APIs have become a vital part of modern web development. They allow applications to communicate and exchange data seamlessly. If you're diving into the world of APIs, you’ve probably heard of cURL. It's a powerful tool for making HTTP requests, but did you know you can harness its power in Python too? In this blog post, we'll explore how to use cURL in Python to make your API interactions smoother and more efficient.
But before we dive in, I want to introduce you to Apidog. It’s a fantastic tool that simplifies API development, testing, and documentation. You can download Apidog for free and start boosting your API game right away!
What is cURL?
cURL is a command-line tool and library for transferring data with URLs. It's widely used for making HTTP requests, and it supports a variety of protocols, including HTTP, HTTPS, FTP, and more. Developers love cURL for its simplicity and versatility.
When you use cURL, you can quickly test endpoints, fetch data, and even automate tasks that involve network operations. For instance, you can use cURL to send a GET request to an API and retrieve data or to post data to a server.
Why Use cURL in Python?
Python is known for its readability and ease of use, making it a popular choice for web development and data science. Integrating cURL with Python allows you to leverage the power of cURL within a Pythonic environment. This combination can make your API requests more manageable and your code more maintainable.
Here are some reasons to use cURL in Python:
- Simplicity: cURL's straightforward syntax makes it easy to learn and use.
- Flexibility: cURL supports various protocols and options, allowing you to handle complex requests.
- Integration: Using cURL in Python lets you integrate HTTP requests seamlessly into your Python applications.
- Efficiency: cURL is highly efficient, making it suitable for high-performance applications.
Getting Started with cURL in Python
To start using cURL in Python, you'll need the pycurl
library. It's a Python interface to cURL, providing a way to execute cURL commands within Python scripts. You can install pycurl
using pip:
pip install pycurl
Once you have pycurl
installed, you're ready to make your first cURL request in Python.
Making Simple API Requests
Let's start with a simple example: making a GET request to an API. We'll use a public API that returns JSON data. Here's how you can do it:
import pycurl
import io
import json
# Define the URL of the API endpoint
url = 'https://api.example.com/data'
# Create a buffer to hold the response
response_buffer = io.BytesIO()
# Initialize a cURL object
curl = pycurl.Curl()
# Set the URL and other options
curl.setopt(curl.URL, url)
curl.setopt(curl.WRITEDATA, response_buffer)
# Perform the request
curl.perform()
# Get the HTTP response code
response_code = curl.getinfo(curl.RESPONSE_CODE)
# Check if the request was successful
if response_code == 200:
# Get the response data
response_data = response_buffer.getvalue().decode('utf-8')
# Parse the JSON data
json_data = json.loads(response_data)
print(json_data)
else:
print(f'Request failed with status code {response_code}')
# Clean up
curl.close()
In this example, we:
- Define the API endpoint URL.
- Create a buffer to store the response.
- Initialize a cURL object.
- Set the URL and other options using
curl.setopt
. - Perform the request with
curl.perform
. - Check the HTTP response code to see if the request was successful.
- Retrieve and parse the JSON data from the response.
- Clean up by closing the cURL object.
Handling Responses
Handling API responses is crucial for processing the data you receive. In the previous example, we checked the HTTP status code and parsed JSON data. Let's expand on this by handling different types of responses and errors.
JSON Responses
Most APIs return JSON data, which is easy to parse in Python. Here's how to handle JSON responses:
if response_code == 200:
response_data = response_buffer.getvalue().decode('utf-8')
try:
json_data = json.loads(response_data)
print(json_data)
except json.JSONDecodeError:
print('Failed to parse JSON response')
else:
print(f'Request failed with status code {response_code}')
Handling Errors
It's essential to handle errors gracefully to ensure your application remains robust. Here's an example of handling different types of errors:
try:
curl.perform()
response_code = curl.getinfo(curl.RESPONSE_CODE)
if response_code == 200:
response_data = response_buffer.getvalue().decode('utf-8')
json_data = json.loads(response_data)
print(json_data)
else:
print(f'Request failed with status code {response_code}')
except pycurl.error as e:
errno, errstr = e.args
print(f'cURL error: {errstr} (errno: {errno})')
finally:
curl.close()
Advanced cURL Options
cURL offers many advanced options to customize your requests. Here are some commonly used options:
- HTTP Headers: You can set custom headers using the
HTTPHEADER
option. - Timeouts: Use the
TIMEOUT
andCONNECTTIMEOUT
options to set timeouts for the request. - Authentication: Use the
USERPWD
option for basic authentication.
Here's an example of setting custom headers and a timeout:
headers = [
'Content-Type: application/json',
'Authorization: Bearer your_token'
]
curl.setopt(curl.HTTPHEADER, headers)
curl.setopt(curl.TIMEOUT, 10)
Error Handling
Handling errors effectively is critical for building reliable applications. Besides checking HTTP status codes, you should handle network errors, timeouts, and other exceptions. Here’s how you can enhance your error handling:
try:
curl.perform()
response_code = curl.getinfo(curl.RESPONSE_CODE)
if response_code == 200:
response_data = response_buffer.getvalue().decode('utf-8')
try:
json_data = json.loads(response_data)
print(json_data)
except json.JSONDecodeError:
print('Failed to parse JSON response')
else:
print(f'Request failed with status code {response_code}')
except pycurl.error as e:
errno, errstr = e.args
print(f'cURL error: {errstr} (errno: {errno})')
finally:
curl.close()
Real-World Examples
To make the most of cURL in Python, let’s explore some real-world examples. We'll cover common use cases like sending POST requests, handling form data, and working with file uploads.
Sending POST Requests
Sending a POST request with cURL in Python is straightforward. You can send JSON data or form data as needed. Here’s how to send a POST request with JSON data:
post_data = json.dumps({'key': 'value'})
headers = ['Content-Type: application/json']
curl.setopt(curl.POST, 1)
curl.setopt(curl.POSTFIELDS, post_data)
curl.setopt(curl.HTTPHEADER, headers)
Handling Form Data
If you need to send form data, you can use the POSTFIELDS
option with a URL-encoded string:
form_data = 'key1=value1&key2=value2'
curl.setopt(curl.POST, 1)
curl.setopt(curl.POSTFIELDS, form_data)
curl.setopt(curl.HTTPHEADER, ['Content-Type: application/x-www-form-urlencoded'])
Working with File Uploads
Uploading files is a common task in web applications. With cURL, you can handle file uploads effortlessly. Here’s how to upload a file using cURL in Python:
curl.setopt(curl.POST, 1)
curl.setopt(curl.HTTPPOST, [
('file', (curl.FORM_FILE, '/path/to/file'))
])
cURL and Apidog
If you are looking for an API tool that can work seamlessly with cURL APIs, then you are in luck!
Introducing to you Apidog - a comprehensive API development platform with complete tools for the entire API lifecycle. With Apidog, you can build, test, mock, and document APIs within a single application!
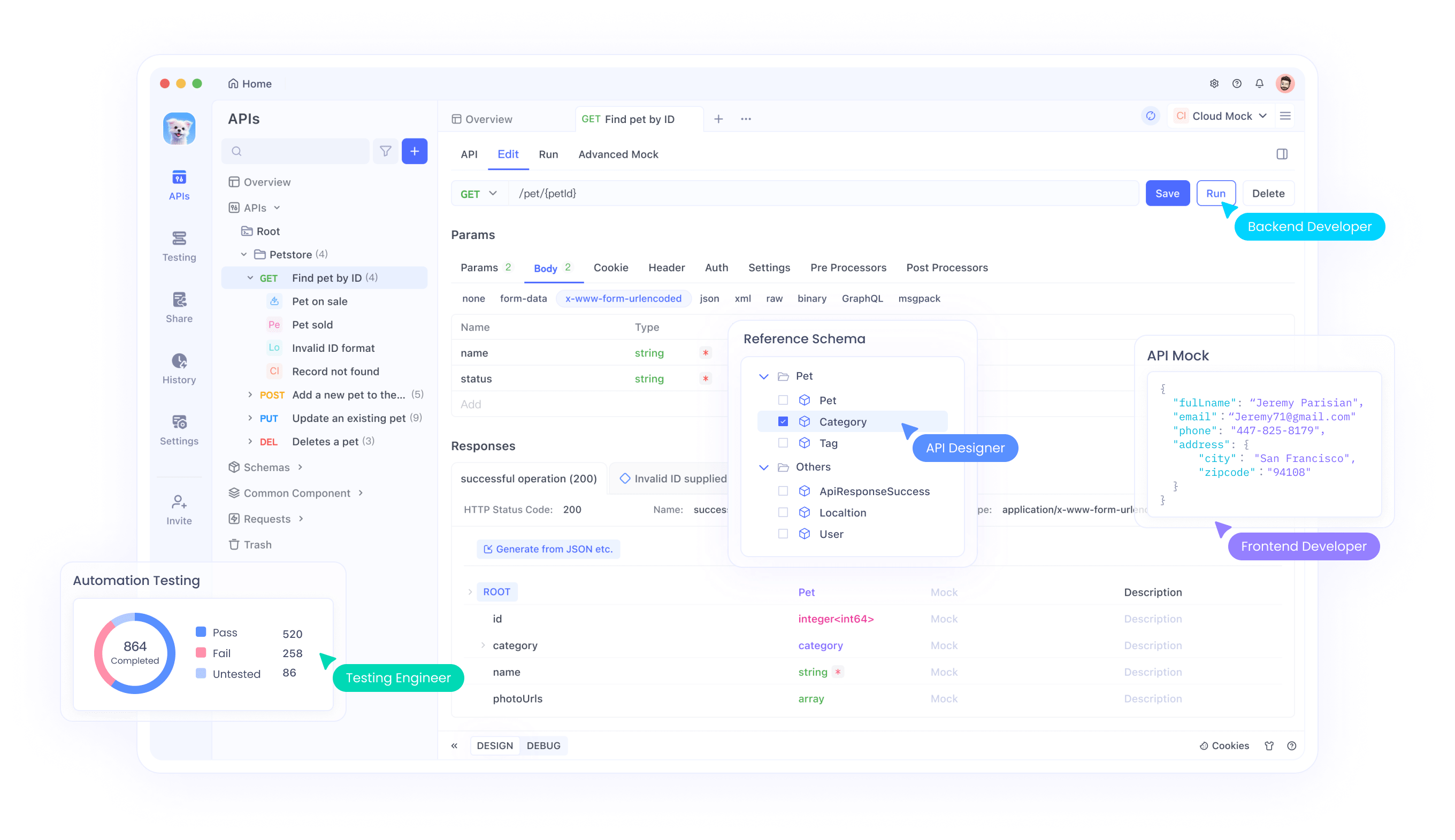
Start Working with cURL APIs by Importing them to Apidog
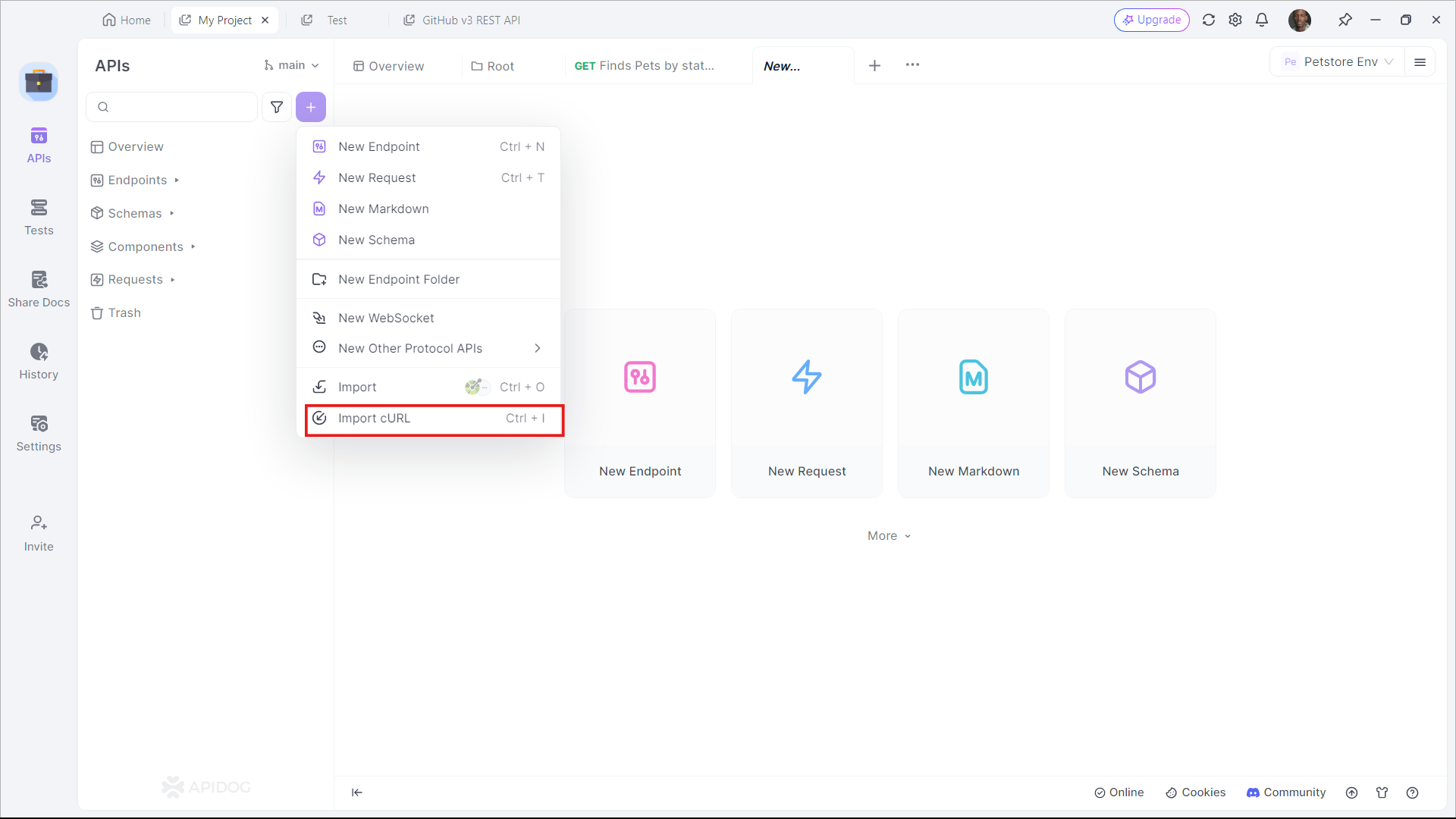
Apidog supports users who wish to import cURL commands to Apidog. In an empty project, click the purple +
button around the top left portion of the Apidog window, and select Import cURL
.
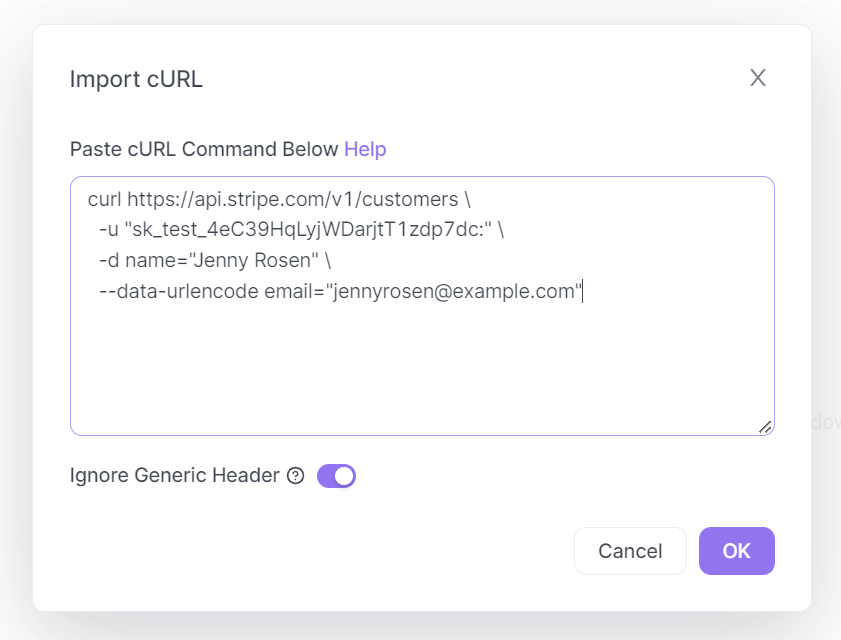
Copy and paste the cURL command into the box displayed on your screen.
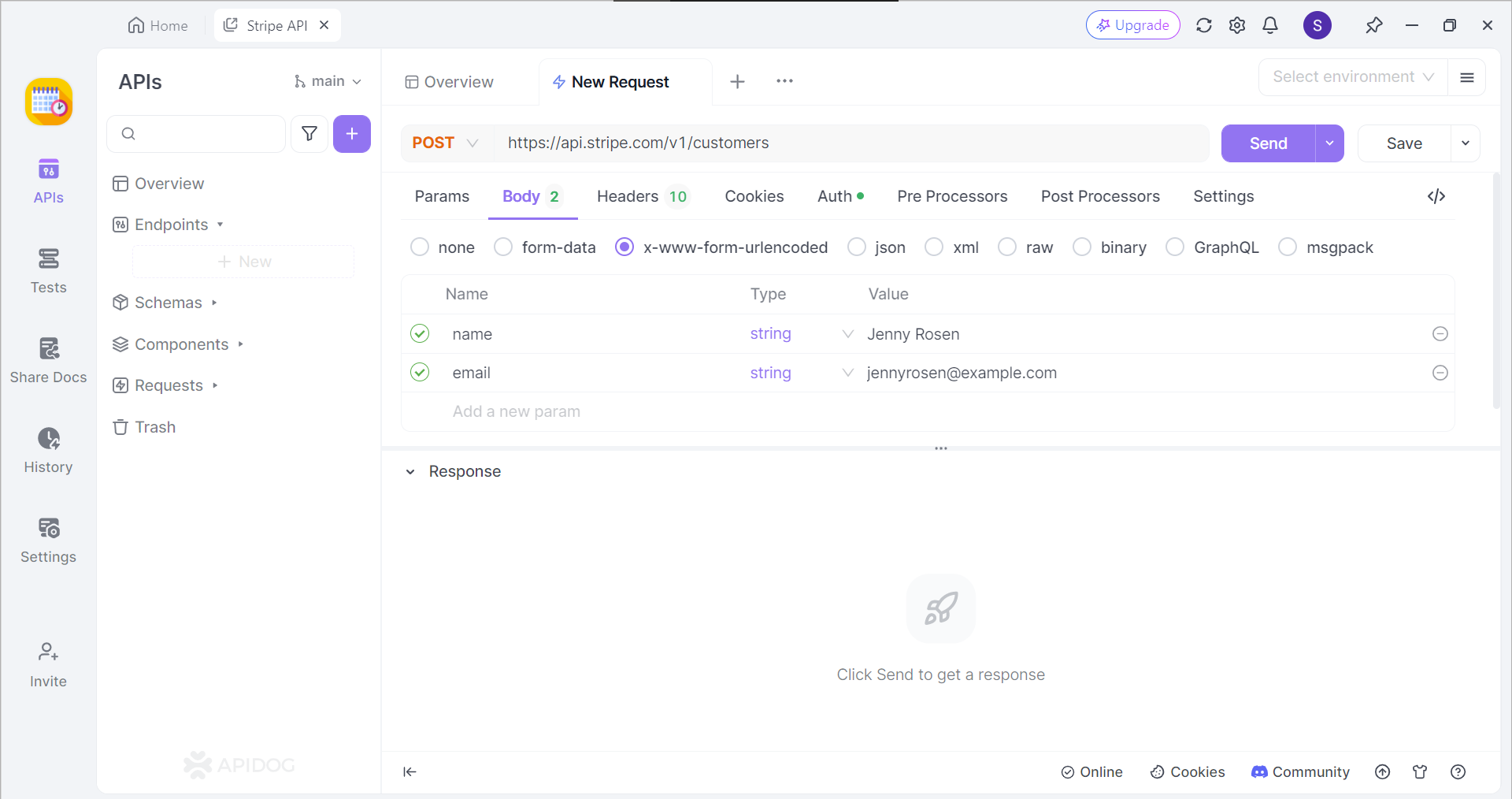
If successful, you should now be able to view the cURL command in the form of an API request.
Generate Python Code Instantly
Apidog can generate the necessary Pythoncode for your application within the blink of an eye.
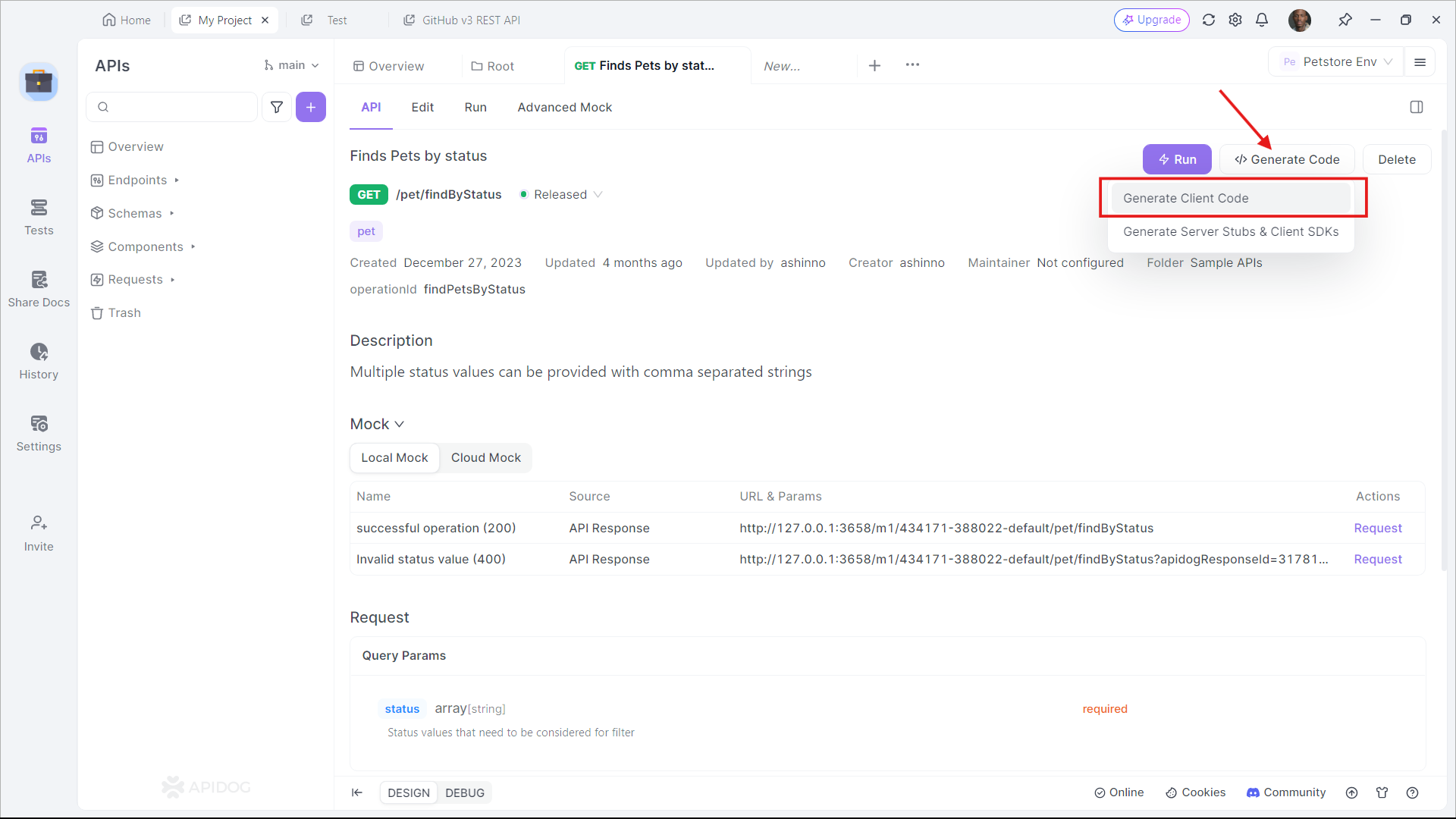
First, locate the </> Generate Code
button on any API or request, and select Generate Client Code
on the drop-down list.
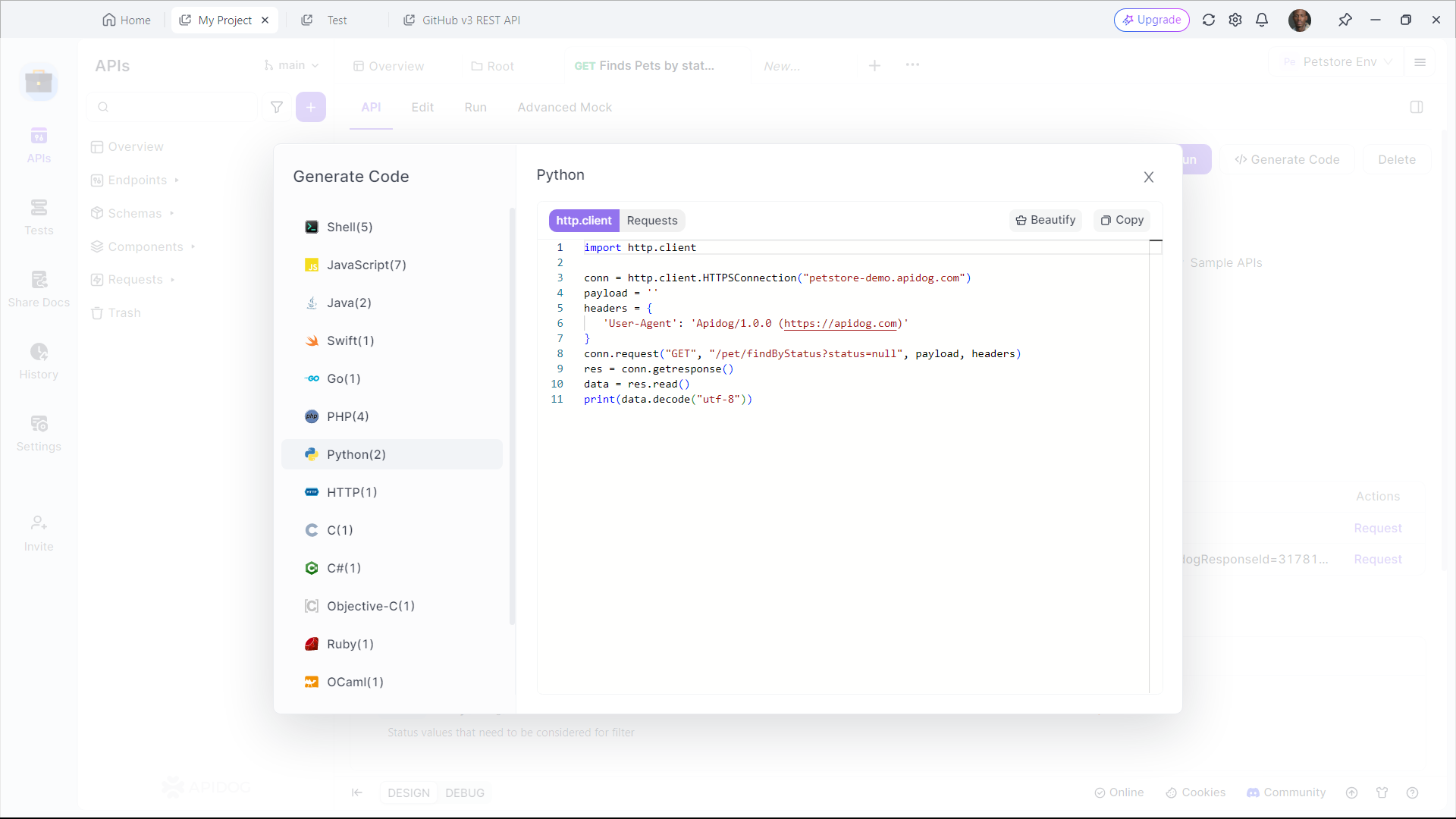
Next, select Python, and find the cURL section. You should now see the generated code for cURL. All you have to do is copy and paste it to your IDE (Integrated Development Environment) and continue developing your application.
Conclusion
Using cURL in Python opens up a world of possibilities for making API requests more efficient and powerful. Whether you're fetching data, sending POST requests, or handling file uploads, cURL provides a versatile and robust solution.
Remember, while cURL is powerful, it's always a good idea to explore tools that can simplify your workflow. Apidog is one such tool that can make your API development, testing, and documentation a breeze. Don’t forget to download Apidog for free and supercharge your API development!
By integrating cURL with Python, you can enhance your applications and streamline your development process. Happy coding!