If you've ever dived into the world of web development, you know how crucial it is to handle parameters effectively. Whether you're a seasoned developer or just starting out, understanding how to pass parameters in FastAPI can significantly boost your efficiency and productivity.
Alright, with that out of the way, let’s dive into the fascinating world of FastAPI and parameter passing.
What is FastAPI?
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. It's designed to be easy to use and learn, while also providing the speed and performance of async programming. FastAPI is perfect for building high-performance applications and microservices. It also integrates seamlessly with modern tools and libraries.
Why FastAPI?
FastAPI boasts several advantages that make it a top choice among developers:
- Speed: It's fast. In fact, it's one of the fastest Python web frameworks available.
- Ease of Use: It’s designed to be intuitive and straightforward.
- Documentation: Automatic generation of interactive API documentation using OpenAPI and JSON Schema.
- Validation: It provides automatic request data validation.
- Async Support: Full support for asynchronous programming.
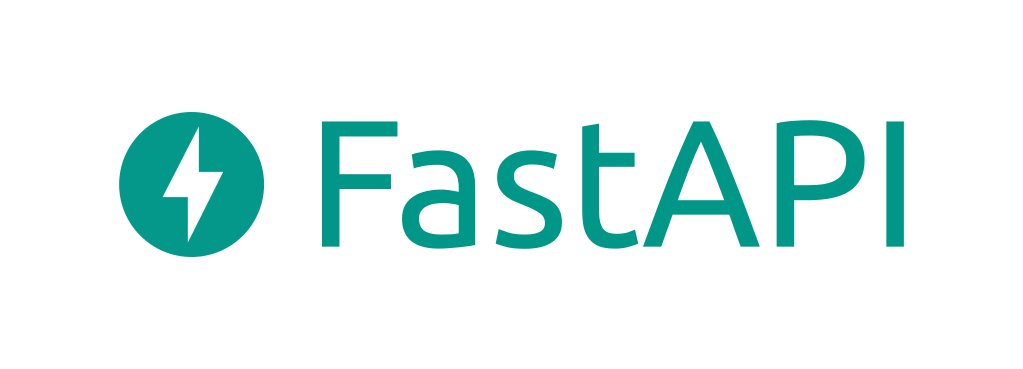
Getting Started with FastAPI
Before we delve into passing parameters, let's ensure you have FastAPI and Uvicorn (an ASGI server) installed. You can install them using pip:
pip install fastapi uvicorn
Now, let’s create a simple FastAPI application to get started:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
You can run this application using Uvicorn:
uvicorn main:app --reload
Open your browser and navigate to http://127.0.0.1:8000
, and you should see {"Hello": "World"}
.
Passing Parameters in FastAPI
In FastAPI, you can pass parameters in several ways. Let's explore each method in detail.
Path Parameters
Path parameters are used to capture variable parts of the URL path. For instance, if you want to capture a user's ID from the URL, you can do it like this:
@app.get("/users/{user_id}")
def read_user(user_id: int):
return {"user_id": user_id}
In this example, user_id
is a path parameter. When you visit http://127.0.0.1:8000/users/1
, the user_id
parameter will be captured and passed to the read_user
function.
Type Hinting
Notice how we used int
type hinting for user_id
. FastAPI uses these type hints to validate the incoming parameters. If you pass a string instead of an integer, FastAPI will return a 422 status code indicating a validation error.
Query Parameters
Query parameters are used to pass optional parameters in the URL. They come after the ?
in the URL. For example:
@app.get("/items/")
def read_item(item_id: int, q: str = None):
return {"item_id": item_id, "q": q}
Here, item_id
is a required query parameter, while q
is optional. You can access this endpoint by visiting http://127.0.0.1:8000/items/?item_id=1&q=search
.
Request Body Parameters
Request body parameters are used to pass JSON data to the API endpoint. You can define a Pydantic model to validate the incoming JSON data. Here's an example:
from pydantic import BaseModel
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the create_item
function expects a JSON object that matches the Item
model. FastAPI will automatically validate the incoming JSON data and return a 422 status code if the data is invalid.
Header Parameters
Header parameters can be accessed using the Header
class from fastapi
. For example:
from fastapi import Header
@app.get("/items/")
def read_item(user_agent: str = Header(None)):
return {"User-Agent": user_agent}
This function will capture the User-Agent
header from the request.
Form Parameters
Form parameters are used to handle form data in the request. You can use the Form
class to capture form data. Here's an example:
from fastapi import Form
@app.post("/login/")
def login(username: str = Form(...), password: str = Form(...)):
return {"username": username}
In this case, the login
function expects form data with username
and password
fields.
File Parameters
File parameters allow you to handle file uploads. You can use the File
class from fastapi
to capture file data. Here's an example:
from fastapi import File, UploadFile
@app.post("/uploadfile/")
async def create_upload_file(file: UploadFile = File(...)):
return {"filename": file.filename}
In this example, the create_upload_file
function expects a file to be uploaded. FastAPI will handle the file upload and provide the file details to the function.
Dependency Injection
FastAPI also supports dependency injection, allowing you to define reusable components that can be injected into your functions. Here's an example:
from fastapi import Depends
def get_token_header():
return {"Authorization": "Bearer my_token"}
@app.get("/items/")
def read_item(token: dict = Depends(get_token_header)):
return token
In this case, the read_item
function depends on the get_token_header
function, which provides the token header.
Combining Parameters
You can combine different types of parameters in a single endpoint. Here's an example that uses path, query, and body parameters:
@app.put("/items/{item_id}")
def update_item(
item_id: int,
q: str = None,
item: Item = Body(...)
):
return {"item_id": item_id, "q": q, "item": item}
This function expects a path parameter (item_id
), a query parameter (q
), and a body parameter (item
).
Handling Parameter Validation
FastAPI uses Pydantic for data validation, which provides a rich set of features for defining and validating data models. You can use Pydantic to enforce constraints on your parameters. For example:
from pydantic import BaseModel, Field
class Item(BaseModel):
name: str
description: str = Field(None, max_length=300)
price: float = Field(..., gt=0)
tax: float = None
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the description
field has a maximum length constraint, and the price
field must be greater than 0.
Advanced Parameter Features
FastAPI provides several advanced features for handling parameters, including:
- Aliases: You can use aliases for your parameters.
- Nested Models: You can use nested Pydantic models for complex data structures.
- Default Values: You can set default values for your parameters.
- Regular Expressions: You can use regular expressions to validate parameters.
Using Aliases
You can define aliases for your parameters using the Field
class from Pydantic. For example:
class Item(BaseModel):
name: str = Field(..., alias="itemName")
description: str = None
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the name
field will be aliased as itemName
.
Nested Models
You can use nested Pydantic models to handle complex data structures. For example:
class SubItem(BaseModel):
name: str
description: str = None
class Item(BaseModel):
name: str
description: str = None
sub_item: SubItem
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the Item
model contains a nested SubItem
model.
Default Values
You can set default values for your parameters. For example:
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = 10.0
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the tax
field has a default value of 10.0.
Regular Expressions
You can use regular expressions to validate your parameters. For example:
from pydantic import Field
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
code: str = Field(..., regex="^[A-Z0-9]+$")
@app.post("/items/")
def create_item(item: Item):
return item
In this case, the code
field
must match the regular expression ^[A-Z0-9]+$
.
Using Apidog with FastAPI to Pass Parameters
Integrating Apidog into your FastAPI development process can significantly enhance your productivity and streamline your API testing workflow. Apidog is a powerful tool that provides a user-friendly interface for testing and debugging your APIs, making it easier to manage and pass parameters effectively.
Simplifying Parameter Management with Apidog
When working with FastAPI, passing parameters correctly is crucial for the functionality of your endpoints. Apidog simplifies this process by providing an intuitive platform where you can visually create and manage your API requests. Here’s how Apidog can help you with parameter management:
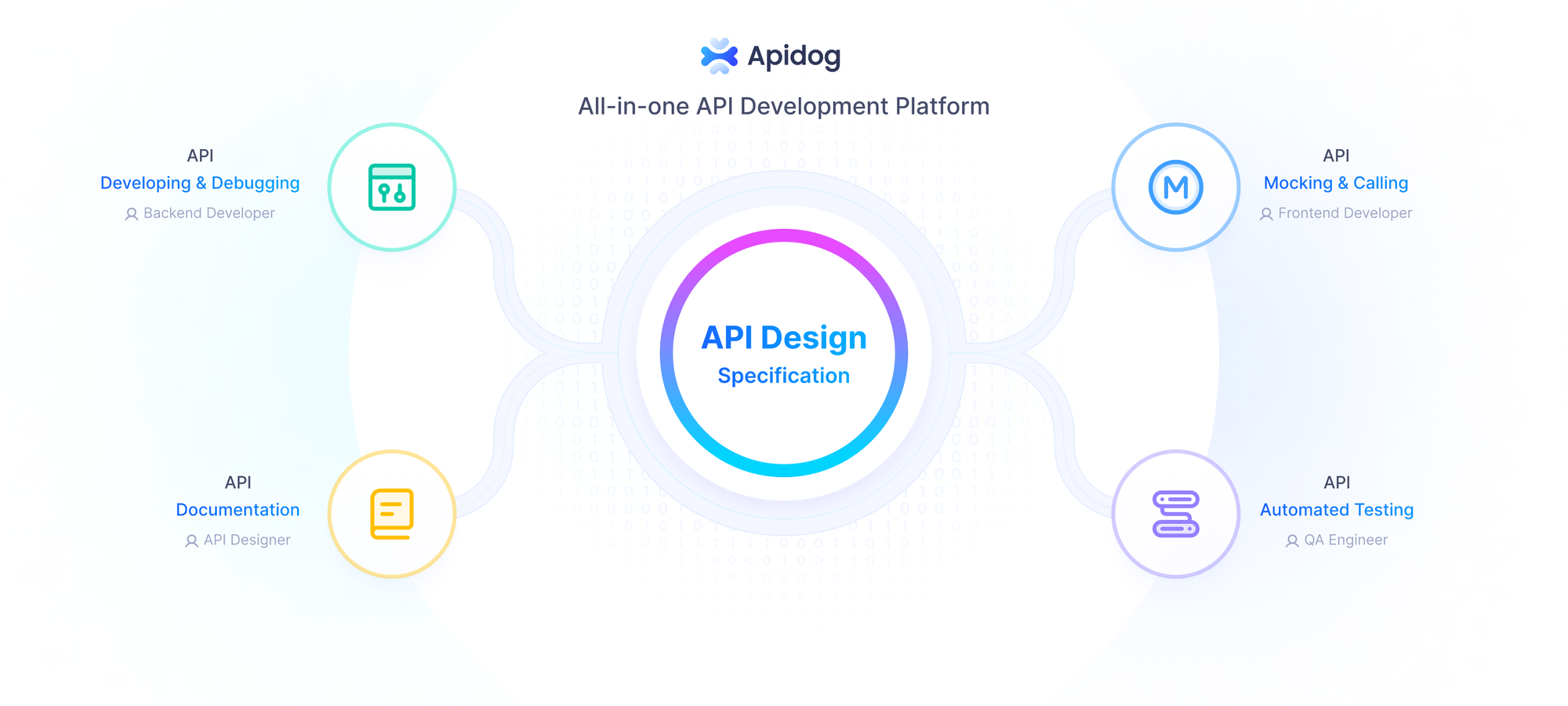
Interactive Documentation: Apidog automatically generates interactive API documentation based on your FastAPI code. This documentation includes all the parameters your endpoints accept, making it easy to understand and test them.
Parameter Testing: You can use Apidog to test different types of parameters, such as path parameters, query parameters, request body parameters, and more. It allows you to input and modify these parameters dynamically, ensuring that your FastAPI endpoints handle them correctly.
Validation Feedback: Apidog provides real-time feedback on parameter validation. If you pass invalid parameters, it highlights the errors and shows detailed messages, helping you quickly identify and fix issues.
Simulating Real-world Scenarios: With Apidog, you can simulate various real-world scenarios by passing different sets of parameters. This is particularly useful for testing edge cases and ensuring your FastAPI application handles all possible inputs gracefully.
Example: Testing Path and Query Parameters with Apidog
Let’s look at an example of how you can use Apidog to test path and query parameters in a FastAPI endpoint.
Suppose you have the following FastAPI endpoint:
from fastapi import FastAPI
app = FastAPI()
@app.get("/items/{item_id}")
def read_item(item_id: int, q: str = None):
return {"item_id": item_id, "q": q}
To test this endpoint with Apidog:
Create a New Request: Open Apidog and create a new request for the GET /items/{item_id}
endpoint.
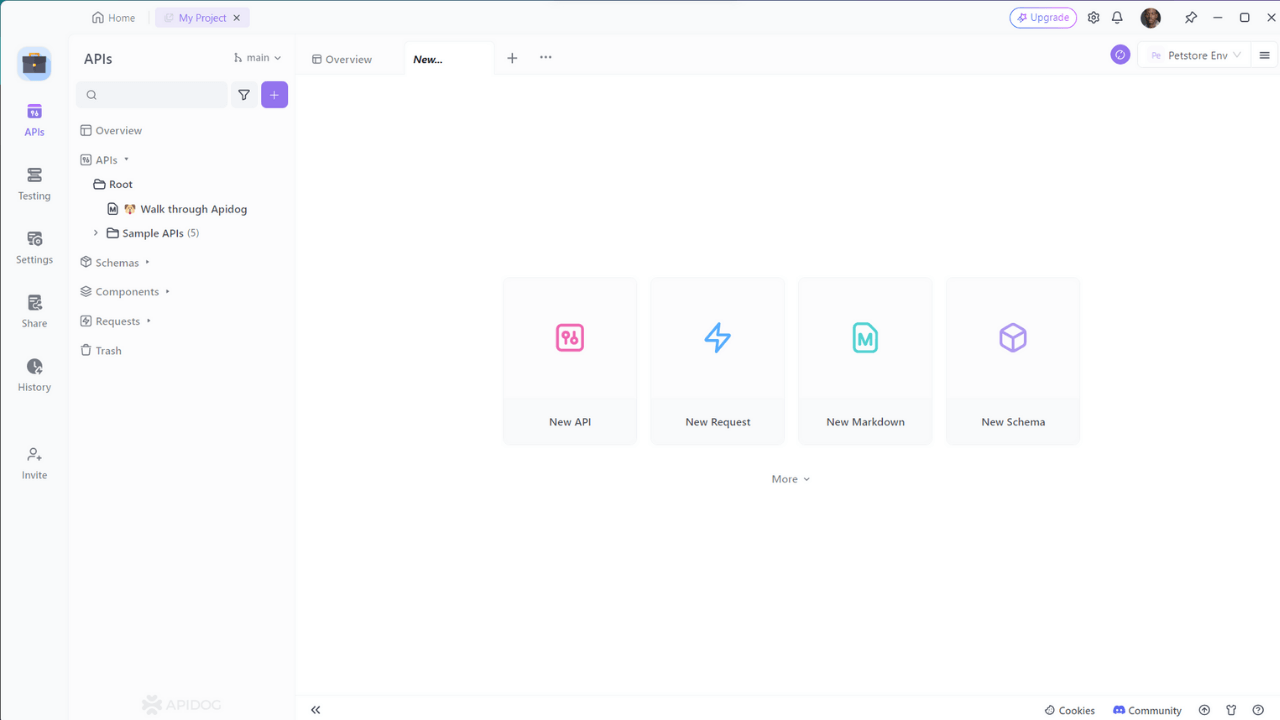
Specify Path Parameters and add Query Parameters: Enter a value for the item_id
path parameter. For example, you might use 123
. Then add a query parameter q
with a value, such as search_term
.
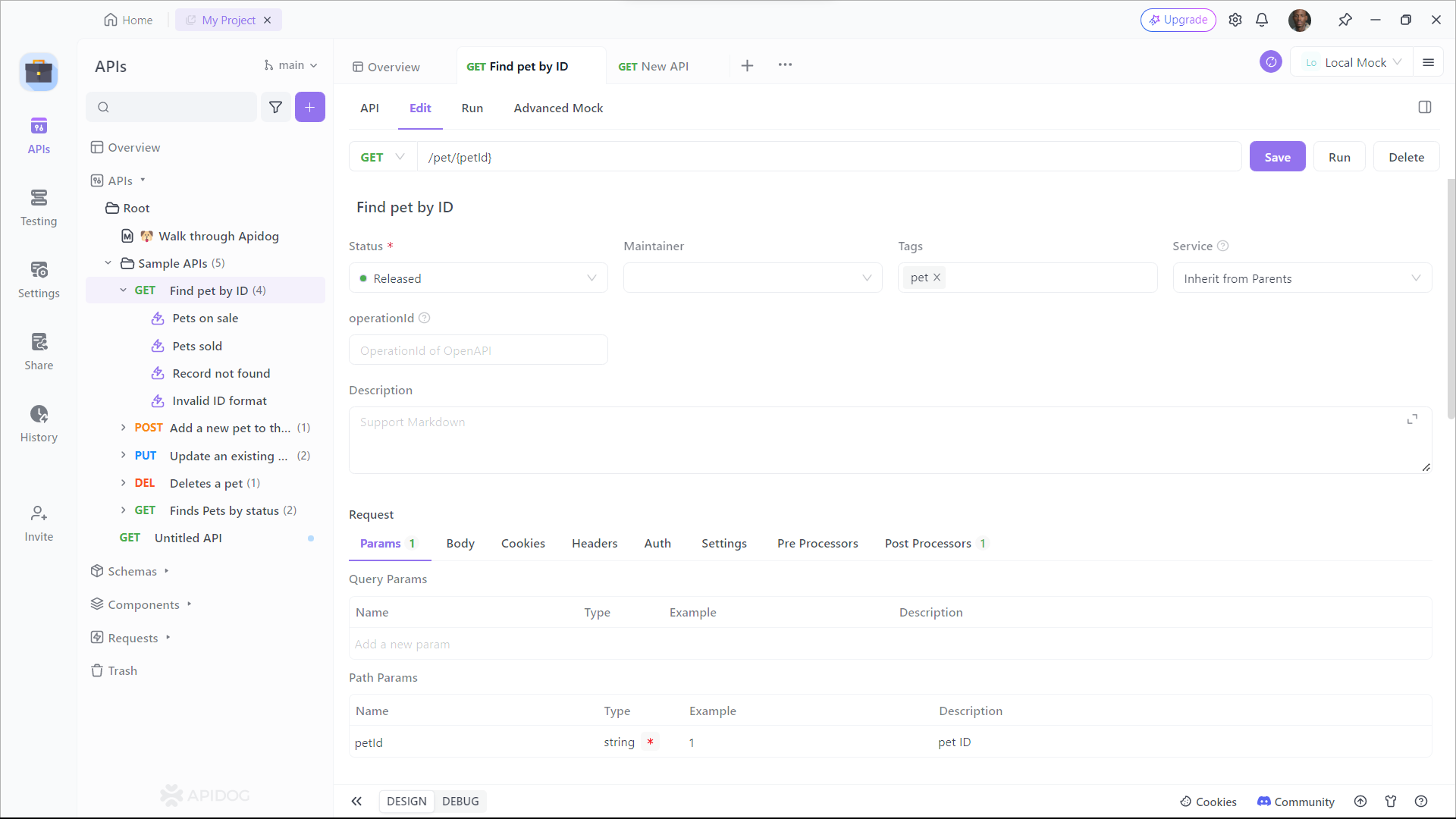
Send the request and observe the response. Apidog will display the returned JSON data, showing how the parameters were handled by FastAPI.
Enhancing Your Workflow
By incorporating Apidog into your FastAPI development workflow, you can ensure that your parameter handling is robust and error-free. The seamless integration of Apidog with FastAPI’s parameter validation features makes it an invaluable tool for both development and testing.
By integrating Apidog into your FastAPI development, you can streamline the process of passing and testing parameters, ensuring your API endpoints are robust and reliable.
Conclusion
Passing parameters in FastAPI is a breeze, thanks to its intuitive design and robust validation features. Whether you're dealing with path parameters, query parameters, or complex JSON data, FastAPI has you covered.
Remember, efficient API development starts with the right tools. Download Apidog for free to streamline your API testing and debugging process. It’s a game-changer for developers working with APIs!
FastAPI is a powerful framework that can significantly boost your productivity and the performance of your applications. With its easy-to-use interface and comprehensive validation features, you can focus on building great applications without worrying about the underlying infrastructure.
Happy coding!