How to Create and Test CRUD REST API
REST API provides a set of principles that guide the design of networked applications. You can create a CRUD REST API by following the steps in this article and document, mock, and test your API with Apidog.
In today’s age, managing data efficiently is highly important. Using CRUD with REST API ensures data operations are streamlined and standardized in modern web development. CRUD (Create, Read, Update, Delete) operations help data interact with the database.
On the other hand, REST (Representational State Transfer) API provides a set of principles that guide the design of networked applications. You can create a CRUD REST API by following the steps in this article and document, mock, and test your API with Apidog.
What is a CRUD API?
A CRUD API is an interface that supports the basic operations of Create, Read, Update, and Delete. It allows users or applications to interact with a database or data storage system by creating, retrieving, updating, and deleting records.
For example, a CRUD API for managing users might have endpoints like:
POST /api/users
: Create a new user.GET /api/users/:id
: Retrieve details of a specific user.PUT /api/users/:id
: Update all details of a specific user.PATCH /api/users/:id
: Update specific details of a user.DELETE /api/users/:id
: Delete a specific user.
How to create a CRUD REST API?
Creating a CRUD REST API involves several steps. We will be creating a simple project using Express.js. Follow all the steps carefully to create a RESTful API successfully.
Pre-requisites:
- You need to have Node.js installed on your machine. You can install it here.
- You need a code editor like Visual Studio Code on your system.
Step 1: Initialize a Node.js project
Open a terminal like a Command prompt or Terminal and type the following commands. mkdir will create your project’s directory, and npm init -y will initialize your project.
Step 2: Install the dependencies
Install the necessary packages for handling HTTP requests and for parsing requests.
Step 3: Create an Express.js app
Create a file named app.js in your project directory and add the following code.
Notice that we have not written our routes yet. We will do that in the next step.
Step 4: Setup your CRUD operations
Define your routes for handling CRUD operations. Put this code inside your app.js as well.
Step 5: Run your app
Now you can run your server.
If your app is created successfully, you can see something like this in your terminal.
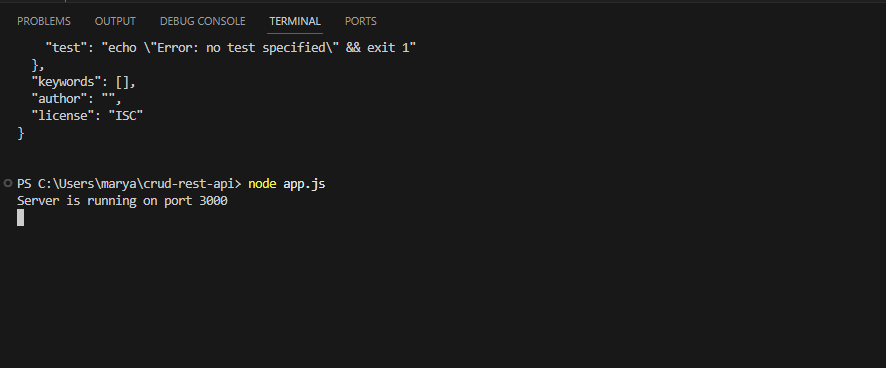
What is Apidog?
Apidog is a versatile API integration platform that simplifies API testing, debugging, design, mocking, and documentation processes. With a user-friendly interface and a rich set of tools, Apidog enhances collaboration within teams working on various projects.
It optimizes API testing, allowing for more thorough evaluations, and facilitates the creation of JSON/XML schemas for test results and responses.
Among its features, Apidog excels in documenting API responses and results, offering customizable layouts for more presentable documentation. The platform also boasts user-friendly API testing tools, enabling the visual addition of assertions and the creation of testing branches.
Collaborative efforts are streamlined through the option to share and work on projects with team members, simplifying the process of handling group APIs. Additionally, Apidog aids in monitoring API activity, and its mocking capabilities eliminate the need for scripting, providing a more efficient development experience.
How to Test your CRUD REST API with Apidog?
Now that you have created your application, you can test your API on Apidog. Apidog provides a graphical interface for creating requests, setting messages and parameters, saving requests, and connecting to API services. The steps to testing your API with Apidog are given below.
1. Create a new project in Apidog. You can name this project anything you like.
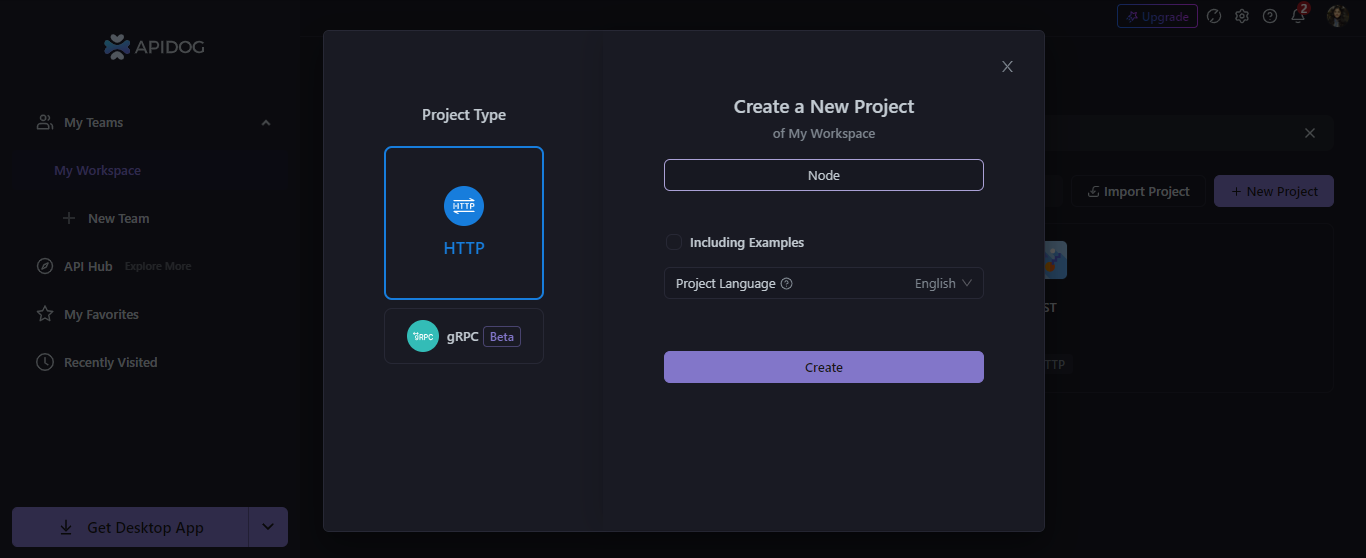
2. Once your project has been created, go to the API section and click on New API.
Click on the + sign next to the search bar to select what kind of API you want to test. In our case, we will be looking at the New API.
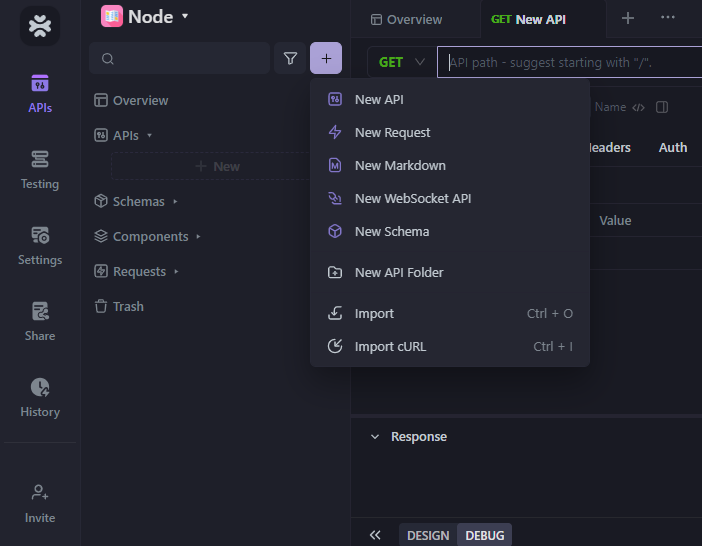
3. Make sure to set your environment according to your requirements. We will be working in a Development Environment for our example.
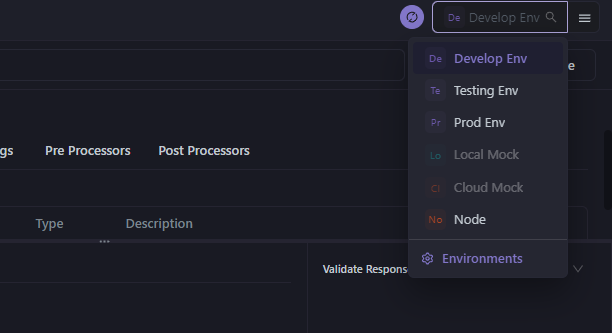
4. Enter your API and click on “Send”. You will be able to see all the responses in your results.
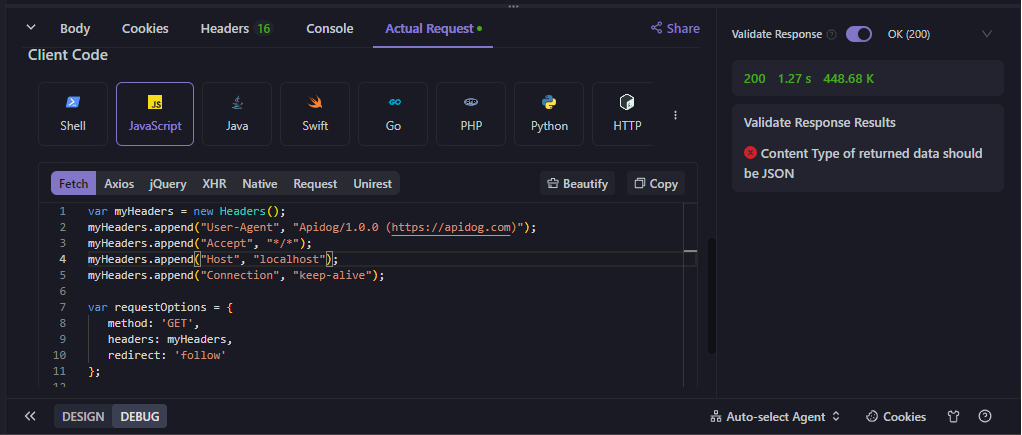
Benefits of using Apidog with CRUD REST API
There are several benefits that Apidog provides when used to test CRUD REST APIs.
API documentation:
Apidog provides tools for generating comprehensive and interactive API documentation for your CRUD REST APIs. It also provides clear and well-structured documentation for developers who want to understand how to use their APIs effectively.
Testing and Debugging:
Apidog offers several features for testing and debugging CRUD operations on your REST API. This can be particularly helpful during development and testing, allowing developers to identify and address issues more efficiently.
API exploration:
Tools like Apidog provides an interactive interface for exploring and interacting with different API endpoints. This can be useful for developers who want to experiment with various requests and understand the expected responses.
Code generation:
Some API tools, including Apidog, might offer code-generation capabilities. This can help developers by automatically generating code snippets in different programming languages based on the API documentation, making integrating the API into their applications easier.
Collaboration and Teamwork:
Apidog facilitates collaboration among team members by providing a centralized platform for API documentation, testing, and discussion. This can improve communication and coordination among developers working on different aspects of the API.
Versioning:
If Apidog includes versioning and change management features, it can assist in keeping track of changes to the API over time. This is important for maintaining backward compatibility and ensuring a smooth transition for API consumers when updates are made.
Security and access control:
Apidog offers features related to API security, such as access control and authentication. This can help manage who has access to the API and enforce security best practices.
Monitoring and Analytics:
Some API tools include monitoring and analytics features that allow developers to track API usage, identify performance issues, and gain insights into how the API is utilized.
Conclusion
In conclusion, developing a CRUD REST API is fundamental to modern web application development. The Create, Read, Update, and Delete operations form the backbone of data management, enabling seamless communication between clients and servers.
By adhering to the RESTful principles, developers ensure a scalable, stateless, and uniform interface for their APIs. As we navigate web development, the importance of well-designed CRUD REST APIs becomes increasingly evident. To make our APIs better, we need to test them thoroughly, and Apidog helps in this regard. It not only tests APIs but also provides clear documentation, analysis and debugging.