Bolster API Security: Mastering AIOHTTP Authentication
APIs are the backbone of modern web applications, but security breaches can wreak havoc. This article dives into AIOHTTP authentication, a powerful tool for securing your APIs. Explore common methods like token-based authentication and explore best practices to safeguard your data and user privacy.
In today's web-driven world, APIs (Application Programming Interfaces) act as the connective tissue between various applications, allowing them to share data and functionality. However, with this convenience comes a critical responsibility: ensuring the security of these APIs. Breaches can expose sensitive data and disrupt core functionalities, causing significant damage.
If you want to apply the proper authentication for your AIOHTTP-based APIs or applications, you can use Apidog to implement the necessary authentication type.
Apidog has countless other useful functionalities that you can utilize for your project's developments - so try them out for free today by clicking the button below! 👇 👇 👇
This article delves into AIOHTTP authentication, a robust toolkit within the AIOHTTP framework that empowers developers to safeguard their APIs. We'll explore various authentication methods and best practices to effectively secure your APIs and prevent unauthorized access.
But before we dive deeper into what AIOHTTP Authentication is, let's review on the individual terms we can find from the phrase that this article revolves around.
Definition of AIOHTTP
AIOHTTP is a Python library designed for building asynchronous HTTP clients and servers, leveraging the power of asyncio, another python library, to handle multiple requests concurrently.
Definition of Authentication
Authentication, in the context of APIs, is the process of verifying the identity of a user or application trying to accesss an API.
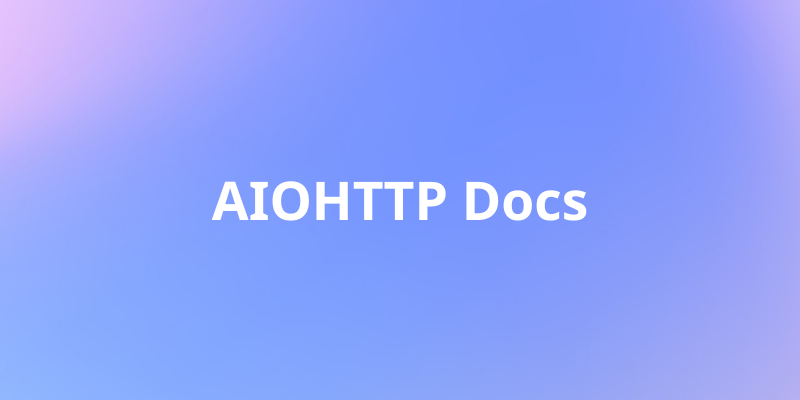
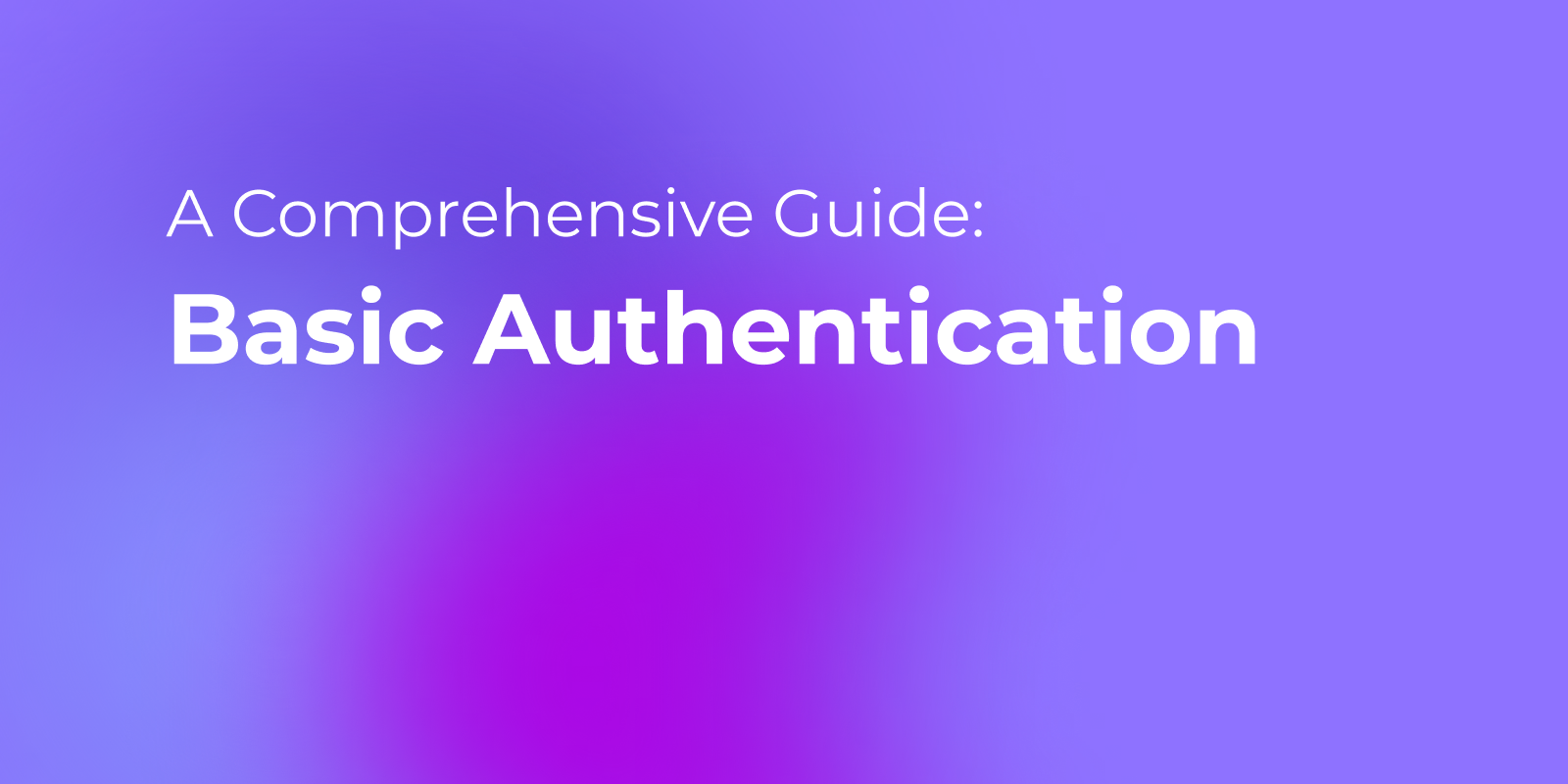
What is AIOHTTP Authentication?
When put together, AIOHTTP authentication refers to the collection of methods and functionalities within the AIOHTTP framework, enabling developers to implement authentication mechanisms for their web applications' APIs.
These mechanisms verify the identity of users or applications that are trying to access API resources, ensuring only authorized access and enhancing overall API security.
Key Aspects of AIOHTTP Authentication
1. Authentication Methods:
AIOHTTP offers support for various authentication methods, providing flexibility to choose the most suitable one for your application's needs. Here are some common methods:
- Basic Authentication: A basic access credential (username and password) is encoded within the request header for verification by the server. This method is considered less secure due to credential transmission in plain text.
- API Key Authentication: A unique API key is provided with the request, often as a header or query parameter. The server validates the key against a predefined list to grant access. This method is simpler to implement but requires careful API key management.
- Token-Based Authentication (JWT, OAuth): JSON Web Tokens (JWT) or protocols like OAuth are used to generate tokens that encapsulate user information and permissions. These tokens are included with the request for verification by the server. This method offers greater security and scalability.
2. Integration with AIOHTTP:
AIOHTTP provides middleware components that seamlessly integrate authentication into your application's workflow. These middleware functions intercept incoming requests, handle authentication logic (verifying credentials or tokens), and grant or deny access based on the outcome.
3. Credential Storage: Credentials (usernames, passwords, API keys) should be securely stored outside of your application code. AIOHTTP doesn't dictate storage mechanisms, but common practices include environment variables, secure configuration files, or dedicated secret management services.
4. User Permissions: AIOHTTP authentication can be integrated with your authorization system to define different permission levels for users. This allows granular control over what data and functionalities users can access within your API.
5. Security Best Practices:
- HTTPS Everywhere: Always use HTTPS for API communication to encrypt data transmission and prevent man-in-the-middle attacks.
- Secure Credential Storage: Implement robust measures to protect sensitive credentials like API keys and access tokens.
- Regular Security Audits: Conduct regular security audits to identify and address any vulnerabilities in your authentication implementation.
- Stay Updated: Keep the AIOHTTP library and any depencies udpated to benefit from security patches and improvements.
Coding Examples for AIOHTTP Authentication
1. Basic Authentication
The code example shows basic authentication using a username and password.
import aiohttp
async def basic_auth_request(username, password, url):
async with aiohttp.ClientSession(auth=aiohttp.BasicAuth(username, password)) as session:
async with session.get(url) as response:
if response.status == 200:
data = await response.json()
print(data)
else:
print(f"Error: {response.status}")
# Example usage
username = "your_username"
password = "your_password"
url = "https://api.example.com/protected_resource"
aiohttp.run(basic_auth_request(username, password, url))
2. API Key Authentication
The code example below showcases sending an API key in the request header.
import aiohttp
async def api_key_request(api_key, url):
headers = {"Authorization": f"Bearer {api_key}"}
async with aiohttp.ClientSession() as session:
async with session.get(url, headers=headers) as response:
if response.status == 200:
data = await response.json()
print(data)
else:
print(f"Error: {response.status}")
# Example usage
api_key = "your_api_key"
url = "https://api.example.com/data"
aiohttp.run(api_key_request(api_key, url))
3. Token-based Authentication (JWT)
The code example below shows sending a JWT token in the request header.
import aiohttp
import jwt
async def jwt_auth_request(token, url):
headers = {"Authorization": f"Bearer {token}"}
async with aiohttp.ClientSession() as session:
async with session.get(url, headers=headers) as response:
if response.status == 200:
data = await response.json()
print(data)
else:
print(f"Error: {response.status}")
# Example usage (assuming you have a JWT generation function)
token = generate_jwt_token(user_id=123)
url = "https://api.example.com/private"
aiohttp.run(jwt_auth_request(token, url))
Apidog - Strengthening API Security by Selecting Authentication Type
A must-know piece of knowledge for all API developers is implementing an authentication type for their APIs. API authentication can be implemented to prevent API exploitation or abuse, and to provide such a a vital component to your APIs, all you need to do is download Apidog, a one-stop solution to your API security problems.
Setting Up API Authentication On Apidog
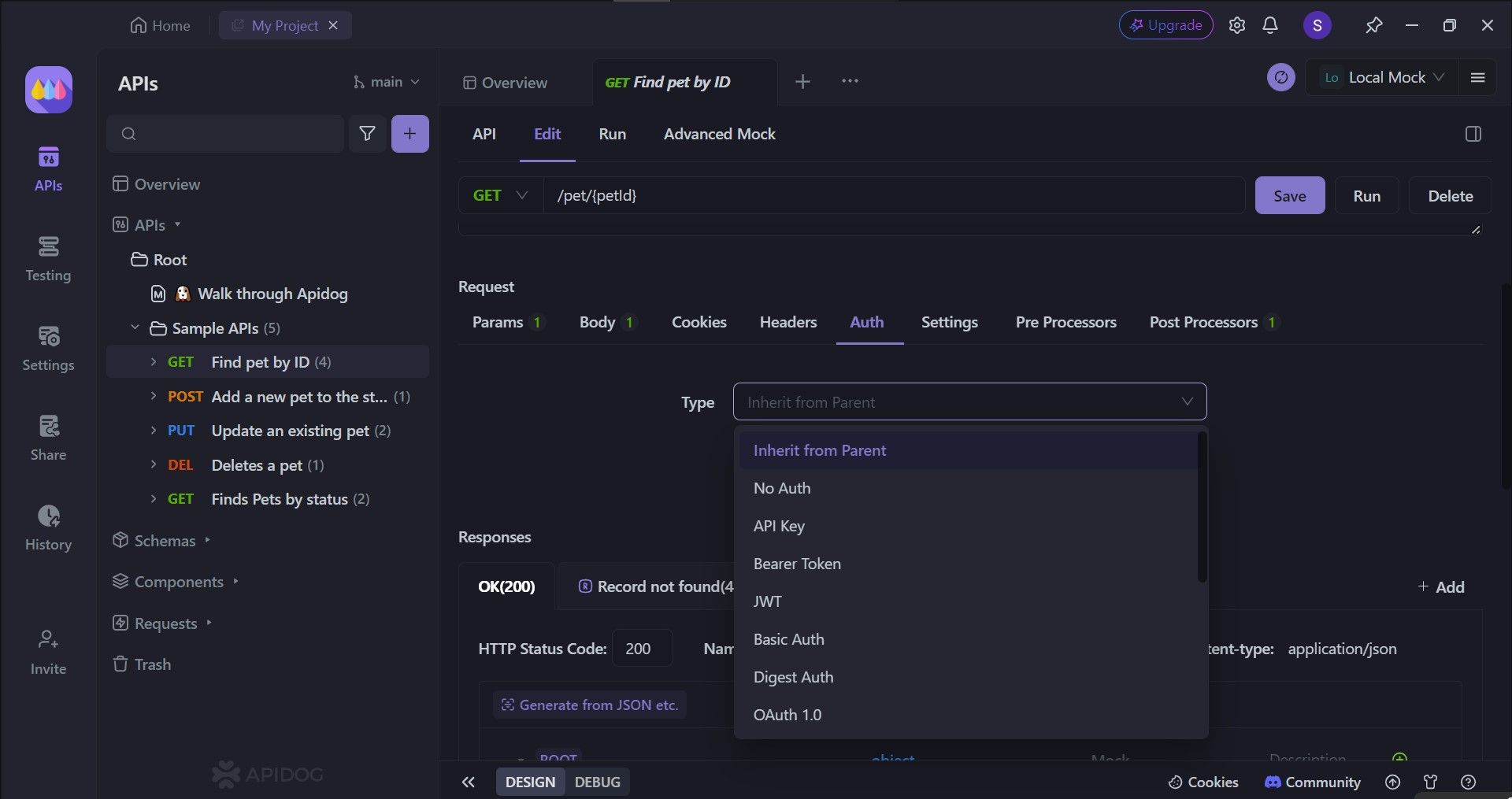
With Apidog, you can edit specific APIs' authentication methods. There are several choices for you to select from. To access this part of Apidog, you need to first:
- Select an API.
- Click the
Edit
heading. - Scroll down to the
Request
section. - Click the
Auth
header. - Finally, select which
Type
of authentication you like.
Generating AIOHTTP Python Code Using Apidog
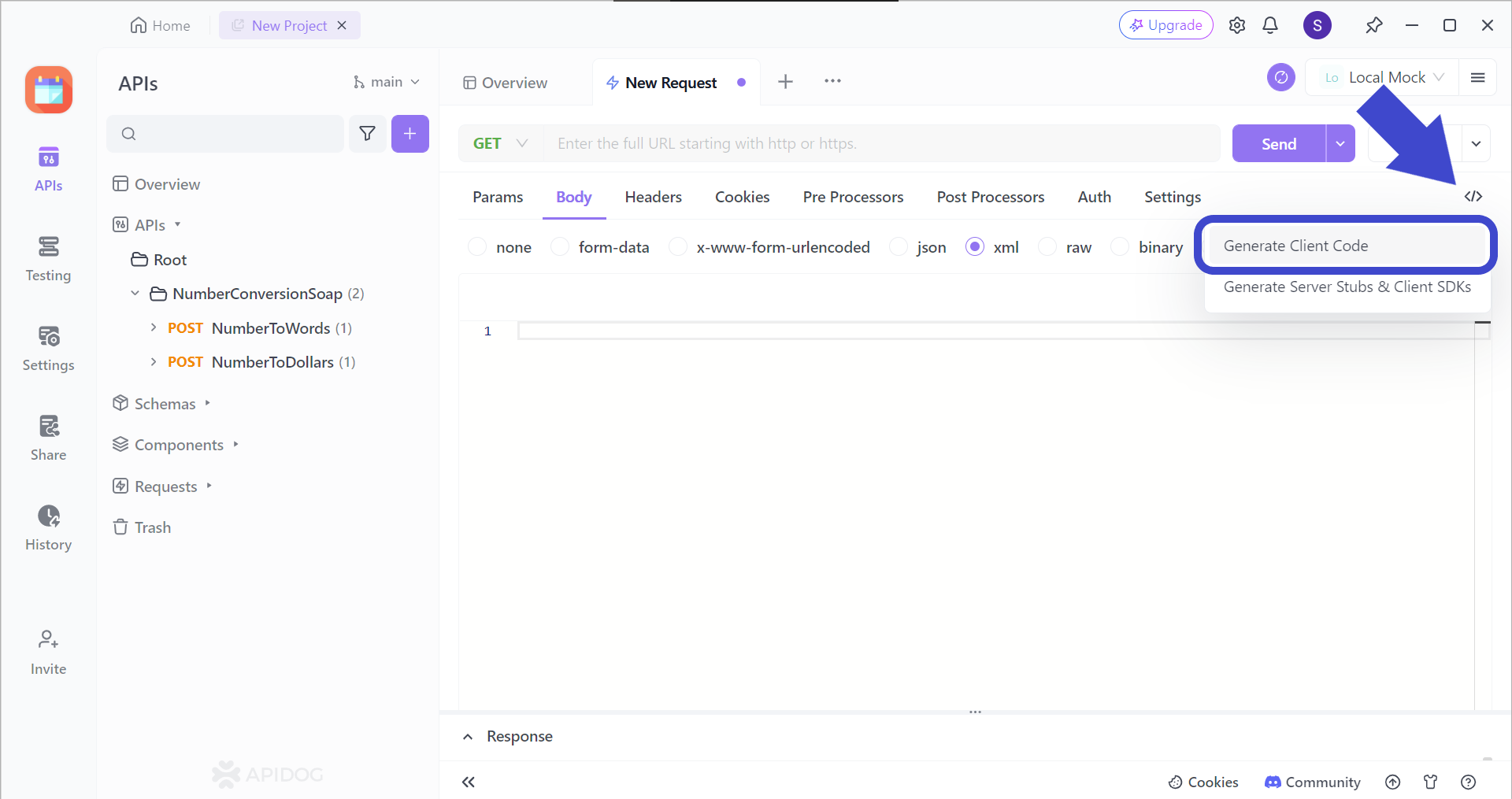
To utilize Apidog's code generation feature, begin by clicking the </>
button found on the top right corner of the Apidog window, and press Generate Client Code
.
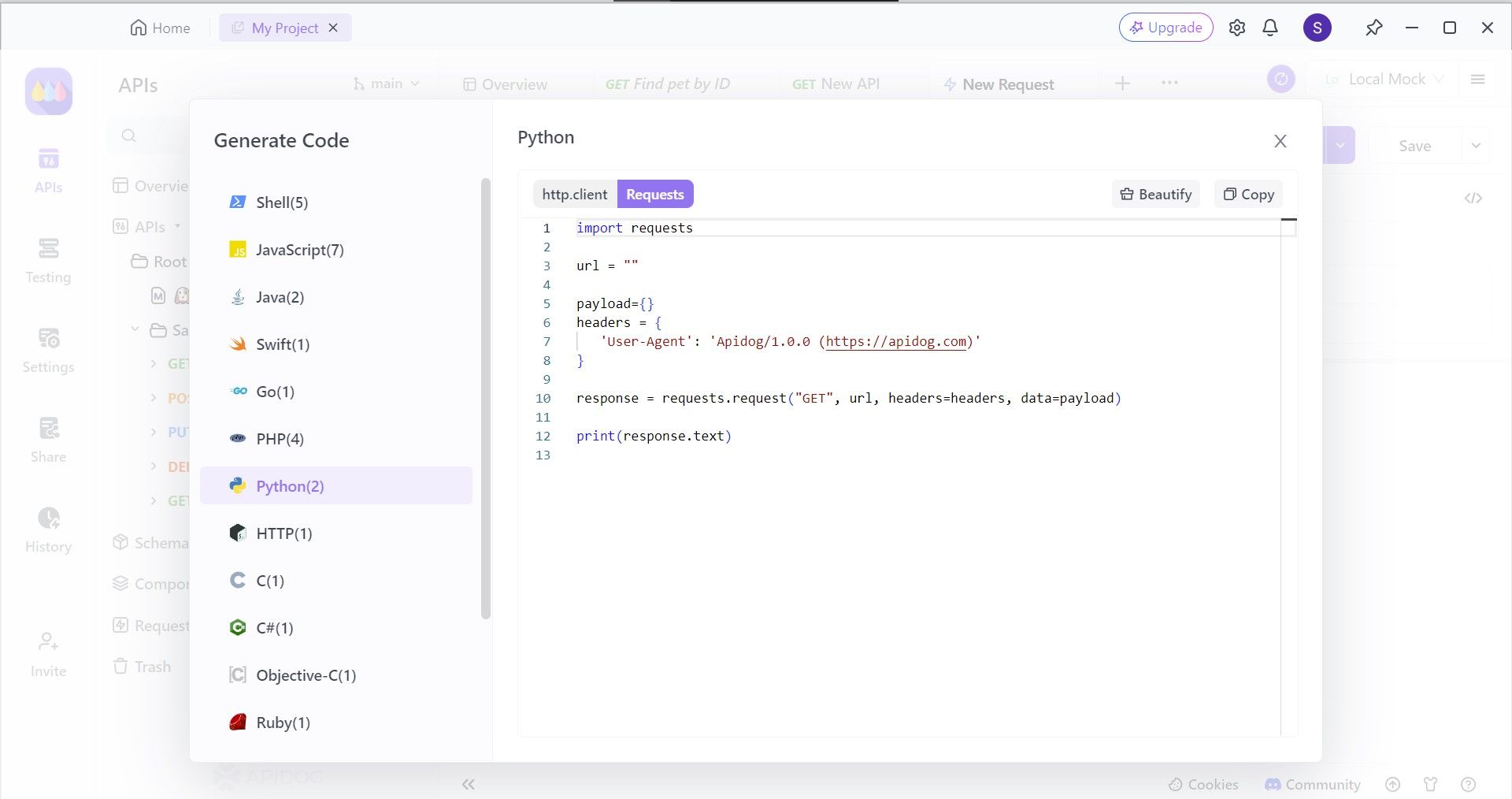
Next, select the Python
section, where you can find different frameworks for the JavaScript language. In this step, select Requests
, and copy the code. You can then paste it over to your IDE to implement the AIOHTTP framework!
Conclusion
By effectively leveraging AIOHTTP authentication, developers can construct a robust security shield for their web applications' APIs. Implementing appropriate authentication methods ensures only authorized users or applications gain access to sensitive data and functionalities. This not only safeguards your application from unauthorized breaches but also fosters trust with users who can be confident their information is protected.
Furthermore, AIOHTTP's flexibility in supporting various authentication methods empowers developers to tailor their security approach to their specific needs. Whether you choose basic authentication, API key authentication, or token-based methods like JWT, AIOHTTP provides the tools and functionalities to create a secure and scalable API environment. By following best practices and staying updated with the latest security advancements, developers can leverage AIOHTTP authentication to empower their APIs and build trust within their applications.
Although setting up proper authentication for your API may sound like a very complex process, it is made simple with Apidog. Aside from selecting what authentication type you would like to implement on your APIs, you can also build, test, mock, and document APIs, all with Apidog!