How to Use FastAPI APIRouters
In this article, we will dive into the world of FastAPI APIRouters and explore how to use FastAPI APIRouters.
FastAPI is a modern and high-performance web framework for building APIs with Python. Its simplicity, speed, and automatic validation of request data using Python-type hints make it a popular choice among developers. One of the powerful features that FastAPI offers is the use of APIRouters, which allows you to organize and structure your API code in a modular and scalable manner.
In this article, we will dive into the world of FastAPI APIRouters and explore how they can streamline your API development process. We'll walk you through the step-by-step process of creating and using APIRouters, showcasing their flexibility and versatility.
Overview of FastAPI APIRouters
One of the critical features of FastAPI is the ability to use APIRouters. APIRouters allow you to organize your API endpoints into modular components, making managing and maintaining your codebase easier. They provide a way to logically group related routes and endpoints together, allowing for better organization and separation of concerns.
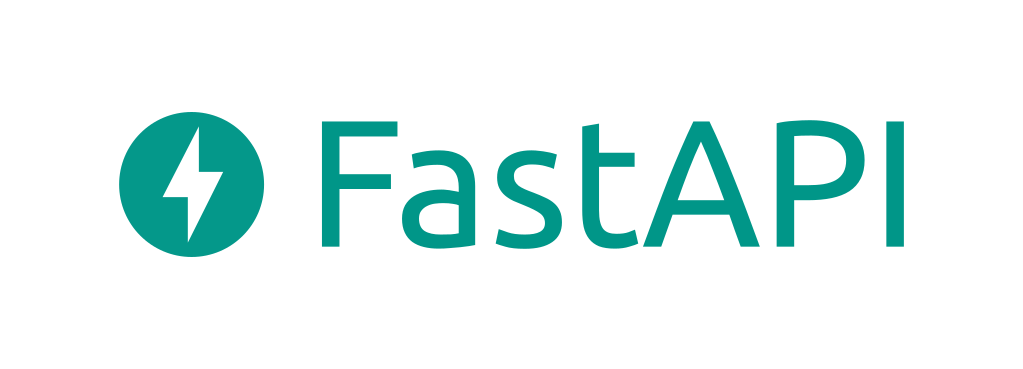
APIRouters in FastAPI are created by instantiating the APIRouter
class from the fastapi
module. This class provides methods to define routes and endpoints, handle request methods and parameters, and mount the router within the FastAPI application.
To create an APIRouter, you simply import the APIRouter
class and instantiate it:
from fastapi import APIRouter
router = APIRouter()
Once you have created the router, you can define routes and endpoints using the various methods provided by the APIRouter
class. These methods include router.get()
, router.post()
, router.put()
, router.delete()
, and so on, which correspond to the different HTTP request methods.
For example, to define a GET endpoint at the path /users
, you can use the router.get()
method:
@router.get("/users")
def get_users():
return {"message": "Get all users"}
You can also define parameters for your routes and endpoints using the same syntax as in regular FastAPI applications. For example, to define a route with a path parameter, you can use the router.get()
method with a path that includes a parameter enclosed in curly braces:
@router.get("/users/{user_id}")
def get_user(user_id: int):
return {"message": f"Get user with ID {user_id}"}
In addition to handling request methods and parameters, APIRouters also provide a way to organize and mount multiple routers within your FastAPI application. This can be done using the app.include_router()
method, which takes an instance of the router as an argument.
For example, if you have multiple APIRouters defined in separate files, you can mount them within your FastAPI application like this:
from fastapi import FastAPI
from .routers import router1, router2
app = FastAPI()
app.include_router(router1)
app.include_router(router2)
This allows you to keep your codebase modular and maintainable, with each router handling a specific set of routes and endpoints.
How to Create APIRouters in FastAPI
In FastAPI, APIRouters are used to organize and group related routes and endpoints together. They provide a way to modularize your application's API and make it more maintainable and scalable. In this section, we will explore how to create APIRouters in FastAPI.
To create an APIRouter, you first need to import it from the FastAPI module. Here's an example:
from fastapi import APIRouter
router = APIRouter()
Once you have created an APIRouter instance, you can start defining routes and endpoints using the router object. The routes and endpoints defined within an APIRouter will be automatically mounted to the main FastAPI application.
Let's take a look at an example of how to define routes and endpoints within an APIRouter:
from fastapi import APIRouter
router = APIRouter()
@router.get("/users")
def get_users():
return {"message": "Get all users"}
@router.get("/users/{user_id}")
def get_user(user_id: int):
return {"message": f"Get user with id {user_id}"}
@router.post("/users")
def create_user(user: dict):
return {"message": f"Create user with data {user}"}
In the above example, we have defined three routes within the APIRouter: /users
, /users/{user_id}
, and /users
. Each route is associated with a specific HTTP request method (GET
or POST
) and a corresponding function that handles the request.
To use the APIRouter in your FastAPI application, you need to mount it to the main application using the include_router()
function. Here's an example:
from fastapi import FastAPI
from .routers import router
app = FastAPI()
app.include_router(router, prefix="/api")
In the above example, we have mounted the APIRouter to the main FastAPI application using the include_router()
function. The prefix
parameter is used to specify a common prefix for all routes defined within the APIRouter.
By organizing your routes and endpoints into separate APIRouters, you can keep your codebase clean and maintainable. It also allows you to easily add new routes and endpoints without modifying the main application code.
Defining Routes and Endpoints in APIRouters
In FastAPI, APIRouters are used to define routes and endpoints for your API. These routers allow you to organize your API endpoints into separate modules or files, making managing and maintaining your codebase easier.
To define routes and endpoints in APIRouters, you must create a new router instance using the APIRouter
class provided by FastAPI. This class allows you to define the routes and endpoints using decorators, similar to how you would define them in the main FastAPI application.
Here's an example of how to define routes and endpoints in an APIRouter:
from fastapi import APIRouter
router = APIRouter()
@router.get("/items")
async def get_items():
return {"message": "Get all items"}
@router.get("/items/{item_id}")
async def get_item(item_id: int):
return {"message": f"Get item with id {item_id}"}
@router.post("/items")
async def create_item(item: Item):
# Code to create an item
return {"message": "Item created successfully"}
In the above example, we first import the APIRouter
class from FastAPI. Then, we create a new instance of the router using APIRouter()
. This creates a new router object that we can use to define our routes and endpoints.
Next, we use the @router.get()
decorator to define a GET endpoint for retrieving all items. The function get_items()
is the handler for this endpoint and will be executed when a GET request is made to the /items
route.
Similarly, we use the @router.get()
decorator to define another GET endpoint for retrieving a specific item based on its ID. The function get_item()
takes an item_id
parameter and will be executed when a GET request is made to the /items/{item_id}
route.
Finally, we use the @router.post()
decorator to define a POST endpoint for creating a new item. The function create_item()
takes an item
parameter, which is of type Item
(a Pydantic model), and will be executed when a POST request is made to the /items
route.
Once you have defined your routes and endpoints in the APIRouter, you can mount it in your main FastAPI application using the app.include_router()
method. This allows you to organize and modularize your API endpoints effectively.
Apidog is an API collaboration platform that combines API documentation, API debugging, API mocking, and API automated testing. With Apidog, we can conveniently debug FastAPI interfaces.
To quickly debug an API endpoint, create a new project, select "Debug Mode" within the project, and fill in the request address. This allows you to send requests rapidly and receive response results. An example of this practice is shown in the figure above.
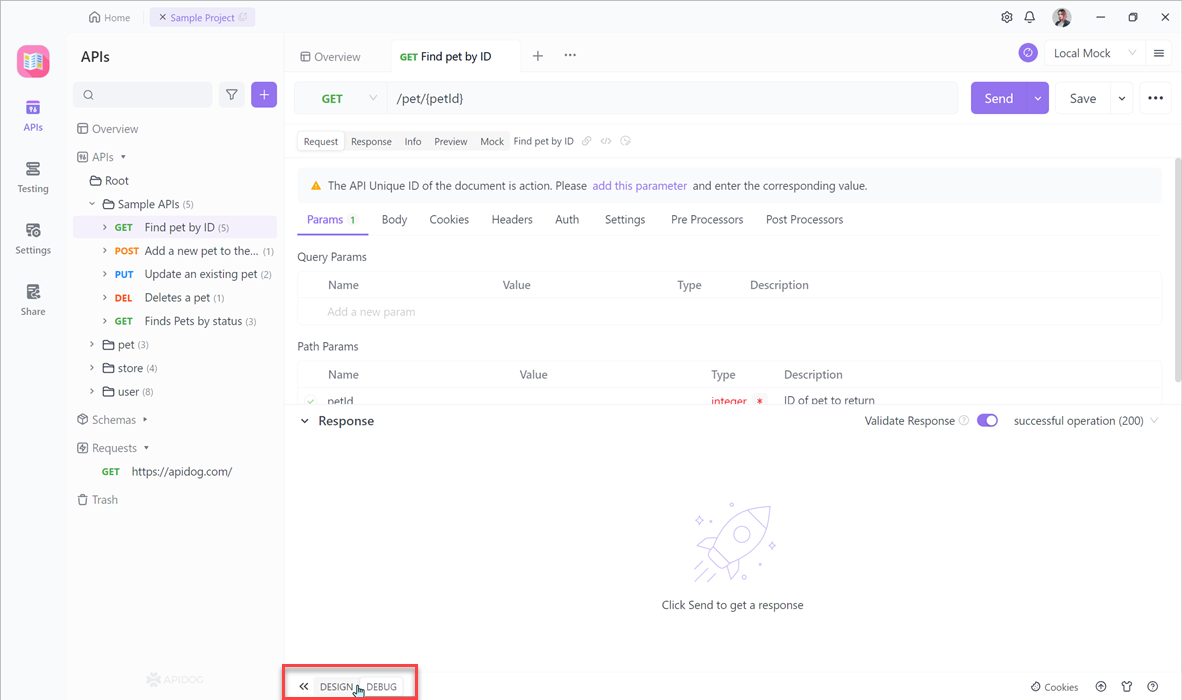
In conclusion
APIRouters in FastAPI provide a convenient way to define routes and endpoints for your API. By using decorators, you can easily define the HTTP methods and parameters for each endpoint. Additionally, organizing your endpoints into separate routers helps to keep your codebase clean and maintainable.