Mastering AJAX PUT Requests: The Key to Efficient API Data Updates
Discover the power of AJAX PUT requests and how they revolutionize data updates in APIs. Learn to create efficient client-side updates, handle responses, and implement best practices for smooth integrations.
In the ever-evolving landscape of web development, mastering the art of AJAX PUT requests stands as a cornerstone for developers seeking to ensure seamless data updates. This blog post delves into the intricacies of AJAX PUT requests, a critical component of the modern API-driven web. We’ll explore how these requests empower developers to efficiently update data on the server without reloading the entire page, leading to a more dynamic and responsive user experience.
Understanding AJAX and its Power
Before we delve into the specifics of PUT requests, let's take a moment to appreciate the beauty of AJAX (Asynchronous JavaScript and XML). AJAX is a set of web development techniques that allows web applications to send and retrieve data from a server asynchronously, without the need for a full page refresh.
This means that you can update specific portions of a web page dynamically, providing a smooth and seamless user experience. No more waiting for entire pages to reload – AJAX enables lightning-fast updates and interactions.
The HTTP Methods: A Brief Overview
In the world of web development, we rely on HTTP (Hypertext Transfer Protocol) to communicate between clients (e.g., web browsers) and servers. HTTP defines a set of methods, or "verbs," that specify the type of action to be performed on a given resource (e.g., a web page, an API endpoint).
The most common HTTP requests are:
- GET: Used to retrieve data from a server
- POST: Used to submit new data to a server
- PUT: Used to update an existing resource on the server
- DELETE: Used to remove a resource from the server
Today, our focus is on the mighty PUT method and how it can be utilized with AJAX to update data in APIs efficiently.
The Power of AJAX PUT Requests
When it comes to updating data in APIs, AJAX PUT requests are a true game-changer. Unlike traditional form submissions, which typically involve a full page refresh, AJAX PUT requests allow you to update specific data points without disrupting the user experience.
Here's how it works:
- Gather the Data: First, you'll need to collect the updated data that you want to send to the API. This could come from form fields, user input, or any other source within your web application.
- Create the AJAX Request: Next, you'll use JavaScript to create an AJAX PUT request to the API endpoint responsible for updating the data. This typically involves constructing an XMLHttpRequest object or using a library like jQuery or Axios.
- Send the Data: Once you've constructed the AJAX request, you can include the updated data in the request body (typically in JSON format) and send it to the API endpoint.
- Handle the Response: After the server processes the request, it will send back a response, which you can handle in your JavaScript code. This response could indicate success, failure, or provide additional data or instructions.
// Function to gather data and send a PUT request
function updateData(apiEndpoint, updatedData) {
// Create a new XMLHttpRequest object
var xhr = new XMLHttpRequest();
// Configure it: PUT-request for the URL /api/endpoint
xhr.open('PUT', apiEndpoint, true);
// Set up the request headers
xhr.setRequestHeader('Content-Type', 'application/json');
// Define what happens on successful data submission
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
// Parse the JSON response
var response = JSON.parse(xhr.responseText);
console.log('Success:', response);
} else {
// Handle errors if the server response was not ok
console.error('The request failed!');
}
};
// Define what happens in case of an error
xhr.onerror = function () {
console.error('The request encountered an error.');
};
// Convert the data to JSON and send it
xhr.send(JSON.stringify(updatedData));
}
// Example usage:
// Assuming you have an API endpoint '/api/update-user' and updated user data
var apiEndpoint = '/api/update-user';
var userData = {
id: '123',
name: 'Alice',
email: 'alice@example.com'
};
// Call the function to update the data
updateData(apiEndpoint, userData);
This code snippet demonstrates the process of sending a PUT request to an API endpoint. It includes gathering the data, creating the AJAX request, sending the data, and handling the response. Remember to replace apiEndpoint
and userData
with your actual API endpoint and the data you wish to update.
By leveraging AJAX PUT requests, you can seamlessly update data in your APIs without causing any disruption to the user's workflow or experience. No more full-page reloads or jarring transitions – just smooth, efficient data updates.
Test Your Ajax Put Request with Apidog
Apidog is an all-in-one API collaboration platform that provides comprehensive tools for API documentation, API debugging, API mocking, and API automated testing. It’s designed to address the challenges of data synchronization across different systems by using a single system and centralized data.
To create and test an AJAX PUT request using Apidog, you can follow these steps:
- Open Apidog and create a new request.
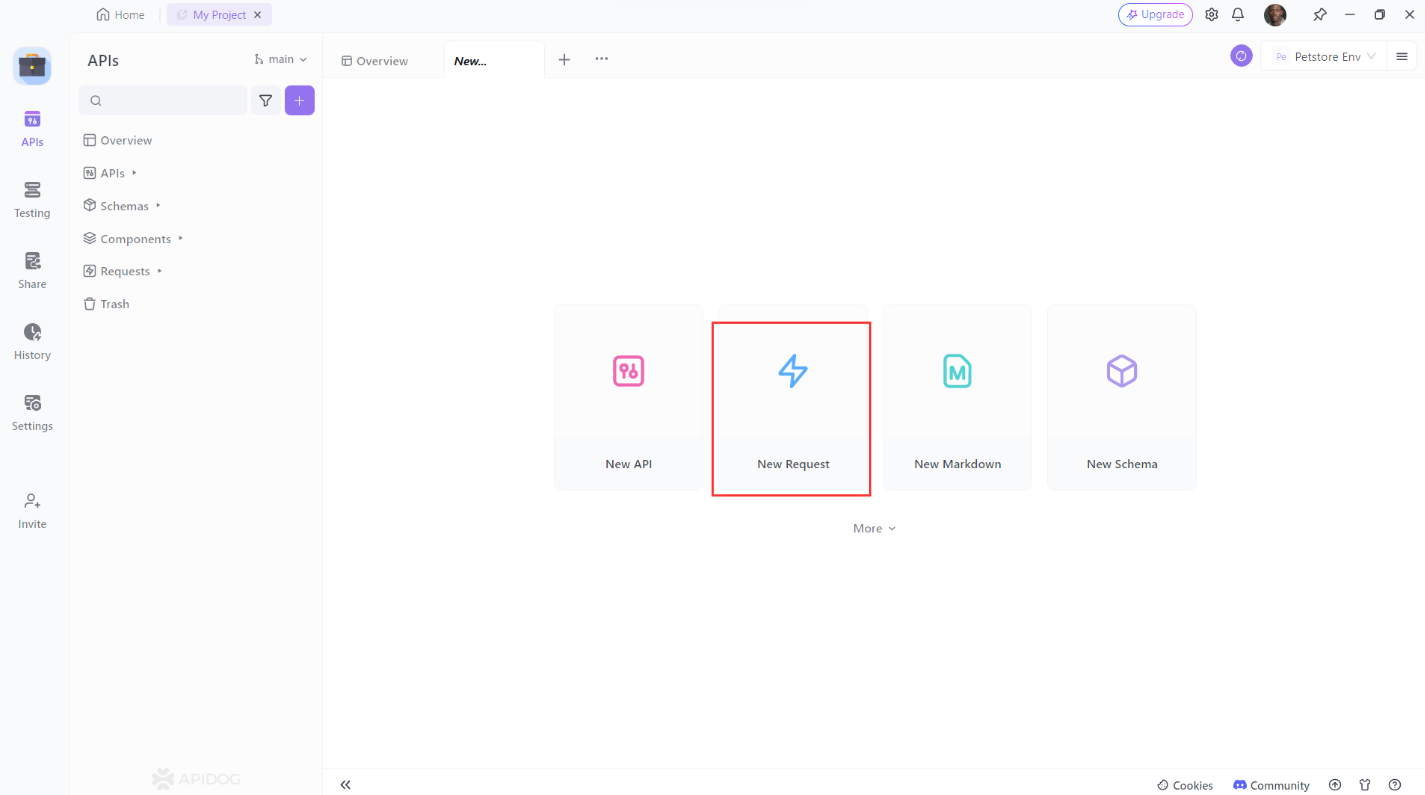
2. Choose PUT as the HTTP method for your request.
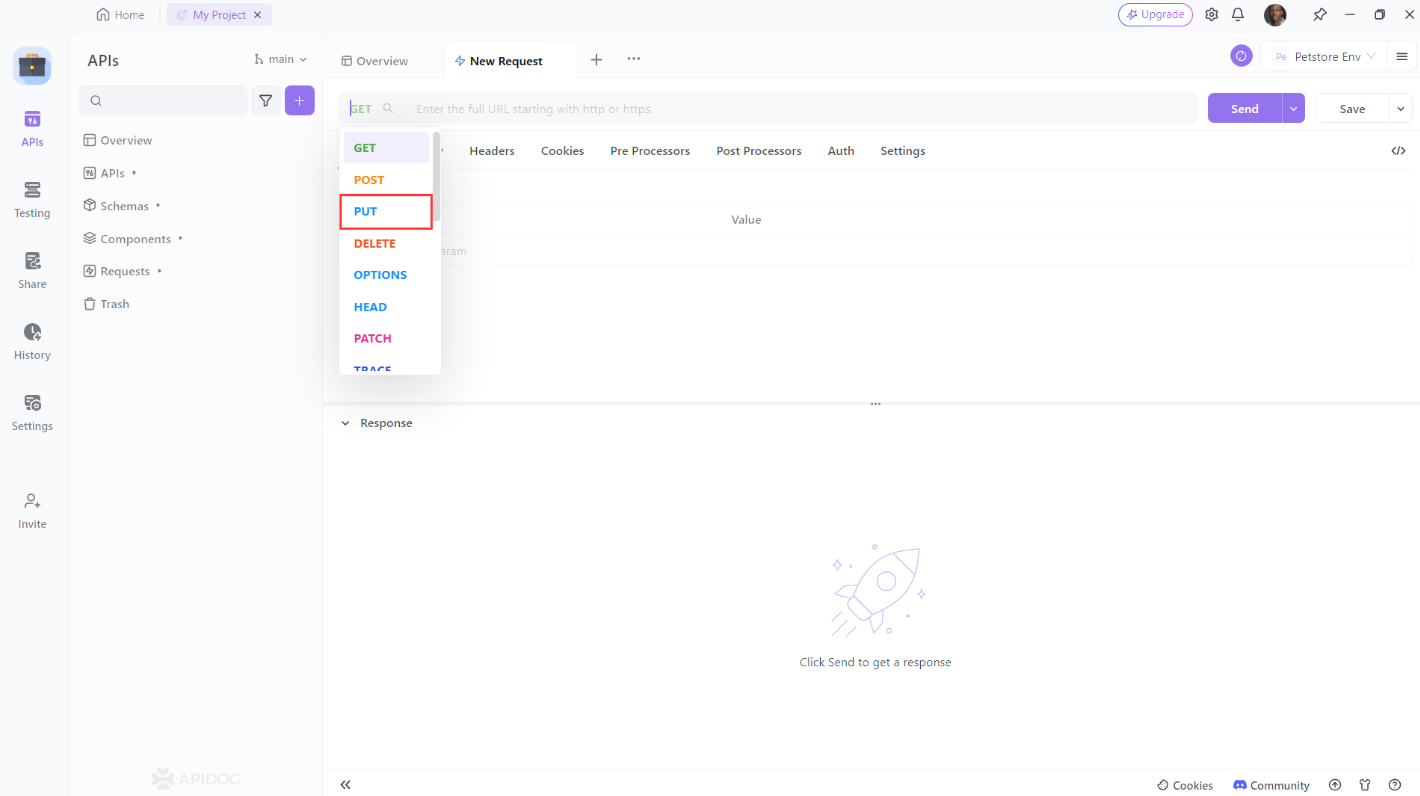
3. Fill in the necessary request details such as the URL and parameters. Apidog will automatically identify the parameters contained in the URL and display them in the request parameter list. Then click on ‘Send’ to execute the PUT request.
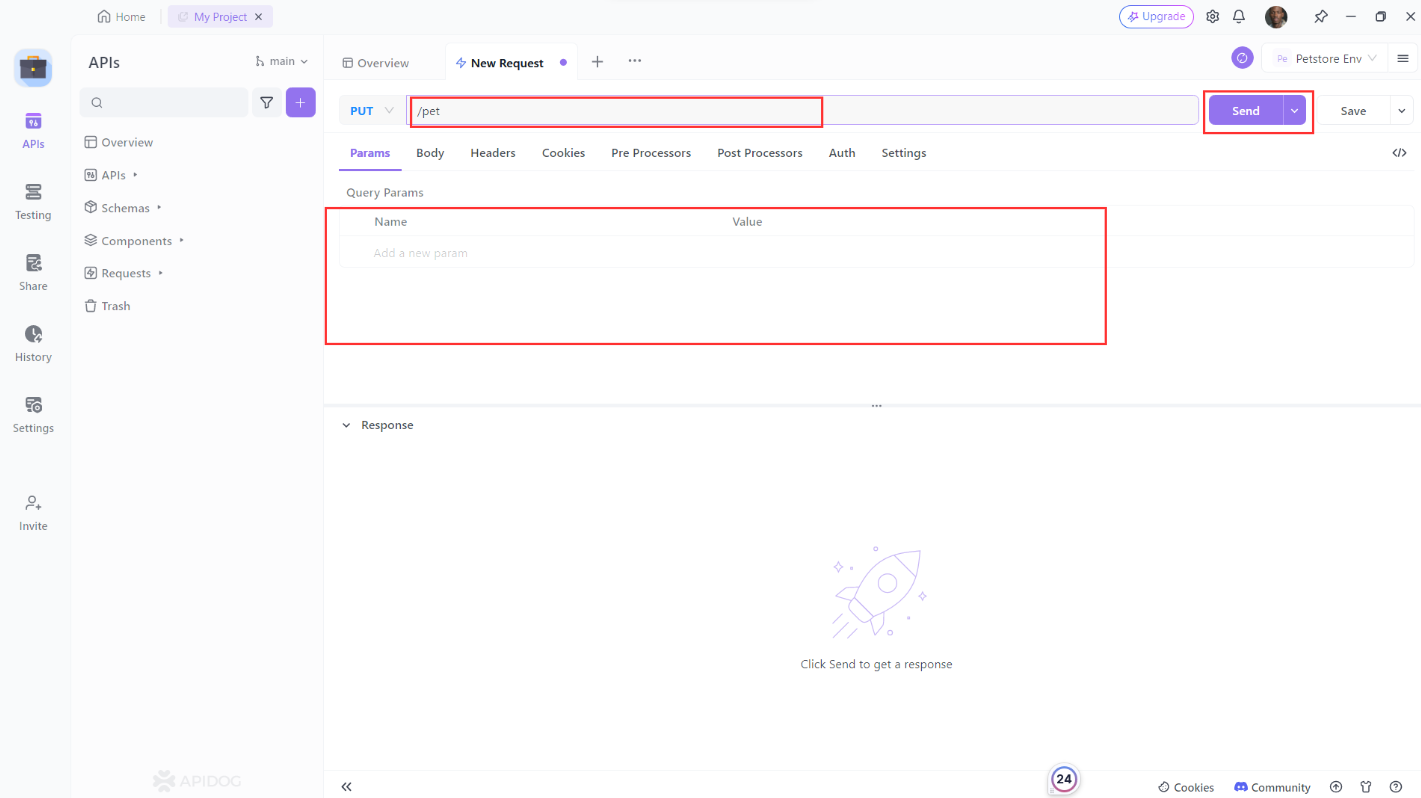
4. Verify that the response is what you expected.
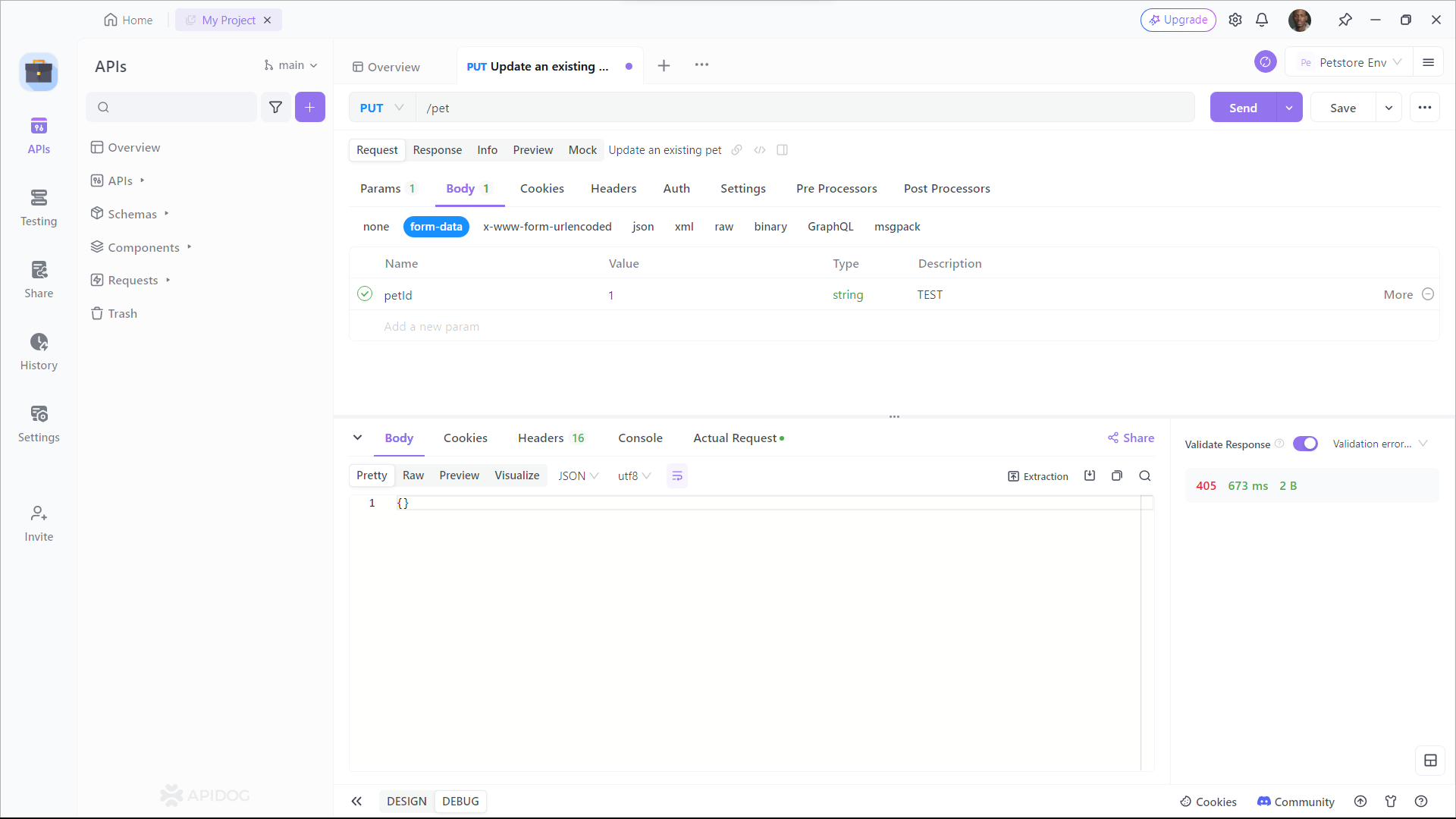
Best Practices and Tips for AJAX PUT
While AJAX PUT requests are incredibly powerful, it's essential to follow best practices to ensure smooth and secure data updates:
- Validate Data: Before sending data to the API, validate it on the client-side to prevent potential security vulnerabilities or data corruption.
- Handle Errors Gracefully: Implement proper error handling mechanisms to gracefully handle API errors, network issues, or other potential problems that may arise during the data update process.
- Implement Authentication and Authorization: Depending on your API's requirements, you may need to include authentication and authorization mechanisms (e.g., API keys, JWT tokens) in your AJAX PUT requests to ensure secure access to protected resources.
- Optimize for Performance: Consider techniques like data compression, caching, and minimizing payload sizes to optimize the performance of your AJAX PUT requests, especially in scenarios where large amounts of data need to be updated.
- Test Thoroughly: Thoroughly test your AJAX PUT request implementations across different browsers, devices, and scenarios to ensure consistent and reliable behavior.
Conclusion:
Embracing AJAX PUT requests is not merely about data manipulation; it’s about harnessing the power of APIs to create fluid, intuitive web applications. Platforms like Apidog streamline this process, offering tools that simplify the creation, testing, and management of APIs.
By mastering AJAX PUT requests and utilizing comprehensive platforms such as Apidog, developers can ensure efficient data updates, leading to a robust and seamless user experience. As we continue to push the boundaries of web development, tools like Apidog will be at the forefront, guiding us towards a more connected and dynamic digital world.