How to Test Django REST Framework
Testing is a crucial aspect of web development, ensuring that your Django Rest Framework (DRF) API functions correctly and efficiently. In this guide, we'll walk you through a step-by-step process on how to test Django Rest Framework, covering unit tests, integration tests with Apidog, and more.
Testing is a crucial aspect of web development, ensuring that your Django Rest Framework (DRF) API functions correctly and efficiently. In this guide, we'll walk you through a step-by-step process on how to test Django Rest Framework, covering unit tests, integration tests with Apidog, and more. By the end of this article, you'll have a solid foundation for building robust and reliable APIs with Django Rest Framework.
Why Test Django Rest Framework?
Before diving into the testing process, let's briefly discuss the importance of testing in the context of Django Rest Framework.
Bug Detection: Testing helps identify and fix bugs early in the development process, preventing issues from reaching production.
Code Confidence: Robust test coverage gives developers confidence in the stability and reliability of their code.
Documentation: Tests serve as living documentation, providing insights into how different components of your API should behave.
Refactoring Support: With a comprehensive test suite, you can refactor your code confidently, knowing that tests will catch any regressions.
How to Test Django REST Framework?
If you have created RESTful APIs with Django REST Framework. Now, let's move on to the practical steps for testing Django Rest Framework.
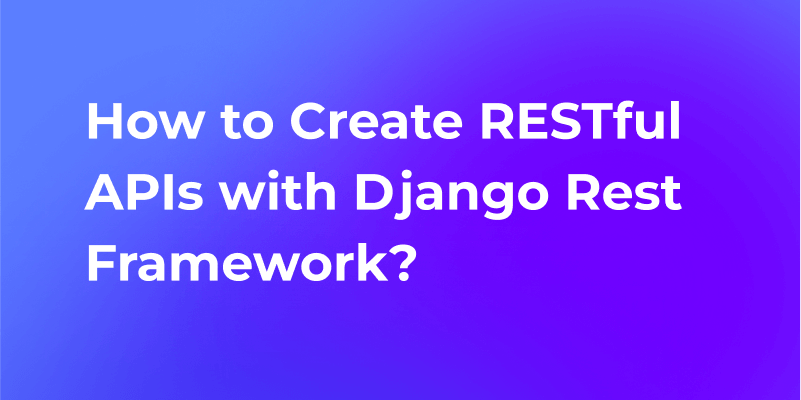
1. Setting Up Your Test Environment
First, make sure you have a virtual environment set up for your Django project. You can create one using:
python -m venv venv
Activate the virtual environment:
- On Windows:
venv\Scripts\activate
- On macOS/Linux:
source venv/bin/activate
Install the required packages:
pip install django djangorestframework
2. Writing Unit Tests
Start by writing unit tests for your serializers, views, and other components. Use the built-in unittest
module or Django's TestCase
class for testing.
# Example unit test for a serializer
from django.test import TestCase
from myapp.serializers import MyModelSerializer
class MyModelSerializerTest(TestCase):
def test_serializer_valid_data(self):
data = {'field1': 'value1', 'field2': 'value2'}
serializer = MyModelSerializer(data=data)
self.assertTrue(serializer.is_valid())
3. Integration Testing with Django Rest Framework's APIClient
Django Rest Framework provides an APIClient
for making test requests to your API.
# Example integration test
from rest_framework.test import APITestCase
from rest_framework import status
from myapp.models import MyModel
class MyModelAPITest(APITestCase):
def setUp(self):
MyModel.objects.create(field1='value1', field2='value2')
def test_get_my_model_list(self):
url = '/api/mymodel/'
response = self.client.get(url)
self.assertEqual(response.status_code, status.HTTP_200_OK)
4. Testing Authentication and Permissions
Ensure that your authentication and permission classes are functioning as expected.
# Example test for authentication
from rest_framework.test import APITestCase
from rest_framework import status
from django.contrib.auth.models import User
from myapp.models import MyModel
class MyModelAPITest(APITestCase):
def setUp(self):
user = User.objects.create_user(username='testuser', password='testpassword')
self.client.force_authenticate(user=user)
def test_authenticated_user_can_create_model(self):
url = '/api/mymodel/'
data = {'field1': 'value1', 'field2': 'value2'}
response = self.client.post(url, data)
self.assertEqual(response.status_code, status.HTTP_201_CREATED)
5. Running Tests and Coverage
Run your tests using:
python manage.py test
To check test coverage, you can use tools like coverage.py
:
pip install coverage
coverage run manage.py test
coverage report
Overview of Apidog
Apidog provides a robust set of features to streamline API development and testing. In terms of testing, Apidog facilitates in-depth examination by allowing users to create and manage JSON/XML schemas for comprehensive test results and responses. Additionally, the platform excels in API documentation capabilities, offering customizable layouts to effectively document various functionalities of the API.
Furthermore, Apidog incorporates visual tools designed specifically for API testing, allowing users to seamlessly add assertions and create test scenarios, ensuring the resilience of their APIs. Beyond testing, Apidog promotes collaboration on API projects by enabling users to share and work together with team members. This collaborative feature enhances teamwork and contributes to more efficient project management.
Integration with Django REST Framework:
Now, let's explore how Apidog integrates with Django REST Framework for effective API testing and management:
Accessing Apidog:
- Begin by visiting Apidog's official website at https://apidog.com/. Users can either sign up for free or download the version compatible with their system to initiate API testing with Apidog.
Creating a New Project:
- Initiate a new project by clicking the "New Project" button on the right side of the interface. Provide a suitable name for the project and select the project type.
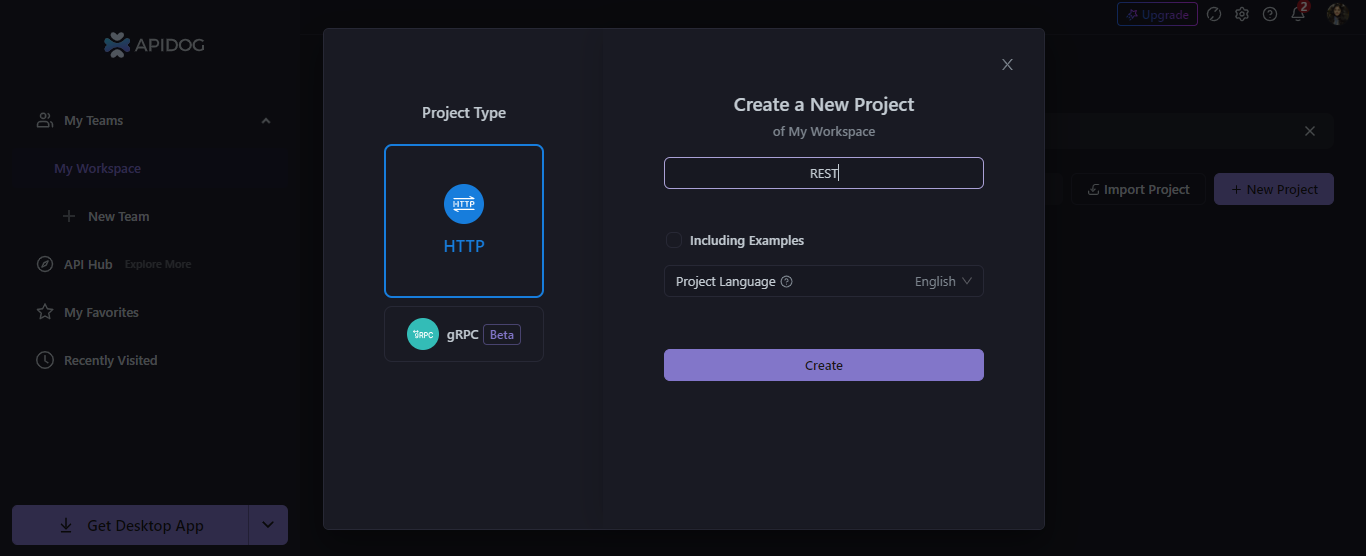
Creating and Testing APIs:
- Users can create new APIs and apply various methods to their requests. For instance, testing the GET method for a localhost can be done by assuming a URL like "http://localhost:8000/api/items/". The platform provides a user-friendly interface to input the URL, send the request, and examine the response.
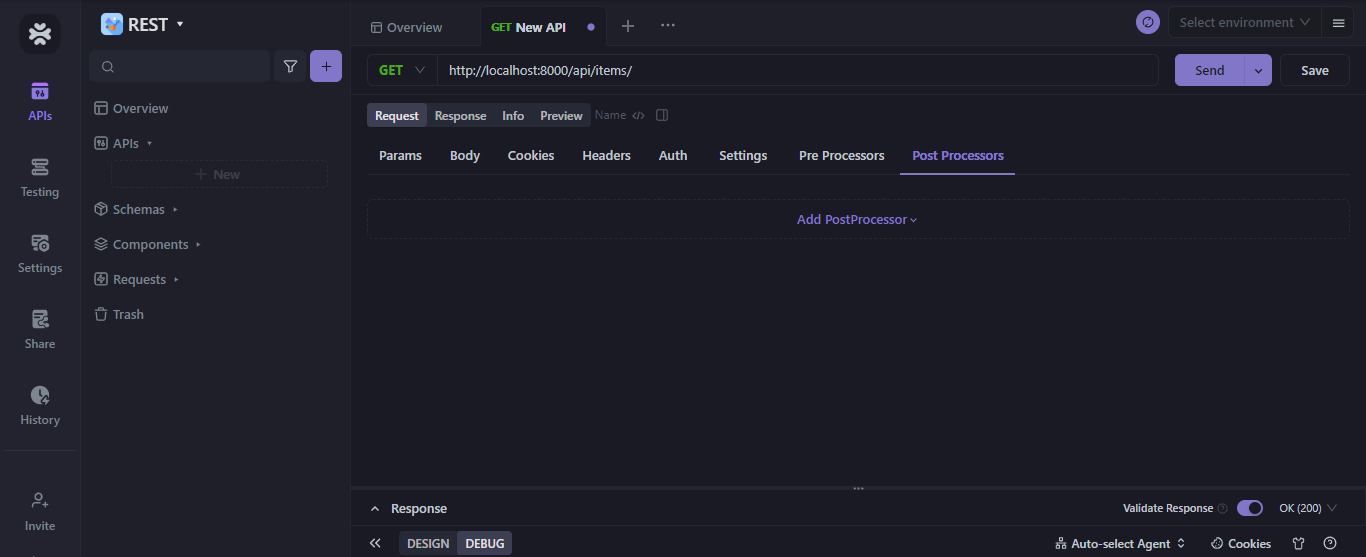
Validating Responses:
- Responses to requests can be checked in the designated Response section. Valid responses are displayed, while validation errors are highlighted in case of issues. It is crucial to ensure that all API-generating steps have been executed accurately
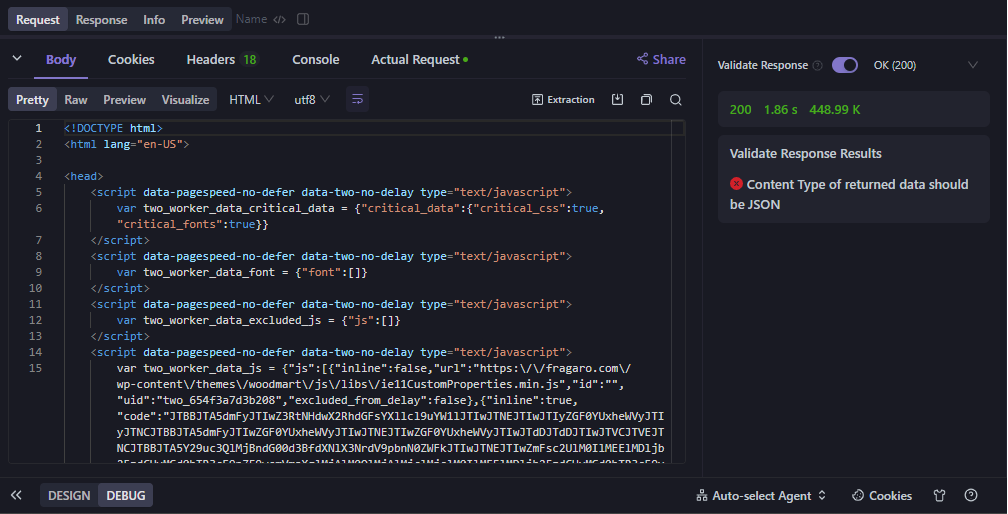
Conclusion:
Testing your Django Rest Framework API is essential for ensuring its reliability and performance. By following these steps, you'll be well-equipped to catch and fix issues early in the development process, ultimately leading to a more robust and maintainable codebase.