How to Send HTTP/2 Request?
In this blog, we'll guide you through the correct way to send HTTP/2 requests, addressing the common misconceptions and challenges you might encounter along the way.
In the ever-evolving world of web communication, it's essential to stay up-to-date with the latest technologies and protocols. One such advancement is HTTP/2, which promises faster and more efficient web browsing.
With its promise of improved performance, it's important to understand how to send an HTTP/2 request effectively. In this blog, we'll walk you through the steps to send an HTTP/2 request and harness the benefits of this modern protocol.
What is HTTP/2
Before we dive into the intricacies of sending HTTP/2 requests, let's briefly recap what HTTP/2 is. HTTP/2 is the second major version of the HTTP network protocol, designed to enhance the performance of websites and web applications. It achieves this by reducing latency and optimizing the way data is transmitted between clients and servers.
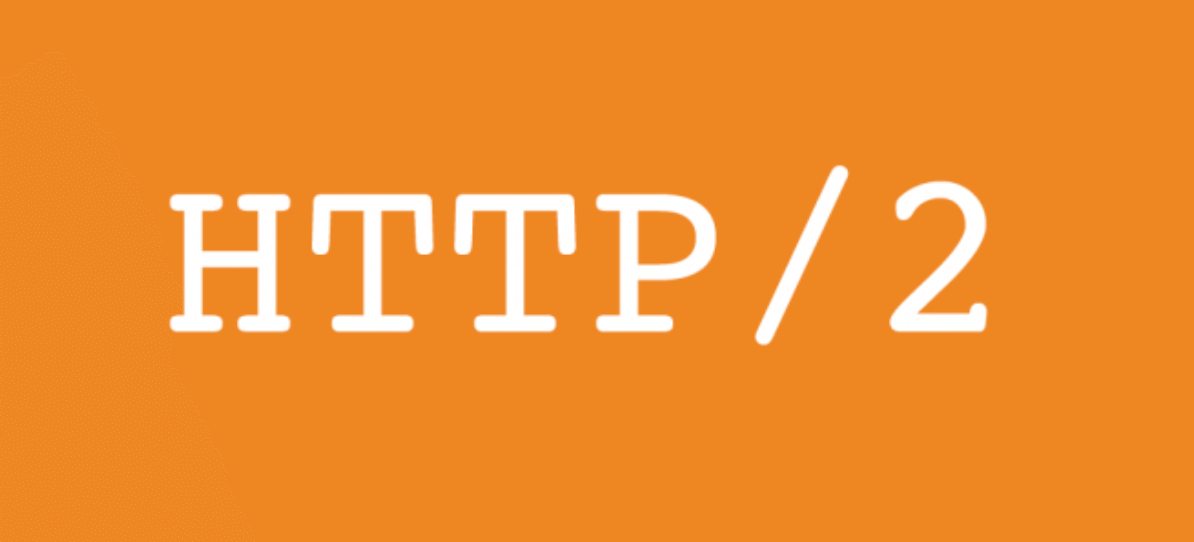
How to Send an HTTP/2 Request: A Comprehensive Guide
Step 1: Ensure HTTP/2 Support
Before diving into sending an HTTP/2 request, it's crucial to ensure that both your client and server support HTTP/2. Fortunately, most modern web browsers and web servers already provide built-in support for this protocol. If you're using a recent version of a popular language like Go or an HTTP library that is up to date, chances are you're good to go.
Step 2: Use the Right Client Library
When making HTTP requests, you'll want to utilize a client library that's HTTP/2-aware. For instance, if you're working with Go, you can make use of the net/http
package. Ensure you're using a recent version of Go to fully leverage the built-in HTTP/2 support it offers.
Step 3: Sending an HTTP/2 Request
Now, let's take a closer look at how to send an HTTP/2 request using Go as an example:
import (
"fmt"
"net/http"
)
func main() {
client := &http.Client{}
req, err := http.NewRequest("GET", "https://example.com/some/url", nil)
if err != nil {
fmt.Println(err)
return
}
// You can specify HTTP/2 as the protocol
req.Header.Set("Connection", "Upgrade, HTTP2-Settings")
req.Header.Set("Upgrade", "h2c")
req.Header.Set("HTTP2-Settings", "AAMAAABkAAQAAP__")
resp, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
// Handle the response as needed
defer resp.Body.Close()
}
This code example demonstrates how to send an HTTP/2 request using the net/http
package in Go. The key is to set the appropriate headers that indicate the use of HTTP/2. By following these steps, you can correctly send an HTTP/2 request from Go.
The Challenge with HTTP/2 in Go
You might be familiar with Go, a popular programming language for building web applications and services. While Go is capable of working with HTTP/2, there's a common misunderstanding regarding how to correctly send HTTP/2 requests from Go.
One common misconception is using thehttputil.DumpRequest
function, as shown in the code snippet below:
dr, _ := httputil.DumpRequest(request, false)
fmt.Println(string(dr))
However, the output of this code does not reflect the actual request sent on the wire, and it's not suitable for checking if HTTP/2 is being used. Here's an example of what it might print:
GET /some/url HTTP/1.1
// some headers
// some cookies
As you can see, it still displays the request in HTTP/1.1 format, which can lead to confusion.
WhyDumpRequest
Is Not Suitable for Checking HTTP/2
The Go documentation forhttputil.DumpRequest
explicitly states:
"DumpRequest returns the given request in its HTTP/1.x wire representation. It should only be used by servers to debug client requests. The returned representation is an approximation only; some details of the initial request are lost while parsing it into an http.Request.
In particular, the order and case of header field names are lost. The order of values in multi-valued headers is kept intact. HTTP/2 requests are dumped in HTTP/1.x form, not in their original binary representations."
In other words,DumpRequest
is designed to return the request in its HTTP/1.x wire representation. It's not suitable for checking if HTTP/2 is being used because it doesn't display the original binary representation of the HTTP/2 request.
Apidog Supports to Send HTTP/2 API
Apidog is your ultimate solution for comprehensive API testing and management while Postman still cannot support HTTP/2.0. This powerful tool simplifies the testing of a wide array of APIs, whether they're based on the HTTP/1.1 protocol or the latest HTTP/2. With its intuitive interface, Apidog allows you to effortlessly create and send requests, streamlining the testing process.
What sets Apidog apart is its optimized communication capabilities. With robust support for HTTP/2, it ensures that your API testing is not only efficient but also highly responsive, significantly reducing latency and enhancing overall performance.
Apidog provides the flexibility to choose between HTTP/1.1 and HTTP/2 connections, enabling you to seamlessly adapt to your API's specific requirements. This adaptability makes it a versatile tool for a wide range of APIs.
To further enhance your experience, Apidog offers user-friendly settings that can be easily customized within the "Settings" tab. This feature ensures that your testing experience is smooth and tailored to your individual preferences. Whether you're involved in API design, testing, or sharing, Apidog is the ideal companion to streamline and optimize your API management tasks.
FAQs of HTTP 2.0 Request
Is HTTP 2.0 still used?
Yes, HTTP/2 is still used and increasingly adopted. Many modern web servers, browsers, and web applications support it, improving web performance and user experience.
Why HTTP/2 is not popular?
HTTP/2 is not as popular as it could be due to various reasons, including limited support on older systems and configurations, and the complexity of transitioning from HTTP/1.1 to HTTP/2. However, it is gaining traction as more web servers and browsers offer support.
What is the HTTP/2 request structure?
The HTTP/2 request structure is similar to HTTP/1.1 but is transmitted differently. It uses binary framing, multiplexing, and header compression to optimize data transfer. Requests include methods (GET, POST, etc.), headers, and the requested resource path.