Pytest Tutorial: API Automated Testing Framework
What is Pytest and how does it work? Pytest is a Python testing framework for writing and executing various software tests.
Python: Automated Testing Framework
Python has several popular automation testing frameworks that can be used for batch, frequent, and repetitive testing. These frameworks encapsulate automation testing code, greatly improving testing efficiency and reusability, reducing repetitive work, and providing extensive test coverage. They do not require manual intervention, thereby reducing the likelihood of errors. Python offers numerous frameworks and libraries that provide support for automated testing.
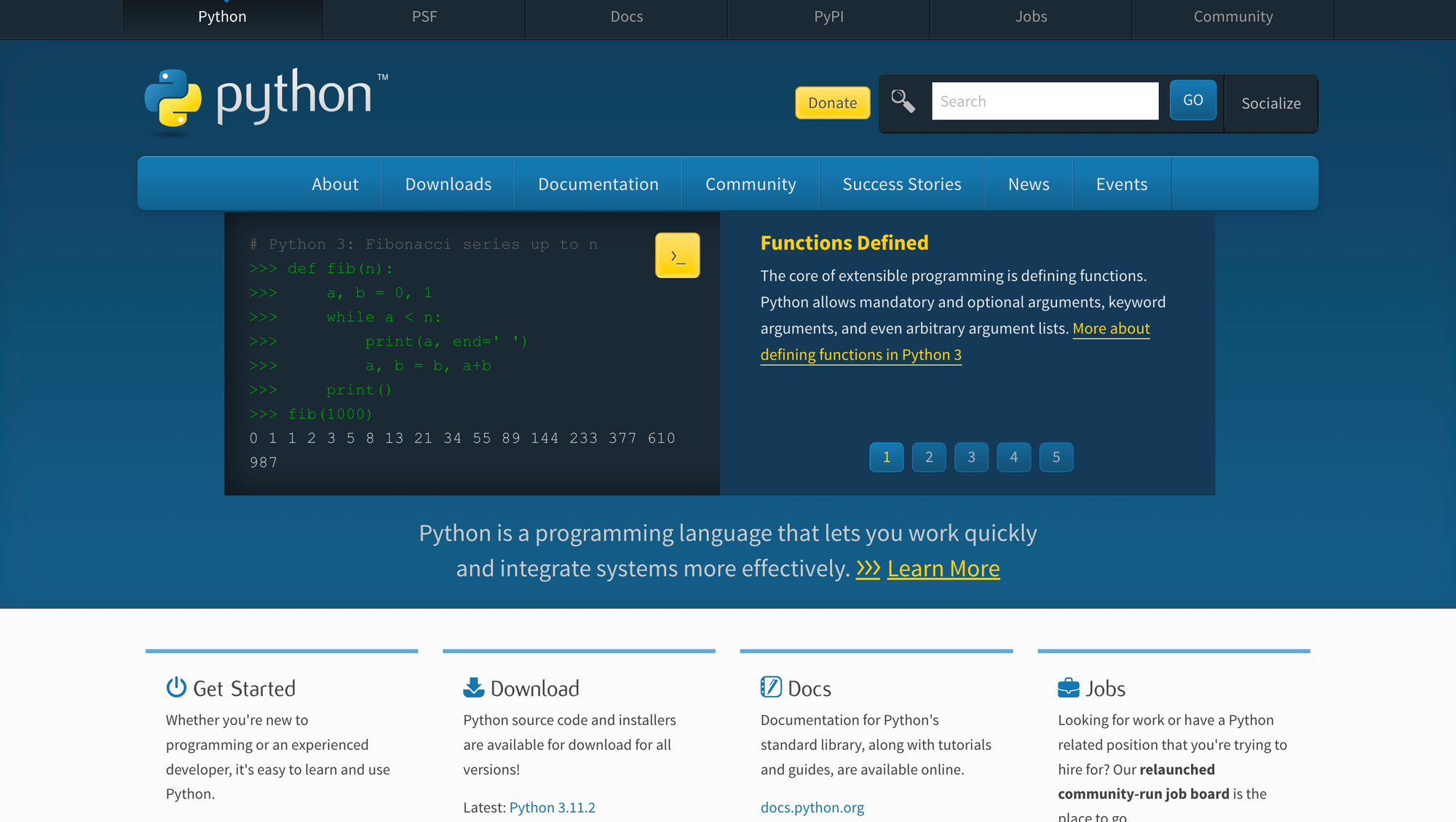
What is Pytest
Pytest is a Python testing framework used for writing and executing various types of software tests, such as unit tests, integration tests, end-to-end tests, and functional tests. It is primarily used for API testing but can also test databases, UI, and other components. It features functionalities like parameterized testing, fixtures, assertion rewriting, and parallel testing. You can install it using the command "pip install Pytest" and verify the installation using the command "Pytest --version".
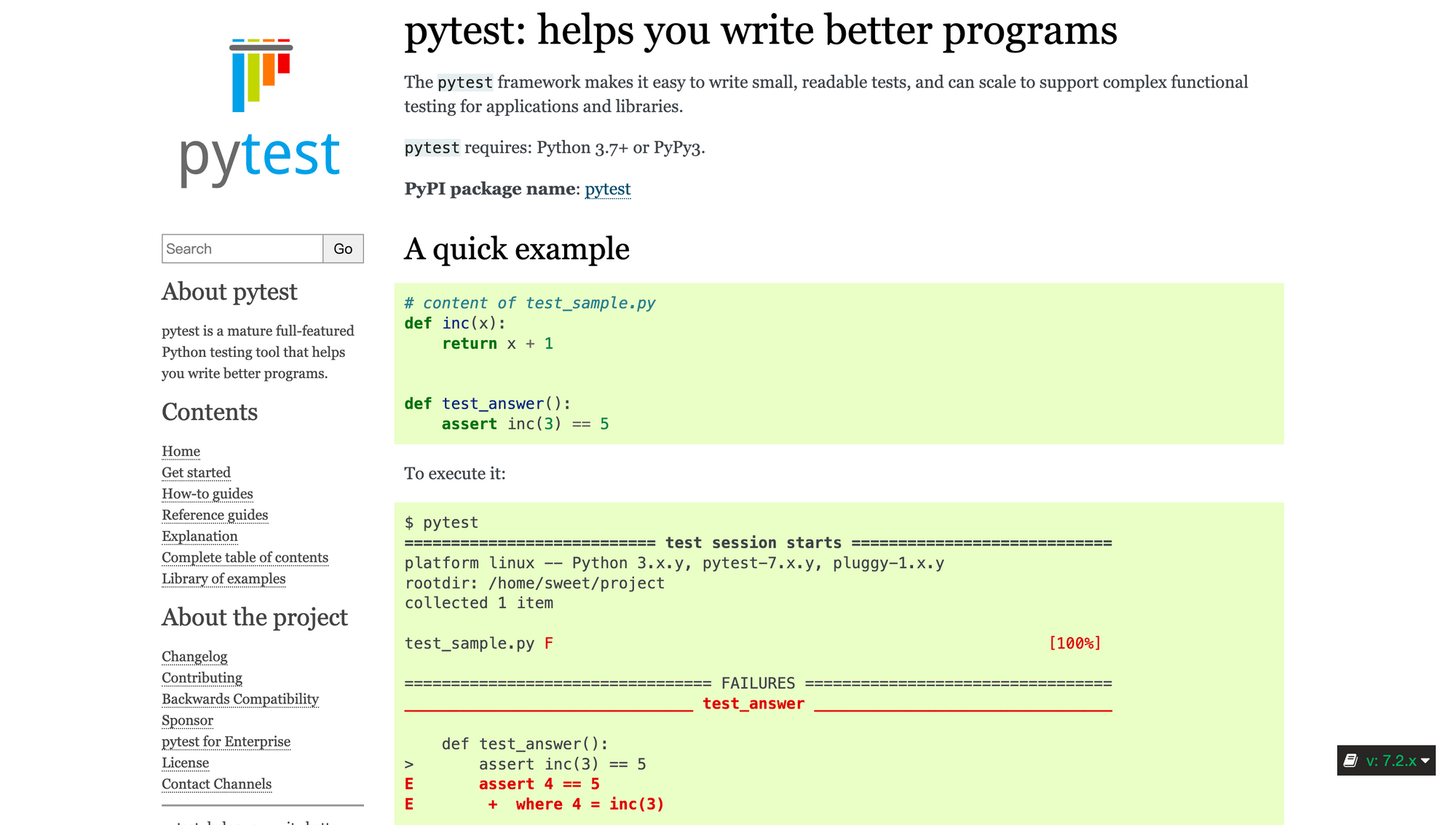
Pros of Pytest:
- Pythonic and Readable: Pytest leverages Python's simplicity and readability, making it accessible even to beginners.
- Broad Testing Scope: While primarily used for API testing, Pytest can also test databases, UI, and other components, providing versatility.
- Rich Ecosystem: Pytest has a vast ecosystem of plugins and extensions that enhance its functionality and integration with other tools.
- Faster Test Execution: Pytest's parallel testing feature allows for faster execution of test cases, improving overall efficiency.
- Detailed Test Reporting: Pytest generates comprehensive test reports, including detailed failure information, aiding in issue identification and resolution.
Cons of Pytest:
- Learning Curve: Although Pytest is beginner-friendly, mastering advanced features and fixtures may require some learning.
- Limited Built-in Functionality: Compared to specialized testing frameworks, Pytest may have limited built-in functionalities for specific testing requirements.
- Dependency on Python: Pytest is tightly coupled with the Python programming language, which may limit its usage in non-Python environments.
How to Perform Python Automation Testing with Pytest
Installing Pytest
You need to install Pytest by using pip.
pip install -U Pytest
After installation, enter it in the terminal and if the version number is displayed, the installation is successful.
Pytest --version
Pytest Use Case Writing Specification
Pytest Test Case Writing Guidelines:
- The files to be tested must start with
test_
or end with_test
. - When running Pytest, it searches for files starting with
test_
or ending with_test
and treats them as test files. - The test class name should start with "Test" and should not have an "init" function, as it may trigger a warning:
ytestCollectionWarning: cannot collect test class 'TestXXX'
. - Test method functions should start with
test_
. - Use assert for assertions.
Running Command
Pytest -k "class name"
Pytest -k "method"
Pytest -k "class name and not method"
Running Modules
Pytest provides multiple running modes, allowing you to run specific modules or even specific methods within a module.
Pytest file name.py
Pytest file name.py::class name
Pytest file name.py::class name::method
Test Code Example
You can write a piece of test code to test it. We need to create a .py file starting with test_.
def setup_module():
print('This is setup_module method, executed only once when there are multiple test classes.')
def teardown_module():
print('This is teardown_module method, executed only once when there are multiple test classes.')
def setup_function():
print('This is setup_function method, executed only once when there are multiple test classes.')
def teardown_function():
print('This is teardown_function method, executed only once when there are multiple test classes.')
def test_five():
print('This is test_five method.')
def test_six():
print('This is test_six method.')
class TestPytest01:
def setup_class(self):
print('Called setup_class1 method.')
def teardown_class(self):
print('Called teardown_class1 method.')
def setup_method(self):
print('Executed setup1 operation before the test method.')
def teardown_method(self):
print('Executed teardown1 operation after the test method.')
def test_one(self):
print('This is test_one method.')
def test_two(self):
print('This is test_two method.')
def setup(self):
print('This is setup method.')
def teardown(self):
print('This is teardown method.')
class TestPytest02:
def setup_class(self):
print('Called setup_class2 method.')
def teardown_class(self):
print('Called teardown_class2 method.')
def setup_method(self):
print('Executed setup2 operation before the test method.')
def teardown_method(self):
print('Executed teardown2 operation after the test method.')
def test_three(self):
print('This is test_three method.')
def test_four(self):
print('This is test_four method.')
Run the above code and you will get the result.
Controlling the order of use cases
If you want to set the order of the use cases, you can use the Pytest-order plugin, combined with the decorator @Pytest.mark.run(order=num)
.
pip install Pytest-ordering
We can write a piece of code to verify this.
import Pytest
class TestPytest:
@Pytest.mark.run(order=-2)
def test_03(self):
print('test_03')
@Pytest.mark.run(order=-3)
def test_01(self):
print('test_01')
@Pytest.mark.run(order=4)
def test_02(self):
print('test_02')
The result of the implementation is:
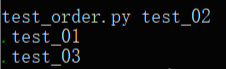
How to Test Python APIs with Apidog
The previous discussion was about testing some function methods written in Python using Pytest. But what if we want to test APIs written in Python? For API testing, here we will sincerely recommend using Apidog.
What is Apidog? Apidog is an all-in-one API collaboration platform that enables API documentation, API debugging, API Mock, and API automation testing and is a more advanced API design/development/testing tool.
Apidog provides a comprehensive API management solution. With Apidog, you can design, debug, test, and collaborate on your APIs from a unified platform, eliminating the problems of switching between tools and inconsistent data.
For example, let's say I have already written a simple API using Python:
/api/test/python
Creating a request
We need to create an API request for the same API we just wrote, filling in:
- Request Path
- Request Method
- Request name
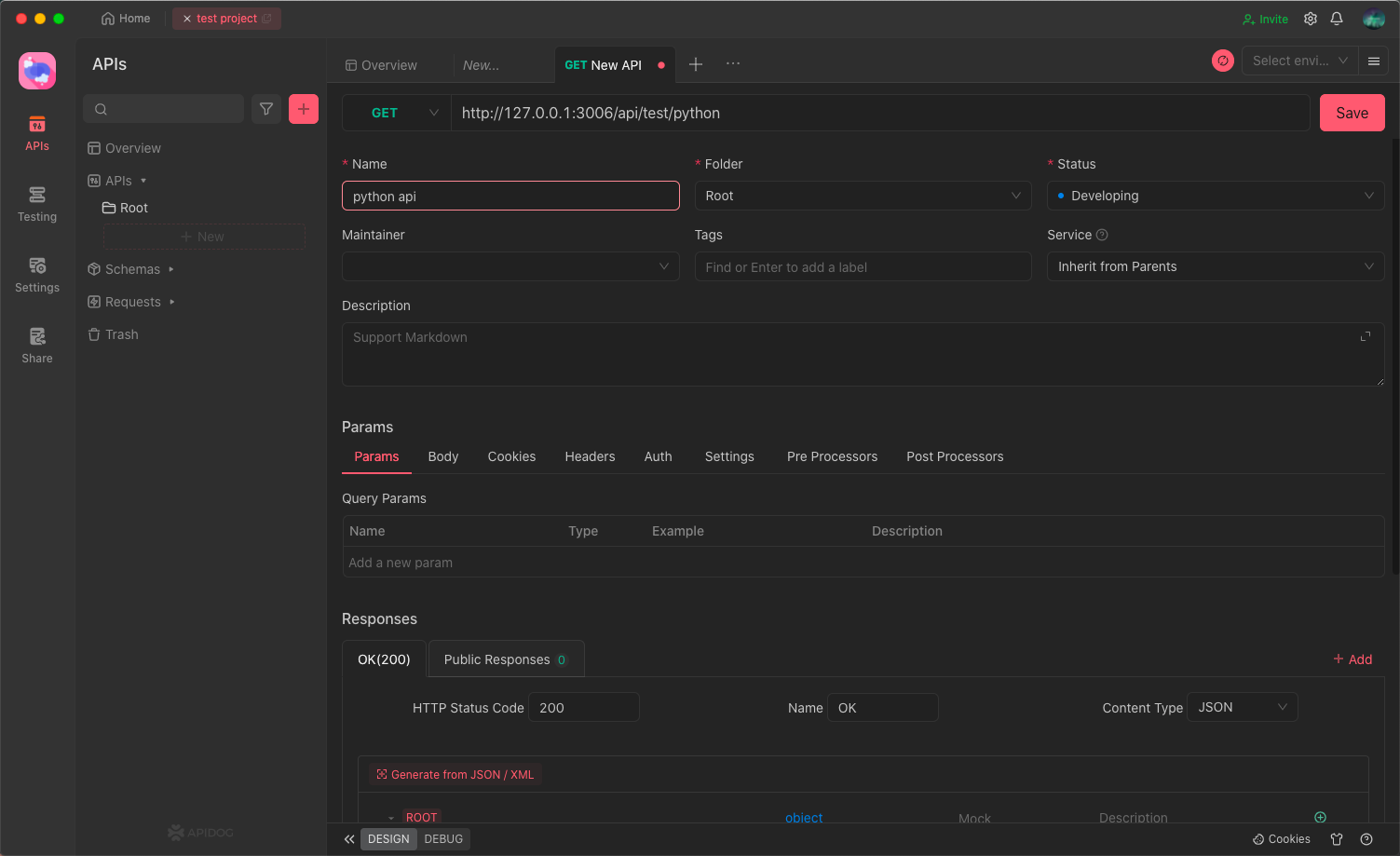
Setting Assertions
Next, we tap on the post-script item and select the custom script. Apidog provides us with visual scripting options.
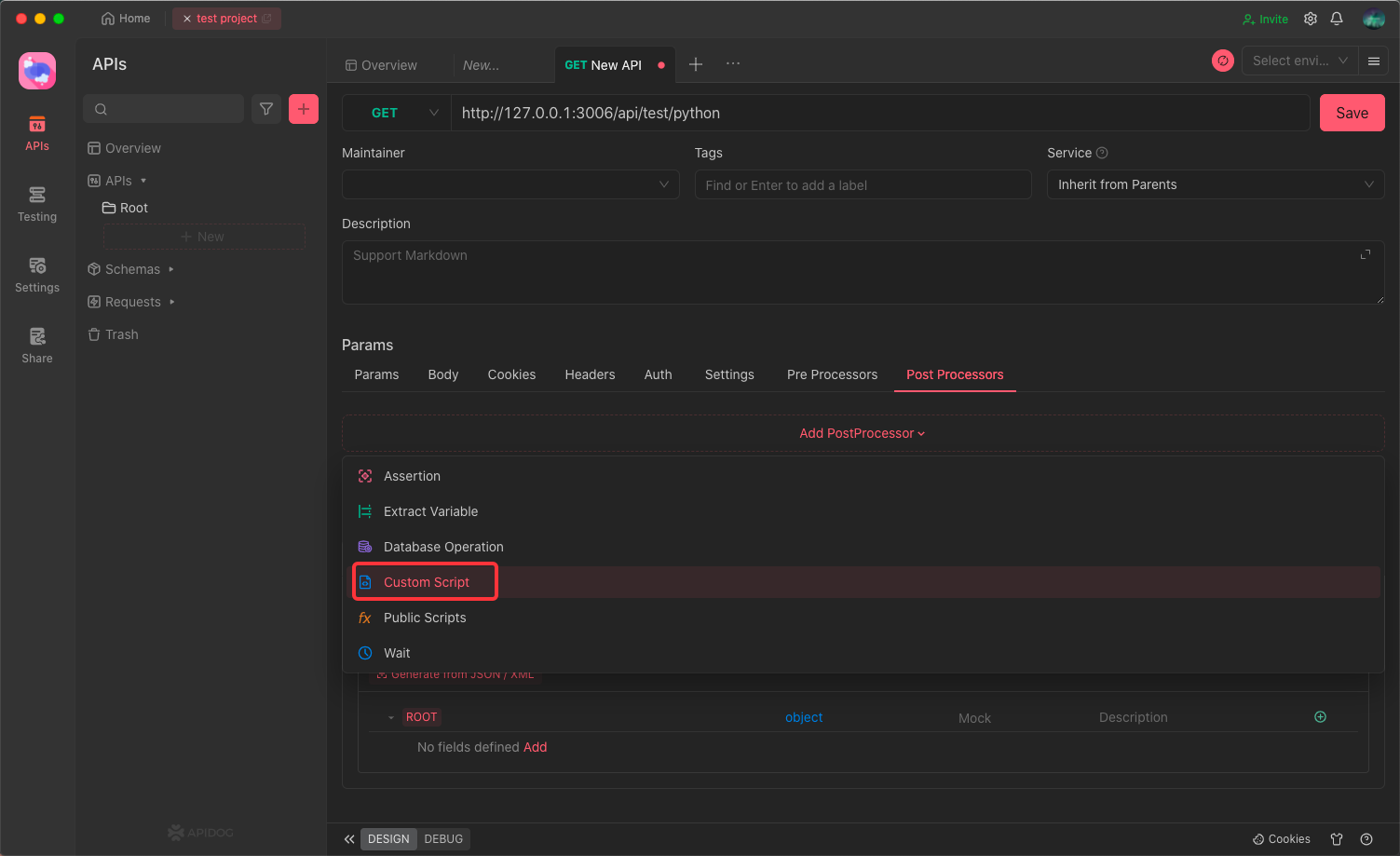
We click on the ready-made script command provided to us by Apidog on the right, and we expect a code of 200 to be returned.
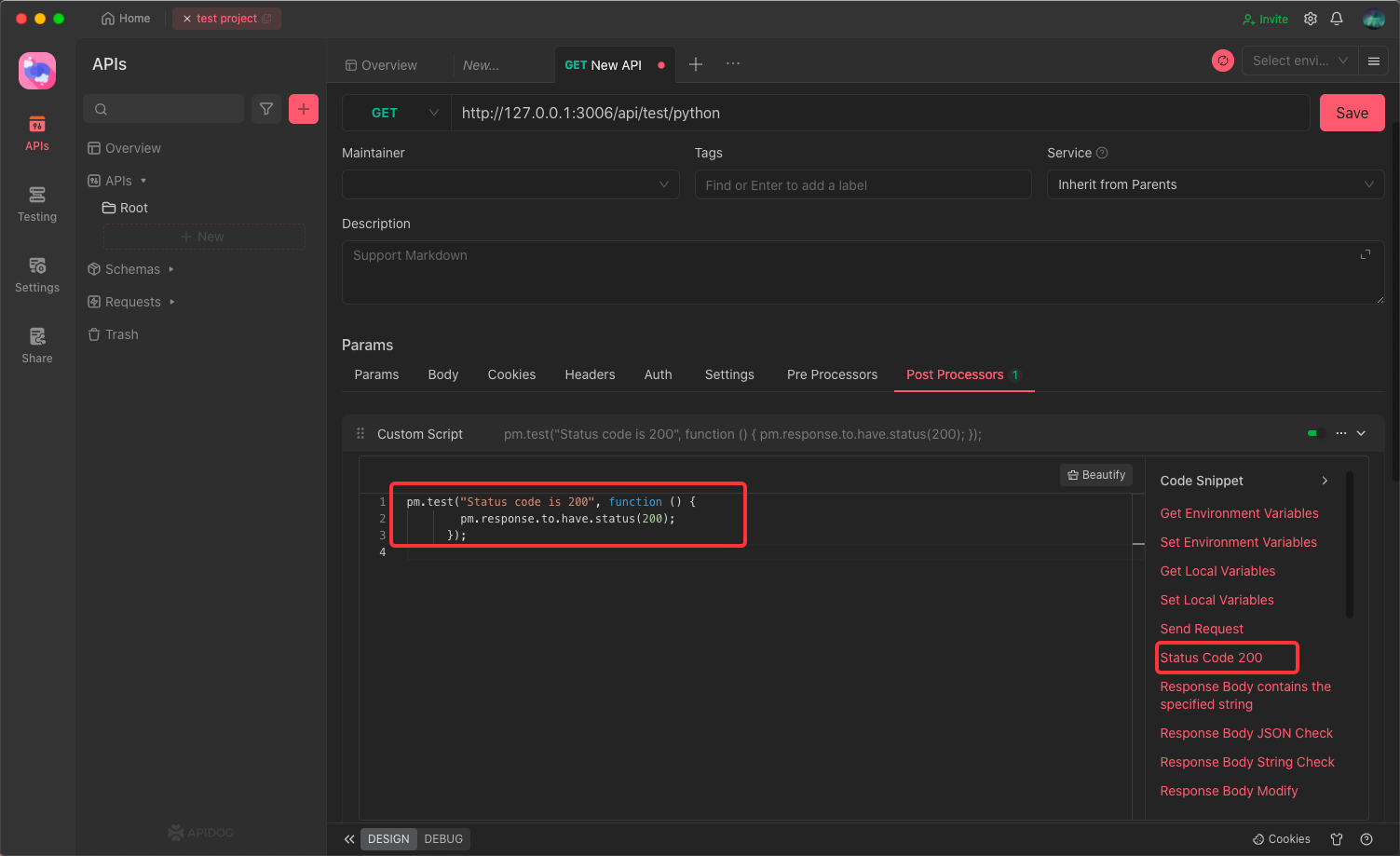
After setting the assertion script, click the Save button.
Run debugging
Go to the Run page and click Send to see the response results and an indication that the assertion passed successfully.
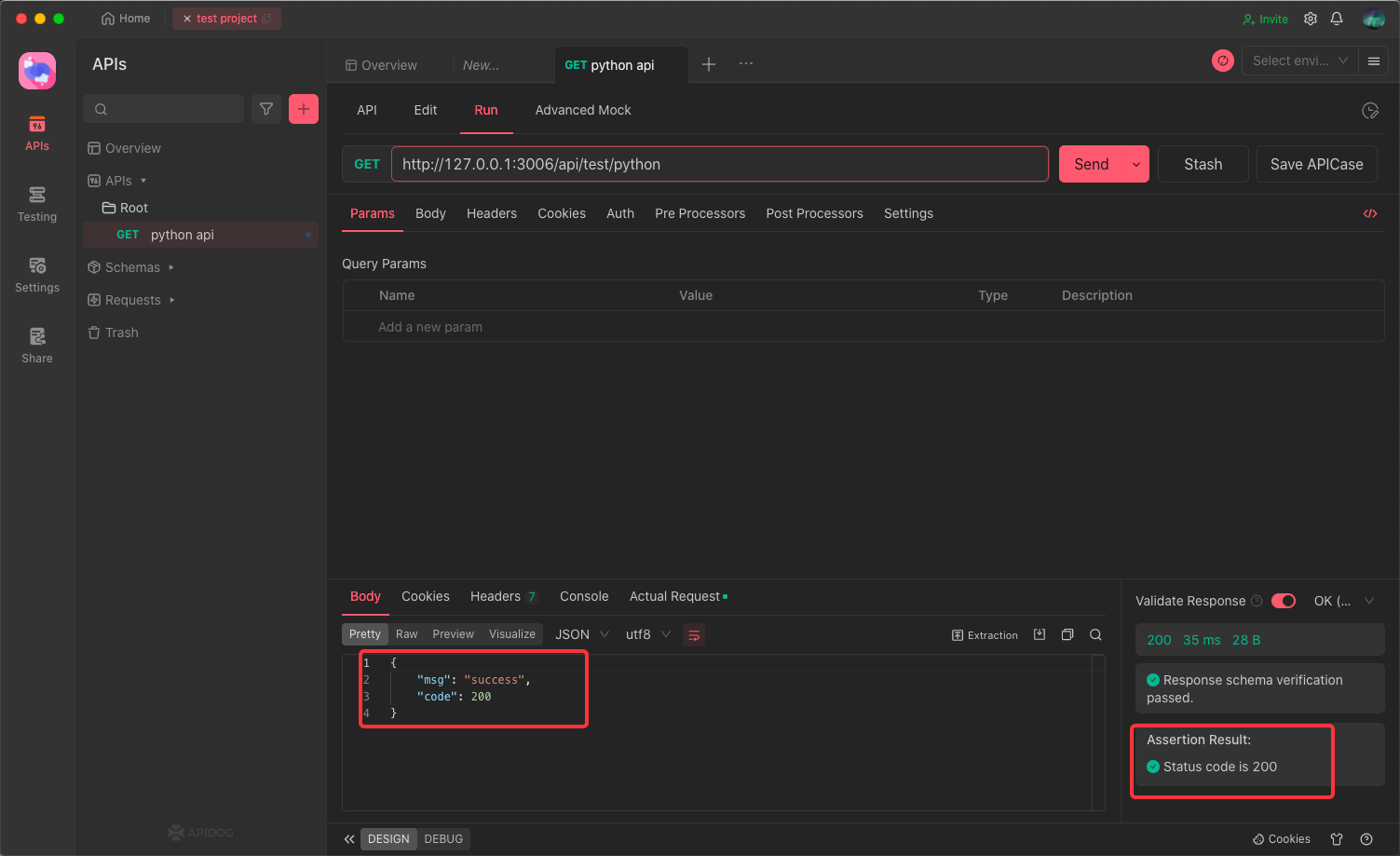