How to post form data with aiohttp ?
Discover the simplicity of sending form data with `aiohttp` in our latest post. Learn how to leverage asynchronous requests in Python for faster, more efficient web applications. Dive into our easy-to-follow guide and elevate your coding skills today!
The ability to handle HTTP requests efficiently is crucial. This guide shines a light on aiohttp
, a robust Python library that excels in managing asynchronous HTTP communications. We’ll delve into the specifics of crafting HTTP POST requests to transmit form data, setting up aiohttp
, and adhering to best practices for creating secure and high-performing web applications. Whether you’re an experienced developer or just starting out, this article will provide you with the tools and knowledge to effectively utilize aiohttp
for your projects, ensuring seamless API interactions and top-notch performance.
What is aiohttp?
aiohttp is an asynchronous HTTP client/server framework written in Python. It leverages the asyncio
library to enable non-blocking network communication, which is particularly useful for handling multiple requests concurrently without slowing down the server. Here are some key features of aiohttp
:
- Asynchronous Support: It supports asynchronous request handling, making it suitable for high-performance web applications.
- Client and Server:
aiohttp
can be used to build both client applications (making HTTP requests) and servers (handling HTTP requests). - WebSockets: It provides support for both client and server WebSockets out-of-the-box, allowing for real-time two-way communication.
- Middlewares and Signals: The server component of
aiohttp
includes middlewares and signals for request processing and response handling. - Pluggable Routing: It allows for flexible routing of HTTP requests to different handlers based on the URL.
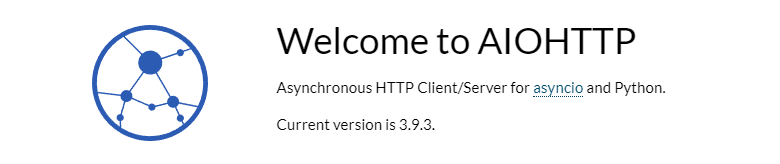
aiohttp
is designed to handle the complexities of modern web services and is a popular choice for developers looking to create scalable and responsive applications.
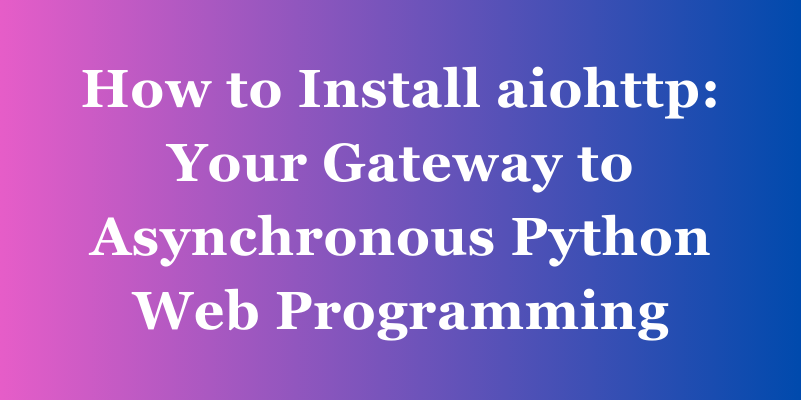
What is a POST Request?
A POST request is used to send data to a server to create or update a resource. The data is included in the body of the request, which allows for more extensive and complex data to be transmitted compared to a GET request.
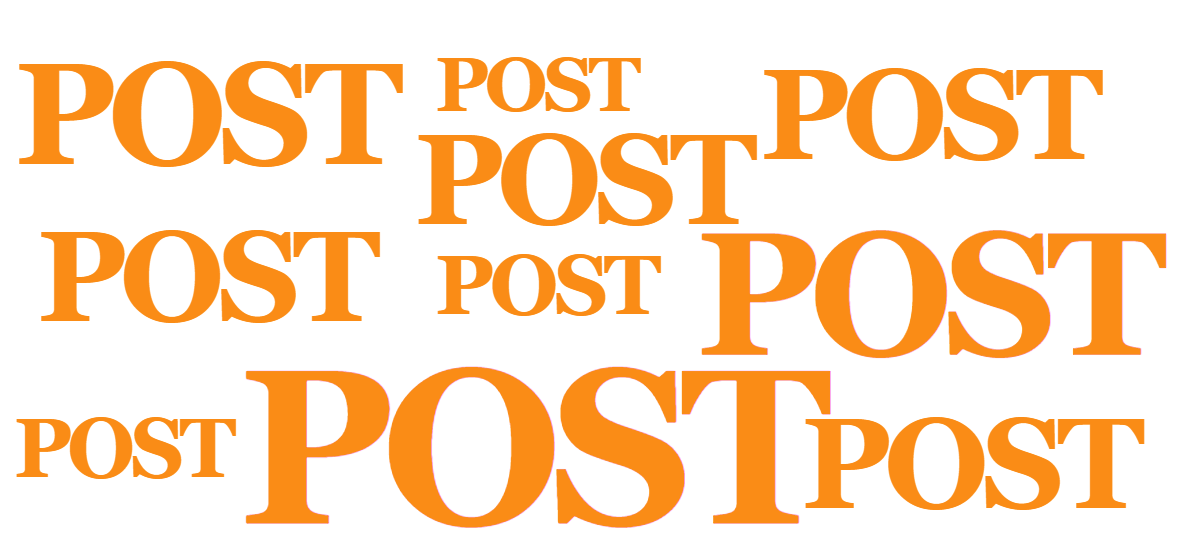
Structure of an HTTP POST Request
An HTTP POST request consists of:
- Start-line: Includes the request method (POST), the request target (URL or path), and the HTTP version.
- Headers: Provide metadata about the request, such as
Content-Type
andContent-Length
. - Empty line: Separates headers from the body.
- Body: Contains the data to be sent to the server.
Here’s a basic example:
POST /path/resource HTTP/1.1
Host: example.com
Content-Type: application/x-www-form-urlencoded
Content-Length: length
field1=value1&field2=value2
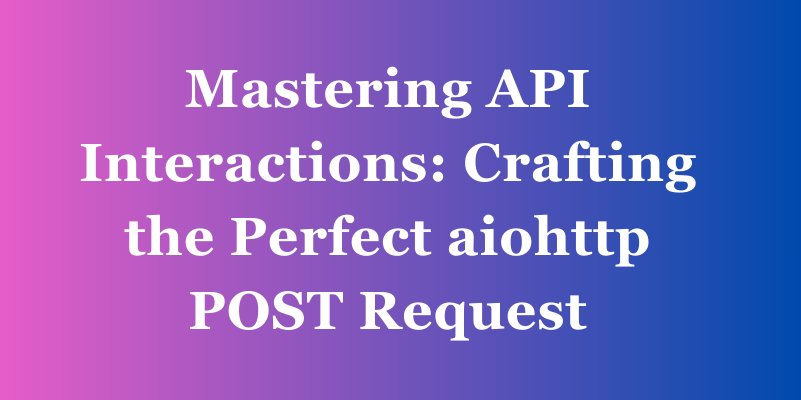
How to post form data in aiohttp
Posting form data using aiohttp
in Python involves creating a FormData
object, adding fields to it, and then sending it with a POST request. Here’s a step-by-step guide:
Import aiohttp and FormData:
import aiohttp
from aiohttp import FormData
Create an instance of FormData and add fields:
data = FormData()
data.add_field('field_name', 'field_value')
# Repeat for each field you want to add
Create a ClientSession and send the POST request:
async def send_post_request():
url = 'your_endpoint_url'
async with aiohttp.ClientSession() as session:
async with session.post(url, data=data) as response:
# Process the response
response_data = await response.text()
return response_data
Run the asynchronous function:If you’re running this code outside of an existing event loop, you’ll need to use asyncio.run()
:
import asyncio
asyncio.run(send_post_request())
Remember to replace 'your_endpoint_url'
, 'field_name'
, and 'field_value'
with the actual URL and form data you intend to send. This is a basic example, and you might need to handle headers, authentication, and other aspects depending on your specific use case.
How to test aiohttp post request with apidog
Testing an aiohttp
POST request with Apidog involves a few steps to ensure that your API is functioning correctly.
Here is how you can use Apidog to test your aiohttp POST request:
- Open Apidog and create a new request.
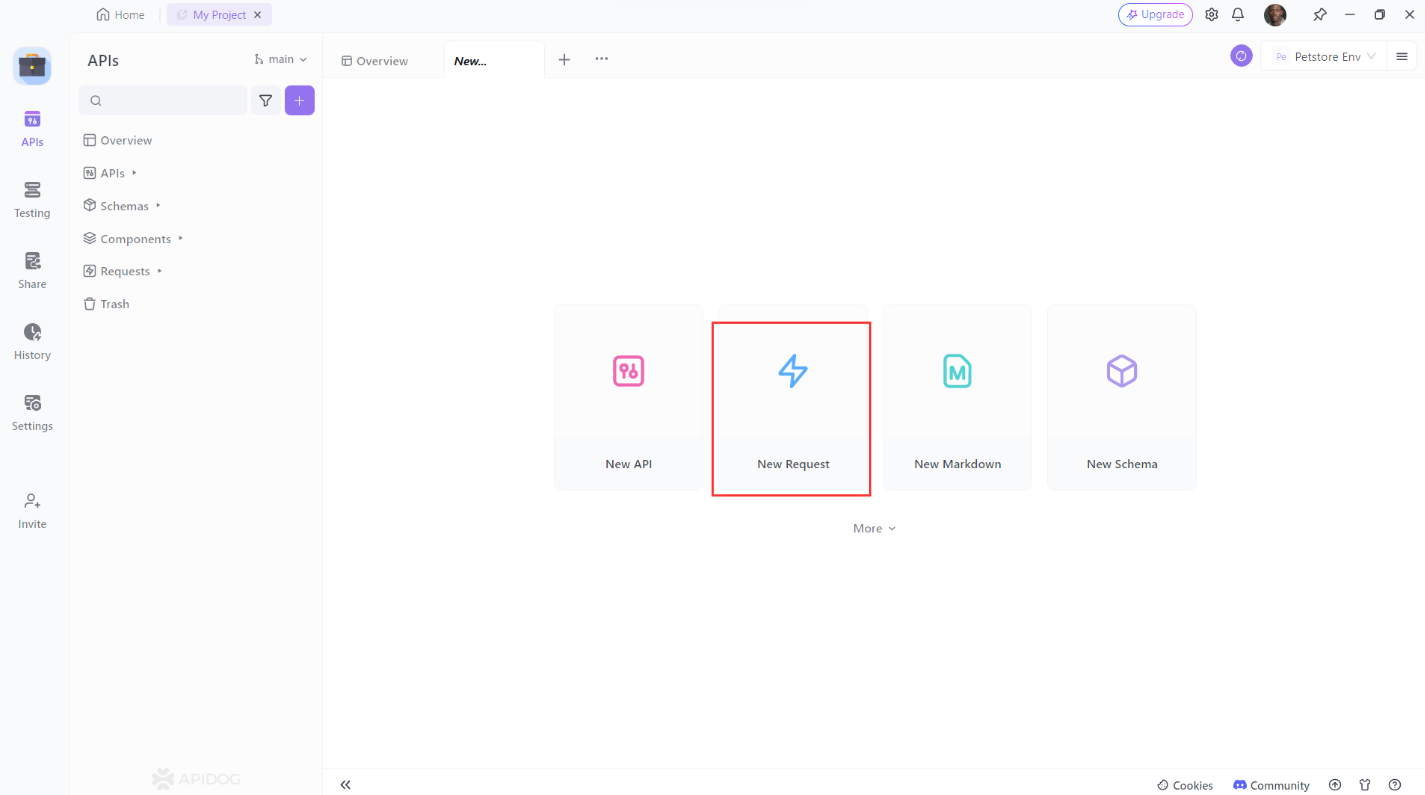
2. Set the request method to POST.
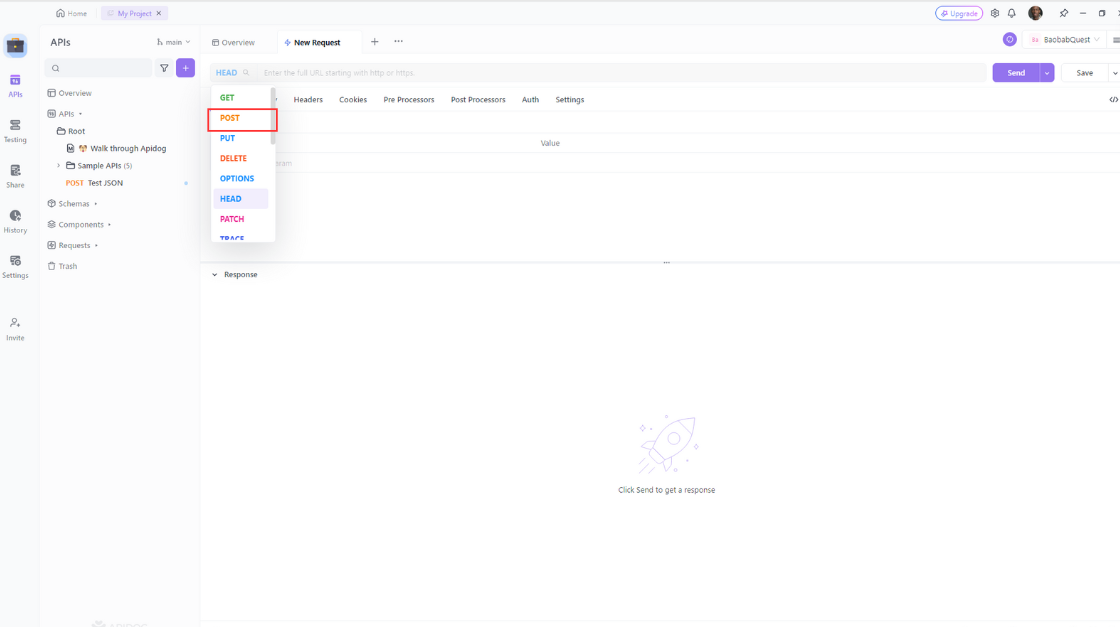
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
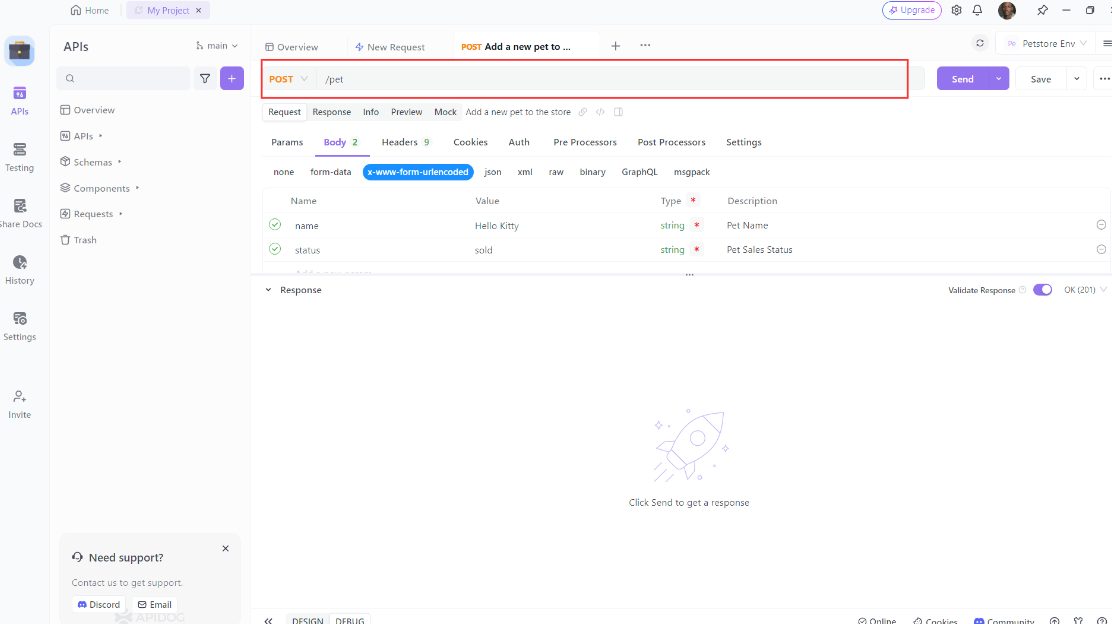
4. Verify that the response is what you expected.
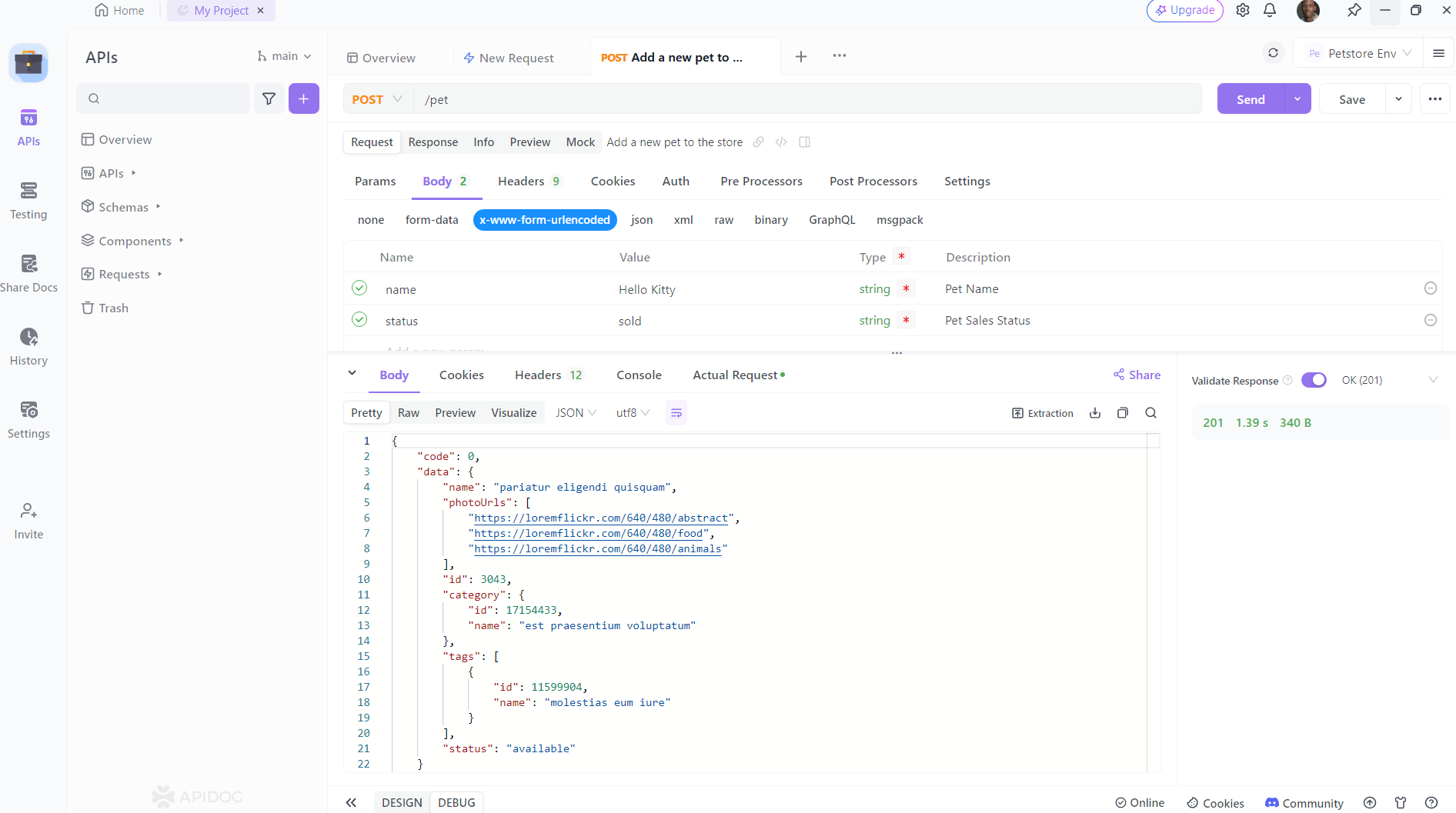
Best Practices for aiohttp POST form data Requests
When working with aiohttp
and POST requests, it’s important to adhere to best practices to ensure security, optimize performance, and maintain clean code.
Ensuring Security and Privacy
- SSL/TLS: Always use
https
to encrypt data in transit. - Session Management: Use
ClientSession
to manage cookies and headers securely. - Input Validation: Validate and sanitize input to prevent injection attacks.
- Error Handling: Implement proper error handling to avoid exposing sensitive information.
Optimizing Performance
- Connection Pooling: Reuse connections with
ClientSession
to reduce latency. - Asynchronous Operations: Leverage
async
andawait
to handle I/O operations without blocking. - Concurrent Requests: Use
asyncio.gather
to make concurrent requests and improve throughput. - Resource Management: Use context managers to ensure resources like sessions are properly closed.
Writing Clean and Maintainable Code
- Code Structure: Organize code with functions and classes to improve readability.
- Comments and Documentation: Comment your code and maintain up-to-date documentation.
- Consistent Style: Follow PEP 8 or other style guides for consistent code formatting.
- Version Control: Use version control systems like Git to track changes and collaborate.
By following these practices, you can create aiohttp
POST requests that are secure, efficient, and easy to maintain. Remember, the key to successful implementation is continuous learning and adapting to new patterns and practices as they emerge in the community.
Conclusion
APIs are the backbone of modern software development, bridging the gap between different systems to enable seamless collaboration. In the Python ecosystem, the aiohttp
library emerges as a pivotal tool for asynchronous HTTP request handling, paving the path for the creation of efficient and scalable web applications. A critical aspect of this is mastering HTTP POST requests to send form data, which, when coupled with best practices and thorough testing using tools like Apidog, forms the foundation of sturdy API development. As the technological landscape advances, proficiency in these areas becomes indispensable for devising cutting-edge solutions and maintaining a competitive edge.