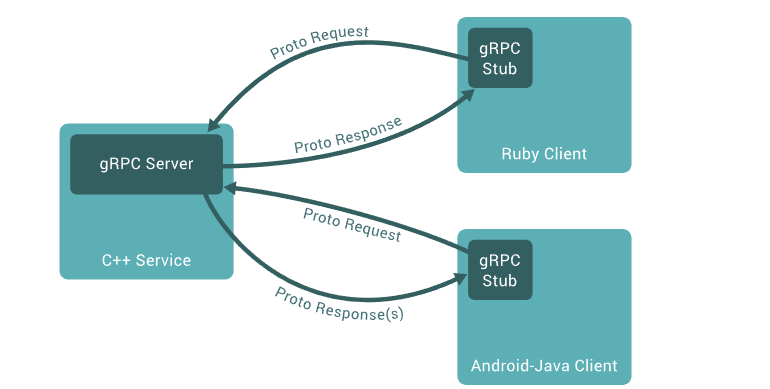
In contrast to REST (Representational State Transfer), which is an architectural style for building web services, gRPC is a protocol that defines how clients and servers communicate with each other. gRPC uses Protocol Buffers, a powerful binary serialization toolset and language, to define the service and message structure. It can automatically generate idiomatic client and server stubs for your service in a variety of languages and platforms.
gRPC is a high-performance, open-source, and cross-platform RPC framework that can be used to connect services in and across data centers, distributed computing, and devices.
Why use gRPC?
gRPC is a modern and high-performance Remote Procedure Call (RPC) framework that is gaining popularity among developers. It provides a robust solution for client-server architectures like APIs and microservices. gRPC uses HTTP/2 as its underlying transport protocol, which makes it faster and more efficient than traditional REST APIs.
Some of the advantages of using gRPC include:
- Performance: gRPC is faster than REST APIs because it uses binary data instead of text data, which reduces the size of the payload and the number of round trips required to complete a request.
- Interoperability: gRPC supports multiple programming languages, which makes it easy to build APIs that can be used across different platforms and devices.
- Ease of use: gRPC provides a simple and intuitive API that makes it easy to build and maintain APIs.
- Streaming: gRPC supports both client-side and server-side streaming, which allows for more efficient communication between clients and servers.
In summary, gRPC is a powerful and efficient RPC framework that provides a modern alternative to traditional REST APIs. It is especially useful for building APIs that require high performance and interoperability across different platforms and devices.
What is flask gRPC?
Flask gRPC is a combination of Flask, a lightweight web framework in Python, and gRPC, a high-performance, open-source universal RPC framework. By integrating gRPC with Flask, you can create microservices in Python that communicate with each other over gRPC, which is particularly useful for building distributed systems and services that need to communicate efficiently.
In a Flask gRPC setup, you would typically have a Flask application that can serve web pages or APIs, and alongside it, you would have gRPC services that can handle more complex or performance-intensive tasks. This allows you to leverage the simplicity of Flask for web interfaces while using gRPC for efficient service-to-service communication.
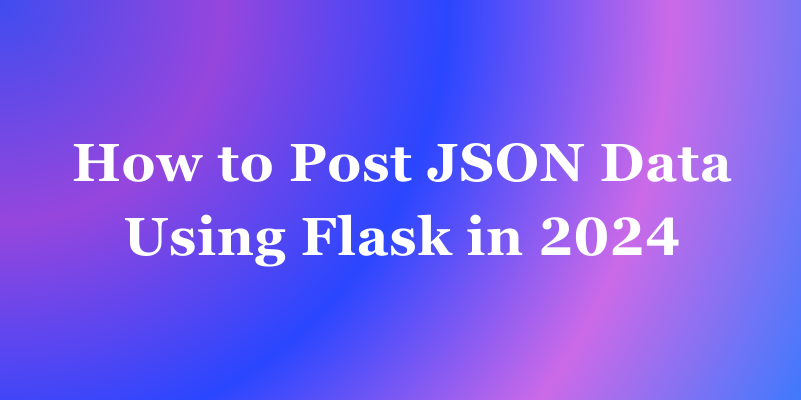
How does Flask gRPC work?
Flask gRPC works by combining the Flask web framework with the gRPC (gRPC Remote Procedure Call) system to create a powerful tool for building microservices. Here’s a step-by-step explanation of how it operates:
Define gRPC Services: You start by defining your gRPC services using Protocol Buffers (protobuf). This involves specifying service methods and their request and response message types in a .proto
file.
Generate Code: Using the protoc
compiler with a gRPC plugin, you generate the server and client code from your .proto
file. This code includes the classes for your service and the methods you defined.
Implement Server: In your Flask application, you implement the server side of your gRPC services. This means writing the actual Python code that performs the operations described by your service methods.
Create gRPC Server: You then create a gRPC server that listens for incoming RPC calls. This server uses the implementation you wrote to handle these calls.
Integrate with Flask: On the Flask side, you set up routes as you normally would for a web application. However, for routes that should handle gRPC calls, you direct those requests to the gRPC server.
Run the Application: When you run your Flask application, it can handle both regular HTTP requests and gRPC calls, allowing for efficient communication between different parts of your system or with other systems.
This setup allows you to take advantage of Flask’s ease of use and gRPC’s performance benefits, making it ideal for microservices architectures.
How to use Flask gRPC
Using Flask with gRPC involves several steps to set up a microservice that can handle both web requests and RPC calls. Here’s a simplified guide to get you started:
Install Necessary Libraries: Make sure you have Flask and the gRPC libraries installed. You can install them using pip:
pip install Flask grpcio
Define Your gRPC Service: Create a .proto
file to define your gRPC service and messages. For example:
syntax = "proto3";
package my_package;
service MyService {
rpc MyMethod (MyRequest) returns (MyResponse) {}
}
message MyRequest {
string message = 1;
}
message MyResponse {
string message = 1;
}
Generate gRPC Code: Use the protoc
compiler to generate Python code from your .proto
file:
python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. my_service.proto
Implement gRPC Server: Write the server-side implementation for your service in Python.
Create a Flask App: Set up a Flask application as you normally would.
Integrate gRPC with Flask: Modify your Flask app to handle gRPC requests by creating a gRPC server and directing appropriate requests to it.
Run Your Application: Start your Flask application, which can now handle both HTTP and gRPC requests.
Here’s a basic example of how your Flask app might look after integrating gRPC:
from flask import Flask
import grpc
import my_service_pb2
import my_service_pb2_grpc
app = Flask(__name__)
@app.route('/')
def home():
channel = grpc.insecure_channel('localhost:50051')
stub = my_service_pb2_grpc.MyServiceStub(channel)
response = stub.MyMethod(my_service_pb2.MyRequest(message='Hello, gRPC!'))
return response.message
if __name__ == '__main__':
app.run()
This code sets up a Flask route that, when visited, sends a gRPC request to a locally running gRPC service and returns the response.
Test your Flask gRPC with Apidog
Testing your Flask gRPC application with Apidog involves a few steps to ensure that your gRPC services are functioning correctly and to debug any issues that may arise.
Server Streaming
Server Streaming, as the name implies, involves sending multiple response data in a single request. For instance, it could involve subscribing to all the transaction price data of stocks within a one-minute timeframe.
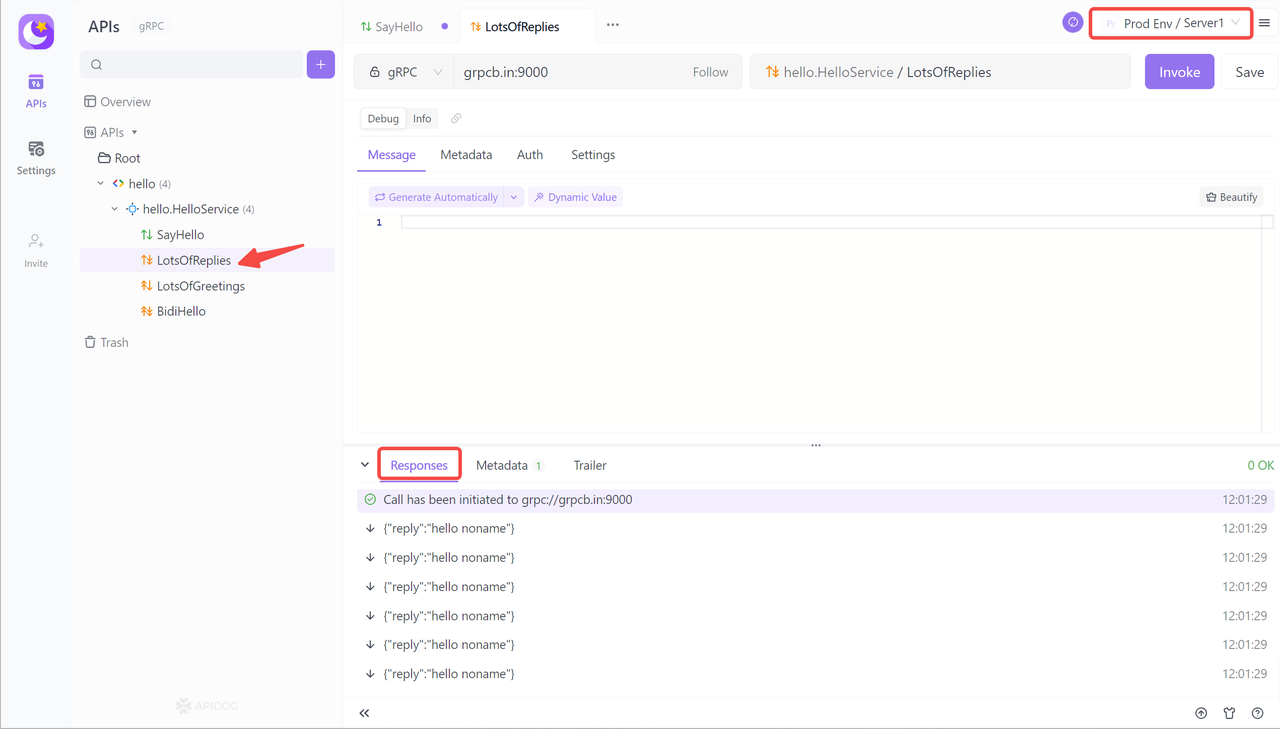
Client Streaming
In this mode, the client can continuously send multiple request messages to the server without waiting for immediate responses. After processing all the requests, the server sends a single response message back to the client. This approach is well-suited for efficiently transmitting large amounts of data in a streaming manner, which helps reduce latency and optimize data exchange.
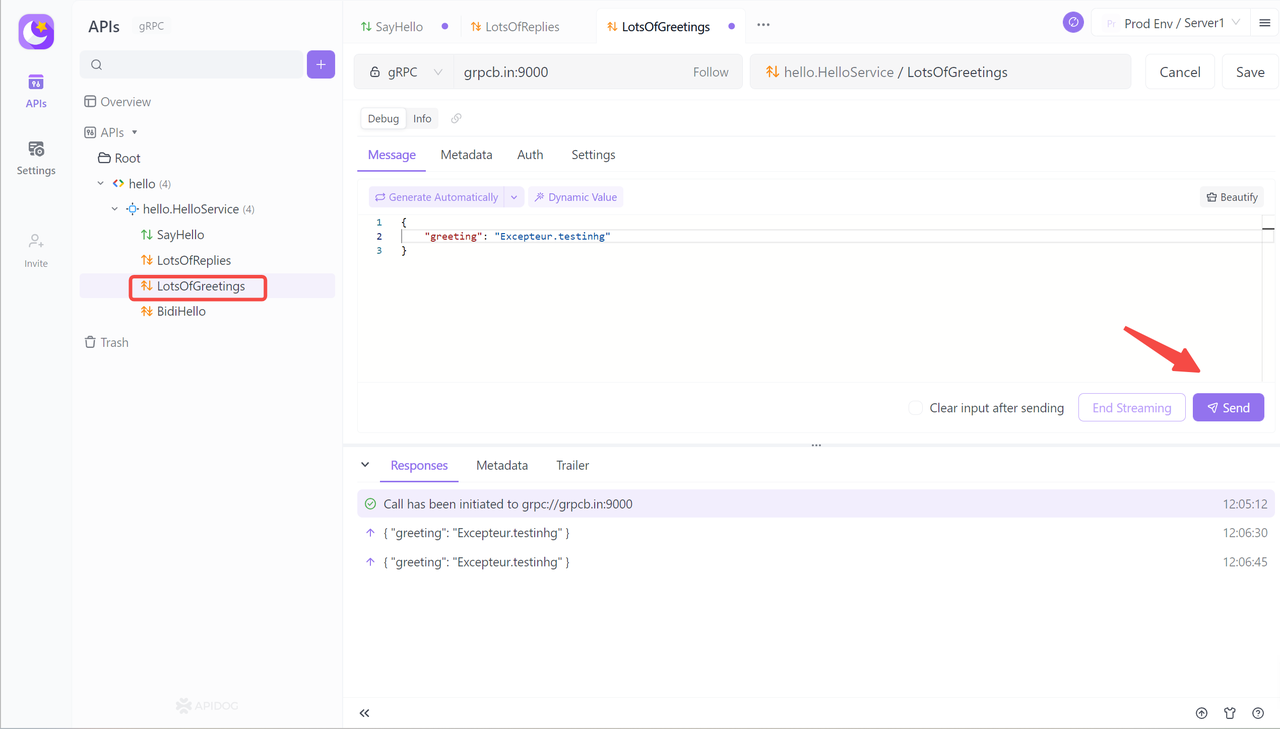
Bidirectional Streaming
Bidirectional Streaming enables clients and servers to establish persistent bidirectional communication and transmit multiple messages simultaneously. It is commonly employed in online games and real-time video call software, and is well-suited for real-time communication and large-scale data transmission scenarios. After initiating the call, the client and the server maintain a session between them and receive real-time responses after sending different request contents.
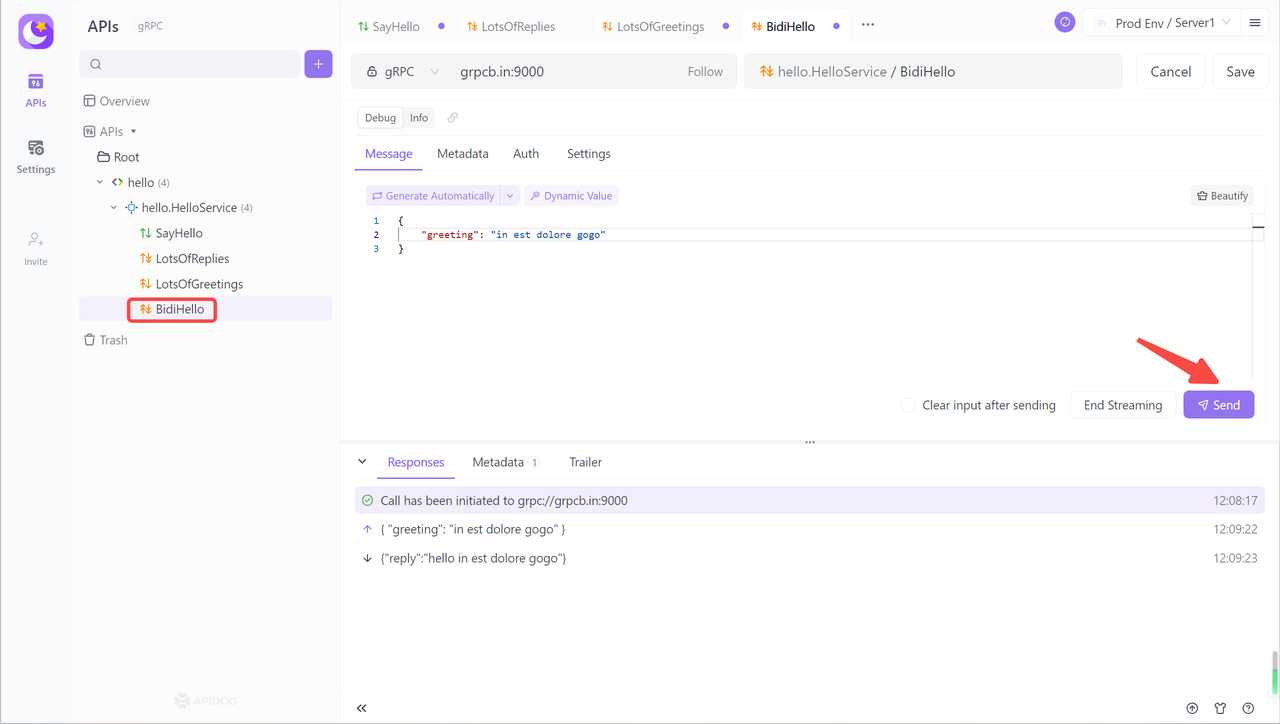
Collaborating on gRPC APIs
Apidog can generate human-readable gRPC interface documents from .proto files, facilitating team collaboration on interfaces. Click the menu button on the right side of the interface to obtain the collaboration link and share it with other team members to align the interface debugging approach.
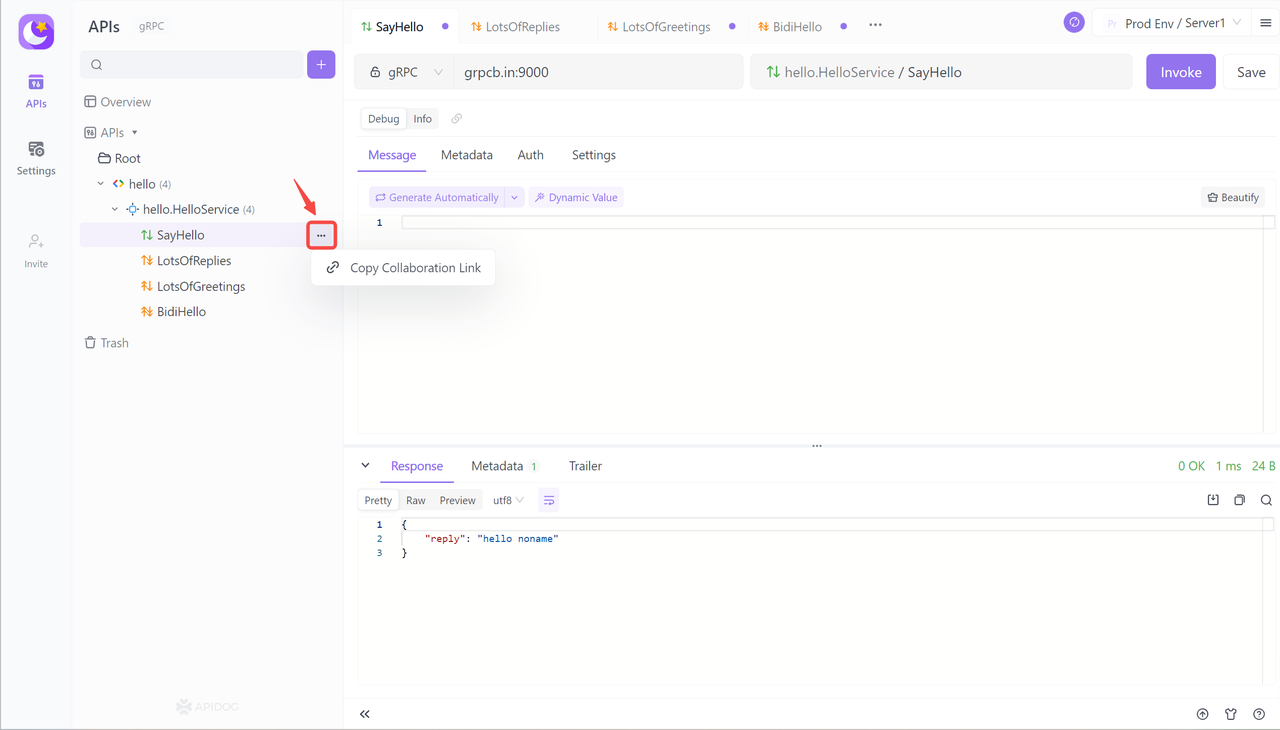
Conclusion
In conclusion, Flask gRPC is a powerful combination that allows developers to build efficient and scalable microservices by leveraging the simplicity of Flask for web interfaces and the performance of gRPC for inter-service communication. By defining services with Protocol Buffers, generating server and client code, and integrating gRPC with a Flask application, developers can create systems that handle both HTTP requests and gRPC calls. Testing these services with tools like Apidog ensures that they work as expected and are ready for deployment in a production environment. This technology stack is particularly useful for distributed systems where performance and communication efficiency are critical.